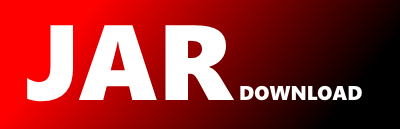
apoc.util.JsonUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apoc-common Show documentation
Show all versions of apoc-common Show documentation
Data types package for Neo4j Procedures
/*
* Copyright (c) "Neo4j"
* Neo4j Sweden AB [http://neo4j.com]
*
* This file is part of Neo4j.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package apoc.util;
import apoc.export.util.DurationValueSerializer;
import apoc.export.util.PointSerializer;
import apoc.export.util.TemporalSerializer;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.MappingIterator;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.module.SimpleModule;
import com.jayway.jsonpath.Configuration;
import com.jayway.jsonpath.JsonPath;
import com.jayway.jsonpath.Option;
import com.jayway.jsonpath.spi.json.JacksonJsonProvider;
import com.jayway.jsonpath.spi.mapper.JacksonMappingProvider;
import java.io.IOException;
import java.io.InputStream;
import java.net.URISyntaxException;
import java.time.temporal.Temporal;
import java.util.EnumSet;
import java.util.List;
import java.util.Map;
import java.util.Spliterators;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
import org.apache.commons.lang3.StringUtils;
import org.neo4j.graphdb.security.URLAccessChecker;
import org.neo4j.graphdb.security.URLAccessValidationError;
import org.neo4j.graphdb.spatial.Point;
import org.neo4j.values.storable.DurationValue;
/**
* @author mh
* @since 04.05.16
*/
public class JsonUtil {
private static final Option[] defaultJsonPathOptions = {Option.DEFAULT_PATH_LEAF_TO_NULL, Option.SUPPRESS_EXCEPTIONS
};
public static final ObjectMapper OBJECT_MAPPER = new ObjectMapper();
public static final String PATH_OPTIONS_ERROR_MESSAGE =
"Invalid pathOptions. The allowed values are: " + EnumSet.allOf(Option.class);
static {
OBJECT_MAPPER.configure(JsonParser.Feature.ALLOW_UNQUOTED_FIELD_NAMES, true);
OBJECT_MAPPER.configure(JsonParser.Feature.AUTO_CLOSE_SOURCE, false);
OBJECT_MAPPER.configure(JsonParser.Feature.ALLOW_SINGLE_QUOTES, true);
OBJECT_MAPPER.configure(JsonParser.Feature.ALLOW_UNQUOTED_FIELD_NAMES, true);
OBJECT_MAPPER.configure(JsonParser.Feature.ALLOW_COMMENTS, true);
OBJECT_MAPPER.configure(JsonParser.Feature.ALLOW_BACKSLASH_ESCAPING_ANY_CHARACTER, true);
OBJECT_MAPPER.configure(JsonParser.Feature.ALLOW_NON_NUMERIC_NUMBERS, true);
OBJECT_MAPPER.enable(DeserializationFeature.USE_LONG_FOR_INTS);
SimpleModule module = new SimpleModule("Neo4jApocSerializer");
module.addSerializer(Point.class, new PointSerializer());
module.addSerializer(Temporal.class, new TemporalSerializer());
module.addSerializer(DurationValue.class, new DurationValueSerializer());
OBJECT_MAPPER.registerModule(module);
}
private static Configuration getJsonPathConfig(List options) {
try {
Option[] opts = options == null
? defaultJsonPathOptions
: options.stream().map(Option::valueOf).toArray(Option[]::new);
return Configuration.builder()
.options(opts)
.jsonProvider(new JacksonJsonProvider(OBJECT_MAPPER))
.mappingProvider(new JacksonMappingProvider(OBJECT_MAPPER))
.build();
} catch (Exception e) {
throw new RuntimeException(PATH_OPTIONS_ERROR_MESSAGE, e);
}
}
public static Stream
© 2015 - 2024 Weber Informatics LLC | Privacy Policy