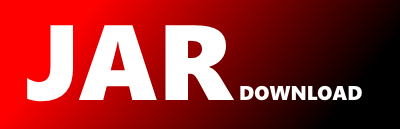
org.neo4j.gds.pricesteiner.PrizeSteinerTreeMemoryEstimateDefinition Maven / Gradle / Ivy
Go to download
International Component for Unicode for Java (ICU4J) is a mature, widely used Java library
providing Unicode and Globalization support
Please wait ...