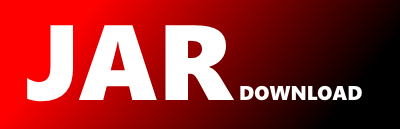
org.neo4j.server.rest.batch.StreamingBatchOperationResults Maven / Gradle / Ivy
/*
* Copyright (c) 2002-2016 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.server.rest.batch;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.util.HashMap;
import java.util.Map;
import javax.servlet.ServletOutputStream;
import javax.servlet.WriteListener;
import javax.ws.rs.core.Response;
import org.codehaus.jackson.JsonGenerator;
/*
* Because the batch operation API operates on the HTTP abstraction
* level, we do not use our normal serialization system for serializing
* its' results.
*
* Doing so would require us to de-serialize each JSON response we get from
* each operation, and we would have to extend our current type safe serialization
* system to incorporate arbitrary responses.
*/
public class StreamingBatchOperationResults
{
public static final int HEAD_BUFFER = 10;
public static final int IS_ERROR = -1;
private final Map locations = new HashMap();
private final JsonGenerator g;
private final ServletOutputStream output;
private ByteArrayOutputStream errorStream;
private int bytesWritten = 0;
private char[] head = new char[HEAD_BUFFER];
public StreamingBatchOperationResults( JsonGenerator g, ServletOutputStream output ) throws IOException {
this.g = g;
this.output = output;
g.writeStartArray();
}
public void startOperation(String from, Integer id) throws IOException {
bytesWritten = 0;
g.writeStartObject();
if (id!=null) g.writeNumberField("id", id);
g.writeStringField("from", from);
g.writeRaw(",\"body\":");
g.flush();
}
public void addOperationResult(int status, Integer id,String location) throws IOException {
finishBody();
if ( location != null ) {
locations.put(id, location);
g.writeStringField("location",location);
}
g.writeNumberField("status",status);
g.writeEndObject();
}
private void finishBody() throws IOException
{
if (bytesWritten == 0 ) {
g.writeRaw("null");
} else if ( bytesWritten getLocations()
{
return locations;
}
public void close() throws IOException {
g.writeEndArray();
g.close();
}
public void writeError( int status, String message ) throws IOException {
if (bytesWritten == 0 || bytesWritten == IS_ERROR) g.writeRaw( "null" );
g.writeNumberField( "status", status );
if (message!=null && !message.trim().equals( Response.Status.fromStatusCode( status ).getReasonPhrase())) g.writeStringField( "message", message);
else {
if (errorStream!=null) {
g.writeStringField( "message", errorStream.toString( StandardCharsets.UTF_8.name() ));
}
}
g.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy