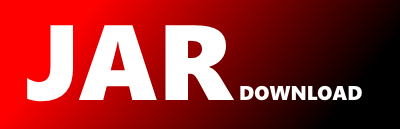
nbnDe11112004033116.impl.EpicurDocumentImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of epicur Show documentation
Show all versions of epicur Show documentation
Java classes (generated with XML-Beans) for XML-Schemas xepicur and epicurlite processing.
See the official URN:NBN Resolver http://nbn-resolving.org or http://persid.org
The newest version!
/*
* An XML document type.
* Localname: epicur
* Namespace: urn:nbn:de:1111-2004033116
* Java type: nbnDe11112004033116.EpicurDocument
*
* Automatically generated - do not modify.
*/
package nbnDe11112004033116.impl;
/**
* A document containing one epicur(@urn:nbn:de:1111-2004033116) element.
*
* This is a complex type.
*/
public class EpicurDocumentImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements nbnDe11112004033116.EpicurDocument
{
private static final long serialVersionUID = 1L;
public EpicurDocumentImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName EPICUR$0 =
new javax.xml.namespace.QName("urn:nbn:de:1111-2004033116", "epicur");
/**
* Gets the "epicur" element
*/
public nbnDe11112004033116.EpicurDocument.Epicur getEpicur()
{
synchronized (monitor())
{
check_orphaned();
nbnDe11112004033116.EpicurDocument.Epicur target = null;
target = (nbnDe11112004033116.EpicurDocument.Epicur)get_store().find_element_user(EPICUR$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "epicur" element
*/
public void setEpicur(nbnDe11112004033116.EpicurDocument.Epicur epicur)
{
synchronized (monitor())
{
check_orphaned();
nbnDe11112004033116.EpicurDocument.Epicur target = null;
target = (nbnDe11112004033116.EpicurDocument.Epicur)get_store().find_element_user(EPICUR$0, 0);
if (target == null)
{
target = (nbnDe11112004033116.EpicurDocument.Epicur)get_store().add_element_user(EPICUR$0);
}
target.set(epicur);
}
}
/**
* Appends and returns a new empty "epicur" element
*/
public nbnDe11112004033116.EpicurDocument.Epicur addNewEpicur()
{
synchronized (monitor())
{
check_orphaned();
nbnDe11112004033116.EpicurDocument.Epicur target = null;
target = (nbnDe11112004033116.EpicurDocument.Epicur)get_store().add_element_user(EPICUR$0);
return target;
}
}
/**
* An XML epicur(@urn:nbn:de:1111-2004033116).
*
* This is a complex type.
*/
public static class EpicurImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements nbnDe11112004033116.EpicurDocument.Epicur
{
private static final long serialVersionUID = 1L;
public EpicurImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName ADMINISTRATIVEDATA$0 =
new javax.xml.namespace.QName("urn:nbn:de:1111-2004033116", "administrative_data");
private static final javax.xml.namespace.QName RECORD$2 =
new javax.xml.namespace.QName("urn:nbn:de:1111-2004033116", "record");
/**
* Gets the "administrative_data" element
*/
public nbnDe11112004033116.AdministrativeDataType getAdministrativeData()
{
synchronized (monitor())
{
check_orphaned();
nbnDe11112004033116.AdministrativeDataType target = null;
target = (nbnDe11112004033116.AdministrativeDataType)get_store().find_element_user(ADMINISTRATIVEDATA$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "administrative_data" element
*/
public void setAdministrativeData(nbnDe11112004033116.AdministrativeDataType administrativeData)
{
synchronized (monitor())
{
check_orphaned();
nbnDe11112004033116.AdministrativeDataType target = null;
target = (nbnDe11112004033116.AdministrativeDataType)get_store().find_element_user(ADMINISTRATIVEDATA$0, 0);
if (target == null)
{
target = (nbnDe11112004033116.AdministrativeDataType)get_store().add_element_user(ADMINISTRATIVEDATA$0);
}
target.set(administrativeData);
}
}
/**
* Appends and returns a new empty "administrative_data" element
*/
public nbnDe11112004033116.AdministrativeDataType addNewAdministrativeData()
{
synchronized (monitor())
{
check_orphaned();
nbnDe11112004033116.AdministrativeDataType target = null;
target = (nbnDe11112004033116.AdministrativeDataType)get_store().add_element_user(ADMINISTRATIVEDATA$0);
return target;
}
}
/**
* Gets array of all "record" elements
*/
public nbnDe11112004033116.RecordType[] getRecordArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(RECORD$2, targetList);
nbnDe11112004033116.RecordType[] result = new nbnDe11112004033116.RecordType[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "record" element
*/
public nbnDe11112004033116.RecordType getRecordArray(int i)
{
synchronized (monitor())
{
check_orphaned();
nbnDe11112004033116.RecordType target = null;
target = (nbnDe11112004033116.RecordType)get_store().find_element_user(RECORD$2, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "record" element
*/
public int sizeOfRecordArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(RECORD$2);
}
}
/**
* Sets array of all "record" element
*/
public void setRecordArray(nbnDe11112004033116.RecordType[] recordArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(recordArray, RECORD$2);
}
}
/**
* Sets ith "record" element
*/
public void setRecordArray(int i, nbnDe11112004033116.RecordType record)
{
synchronized (monitor())
{
check_orphaned();
nbnDe11112004033116.RecordType target = null;
target = (nbnDe11112004033116.RecordType)get_store().find_element_user(RECORD$2, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.set(record);
}
}
/**
* Inserts and returns a new empty value (as xml) as the ith "record" element
*/
public nbnDe11112004033116.RecordType insertNewRecord(int i)
{
synchronized (monitor())
{
check_orphaned();
nbnDe11112004033116.RecordType target = null;
target = (nbnDe11112004033116.RecordType)get_store().insert_element_user(RECORD$2, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "record" element
*/
public nbnDe11112004033116.RecordType addNewRecord()
{
synchronized (monitor())
{
check_orphaned();
nbnDe11112004033116.RecordType target = null;
target = (nbnDe11112004033116.RecordType)get_store().add_element_user(RECORD$2);
return target;
}
}
/**
* Removes the ith "record" element
*/
public void removeRecord(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(RECORD$2, i);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy