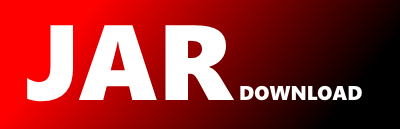
apoc.export.util.MetaInformation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apoc-common Show documentation
Show all versions of apoc-common Show documentation
Data types package for Neo4j Procedures
/*
* Copyright (c) "Neo4j"
* Neo4j Sweden AB [http://neo4j.com]
*
* This file is part of Neo4j.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package apoc.export.util;
import static apoc.gephi.GephiFormatUtils.getCaption;
import static apoc.meta.tablesforlabels.PropertyTracker.typeMappings;
import static apoc.util.collection.Iterables.stream;
import static java.util.Arrays.asList;
import apoc.meta.Types;
import apoc.util.MapUtil;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
import org.apache.commons.lang3.ClassUtils;
import org.neo4j.cypher.export.SubGraph;
import org.neo4j.graphdb.Entity;
import org.neo4j.graphdb.GraphDatabaseService;
import org.neo4j.graphdb.Label;
import org.neo4j.graphdb.Node;
import org.neo4j.graphdb.Relationship;
import org.neo4j.graphdb.RelationshipType;
import org.neo4j.graphdb.ResultTransformer;
/**
* @author mh
* @since 19.01.14
*/
public class MetaInformation {
private static final Map REVERSED_TYPE_MAP = MapUtil.invertMap(typeMappings);
public static Map collectPropTypesForNodes(
SubGraph graph, GraphDatabaseService db, ExportConfig config) {
if (!config.isSampling()) {
Map propTypes = new LinkedHashMap<>();
for (Node node : graph.getNodes()) {
updateKeyTypes(propTypes, node);
}
return propTypes;
}
final Map conf = config.getSamplingConfig();
conf.putIfAbsent(
"includeLabels",
stream(graph.getAllLabelsInUse()).map(Label::name).collect(Collectors.toList()));
return db.executeTransactionally(
"CALL apoc.meta.nodeTypeProperties($conf)", Map.of("conf", conf), getMapResultTransformer());
}
public static Map collectPropTypesForRelationships(
SubGraph graph, GraphDatabaseService db, ExportConfig config) {
if (!config.isSampling()) {
Map propTypes = new LinkedHashMap<>();
for (Relationship relationship : graph.getRelationships()) {
updateKeyTypes(propTypes, relationship);
}
return propTypes;
}
final Map conf = config.getSamplingConfig();
conf.putIfAbsent(
"includeRels",
stream(graph.getAllRelationshipTypesInUse())
.map(RelationshipType::name)
.collect(Collectors.toList()));
return db.executeTransactionally(
"CALL apoc.meta.relTypeProperties($conf)", Map.of("conf", conf), getMapResultTransformer());
}
private static ResultTransformer
© 2015 - 2024 Weber Informatics LLC | Privacy Policy