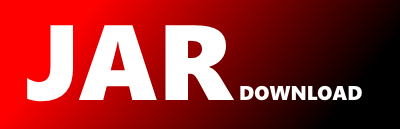
org.nbnResolving.epicurlite.impl.EpicurliteDocumentImpl Maven / Gradle / Ivy
/*
* An XML document type.
* Localname: epicurlite
* Namespace: http://nbn-resolving.org/epicurlite
* Java type: org.nbnResolving.epicurlite.EpicurliteDocument
*
* Automatically generated - do not modify.
*/
package org.nbnResolving.epicurlite.impl;
/**
* A document containing one epicurlite(@http://nbn-resolving.org/epicurlite) element.
*
* This is a complex type.
*/
public class EpicurliteDocumentImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements org.nbnResolving.epicurlite.EpicurliteDocument
{
private static final long serialVersionUID = 1L;
public EpicurliteDocumentImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName EPICURLITE$0 =
new javax.xml.namespace.QName("http://nbn-resolving.org/epicurlite", "epicurlite");
/**
* Gets the "epicurlite" element
*/
public org.nbnResolving.epicurlite.EpicurliteDocument.Epicurlite getEpicurlite()
{
synchronized (monitor())
{
check_orphaned();
org.nbnResolving.epicurlite.EpicurliteDocument.Epicurlite target = null;
target = (org.nbnResolving.epicurlite.EpicurliteDocument.Epicurlite)get_store().find_element_user(EPICURLITE$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "epicurlite" element
*/
public void setEpicurlite(org.nbnResolving.epicurlite.EpicurliteDocument.Epicurlite epicurlite)
{
synchronized (monitor())
{
check_orphaned();
org.nbnResolving.epicurlite.EpicurliteDocument.Epicurlite target = null;
target = (org.nbnResolving.epicurlite.EpicurliteDocument.Epicurlite)get_store().find_element_user(EPICURLITE$0, 0);
if (target == null)
{
target = (org.nbnResolving.epicurlite.EpicurliteDocument.Epicurlite)get_store().add_element_user(EPICURLITE$0);
}
target.set(epicurlite);
}
}
/**
* Appends and returns a new empty "epicurlite" element
*/
public org.nbnResolving.epicurlite.EpicurliteDocument.Epicurlite addNewEpicurlite()
{
synchronized (monitor())
{
check_orphaned();
org.nbnResolving.epicurlite.EpicurliteDocument.Epicurlite target = null;
target = (org.nbnResolving.epicurlite.EpicurliteDocument.Epicurlite)get_store().add_element_user(EPICURLITE$0);
return target;
}
}
/**
* An XML epicurlite(@http://nbn-resolving.org/epicurlite).
*
* This is a complex type.
*/
public static class EpicurliteImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements org.nbnResolving.epicurlite.EpicurliteDocument.Epicurlite
{
private static final long serialVersionUID = 1L;
public EpicurliteImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName LOGIN$0 =
new javax.xml.namespace.QName("http://nbn-resolving.org/epicurlite", "login");
private static final javax.xml.namespace.QName PASSWORD$2 =
new javax.xml.namespace.QName("http://nbn-resolving.org/epicurlite", "password");
private static final javax.xml.namespace.QName IDENTIFIER$4 =
new javax.xml.namespace.QName("http://nbn-resolving.org/epicurlite", "identifier");
private static final javax.xml.namespace.QName RESOURCE$6 =
new javax.xml.namespace.QName("http://nbn-resolving.org/epicurlite", "resource");
/**
* Gets the "login" element
*/
public java.lang.String getLogin()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(LOGIN$0, 0);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "login" element
*/
public org.apache.xmlbeans.XmlToken xgetLogin()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlToken target = null;
target = (org.apache.xmlbeans.XmlToken)get_store().find_element_user(LOGIN$0, 0);
return target;
}
}
/**
* Sets the "login" element
*/
public void setLogin(java.lang.String login)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(LOGIN$0, 0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_element_user(LOGIN$0);
}
target.setStringValue(login);
}
}
/**
* Sets (as xml) the "login" element
*/
public void xsetLogin(org.apache.xmlbeans.XmlToken login)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlToken target = null;
target = (org.apache.xmlbeans.XmlToken)get_store().find_element_user(LOGIN$0, 0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlToken)get_store().add_element_user(LOGIN$0);
}
target.set(login);
}
}
/**
* Gets the "password" element
*/
public java.lang.String getPassword()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(PASSWORD$2, 0);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "password" element
*/
public org.apache.xmlbeans.XmlToken xgetPassword()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlToken target = null;
target = (org.apache.xmlbeans.XmlToken)get_store().find_element_user(PASSWORD$2, 0);
return target;
}
}
/**
* Sets the "password" element
*/
public void setPassword(java.lang.String password)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(PASSWORD$2, 0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_element_user(PASSWORD$2);
}
target.setStringValue(password);
}
}
/**
* Sets (as xml) the "password" element
*/
public void xsetPassword(org.apache.xmlbeans.XmlToken password)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlToken target = null;
target = (org.apache.xmlbeans.XmlToken)get_store().find_element_user(PASSWORD$2, 0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlToken)get_store().add_element_user(PASSWORD$2);
}
target.set(password);
}
}
/**
* Gets the "identifier" element
*/
public org.nbnResolving.epicurlite.IdentifierType getIdentifier()
{
synchronized (monitor())
{
check_orphaned();
org.nbnResolving.epicurlite.IdentifierType target = null;
target = (org.nbnResolving.epicurlite.IdentifierType)get_store().find_element_user(IDENTIFIER$4, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "identifier" element
*/
public boolean isSetIdentifier()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(IDENTIFIER$4) != 0;
}
}
/**
* Sets the "identifier" element
*/
public void setIdentifier(org.nbnResolving.epicurlite.IdentifierType identifier)
{
synchronized (monitor())
{
check_orphaned();
org.nbnResolving.epicurlite.IdentifierType target = null;
target = (org.nbnResolving.epicurlite.IdentifierType)get_store().find_element_user(IDENTIFIER$4, 0);
if (target == null)
{
target = (org.nbnResolving.epicurlite.IdentifierType)get_store().add_element_user(IDENTIFIER$4);
}
target.set(identifier);
}
}
/**
* Appends and returns a new empty "identifier" element
*/
public org.nbnResolving.epicurlite.IdentifierType addNewIdentifier()
{
synchronized (monitor())
{
check_orphaned();
org.nbnResolving.epicurlite.IdentifierType target = null;
target = (org.nbnResolving.epicurlite.IdentifierType)get_store().add_element_user(IDENTIFIER$4);
return target;
}
}
/**
* Unsets the "identifier" element
*/
public void unsetIdentifier()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(IDENTIFIER$4, 0);
}
}
/**
* Gets array of all "resource" elements
*/
public org.nbnResolving.epicurlite.ResourceType[] getResourceArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(RESOURCE$6, targetList);
org.nbnResolving.epicurlite.ResourceType[] result = new org.nbnResolving.epicurlite.ResourceType[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "resource" element
*/
public org.nbnResolving.epicurlite.ResourceType getResourceArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.nbnResolving.epicurlite.ResourceType target = null;
target = (org.nbnResolving.epicurlite.ResourceType)get_store().find_element_user(RESOURCE$6, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "resource" element
*/
public int sizeOfResourceArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(RESOURCE$6);
}
}
/**
* Sets array of all "resource" element
*/
public void setResourceArray(org.nbnResolving.epicurlite.ResourceType[] resourceArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(resourceArray, RESOURCE$6);
}
}
/**
* Sets ith "resource" element
*/
public void setResourceArray(int i, org.nbnResolving.epicurlite.ResourceType resource)
{
synchronized (monitor())
{
check_orphaned();
org.nbnResolving.epicurlite.ResourceType target = null;
target = (org.nbnResolving.epicurlite.ResourceType)get_store().find_element_user(RESOURCE$6, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.set(resource);
}
}
/**
* Inserts and returns a new empty value (as xml) as the ith "resource" element
*/
public org.nbnResolving.epicurlite.ResourceType insertNewResource(int i)
{
synchronized (monitor())
{
check_orphaned();
org.nbnResolving.epicurlite.ResourceType target = null;
target = (org.nbnResolving.epicurlite.ResourceType)get_store().insert_element_user(RESOURCE$6, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "resource" element
*/
public org.nbnResolving.epicurlite.ResourceType addNewResource()
{
synchronized (monitor())
{
check_orphaned();
org.nbnResolving.epicurlite.ResourceType target = null;
target = (org.nbnResolving.epicurlite.ResourceType)get_store().add_element_user(RESOURCE$6);
return target;
}
}
/**
* Removes the ith "resource" element
*/
public void removeResource(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(RESOURCE$6, i);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy