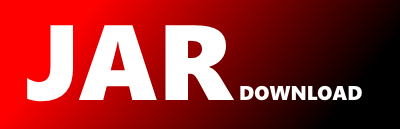
apoc.meta.MetaConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apoc-common Show documentation
Show all versions of apoc-common Show documentation
Data types package for Neo4j Procedures
package apoc.meta;
import apoc.util.Util;
import org.neo4j.graphdb.Label;
import org.neo4j.graphdb.Relationship;
import org.neo4j.graphdb.RelationshipType;
import java.util.*;
public class MetaConfig {
private final Set includesLabels;
private final Set includesRels;
private final Set excludes;
private final Set excludeRels;
private final long maxRels;
private final long sample;
private final boolean addRelationshipsBetweenNodes;
/**
* A map of values, with the following keys and meanings.
* - labels: a list of strings, which are allowlisted node labels. If this list
* is specified **only these labels** will be examined.
* - rels: a list of strings, which are allowlisted rel types. If this list is
* specified, **only these reltypes** will be examined.
* - excludes: a list of strings, which are node labels. This
* works like a denylist: if listed here, the thing won't be considered. Everything
* else (subject to the allowlist) will be.
* - sample: a long number, i.e. "1 in (SAMPLE)". If set to 1000 this means that
* every 1000th node will be examined. It does **not** mean that a total of 1000 nodes
* will be sampled.
* - maxRels: the maximum number of relationships of a given type to look at.
* @param config
*/
public MetaConfig(Map config) {
config = config != null ? config : Collections.emptyMap();
// To maintain backwards compatibility, need to still support "labels", "rels" and "excludes" for "includeLabels", "includeRels" and "excludeLabels" respectively.
Set includesLabelsLocal = new HashSet<>((Collection)config.getOrDefault("labels",Collections.EMPTY_SET));
Set includesRelsLocal = new HashSet<>((Collection)config.getOrDefault("rels",Collections.EMPTY_SET));
Set excludesLocal = new HashSet<>((Collection)config.getOrDefault("excludes",Collections.EMPTY_SET));
if (includesLabelsLocal.isEmpty()) {
includesLabelsLocal = new HashSet<>((Collection)config.getOrDefault("includeLabels",Collections.EMPTY_SET));
}
if (includesRelsLocal.isEmpty()) {
includesRelsLocal = new HashSet<>((Collection)config.getOrDefault("includeRels",Collections.EMPTY_SET));
}
if (excludesLocal.isEmpty()) {
excludesLocal = new HashSet<>((Collection)config.getOrDefault("excludeLabels",Collections.EMPTY_SET));
}
this.includesLabels = includesLabelsLocal;
this.includesRels = includesRelsLocal;
this.excludes = excludesLocal;
this.excludeRels = new HashSet<>((Collection)config.getOrDefault("excludeRels",Collections.EMPTY_SET));
this.sample = (long) config.getOrDefault("sample", 1000L);
this.maxRels = (long) config.getOrDefault("maxRels", 100L);
this.addRelationshipsBetweenNodes = Util.toBoolean(config.getOrDefault("addRelationshipsBetweenNodes", true));
}
public Set getIncludesLabels() {
return includesLabels;
}
public Set getIncludesRels() {
return includesRels;
}
public Set getExcludes() {
return excludes;
}
public Set getExcludeRels() {
return excludeRels;
}
public long getSample() {
return sample;
}
public long getMaxRels() {
return maxRels;
}
/**
* @param l
* @return true if the label matches the mask expressed by this object, false otherwise.
*/
public boolean matches(Label l) {
if (getExcludes().contains(l.name())) { return false; }
if (getIncludesLabels().isEmpty()) { return true; }
return getIncludesLabels().contains(l.name());
}
/**
* @param labels
* @return true if any of the labels matches the mask expressed by this object, false otherwise.
*/
public boolean matches(Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy