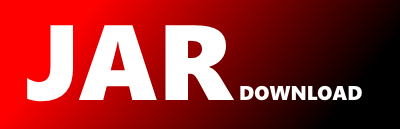
apoc.result.VirtualNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apoc-common Show documentation
Show all versions of apoc-common Show documentation
Data types package for Neo4j Procedures
/*
* Copyright (c) "Neo4j"
* Neo4j Sweden AB [http://neo4j.com]
*
* This file is part of Neo4j.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package apoc.result;
import static java.util.Arrays.asList;
import apoc.util.Util;
import apoc.util.collection.FilteringIterable;
import apoc.util.collection.Iterables;
import java.util.*;
import java.util.concurrent.atomic.AtomicLong;
import java.util.stream.Collectors;
import org.neo4j.graphdb.*;
/**
* @author mh
* @since 16.03.16
*/
public class VirtualNode implements Node {
public static final String ERROR_NODE_NULL = "The inserted Node is null";
private static AtomicLong MIN_ID = new AtomicLong(-1);
private final Set labels = new LinkedHashSet<>();
private final Map props = new HashMap<>();
private final List rels = new ArrayList<>();
private final long id;
private final String elementId;
public VirtualNode(Label[] labels, Map props) {
// to not overlap this ids with ids from VirtualNode(Node node, List propertyNames)
this.id = MIN_ID.decrementAndGet();
addLabels(asList(labels));
this.props.putAll(props);
this.elementId = null;
}
@SuppressWarnings("unused") // used from extended
public VirtualNode(long nodeId, Label[] labels, Map props) {
this.id = nodeId;
addLabels(asList(labels));
this.props.putAll(props);
this.elementId = null;
}
public VirtualNode(long nodeId) {
this.id = nodeId;
this.elementId = null;
}
public VirtualNode(Node node, List propertyNames) {
Objects.requireNonNull(node, ERROR_NODE_NULL);
final long id = node.getId();
// if node is already virtual, we return the same id
this.id = id < 0 ? id : -id - 1;
// to not overlap this ids with ids from VirtualNode(Label[] labels, Map props)
MIN_ID.updateAndGet(x -> Math.min(x, this.id));
this.labels.addAll(Util.labelStrings(node));
String[] keys = propertyNames.toArray(new String[propertyNames.size()]);
this.props.putAll(node.getProperties(keys));
this.elementId = node.getElementId();
}
public static VirtualNode from(Node node) {
return new VirtualNode(node, Iterables.asList(node.getPropertyKeys()));
}
@Override
public long getId() {
return id;
}
@Override
public String getElementId() {
return elementId != null ? elementId : String.valueOf(id);
}
@Override
public void delete() {
for (Relationship rel : rels) {
rel.delete();
}
}
@Override
public ResourceIterable getRelationships() {
return Iterables.asResourceIterable(rels);
}
@Override
public boolean hasRelationship() {
return !rels.isEmpty();
}
@Override
public ResourceIterable getRelationships(RelationshipType... relationshipTypes) {
return Iterables.asResourceIterable(new FilteringIterable<>(rels, (r) -> isType(r, relationshipTypes)));
}
private boolean isType(Relationship r, RelationshipType... relationshipTypes) {
for (RelationshipType type : relationshipTypes) {
if (r.isType(type)) return true;
}
return false;
}
@Override
public ResourceIterable getRelationships(Direction direction, RelationshipType... relationshipTypes) {
return Iterables.asResourceIterable(
new FilteringIterable<>(rels, (r) -> isType(r, relationshipTypes) && isDirection(r, direction)));
}
private boolean isDirection(Relationship r, Direction direction) {
return direction == Direction.BOTH
|| direction == Direction.OUTGOING && r.getStartNode().equals(this)
|| direction == Direction.INCOMING && r.getEndNode().equals(this);
}
@Override
public boolean hasRelationship(RelationshipType... relationshipTypes) {
return getRelationships(relationshipTypes).iterator().hasNext();
}
@Override
public boolean hasRelationship(Direction direction, RelationshipType... relationshipTypes) {
return getRelationships(direction, relationshipTypes).iterator().hasNext();
}
@Override
public ResourceIterable getRelationships(Direction direction) {
return Iterables.asResourceIterable(new FilteringIterable<>(rels, (r) -> isDirection(r, direction)));
}
@Override
public boolean hasRelationship(Direction direction) {
return getRelationships(direction).iterator().hasNext();
}
@Override
public Relationship getSingleRelationship(RelationshipType relationshipType, Direction direction) {
return Iterables.single(getRelationships(direction, relationshipType));
}
@Override
public VirtualRelationship createRelationshipTo(Node node, RelationshipType relationshipType) {
VirtualRelationship rel = new VirtualRelationship(this, node, relationshipType);
rels.add(rel);
if (node
instanceof
VirtualNode) { // register the inverse relationship into the target virtual node only if it is not a
// self relationship
VirtualNode target = (VirtualNode) node;
if (!target.rels.contains(rel)) {
target.rels.add(rel);
}
}
return rel;
}
public VirtualRelationship createRelationshipFrom(Node start, RelationshipType relationshipType) {
VirtualRelationship rel = new VirtualRelationship(start, this, relationshipType);
rels.add(rel);
if (start
instanceof
VirtualNode) { // register the inverse relationship into the start virtual node only if it is not a self
// relationship
VirtualNode startVirtual = (VirtualNode) start;
if (!startVirtual.rels.contains(rel)) {
startVirtual.rels.add(rel);
}
}
return rel;
}
@Override
public Iterable getRelationshipTypes() {
return rels.stream().map(Relationship::getType).collect(Collectors.toList());
}
@Override
public int getDegree() {
return rels.size();
}
@Override
public int getDegree(RelationshipType relationshipType) {
return (int) Iterables.count(getRelationships(relationshipType));
}
@Override
public int getDegree(Direction direction) {
return (int) Iterables.count(getRelationships(direction));
}
@Override
public int getDegree(RelationshipType relationshipType, Direction direction) {
return (int) Iterables.count(getRelationships(direction, relationshipType));
}
@Override
public void addLabel(Label label) {
labels.add(label.name());
}
public void addLabels(Iterable
© 2015 - 2024 Weber Informatics LLC | Privacy Policy