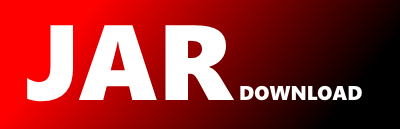
org.neo4j.driver.internal.util.Iterables Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neo4j-java-driver Show documentation
Show all versions of neo4j-java-driver Show documentation
Access to the Neo4j graph database through Java
/*
* Copyright (c) "Neo4j"
* Neo4j Sweden AB [https://neo4j.com]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.neo4j.driver.internal.util;
import java.util.AbstractQueue;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Queue;
import java.util.function.Function;
public class Iterables {
@SuppressWarnings("rawtypes")
private static final Queue EMPTY_QUEUE = new EmptyQueue();
private static final float DEFAULT_HASH_MAP_LOAD_FACTOR = 0.75F;
public static int count(Iterable> it) {
if (it instanceof Collection) {
return ((Collection>) it).size();
}
var size = 0;
for (Object ignored : it) {
size++;
}
return size;
}
public static List asList(Iterable it) {
if (it instanceof List) {
return (List) it;
}
List list = new ArrayList<>();
for (var t : it) {
list.add(t);
}
return list;
}
public static T single(Iterable it) {
var iterator = it.iterator();
if (!iterator.hasNext()) {
throw new IllegalArgumentException("Given iterable is empty");
}
var result = iterator.next();
if (iterator.hasNext()) {
throw new IllegalArgumentException("Given iterable contains more than one element: " + it);
}
return result;
}
public static Iterable map(final Iterable it, final Function f) {
return () -> {
final var aIterator = it.iterator();
return new Iterator<>() {
@Override
public boolean hasNext() {
return aIterator.hasNext();
}
@Override
public B next() {
return f.apply(aIterator.next());
}
@Override
public void remove() {
aIterator.remove();
}
};
};
}
@SuppressWarnings("unchecked")
public static Queue emptyQueue() {
return (Queue) EMPTY_QUEUE;
}
public static HashMap newHashMapWithSize(int expectedSize) {
return new HashMap<>(hashMapCapacity(expectedSize));
}
public static LinkedHashMap newLinkedHashMapWithSize(int expectedSize) {
return new LinkedHashMap<>(hashMapCapacity(expectedSize));
}
private static int hashMapCapacity(int expectedSize) {
if (expectedSize < 3) {
if (expectedSize < 0) {
throw new IllegalArgumentException("Illegal map size: " + expectedSize);
}
return expectedSize + 1;
}
return (int) ((float) expectedSize / DEFAULT_HASH_MAP_LOAD_FACTOR + 1.0F);
}
private static class EmptyQueue extends AbstractQueue {
@Override
public Iterator iterator() {
return Collections.emptyIterator();
}
@Override
public int size() {
return 0;
}
@Override
public boolean offer(T t) {
throw new UnsupportedOperationException();
}
@Override
public T poll() {
return null;
}
@Override
public T peek() {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy