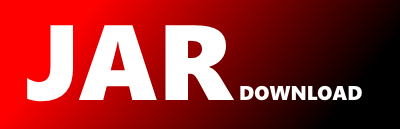
apoc.agg.Rollup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apoc-extended Show documentation
Show all versions of apoc-extended Show documentation
Extended package for Neo4j Procedures
package apoc.agg;
import apoc.Extended;
import apoc.util.ExtendedListUtils;
import apoc.util.Util;
import org.neo4j.graphdb.Entity;
import org.neo4j.procedure.Description;
import org.neo4j.procedure.Name;
import org.neo4j.procedure.UserAggregationFunction;
import org.neo4j.procedure.UserAggregationResult;
import org.neo4j.procedure.UserAggregationUpdate;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import static apoc.agg.AggregationUtil.updateAggregationValues;
@Extended
public class Rollup {
public static final String NULL_ROLLUP = "[NULL]";
@UserAggregationFunction("apoc.agg.rollup")
@Description("apoc.agg.rollup(, [groupKeys], [aggKeys])" +
"\n Emulate an Oracle/Mysql rollup command: `ROLLUP groupKeys, SUM(aggKey1), AVG(aggKey1), COUNT(aggKey1), SUM(aggKey2), AVG(aggKey2), ... `")
public RollupFunction rollup() {
return new RollupFunction();
}
public static class RollupFunction {
// Function to generate all combinations of a list with "TEST" as a placeholder
public static List> generateCombinationsWithPlaceholder(List elements) {
List> result = new ArrayList<>();
generateCombinationsWithPlaceholder(elements, 0, new ArrayList<>(), result);
return result;
}
// Helper function for generating combinations recursively
private static void generateCombinationsWithPlaceholder(List elements, int index, List current, List> result) {
if (index == elements.size()) {
result.add(new ArrayList<>(current));
return;
}
current.add(elements.get(index));
generateCombinationsWithPlaceholder(elements, index + 1, current, result);
current.remove(current.size() - 1);
// Add "NULL" as a combination placeholder
current.add((T) NULL_ROLLUP);
generateCombinationsWithPlaceholder(elements, index + 1, current, result);
current.remove(current.size() - 1);
}
private final Map result = new HashMap<>();
private final Map, Map> rolledUpData = new HashMap<>();
private List groupKeysRes = null;
@UserAggregationUpdate
public void aggregate(
@Name("value") Object value,
@Name(value = "groupKeys") List groupKeys,
@Name(value = "aggKeys") List aggKeys,
@Name(value = "config", defaultValue = "{}") Map config) {
boolean cube = Util.toBoolean(config.get("cube"));
Entity entity = (Entity) value;
if (groupKeys.isEmpty()) {
return;
}
groupKeysRes = groupKeys;
/*
if true:
emulate the CUBE command: https://docs.oracle.com/cd/F49540_01/DOC/server.815/a68003/rollup_c.htm#32311
else:
emulate the ROLLUP command: https://docs.oracle.com/cd/F49540_01/DOC/server.815/a68003/rollup_c.htm#32084
*/
if (cube) {
List> groupingSets = generateCombinationsWithPlaceholder(groupKeys);
for (List groupKey : groupingSets) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy