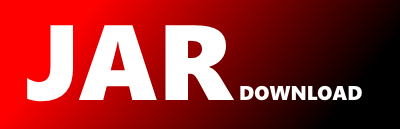
org.neo4j.driver.internal.bolt.basicimpl.NettyBoltConnectionProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neo4j-java-driver Show documentation
Show all versions of neo4j-java-driver Show documentation
Access to the Neo4j graph database through Java
The newest version!
/*
* Copyright (c) "Neo4j"
* Neo4j Sweden AB [https://neo4j.com]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.neo4j.driver.internal.bolt.basicimpl;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.local.LocalAddress;
import io.netty.util.internal.logging.InternalLoggerFactory;
import java.time.Clock;
import java.util.Collections;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionException;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.atomic.AtomicReference;
import java.util.function.Consumer;
import java.util.function.Supplier;
import org.neo4j.driver.Value;
import org.neo4j.driver.internal.bolt.api.AccessMode;
import org.neo4j.driver.internal.bolt.api.BoltAgent;
import org.neo4j.driver.internal.bolt.api.BoltConnection;
import org.neo4j.driver.internal.bolt.api.BoltConnectionProvider;
import org.neo4j.driver.internal.bolt.api.BoltProtocolVersion;
import org.neo4j.driver.internal.bolt.api.BoltServerAddress;
import org.neo4j.driver.internal.bolt.api.DatabaseName;
import org.neo4j.driver.internal.bolt.api.DomainNameResolver;
import org.neo4j.driver.internal.bolt.api.LoggingProvider;
import org.neo4j.driver.internal.bolt.api.MetricsListener;
import org.neo4j.driver.internal.bolt.api.NotificationConfig;
import org.neo4j.driver.internal.bolt.api.RoutingContext;
import org.neo4j.driver.internal.bolt.api.SecurityPlan;
import org.neo4j.driver.internal.bolt.api.exception.MinVersionAcquisitionException;
import org.neo4j.driver.internal.bolt.basicimpl.messaging.v4.BoltProtocolV4;
import org.neo4j.driver.internal.bolt.basicimpl.messaging.v51.BoltProtocolV51;
import org.neo4j.driver.internal.bolt.basicimpl.util.FutureUtil;
public final class NettyBoltConnectionProvider implements BoltConnectionProvider {
private final LoggingProvider logging;
private final System.Logger log;
private final ConnectionProvider connectionProvider;
private BoltServerAddress address;
private RoutingContext routingContext;
private BoltAgent boltAgent;
private String userAgent;
private int connectTimeoutMillis;
private CompletableFuture closeFuture;
private MetricsListener metricsListener;
private final Clock clock;
public NettyBoltConnectionProvider(
EventLoopGroup eventLoopGroup,
Clock clock,
DomainNameResolver domainNameResolver,
LocalAddress localAddress,
LoggingProvider logging) {
Objects.requireNonNull(eventLoopGroup);
this.clock = Objects.requireNonNull(clock);
this.logging = Objects.requireNonNull(logging);
this.log = logging.getLog(getClass());
this.connectionProvider =
ConnectionProviders.netty(eventLoopGroup, clock, domainNameResolver, localAddress, logging);
}
@Override
public CompletionStage init(
BoltServerAddress address,
RoutingContext routingContext,
BoltAgent boltAgent,
String userAgent,
int connectTimeoutMillis,
MetricsListener metricsListener) {
this.address = address;
this.routingContext = routingContext;
this.boltAgent = boltAgent;
this.userAgent = userAgent;
this.connectTimeoutMillis = connectTimeoutMillis;
this.metricsListener = NoopMetricsListener.getInstance();
InternalLoggerFactory.setDefaultFactory(new NettyLogging(logging));
return CompletableFuture.completedStage(null);
}
@Override
public CompletionStage connect(
SecurityPlan securityPlan,
DatabaseName databaseName,
Supplier>> authMapStageSupplier,
AccessMode mode,
Set bookmarks,
String impersonatedUser,
BoltProtocolVersion minVersion,
NotificationConfig notificationConfig,
Consumer databaseNameConsumer) {
synchronized (this) {
if (closeFuture != null) {
return CompletableFuture.failedFuture(new IllegalStateException("Connection provider is closed."));
}
}
var latestAuthMillisFuture = new CompletableFuture();
var authMapRef = new AtomicReference
© 2015 - 2024 Weber Informatics LLC | Privacy Policy