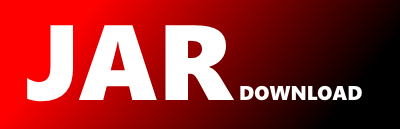
apoc.load.Mapping Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apoc-common Show documentation
Show all versions of apoc-common Show documentation
Data types package for Neo4j Procedures
/*
* Copyright (c) "Neo4j"
* Neo4j Sweden AB [http://neo4j.com]
*
* This file is part of Neo4j.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package apoc.load;
import static apoc.ApocConfig.apocConfig;
import static apoc.util.Util.parseCharFromConfig;
import static java.util.Collections.emptyList;
import static java.util.Collections.emptyMap;
import static org.neo4j.configuration.GraphDatabaseSettings.db_temporal_timezone;
import apoc.meta.Types;
import apoc.util.Util;
import java.time.ZoneId;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.function.Supplier;
import java.util.regex.Pattern;
import java.util.stream.Collectors;
import org.apache.commons.lang3.StringUtils;
import org.neo4j.values.storable.DateTimeValue;
import org.neo4j.values.storable.DateValue;
import org.neo4j.values.storable.DurationValue;
import org.neo4j.values.storable.LocalDateTimeValue;
import org.neo4j.values.storable.LocalTimeValue;
import org.neo4j.values.storable.TimeValue;
public class Mapping {
final String name;
final Collection nullValues;
final Types type;
final boolean array;
final boolean ignore;
final char arraySep;
private final Pattern arrayPattern;
private final Map optionalData;
public Mapping(String name, Map mapping, char arraySep, boolean ignore) {
this.name = mapping.getOrDefault("name", name).toString();
this.array = (Boolean) mapping.getOrDefault("array", false);
this.optionalData = (Map) mapping.getOrDefault("optionalData", emptyMap());
this.ignore = (Boolean) mapping.getOrDefault("ignore", ignore);
this.nullValues = (Collection) mapping.getOrDefault("nullValues", emptyList());
this.arraySep = parseCharFromConfig(mapping, "arraySep", arraySep);
this.type = Types.from(mapping.getOrDefault("type", "STRING").toString());
this.arrayPattern = Pattern.compile(String.valueOf(this.arraySep), Pattern.LITERAL);
if (this.type == null) {
// Call this out to the user explicitly because deep inside of LoadCSV and others you will get
// NPEs that are hard to spot if this is allowed to go through.
throw new RuntimeException("In specified mapping, there is no type by the name "
+ mapping.getOrDefault("type", "STRING").toString());
}
}
public Object convert(String value) {
return array ? convertArray(value) : convertType(value);
}
private Object convertArray(String value) {
String[] values = arrayPattern.split(value);
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy