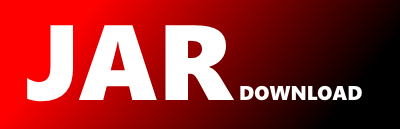
org.neo4j.driver.internal.async.HandshakeHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neo4j-java-driver Show documentation
Show all versions of neo4j-java-driver Show documentation
Access to the Neo4j graph database through Java
/*
* Copyright (c) 2002-2018 "Neo4j,"
* Neo4j Sweden AB [http://neo4j.com]
*
* This file is part of Neo4j.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.neo4j.driver.internal.async;
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelPipeline;
import io.netty.channel.ChannelPromise;
import io.netty.handler.codec.DecoderException;
import io.netty.handler.codec.ReplayingDecoder;
import java.util.List;
import javax.net.ssl.SSLHandshakeException;
import org.neo4j.driver.internal.logging.ChannelActivityLogger;
import org.neo4j.driver.internal.messaging.MessageFormat;
import org.neo4j.driver.internal.messaging.PackStreamMessageFormatV1;
import org.neo4j.driver.internal.messaging.PackStreamMessageFormatV2;
import org.neo4j.driver.internal.util.ErrorUtil;
import org.neo4j.driver.v1.Logger;
import org.neo4j.driver.v1.Logging;
import org.neo4j.driver.v1.exceptions.ClientException;
import org.neo4j.driver.v1.exceptions.SecurityException;
import org.neo4j.driver.v1.exceptions.ServiceUnavailableException;
import static org.neo4j.driver.internal.async.BoltProtocolUtil.HTTP;
import static org.neo4j.driver.internal.async.BoltProtocolUtil.NO_PROTOCOL_VERSION;
import static org.neo4j.driver.internal.async.BoltProtocolUtil.PROTOCOL_VERSION_1;
import static org.neo4j.driver.internal.async.BoltProtocolUtil.PROTOCOL_VERSION_2;
public class HandshakeHandler extends ReplayingDecoder
{
private final ChannelPipelineBuilder pipelineBuilder;
private final ChannelPromise handshakeCompletedPromise;
private final Logging logging;
private boolean failed;
private Logger log;
public HandshakeHandler( ChannelPipelineBuilder pipelineBuilder, ChannelPromise handshakeCompletedPromise,
Logging logging )
{
this.pipelineBuilder = pipelineBuilder;
this.handshakeCompletedPromise = handshakeCompletedPromise;
this.logging = logging;
}
@Override
public void handlerAdded( ChannelHandlerContext ctx )
{
log = new ChannelActivityLogger( ctx.channel(), logging, getClass() );
}
@Override
protected void handlerRemoved0( ChannelHandlerContext ctx )
{
failed = false;
log = null;
}
@Override
public void channelInactive( ChannelHandlerContext ctx )
{
log.debug( "Channel is inactive" );
if ( !failed )
{
// channel became inactive while doing bolt handshake, not because of some previous error
ServiceUnavailableException error = ErrorUtil.newConnectionTerminatedError();
fail( ctx, error );
}
}
@Override
public void exceptionCaught( ChannelHandlerContext ctx, Throwable error )
{
if ( failed )
{
log.warn( "Another fatal error occurred in the pipeline", error );
}
else
{
failed = true;
Throwable cause = transformError( error );
fail( ctx, cause );
}
}
@Override
protected void decode( ChannelHandlerContext ctx, ByteBuf in, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy