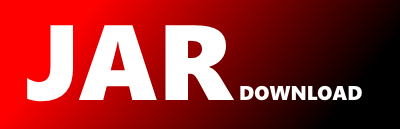
org.linuxstuff.mojo.licensing.model.ArtifactWithLicenses Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of licensing-maven-plugin Show documentation
Show all versions of licensing-maven-plugin Show documentation
Forked from http://github.com/idcmp/licensing-maven-plugin
package org.linuxstuff.mojo.licensing.model;
import java.util.HashSet;
import java.util.Set;
import com.thoughtworks.xstream.annotations.XStreamAlias;
import com.thoughtworks.xstream.annotations.XStreamAsAttribute;
import com.thoughtworks.xstream.annotations.XStreamImplicit;
@XStreamAlias("artifact")
public class ArtifactWithLicenses {
@XStreamAsAttribute
@XStreamAlias("id")
private String artifactId;
@XStreamAsAttribute
@XStreamAlias("name")
private String name;
@XStreamImplicit(itemFieldName = "license")
private Set licenses;
public ArtifactWithLicenses() {
licenses = new HashSet();
}
public ArtifactWithLicenses(String artifactId) {
this.artifactId = artifactId;
this.licenses = new HashSet();
}
public ArtifactWithLicenses(String artifactId, Set licenses) {
this.artifactId = artifactId;
this.licenses = licenses;
}
public ArtifactWithLicenses(String artifactId, String name) {
licenses = new HashSet();
this.artifactId = artifactId;
this.name = name;
}
public void combineWith(ArtifactWithLicenses other) {
licenses.addAll(other.getLicenses());
}
public String getArtifactId() {
return artifactId;
}
public Set getLicenses() {
return licenses;
}
public void addLicense(String license) {
licenses.add(license);
}
public void setArtifactId(String artifactId) {
this.artifactId = artifactId;
}
public void setLicenses(Set licenses) {
this.licenses = licenses;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((artifactId == null) ? 0 : artifactId.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
ArtifactWithLicenses other = (ArtifactWithLicenses) obj;
if (artifactId == null) {
if (other.artifactId != null)
return false;
} else if (!artifactId.equals(other.artifactId))
return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy