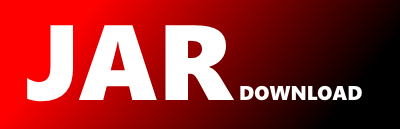
org.neo4j.driver.Logging Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neo4j-java-driver Show documentation
Show all versions of neo4j-java-driver Show documentation
Access to the Neo4j graph database through Java
The newest version!
/*
* Copyright (c) "Neo4j"
* Neo4j Sweden AB [https://neo4j.com]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.neo4j.driver;
import static org.neo4j.driver.internal.logging.DevNullLogging.DEV_NULL_LOGGING;
import java.util.logging.ConsoleHandler;
import java.util.logging.Level;
import org.neo4j.driver.internal.logging.ConsoleLogging;
import org.neo4j.driver.internal.logging.JULogging;
import org.neo4j.driver.internal.logging.Slf4jLogging;
/**
* Accessor for {@link Logger} instances. Configured once for a driver instance using {@link Config.ConfigBuilder#withLogging(Logging)} builder method.
* Users are expected to either implement this interface or use one of the existing implementations (available via static methods in this interface):
*
* - {@link #slf4j() SLF4J logging} - uses available SLF4J binding (Logback, Log4j, etc.) fails when no SLF4J implementation is available. Uses
* application's logging configuration from XML or other type of configuration file. This logging method is the preferred one and relies on the SLF4J
* implementation available in the classpath or modulepath.
* - {@link #javaUtilLogging(Level) Java Logging API (JUL)} - uses {@link java.util.logging.Logger} created via
* {@link java.util.logging.Logger#getLogger(String)}. Global java util logging configuration applies. This logging method is suitable when application
* uses JUL for logging and explicitly configures it.
* - {@link #console(Level) Console logging} - uses {@link ConsoleHandler} with the specified {@link Level logging level} to print messages to {@code
* System.err}. This logging method is suitable for quick debugging or prototyping.
* - {@link #none() No logging} - implementation that discards all logged messages. This logging method is suitable for testing to make driver produce
* no output.
*
*
* Driver logging API defines the following log levels: ERROR, INFO, WARN, DEBUG and TRACE. They are similar to levels defined by SLF4J but different from
* log levels defined for {@link java.util.logging}. The following mapping takes place:
*
* Driver and JUL log levels
*
* Driver
* java.util.logging
*
*
* ERROR
* SEVERE
*
*
* INFO
* INFO, CONFIG
*
*
* WARN
* WARNING
*
*
* DEBUG
* FINE, FINER
*
*
* TRACE
* FINEST
*
*
*
* Example of driver configuration with SLF4J logging:
*
* {@code
* Driver driver = GraphDatabase.driver("neo4j://localhost:7687",
* AuthTokens.basic("neo4j", "password"),
* Config.builder().withLogging(Logging.slf4j()).build());
* }
*
*
* @see Logger
* @see Config.ConfigBuilder#withLogging(Logging)
*/
public interface Logging {
/**
* Obtain a {@link Logger} instance by class, its name will be the fully qualified name of the class.
*
* @param clazz class whose name should be used as the {@link Logger} name.
* @return {@link Logger} instance
*/
default Logger getLog(Class> clazz) {
var canonicalName = clazz.getCanonicalName();
return getLog(canonicalName != null ? canonicalName : clazz.getName());
}
/**
* Obtain a {@link Logger} instance by name.
*
* @param name name of a {@link Logger}
* @return {@link Logger} instance
*/
Logger getLog(String name);
/**
* Create logging implementation that uses SLF4J.
*
* @return new logging implementation.
* @throws IllegalStateException if SLF4J is not available.
*/
static Logging slf4j() {
var unavailabilityError = Slf4jLogging.checkAvailability();
if (unavailabilityError != null) {
throw unavailabilityError;
}
return new Slf4jLogging();
}
/**
* Create logging implementation that uses {@link java.util.logging}.
*
* @param level the log level.
* @return new logging implementation.
*/
static Logging javaUtilLogging(Level level) {
return new JULogging(level);
}
/**
* Create logging implementation that uses {@link java.util.logging} to log to {@code System.err}.
*
* @param level the log level.
* @return new logging implementation.
*/
static Logging console(Level level) {
return new ConsoleLogging(level);
}
/**
* Create logging implementation that discards all messages and logs nothing.
*
* @return new logging implementation.
*/
@SuppressWarnings("SameReturnValue")
static Logging none() {
return DEV_NULL_LOGGING;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy