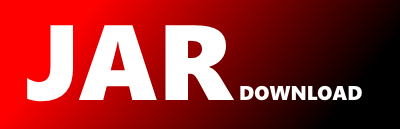
org.linuxstuff.mojo.licensing.HelpMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of licensing-maven-plugin Show documentation
Show all versions of licensing-maven-plugin Show documentation
Forked from http://github.com/idcmp/licensing-maven-plugin
package org.linuxstuff.mojo.licensing;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
/**
* Display help information on licensing-maven-plugin.
Call mvn licensing:help -Ddetail=true -Dgoal=<goal-name>
to display parameter details.
*
* @version generated on Mon Nov 05 15:58:56 CET 2012
* @author org.apache.maven.tools.plugin.generator.PluginHelpGenerator (version 2.9)
* @goal help
* @requiresProject false
* @threadSafe
*/
public class HelpMojo
extends AbstractMojo
{
/**
* If true
, display all settable properties for each goal.
*
* @parameter expression="${detail}" default-value="false"
*/
private boolean detail;
/**
* The name of the goal for which to show help. If unspecified, all goals will be displayed.
*
* @parameter expression="${goal}"
*/
private java.lang.String goal;
/**
* The maximum length of a display line, should be positive.
*
* @parameter expression="${lineLength}" default-value="80"
*/
private int lineLength;
/**
* The number of spaces per indentation level, should be positive.
*
* @parameter expression="${indentSize}" default-value="2"
*/
private int indentSize;
/** {@inheritDoc} */
public void execute()
throws MojoExecutionException
{
if ( lineLength <= 0 )
{
getLog().warn( "The parameter 'lineLength' should be positive, using '80' as default." );
lineLength = 80;
}
if ( indentSize <= 0 )
{
getLog().warn( "The parameter 'indentSize' should be positive, using '2' as default." );
indentSize = 2;
}
StringBuffer sb = new StringBuffer();
append( sb, "org.neo4j.build.plugins:licensing-maven-plugin:1.7.5", 0 );
append( sb, "", 0 );
append( sb, "Licensing Maven Mojo", 0 );
append( sb, "Forked from http://github.com/idcmp/licensing-maven-plugin", 1 );
append( sb, "", 0 );
if ( goal == null || goal.length() <= 0 )
{
append( sb, "This plugin has 4 goals:", 0 );
append( sb, "", 0 );
}
if ( goal == null || goal.length() <= 0 || "aggregate".equals( goal ) )
{
append( sb, "licensing:aggregate", 0 );
append( sb, "Aggregate mojo. Will walk your reactor building in memory licensing reports making them into one giant report. This mojo does not check for missing or disliked artifacts (use CheckMojo for that).", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "aggregatedThirdPartyLicensingFilename (Default: aggregated-third-party-licensing.xml)", 2 );
append( sb, "The name of the the XML file which contains the aggregated licensing information for artifacts.", 3 );
append( sb, "Expression: ${aggregatedThirdPartyLicensingFilename}", 3 );
append( sb, "", 0 );
append( sb, "appendText", 2 );
append( sb, "File to append to the text-based report.", 3 );
append( sb, "Expression: ${appendText}", 3 );
append( sb, "", 0 );
append( sb, "checkExistingLicensesFile", 2 );
append( sb, "File to append to the text-based report.", 3 );
append( sb, "Expression: ${checkExistingLicensesFile}", 3 );
append( sb, "", 0 );
append( sb, "checkExistingNoticeFile", 2 );
append( sb, "File to append to the text-based report.", 3 );
append( sb, "Expression: ${checkExistingNoticeFile}", 3 );
append( sb, "", 0 );
append( sb, "excludedArtifacts", 2 );
append( sb, "A filter to exclude some ArtifactsIds", 3 );
append( sb, "Expression: ${licensing.excludedArtifacts}", 3 );
append( sb, "", 0 );
append( sb, "excludedGroups", 2 );
append( sb, "A filter to exclude some GroupIds", 3 );
append( sb, "Expression: ${licensing.excludedGroups}", 3 );
append( sb, "", 0 );
append( sb, "excludedScopes (Default: system)", 2 );
append( sb, "A filter to exclude some scopes.", 3 );
append( sb, "Expression: ${licensing.excludedScopes}", 3 );
append( sb, "", 0 );
append( sb, "failIfDisliked (Default: true)", 2 );
append( sb, "A fail the build if any artifacts have disliked licenses.", 3 );
append( sb, "Expression: ${failIfDisliked}", 3 );
append( sb, "", 0 );
append( sb, "failIfMissing (Default: true)", 2 );
append( sb, "A fail the build if any artifacts are missing licensing information.", 3 );
append( sb, "Expression: ${failIfMissing}", 3 );
append( sb, "", 0 );
append( sb, "includedArtifacts", 2 );
append( sb, "A filter to include only some ArtifactsIds", 3 );
append( sb, "Expression: ${licensing.includedArtifacts}", 3 );
append( sb, "", 0 );
append( sb, "includedGroups", 2 );
append( sb, "A filter to include only some GroupIds", 3 );
append( sb, "Expression: ${licensing.includedGroups}", 3 );
append( sb, "", 0 );
append( sb, "includedScopes", 2 );
append( sb, "A filter to include only some scopes, if let empty then all scopes will be used (no filter).", 3 );
append( sb, "Expression: ${licensing.includedScopes}", 3 );
append( sb, "", 0 );
append( sb, "includeOnlyLikedInReport (Default: true)", 2 );
append( sb, "If using liked licenses, only use those in the report.", 3 );
append( sb, "Expression: ${includeOnlyLikedInReport}", 3 );
append( sb, "", 0 );
append( sb, "includeTransitiveDependencies (Default: true)", 2 );
append( sb, "Include transitive dependencies when downloading license files.", 3 );
append( sb, "", 0 );
append( sb, "licensingRequirementFiles", 2 );
append( sb, "Location of license requirement XML files.", 3 );
append( sb, "", 0 );
append( sb, "listPrependText", 2 );
append( sb, "File to prepend to the text-based report with full license texts.", 3 );
append( sb, "Expression: ${listPrependText}", 3 );
append( sb, "", 0 );
append( sb, "listReport", 2 );
append( sb, "The name of the output txt file which contains full text licenses. Only kicks in as part of generating a plain text report.", 3 );
append( sb, "Expression: ${listReport}", 3 );
append( sb, "", 0 );
append( sb, "overwrite (Default: false)", 2 );
append( sb, "Overwrite existing license/notice.txt files.", 3 );
append( sb, "Expression: ${overwrite}", 3 );
append( sb, "", 0 );
append( sb, "plainTextReport (Default: false)", 2 );
append( sb, "Output the result as a plain text file.", 3 );
append( sb, "Expression: ${writeTextReport}", 3 );
append( sb, "", 0 );
append( sb, "prependText", 2 );
append( sb, "File to prepend to the text-based report.", 3 );
append( sb, "Expression: ${prependText}", 3 );
append( sb, "", 0 );
append( sb, "skip (Default: false)", 2 );
append( sb, "Should we skip doing licensing checks?", 3 );
append( sb, "Expression: ${licensing.skip}", 3 );
append( sb, "", 0 );
append( sb, "thirdPartyLicensingFilename (Default: third-party-licensing.xml)", 2 );
append( sb, "The name of the the XML file which contains licensing information one artifact.", 3 );
append( sb, "Expression: ${thirdPartyLicensingFilename}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "check".equals( goal ) )
{
append( sb, "licensing:check", 0 );
append( sb, "Determine licensing information of all dependencies. This is generally obtained by dependencies providing a license block in their POM. However this plugin supports a requirements file which can supplement licensing information for artifacts missing licensing information.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "aggregatedThirdPartyLicensingFilename (Default: aggregated-third-party-licensing.xml)", 2 );
append( sb, "The name of the the XML file which contains the aggregated licensing information for artifacts.", 3 );
append( sb, "Expression: ${aggregatedThirdPartyLicensingFilename}", 3 );
append( sb, "", 0 );
append( sb, "appendText", 2 );
append( sb, "File to append to the text-based report.", 3 );
append( sb, "Expression: ${appendText}", 3 );
append( sb, "", 0 );
append( sb, "checkExistingLicensesFile", 2 );
append( sb, "File to append to the text-based report.", 3 );
append( sb, "Expression: ${checkExistingLicensesFile}", 3 );
append( sb, "", 0 );
append( sb, "checkExistingNoticeFile", 2 );
append( sb, "File to append to the text-based report.", 3 );
append( sb, "Expression: ${checkExistingNoticeFile}", 3 );
append( sb, "", 0 );
append( sb, "excludedArtifacts", 2 );
append( sb, "A filter to exclude some ArtifactsIds", 3 );
append( sb, "Expression: ${licensing.excludedArtifacts}", 3 );
append( sb, "", 0 );
append( sb, "excludedGroups", 2 );
append( sb, "A filter to exclude some GroupIds", 3 );
append( sb, "Expression: ${licensing.excludedGroups}", 3 );
append( sb, "", 0 );
append( sb, "excludedScopes (Default: system)", 2 );
append( sb, "A filter to exclude some scopes.", 3 );
append( sb, "Expression: ${licensing.excludedScopes}", 3 );
append( sb, "", 0 );
append( sb, "failIfDisliked (Default: true)", 2 );
append( sb, "A fail the build if any artifacts have disliked licenses.", 3 );
append( sb, "Expression: ${failIfDisliked}", 3 );
append( sb, "", 0 );
append( sb, "failIfMissing (Default: true)", 2 );
append( sb, "A fail the build if any artifacts are missing licensing information.", 3 );
append( sb, "Expression: ${failIfMissing}", 3 );
append( sb, "", 0 );
append( sb, "includedArtifacts", 2 );
append( sb, "A filter to include only some ArtifactsIds", 3 );
append( sb, "Expression: ${licensing.includedArtifacts}", 3 );
append( sb, "", 0 );
append( sb, "includedGroups", 2 );
append( sb, "A filter to include only some GroupIds", 3 );
append( sb, "Expression: ${licensing.includedGroups}", 3 );
append( sb, "", 0 );
append( sb, "includedScopes", 2 );
append( sb, "A filter to include only some scopes, if let empty then all scopes will be used (no filter).", 3 );
append( sb, "Expression: ${licensing.includedScopes}", 3 );
append( sb, "", 0 );
append( sb, "includeOnlyLikedInReport (Default: true)", 2 );
append( sb, "If using liked licenses, only use those in the report.", 3 );
append( sb, "Expression: ${includeOnlyLikedInReport}", 3 );
append( sb, "", 0 );
append( sb, "includeTransitiveDependencies (Default: true)", 2 );
append( sb, "Include transitive dependencies when downloading license files.", 3 );
append( sb, "", 0 );
append( sb, "licensingRequirementFiles", 2 );
append( sb, "Location of license requirement XML files.", 3 );
append( sb, "", 0 );
append( sb, "listPrependText", 2 );
append( sb, "File to prepend to the text-based report with full license texts.", 3 );
append( sb, "Expression: ${listPrependText}", 3 );
append( sb, "", 0 );
append( sb, "listReport", 2 );
append( sb, "The name of the output txt file which contains full text licenses. Only kicks in as part of generating a plain text report.", 3 );
append( sb, "Expression: ${listReport}", 3 );
append( sb, "", 0 );
append( sb, "overwrite (Default: false)", 2 );
append( sb, "Overwrite existing license/notice.txt files.", 3 );
append( sb, "Expression: ${overwrite}", 3 );
append( sb, "", 0 );
append( sb, "plainTextReport (Default: false)", 2 );
append( sb, "Output the result as a plain text file.", 3 );
append( sb, "Expression: ${writeTextReport}", 3 );
append( sb, "", 0 );
append( sb, "prependText", 2 );
append( sb, "File to prepend to the text-based report.", 3 );
append( sb, "Expression: ${prependText}", 3 );
append( sb, "", 0 );
append( sb, "skip (Default: false)", 2 );
append( sb, "Should we skip doing licensing checks?", 3 );
append( sb, "Expression: ${licensing.skip}", 3 );
append( sb, "", 0 );
append( sb, "thirdPartyLicensingFilename (Default: third-party-licensing.xml)", 2 );
append( sb, "The name of the the XML file which contains licensing information one artifact.", 3 );
append( sb, "Expression: ${thirdPartyLicensingFilename}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "collect-reports".equals( goal ) )
{
append( sb, "licensing:collect-reports", 0 );
append( sb, "(no description available)", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "aggregatedThirdPartyLicensingFilename (Default: aggregated-third-party-licensing.xml)", 2 );
append( sb, "The name of the the XML file which contains the aggregated licensing information for artifacts.", 3 );
append( sb, "Expression: ${aggregatedThirdPartyLicensingFilename}", 3 );
append( sb, "", 0 );
append( sb, "excludedArtifacts", 2 );
append( sb, "A filter to exclude some ArtifactsIds", 3 );
append( sb, "Expression: ${licensing.excludedArtifacts}", 3 );
append( sb, "", 0 );
append( sb, "excludedGroups", 2 );
append( sb, "A filter to exclude some GroupIds", 3 );
append( sb, "Expression: ${licensing.excludedGroups}", 3 );
append( sb, "", 0 );
append( sb, "excludedScopes (Default: system)", 2 );
append( sb, "A filter to exclude some scopes.", 3 );
append( sb, "Expression: ${licensing.excludedScopes}", 3 );
append( sb, "", 0 );
append( sb, "includedArtifacts", 2 );
append( sb, "A filter to include only some ArtifactsIds", 3 );
append( sb, "Expression: ${licensing.includedArtifacts}", 3 );
append( sb, "", 0 );
append( sb, "includedGroups", 2 );
append( sb, "A filter to include only some GroupIds", 3 );
append( sb, "Expression: ${licensing.includedGroups}", 3 );
append( sb, "", 0 );
append( sb, "includedScopes", 2 );
append( sb, "A filter to include only some scopes, if let empty then all scopes will be used (no filter).", 3 );
append( sb, "Expression: ${licensing.includedScopes}", 3 );
append( sb, "", 0 );
append( sb, "includeTransitiveDependencies (Default: true)", 2 );
append( sb, "Include transitive dependencies when downloading license files.", 3 );
append( sb, "", 0 );
append( sb, "licensingRequirementFiles", 2 );
append( sb, "Location of license requirement XML files.", 3 );
append( sb, "", 0 );
append( sb, "skip (Default: false)", 2 );
append( sb, "Should we skip doing licensing checks?", 3 );
append( sb, "Expression: ${licensing.skip}", 3 );
append( sb, "", 0 );
append( sb, "thirdPartyLicensingFilename (Default: third-party-licensing.xml)", 2 );
append( sb, "The name of the the XML file which contains licensing information one artifact.", 3 );
append( sb, "Expression: ${thirdPartyLicensingFilename}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "help".equals( goal ) )
{
append( sb, "licensing:help", 0 );
append( sb, "Display help information on licensing-maven-plugin.\nCall\n\u00a0\u00a0mvn\u00a0licensing:help\u00a0-Ddetail=true\u00a0-Dgoal=\nto display parameter details.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "detail (Default: false)", 2 );
append( sb, "If true, display all settable properties for each goal.", 3 );
append( sb, "Expression: ${detail}", 3 );
append( sb, "", 0 );
append( sb, "goal", 2 );
append( sb, "The name of the goal for which to show help. If unspecified, all goals will be displayed.", 3 );
append( sb, "Expression: ${goal}", 3 );
append( sb, "", 0 );
append( sb, "indentSize (Default: 2)", 2 );
append( sb, "The number of spaces per indentation level, should be positive.", 3 );
append( sb, "Expression: ${indentSize}", 3 );
append( sb, "", 0 );
append( sb, "lineLength (Default: 80)", 2 );
append( sb, "The maximum length of a display line, should be positive.", 3 );
append( sb, "Expression: ${lineLength}", 3 );
append( sb, "", 0 );
}
}
if ( getLog().isInfoEnabled() )
{
getLog().info( sb.toString() );
}
}
/**
* Repeat a String n
times to form a new string.
*
* @param str String to repeat
* @param repeat number of times to repeat str
* @return String with repeated String
* @throws NegativeArraySizeException if repeat < 0
* @throws NullPointerException if str is null
*/
private static String repeat( String str, int repeat )
{
StringBuffer buffer = new StringBuffer( repeat * str.length() );
for ( int i = 0; i < repeat; i++ )
{
buffer.append( str );
}
return buffer.toString();
}
/**
* Append a description to the buffer by respecting the indentSize and lineLength parameters.
* Note: The last character is always a new line.
*
* @param sb The buffer to append the description, not null
.
* @param description The description, not null
.
* @param indent The base indentation level of each line, must not be negative.
*/
private void append( StringBuffer sb, String description, int indent )
{
for ( Iterator it = toLines( description, indent, indentSize, lineLength ).iterator(); it.hasNext(); )
{
sb.append( it.next().toString() ).append( '\n' );
}
}
/**
* Splits the specified text into lines of convenient display length.
*
* @param text The text to split into lines, must not be null
.
* @param indent The base indentation level of each line, must not be negative.
* @param indentSize The size of each indentation, must not be negative.
* @param lineLength The length of the line, must not be negative.
* @return The sequence of display lines, never null
.
* @throws NegativeArraySizeException if indent < 0
*/
private static List toLines( String text, int indent, int indentSize, int lineLength )
{
List lines = new ArrayList();
String ind = repeat( "\t", indent );
String[] plainLines = text.split( "(\r\n)|(\r)|(\n)" );
for ( int i = 0; i < plainLines.length; i++ )
{
toLines( lines, ind + plainLines[i], indentSize, lineLength );
}
return lines;
}
/**
* Adds the specified line to the output sequence, performing line wrapping if necessary.
*
* @param lines The sequence of display lines, must not be null
.
* @param line The line to add, must not be null
.
* @param indentSize The size of each indentation, must not be negative.
* @param lineLength The length of the line, must not be negative.
*/
private static void toLines( List lines, String line, int indentSize, int lineLength )
{
int lineIndent = getIndentLevel( line );
StringBuffer buf = new StringBuffer( 256 );
String[] tokens = line.split( " +" );
for ( int i = 0; i < tokens.length; i++ )
{
String token = tokens[i];
if ( i > 0 )
{
if ( buf.length() + token.length() >= lineLength )
{
lines.add( buf.toString() );
buf.setLength( 0 );
buf.append( repeat( " ", lineIndent * indentSize ) );
}
else
{
buf.append( ' ' );
}
}
for ( int j = 0; j < token.length(); j++ )
{
char c = token.charAt( j );
if ( c == '\t' )
{
buf.append( repeat( " ", indentSize - buf.length() % indentSize ) );
}
else if ( c == '\u00A0' )
{
buf.append( ' ' );
}
else
{
buf.append( c );
}
}
}
lines.add( buf.toString() );
}
/**
* Gets the indentation level of the specified line.
*
* @param line The line whose indentation level should be retrieved, must not be null
.
* @return The indentation level of the line.
*/
private static int getIndentLevel( String line )
{
int level = 0;
for ( int i = 0; i < line.length() && line.charAt( i ) == '\t'; i++ )
{
level++;
}
for ( int i = level + 1; i <= level + 4 && i < line.length(); i++ )
{
if ( line.charAt( i ) == '\t' )
{
level++;
break;
}
}
return level;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy