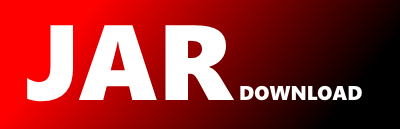
org.neo4j.cypher.internal.parser.v6.Cypher6Parser Maven / Gradle / Ivy
/*
* Copyright (c) "Neo4j"
* Neo4j Sweden AB [https://neo4j.com]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated from org/neo4j/cypher/internal/parser/v6/Cypher6Parser.g4 by ANTLR 4.13.2
package org.neo4j.cypher.internal.parser.v6;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast", "CheckReturnValue", "this-escape"})
public class Cypher6Parser extends Parser {
static { RuntimeMetaData.checkVersion("4.13.2", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
SPACE=1, SINGLE_LINE_COMMENT=2, MULTI_LINE_COMMENT=3, DECIMAL_DOUBLE=4,
UNSIGNED_DECIMAL_INTEGER=5, UNSIGNED_HEX_INTEGER=6, UNSIGNED_OCTAL_INTEGER=7,
STRING_LITERAL1=8, STRING_LITERAL2=9, ESCAPED_SYMBOLIC_NAME=10, ACCESS=11,
ACTIVE=12, ADMIN=13, ADMINISTRATOR=14, ALIAS=15, ALIASES=16, ALL_SHORTEST_PATHS=17,
ALL=18, ALTER=19, AND=20, ANY=21, ARRAY=22, AS=23, ASC=24, ASCENDING=25,
ASSIGN=26, AT=27, AUTH=28, BAR=29, BINDINGS=30, BOOL=31, BOOLEAN=32, BOOSTED=33,
BOTH=34, BREAK=35, BTREE=36, BUILT=37, BY=38, CALL=39, CASCADE=40, CASE=41,
CHANGE=42, CIDR=43, COLLECT=44, COLON=45, COLONCOLON=46, COMMA=47, COMMAND=48,
COMMANDS=49, COMPOSITE=50, CONCURRENT=51, CONSTRAINT=52, CONSTRAINTS=53,
CONTAINS=54, COPY=55, CONTINUE=56, COUNT=57, CREATE=58, CSV=59, CURRENT=60,
DATA=61, DATABASE=62, DATABASES=63, DATE=64, DATETIME=65, DBMS=66, DEALLOCATE=67,
DEFAULT=68, DEFINED=69, DELETE=70, DENY=71, DESC=72, DESCENDING=73, DESTROY=74,
DETACH=75, DIFFERENT=76, DOLLAR=77, DISTINCT=78, DIVIDE=79, DOT=80, DOTDOT=81,
DOUBLEBAR=82, DRIVER=83, DROP=84, DRYRUN=85, DUMP=86, DURATION=87, EACH=88,
EDGE=89, ENABLE=90, ELEMENT=91, ELEMENTS=92, ELSE=93, ENCRYPTED=94, END=95,
ENDS=96, EQ=97, EXECUTABLE=98, EXECUTE=99, EXIST=100, EXISTENCE=101, EXISTS=102,
ERROR=103, FAIL=104, FALSE=105, FIELDTERMINATOR=106, FINISH=107, FLOAT=108,
FOR=109, FOREACH=110, FROM=111, FULLTEXT=112, FUNCTION=113, FUNCTIONS=114,
GE=115, GRANT=116, GRAPH=117, GRAPHS=118, GROUP=119, GROUPS=120, GT=121,
HEADERS=122, HOME=123, ID=124, IF=125, IMPERSONATE=126, IMMUTABLE=127,
IN=128, INDEX=129, INDEXES=130, INF=131, INFINITY=132, INSERT=133, INT=134,
INTEGER=135, IS=136, JOIN=137, KEY=138, LABEL=139, LABELS=140, AMPERSAND=141,
EXCLAMATION_MARK=142, LBRACKET=143, LCURLY=144, LE=145, LEADING=146, LIMITROWS=147,
LIST=148, LOAD=149, LOCAL=150, LOOKUP=151, LPAREN=152, LT=153, MANAGEMENT=154,
MAP=155, MATCH=156, MERGE=157, MINUS=158, PERCENT=159, INVALID_NEQ=160,
NEQ=161, NAME=162, NAMES=163, NAN=164, NFC=165, NFD=166, NFKC=167, NFKD=168,
NEW=169, NODE=170, NODETACH=171, NODES=172, NONE=173, NORMALIZE=174, NORMALIZED=175,
NOT=176, NOTHING=177, NOWAIT=178, NULL=179, OF=180, OFFSET=181, ON=182,
ONLY=183, OPTIONAL=184, OPTIONS=185, OPTION=186, OR=187, ORDER=188, PASSWORD=189,
PASSWORDS=190, PATH=191, PATHS=192, PLAINTEXT=193, PLUS=194, PLUSEQUAL=195,
POINT=196, POPULATED=197, POW=198, PRIMARY=199, PRIMARIES=200, PRIVILEGE=201,
PRIVILEGES=202, PROCEDURE=203, PROCEDURES=204, PROPERTIES=205, PROPERTY=206,
PROVIDER=207, PROVIDERS=208, QUESTION=209, RANGE=210, RBRACKET=211, RCURLY=212,
READ=213, REALLOCATE=214, REDUCE=215, RENAME=216, REGEQ=217, REL=218,
RELATIONSHIP=219, RELATIONSHIPS=220, REMOVE=221, REPEATABLE=222, REPLACE=223,
REPORT=224, REQUIRE=225, REQUIRED=226, RESTRICT=227, RETURN=228, REVOKE=229,
ROLE=230, ROLES=231, ROW=232, ROWS=233, RPAREN=234, SCAN=235, SEC=236,
SECOND=237, SECONDARY=238, SECONDARIES=239, SECONDS=240, SEEK=241, SEMICOLON=242,
SERVER=243, SERVERS=244, SET=245, SETTING=246, SETTINGS=247, SHORTEST_PATH=248,
SHORTEST=249, SHOW=250, SIGNED=251, SINGLE=252, SKIPROWS=253, START=254,
STARTS=255, STATUS=256, STOP=257, STRING=258, SUPPORTED=259, SUSPENDED=260,
TARGET=261, TERMINATE=262, TEXT=263, THEN=264, TIME=265, TIMES=266, TIMESTAMP=267,
TIMEZONE=268, TO=269, TOPOLOGY=270, TRAILING=271, TRANSACTION=272, TRANSACTIONS=273,
TRAVERSE=274, TRIM=275, TRUE=276, TYPE=277, TYPED=278, TYPES=279, UNION=280,
UNIQUE=281, UNIQUENESS=282, UNWIND=283, URL=284, USE=285, USER=286, USERS=287,
USING=288, VALUE=289, VARCHAR=290, VECTOR=291, VERTEX=292, WAIT=293, WHEN=294,
WHERE=295, WITH=296, WITHOUT=297, WRITE=298, XOR=299, YIELD=300, ZONE=301,
ZONED=302, IDENTIFIER=303, EXTENDED_IDENTIFIER=304, ARROW_LINE=305, ARROW_LEFT_HEAD=306,
ARROW_RIGHT_HEAD=307, ErrorChar=308;
public static final int
RULE_statements = 0, RULE_statement = 1, RULE_regularQuery = 2, RULE_singleQuery = 3,
RULE_clause = 4, RULE_useClause = 5, RULE_graphReference = 6, RULE_finishClause = 7,
RULE_returnClause = 8, RULE_returnBody = 9, RULE_returnItem = 10, RULE_returnItems = 11,
RULE_orderItem = 12, RULE_ascToken = 13, RULE_descToken = 14, RULE_orderBy = 15,
RULE_skip = 16, RULE_limit = 17, RULE_whereClause = 18, RULE_withClause = 19,
RULE_createClause = 20, RULE_insertClause = 21, RULE_setClause = 22, RULE_setItem = 23,
RULE_removeClause = 24, RULE_removeItem = 25, RULE_deleteClause = 26,
RULE_matchClause = 27, RULE_matchMode = 28, RULE_hint = 29, RULE_mergeClause = 30,
RULE_mergeAction = 31, RULE_unwindClause = 32, RULE_callClause = 33, RULE_procedureName = 34,
RULE_procedureArgument = 35, RULE_procedureResultItem = 36, RULE_loadCSVClause = 37,
RULE_foreachClause = 38, RULE_subqueryClause = 39, RULE_subqueryScope = 40,
RULE_subqueryInTransactionsParameters = 41, RULE_subqueryInTransactionsBatchParameters = 42,
RULE_subqueryInTransactionsErrorParameters = 43, RULE_subqueryInTransactionsReportParameters = 44,
RULE_orderBySkipLimitClause = 45, RULE_patternList = 46, RULE_insertPatternList = 47,
RULE_pattern = 48, RULE_insertPattern = 49, RULE_quantifier = 50, RULE_anonymousPattern = 51,
RULE_shortestPathPattern = 52, RULE_patternElement = 53, RULE_selector = 54,
RULE_groupToken = 55, RULE_pathToken = 56, RULE_pathPatternNonEmpty = 57,
RULE_nodePattern = 58, RULE_insertNodePattern = 59, RULE_parenthesizedPath = 60,
RULE_nodeLabels = 61, RULE_nodeLabelsIs = 62, RULE_dynamicExpression = 63,
RULE_dynamicLabelType = 64, RULE_labelType = 65, RULE_relType = 66, RULE_labelOrRelType = 67,
RULE_properties = 68, RULE_relationshipPattern = 69, RULE_insertRelationshipPattern = 70,
RULE_leftArrow = 71, RULE_arrowLine = 72, RULE_rightArrow = 73, RULE_pathLength = 74,
RULE_labelExpression = 75, RULE_labelExpression4 = 76, RULE_labelExpression4Is = 77,
RULE_labelExpression3 = 78, RULE_labelExpression3Is = 79, RULE_labelExpression2 = 80,
RULE_labelExpression2Is = 81, RULE_labelExpression1 = 82, RULE_labelExpression1Is = 83,
RULE_insertNodeLabelExpression = 84, RULE_insertRelationshipLabelExpression = 85,
RULE_expression = 86, RULE_expression11 = 87, RULE_expression10 = 88,
RULE_expression9 = 89, RULE_expression8 = 90, RULE_expression7 = 91, RULE_comparisonExpression6 = 92,
RULE_normalForm = 93, RULE_expression6 = 94, RULE_expression5 = 95, RULE_expression4 = 96,
RULE_expression3 = 97, RULE_expression2 = 98, RULE_postFix = 99, RULE_property = 100,
RULE_dynamicProperty = 101, RULE_propertyExpression = 102, RULE_dynamicPropertyExpression = 103,
RULE_expression1 = 104, RULE_literal = 105, RULE_caseExpression = 106,
RULE_caseAlternative = 107, RULE_extendedCaseExpression = 108, RULE_extendedCaseAlternative = 109,
RULE_extendedWhen = 110, RULE_listComprehension = 111, RULE_patternComprehension = 112,
RULE_reduceExpression = 113, RULE_listItemsPredicate = 114, RULE_normalizeFunction = 115,
RULE_trimFunction = 116, RULE_patternExpression = 117, RULE_shortestPathExpression = 118,
RULE_parenthesizedExpression = 119, RULE_mapProjection = 120, RULE_mapProjectionElement = 121,
RULE_countStar = 122, RULE_existsExpression = 123, RULE_countExpression = 124,
RULE_collectExpression = 125, RULE_numberLiteral = 126, RULE_signedIntegerLiteral = 127,
RULE_listLiteral = 128, RULE_propertyKeyName = 129, RULE_parameter = 130,
RULE_parameterName = 131, RULE_functionInvocation = 132, RULE_functionArgument = 133,
RULE_functionName = 134, RULE_namespace = 135, RULE_variable = 136, RULE_nonEmptyNameList = 137,
RULE_type = 138, RULE_typePart = 139, RULE_typeName = 140, RULE_typeNullability = 141,
RULE_typeListSuffix = 142, RULE_command = 143, RULE_createCommand = 144,
RULE_dropCommand = 145, RULE_showCommand = 146, RULE_showCommandYield = 147,
RULE_yieldItem = 148, RULE_yieldSkip = 149, RULE_yieldLimit = 150, RULE_yieldClause = 151,
RULE_commandOptions = 152, RULE_terminateCommand = 153, RULE_composableCommandClauses = 154,
RULE_composableShowCommandClauses = 155, RULE_showIndexCommand = 156,
RULE_showIndexType = 157, RULE_showIndexesEnd = 158, RULE_showConstraintCommand = 159,
RULE_showConstraintEntity = 160, RULE_constraintExistType = 161, RULE_showConstraintsEnd = 162,
RULE_showProcedures = 163, RULE_showFunctions = 164, RULE_functionToken = 165,
RULE_executableBy = 166, RULE_showFunctionsType = 167, RULE_showTransactions = 168,
RULE_terminateTransactions = 169, RULE_showSettings = 170, RULE_settingToken = 171,
RULE_namesAndClauses = 172, RULE_stringsOrExpression = 173, RULE_commandNodePattern = 174,
RULE_commandRelPattern = 175, RULE_createConstraint = 176, RULE_constraintType = 177,
RULE_dropConstraint = 178, RULE_createIndex = 179, RULE_createIndex_ = 180,
RULE_createFulltextIndex = 181, RULE_fulltextNodePattern = 182, RULE_fulltextRelPattern = 183,
RULE_createLookupIndex = 184, RULE_lookupIndexNodePattern = 185, RULE_lookupIndexRelPattern = 186,
RULE_dropIndex = 187, RULE_propertyList = 188, RULE_enclosedPropertyList = 189,
RULE_alterCommand = 190, RULE_renameCommand = 191, RULE_grantCommand = 192,
RULE_denyCommand = 193, RULE_revokeCommand = 194, RULE_userNames = 195,
RULE_roleNames = 196, RULE_roleToken = 197, RULE_enableServerCommand = 198,
RULE_alterServer = 199, RULE_renameServer = 200, RULE_dropServer = 201,
RULE_showServers = 202, RULE_allocationCommand = 203, RULE_deallocateDatabaseFromServers = 204,
RULE_reallocateDatabases = 205, RULE_createRole = 206, RULE_dropRole = 207,
RULE_renameRole = 208, RULE_showRoles = 209, RULE_grantRole = 210, RULE_revokeRole = 211,
RULE_createUser = 212, RULE_dropUser = 213, RULE_renameUser = 214, RULE_alterCurrentUser = 215,
RULE_alterUser = 216, RULE_removeNamedProvider = 217, RULE_password = 218,
RULE_passwordOnly = 219, RULE_passwordExpression = 220, RULE_passwordChangeRequired = 221,
RULE_userStatus = 222, RULE_homeDatabase = 223, RULE_setAuthClause = 224,
RULE_userAuthAttribute = 225, RULE_showUsers = 226, RULE_showCurrentUser = 227,
RULE_showSupportedPrivileges = 228, RULE_showPrivileges = 229, RULE_showRolePrivileges = 230,
RULE_showUserPrivileges = 231, RULE_privilegeAsCommand = 232, RULE_privilegeToken = 233,
RULE_privilege = 234, RULE_allPrivilege = 235, RULE_allPrivilegeType = 236,
RULE_allPrivilegeTarget = 237, RULE_createPrivilege = 238, RULE_createPrivilegeForDatabase = 239,
RULE_createNodePrivilegeToken = 240, RULE_createRelPrivilegeToken = 241,
RULE_createPropertyPrivilegeToken = 242, RULE_actionForDBMS = 243, RULE_dropPrivilege = 244,
RULE_loadPrivilege = 245, RULE_showPrivilege = 246, RULE_setPrivilege = 247,
RULE_passwordToken = 248, RULE_removePrivilege = 249, RULE_writePrivilege = 250,
RULE_databasePrivilege = 251, RULE_dbmsPrivilege = 252, RULE_dbmsPrivilegeExecute = 253,
RULE_adminToken = 254, RULE_procedureToken = 255, RULE_indexToken = 256,
RULE_constraintToken = 257, RULE_transactionToken = 258, RULE_userQualifier = 259,
RULE_executeFunctionQualifier = 260, RULE_executeProcedureQualifier = 261,
RULE_settingQualifier = 262, RULE_globs = 263, RULE_glob = 264, RULE_globRecursive = 265,
RULE_globPart = 266, RULE_qualifiedGraphPrivilegesWithProperty = 267,
RULE_qualifiedGraphPrivileges = 268, RULE_labelsResource = 269, RULE_propertiesResource = 270,
RULE_nonEmptyStringList = 271, RULE_graphQualifier = 272, RULE_graphQualifierToken = 273,
RULE_relToken = 274, RULE_elementToken = 275, RULE_nodeToken = 276, RULE_databaseScope = 277,
RULE_graphScope = 278, RULE_createCompositeDatabase = 279, RULE_createDatabase = 280,
RULE_primaryTopology = 281, RULE_primaryToken = 282, RULE_secondaryTopology = 283,
RULE_secondaryToken = 284, RULE_dropDatabase = 285, RULE_aliasAction = 286,
RULE_alterDatabase = 287, RULE_alterDatabaseAccess = 288, RULE_alterDatabaseTopology = 289,
RULE_alterDatabaseOption = 290, RULE_startDatabase = 291, RULE_stopDatabase = 292,
RULE_waitClause = 293, RULE_secondsToken = 294, RULE_showDatabase = 295,
RULE_aliasName = 296, RULE_databaseName = 297, RULE_createAlias = 298,
RULE_dropAlias = 299, RULE_alterAlias = 300, RULE_alterAliasTarget = 301,
RULE_alterAliasUser = 302, RULE_alterAliasPassword = 303, RULE_alterAliasDriver = 304,
RULE_alterAliasProperties = 305, RULE_showAliases = 306, RULE_symbolicNameOrStringParameter = 307,
RULE_commandNameExpression = 308, RULE_symbolicNameOrStringParameterList = 309,
RULE_symbolicAliasNameList = 310, RULE_symbolicAliasNameOrParameter = 311,
RULE_symbolicAliasName = 312, RULE_stringListLiteral = 313, RULE_stringList = 314,
RULE_stringLiteral = 315, RULE_stringOrParameterExpression = 316, RULE_stringOrParameter = 317,
RULE_mapOrParameter = 318, RULE_map = 319, RULE_symbolicNameString = 320,
RULE_escapedSymbolicNameString = 321, RULE_unescapedSymbolicNameString = 322,
RULE_symbolicLabelNameString = 323, RULE_unescapedLabelSymbolicNameString = 324,
RULE_endOfFile = 325;
private static String[] makeRuleNames() {
return new String[] {
"statements", "statement", "regularQuery", "singleQuery", "clause", "useClause",
"graphReference", "finishClause", "returnClause", "returnBody", "returnItem",
"returnItems", "orderItem", "ascToken", "descToken", "orderBy", "skip",
"limit", "whereClause", "withClause", "createClause", "insertClause",
"setClause", "setItem", "removeClause", "removeItem", "deleteClause",
"matchClause", "matchMode", "hint", "mergeClause", "mergeAction", "unwindClause",
"callClause", "procedureName", "procedureArgument", "procedureResultItem",
"loadCSVClause", "foreachClause", "subqueryClause", "subqueryScope",
"subqueryInTransactionsParameters", "subqueryInTransactionsBatchParameters",
"subqueryInTransactionsErrorParameters", "subqueryInTransactionsReportParameters",
"orderBySkipLimitClause", "patternList", "insertPatternList", "pattern",
"insertPattern", "quantifier", "anonymousPattern", "shortestPathPattern",
"patternElement", "selector", "groupToken", "pathToken", "pathPatternNonEmpty",
"nodePattern", "insertNodePattern", "parenthesizedPath", "nodeLabels",
"nodeLabelsIs", "dynamicExpression", "dynamicLabelType", "labelType",
"relType", "labelOrRelType", "properties", "relationshipPattern", "insertRelationshipPattern",
"leftArrow", "arrowLine", "rightArrow", "pathLength", "labelExpression",
"labelExpression4", "labelExpression4Is", "labelExpression3", "labelExpression3Is",
"labelExpression2", "labelExpression2Is", "labelExpression1", "labelExpression1Is",
"insertNodeLabelExpression", "insertRelationshipLabelExpression", "expression",
"expression11", "expression10", "expression9", "expression8", "expression7",
"comparisonExpression6", "normalForm", "expression6", "expression5",
"expression4", "expression3", "expression2", "postFix", "property", "dynamicProperty",
"propertyExpression", "dynamicPropertyExpression", "expression1", "literal",
"caseExpression", "caseAlternative", "extendedCaseExpression", "extendedCaseAlternative",
"extendedWhen", "listComprehension", "patternComprehension", "reduceExpression",
"listItemsPredicate", "normalizeFunction", "trimFunction", "patternExpression",
"shortestPathExpression", "parenthesizedExpression", "mapProjection",
"mapProjectionElement", "countStar", "existsExpression", "countExpression",
"collectExpression", "numberLiteral", "signedIntegerLiteral", "listLiteral",
"propertyKeyName", "parameter", "parameterName", "functionInvocation",
"functionArgument", "functionName", "namespace", "variable", "nonEmptyNameList",
"type", "typePart", "typeName", "typeNullability", "typeListSuffix",
"command", "createCommand", "dropCommand", "showCommand", "showCommandYield",
"yieldItem", "yieldSkip", "yieldLimit", "yieldClause", "commandOptions",
"terminateCommand", "composableCommandClauses", "composableShowCommandClauses",
"showIndexCommand", "showIndexType", "showIndexesEnd", "showConstraintCommand",
"showConstraintEntity", "constraintExistType", "showConstraintsEnd",
"showProcedures", "showFunctions", "functionToken", "executableBy", "showFunctionsType",
"showTransactions", "terminateTransactions", "showSettings", "settingToken",
"namesAndClauses", "stringsOrExpression", "commandNodePattern", "commandRelPattern",
"createConstraint", "constraintType", "dropConstraint", "createIndex",
"createIndex_", "createFulltextIndex", "fulltextNodePattern", "fulltextRelPattern",
"createLookupIndex", "lookupIndexNodePattern", "lookupIndexRelPattern",
"dropIndex", "propertyList", "enclosedPropertyList", "alterCommand",
"renameCommand", "grantCommand", "denyCommand", "revokeCommand", "userNames",
"roleNames", "roleToken", "enableServerCommand", "alterServer", "renameServer",
"dropServer", "showServers", "allocationCommand", "deallocateDatabaseFromServers",
"reallocateDatabases", "createRole", "dropRole", "renameRole", "showRoles",
"grantRole", "revokeRole", "createUser", "dropUser", "renameUser", "alterCurrentUser",
"alterUser", "removeNamedProvider", "password", "passwordOnly", "passwordExpression",
"passwordChangeRequired", "userStatus", "homeDatabase", "setAuthClause",
"userAuthAttribute", "showUsers", "showCurrentUser", "showSupportedPrivileges",
"showPrivileges", "showRolePrivileges", "showUserPrivileges", "privilegeAsCommand",
"privilegeToken", "privilege", "allPrivilege", "allPrivilegeType", "allPrivilegeTarget",
"createPrivilege", "createPrivilegeForDatabase", "createNodePrivilegeToken",
"createRelPrivilegeToken", "createPropertyPrivilegeToken", "actionForDBMS",
"dropPrivilege", "loadPrivilege", "showPrivilege", "setPrivilege", "passwordToken",
"removePrivilege", "writePrivilege", "databasePrivilege", "dbmsPrivilege",
"dbmsPrivilegeExecute", "adminToken", "procedureToken", "indexToken",
"constraintToken", "transactionToken", "userQualifier", "executeFunctionQualifier",
"executeProcedureQualifier", "settingQualifier", "globs", "glob", "globRecursive",
"globPart", "qualifiedGraphPrivilegesWithProperty", "qualifiedGraphPrivileges",
"labelsResource", "propertiesResource", "nonEmptyStringList", "graphQualifier",
"graphQualifierToken", "relToken", "elementToken", "nodeToken", "databaseScope",
"graphScope", "createCompositeDatabase", "createDatabase", "primaryTopology",
"primaryToken", "secondaryTopology", "secondaryToken", "dropDatabase",
"aliasAction", "alterDatabase", "alterDatabaseAccess", "alterDatabaseTopology",
"alterDatabaseOption", "startDatabase", "stopDatabase", "waitClause",
"secondsToken", "showDatabase", "aliasName", "databaseName", "createAlias",
"dropAlias", "alterAlias", "alterAliasTarget", "alterAliasUser", "alterAliasPassword",
"alterAliasDriver", "alterAliasProperties", "showAliases", "symbolicNameOrStringParameter",
"commandNameExpression", "symbolicNameOrStringParameterList", "symbolicAliasNameList",
"symbolicAliasNameOrParameter", "symbolicAliasName", "stringListLiteral",
"stringList", "stringLiteral", "stringOrParameterExpression", "stringOrParameter",
"mapOrParameter", "map", "symbolicNameString", "escapedSymbolicNameString",
"unescapedSymbolicNameString", "symbolicLabelNameString", "unescapedLabelSymbolicNameString",
"endOfFile"
};
}
public static final String[] ruleNames = makeRuleNames();
private static String[] makeLiteralNames() {
return new String[] {
null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, "'|'", null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, "':'", "'::'",
"','", null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, "'$'", null, "'/'", "'.'", "'..'",
"'||'", null, null, null, null, null, null, null, null, null, null, null,
null, null, null, "'='", null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, "'>='", null, null,
null, null, null, "'>'", null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, "'&'",
"'!'", "'['", "'{'", "'<='", null, null, null, null, null, null, "'('",
"'<'", null, null, null, null, "'-'", "'%'", "'!='", "'<>'", null, null,
null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, "'+'", "'+='", null, null, "'^'",
null, null, null, null, null, null, null, null, null, null, "'?'", null,
"']'", "'}'", null, null, null, null, "'=~'", null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null,
"')'", null, null, null, null, null, null, null, "';'", null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, "'*'"
};
}
private static final String[] _LITERAL_NAMES = makeLiteralNames();
private static String[] makeSymbolicNames() {
return new String[] {
null, "SPACE", "SINGLE_LINE_COMMENT", "MULTI_LINE_COMMENT", "DECIMAL_DOUBLE",
"UNSIGNED_DECIMAL_INTEGER", "UNSIGNED_HEX_INTEGER", "UNSIGNED_OCTAL_INTEGER",
"STRING_LITERAL1", "STRING_LITERAL2", "ESCAPED_SYMBOLIC_NAME", "ACCESS",
"ACTIVE", "ADMIN", "ADMINISTRATOR", "ALIAS", "ALIASES", "ALL_SHORTEST_PATHS",
"ALL", "ALTER", "AND", "ANY", "ARRAY", "AS", "ASC", "ASCENDING", "ASSIGN",
"AT", "AUTH", "BAR", "BINDINGS", "BOOL", "BOOLEAN", "BOOSTED", "BOTH",
"BREAK", "BTREE", "BUILT", "BY", "CALL", "CASCADE", "CASE", "CHANGE",
"CIDR", "COLLECT", "COLON", "COLONCOLON", "COMMA", "COMMAND", "COMMANDS",
"COMPOSITE", "CONCURRENT", "CONSTRAINT", "CONSTRAINTS", "CONTAINS", "COPY",
"CONTINUE", "COUNT", "CREATE", "CSV", "CURRENT", "DATA", "DATABASE",
"DATABASES", "DATE", "DATETIME", "DBMS", "DEALLOCATE", "DEFAULT", "DEFINED",
"DELETE", "DENY", "DESC", "DESCENDING", "DESTROY", "DETACH", "DIFFERENT",
"DOLLAR", "DISTINCT", "DIVIDE", "DOT", "DOTDOT", "DOUBLEBAR", "DRIVER",
"DROP", "DRYRUN", "DUMP", "DURATION", "EACH", "EDGE", "ENABLE", "ELEMENT",
"ELEMENTS", "ELSE", "ENCRYPTED", "END", "ENDS", "EQ", "EXECUTABLE", "EXECUTE",
"EXIST", "EXISTENCE", "EXISTS", "ERROR", "FAIL", "FALSE", "FIELDTERMINATOR",
"FINISH", "FLOAT", "FOR", "FOREACH", "FROM", "FULLTEXT", "FUNCTION",
"FUNCTIONS", "GE", "GRANT", "GRAPH", "GRAPHS", "GROUP", "GROUPS", "GT",
"HEADERS", "HOME", "ID", "IF", "IMPERSONATE", "IMMUTABLE", "IN", "INDEX",
"INDEXES", "INF", "INFINITY", "INSERT", "INT", "INTEGER", "IS", "JOIN",
"KEY", "LABEL", "LABELS", "AMPERSAND", "EXCLAMATION_MARK", "LBRACKET",
"LCURLY", "LE", "LEADING", "LIMITROWS", "LIST", "LOAD", "LOCAL", "LOOKUP",
"LPAREN", "LT", "MANAGEMENT", "MAP", "MATCH", "MERGE", "MINUS", "PERCENT",
"INVALID_NEQ", "NEQ", "NAME", "NAMES", "NAN", "NFC", "NFD", "NFKC", "NFKD",
"NEW", "NODE", "NODETACH", "NODES", "NONE", "NORMALIZE", "NORMALIZED",
"NOT", "NOTHING", "NOWAIT", "NULL", "OF", "OFFSET", "ON", "ONLY", "OPTIONAL",
"OPTIONS", "OPTION", "OR", "ORDER", "PASSWORD", "PASSWORDS", "PATH",
"PATHS", "PLAINTEXT", "PLUS", "PLUSEQUAL", "POINT", "POPULATED", "POW",
"PRIMARY", "PRIMARIES", "PRIVILEGE", "PRIVILEGES", "PROCEDURE", "PROCEDURES",
"PROPERTIES", "PROPERTY", "PROVIDER", "PROVIDERS", "QUESTION", "RANGE",
"RBRACKET", "RCURLY", "READ", "REALLOCATE", "REDUCE", "RENAME", "REGEQ",
"REL", "RELATIONSHIP", "RELATIONSHIPS", "REMOVE", "REPEATABLE", "REPLACE",
"REPORT", "REQUIRE", "REQUIRED", "RESTRICT", "RETURN", "REVOKE", "ROLE",
"ROLES", "ROW", "ROWS", "RPAREN", "SCAN", "SEC", "SECOND", "SECONDARY",
"SECONDARIES", "SECONDS", "SEEK", "SEMICOLON", "SERVER", "SERVERS", "SET",
"SETTING", "SETTINGS", "SHORTEST_PATH", "SHORTEST", "SHOW", "SIGNED",
"SINGLE", "SKIPROWS", "START", "STARTS", "STATUS", "STOP", "STRING",
"SUPPORTED", "SUSPENDED", "TARGET", "TERMINATE", "TEXT", "THEN", "TIME",
"TIMES", "TIMESTAMP", "TIMEZONE", "TO", "TOPOLOGY", "TRAILING", "TRANSACTION",
"TRANSACTIONS", "TRAVERSE", "TRIM", "TRUE", "TYPE", "TYPED", "TYPES",
"UNION", "UNIQUE", "UNIQUENESS", "UNWIND", "URL", "USE", "USER", "USERS",
"USING", "VALUE", "VARCHAR", "VECTOR", "VERTEX", "WAIT", "WHEN", "WHERE",
"WITH", "WITHOUT", "WRITE", "XOR", "YIELD", "ZONE", "ZONED", "IDENTIFIER",
"EXTENDED_IDENTIFIER", "ARROW_LINE", "ARROW_LEFT_HEAD", "ARROW_RIGHT_HEAD",
"ErrorChar"
};
}
private static final String[] _SYMBOLIC_NAMES = makeSymbolicNames();
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "Cypher6Parser.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
public Cypher6Parser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
@SuppressWarnings("CheckReturnValue")
public static class StatementsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List statement() {
return getRuleContexts(StatementContext.class);
}
public StatementContext statement(int i) {
return getRuleContext(StatementContext.class,i);
}
public TerminalNode EOF() { return getToken(Cypher6Parser.EOF, 0); }
public List SEMICOLON() { return getTokens(Cypher6Parser.SEMICOLON); }
public TerminalNode SEMICOLON(int i) {
return getToken(Cypher6Parser.SEMICOLON, i);
}
public StatementsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_statements; }
}
public final StatementsContext statements() throws RecognitionException {
StatementsContext _localctx = new StatementsContext(_ctx, getState());
enterRule(_localctx, 0, RULE_statements);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(652);
statement();
setState(657);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,0,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(653);
match(SEMICOLON);
setState(654);
statement();
}
}
}
setState(659);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,0,_ctx);
}
setState(661);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==SEMICOLON) {
{
setState(660);
match(SEMICOLON);
}
}
setState(663);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StatementContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public CommandContext command() {
return getRuleContext(CommandContext.class,0);
}
public RegularQueryContext regularQuery() {
return getRuleContext(RegularQueryContext.class,0);
}
public StatementContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_statement; }
}
public final StatementContext statement() throws RecognitionException {
StatementContext _localctx = new StatementContext(_ctx, getState());
enterRule(_localctx, 2, RULE_statement);
try {
setState(667);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,2,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(665);
command();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(666);
regularQuery();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RegularQueryContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List singleQuery() {
return getRuleContexts(SingleQueryContext.class);
}
public SingleQueryContext singleQuery(int i) {
return getRuleContext(SingleQueryContext.class,i);
}
public List UNION() { return getTokens(Cypher6Parser.UNION); }
public TerminalNode UNION(int i) {
return getToken(Cypher6Parser.UNION, i);
}
public List ALL() { return getTokens(Cypher6Parser.ALL); }
public TerminalNode ALL(int i) {
return getToken(Cypher6Parser.ALL, i);
}
public List DISTINCT() { return getTokens(Cypher6Parser.DISTINCT); }
public TerminalNode DISTINCT(int i) {
return getToken(Cypher6Parser.DISTINCT, i);
}
public RegularQueryContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_regularQuery; }
}
public final RegularQueryContext regularQuery() throws RecognitionException {
RegularQueryContext _localctx = new RegularQueryContext(_ctx, getState());
enterRule(_localctx, 4, RULE_regularQuery);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(669);
singleQuery();
setState(677);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==UNION) {
{
{
setState(670);
match(UNION);
setState(672);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==ALL || _la==DISTINCT) {
{
setState(671);
_la = _input.LA(1);
if ( !(_la==ALL || _la==DISTINCT) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(674);
singleQuery();
}
}
setState(679);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SingleQueryContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List clause() {
return getRuleContexts(ClauseContext.class);
}
public ClauseContext clause(int i) {
return getRuleContext(ClauseContext.class,i);
}
public SingleQueryContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_singleQuery; }
}
public final SingleQueryContext singleQuery() throws RecognitionException {
SingleQueryContext _localctx = new SingleQueryContext(_ctx, getState());
enterRule(_localctx, 6, RULE_singleQuery);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(681);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(680);
clause();
}
}
setState(683);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( ((((_la - 39)) & ~0x3f) == 0 && ((1L << (_la - 39)) & 70867484673L) != 0) || ((((_la - 107)) & ~0x3f) == 0 && ((1L << (_la - 107)) & 1694347485511689L) != 0) || ((((_la - 171)) & ~0x3f) == 0 && ((1L << (_la - 171)) & 145241087982838785L) != 0) || ((((_la - 245)) & ~0x3f) == 0 && ((1L << (_la - 245)) & 2253174203220225L) != 0) );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public UseClauseContext useClause() {
return getRuleContext(UseClauseContext.class,0);
}
public FinishClauseContext finishClause() {
return getRuleContext(FinishClauseContext.class,0);
}
public ReturnClauseContext returnClause() {
return getRuleContext(ReturnClauseContext.class,0);
}
public CreateClauseContext createClause() {
return getRuleContext(CreateClauseContext.class,0);
}
public InsertClauseContext insertClause() {
return getRuleContext(InsertClauseContext.class,0);
}
public DeleteClauseContext deleteClause() {
return getRuleContext(DeleteClauseContext.class,0);
}
public SetClauseContext setClause() {
return getRuleContext(SetClauseContext.class,0);
}
public RemoveClauseContext removeClause() {
return getRuleContext(RemoveClauseContext.class,0);
}
public MatchClauseContext matchClause() {
return getRuleContext(MatchClauseContext.class,0);
}
public MergeClauseContext mergeClause() {
return getRuleContext(MergeClauseContext.class,0);
}
public WithClauseContext withClause() {
return getRuleContext(WithClauseContext.class,0);
}
public UnwindClauseContext unwindClause() {
return getRuleContext(UnwindClauseContext.class,0);
}
public CallClauseContext callClause() {
return getRuleContext(CallClauseContext.class,0);
}
public SubqueryClauseContext subqueryClause() {
return getRuleContext(SubqueryClauseContext.class,0);
}
public LoadCSVClauseContext loadCSVClause() {
return getRuleContext(LoadCSVClauseContext.class,0);
}
public ForeachClauseContext foreachClause() {
return getRuleContext(ForeachClauseContext.class,0);
}
public OrderBySkipLimitClauseContext orderBySkipLimitClause() {
return getRuleContext(OrderBySkipLimitClauseContext.class,0);
}
public ClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_clause; }
}
public final ClauseContext clause() throws RecognitionException {
ClauseContext _localctx = new ClauseContext(_ctx, getState());
enterRule(_localctx, 8, RULE_clause);
try {
setState(702);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,6,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(685);
useClause();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(686);
finishClause();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(687);
returnClause();
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(688);
createClause();
}
break;
case 5:
enterOuterAlt(_localctx, 5);
{
setState(689);
insertClause();
}
break;
case 6:
enterOuterAlt(_localctx, 6);
{
setState(690);
deleteClause();
}
break;
case 7:
enterOuterAlt(_localctx, 7);
{
setState(691);
setClause();
}
break;
case 8:
enterOuterAlt(_localctx, 8);
{
setState(692);
removeClause();
}
break;
case 9:
enterOuterAlt(_localctx, 9);
{
setState(693);
matchClause();
}
break;
case 10:
enterOuterAlt(_localctx, 10);
{
setState(694);
mergeClause();
}
break;
case 11:
enterOuterAlt(_localctx, 11);
{
setState(695);
withClause();
}
break;
case 12:
enterOuterAlt(_localctx, 12);
{
setState(696);
unwindClause();
}
break;
case 13:
enterOuterAlt(_localctx, 13);
{
setState(697);
callClause();
}
break;
case 14:
enterOuterAlt(_localctx, 14);
{
setState(698);
subqueryClause();
}
break;
case 15:
enterOuterAlt(_localctx, 15);
{
setState(699);
loadCSVClause();
}
break;
case 16:
enterOuterAlt(_localctx, 16);
{
setState(700);
foreachClause();
}
break;
case 17:
enterOuterAlt(_localctx, 17);
{
setState(701);
orderBySkipLimitClause();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UseClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USE() { return getToken(Cypher6Parser.USE, 0); }
public GraphReferenceContext graphReference() {
return getRuleContext(GraphReferenceContext.class,0);
}
public TerminalNode GRAPH() { return getToken(Cypher6Parser.GRAPH, 0); }
public UseClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_useClause; }
}
public final UseClauseContext useClause() throws RecognitionException {
UseClauseContext _localctx = new UseClauseContext(_ctx, getState());
enterRule(_localctx, 10, RULE_useClause);
try {
enterOuterAlt(_localctx, 1);
{
setState(704);
match(USE);
setState(706);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,7,_ctx) ) {
case 1:
{
setState(705);
match(GRAPH);
}
break;
}
setState(708);
graphReference();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GraphReferenceContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public GraphReferenceContext graphReference() {
return getRuleContext(GraphReferenceContext.class,0);
}
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public FunctionInvocationContext functionInvocation() {
return getRuleContext(FunctionInvocationContext.class,0);
}
public SymbolicAliasNameContext symbolicAliasName() {
return getRuleContext(SymbolicAliasNameContext.class,0);
}
public GraphReferenceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_graphReference; }
}
public final GraphReferenceContext graphReference() throws RecognitionException {
GraphReferenceContext _localctx = new GraphReferenceContext(_ctx, getState());
enterRule(_localctx, 12, RULE_graphReference);
try {
setState(716);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,8,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(710);
match(LPAREN);
setState(711);
graphReference();
setState(712);
match(RPAREN);
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(714);
functionInvocation();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(715);
symbolicAliasName();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FinishClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode FINISH() { return getToken(Cypher6Parser.FINISH, 0); }
public FinishClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_finishClause; }
}
public final FinishClauseContext finishClause() throws RecognitionException {
FinishClauseContext _localctx = new FinishClauseContext(_ctx, getState());
enterRule(_localctx, 14, RULE_finishClause);
try {
enterOuterAlt(_localctx, 1);
{
setState(718);
match(FINISH);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ReturnClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode RETURN() { return getToken(Cypher6Parser.RETURN, 0); }
public ReturnBodyContext returnBody() {
return getRuleContext(ReturnBodyContext.class,0);
}
public ReturnClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_returnClause; }
}
public final ReturnClauseContext returnClause() throws RecognitionException {
ReturnClauseContext _localctx = new ReturnClauseContext(_ctx, getState());
enterRule(_localctx, 16, RULE_returnClause);
try {
enterOuterAlt(_localctx, 1);
{
setState(720);
match(RETURN);
setState(721);
returnBody();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ReturnBodyContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ReturnItemsContext returnItems() {
return getRuleContext(ReturnItemsContext.class,0);
}
public TerminalNode DISTINCT() { return getToken(Cypher6Parser.DISTINCT, 0); }
public OrderByContext orderBy() {
return getRuleContext(OrderByContext.class,0);
}
public SkipContext skip() {
return getRuleContext(SkipContext.class,0);
}
public LimitContext limit() {
return getRuleContext(LimitContext.class,0);
}
public ReturnBodyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_returnBody; }
}
public final ReturnBodyContext returnBody() throws RecognitionException {
ReturnBodyContext _localctx = new ReturnBodyContext(_ctx, getState());
enterRule(_localctx, 18, RULE_returnBody);
try {
enterOuterAlt(_localctx, 1);
{
setState(724);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,9,_ctx) ) {
case 1:
{
setState(723);
match(DISTINCT);
}
break;
}
setState(726);
returnItems();
setState(728);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,10,_ctx) ) {
case 1:
{
setState(727);
orderBy();
}
break;
}
setState(731);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,11,_ctx) ) {
case 1:
{
setState(730);
skip();
}
break;
}
setState(734);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,12,_ctx) ) {
case 1:
{
setState(733);
limit();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ReturnItemContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode AS() { return getToken(Cypher6Parser.AS, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public ReturnItemContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_returnItem; }
}
public final ReturnItemContext returnItem() throws RecognitionException {
ReturnItemContext _localctx = new ReturnItemContext(_ctx, getState());
enterRule(_localctx, 20, RULE_returnItem);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(736);
expression();
setState(739);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AS) {
{
setState(737);
match(AS);
setState(738);
variable();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ReturnItemsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public List returnItem() {
return getRuleContexts(ReturnItemContext.class);
}
public ReturnItemContext returnItem(int i) {
return getRuleContext(ReturnItemContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public ReturnItemsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_returnItems; }
}
public final ReturnItemsContext returnItems() throws RecognitionException {
ReturnItemsContext _localctx = new ReturnItemsContext(_ctx, getState());
enterRule(_localctx, 22, RULE_returnItems);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(743);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(741);
match(TIMES);
}
break;
case DECIMAL_DOUBLE:
case UNSIGNED_DECIMAL_INTEGER:
case UNSIGNED_HEX_INTEGER:
case UNSIGNED_OCTAL_INTEGER:
case STRING_LITERAL1:
case STRING_LITERAL2:
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LBRACKET:
case LCURLY:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case LPAREN:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case MINUS:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case PLUS:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
{
setState(742);
returnItem();
}
break;
default:
throw new NoViableAltException(this);
}
setState(749);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(745);
match(COMMA);
setState(746);
returnItem();
}
}
setState(751);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class OrderItemContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public AscTokenContext ascToken() {
return getRuleContext(AscTokenContext.class,0);
}
public DescTokenContext descToken() {
return getRuleContext(DescTokenContext.class,0);
}
public OrderItemContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_orderItem; }
}
public final OrderItemContext orderItem() throws RecognitionException {
OrderItemContext _localctx = new OrderItemContext(_ctx, getState());
enterRule(_localctx, 24, RULE_orderItem);
try {
enterOuterAlt(_localctx, 1);
{
setState(752);
expression();
setState(755);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ASC:
case ASCENDING:
{
setState(753);
ascToken();
}
break;
case DESC:
case DESCENDING:
{
setState(754);
descToken();
}
break;
case EOF:
case CALL:
case COMMA:
case CREATE:
case DELETE:
case DETACH:
case FINISH:
case FOREACH:
case INSERT:
case LIMITROWS:
case LOAD:
case MATCH:
case MERGE:
case NODETACH:
case OFFSET:
case OPTIONAL:
case ORDER:
case RCURLY:
case REMOVE:
case RETURN:
case RPAREN:
case SEMICOLON:
case SET:
case SHOW:
case SKIPROWS:
case TERMINATE:
case UNION:
case UNWIND:
case USE:
case WHERE:
case WITH:
break;
default:
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AscTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ASC() { return getToken(Cypher6Parser.ASC, 0); }
public TerminalNode ASCENDING() { return getToken(Cypher6Parser.ASCENDING, 0); }
public AscTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_ascToken; }
}
public final AscTokenContext ascToken() throws RecognitionException {
AscTokenContext _localctx = new AscTokenContext(_ctx, getState());
enterRule(_localctx, 26, RULE_ascToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(757);
_la = _input.LA(1);
if ( !(_la==ASC || _la==ASCENDING) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DescTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DESC() { return getToken(Cypher6Parser.DESC, 0); }
public TerminalNode DESCENDING() { return getToken(Cypher6Parser.DESCENDING, 0); }
public DescTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_descToken; }
}
public final DescTokenContext descToken() throws RecognitionException {
DescTokenContext _localctx = new DescTokenContext(_ctx, getState());
enterRule(_localctx, 28, RULE_descToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(759);
_la = _input.LA(1);
if ( !(_la==DESC || _la==DESCENDING) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class OrderByContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ORDER() { return getToken(Cypher6Parser.ORDER, 0); }
public TerminalNode BY() { return getToken(Cypher6Parser.BY, 0); }
public List orderItem() {
return getRuleContexts(OrderItemContext.class);
}
public OrderItemContext orderItem(int i) {
return getRuleContext(OrderItemContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public OrderByContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_orderBy; }
}
public final OrderByContext orderBy() throws RecognitionException {
OrderByContext _localctx = new OrderByContext(_ctx, getState());
enterRule(_localctx, 30, RULE_orderBy);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(761);
match(ORDER);
setState(762);
match(BY);
setState(763);
orderItem();
setState(768);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(764);
match(COMMA);
setState(765);
orderItem();
}
}
setState(770);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SkipContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode OFFSET() { return getToken(Cypher6Parser.OFFSET, 0); }
public TerminalNode SKIPROWS() { return getToken(Cypher6Parser.SKIPROWS, 0); }
public SkipContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_skip; }
}
public final SkipContext skip() throws RecognitionException {
SkipContext _localctx = new SkipContext(_ctx, getState());
enterRule(_localctx, 32, RULE_skip);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(771);
_la = _input.LA(1);
if ( !(_la==OFFSET || _la==SKIPROWS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(772);
expression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LimitContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LIMITROWS() { return getToken(Cypher6Parser.LIMITROWS, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public LimitContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_limit; }
}
public final LimitContext limit() throws RecognitionException {
LimitContext _localctx = new LimitContext(_ctx, getState());
enterRule(_localctx, 34, RULE_limit);
try {
enterOuterAlt(_localctx, 1);
{
setState(774);
match(LIMITROWS);
setState(775);
expression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class WhereClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode WHERE() { return getToken(Cypher6Parser.WHERE, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public WhereClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_whereClause; }
}
public final WhereClauseContext whereClause() throws RecognitionException {
WhereClauseContext _localctx = new WhereClauseContext(_ctx, getState());
enterRule(_localctx, 36, RULE_whereClause);
try {
enterOuterAlt(_localctx, 1);
{
setState(777);
match(WHERE);
setState(778);
expression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class WithClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode WITH() { return getToken(Cypher6Parser.WITH, 0); }
public ReturnBodyContext returnBody() {
return getRuleContext(ReturnBodyContext.class,0);
}
public WhereClauseContext whereClause() {
return getRuleContext(WhereClauseContext.class,0);
}
public WithClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_withClause; }
}
public final WithClauseContext withClause() throws RecognitionException {
WithClauseContext _localctx = new WithClauseContext(_ctx, getState());
enterRule(_localctx, 38, RULE_withClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(780);
match(WITH);
setState(781);
returnBody();
setState(783);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE) {
{
setState(782);
whereClause();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CREATE() { return getToken(Cypher6Parser.CREATE, 0); }
public PatternListContext patternList() {
return getRuleContext(PatternListContext.class,0);
}
public CreateClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createClause; }
}
public final CreateClauseContext createClause() throws RecognitionException {
CreateClauseContext _localctx = new CreateClauseContext(_ctx, getState());
enterRule(_localctx, 40, RULE_createClause);
try {
enterOuterAlt(_localctx, 1);
{
setState(785);
match(CREATE);
setState(786);
patternList();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InsertClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode INSERT() { return getToken(Cypher6Parser.INSERT, 0); }
public InsertPatternListContext insertPatternList() {
return getRuleContext(InsertPatternListContext.class,0);
}
public InsertClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_insertClause; }
}
public final InsertClauseContext insertClause() throws RecognitionException {
InsertClauseContext _localctx = new InsertClauseContext(_ctx, getState());
enterRule(_localctx, 42, RULE_insertClause);
try {
enterOuterAlt(_localctx, 1);
{
setState(788);
match(INSERT);
setState(789);
insertPatternList();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SetClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SET() { return getToken(Cypher6Parser.SET, 0); }
public List setItem() {
return getRuleContexts(SetItemContext.class);
}
public SetItemContext setItem(int i) {
return getRuleContext(SetItemContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public SetClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_setClause; }
}
public final SetClauseContext setClause() throws RecognitionException {
SetClauseContext _localctx = new SetClauseContext(_ctx, getState());
enterRule(_localctx, 44, RULE_setClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(791);
match(SET);
setState(792);
setItem();
setState(797);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(793);
match(COMMA);
setState(794);
setItem();
}
}
setState(799);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SetItemContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SetItemContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_setItem; }
public SetItemContext() { }
public void copyFrom(SetItemContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class SetPropContext extends SetItemContext {
public PropertyExpressionContext propertyExpression() {
return getRuleContext(PropertyExpressionContext.class,0);
}
public TerminalNode EQ() { return getToken(Cypher6Parser.EQ, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public SetPropContext(SetItemContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class AddPropContext extends SetItemContext {
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public TerminalNode PLUSEQUAL() { return getToken(Cypher6Parser.PLUSEQUAL, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public AddPropContext(SetItemContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class SetDynamicPropContext extends SetItemContext {
public DynamicPropertyExpressionContext dynamicPropertyExpression() {
return getRuleContext(DynamicPropertyExpressionContext.class,0);
}
public TerminalNode EQ() { return getToken(Cypher6Parser.EQ, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public SetDynamicPropContext(SetItemContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class SetPropsContext extends SetItemContext {
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public TerminalNode EQ() { return getToken(Cypher6Parser.EQ, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public SetPropsContext(SetItemContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class SetLabelsContext extends SetItemContext {
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public NodeLabelsContext nodeLabels() {
return getRuleContext(NodeLabelsContext.class,0);
}
public SetLabelsContext(SetItemContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class SetLabelsIsContext extends SetItemContext {
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public NodeLabelsIsContext nodeLabelsIs() {
return getRuleContext(NodeLabelsIsContext.class,0);
}
public SetLabelsIsContext(SetItemContext ctx) { copyFrom(ctx); }
}
public final SetItemContext setItem() throws RecognitionException {
SetItemContext _localctx = new SetItemContext(_ctx, getState());
enterRule(_localctx, 46, RULE_setItem);
try {
setState(822);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,20,_ctx) ) {
case 1:
_localctx = new SetPropContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(800);
propertyExpression();
setState(801);
match(EQ);
setState(802);
expression();
}
break;
case 2:
_localctx = new SetDynamicPropContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(804);
dynamicPropertyExpression();
setState(805);
match(EQ);
setState(806);
expression();
}
break;
case 3:
_localctx = new SetPropsContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(808);
variable();
setState(809);
match(EQ);
setState(810);
expression();
}
break;
case 4:
_localctx = new AddPropContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(812);
variable();
setState(813);
match(PLUSEQUAL);
setState(814);
expression();
}
break;
case 5:
_localctx = new SetLabelsContext(_localctx);
enterOuterAlt(_localctx, 5);
{
setState(816);
variable();
setState(817);
nodeLabels();
}
break;
case 6:
_localctx = new SetLabelsIsContext(_localctx);
enterOuterAlt(_localctx, 6);
{
setState(819);
variable();
setState(820);
nodeLabelsIs();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RemoveClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode REMOVE() { return getToken(Cypher6Parser.REMOVE, 0); }
public List removeItem() {
return getRuleContexts(RemoveItemContext.class);
}
public RemoveItemContext removeItem(int i) {
return getRuleContext(RemoveItemContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public RemoveClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_removeClause; }
}
public final RemoveClauseContext removeClause() throws RecognitionException {
RemoveClauseContext _localctx = new RemoveClauseContext(_ctx, getState());
enterRule(_localctx, 48, RULE_removeClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(824);
match(REMOVE);
setState(825);
removeItem();
setState(830);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(826);
match(COMMA);
setState(827);
removeItem();
}
}
setState(832);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RemoveItemContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public RemoveItemContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_removeItem; }
public RemoveItemContext() { }
public void copyFrom(RemoveItemContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class RemoveLabelsIsContext extends RemoveItemContext {
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public NodeLabelsIsContext nodeLabelsIs() {
return getRuleContext(NodeLabelsIsContext.class,0);
}
public RemoveLabelsIsContext(RemoveItemContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class RemoveDynamicPropContext extends RemoveItemContext {
public DynamicPropertyExpressionContext dynamicPropertyExpression() {
return getRuleContext(DynamicPropertyExpressionContext.class,0);
}
public RemoveDynamicPropContext(RemoveItemContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class RemoveLabelsContext extends RemoveItemContext {
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public NodeLabelsContext nodeLabels() {
return getRuleContext(NodeLabelsContext.class,0);
}
public RemoveLabelsContext(RemoveItemContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class RemovePropContext extends RemoveItemContext {
public PropertyExpressionContext propertyExpression() {
return getRuleContext(PropertyExpressionContext.class,0);
}
public RemovePropContext(RemoveItemContext ctx) { copyFrom(ctx); }
}
public final RemoveItemContext removeItem() throws RecognitionException {
RemoveItemContext _localctx = new RemoveItemContext(_ctx, getState());
enterRule(_localctx, 50, RULE_removeItem);
try {
setState(841);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,22,_ctx) ) {
case 1:
_localctx = new RemovePropContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(833);
propertyExpression();
}
break;
case 2:
_localctx = new RemoveDynamicPropContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(834);
dynamicPropertyExpression();
}
break;
case 3:
_localctx = new RemoveLabelsContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(835);
variable();
setState(836);
nodeLabels();
}
break;
case 4:
_localctx = new RemoveLabelsIsContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(838);
variable();
setState(839);
nodeLabelsIs();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DeleteClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DELETE() { return getToken(Cypher6Parser.DELETE, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public TerminalNode DETACH() { return getToken(Cypher6Parser.DETACH, 0); }
public TerminalNode NODETACH() { return getToken(Cypher6Parser.NODETACH, 0); }
public DeleteClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_deleteClause; }
}
public final DeleteClauseContext deleteClause() throws RecognitionException {
DeleteClauseContext _localctx = new DeleteClauseContext(_ctx, getState());
enterRule(_localctx, 52, RULE_deleteClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(844);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DETACH || _la==NODETACH) {
{
setState(843);
_la = _input.LA(1);
if ( !(_la==DETACH || _la==NODETACH) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(846);
match(DELETE);
setState(847);
expression();
setState(852);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(848);
match(COMMA);
setState(849);
expression();
}
}
setState(854);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MatchClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode MATCH() { return getToken(Cypher6Parser.MATCH, 0); }
public PatternListContext patternList() {
return getRuleContext(PatternListContext.class,0);
}
public TerminalNode OPTIONAL() { return getToken(Cypher6Parser.OPTIONAL, 0); }
public MatchModeContext matchMode() {
return getRuleContext(MatchModeContext.class,0);
}
public List hint() {
return getRuleContexts(HintContext.class);
}
public HintContext hint(int i) {
return getRuleContext(HintContext.class,i);
}
public WhereClauseContext whereClause() {
return getRuleContext(WhereClauseContext.class,0);
}
public MatchClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_matchClause; }
}
public final MatchClauseContext matchClause() throws RecognitionException {
MatchClauseContext _localctx = new MatchClauseContext(_ctx, getState());
enterRule(_localctx, 54, RULE_matchClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(856);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==OPTIONAL) {
{
setState(855);
match(OPTIONAL);
}
}
setState(858);
match(MATCH);
setState(860);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,26,_ctx) ) {
case 1:
{
setState(859);
matchMode();
}
break;
}
setState(862);
patternList();
setState(866);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==USING) {
{
{
setState(863);
hint();
}
}
setState(868);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(870);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE) {
{
setState(869);
whereClause();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MatchModeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode REPEATABLE() { return getToken(Cypher6Parser.REPEATABLE, 0); }
public TerminalNode ELEMENT() { return getToken(Cypher6Parser.ELEMENT, 0); }
public TerminalNode ELEMENTS() { return getToken(Cypher6Parser.ELEMENTS, 0); }
public TerminalNode BINDINGS() { return getToken(Cypher6Parser.BINDINGS, 0); }
public TerminalNode DIFFERENT() { return getToken(Cypher6Parser.DIFFERENT, 0); }
public TerminalNode RELATIONSHIP() { return getToken(Cypher6Parser.RELATIONSHIP, 0); }
public TerminalNode RELATIONSHIPS() { return getToken(Cypher6Parser.RELATIONSHIPS, 0); }
public MatchModeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_matchMode; }
}
public final MatchModeContext matchMode() throws RecognitionException {
MatchModeContext _localctx = new MatchModeContext(_ctx, getState());
enterRule(_localctx, 56, RULE_matchMode);
try {
setState(888);
_errHandler.sync(this);
switch (_input.LA(1)) {
case REPEATABLE:
enterOuterAlt(_localctx, 1);
{
setState(872);
match(REPEATABLE);
setState(878);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ELEMENT:
{
setState(873);
match(ELEMENT);
setState(875);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,29,_ctx) ) {
case 1:
{
setState(874);
match(BINDINGS);
}
break;
}
}
break;
case ELEMENTS:
{
setState(877);
match(ELEMENTS);
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
case DIFFERENT:
enterOuterAlt(_localctx, 2);
{
setState(880);
match(DIFFERENT);
setState(886);
_errHandler.sync(this);
switch (_input.LA(1)) {
case RELATIONSHIP:
{
setState(881);
match(RELATIONSHIP);
setState(883);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,31,_ctx) ) {
case 1:
{
setState(882);
match(BINDINGS);
}
break;
}
}
break;
case RELATIONSHIPS:
{
setState(885);
match(RELATIONSHIPS);
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class HintContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USING() { return getToken(Cypher6Parser.USING, 0); }
public TerminalNode JOIN() { return getToken(Cypher6Parser.JOIN, 0); }
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public NonEmptyNameListContext nonEmptyNameList() {
return getRuleContext(NonEmptyNameListContext.class,0);
}
public TerminalNode SCAN() { return getToken(Cypher6Parser.SCAN, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public LabelOrRelTypeContext labelOrRelType() {
return getRuleContext(LabelOrRelTypeContext.class,0);
}
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public TerminalNode INDEX() { return getToken(Cypher6Parser.INDEX, 0); }
public TerminalNode BTREE() { return getToken(Cypher6Parser.BTREE, 0); }
public TerminalNode TEXT() { return getToken(Cypher6Parser.TEXT, 0); }
public TerminalNode RANGE() { return getToken(Cypher6Parser.RANGE, 0); }
public TerminalNode POINT() { return getToken(Cypher6Parser.POINT, 0); }
public TerminalNode SEEK() { return getToken(Cypher6Parser.SEEK, 0); }
public HintContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_hint; }
}
public final HintContext hint() throws RecognitionException {
HintContext _localctx = new HintContext(_ctx, getState());
enterRule(_localctx, 58, RULE_hint);
try {
enterOuterAlt(_localctx, 1);
{
setState(890);
match(USING);
setState(918);
_errHandler.sync(this);
switch (_input.LA(1)) {
case BTREE:
case INDEX:
case POINT:
case RANGE:
case TEXT:
{
{
setState(900);
_errHandler.sync(this);
switch (_input.LA(1)) {
case INDEX:
{
setState(891);
match(INDEX);
}
break;
case BTREE:
{
setState(892);
match(BTREE);
setState(893);
match(INDEX);
}
break;
case TEXT:
{
setState(894);
match(TEXT);
setState(895);
match(INDEX);
}
break;
case RANGE:
{
setState(896);
match(RANGE);
setState(897);
match(INDEX);
}
break;
case POINT:
{
setState(898);
match(POINT);
setState(899);
match(INDEX);
}
break;
default:
throw new NoViableAltException(this);
}
setState(903);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,35,_ctx) ) {
case 1:
{
setState(902);
match(SEEK);
}
break;
}
setState(905);
variable();
setState(906);
labelOrRelType();
setState(907);
match(LPAREN);
setState(908);
nonEmptyNameList();
setState(909);
match(RPAREN);
}
}
break;
case JOIN:
{
setState(911);
match(JOIN);
setState(912);
match(ON);
setState(913);
nonEmptyNameList();
}
break;
case SCAN:
{
setState(914);
match(SCAN);
setState(915);
variable();
setState(916);
labelOrRelType();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MergeClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode MERGE() { return getToken(Cypher6Parser.MERGE, 0); }
public PatternContext pattern() {
return getRuleContext(PatternContext.class,0);
}
public List mergeAction() {
return getRuleContexts(MergeActionContext.class);
}
public MergeActionContext mergeAction(int i) {
return getRuleContext(MergeActionContext.class,i);
}
public MergeClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_mergeClause; }
}
public final MergeClauseContext mergeClause() throws RecognitionException {
MergeClauseContext _localctx = new MergeClauseContext(_ctx, getState());
enterRule(_localctx, 60, RULE_mergeClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(920);
match(MERGE);
setState(921);
pattern();
setState(925);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==ON) {
{
{
setState(922);
mergeAction();
}
}
setState(927);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MergeActionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public SetClauseContext setClause() {
return getRuleContext(SetClauseContext.class,0);
}
public TerminalNode MATCH() { return getToken(Cypher6Parser.MATCH, 0); }
public TerminalNode CREATE() { return getToken(Cypher6Parser.CREATE, 0); }
public MergeActionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_mergeAction; }
}
public final MergeActionContext mergeAction() throws RecognitionException {
MergeActionContext _localctx = new MergeActionContext(_ctx, getState());
enterRule(_localctx, 62, RULE_mergeAction);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(928);
match(ON);
setState(929);
_la = _input.LA(1);
if ( !(_la==CREATE || _la==MATCH) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(930);
setClause();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UnwindClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode UNWIND() { return getToken(Cypher6Parser.UNWIND, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode AS() { return getToken(Cypher6Parser.AS, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public UnwindClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_unwindClause; }
}
public final UnwindClauseContext unwindClause() throws RecognitionException {
UnwindClauseContext _localctx = new UnwindClauseContext(_ctx, getState());
enterRule(_localctx, 64, RULE_unwindClause);
try {
enterOuterAlt(_localctx, 1);
{
setState(932);
match(UNWIND);
setState(933);
expression();
setState(934);
match(AS);
setState(935);
variable();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CallClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CALL() { return getToken(Cypher6Parser.CALL, 0); }
public ProcedureNameContext procedureName() {
return getRuleContext(ProcedureNameContext.class,0);
}
public TerminalNode OPTIONAL() { return getToken(Cypher6Parser.OPTIONAL, 0); }
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public TerminalNode YIELD() { return getToken(Cypher6Parser.YIELD, 0); }
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public List procedureResultItem() {
return getRuleContexts(ProcedureResultItemContext.class);
}
public ProcedureResultItemContext procedureResultItem(int i) {
return getRuleContext(ProcedureResultItemContext.class,i);
}
public List procedureArgument() {
return getRuleContexts(ProcedureArgumentContext.class);
}
public ProcedureArgumentContext procedureArgument(int i) {
return getRuleContext(ProcedureArgumentContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public WhereClauseContext whereClause() {
return getRuleContext(WhereClauseContext.class,0);
}
public CallClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_callClause; }
}
public final CallClauseContext callClause() throws RecognitionException {
CallClauseContext _localctx = new CallClauseContext(_ctx, getState());
enterRule(_localctx, 66, RULE_callClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(938);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==OPTIONAL) {
{
setState(937);
match(OPTIONAL);
}
}
setState(940);
match(CALL);
setState(941);
procedureName();
setState(954);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LPAREN) {
{
setState(942);
match(LPAREN);
setState(951);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -246291141492752L) != 0) || ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -146366996479967233L) != 0) || ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -15066095617L) != 0) || ((((_la - 192)) & ~0x3f) == 0 && ((1L << (_la - 192)) & -1130297988612169L) != 0) || ((((_la - 256)) & ~0x3f) == 0 && ((1L << (_la - 256)) & 281474976709631L) != 0)) {
{
setState(943);
procedureArgument();
setState(948);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(944);
match(COMMA);
setState(945);
procedureArgument();
}
}
setState(950);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(953);
match(RPAREN);
}
}
setState(971);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==YIELD) {
{
setState(956);
match(YIELD);
setState(969);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(957);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
{
setState(958);
procedureResultItem();
setState(963);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(959);
match(COMMA);
setState(960);
procedureResultItem();
}
}
setState(965);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(967);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE) {
{
setState(966);
whereClause();
}
}
}
break;
default:
throw new NoViableAltException(this);
}
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ProcedureNameContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public NamespaceContext namespace() {
return getRuleContext(NamespaceContext.class,0);
}
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public ProcedureNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_procedureName; }
}
public final ProcedureNameContext procedureName() throws RecognitionException {
ProcedureNameContext _localctx = new ProcedureNameContext(_ctx, getState());
enterRule(_localctx, 68, RULE_procedureName);
try {
enterOuterAlt(_localctx, 1);
{
setState(973);
namespace();
setState(974);
symbolicNameString();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ProcedureArgumentContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public ProcedureArgumentContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_procedureArgument; }
}
public final ProcedureArgumentContext procedureArgument() throws RecognitionException {
ProcedureArgumentContext _localctx = new ProcedureArgumentContext(_ctx, getState());
enterRule(_localctx, 70, RULE_procedureArgument);
try {
enterOuterAlt(_localctx, 1);
{
setState(976);
expression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ProcedureResultItemContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public TerminalNode AS() { return getToken(Cypher6Parser.AS, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public ProcedureResultItemContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_procedureResultItem; }
}
public final ProcedureResultItemContext procedureResultItem() throws RecognitionException {
ProcedureResultItemContext _localctx = new ProcedureResultItemContext(_ctx, getState());
enterRule(_localctx, 72, RULE_procedureResultItem);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(978);
symbolicNameString();
setState(981);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AS) {
{
setState(979);
match(AS);
setState(980);
variable();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LoadCSVClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LOAD() { return getToken(Cypher6Parser.LOAD, 0); }
public TerminalNode CSV() { return getToken(Cypher6Parser.CSV, 0); }
public TerminalNode FROM() { return getToken(Cypher6Parser.FROM, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode AS() { return getToken(Cypher6Parser.AS, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public TerminalNode WITH() { return getToken(Cypher6Parser.WITH, 0); }
public TerminalNode HEADERS() { return getToken(Cypher6Parser.HEADERS, 0); }
public TerminalNode FIELDTERMINATOR() { return getToken(Cypher6Parser.FIELDTERMINATOR, 0); }
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public LoadCSVClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_loadCSVClause; }
}
public final LoadCSVClauseContext loadCSVClause() throws RecognitionException {
LoadCSVClauseContext _localctx = new LoadCSVClauseContext(_ctx, getState());
enterRule(_localctx, 74, RULE_loadCSVClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(983);
match(LOAD);
setState(984);
match(CSV);
setState(987);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WITH) {
{
setState(985);
match(WITH);
setState(986);
match(HEADERS);
}
}
setState(989);
match(FROM);
setState(990);
expression();
setState(991);
match(AS);
setState(992);
variable();
setState(995);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==FIELDTERMINATOR) {
{
setState(993);
match(FIELDTERMINATOR);
setState(994);
stringLiteral();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ForeachClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode FOREACH() { return getToken(Cypher6Parser.FOREACH, 0); }
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public TerminalNode IN() { return getToken(Cypher6Parser.IN, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode BAR() { return getToken(Cypher6Parser.BAR, 0); }
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public List clause() {
return getRuleContexts(ClauseContext.class);
}
public ClauseContext clause(int i) {
return getRuleContext(ClauseContext.class,i);
}
public ForeachClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_foreachClause; }
}
public final ForeachClauseContext foreachClause() throws RecognitionException {
ForeachClauseContext _localctx = new ForeachClauseContext(_ctx, getState());
enterRule(_localctx, 76, RULE_foreachClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(997);
match(FOREACH);
setState(998);
match(LPAREN);
setState(999);
variable();
setState(1000);
match(IN);
setState(1001);
expression();
setState(1002);
match(BAR);
setState(1004);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(1003);
clause();
}
}
setState(1006);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( ((((_la - 39)) & ~0x3f) == 0 && ((1L << (_la - 39)) & 70867484673L) != 0) || ((((_la - 107)) & ~0x3f) == 0 && ((1L << (_la - 107)) & 1694347485511689L) != 0) || ((((_la - 171)) & ~0x3f) == 0 && ((1L << (_la - 171)) & 145241087982838785L) != 0) || ((((_la - 245)) & ~0x3f) == 0 && ((1L << (_la - 245)) & 2253174203220225L) != 0) );
setState(1008);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SubqueryClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CALL() { return getToken(Cypher6Parser.CALL, 0); }
public TerminalNode LCURLY() { return getToken(Cypher6Parser.LCURLY, 0); }
public RegularQueryContext regularQuery() {
return getRuleContext(RegularQueryContext.class,0);
}
public TerminalNode RCURLY() { return getToken(Cypher6Parser.RCURLY, 0); }
public TerminalNode OPTIONAL() { return getToken(Cypher6Parser.OPTIONAL, 0); }
public SubqueryScopeContext subqueryScope() {
return getRuleContext(SubqueryScopeContext.class,0);
}
public SubqueryInTransactionsParametersContext subqueryInTransactionsParameters() {
return getRuleContext(SubqueryInTransactionsParametersContext.class,0);
}
public SubqueryClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_subqueryClause; }
}
public final SubqueryClauseContext subqueryClause() throws RecognitionException {
SubqueryClauseContext _localctx = new SubqueryClauseContext(_ctx, getState());
enterRule(_localctx, 78, RULE_subqueryClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1011);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==OPTIONAL) {
{
setState(1010);
match(OPTIONAL);
}
}
setState(1013);
match(CALL);
setState(1015);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LPAREN) {
{
setState(1014);
subqueryScope();
}
}
setState(1017);
match(LCURLY);
setState(1018);
regularQuery();
setState(1019);
match(RCURLY);
setState(1021);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IN) {
{
setState(1020);
subqueryInTransactionsParameters();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SubqueryScopeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public List variable() {
return getRuleContexts(VariableContext.class);
}
public VariableContext variable(int i) {
return getRuleContext(VariableContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public SubqueryScopeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_subqueryScope; }
}
public final SubqueryScopeContext subqueryScope() throws RecognitionException {
SubqueryScopeContext _localctx = new SubqueryScopeContext(_ctx, getState());
enterRule(_localctx, 80, RULE_subqueryScope);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1023);
match(LPAREN);
setState(1033);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(1024);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
{
setState(1025);
variable();
setState(1030);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(1026);
match(COMMA);
setState(1027);
variable();
}
}
setState(1032);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
break;
case RPAREN:
break;
default:
break;
}
setState(1035);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SubqueryInTransactionsParametersContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode IN() { return getToken(Cypher6Parser.IN, 0); }
public TerminalNode TRANSACTIONS() { return getToken(Cypher6Parser.TRANSACTIONS, 0); }
public TerminalNode CONCURRENT() { return getToken(Cypher6Parser.CONCURRENT, 0); }
public List subqueryInTransactionsBatchParameters() {
return getRuleContexts(SubqueryInTransactionsBatchParametersContext.class);
}
public SubqueryInTransactionsBatchParametersContext subqueryInTransactionsBatchParameters(int i) {
return getRuleContext(SubqueryInTransactionsBatchParametersContext.class,i);
}
public List subqueryInTransactionsErrorParameters() {
return getRuleContexts(SubqueryInTransactionsErrorParametersContext.class);
}
public SubqueryInTransactionsErrorParametersContext subqueryInTransactionsErrorParameters(int i) {
return getRuleContext(SubqueryInTransactionsErrorParametersContext.class,i);
}
public List subqueryInTransactionsReportParameters() {
return getRuleContexts(SubqueryInTransactionsReportParametersContext.class);
}
public SubqueryInTransactionsReportParametersContext subqueryInTransactionsReportParameters(int i) {
return getRuleContext(SubqueryInTransactionsReportParametersContext.class,i);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public SubqueryInTransactionsParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_subqueryInTransactionsParameters; }
}
public final SubqueryInTransactionsParametersContext subqueryInTransactionsParameters() throws RecognitionException {
SubqueryInTransactionsParametersContext _localctx = new SubqueryInTransactionsParametersContext(_ctx, getState());
enterRule(_localctx, 82, RULE_subqueryInTransactionsParameters);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1037);
match(IN);
setState(1042);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,56,_ctx) ) {
case 1:
{
setState(1039);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,55,_ctx) ) {
case 1:
{
setState(1038);
expression();
}
break;
}
setState(1041);
match(CONCURRENT);
}
break;
}
setState(1044);
match(TRANSACTIONS);
setState(1050);
_errHandler.sync(this);
_la = _input.LA(1);
while (((((_la - 180)) & ~0x3f) == 0 && ((1L << (_la - 180)) & 17592186044421L) != 0)) {
{
setState(1048);
_errHandler.sync(this);
switch (_input.LA(1)) {
case OF:
{
setState(1045);
subqueryInTransactionsBatchParameters();
}
break;
case ON:
{
setState(1046);
subqueryInTransactionsErrorParameters();
}
break;
case REPORT:
{
setState(1047);
subqueryInTransactionsReportParameters();
}
break;
default:
throw new NoViableAltException(this);
}
}
setState(1052);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SubqueryInTransactionsBatchParametersContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode OF() { return getToken(Cypher6Parser.OF, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode ROW() { return getToken(Cypher6Parser.ROW, 0); }
public TerminalNode ROWS() { return getToken(Cypher6Parser.ROWS, 0); }
public SubqueryInTransactionsBatchParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_subqueryInTransactionsBatchParameters; }
}
public final SubqueryInTransactionsBatchParametersContext subqueryInTransactionsBatchParameters() throws RecognitionException {
SubqueryInTransactionsBatchParametersContext _localctx = new SubqueryInTransactionsBatchParametersContext(_ctx, getState());
enterRule(_localctx, 84, RULE_subqueryInTransactionsBatchParameters);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1053);
match(OF);
setState(1054);
expression();
setState(1055);
_la = _input.LA(1);
if ( !(_la==ROW || _la==ROWS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SubqueryInTransactionsErrorParametersContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public TerminalNode ERROR() { return getToken(Cypher6Parser.ERROR, 0); }
public TerminalNode CONTINUE() { return getToken(Cypher6Parser.CONTINUE, 0); }
public TerminalNode BREAK() { return getToken(Cypher6Parser.BREAK, 0); }
public TerminalNode FAIL() { return getToken(Cypher6Parser.FAIL, 0); }
public SubqueryInTransactionsErrorParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_subqueryInTransactionsErrorParameters; }
}
public final SubqueryInTransactionsErrorParametersContext subqueryInTransactionsErrorParameters() throws RecognitionException {
SubqueryInTransactionsErrorParametersContext _localctx = new SubqueryInTransactionsErrorParametersContext(_ctx, getState());
enterRule(_localctx, 86, RULE_subqueryInTransactionsErrorParameters);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1057);
match(ON);
setState(1058);
match(ERROR);
setState(1059);
_la = _input.LA(1);
if ( !(_la==BREAK || _la==CONTINUE || _la==FAIL) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SubqueryInTransactionsReportParametersContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode REPORT() { return getToken(Cypher6Parser.REPORT, 0); }
public TerminalNode STATUS() { return getToken(Cypher6Parser.STATUS, 0); }
public TerminalNode AS() { return getToken(Cypher6Parser.AS, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public SubqueryInTransactionsReportParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_subqueryInTransactionsReportParameters; }
}
public final SubqueryInTransactionsReportParametersContext subqueryInTransactionsReportParameters() throws RecognitionException {
SubqueryInTransactionsReportParametersContext _localctx = new SubqueryInTransactionsReportParametersContext(_ctx, getState());
enterRule(_localctx, 88, RULE_subqueryInTransactionsReportParameters);
try {
enterOuterAlt(_localctx, 1);
{
setState(1061);
match(REPORT);
setState(1062);
match(STATUS);
setState(1063);
match(AS);
setState(1064);
variable();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class OrderBySkipLimitClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public OrderByContext orderBy() {
return getRuleContext(OrderByContext.class,0);
}
public SkipContext skip() {
return getRuleContext(SkipContext.class,0);
}
public LimitContext limit() {
return getRuleContext(LimitContext.class,0);
}
public OrderBySkipLimitClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_orderBySkipLimitClause; }
}
public final OrderBySkipLimitClauseContext orderBySkipLimitClause() throws RecognitionException {
OrderBySkipLimitClauseContext _localctx = new OrderBySkipLimitClauseContext(_ctx, getState());
enterRule(_localctx, 90, RULE_orderBySkipLimitClause);
try {
setState(1078);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ORDER:
enterOuterAlt(_localctx, 1);
{
setState(1066);
orderBy();
setState(1068);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,59,_ctx) ) {
case 1:
{
setState(1067);
skip();
}
break;
}
setState(1071);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,60,_ctx) ) {
case 1:
{
setState(1070);
limit();
}
break;
}
}
break;
case OFFSET:
case SKIPROWS:
enterOuterAlt(_localctx, 2);
{
setState(1073);
skip();
setState(1075);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,61,_ctx) ) {
case 1:
{
setState(1074);
limit();
}
break;
}
}
break;
case LIMITROWS:
enterOuterAlt(_localctx, 3);
{
setState(1077);
limit();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PatternListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List pattern() {
return getRuleContexts(PatternContext.class);
}
public PatternContext pattern(int i) {
return getRuleContext(PatternContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public PatternListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_patternList; }
}
public final PatternListContext patternList() throws RecognitionException {
PatternListContext _localctx = new PatternListContext(_ctx, getState());
enterRule(_localctx, 92, RULE_patternList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1080);
pattern();
setState(1085);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(1081);
match(COMMA);
setState(1082);
pattern();
}
}
setState(1087);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InsertPatternListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List insertPattern() {
return getRuleContexts(InsertPatternContext.class);
}
public InsertPatternContext insertPattern(int i) {
return getRuleContext(InsertPatternContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public InsertPatternListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_insertPatternList; }
}
public final InsertPatternListContext insertPatternList() throws RecognitionException {
InsertPatternListContext _localctx = new InsertPatternListContext(_ctx, getState());
enterRule(_localctx, 94, RULE_insertPatternList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1088);
insertPattern();
setState(1093);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(1089);
match(COMMA);
setState(1090);
insertPattern();
}
}
setState(1095);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public AnonymousPatternContext anonymousPattern() {
return getRuleContext(AnonymousPatternContext.class,0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public TerminalNode EQ() { return getToken(Cypher6Parser.EQ, 0); }
public SelectorContext selector() {
return getRuleContext(SelectorContext.class,0);
}
public PatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_pattern; }
}
public final PatternContext pattern() throws RecognitionException {
PatternContext _localctx = new PatternContext(_ctx, getState());
enterRule(_localctx, 96, RULE_pattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1099);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,65,_ctx) ) {
case 1:
{
setState(1096);
variable();
setState(1097);
match(EQ);
}
break;
}
setState(1102);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==ALL || _la==ANY || _la==SHORTEST) {
{
setState(1101);
selector();
}
}
setState(1104);
anonymousPattern();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InsertPatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List insertNodePattern() {
return getRuleContexts(InsertNodePatternContext.class);
}
public InsertNodePatternContext insertNodePattern(int i) {
return getRuleContext(InsertNodePatternContext.class,i);
}
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public TerminalNode EQ() { return getToken(Cypher6Parser.EQ, 0); }
public List insertRelationshipPattern() {
return getRuleContexts(InsertRelationshipPatternContext.class);
}
public InsertRelationshipPatternContext insertRelationshipPattern(int i) {
return getRuleContext(InsertRelationshipPatternContext.class,i);
}
public InsertPatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_insertPattern; }
}
public final InsertPatternContext insertPattern() throws RecognitionException {
InsertPatternContext _localctx = new InsertPatternContext(_ctx, getState());
enterRule(_localctx, 98, RULE_insertPattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1109);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -246291141493760L) != 0) || ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -146366996479975425L) != 0) || ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -16156712961L) != 0) || ((((_la - 192)) & ~0x3f) == 0 && ((1L << (_la - 192)) & -1130297988612173L) != 0) || ((((_la - 256)) & ~0x3f) == 0 && ((1L << (_la - 256)) & 281474976709631L) != 0)) {
{
setState(1106);
symbolicNameString();
setState(1107);
match(EQ);
}
}
setState(1111);
insertNodePattern();
setState(1117);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==LT || _la==MINUS || _la==ARROW_LINE || _la==ARROW_LEFT_HEAD) {
{
{
setState(1112);
insertRelationshipPattern();
setState(1113);
insertNodePattern();
}
}
setState(1119);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class QuantifierContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public Token from;
public Token to;
public TerminalNode LCURLY() { return getToken(Cypher6Parser.LCURLY, 0); }
public List UNSIGNED_DECIMAL_INTEGER() { return getTokens(Cypher6Parser.UNSIGNED_DECIMAL_INTEGER); }
public TerminalNode UNSIGNED_DECIMAL_INTEGER(int i) {
return getToken(Cypher6Parser.UNSIGNED_DECIMAL_INTEGER, i);
}
public TerminalNode RCURLY() { return getToken(Cypher6Parser.RCURLY, 0); }
public TerminalNode COMMA() { return getToken(Cypher6Parser.COMMA, 0); }
public TerminalNode PLUS() { return getToken(Cypher6Parser.PLUS, 0); }
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public QuantifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_quantifier; }
}
public final QuantifierContext quantifier() throws RecognitionException {
QuantifierContext _localctx = new QuantifierContext(_ctx, getState());
enterRule(_localctx, 100, RULE_quantifier);
int _la;
try {
setState(1134);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,71,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(1120);
match(LCURLY);
setState(1121);
match(UNSIGNED_DECIMAL_INTEGER);
setState(1122);
match(RCURLY);
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(1123);
match(LCURLY);
setState(1125);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==UNSIGNED_DECIMAL_INTEGER) {
{
setState(1124);
((QuantifierContext)_localctx).from = match(UNSIGNED_DECIMAL_INTEGER);
}
}
setState(1127);
match(COMMA);
setState(1129);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==UNSIGNED_DECIMAL_INTEGER) {
{
setState(1128);
((QuantifierContext)_localctx).to = match(UNSIGNED_DECIMAL_INTEGER);
}
}
setState(1131);
match(RCURLY);
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(1132);
match(PLUS);
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(1133);
match(TIMES);
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AnonymousPatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ShortestPathPatternContext shortestPathPattern() {
return getRuleContext(ShortestPathPatternContext.class,0);
}
public PatternElementContext patternElement() {
return getRuleContext(PatternElementContext.class,0);
}
public AnonymousPatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_anonymousPattern; }
}
public final AnonymousPatternContext anonymousPattern() throws RecognitionException {
AnonymousPatternContext _localctx = new AnonymousPatternContext(_ctx, getState());
enterRule(_localctx, 102, RULE_anonymousPattern);
try {
setState(1138);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALL_SHORTEST_PATHS:
case SHORTEST_PATH:
enterOuterAlt(_localctx, 1);
{
setState(1136);
shortestPathPattern();
}
break;
case LPAREN:
enterOuterAlt(_localctx, 2);
{
setState(1137);
patternElement();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShortestPathPatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public PatternElementContext patternElement() {
return getRuleContext(PatternElementContext.class,0);
}
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public TerminalNode SHORTEST_PATH() { return getToken(Cypher6Parser.SHORTEST_PATH, 0); }
public TerminalNode ALL_SHORTEST_PATHS() { return getToken(Cypher6Parser.ALL_SHORTEST_PATHS, 0); }
public ShortestPathPatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_shortestPathPattern; }
}
public final ShortestPathPatternContext shortestPathPattern() throws RecognitionException {
ShortestPathPatternContext _localctx = new ShortestPathPatternContext(_ctx, getState());
enterRule(_localctx, 104, RULE_shortestPathPattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1140);
_la = _input.LA(1);
if ( !(_la==ALL_SHORTEST_PATHS || _la==SHORTEST_PATH) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1141);
match(LPAREN);
setState(1142);
patternElement();
setState(1143);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PatternElementContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List nodePattern() {
return getRuleContexts(NodePatternContext.class);
}
public NodePatternContext nodePattern(int i) {
return getRuleContext(NodePatternContext.class,i);
}
public List parenthesizedPath() {
return getRuleContexts(ParenthesizedPathContext.class);
}
public ParenthesizedPathContext parenthesizedPath(int i) {
return getRuleContext(ParenthesizedPathContext.class,i);
}
public List relationshipPattern() {
return getRuleContexts(RelationshipPatternContext.class);
}
public RelationshipPatternContext relationshipPattern(int i) {
return getRuleContext(RelationshipPatternContext.class,i);
}
public List quantifier() {
return getRuleContexts(QuantifierContext.class);
}
public QuantifierContext quantifier(int i) {
return getRuleContext(QuantifierContext.class,i);
}
public PatternElementContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_patternElement; }
}
public final PatternElementContext patternElement() throws RecognitionException {
PatternElementContext _localctx = new PatternElementContext(_ctx, getState());
enterRule(_localctx, 106, RULE_patternElement);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1158);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
setState(1158);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,75,_ctx) ) {
case 1:
{
setState(1145);
nodePattern();
setState(1154);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==LT || _la==MINUS || _la==ARROW_LINE || _la==ARROW_LEFT_HEAD) {
{
{
setState(1146);
relationshipPattern();
setState(1148);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LCURLY || _la==PLUS || _la==TIMES) {
{
setState(1147);
quantifier();
}
}
setState(1150);
nodePattern();
}
}
setState(1156);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
break;
case 2:
{
setState(1157);
parenthesizedPath();
}
break;
}
}
setState(1160);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==LPAREN );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SelectorContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SelectorContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_selector; }
public SelectorContext() { }
public void copyFrom(SelectorContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class AllShortestPathContext extends SelectorContext {
public TerminalNode ALL() { return getToken(Cypher6Parser.ALL, 0); }
public TerminalNode SHORTEST() { return getToken(Cypher6Parser.SHORTEST, 0); }
public PathTokenContext pathToken() {
return getRuleContext(PathTokenContext.class,0);
}
public AllShortestPathContext(SelectorContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class AnyPathContext extends SelectorContext {
public TerminalNode ANY() { return getToken(Cypher6Parser.ANY, 0); }
public TerminalNode UNSIGNED_DECIMAL_INTEGER() { return getToken(Cypher6Parser.UNSIGNED_DECIMAL_INTEGER, 0); }
public PathTokenContext pathToken() {
return getRuleContext(PathTokenContext.class,0);
}
public AnyPathContext(SelectorContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class ShortestGroupContext extends SelectorContext {
public TerminalNode SHORTEST() { return getToken(Cypher6Parser.SHORTEST, 0); }
public GroupTokenContext groupToken() {
return getRuleContext(GroupTokenContext.class,0);
}
public TerminalNode UNSIGNED_DECIMAL_INTEGER() { return getToken(Cypher6Parser.UNSIGNED_DECIMAL_INTEGER, 0); }
public PathTokenContext pathToken() {
return getRuleContext(PathTokenContext.class,0);
}
public ShortestGroupContext(SelectorContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class AnyShortestPathContext extends SelectorContext {
public TerminalNode ANY() { return getToken(Cypher6Parser.ANY, 0); }
public TerminalNode SHORTEST() { return getToken(Cypher6Parser.SHORTEST, 0); }
public PathTokenContext pathToken() {
return getRuleContext(PathTokenContext.class,0);
}
public TerminalNode UNSIGNED_DECIMAL_INTEGER() { return getToken(Cypher6Parser.UNSIGNED_DECIMAL_INTEGER, 0); }
public AnyShortestPathContext(SelectorContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class AllPathContext extends SelectorContext {
public TerminalNode ALL() { return getToken(Cypher6Parser.ALL, 0); }
public PathTokenContext pathToken() {
return getRuleContext(PathTokenContext.class,0);
}
public AllPathContext(SelectorContext ctx) { copyFrom(ctx); }
}
public final SelectorContext selector() throws RecognitionException {
SelectorContext _localctx = new SelectorContext(_ctx, getState());
enterRule(_localctx, 108, RULE_selector);
int _la;
try {
setState(1196);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,85,_ctx) ) {
case 1:
_localctx = new AnyShortestPathContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(1162);
match(ANY);
setState(1163);
match(SHORTEST);
setState(1165);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==PATH || _la==PATHS) {
{
setState(1164);
pathToken();
}
}
}
break;
case 2:
_localctx = new AllShortestPathContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(1167);
match(ALL);
setState(1168);
match(SHORTEST);
setState(1170);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==PATH || _la==PATHS) {
{
setState(1169);
pathToken();
}
}
}
break;
case 3:
_localctx = new AnyPathContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(1172);
match(ANY);
setState(1174);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==UNSIGNED_DECIMAL_INTEGER) {
{
setState(1173);
match(UNSIGNED_DECIMAL_INTEGER);
}
}
setState(1177);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==PATH || _la==PATHS) {
{
setState(1176);
pathToken();
}
}
}
break;
case 4:
_localctx = new AllPathContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(1179);
match(ALL);
setState(1181);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==PATH || _la==PATHS) {
{
setState(1180);
pathToken();
}
}
}
break;
case 5:
_localctx = new ShortestGroupContext(_localctx);
enterOuterAlt(_localctx, 5);
{
setState(1183);
match(SHORTEST);
setState(1185);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==UNSIGNED_DECIMAL_INTEGER) {
{
setState(1184);
match(UNSIGNED_DECIMAL_INTEGER);
}
}
setState(1188);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==PATH || _la==PATHS) {
{
setState(1187);
pathToken();
}
}
setState(1190);
groupToken();
}
break;
case 6:
_localctx = new AnyShortestPathContext(_localctx);
enterOuterAlt(_localctx, 6);
{
setState(1191);
match(SHORTEST);
setState(1192);
match(UNSIGNED_DECIMAL_INTEGER);
setState(1194);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==PATH || _la==PATHS) {
{
setState(1193);
pathToken();
}
}
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GroupTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode GROUP() { return getToken(Cypher6Parser.GROUP, 0); }
public TerminalNode GROUPS() { return getToken(Cypher6Parser.GROUPS, 0); }
public GroupTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_groupToken; }
}
public final GroupTokenContext groupToken() throws RecognitionException {
GroupTokenContext _localctx = new GroupTokenContext(_ctx, getState());
enterRule(_localctx, 110, RULE_groupToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1198);
_la = _input.LA(1);
if ( !(_la==GROUP || _la==GROUPS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PathTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PATH() { return getToken(Cypher6Parser.PATH, 0); }
public TerminalNode PATHS() { return getToken(Cypher6Parser.PATHS, 0); }
public PathTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_pathToken; }
}
public final PathTokenContext pathToken() throws RecognitionException {
PathTokenContext _localctx = new PathTokenContext(_ctx, getState());
enterRule(_localctx, 112, RULE_pathToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1200);
_la = _input.LA(1);
if ( !(_la==PATH || _la==PATHS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PathPatternNonEmptyContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List nodePattern() {
return getRuleContexts(NodePatternContext.class);
}
public NodePatternContext nodePattern(int i) {
return getRuleContext(NodePatternContext.class,i);
}
public List relationshipPattern() {
return getRuleContexts(RelationshipPatternContext.class);
}
public RelationshipPatternContext relationshipPattern(int i) {
return getRuleContext(RelationshipPatternContext.class,i);
}
public PathPatternNonEmptyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_pathPatternNonEmpty; }
}
public final PathPatternNonEmptyContext pathPatternNonEmpty() throws RecognitionException {
PathPatternNonEmptyContext _localctx = new PathPatternNonEmptyContext(_ctx, getState());
enterRule(_localctx, 114, RULE_pathPatternNonEmpty);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1202);
nodePattern();
setState(1206);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(1203);
relationshipPattern();
setState(1204);
nodePattern();
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(1208);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,86,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NodePatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public LabelExpressionContext labelExpression() {
return getRuleContext(LabelExpressionContext.class,0);
}
public PropertiesContext properties() {
return getRuleContext(PropertiesContext.class,0);
}
public TerminalNode WHERE() { return getToken(Cypher6Parser.WHERE, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public NodePatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_nodePattern; }
}
public final NodePatternContext nodePattern() throws RecognitionException {
NodePatternContext _localctx = new NodePatternContext(_ctx, getState());
enterRule(_localctx, 116, RULE_nodePattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1210);
match(LPAREN);
setState(1212);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,87,_ctx) ) {
case 1:
{
setState(1211);
variable();
}
break;
}
setState(1215);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COLON || _la==IS) {
{
setState(1214);
labelExpression();
}
}
setState(1218);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DOLLAR || _la==LCURLY) {
{
setState(1217);
properties();
}
}
setState(1222);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE) {
{
setState(1220);
match(WHERE);
setState(1221);
expression();
}
}
setState(1224);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InsertNodePatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public InsertNodeLabelExpressionContext insertNodeLabelExpression() {
return getRuleContext(InsertNodeLabelExpressionContext.class,0);
}
public MapContext map() {
return getRuleContext(MapContext.class,0);
}
public InsertNodePatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_insertNodePattern; }
}
public final InsertNodePatternContext insertNodePattern() throws RecognitionException {
InsertNodePatternContext _localctx = new InsertNodePatternContext(_ctx, getState());
enterRule(_localctx, 118, RULE_insertNodePattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1226);
match(LPAREN);
setState(1228);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,91,_ctx) ) {
case 1:
{
setState(1227);
variable();
}
break;
}
setState(1231);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COLON || _la==IS) {
{
setState(1230);
insertNodeLabelExpression();
}
}
setState(1234);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LCURLY) {
{
setState(1233);
map();
}
}
setState(1236);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParenthesizedPathContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public PatternContext pattern() {
return getRuleContext(PatternContext.class,0);
}
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public TerminalNode WHERE() { return getToken(Cypher6Parser.WHERE, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public QuantifierContext quantifier() {
return getRuleContext(QuantifierContext.class,0);
}
public ParenthesizedPathContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parenthesizedPath; }
}
public final ParenthesizedPathContext parenthesizedPath() throws RecognitionException {
ParenthesizedPathContext _localctx = new ParenthesizedPathContext(_ctx, getState());
enterRule(_localctx, 120, RULE_parenthesizedPath);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1238);
match(LPAREN);
setState(1239);
pattern();
setState(1242);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE) {
{
setState(1240);
match(WHERE);
setState(1241);
expression();
}
}
setState(1244);
match(RPAREN);
setState(1246);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LCURLY || _la==PLUS || _la==TIMES) {
{
setState(1245);
quantifier();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NodeLabelsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List labelType() {
return getRuleContexts(LabelTypeContext.class);
}
public LabelTypeContext labelType(int i) {
return getRuleContext(LabelTypeContext.class,i);
}
public List dynamicLabelType() {
return getRuleContexts(DynamicLabelTypeContext.class);
}
public DynamicLabelTypeContext dynamicLabelType(int i) {
return getRuleContext(DynamicLabelTypeContext.class,i);
}
public NodeLabelsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_nodeLabels; }
}
public final NodeLabelsContext nodeLabels() throws RecognitionException {
NodeLabelsContext _localctx = new NodeLabelsContext(_ctx, getState());
enterRule(_localctx, 122, RULE_nodeLabels);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1250);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
setState(1250);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,96,_ctx) ) {
case 1:
{
setState(1248);
labelType();
}
break;
case 2:
{
setState(1249);
dynamicLabelType();
}
break;
}
}
setState(1252);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==COLON );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NodeLabelsIsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode IS() { return getToken(Cypher6Parser.IS, 0); }
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public DynamicExpressionContext dynamicExpression() {
return getRuleContext(DynamicExpressionContext.class,0);
}
public List labelType() {
return getRuleContexts(LabelTypeContext.class);
}
public LabelTypeContext labelType(int i) {
return getRuleContext(LabelTypeContext.class,i);
}
public List dynamicLabelType() {
return getRuleContexts(DynamicLabelTypeContext.class);
}
public DynamicLabelTypeContext dynamicLabelType(int i) {
return getRuleContext(DynamicLabelTypeContext.class,i);
}
public NodeLabelsIsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_nodeLabelsIs; }
}
public final NodeLabelsIsContext nodeLabelsIs() throws RecognitionException {
NodeLabelsIsContext _localctx = new NodeLabelsIsContext(_ctx, getState());
enterRule(_localctx, 124, RULE_nodeLabelsIs);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1254);
match(IS);
setState(1257);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
{
setState(1255);
symbolicNameString();
}
break;
case DOLLAR:
{
setState(1256);
dynamicExpression();
}
break;
default:
throw new NoViableAltException(this);
}
setState(1263);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COLON) {
{
setState(1261);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,99,_ctx) ) {
case 1:
{
setState(1259);
labelType();
}
break;
case 2:
{
setState(1260);
dynamicLabelType();
}
break;
}
}
setState(1265);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DynamicExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DOLLAR() { return getToken(Cypher6Parser.DOLLAR, 0); }
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public DynamicExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dynamicExpression; }
}
public final DynamicExpressionContext dynamicExpression() throws RecognitionException {
DynamicExpressionContext _localctx = new DynamicExpressionContext(_ctx, getState());
enterRule(_localctx, 126, RULE_dynamicExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(1266);
match(DOLLAR);
setState(1267);
match(LPAREN);
setState(1268);
expression();
setState(1269);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DynamicLabelTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COLON() { return getToken(Cypher6Parser.COLON, 0); }
public DynamicExpressionContext dynamicExpression() {
return getRuleContext(DynamicExpressionContext.class,0);
}
public DynamicLabelTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dynamicLabelType; }
}
public final DynamicLabelTypeContext dynamicLabelType() throws RecognitionException {
DynamicLabelTypeContext _localctx = new DynamicLabelTypeContext(_ctx, getState());
enterRule(_localctx, 128, RULE_dynamicLabelType);
try {
enterOuterAlt(_localctx, 1);
{
setState(1271);
match(COLON);
setState(1272);
dynamicExpression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COLON() { return getToken(Cypher6Parser.COLON, 0); }
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public LabelTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_labelType; }
}
public final LabelTypeContext labelType() throws RecognitionException {
LabelTypeContext _localctx = new LabelTypeContext(_ctx, getState());
enterRule(_localctx, 130, RULE_labelType);
try {
enterOuterAlt(_localctx, 1);
{
setState(1274);
match(COLON);
setState(1275);
symbolicNameString();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RelTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COLON() { return getToken(Cypher6Parser.COLON, 0); }
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public RelTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_relType; }
}
public final RelTypeContext relType() throws RecognitionException {
RelTypeContext _localctx = new RelTypeContext(_ctx, getState());
enterRule(_localctx, 132, RULE_relType);
try {
enterOuterAlt(_localctx, 1);
{
setState(1277);
match(COLON);
setState(1278);
symbolicNameString();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelOrRelTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COLON() { return getToken(Cypher6Parser.COLON, 0); }
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public LabelOrRelTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_labelOrRelType; }
}
public final LabelOrRelTypeContext labelOrRelType() throws RecognitionException {
LabelOrRelTypeContext _localctx = new LabelOrRelTypeContext(_ctx, getState());
enterRule(_localctx, 134, RULE_labelOrRelType);
try {
enterOuterAlt(_localctx, 1);
{
setState(1280);
match(COLON);
setState(1281);
symbolicNameString();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PropertiesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public MapContext map() {
return getRuleContext(MapContext.class,0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class,0);
}
public PropertiesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_properties; }
}
public final PropertiesContext properties() throws RecognitionException {
PropertiesContext _localctx = new PropertiesContext(_ctx, getState());
enterRule(_localctx, 136, RULE_properties);
try {
setState(1285);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LCURLY:
enterOuterAlt(_localctx, 1);
{
setState(1283);
map();
}
break;
case DOLLAR:
enterOuterAlt(_localctx, 2);
{
setState(1284);
parameter("ANY");
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RelationshipPatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List arrowLine() {
return getRuleContexts(ArrowLineContext.class);
}
public ArrowLineContext arrowLine(int i) {
return getRuleContext(ArrowLineContext.class,i);
}
public LeftArrowContext leftArrow() {
return getRuleContext(LeftArrowContext.class,0);
}
public TerminalNode LBRACKET() { return getToken(Cypher6Parser.LBRACKET, 0); }
public TerminalNode RBRACKET() { return getToken(Cypher6Parser.RBRACKET, 0); }
public RightArrowContext rightArrow() {
return getRuleContext(RightArrowContext.class,0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public LabelExpressionContext labelExpression() {
return getRuleContext(LabelExpressionContext.class,0);
}
public PathLengthContext pathLength() {
return getRuleContext(PathLengthContext.class,0);
}
public PropertiesContext properties() {
return getRuleContext(PropertiesContext.class,0);
}
public TerminalNode WHERE() { return getToken(Cypher6Parser.WHERE, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public RelationshipPatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_relationshipPattern; }
}
public final RelationshipPatternContext relationshipPattern() throws RecognitionException {
RelationshipPatternContext _localctx = new RelationshipPatternContext(_ctx, getState());
enterRule(_localctx, 138, RULE_relationshipPattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1288);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LT || _la==ARROW_LEFT_HEAD) {
{
setState(1287);
leftArrow();
}
}
setState(1290);
arrowLine();
setState(1309);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LBRACKET) {
{
setState(1291);
match(LBRACKET);
setState(1293);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,103,_ctx) ) {
case 1:
{
setState(1292);
variable();
}
break;
}
setState(1296);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COLON || _la==IS) {
{
setState(1295);
labelExpression();
}
}
setState(1299);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==TIMES) {
{
setState(1298);
pathLength();
}
}
setState(1302);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DOLLAR || _la==LCURLY) {
{
setState(1301);
properties();
}
}
setState(1306);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE) {
{
setState(1304);
match(WHERE);
setState(1305);
expression();
}
}
setState(1308);
match(RBRACKET);
}
}
setState(1311);
arrowLine();
setState(1313);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==GT || _la==ARROW_RIGHT_HEAD) {
{
setState(1312);
rightArrow();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InsertRelationshipPatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List arrowLine() {
return getRuleContexts(ArrowLineContext.class);
}
public ArrowLineContext arrowLine(int i) {
return getRuleContext(ArrowLineContext.class,i);
}
public TerminalNode LBRACKET() { return getToken(Cypher6Parser.LBRACKET, 0); }
public InsertRelationshipLabelExpressionContext insertRelationshipLabelExpression() {
return getRuleContext(InsertRelationshipLabelExpressionContext.class,0);
}
public TerminalNode RBRACKET() { return getToken(Cypher6Parser.RBRACKET, 0); }
public LeftArrowContext leftArrow() {
return getRuleContext(LeftArrowContext.class,0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public MapContext map() {
return getRuleContext(MapContext.class,0);
}
public RightArrowContext rightArrow() {
return getRuleContext(RightArrowContext.class,0);
}
public InsertRelationshipPatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_insertRelationshipPattern; }
}
public final InsertRelationshipPatternContext insertRelationshipPattern() throws RecognitionException {
InsertRelationshipPatternContext _localctx = new InsertRelationshipPatternContext(_ctx, getState());
enterRule(_localctx, 140, RULE_insertRelationshipPattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1316);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LT || _la==ARROW_LEFT_HEAD) {
{
setState(1315);
leftArrow();
}
}
setState(1318);
arrowLine();
setState(1319);
match(LBRACKET);
setState(1321);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,111,_ctx) ) {
case 1:
{
setState(1320);
variable();
}
break;
}
setState(1323);
insertRelationshipLabelExpression();
setState(1325);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LCURLY) {
{
setState(1324);
map();
}
}
setState(1327);
match(RBRACKET);
setState(1328);
arrowLine();
setState(1330);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==GT || _la==ARROW_RIGHT_HEAD) {
{
setState(1329);
rightArrow();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LeftArrowContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LT() { return getToken(Cypher6Parser.LT, 0); }
public TerminalNode ARROW_LEFT_HEAD() { return getToken(Cypher6Parser.ARROW_LEFT_HEAD, 0); }
public LeftArrowContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_leftArrow; }
}
public final LeftArrowContext leftArrow() throws RecognitionException {
LeftArrowContext _localctx = new LeftArrowContext(_ctx, getState());
enterRule(_localctx, 142, RULE_leftArrow);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1332);
_la = _input.LA(1);
if ( !(_la==LT || _la==ARROW_LEFT_HEAD) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ArrowLineContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ARROW_LINE() { return getToken(Cypher6Parser.ARROW_LINE, 0); }
public TerminalNode MINUS() { return getToken(Cypher6Parser.MINUS, 0); }
public ArrowLineContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_arrowLine; }
}
public final ArrowLineContext arrowLine() throws RecognitionException {
ArrowLineContext _localctx = new ArrowLineContext(_ctx, getState());
enterRule(_localctx, 144, RULE_arrowLine);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1334);
_la = _input.LA(1);
if ( !(_la==MINUS || _la==ARROW_LINE) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RightArrowContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode GT() { return getToken(Cypher6Parser.GT, 0); }
public TerminalNode ARROW_RIGHT_HEAD() { return getToken(Cypher6Parser.ARROW_RIGHT_HEAD, 0); }
public RightArrowContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_rightArrow; }
}
public final RightArrowContext rightArrow() throws RecognitionException {
RightArrowContext _localctx = new RightArrowContext(_ctx, getState());
enterRule(_localctx, 146, RULE_rightArrow);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1336);
_la = _input.LA(1);
if ( !(_la==GT || _la==ARROW_RIGHT_HEAD) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PathLengthContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public Token from;
public Token to;
public Token single;
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public TerminalNode DOTDOT() { return getToken(Cypher6Parser.DOTDOT, 0); }
public List UNSIGNED_DECIMAL_INTEGER() { return getTokens(Cypher6Parser.UNSIGNED_DECIMAL_INTEGER); }
public TerminalNode UNSIGNED_DECIMAL_INTEGER(int i) {
return getToken(Cypher6Parser.UNSIGNED_DECIMAL_INTEGER, i);
}
public PathLengthContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_pathLength; }
}
public final PathLengthContext pathLength() throws RecognitionException {
PathLengthContext _localctx = new PathLengthContext(_ctx, getState());
enterRule(_localctx, 148, RULE_pathLength);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1338);
match(TIMES);
setState(1347);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,116,_ctx) ) {
case 1:
{
setState(1340);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==UNSIGNED_DECIMAL_INTEGER) {
{
setState(1339);
((PathLengthContext)_localctx).from = match(UNSIGNED_DECIMAL_INTEGER);
}
}
setState(1342);
match(DOTDOT);
setState(1344);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==UNSIGNED_DECIMAL_INTEGER) {
{
setState(1343);
((PathLengthContext)_localctx).to = match(UNSIGNED_DECIMAL_INTEGER);
}
}
}
break;
case 2:
{
setState(1346);
((PathLengthContext)_localctx).single = match(UNSIGNED_DECIMAL_INTEGER);
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COLON() { return getToken(Cypher6Parser.COLON, 0); }
public LabelExpression4Context labelExpression4() {
return getRuleContext(LabelExpression4Context.class,0);
}
public TerminalNode IS() { return getToken(Cypher6Parser.IS, 0); }
public LabelExpression4IsContext labelExpression4Is() {
return getRuleContext(LabelExpression4IsContext.class,0);
}
public LabelExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_labelExpression; }
}
public final LabelExpressionContext labelExpression() throws RecognitionException {
LabelExpressionContext _localctx = new LabelExpressionContext(_ctx, getState());
enterRule(_localctx, 150, RULE_labelExpression);
try {
setState(1353);
_errHandler.sync(this);
switch (_input.LA(1)) {
case COLON:
enterOuterAlt(_localctx, 1);
{
setState(1349);
match(COLON);
setState(1350);
labelExpression4();
}
break;
case IS:
enterOuterAlt(_localctx, 2);
{
setState(1351);
match(IS);
setState(1352);
labelExpression4Is();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpression4Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List labelExpression3() {
return getRuleContexts(LabelExpression3Context.class);
}
public LabelExpression3Context labelExpression3(int i) {
return getRuleContext(LabelExpression3Context.class,i);
}
public List BAR() { return getTokens(Cypher6Parser.BAR); }
public TerminalNode BAR(int i) {
return getToken(Cypher6Parser.BAR, i);
}
public List COLON() { return getTokens(Cypher6Parser.COLON); }
public TerminalNode COLON(int i) {
return getToken(Cypher6Parser.COLON, i);
}
public LabelExpression4Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_labelExpression4; }
}
public final LabelExpression4Context labelExpression4() throws RecognitionException {
LabelExpression4Context _localctx = new LabelExpression4Context(_ctx, getState());
enterRule(_localctx, 152, RULE_labelExpression4);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1355);
labelExpression3();
setState(1363);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,119,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1356);
match(BAR);
setState(1358);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COLON) {
{
setState(1357);
match(COLON);
}
}
setState(1360);
labelExpression3();
}
}
}
setState(1365);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,119,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpression4IsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List labelExpression3Is() {
return getRuleContexts(LabelExpression3IsContext.class);
}
public LabelExpression3IsContext labelExpression3Is(int i) {
return getRuleContext(LabelExpression3IsContext.class,i);
}
public List BAR() { return getTokens(Cypher6Parser.BAR); }
public TerminalNode BAR(int i) {
return getToken(Cypher6Parser.BAR, i);
}
public List COLON() { return getTokens(Cypher6Parser.COLON); }
public TerminalNode COLON(int i) {
return getToken(Cypher6Parser.COLON, i);
}
public LabelExpression4IsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_labelExpression4Is; }
}
public final LabelExpression4IsContext labelExpression4Is() throws RecognitionException {
LabelExpression4IsContext _localctx = new LabelExpression4IsContext(_ctx, getState());
enterRule(_localctx, 154, RULE_labelExpression4Is);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1366);
labelExpression3Is();
setState(1374);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,121,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1367);
match(BAR);
setState(1369);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COLON) {
{
setState(1368);
match(COLON);
}
}
setState(1371);
labelExpression3Is();
}
}
}
setState(1376);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,121,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpression3Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List labelExpression2() {
return getRuleContexts(LabelExpression2Context.class);
}
public LabelExpression2Context labelExpression2(int i) {
return getRuleContext(LabelExpression2Context.class,i);
}
public List AMPERSAND() { return getTokens(Cypher6Parser.AMPERSAND); }
public TerminalNode AMPERSAND(int i) {
return getToken(Cypher6Parser.AMPERSAND, i);
}
public List COLON() { return getTokens(Cypher6Parser.COLON); }
public TerminalNode COLON(int i) {
return getToken(Cypher6Parser.COLON, i);
}
public LabelExpression3Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_labelExpression3; }
}
public final LabelExpression3Context labelExpression3() throws RecognitionException {
LabelExpression3Context _localctx = new LabelExpression3Context(_ctx, getState());
enterRule(_localctx, 156, RULE_labelExpression3);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1377);
labelExpression2();
setState(1382);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,122,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1378);
_la = _input.LA(1);
if ( !(_la==COLON || _la==AMPERSAND) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1379);
labelExpression2();
}
}
}
setState(1384);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,122,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpression3IsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List labelExpression2Is() {
return getRuleContexts(LabelExpression2IsContext.class);
}
public LabelExpression2IsContext labelExpression2Is(int i) {
return getRuleContext(LabelExpression2IsContext.class,i);
}
public List AMPERSAND() { return getTokens(Cypher6Parser.AMPERSAND); }
public TerminalNode AMPERSAND(int i) {
return getToken(Cypher6Parser.AMPERSAND, i);
}
public List COLON() { return getTokens(Cypher6Parser.COLON); }
public TerminalNode COLON(int i) {
return getToken(Cypher6Parser.COLON, i);
}
public LabelExpression3IsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_labelExpression3Is; }
}
public final LabelExpression3IsContext labelExpression3Is() throws RecognitionException {
LabelExpression3IsContext _localctx = new LabelExpression3IsContext(_ctx, getState());
enterRule(_localctx, 158, RULE_labelExpression3Is);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1385);
labelExpression2Is();
setState(1390);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,123,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1386);
_la = _input.LA(1);
if ( !(_la==COLON || _la==AMPERSAND) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1387);
labelExpression2Is();
}
}
}
setState(1392);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,123,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpression2Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public LabelExpression1Context labelExpression1() {
return getRuleContext(LabelExpression1Context.class,0);
}
public List EXCLAMATION_MARK() { return getTokens(Cypher6Parser.EXCLAMATION_MARK); }
public TerminalNode EXCLAMATION_MARK(int i) {
return getToken(Cypher6Parser.EXCLAMATION_MARK, i);
}
public LabelExpression2Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_labelExpression2; }
}
public final LabelExpression2Context labelExpression2() throws RecognitionException {
LabelExpression2Context _localctx = new LabelExpression2Context(_ctx, getState());
enterRule(_localctx, 160, RULE_labelExpression2);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1396);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==EXCLAMATION_MARK) {
{
{
setState(1393);
match(EXCLAMATION_MARK);
}
}
setState(1398);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1399);
labelExpression1();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpression2IsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public LabelExpression1IsContext labelExpression1Is() {
return getRuleContext(LabelExpression1IsContext.class,0);
}
public List EXCLAMATION_MARK() { return getTokens(Cypher6Parser.EXCLAMATION_MARK); }
public TerminalNode EXCLAMATION_MARK(int i) {
return getToken(Cypher6Parser.EXCLAMATION_MARK, i);
}
public LabelExpression2IsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_labelExpression2Is; }
}
public final LabelExpression2IsContext labelExpression2Is() throws RecognitionException {
LabelExpression2IsContext _localctx = new LabelExpression2IsContext(_ctx, getState());
enterRule(_localctx, 162, RULE_labelExpression2Is);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1404);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==EXCLAMATION_MARK) {
{
{
setState(1401);
match(EXCLAMATION_MARK);
}
}
setState(1406);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1407);
labelExpression1Is();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpression1Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public LabelExpression1Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_labelExpression1; }
public LabelExpression1Context() { }
public void copyFrom(LabelExpression1Context ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class AnyLabelContext extends LabelExpression1Context {
public TerminalNode PERCENT() { return getToken(Cypher6Parser.PERCENT, 0); }
public AnyLabelContext(LabelExpression1Context ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class LabelNameContext extends LabelExpression1Context {
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public LabelNameContext(LabelExpression1Context ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class ParenthesizedLabelExpressionContext extends LabelExpression1Context {
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public LabelExpression4Context labelExpression4() {
return getRuleContext(LabelExpression4Context.class,0);
}
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public ParenthesizedLabelExpressionContext(LabelExpression1Context ctx) { copyFrom(ctx); }
}
public final LabelExpression1Context labelExpression1() throws RecognitionException {
LabelExpression1Context _localctx = new LabelExpression1Context(_ctx, getState());
enterRule(_localctx, 164, RULE_labelExpression1);
try {
setState(1415);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LPAREN:
_localctx = new ParenthesizedLabelExpressionContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(1409);
match(LPAREN);
setState(1410);
labelExpression4();
setState(1411);
match(RPAREN);
}
break;
case PERCENT:
_localctx = new AnyLabelContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(1413);
match(PERCENT);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
_localctx = new LabelNameContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(1414);
symbolicNameString();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpression1IsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public LabelExpression1IsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_labelExpression1Is; }
public LabelExpression1IsContext() { }
public void copyFrom(LabelExpression1IsContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ParenthesizedLabelExpressionIsContext extends LabelExpression1IsContext {
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public LabelExpression4IsContext labelExpression4Is() {
return getRuleContext(LabelExpression4IsContext.class,0);
}
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public ParenthesizedLabelExpressionIsContext(LabelExpression1IsContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class AnyLabelIsContext extends LabelExpression1IsContext {
public TerminalNode PERCENT() { return getToken(Cypher6Parser.PERCENT, 0); }
public AnyLabelIsContext(LabelExpression1IsContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class LabelNameIsContext extends LabelExpression1IsContext {
public SymbolicLabelNameStringContext symbolicLabelNameString() {
return getRuleContext(SymbolicLabelNameStringContext.class,0);
}
public LabelNameIsContext(LabelExpression1IsContext ctx) { copyFrom(ctx); }
}
public final LabelExpression1IsContext labelExpression1Is() throws RecognitionException {
LabelExpression1IsContext _localctx = new LabelExpression1IsContext(_ctx, getState());
enterRule(_localctx, 166, RULE_labelExpression1Is);
try {
setState(1423);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LPAREN:
_localctx = new ParenthesizedLabelExpressionIsContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(1417);
match(LPAREN);
setState(1418);
labelExpression4Is();
setState(1419);
match(RPAREN);
}
break;
case PERCENT:
_localctx = new AnyLabelIsContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(1421);
match(PERCENT);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NOTHING:
case NOWAIT:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
_localctx = new LabelNameIsContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(1422);
symbolicLabelNameString();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InsertNodeLabelExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class,i);
}
public List COLON() { return getTokens(Cypher6Parser.COLON); }
public TerminalNode COLON(int i) {
return getToken(Cypher6Parser.COLON, i);
}
public TerminalNode IS() { return getToken(Cypher6Parser.IS, 0); }
public List AMPERSAND() { return getTokens(Cypher6Parser.AMPERSAND); }
public TerminalNode AMPERSAND(int i) {
return getToken(Cypher6Parser.AMPERSAND, i);
}
public InsertNodeLabelExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_insertNodeLabelExpression; }
}
public final InsertNodeLabelExpressionContext insertNodeLabelExpression() throws RecognitionException {
InsertNodeLabelExpressionContext _localctx = new InsertNodeLabelExpressionContext(_ctx, getState());
enterRule(_localctx, 168, RULE_insertNodeLabelExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1425);
_la = _input.LA(1);
if ( !(_la==COLON || _la==IS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1426);
symbolicNameString();
setState(1431);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COLON || _la==AMPERSAND) {
{
{
setState(1427);
_la = _input.LA(1);
if ( !(_la==COLON || _la==AMPERSAND) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1428);
symbolicNameString();
}
}
setState(1433);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InsertRelationshipLabelExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public TerminalNode COLON() { return getToken(Cypher6Parser.COLON, 0); }
public TerminalNode IS() { return getToken(Cypher6Parser.IS, 0); }
public InsertRelationshipLabelExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_insertRelationshipLabelExpression; }
}
public final InsertRelationshipLabelExpressionContext insertRelationshipLabelExpression() throws RecognitionException {
InsertRelationshipLabelExpressionContext _localctx = new InsertRelationshipLabelExpressionContext(_ctx, getState());
enterRule(_localctx, 170, RULE_insertRelationshipLabelExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1434);
_la = _input.LA(1);
if ( !(_la==COLON || _la==IS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1435);
symbolicNameString();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List expression11() {
return getRuleContexts(Expression11Context.class);
}
public Expression11Context expression11(int i) {
return getRuleContext(Expression11Context.class,i);
}
public List OR() { return getTokens(Cypher6Parser.OR); }
public TerminalNode OR(int i) {
return getToken(Cypher6Parser.OR, i);
}
public ExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression; }
}
public final ExpressionContext expression() throws RecognitionException {
ExpressionContext _localctx = new ExpressionContext(_ctx, getState());
enterRule(_localctx, 172, RULE_expression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1437);
expression11();
setState(1442);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==OR) {
{
{
setState(1438);
match(OR);
setState(1439);
expression11();
}
}
setState(1444);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression11Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List expression10() {
return getRuleContexts(Expression10Context.class);
}
public Expression10Context expression10(int i) {
return getRuleContext(Expression10Context.class,i);
}
public List XOR() { return getTokens(Cypher6Parser.XOR); }
public TerminalNode XOR(int i) {
return getToken(Cypher6Parser.XOR, i);
}
public Expression11Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression11; }
}
public final Expression11Context expression11() throws RecognitionException {
Expression11Context _localctx = new Expression11Context(_ctx, getState());
enterRule(_localctx, 174, RULE_expression11);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1445);
expression10();
setState(1450);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==XOR) {
{
{
setState(1446);
match(XOR);
setState(1447);
expression10();
}
}
setState(1452);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression10Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List expression9() {
return getRuleContexts(Expression9Context.class);
}
public Expression9Context expression9(int i) {
return getRuleContext(Expression9Context.class,i);
}
public List AND() { return getTokens(Cypher6Parser.AND); }
public TerminalNode AND(int i) {
return getToken(Cypher6Parser.AND, i);
}
public Expression10Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression10; }
}
public final Expression10Context expression10() throws RecognitionException {
Expression10Context _localctx = new Expression10Context(_ctx, getState());
enterRule(_localctx, 176, RULE_expression10);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1453);
expression9();
setState(1458);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==AND) {
{
{
setState(1454);
match(AND);
setState(1455);
expression9();
}
}
setState(1460);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression9Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public Expression8Context expression8() {
return getRuleContext(Expression8Context.class,0);
}
public List NOT() { return getTokens(Cypher6Parser.NOT); }
public TerminalNode NOT(int i) {
return getToken(Cypher6Parser.NOT, i);
}
public Expression9Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression9; }
}
public final Expression9Context expression9() throws RecognitionException {
Expression9Context _localctx = new Expression9Context(_ctx, getState());
enterRule(_localctx, 178, RULE_expression9);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1464);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,132,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1461);
match(NOT);
}
}
}
setState(1466);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,132,_ctx);
}
setState(1467);
expression8();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression8Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List expression7() {
return getRuleContexts(Expression7Context.class);
}
public Expression7Context expression7(int i) {
return getRuleContext(Expression7Context.class,i);
}
public List EQ() { return getTokens(Cypher6Parser.EQ); }
public TerminalNode EQ(int i) {
return getToken(Cypher6Parser.EQ, i);
}
public List INVALID_NEQ() { return getTokens(Cypher6Parser.INVALID_NEQ); }
public TerminalNode INVALID_NEQ(int i) {
return getToken(Cypher6Parser.INVALID_NEQ, i);
}
public List NEQ() { return getTokens(Cypher6Parser.NEQ); }
public TerminalNode NEQ(int i) {
return getToken(Cypher6Parser.NEQ, i);
}
public List LE() { return getTokens(Cypher6Parser.LE); }
public TerminalNode LE(int i) {
return getToken(Cypher6Parser.LE, i);
}
public List GE() { return getTokens(Cypher6Parser.GE); }
public TerminalNode GE(int i) {
return getToken(Cypher6Parser.GE, i);
}
public List LT() { return getTokens(Cypher6Parser.LT); }
public TerminalNode LT(int i) {
return getToken(Cypher6Parser.LT, i);
}
public List GT() { return getTokens(Cypher6Parser.GT); }
public TerminalNode GT(int i) {
return getToken(Cypher6Parser.GT, i);
}
public Expression8Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression8; }
}
public final Expression8Context expression8() throws RecognitionException {
Expression8Context _localctx = new Expression8Context(_ctx, getState());
enterRule(_localctx, 180, RULE_expression8);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1469);
expression7();
setState(1474);
_errHandler.sync(this);
_la = _input.LA(1);
while (((((_la - 97)) & ~0x3f) == 0 && ((1L << (_la - 97)) & -9151032967823097855L) != 0) || _la==NEQ) {
{
{
setState(1470);
_la = _input.LA(1);
if ( !(((((_la - 97)) & ~0x3f) == 0 && ((1L << (_la - 97)) & -9151032967823097855L) != 0) || _la==NEQ) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1471);
expression7();
}
}
setState(1476);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression7Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public Expression6Context expression6() {
return getRuleContext(Expression6Context.class,0);
}
public ComparisonExpression6Context comparisonExpression6() {
return getRuleContext(ComparisonExpression6Context.class,0);
}
public Expression7Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression7; }
}
public final Expression7Context expression7() throws RecognitionException {
Expression7Context _localctx = new Expression7Context(_ctx, getState());
enterRule(_localctx, 182, RULE_expression7);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1477);
expression6();
setState(1479);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COLONCOLON || _la==CONTAINS || ((((_la - 96)) & ~0x3f) == 0 && ((1L << (_la - 96)) & 1103806595073L) != 0) || _la==REGEQ || _la==STARTS) {
{
setState(1478);
comparisonExpression6();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ComparisonExpression6Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ComparisonExpression6Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_comparisonExpression6; }
public ComparisonExpression6Context() { }
public void copyFrom(ComparisonExpression6Context ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class TypeComparisonContext extends ComparisonExpression6Context {
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public TerminalNode IS() { return getToken(Cypher6Parser.IS, 0); }
public TerminalNode COLONCOLON() { return getToken(Cypher6Parser.COLONCOLON, 0); }
public TerminalNode TYPED() { return getToken(Cypher6Parser.TYPED, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public TypeComparisonContext(ComparisonExpression6Context ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class StringAndListComparisonContext extends ComparisonExpression6Context {
public Expression6Context expression6() {
return getRuleContext(Expression6Context.class,0);
}
public TerminalNode REGEQ() { return getToken(Cypher6Parser.REGEQ, 0); }
public TerminalNode STARTS() { return getToken(Cypher6Parser.STARTS, 0); }
public TerminalNode WITH() { return getToken(Cypher6Parser.WITH, 0); }
public TerminalNode ENDS() { return getToken(Cypher6Parser.ENDS, 0); }
public TerminalNode CONTAINS() { return getToken(Cypher6Parser.CONTAINS, 0); }
public TerminalNode IN() { return getToken(Cypher6Parser.IN, 0); }
public StringAndListComparisonContext(ComparisonExpression6Context ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class NormalFormComparisonContext extends ComparisonExpression6Context {
public TerminalNode IS() { return getToken(Cypher6Parser.IS, 0); }
public TerminalNode NORMALIZED() { return getToken(Cypher6Parser.NORMALIZED, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public NormalFormContext normalForm() {
return getRuleContext(NormalFormContext.class,0);
}
public NormalFormComparisonContext(ComparisonExpression6Context ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class NullComparisonContext extends ComparisonExpression6Context {
public TerminalNode IS() { return getToken(Cypher6Parser.IS, 0); }
public TerminalNode NULL() { return getToken(Cypher6Parser.NULL, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public NullComparisonContext(ComparisonExpression6Context ctx) { copyFrom(ctx); }
}
public final ComparisonExpression6Context comparisonExpression6() throws RecognitionException {
ComparisonExpression6Context _localctx = new ComparisonExpression6Context(_ctx, getState());
enterRule(_localctx, 184, RULE_comparisonExpression6);
int _la;
try {
setState(1513);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,141,_ctx) ) {
case 1:
_localctx = new StringAndListComparisonContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(1488);
_errHandler.sync(this);
switch (_input.LA(1)) {
case REGEQ:
{
setState(1481);
match(REGEQ);
}
break;
case STARTS:
{
setState(1482);
match(STARTS);
setState(1483);
match(WITH);
}
break;
case ENDS:
{
setState(1484);
match(ENDS);
setState(1485);
match(WITH);
}
break;
case CONTAINS:
{
setState(1486);
match(CONTAINS);
}
break;
case IN:
{
setState(1487);
match(IN);
}
break;
default:
throw new NoViableAltException(this);
}
setState(1490);
expression6();
}
break;
case 2:
_localctx = new NullComparisonContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(1491);
match(IS);
setState(1493);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==NOT) {
{
setState(1492);
match(NOT);
}
}
setState(1495);
match(NULL);
}
break;
case 3:
_localctx = new TypeComparisonContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(1502);
_errHandler.sync(this);
switch (_input.LA(1)) {
case IS:
{
setState(1496);
match(IS);
setState(1498);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==NOT) {
{
setState(1497);
match(NOT);
}
}
setState(1500);
_la = _input.LA(1);
if ( !(_la==COLONCOLON || _la==TYPED) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
case COLONCOLON:
{
setState(1501);
match(COLONCOLON);
}
break;
default:
throw new NoViableAltException(this);
}
setState(1504);
type();
}
break;
case 4:
_localctx = new NormalFormComparisonContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(1505);
match(IS);
setState(1507);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==NOT) {
{
setState(1506);
match(NOT);
}
}
setState(1510);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 165)) & ~0x3f) == 0 && ((1L << (_la - 165)) & 15L) != 0)) {
{
setState(1509);
normalForm();
}
}
setState(1512);
match(NORMALIZED);
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NormalFormContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode NFC() { return getToken(Cypher6Parser.NFC, 0); }
public TerminalNode NFD() { return getToken(Cypher6Parser.NFD, 0); }
public TerminalNode NFKC() { return getToken(Cypher6Parser.NFKC, 0); }
public TerminalNode NFKD() { return getToken(Cypher6Parser.NFKD, 0); }
public NormalFormContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_normalForm; }
}
public final NormalFormContext normalForm() throws RecognitionException {
NormalFormContext _localctx = new NormalFormContext(_ctx, getState());
enterRule(_localctx, 186, RULE_normalForm);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1515);
_la = _input.LA(1);
if ( !(((((_la - 165)) & ~0x3f) == 0 && ((1L << (_la - 165)) & 15L) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression6Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List expression5() {
return getRuleContexts(Expression5Context.class);
}
public Expression5Context expression5(int i) {
return getRuleContext(Expression5Context.class,i);
}
public List PLUS() { return getTokens(Cypher6Parser.PLUS); }
public TerminalNode PLUS(int i) {
return getToken(Cypher6Parser.PLUS, i);
}
public List MINUS() { return getTokens(Cypher6Parser.MINUS); }
public TerminalNode MINUS(int i) {
return getToken(Cypher6Parser.MINUS, i);
}
public List DOUBLEBAR() { return getTokens(Cypher6Parser.DOUBLEBAR); }
public TerminalNode DOUBLEBAR(int i) {
return getToken(Cypher6Parser.DOUBLEBAR, i);
}
public Expression6Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression6; }
}
public final Expression6Context expression6() throws RecognitionException {
Expression6Context _localctx = new Expression6Context(_ctx, getState());
enterRule(_localctx, 188, RULE_expression6);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1517);
expression5();
setState(1522);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==DOUBLEBAR || _la==MINUS || _la==PLUS) {
{
{
setState(1518);
_la = _input.LA(1);
if ( !(_la==DOUBLEBAR || _la==MINUS || _la==PLUS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1519);
expression5();
}
}
setState(1524);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression5Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List expression4() {
return getRuleContexts(Expression4Context.class);
}
public Expression4Context expression4(int i) {
return getRuleContext(Expression4Context.class,i);
}
public List TIMES() { return getTokens(Cypher6Parser.TIMES); }
public TerminalNode TIMES(int i) {
return getToken(Cypher6Parser.TIMES, i);
}
public List DIVIDE() { return getTokens(Cypher6Parser.DIVIDE); }
public TerminalNode DIVIDE(int i) {
return getToken(Cypher6Parser.DIVIDE, i);
}
public List PERCENT() { return getTokens(Cypher6Parser.PERCENT); }
public TerminalNode PERCENT(int i) {
return getToken(Cypher6Parser.PERCENT, i);
}
public Expression5Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression5; }
}
public final Expression5Context expression5() throws RecognitionException {
Expression5Context _localctx = new Expression5Context(_ctx, getState());
enterRule(_localctx, 190, RULE_expression5);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1525);
expression4();
setState(1530);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==DIVIDE || _la==PERCENT || _la==TIMES) {
{
{
setState(1526);
_la = _input.LA(1);
if ( !(_la==DIVIDE || _la==PERCENT || _la==TIMES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1527);
expression4();
}
}
setState(1532);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression4Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List expression3() {
return getRuleContexts(Expression3Context.class);
}
public Expression3Context expression3(int i) {
return getRuleContext(Expression3Context.class,i);
}
public List POW() { return getTokens(Cypher6Parser.POW); }
public TerminalNode POW(int i) {
return getToken(Cypher6Parser.POW, i);
}
public Expression4Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression4; }
}
public final Expression4Context expression4() throws RecognitionException {
Expression4Context _localctx = new Expression4Context(_ctx, getState());
enterRule(_localctx, 192, RULE_expression4);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1533);
expression3();
setState(1538);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==POW) {
{
{
setState(1534);
match(POW);
setState(1535);
expression3();
}
}
setState(1540);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression3Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public Expression2Context expression2() {
return getRuleContext(Expression2Context.class,0);
}
public TerminalNode PLUS() { return getToken(Cypher6Parser.PLUS, 0); }
public TerminalNode MINUS() { return getToken(Cypher6Parser.MINUS, 0); }
public Expression3Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression3; }
}
public final Expression3Context expression3() throws RecognitionException {
Expression3Context _localctx = new Expression3Context(_ctx, getState());
enterRule(_localctx, 194, RULE_expression3);
int _la;
try {
setState(1544);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,145,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(1541);
expression2();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(1542);
_la = _input.LA(1);
if ( !(_la==MINUS || _la==PLUS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1543);
expression2();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression2Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public Expression1Context expression1() {
return getRuleContext(Expression1Context.class,0);
}
public List postFix() {
return getRuleContexts(PostFixContext.class);
}
public PostFixContext postFix(int i) {
return getRuleContext(PostFixContext.class,i);
}
public Expression2Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression2; }
}
public final Expression2Context expression2() throws RecognitionException {
Expression2Context _localctx = new Expression2Context(_ctx, getState());
enterRule(_localctx, 196, RULE_expression2);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1546);
expression1();
setState(1550);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,146,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1547);
postFix();
}
}
}
setState(1552);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,146,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PostFixContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public PostFixContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_postFix; }
public PostFixContext() { }
public void copyFrom(PostFixContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class IndexPostfixContext extends PostFixContext {
public TerminalNode LBRACKET() { return getToken(Cypher6Parser.LBRACKET, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode RBRACKET() { return getToken(Cypher6Parser.RBRACKET, 0); }
public IndexPostfixContext(PostFixContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class PropertyPostfixContext extends PostFixContext {
public PropertyContext property() {
return getRuleContext(PropertyContext.class,0);
}
public PropertyPostfixContext(PostFixContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class LabelPostfixContext extends PostFixContext {
public LabelExpressionContext labelExpression() {
return getRuleContext(LabelExpressionContext.class,0);
}
public LabelPostfixContext(PostFixContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class RangePostfixContext extends PostFixContext {
public ExpressionContext fromExp;
public ExpressionContext toExp;
public TerminalNode LBRACKET() { return getToken(Cypher6Parser.LBRACKET, 0); }
public TerminalNode DOTDOT() { return getToken(Cypher6Parser.DOTDOT, 0); }
public TerminalNode RBRACKET() { return getToken(Cypher6Parser.RBRACKET, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public RangePostfixContext(PostFixContext ctx) { copyFrom(ctx); }
}
public final PostFixContext postFix() throws RecognitionException {
PostFixContext _localctx = new PostFixContext(_ctx, getState());
enterRule(_localctx, 198, RULE_postFix);
int _la;
try {
setState(1568);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,149,_ctx) ) {
case 1:
_localctx = new PropertyPostfixContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(1553);
property();
}
break;
case 2:
_localctx = new LabelPostfixContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(1554);
labelExpression();
}
break;
case 3:
_localctx = new IndexPostfixContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(1555);
match(LBRACKET);
setState(1556);
expression();
setState(1557);
match(RBRACKET);
}
break;
case 4:
_localctx = new RangePostfixContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(1559);
match(LBRACKET);
setState(1561);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -246291141492752L) != 0) || ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -146366996479967233L) != 0) || ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -15066095617L) != 0) || ((((_la - 192)) & ~0x3f) == 0 && ((1L << (_la - 192)) & -1130297988612169L) != 0) || ((((_la - 256)) & ~0x3f) == 0 && ((1L << (_la - 256)) & 281474976709631L) != 0)) {
{
setState(1560);
((RangePostfixContext)_localctx).fromExp = expression();
}
}
setState(1563);
match(DOTDOT);
setState(1565);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -246291141492752L) != 0) || ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -146366996479967233L) != 0) || ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -15066095617L) != 0) || ((((_la - 192)) & ~0x3f) == 0 && ((1L << (_la - 192)) & -1130297988612169L) != 0) || ((((_la - 256)) & ~0x3f) == 0 && ((1L << (_la - 256)) & 281474976709631L) != 0)) {
{
setState(1564);
((RangePostfixContext)_localctx).toExp = expression();
}
}
setState(1567);
match(RBRACKET);
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PropertyContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DOT() { return getToken(Cypher6Parser.DOT, 0); }
public PropertyKeyNameContext propertyKeyName() {
return getRuleContext(PropertyKeyNameContext.class,0);
}
public PropertyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_property; }
}
public final PropertyContext property() throws RecognitionException {
PropertyContext _localctx = new PropertyContext(_ctx, getState());
enterRule(_localctx, 200, RULE_property);
try {
enterOuterAlt(_localctx, 1);
{
setState(1570);
match(DOT);
setState(1571);
propertyKeyName();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DynamicPropertyContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LBRACKET() { return getToken(Cypher6Parser.LBRACKET, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode RBRACKET() { return getToken(Cypher6Parser.RBRACKET, 0); }
public DynamicPropertyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dynamicProperty; }
}
public final DynamicPropertyContext dynamicProperty() throws RecognitionException {
DynamicPropertyContext _localctx = new DynamicPropertyContext(_ctx, getState());
enterRule(_localctx, 202, RULE_dynamicProperty);
try {
enterOuterAlt(_localctx, 1);
{
setState(1573);
match(LBRACKET);
setState(1574);
expression();
setState(1575);
match(RBRACKET);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PropertyExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public Expression1Context expression1() {
return getRuleContext(Expression1Context.class,0);
}
public List property() {
return getRuleContexts(PropertyContext.class);
}
public PropertyContext property(int i) {
return getRuleContext(PropertyContext.class,i);
}
public PropertyExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_propertyExpression; }
}
public final PropertyExpressionContext propertyExpression() throws RecognitionException {
PropertyExpressionContext _localctx = new PropertyExpressionContext(_ctx, getState());
enterRule(_localctx, 204, RULE_propertyExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1577);
expression1();
setState(1579);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(1578);
property();
}
}
setState(1581);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==DOT );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DynamicPropertyExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public Expression1Context expression1() {
return getRuleContext(Expression1Context.class,0);
}
public DynamicPropertyContext dynamicProperty() {
return getRuleContext(DynamicPropertyContext.class,0);
}
public DynamicPropertyExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dynamicPropertyExpression; }
}
public final DynamicPropertyExpressionContext dynamicPropertyExpression() throws RecognitionException {
DynamicPropertyExpressionContext _localctx = new DynamicPropertyExpressionContext(_ctx, getState());
enterRule(_localctx, 206, RULE_dynamicPropertyExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(1583);
expression1();
setState(1584);
dynamicProperty();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression1Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public LiteralContext literal() {
return getRuleContext(LiteralContext.class,0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class,0);
}
public CaseExpressionContext caseExpression() {
return getRuleContext(CaseExpressionContext.class,0);
}
public ExtendedCaseExpressionContext extendedCaseExpression() {
return getRuleContext(ExtendedCaseExpressionContext.class,0);
}
public CountStarContext countStar() {
return getRuleContext(CountStarContext.class,0);
}
public ExistsExpressionContext existsExpression() {
return getRuleContext(ExistsExpressionContext.class,0);
}
public CountExpressionContext countExpression() {
return getRuleContext(CountExpressionContext.class,0);
}
public CollectExpressionContext collectExpression() {
return getRuleContext(CollectExpressionContext.class,0);
}
public MapProjectionContext mapProjection() {
return getRuleContext(MapProjectionContext.class,0);
}
public ListComprehensionContext listComprehension() {
return getRuleContext(ListComprehensionContext.class,0);
}
public ListLiteralContext listLiteral() {
return getRuleContext(ListLiteralContext.class,0);
}
public PatternComprehensionContext patternComprehension() {
return getRuleContext(PatternComprehensionContext.class,0);
}
public ReduceExpressionContext reduceExpression() {
return getRuleContext(ReduceExpressionContext.class,0);
}
public ListItemsPredicateContext listItemsPredicate() {
return getRuleContext(ListItemsPredicateContext.class,0);
}
public NormalizeFunctionContext normalizeFunction() {
return getRuleContext(NormalizeFunctionContext.class,0);
}
public TrimFunctionContext trimFunction() {
return getRuleContext(TrimFunctionContext.class,0);
}
public PatternExpressionContext patternExpression() {
return getRuleContext(PatternExpressionContext.class,0);
}
public ShortestPathExpressionContext shortestPathExpression() {
return getRuleContext(ShortestPathExpressionContext.class,0);
}
public ParenthesizedExpressionContext parenthesizedExpression() {
return getRuleContext(ParenthesizedExpressionContext.class,0);
}
public FunctionInvocationContext functionInvocation() {
return getRuleContext(FunctionInvocationContext.class,0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public Expression1Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression1; }
}
public final Expression1Context expression1() throws RecognitionException {
Expression1Context _localctx = new Expression1Context(_ctx, getState());
enterRule(_localctx, 208, RULE_expression1);
try {
setState(1607);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,151,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(1586);
literal();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(1587);
parameter("ANY");
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(1588);
caseExpression();
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(1589);
extendedCaseExpression();
}
break;
case 5:
enterOuterAlt(_localctx, 5);
{
setState(1590);
countStar();
}
break;
case 6:
enterOuterAlt(_localctx, 6);
{
setState(1591);
existsExpression();
}
break;
case 7:
enterOuterAlt(_localctx, 7);
{
setState(1592);
countExpression();
}
break;
case 8:
enterOuterAlt(_localctx, 8);
{
setState(1593);
collectExpression();
}
break;
case 9:
enterOuterAlt(_localctx, 9);
{
setState(1594);
mapProjection();
}
break;
case 10:
enterOuterAlt(_localctx, 10);
{
setState(1595);
listComprehension();
}
break;
case 11:
enterOuterAlt(_localctx, 11);
{
setState(1596);
listLiteral();
}
break;
case 12:
enterOuterAlt(_localctx, 12);
{
setState(1597);
patternComprehension();
}
break;
case 13:
enterOuterAlt(_localctx, 13);
{
setState(1598);
reduceExpression();
}
break;
case 14:
enterOuterAlt(_localctx, 14);
{
setState(1599);
listItemsPredicate();
}
break;
case 15:
enterOuterAlt(_localctx, 15);
{
setState(1600);
normalizeFunction();
}
break;
case 16:
enterOuterAlt(_localctx, 16);
{
setState(1601);
trimFunction();
}
break;
case 17:
enterOuterAlt(_localctx, 17);
{
setState(1602);
patternExpression();
}
break;
case 18:
enterOuterAlt(_localctx, 18);
{
setState(1603);
shortestPathExpression();
}
break;
case 19:
enterOuterAlt(_localctx, 19);
{
setState(1604);
parenthesizedExpression();
}
break;
case 20:
enterOuterAlt(_localctx, 20);
{
setState(1605);
functionInvocation();
}
break;
case 21:
enterOuterAlt(_localctx, 21);
{
setState(1606);
variable();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LiteralContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public LiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_literal; }
public LiteralContext() { }
public void copyFrom(LiteralContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class NummericLiteralContext extends LiteralContext {
public NumberLiteralContext numberLiteral() {
return getRuleContext(NumberLiteralContext.class,0);
}
public NummericLiteralContext(LiteralContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class BooleanLiteralContext extends LiteralContext {
public TerminalNode TRUE() { return getToken(Cypher6Parser.TRUE, 0); }
public TerminalNode FALSE() { return getToken(Cypher6Parser.FALSE, 0); }
public BooleanLiteralContext(LiteralContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class KeywordLiteralContext extends LiteralContext {
public TerminalNode INF() { return getToken(Cypher6Parser.INF, 0); }
public TerminalNode INFINITY() { return getToken(Cypher6Parser.INFINITY, 0); }
public TerminalNode NAN() { return getToken(Cypher6Parser.NAN, 0); }
public TerminalNode NULL() { return getToken(Cypher6Parser.NULL, 0); }
public KeywordLiteralContext(LiteralContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class OtherLiteralContext extends LiteralContext {
public MapContext map() {
return getRuleContext(MapContext.class,0);
}
public OtherLiteralContext(LiteralContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class StringsLiteralContext extends LiteralContext {
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public StringsLiteralContext(LiteralContext ctx) { copyFrom(ctx); }
}
public final LiteralContext literal() throws RecognitionException {
LiteralContext _localctx = new LiteralContext(_ctx, getState());
enterRule(_localctx, 210, RULE_literal);
try {
setState(1618);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DECIMAL_DOUBLE:
case UNSIGNED_DECIMAL_INTEGER:
case UNSIGNED_HEX_INTEGER:
case UNSIGNED_OCTAL_INTEGER:
case MINUS:
_localctx = new NummericLiteralContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(1609);
numberLiteral();
}
break;
case STRING_LITERAL1:
case STRING_LITERAL2:
_localctx = new StringsLiteralContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(1610);
stringLiteral();
}
break;
case LCURLY:
_localctx = new OtherLiteralContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(1611);
map();
}
break;
case TRUE:
_localctx = new BooleanLiteralContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(1612);
match(TRUE);
}
break;
case FALSE:
_localctx = new BooleanLiteralContext(_localctx);
enterOuterAlt(_localctx, 5);
{
setState(1613);
match(FALSE);
}
break;
case INF:
_localctx = new KeywordLiteralContext(_localctx);
enterOuterAlt(_localctx, 6);
{
setState(1614);
match(INF);
}
break;
case INFINITY:
_localctx = new KeywordLiteralContext(_localctx);
enterOuterAlt(_localctx, 7);
{
setState(1615);
match(INFINITY);
}
break;
case NAN:
_localctx = new KeywordLiteralContext(_localctx);
enterOuterAlt(_localctx, 8);
{
setState(1616);
match(NAN);
}
break;
case NULL:
_localctx = new KeywordLiteralContext(_localctx);
enterOuterAlt(_localctx, 9);
{
setState(1617);
match(NULL);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CaseExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CASE() { return getToken(Cypher6Parser.CASE, 0); }
public TerminalNode END() { return getToken(Cypher6Parser.END, 0); }
public List caseAlternative() {
return getRuleContexts(CaseAlternativeContext.class);
}
public CaseAlternativeContext caseAlternative(int i) {
return getRuleContext(CaseAlternativeContext.class,i);
}
public TerminalNode ELSE() { return getToken(Cypher6Parser.ELSE, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public CaseExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_caseExpression; }
}
public final CaseExpressionContext caseExpression() throws RecognitionException {
CaseExpressionContext _localctx = new CaseExpressionContext(_ctx, getState());
enterRule(_localctx, 212, RULE_caseExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1620);
match(CASE);
setState(1622);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(1621);
caseAlternative();
}
}
setState(1624);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==WHEN );
setState(1628);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==ELSE) {
{
setState(1626);
match(ELSE);
setState(1627);
expression();
}
}
setState(1630);
match(END);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CaseAlternativeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode WHEN() { return getToken(Cypher6Parser.WHEN, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public TerminalNode THEN() { return getToken(Cypher6Parser.THEN, 0); }
public CaseAlternativeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_caseAlternative; }
}
public final CaseAlternativeContext caseAlternative() throws RecognitionException {
CaseAlternativeContext _localctx = new CaseAlternativeContext(_ctx, getState());
enterRule(_localctx, 214, RULE_caseAlternative);
try {
enterOuterAlt(_localctx, 1);
{
setState(1632);
match(WHEN);
setState(1633);
expression();
setState(1634);
match(THEN);
setState(1635);
expression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExtendedCaseExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext elseExp;
public TerminalNode CASE() { return getToken(Cypher6Parser.CASE, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public TerminalNode END() { return getToken(Cypher6Parser.END, 0); }
public List extendedCaseAlternative() {
return getRuleContexts(ExtendedCaseAlternativeContext.class);
}
public ExtendedCaseAlternativeContext extendedCaseAlternative(int i) {
return getRuleContext(ExtendedCaseAlternativeContext.class,i);
}
public TerminalNode ELSE() { return getToken(Cypher6Parser.ELSE, 0); }
public ExtendedCaseExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_extendedCaseExpression; }
}
public final ExtendedCaseExpressionContext extendedCaseExpression() throws RecognitionException {
ExtendedCaseExpressionContext _localctx = new ExtendedCaseExpressionContext(_ctx, getState());
enterRule(_localctx, 216, RULE_extendedCaseExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1637);
match(CASE);
setState(1638);
expression();
setState(1640);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(1639);
extendedCaseAlternative();
}
}
setState(1642);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==WHEN );
setState(1646);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==ELSE) {
{
setState(1644);
match(ELSE);
setState(1645);
((ExtendedCaseExpressionContext)_localctx).elseExp = expression();
}
}
setState(1648);
match(END);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExtendedCaseAlternativeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode WHEN() { return getToken(Cypher6Parser.WHEN, 0); }
public List extendedWhen() {
return getRuleContexts(ExtendedWhenContext.class);
}
public ExtendedWhenContext extendedWhen(int i) {
return getRuleContext(ExtendedWhenContext.class,i);
}
public TerminalNode THEN() { return getToken(Cypher6Parser.THEN, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public ExtendedCaseAlternativeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_extendedCaseAlternative; }
}
public final ExtendedCaseAlternativeContext extendedCaseAlternative() throws RecognitionException {
ExtendedCaseAlternativeContext _localctx = new ExtendedCaseAlternativeContext(_ctx, getState());
enterRule(_localctx, 218, RULE_extendedCaseAlternative);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1650);
match(WHEN);
setState(1651);
extendedWhen();
setState(1656);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(1652);
match(COMMA);
setState(1653);
extendedWhen();
}
}
setState(1658);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1659);
match(THEN);
setState(1660);
expression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExtendedWhenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExtendedWhenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_extendedWhen; }
public ExtendedWhenContext() { }
public void copyFrom(ExtendedWhenContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class WhenStringOrListContext extends ExtendedWhenContext {
public Expression6Context expression6() {
return getRuleContext(Expression6Context.class,0);
}
public TerminalNode REGEQ() { return getToken(Cypher6Parser.REGEQ, 0); }
public TerminalNode STARTS() { return getToken(Cypher6Parser.STARTS, 0); }
public TerminalNode WITH() { return getToken(Cypher6Parser.WITH, 0); }
public TerminalNode ENDS() { return getToken(Cypher6Parser.ENDS, 0); }
public WhenStringOrListContext(ExtendedWhenContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class WhenTypeContext extends ExtendedWhenContext {
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public TerminalNode IS() { return getToken(Cypher6Parser.IS, 0); }
public TerminalNode TYPED() { return getToken(Cypher6Parser.TYPED, 0); }
public TerminalNode COLONCOLON() { return getToken(Cypher6Parser.COLONCOLON, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public WhenTypeContext(ExtendedWhenContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class WhenFormContext extends ExtendedWhenContext {
public TerminalNode IS() { return getToken(Cypher6Parser.IS, 0); }
public TerminalNode NORMALIZED() { return getToken(Cypher6Parser.NORMALIZED, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public NormalFormContext normalForm() {
return getRuleContext(NormalFormContext.class,0);
}
public WhenFormContext(ExtendedWhenContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class WhenNullContext extends ExtendedWhenContext {
public TerminalNode IS() { return getToken(Cypher6Parser.IS, 0); }
public TerminalNode NULL() { return getToken(Cypher6Parser.NULL, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public WhenNullContext(ExtendedWhenContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class WhenEqualsContext extends ExtendedWhenContext {
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public WhenEqualsContext(ExtendedWhenContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class WhenComparatorContext extends ExtendedWhenContext {
public Expression7Context expression7() {
return getRuleContext(Expression7Context.class,0);
}
public TerminalNode EQ() { return getToken(Cypher6Parser.EQ, 0); }
public TerminalNode NEQ() { return getToken(Cypher6Parser.NEQ, 0); }
public TerminalNode INVALID_NEQ() { return getToken(Cypher6Parser.INVALID_NEQ, 0); }
public TerminalNode LE() { return getToken(Cypher6Parser.LE, 0); }
public TerminalNode GE() { return getToken(Cypher6Parser.GE, 0); }
public TerminalNode LT() { return getToken(Cypher6Parser.LT, 0); }
public TerminalNode GT() { return getToken(Cypher6Parser.GT, 0); }
public WhenComparatorContext(ExtendedWhenContext ctx) { copyFrom(ctx); }
}
public final ExtendedWhenContext extendedWhen() throws RecognitionException {
ExtendedWhenContext _localctx = new ExtendedWhenContext(_ctx, getState());
enterRule(_localctx, 220, RULE_extendedWhen);
int _la;
try {
setState(1695);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,164,_ctx) ) {
case 1:
_localctx = new WhenStringOrListContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(1667);
_errHandler.sync(this);
switch (_input.LA(1)) {
case REGEQ:
{
setState(1662);
match(REGEQ);
}
break;
case STARTS:
{
setState(1663);
match(STARTS);
setState(1664);
match(WITH);
}
break;
case ENDS:
{
setState(1665);
match(ENDS);
setState(1666);
match(WITH);
}
break;
default:
throw new NoViableAltException(this);
}
setState(1669);
expression6();
}
break;
case 2:
_localctx = new WhenNullContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(1670);
match(IS);
setState(1672);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==NOT) {
{
setState(1671);
match(NOT);
}
}
setState(1674);
match(NULL);
}
break;
case 3:
_localctx = new WhenTypeContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(1681);
_errHandler.sync(this);
switch (_input.LA(1)) {
case IS:
{
setState(1675);
match(IS);
setState(1677);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==NOT) {
{
setState(1676);
match(NOT);
}
}
setState(1679);
match(TYPED);
}
break;
case COLONCOLON:
{
setState(1680);
match(COLONCOLON);
}
break;
default:
throw new NoViableAltException(this);
}
setState(1683);
type();
}
break;
case 4:
_localctx = new WhenFormContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(1684);
match(IS);
setState(1686);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==NOT) {
{
setState(1685);
match(NOT);
}
}
setState(1689);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 165)) & ~0x3f) == 0 && ((1L << (_la - 165)) & 15L) != 0)) {
{
setState(1688);
normalForm();
}
}
setState(1691);
match(NORMALIZED);
}
break;
case 5:
_localctx = new WhenComparatorContext(_localctx);
enterOuterAlt(_localctx, 5);
{
setState(1692);
_la = _input.LA(1);
if ( !(((((_la - 97)) & ~0x3f) == 0 && ((1L << (_la - 97)) & -9151032967823097855L) != 0) || _la==NEQ) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1693);
expression7();
}
break;
case 6:
_localctx = new WhenEqualsContext(_localctx);
enterOuterAlt(_localctx, 6);
{
setState(1694);
expression();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ListComprehensionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext whereExp;
public ExpressionContext barExp;
public TerminalNode LBRACKET() { return getToken(Cypher6Parser.LBRACKET, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public TerminalNode IN() { return getToken(Cypher6Parser.IN, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public TerminalNode RBRACKET() { return getToken(Cypher6Parser.RBRACKET, 0); }
public TerminalNode BAR() { return getToken(Cypher6Parser.BAR, 0); }
public TerminalNode WHERE() { return getToken(Cypher6Parser.WHERE, 0); }
public ListComprehensionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_listComprehension; }
}
public final ListComprehensionContext listComprehension() throws RecognitionException {
ListComprehensionContext _localctx = new ListComprehensionContext(_ctx, getState());
enterRule(_localctx, 222, RULE_listComprehension);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1697);
match(LBRACKET);
setState(1698);
variable();
setState(1699);
match(IN);
setState(1700);
expression();
setState(1711);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,167,_ctx) ) {
case 1:
{
setState(1703);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE) {
{
setState(1701);
match(WHERE);
setState(1702);
((ListComprehensionContext)_localctx).whereExp = expression();
}
}
setState(1705);
match(BAR);
setState(1706);
((ListComprehensionContext)_localctx).barExp = expression();
}
break;
case 2:
{
setState(1709);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE) {
{
setState(1707);
match(WHERE);
setState(1708);
((ListComprehensionContext)_localctx).whereExp = expression();
}
}
}
break;
}
setState(1713);
match(RBRACKET);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PatternComprehensionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext whereExp;
public ExpressionContext barExp;
public TerminalNode LBRACKET() { return getToken(Cypher6Parser.LBRACKET, 0); }
public PathPatternNonEmptyContext pathPatternNonEmpty() {
return getRuleContext(PathPatternNonEmptyContext.class,0);
}
public TerminalNode BAR() { return getToken(Cypher6Parser.BAR, 0); }
public TerminalNode RBRACKET() { return getToken(Cypher6Parser.RBRACKET, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public TerminalNode EQ() { return getToken(Cypher6Parser.EQ, 0); }
public TerminalNode WHERE() { return getToken(Cypher6Parser.WHERE, 0); }
public PatternComprehensionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_patternComprehension; }
}
public final PatternComprehensionContext patternComprehension() throws RecognitionException {
PatternComprehensionContext _localctx = new PatternComprehensionContext(_ctx, getState());
enterRule(_localctx, 224, RULE_patternComprehension);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1715);
match(LBRACKET);
setState(1719);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -246291141493760L) != 0) || ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -146366996479975425L) != 0) || ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -16156712961L) != 0) || ((((_la - 192)) & ~0x3f) == 0 && ((1L << (_la - 192)) & -1130297988612173L) != 0) || ((((_la - 256)) & ~0x3f) == 0 && ((1L << (_la - 256)) & 281474976709631L) != 0)) {
{
setState(1716);
variable();
setState(1717);
match(EQ);
}
}
setState(1721);
pathPatternNonEmpty();
setState(1724);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE) {
{
setState(1722);
match(WHERE);
setState(1723);
((PatternComprehensionContext)_localctx).whereExp = expression();
}
}
setState(1726);
match(BAR);
setState(1727);
((PatternComprehensionContext)_localctx).barExp = expression();
setState(1728);
match(RBRACKET);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ReduceExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode REDUCE() { return getToken(Cypher6Parser.REDUCE, 0); }
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public List variable() {
return getRuleContexts(VariableContext.class);
}
public VariableContext variable(int i) {
return getRuleContext(VariableContext.class,i);
}
public TerminalNode EQ() { return getToken(Cypher6Parser.EQ, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public TerminalNode COMMA() { return getToken(Cypher6Parser.COMMA, 0); }
public TerminalNode IN() { return getToken(Cypher6Parser.IN, 0); }
public TerminalNode BAR() { return getToken(Cypher6Parser.BAR, 0); }
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public ReduceExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_reduceExpression; }
}
public final ReduceExpressionContext reduceExpression() throws RecognitionException {
ReduceExpressionContext _localctx = new ReduceExpressionContext(_ctx, getState());
enterRule(_localctx, 226, RULE_reduceExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(1730);
match(REDUCE);
setState(1731);
match(LPAREN);
setState(1732);
variable();
setState(1733);
match(EQ);
setState(1734);
expression();
setState(1735);
match(COMMA);
setState(1736);
variable();
setState(1737);
match(IN);
setState(1738);
expression();
setState(1739);
match(BAR);
setState(1740);
expression();
setState(1741);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ListItemsPredicateContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext inExp;
public ExpressionContext whereExp;
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public TerminalNode IN() { return getToken(Cypher6Parser.IN, 0); }
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public TerminalNode ALL() { return getToken(Cypher6Parser.ALL, 0); }
public TerminalNode ANY() { return getToken(Cypher6Parser.ANY, 0); }
public TerminalNode NONE() { return getToken(Cypher6Parser.NONE, 0); }
public TerminalNode SINGLE() { return getToken(Cypher6Parser.SINGLE, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public TerminalNode WHERE() { return getToken(Cypher6Parser.WHERE, 0); }
public ListItemsPredicateContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_listItemsPredicate; }
}
public final ListItemsPredicateContext listItemsPredicate() throws RecognitionException {
ListItemsPredicateContext _localctx = new ListItemsPredicateContext(_ctx, getState());
enterRule(_localctx, 228, RULE_listItemsPredicate);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1743);
_la = _input.LA(1);
if ( !(_la==ALL || _la==ANY || _la==NONE || _la==SINGLE) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1744);
match(LPAREN);
setState(1745);
variable();
setState(1746);
match(IN);
setState(1747);
((ListItemsPredicateContext)_localctx).inExp = expression();
setState(1750);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE) {
{
setState(1748);
match(WHERE);
setState(1749);
((ListItemsPredicateContext)_localctx).whereExp = expression();
}
}
setState(1752);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NormalizeFunctionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode NORMALIZE() { return getToken(Cypher6Parser.NORMALIZE, 0); }
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public TerminalNode COMMA() { return getToken(Cypher6Parser.COMMA, 0); }
public NormalFormContext normalForm() {
return getRuleContext(NormalFormContext.class,0);
}
public NormalizeFunctionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_normalizeFunction; }
}
public final NormalizeFunctionContext normalizeFunction() throws RecognitionException {
NormalizeFunctionContext _localctx = new NormalizeFunctionContext(_ctx, getState());
enterRule(_localctx, 230, RULE_normalizeFunction);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1754);
match(NORMALIZE);
setState(1755);
match(LPAREN);
setState(1756);
expression();
setState(1759);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMMA) {
{
setState(1757);
match(COMMA);
setState(1758);
normalForm();
}
}
setState(1761);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TrimFunctionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext trimCharacterString;
public ExpressionContext trimSource;
public TerminalNode TRIM() { return getToken(Cypher6Parser.TRIM, 0); }
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public TerminalNode FROM() { return getToken(Cypher6Parser.FROM, 0); }
public TerminalNode BOTH() { return getToken(Cypher6Parser.BOTH, 0); }
public TerminalNode LEADING() { return getToken(Cypher6Parser.LEADING, 0); }
public TerminalNode TRAILING() { return getToken(Cypher6Parser.TRAILING, 0); }
public TrimFunctionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_trimFunction; }
}
public final TrimFunctionContext trimFunction() throws RecognitionException {
TrimFunctionContext _localctx = new TrimFunctionContext(_ctx, getState());
enterRule(_localctx, 232, RULE_trimFunction);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1763);
match(TRIM);
setState(1764);
match(LPAREN);
setState(1772);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,174,_ctx) ) {
case 1:
{
setState(1766);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,172,_ctx) ) {
case 1:
{
setState(1765);
_la = _input.LA(1);
if ( !(_la==BOTH || _la==LEADING || _la==TRAILING) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
}
setState(1769);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,173,_ctx) ) {
case 1:
{
setState(1768);
((TrimFunctionContext)_localctx).trimCharacterString = expression();
}
break;
}
setState(1771);
match(FROM);
}
break;
}
setState(1774);
((TrimFunctionContext)_localctx).trimSource = expression();
setState(1775);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PatternExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public PathPatternNonEmptyContext pathPatternNonEmpty() {
return getRuleContext(PathPatternNonEmptyContext.class,0);
}
public PatternExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_patternExpression; }
}
public final PatternExpressionContext patternExpression() throws RecognitionException {
PatternExpressionContext _localctx = new PatternExpressionContext(_ctx, getState());
enterRule(_localctx, 234, RULE_patternExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(1777);
pathPatternNonEmpty();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShortestPathExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ShortestPathPatternContext shortestPathPattern() {
return getRuleContext(ShortestPathPatternContext.class,0);
}
public ShortestPathExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_shortestPathExpression; }
}
public final ShortestPathExpressionContext shortestPathExpression() throws RecognitionException {
ShortestPathExpressionContext _localctx = new ShortestPathExpressionContext(_ctx, getState());
enterRule(_localctx, 236, RULE_shortestPathExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(1779);
shortestPathPattern();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParenthesizedExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public ParenthesizedExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parenthesizedExpression; }
}
public final ParenthesizedExpressionContext parenthesizedExpression() throws RecognitionException {
ParenthesizedExpressionContext _localctx = new ParenthesizedExpressionContext(_ctx, getState());
enterRule(_localctx, 238, RULE_parenthesizedExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(1781);
match(LPAREN);
setState(1782);
expression();
setState(1783);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MapProjectionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public TerminalNode LCURLY() { return getToken(Cypher6Parser.LCURLY, 0); }
public TerminalNode RCURLY() { return getToken(Cypher6Parser.RCURLY, 0); }
public List mapProjectionElement() {
return getRuleContexts(MapProjectionElementContext.class);
}
public MapProjectionElementContext mapProjectionElement(int i) {
return getRuleContext(MapProjectionElementContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public MapProjectionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_mapProjection; }
}
public final MapProjectionContext mapProjection() throws RecognitionException {
MapProjectionContext _localctx = new MapProjectionContext(_ctx, getState());
enterRule(_localctx, 240, RULE_mapProjection);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1785);
variable();
setState(1786);
match(LCURLY);
setState(1795);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -246291141493760L) != 0) || ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -146366996479909889L) != 0) || ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -16156712961L) != 0) || ((((_la - 192)) & ~0x3f) == 0 && ((1L << (_la - 192)) & -1130297988612173L) != 0) || ((((_la - 256)) & ~0x3f) == 0 && ((1L << (_la - 256)) & 281474976709631L) != 0)) {
{
setState(1787);
mapProjectionElement();
setState(1792);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(1788);
match(COMMA);
setState(1789);
mapProjectionElement();
}
}
setState(1794);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(1797);
match(RCURLY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MapProjectionElementContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public PropertyKeyNameContext propertyKeyName() {
return getRuleContext(PropertyKeyNameContext.class,0);
}
public TerminalNode COLON() { return getToken(Cypher6Parser.COLON, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public PropertyContext property() {
return getRuleContext(PropertyContext.class,0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public TerminalNode DOT() { return getToken(Cypher6Parser.DOT, 0); }
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public MapProjectionElementContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_mapProjectionElement; }
}
public final MapProjectionElementContext mapProjectionElement() throws RecognitionException {
MapProjectionElementContext _localctx = new MapProjectionElementContext(_ctx, getState());
enterRule(_localctx, 242, RULE_mapProjectionElement);
try {
setState(1807);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,177,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(1799);
propertyKeyName();
setState(1800);
match(COLON);
setState(1801);
expression();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(1803);
property();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(1804);
variable();
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(1805);
match(DOT);
setState(1806);
match(TIMES);
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CountStarContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COUNT() { return getToken(Cypher6Parser.COUNT, 0); }
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public CountStarContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_countStar; }
}
public final CountStarContext countStar() throws RecognitionException {
CountStarContext _localctx = new CountStarContext(_ctx, getState());
enterRule(_localctx, 244, RULE_countStar);
try {
enterOuterAlt(_localctx, 1);
{
setState(1809);
match(COUNT);
setState(1810);
match(LPAREN);
setState(1811);
match(TIMES);
setState(1812);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExistsExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public TerminalNode LCURLY() { return getToken(Cypher6Parser.LCURLY, 0); }
public TerminalNode RCURLY() { return getToken(Cypher6Parser.RCURLY, 0); }
public RegularQueryContext regularQuery() {
return getRuleContext(RegularQueryContext.class,0);
}
public PatternListContext patternList() {
return getRuleContext(PatternListContext.class,0);
}
public MatchModeContext matchMode() {
return getRuleContext(MatchModeContext.class,0);
}
public WhereClauseContext whereClause() {
return getRuleContext(WhereClauseContext.class,0);
}
public ExistsExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_existsExpression; }
}
public final ExistsExpressionContext existsExpression() throws RecognitionException {
ExistsExpressionContext _localctx = new ExistsExpressionContext(_ctx, getState());
enterRule(_localctx, 246, RULE_existsExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1814);
match(EXISTS);
setState(1815);
match(LCURLY);
setState(1824);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,180,_ctx) ) {
case 1:
{
setState(1816);
regularQuery();
}
break;
case 2:
{
setState(1818);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,178,_ctx) ) {
case 1:
{
setState(1817);
matchMode();
}
break;
}
setState(1820);
patternList();
setState(1822);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE) {
{
setState(1821);
whereClause();
}
}
}
break;
}
setState(1826);
match(RCURLY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CountExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COUNT() { return getToken(Cypher6Parser.COUNT, 0); }
public TerminalNode LCURLY() { return getToken(Cypher6Parser.LCURLY, 0); }
public TerminalNode RCURLY() { return getToken(Cypher6Parser.RCURLY, 0); }
public RegularQueryContext regularQuery() {
return getRuleContext(RegularQueryContext.class,0);
}
public PatternListContext patternList() {
return getRuleContext(PatternListContext.class,0);
}
public MatchModeContext matchMode() {
return getRuleContext(MatchModeContext.class,0);
}
public WhereClauseContext whereClause() {
return getRuleContext(WhereClauseContext.class,0);
}
public CountExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_countExpression; }
}
public final CountExpressionContext countExpression() throws RecognitionException {
CountExpressionContext _localctx = new CountExpressionContext(_ctx, getState());
enterRule(_localctx, 248, RULE_countExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1828);
match(COUNT);
setState(1829);
match(LCURLY);
setState(1838);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,183,_ctx) ) {
case 1:
{
setState(1830);
regularQuery();
}
break;
case 2:
{
setState(1832);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,181,_ctx) ) {
case 1:
{
setState(1831);
matchMode();
}
break;
}
setState(1834);
patternList();
setState(1836);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE) {
{
setState(1835);
whereClause();
}
}
}
break;
}
setState(1840);
match(RCURLY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CollectExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COLLECT() { return getToken(Cypher6Parser.COLLECT, 0); }
public TerminalNode LCURLY() { return getToken(Cypher6Parser.LCURLY, 0); }
public RegularQueryContext regularQuery() {
return getRuleContext(RegularQueryContext.class,0);
}
public TerminalNode RCURLY() { return getToken(Cypher6Parser.RCURLY, 0); }
public CollectExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_collectExpression; }
}
public final CollectExpressionContext collectExpression() throws RecognitionException {
CollectExpressionContext _localctx = new CollectExpressionContext(_ctx, getState());
enterRule(_localctx, 250, RULE_collectExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(1842);
match(COLLECT);
setState(1843);
match(LCURLY);
setState(1844);
regularQuery();
setState(1845);
match(RCURLY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NumberLiteralContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DECIMAL_DOUBLE() { return getToken(Cypher6Parser.DECIMAL_DOUBLE, 0); }
public TerminalNode UNSIGNED_DECIMAL_INTEGER() { return getToken(Cypher6Parser.UNSIGNED_DECIMAL_INTEGER, 0); }
public TerminalNode UNSIGNED_HEX_INTEGER() { return getToken(Cypher6Parser.UNSIGNED_HEX_INTEGER, 0); }
public TerminalNode UNSIGNED_OCTAL_INTEGER() { return getToken(Cypher6Parser.UNSIGNED_OCTAL_INTEGER, 0); }
public TerminalNode MINUS() { return getToken(Cypher6Parser.MINUS, 0); }
public NumberLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_numberLiteral; }
}
public final NumberLiteralContext numberLiteral() throws RecognitionException {
NumberLiteralContext _localctx = new NumberLiteralContext(_ctx, getState());
enterRule(_localctx, 252, RULE_numberLiteral);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1848);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==MINUS) {
{
setState(1847);
match(MINUS);
}
}
setState(1850);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & 240L) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SignedIntegerLiteralContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode UNSIGNED_DECIMAL_INTEGER() { return getToken(Cypher6Parser.UNSIGNED_DECIMAL_INTEGER, 0); }
public TerminalNode MINUS() { return getToken(Cypher6Parser.MINUS, 0); }
public SignedIntegerLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_signedIntegerLiteral; }
}
public final SignedIntegerLiteralContext signedIntegerLiteral() throws RecognitionException {
SignedIntegerLiteralContext _localctx = new SignedIntegerLiteralContext(_ctx, getState());
enterRule(_localctx, 254, RULE_signedIntegerLiteral);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1853);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==MINUS) {
{
setState(1852);
match(MINUS);
}
}
setState(1855);
match(UNSIGNED_DECIMAL_INTEGER);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ListLiteralContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LBRACKET() { return getToken(Cypher6Parser.LBRACKET, 0); }
public TerminalNode RBRACKET() { return getToken(Cypher6Parser.RBRACKET, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public ListLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_listLiteral; }
}
public final ListLiteralContext listLiteral() throws RecognitionException {
ListLiteralContext _localctx = new ListLiteralContext(_ctx, getState());
enterRule(_localctx, 256, RULE_listLiteral);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1857);
match(LBRACKET);
setState(1866);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -246291141492752L) != 0) || ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -146366996479967233L) != 0) || ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -15066095617L) != 0) || ((((_la - 192)) & ~0x3f) == 0 && ((1L << (_la - 192)) & -1130297988612169L) != 0) || ((((_la - 256)) & ~0x3f) == 0 && ((1L << (_la - 256)) & 281474976709631L) != 0)) {
{
setState(1858);
expression();
setState(1863);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(1859);
match(COMMA);
setState(1860);
expression();
}
}
setState(1865);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(1868);
match(RBRACKET);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PropertyKeyNameContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public PropertyKeyNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_propertyKeyName; }
}
public final PropertyKeyNameContext propertyKeyName() throws RecognitionException {
PropertyKeyNameContext _localctx = new PropertyKeyNameContext(_ctx, getState());
enterRule(_localctx, 258, RULE_propertyKeyName);
try {
enterOuterAlt(_localctx, 1);
{
setState(1870);
symbolicNameString();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParameterContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public String paramType;
public TerminalNode DOLLAR() { return getToken(Cypher6Parser.DOLLAR, 0); }
public ParameterNameContext parameterName() {
return getRuleContext(ParameterNameContext.class,0);
}
public ParameterContext(ParserRuleContext parent, int invokingState) { super(parent, invokingState); }
public ParameterContext(ParserRuleContext parent, int invokingState, String paramType) {
super(parent, invokingState);
this.paramType = paramType;
}
@Override public int getRuleIndex() { return RULE_parameter; }
}
public final ParameterContext parameter(String paramType) throws RecognitionException {
ParameterContext _localctx = new ParameterContext(_ctx, getState(), paramType);
enterRule(_localctx, 260, RULE_parameter);
try {
enterOuterAlt(_localctx, 1);
{
setState(1872);
match(DOLLAR);
setState(1873);
parameterName(paramType);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParameterNameContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public String paramType;
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public TerminalNode UNSIGNED_DECIMAL_INTEGER() { return getToken(Cypher6Parser.UNSIGNED_DECIMAL_INTEGER, 0); }
public TerminalNode UNSIGNED_OCTAL_INTEGER() { return getToken(Cypher6Parser.UNSIGNED_OCTAL_INTEGER, 0); }
public TerminalNode EXTENDED_IDENTIFIER() { return getToken(Cypher6Parser.EXTENDED_IDENTIFIER, 0); }
public ParameterNameContext(ParserRuleContext parent, int invokingState) { super(parent, invokingState); }
public ParameterNameContext(ParserRuleContext parent, int invokingState, String paramType) {
super(parent, invokingState);
this.paramType = paramType;
}
@Override public int getRuleIndex() { return RULE_parameterName; }
}
public final ParameterNameContext parameterName(String paramType) throws RecognitionException {
ParameterNameContext _localctx = new ParameterNameContext(_ctx, getState(), paramType);
enterRule(_localctx, 262, RULE_parameterName);
try {
enterOuterAlt(_localctx, 1);
{
setState(1879);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
{
setState(1875);
symbolicNameString();
}
break;
case UNSIGNED_DECIMAL_INTEGER:
{
setState(1876);
match(UNSIGNED_DECIMAL_INTEGER);
}
break;
case UNSIGNED_OCTAL_INTEGER:
{
setState(1877);
match(UNSIGNED_OCTAL_INTEGER);
}
break;
case EXTENDED_IDENTIFIER:
{
setState(1878);
match(EXTENDED_IDENTIFIER);
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FunctionInvocationContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public FunctionNameContext functionName() {
return getRuleContext(FunctionNameContext.class,0);
}
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public List functionArgument() {
return getRuleContexts(FunctionArgumentContext.class);
}
public FunctionArgumentContext functionArgument(int i) {
return getRuleContext(FunctionArgumentContext.class,i);
}
public TerminalNode DISTINCT() { return getToken(Cypher6Parser.DISTINCT, 0); }
public TerminalNode ALL() { return getToken(Cypher6Parser.ALL, 0); }
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public FunctionInvocationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_functionInvocation; }
}
public final FunctionInvocationContext functionInvocation() throws RecognitionException {
FunctionInvocationContext _localctx = new FunctionInvocationContext(_ctx, getState());
enterRule(_localctx, 264, RULE_functionInvocation);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1881);
functionName();
setState(1882);
match(LPAREN);
setState(1884);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,189,_ctx) ) {
case 1:
{
setState(1883);
_la = _input.LA(1);
if ( !(_la==ALL || _la==DISTINCT) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
}
setState(1894);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -246291141492752L) != 0) || ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -146366996479967233L) != 0) || ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -15066095617L) != 0) || ((((_la - 192)) & ~0x3f) == 0 && ((1L << (_la - 192)) & -1130297988612169L) != 0) || ((((_la - 256)) & ~0x3f) == 0 && ((1L << (_la - 256)) & 281474976709631L) != 0)) {
{
setState(1886);
functionArgument();
setState(1891);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(1887);
match(COMMA);
setState(1888);
functionArgument();
}
}
setState(1893);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(1896);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FunctionArgumentContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public FunctionArgumentContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_functionArgument; }
}
public final FunctionArgumentContext functionArgument() throws RecognitionException {
FunctionArgumentContext _localctx = new FunctionArgumentContext(_ctx, getState());
enterRule(_localctx, 266, RULE_functionArgument);
try {
enterOuterAlt(_localctx, 1);
{
setState(1898);
expression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FunctionNameContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public NamespaceContext namespace() {
return getRuleContext(NamespaceContext.class,0);
}
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public FunctionNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_functionName; }
}
public final FunctionNameContext functionName() throws RecognitionException {
FunctionNameContext _localctx = new FunctionNameContext(_ctx, getState());
enterRule(_localctx, 268, RULE_functionName);
try {
enterOuterAlt(_localctx, 1);
{
setState(1900);
namespace();
setState(1901);
symbolicNameString();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NamespaceContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class,i);
}
public List DOT() { return getTokens(Cypher6Parser.DOT); }
public TerminalNode DOT(int i) {
return getToken(Cypher6Parser.DOT, i);
}
public NamespaceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_namespace; }
}
public final NamespaceContext namespace() throws RecognitionException {
NamespaceContext _localctx = new NamespaceContext(_ctx, getState());
enterRule(_localctx, 270, RULE_namespace);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1908);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,192,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1903);
symbolicNameString();
setState(1904);
match(DOT);
}
}
}
setState(1910);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,192,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class VariableContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public VariableContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_variable; }
}
public final VariableContext variable() throws RecognitionException {
VariableContext _localctx = new VariableContext(_ctx, getState());
enterRule(_localctx, 272, RULE_variable);
try {
enterOuterAlt(_localctx, 1);
{
setState(1911);
symbolicNameString();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NonEmptyNameListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public NonEmptyNameListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_nonEmptyNameList; }
}
public final NonEmptyNameListContext nonEmptyNameList() throws RecognitionException {
NonEmptyNameListContext _localctx = new NonEmptyNameListContext(_ctx, getState());
enterRule(_localctx, 274, RULE_nonEmptyNameList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1913);
symbolicNameString();
setState(1918);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(1914);
match(COMMA);
setState(1915);
symbolicNameString();
}
}
setState(1920);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List typePart() {
return getRuleContexts(TypePartContext.class);
}
public TypePartContext typePart(int i) {
return getRuleContext(TypePartContext.class,i);
}
public List BAR() { return getTokens(Cypher6Parser.BAR); }
public TerminalNode BAR(int i) {
return getToken(Cypher6Parser.BAR, i);
}
public TypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_type; }
}
public final TypeContext type() throws RecognitionException {
TypeContext _localctx = new TypeContext(_ctx, getState());
enterRule(_localctx, 276, RULE_type);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1921);
typePart();
setState(1926);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,194,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(1922);
match(BAR);
setState(1923);
typePart();
}
}
}
setState(1928);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,194,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypePartContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TypeNameContext typeName() {
return getRuleContext(TypeNameContext.class,0);
}
public TypeNullabilityContext typeNullability() {
return getRuleContext(TypeNullabilityContext.class,0);
}
public List typeListSuffix() {
return getRuleContexts(TypeListSuffixContext.class);
}
public TypeListSuffixContext typeListSuffix(int i) {
return getRuleContext(TypeListSuffixContext.class,i);
}
public TypePartContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_typePart; }
}
public final TypePartContext typePart() throws RecognitionException {
TypePartContext _localctx = new TypePartContext(_ctx, getState());
enterRule(_localctx, 278, RULE_typePart);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1929);
typeName();
setState(1931);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==EXCLAMATION_MARK || _la==NOT) {
{
setState(1930);
typeNullability();
}
}
setState(1936);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==ARRAY || _la==LIST) {
{
{
setState(1933);
typeListSuffix();
}
}
setState(1938);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeNameContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode NOTHING() { return getToken(Cypher6Parser.NOTHING, 0); }
public TerminalNode NULL() { return getToken(Cypher6Parser.NULL, 0); }
public TerminalNode BOOL() { return getToken(Cypher6Parser.BOOL, 0); }
public TerminalNode BOOLEAN() { return getToken(Cypher6Parser.BOOLEAN, 0); }
public TerminalNode VARCHAR() { return getToken(Cypher6Parser.VARCHAR, 0); }
public TerminalNode STRING() { return getToken(Cypher6Parser.STRING, 0); }
public TerminalNode INT() { return getToken(Cypher6Parser.INT, 0); }
public TerminalNode INTEGER() { return getToken(Cypher6Parser.INTEGER, 0); }
public TerminalNode SIGNED() { return getToken(Cypher6Parser.SIGNED, 0); }
public TerminalNode FLOAT() { return getToken(Cypher6Parser.FLOAT, 0); }
public TerminalNode DATE() { return getToken(Cypher6Parser.DATE, 0); }
public TerminalNode LOCAL() { return getToken(Cypher6Parser.LOCAL, 0); }
public List TIME() { return getTokens(Cypher6Parser.TIME); }
public TerminalNode TIME(int i) {
return getToken(Cypher6Parser.TIME, i);
}
public TerminalNode DATETIME() { return getToken(Cypher6Parser.DATETIME, 0); }
public TerminalNode ZONED() { return getToken(Cypher6Parser.ZONED, 0); }
public TerminalNode WITHOUT() { return getToken(Cypher6Parser.WITHOUT, 0); }
public TerminalNode WITH() { return getToken(Cypher6Parser.WITH, 0); }
public TerminalNode TIMEZONE() { return getToken(Cypher6Parser.TIMEZONE, 0); }
public TerminalNode ZONE() { return getToken(Cypher6Parser.ZONE, 0); }
public TerminalNode TIMESTAMP() { return getToken(Cypher6Parser.TIMESTAMP, 0); }
public TerminalNode DURATION() { return getToken(Cypher6Parser.DURATION, 0); }
public TerminalNode POINT() { return getToken(Cypher6Parser.POINT, 0); }
public TerminalNode NODE() { return getToken(Cypher6Parser.NODE, 0); }
public TerminalNode VERTEX() { return getToken(Cypher6Parser.VERTEX, 0); }
public TerminalNode RELATIONSHIP() { return getToken(Cypher6Parser.RELATIONSHIP, 0); }
public TerminalNode EDGE() { return getToken(Cypher6Parser.EDGE, 0); }
public TerminalNode MAP() { return getToken(Cypher6Parser.MAP, 0); }
public TerminalNode LT() { return getToken(Cypher6Parser.LT, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public TerminalNode GT() { return getToken(Cypher6Parser.GT, 0); }
public TerminalNode LIST() { return getToken(Cypher6Parser.LIST, 0); }
public TerminalNode ARRAY() { return getToken(Cypher6Parser.ARRAY, 0); }
public TerminalNode PATH() { return getToken(Cypher6Parser.PATH, 0); }
public TerminalNode PATHS() { return getToken(Cypher6Parser.PATHS, 0); }
public TerminalNode PROPERTY() { return getToken(Cypher6Parser.PROPERTY, 0); }
public TerminalNode VALUE() { return getToken(Cypher6Parser.VALUE, 0); }
public TerminalNode ANY() { return getToken(Cypher6Parser.ANY, 0); }
public TypeNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_typeName; }
}
public final TypeNameContext typeName() throws RecognitionException {
TypeNameContext _localctx = new TypeNameContext(_ctx, getState());
enterRule(_localctx, 280, RULE_typeName);
int _la;
try {
setState(2004);
_errHandler.sync(this);
switch (_input.LA(1)) {
case NOTHING:
enterOuterAlt(_localctx, 1);
{
setState(1939);
match(NOTHING);
}
break;
case NULL:
enterOuterAlt(_localctx, 2);
{
setState(1940);
match(NULL);
}
break;
case BOOL:
enterOuterAlt(_localctx, 3);
{
setState(1941);
match(BOOL);
}
break;
case BOOLEAN:
enterOuterAlt(_localctx, 4);
{
setState(1942);
match(BOOLEAN);
}
break;
case VARCHAR:
enterOuterAlt(_localctx, 5);
{
setState(1943);
match(VARCHAR);
}
break;
case STRING:
enterOuterAlt(_localctx, 6);
{
setState(1944);
match(STRING);
}
break;
case INT:
enterOuterAlt(_localctx, 7);
{
setState(1945);
match(INT);
}
break;
case INTEGER:
case SIGNED:
enterOuterAlt(_localctx, 8);
{
setState(1947);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==SIGNED) {
{
setState(1946);
match(SIGNED);
}
}
setState(1949);
match(INTEGER);
}
break;
case FLOAT:
enterOuterAlt(_localctx, 9);
{
setState(1950);
match(FLOAT);
}
break;
case DATE:
enterOuterAlt(_localctx, 10);
{
setState(1951);
match(DATE);
}
break;
case LOCAL:
enterOuterAlt(_localctx, 11);
{
setState(1952);
match(LOCAL);
setState(1953);
_la = _input.LA(1);
if ( !(_la==DATETIME || _la==TIME) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
case ZONED:
enterOuterAlt(_localctx, 12);
{
setState(1954);
match(ZONED);
setState(1955);
_la = _input.LA(1);
if ( !(_la==DATETIME || _la==TIME) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
case TIME:
enterOuterAlt(_localctx, 13);
{
setState(1956);
match(TIME);
setState(1957);
_la = _input.LA(1);
if ( !(_la==WITH || _la==WITHOUT) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1961);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMEZONE:
{
setState(1958);
match(TIMEZONE);
}
break;
case TIME:
{
setState(1959);
match(TIME);
setState(1960);
match(ZONE);
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
case TIMESTAMP:
enterOuterAlt(_localctx, 14);
{
setState(1963);
match(TIMESTAMP);
setState(1964);
_la = _input.LA(1);
if ( !(_la==WITH || _la==WITHOUT) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1968);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMEZONE:
{
setState(1965);
match(TIMEZONE);
}
break;
case TIME:
{
setState(1966);
match(TIME);
setState(1967);
match(ZONE);
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
case DURATION:
enterOuterAlt(_localctx, 15);
{
setState(1970);
match(DURATION);
}
break;
case POINT:
enterOuterAlt(_localctx, 16);
{
setState(1971);
match(POINT);
}
break;
case NODE:
enterOuterAlt(_localctx, 17);
{
setState(1972);
match(NODE);
}
break;
case VERTEX:
enterOuterAlt(_localctx, 18);
{
setState(1973);
match(VERTEX);
}
break;
case RELATIONSHIP:
enterOuterAlt(_localctx, 19);
{
setState(1974);
match(RELATIONSHIP);
}
break;
case EDGE:
enterOuterAlt(_localctx, 20);
{
setState(1975);
match(EDGE);
}
break;
case MAP:
enterOuterAlt(_localctx, 21);
{
setState(1976);
match(MAP);
}
break;
case ARRAY:
case LIST:
enterOuterAlt(_localctx, 22);
{
setState(1977);
_la = _input.LA(1);
if ( !(_la==ARRAY || _la==LIST) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1978);
match(LT);
setState(1979);
type();
setState(1980);
match(GT);
}
break;
case PATH:
enterOuterAlt(_localctx, 23);
{
setState(1982);
match(PATH);
}
break;
case PATHS:
enterOuterAlt(_localctx, 24);
{
setState(1983);
match(PATHS);
}
break;
case PROPERTY:
enterOuterAlt(_localctx, 25);
{
setState(1984);
match(PROPERTY);
setState(1985);
match(VALUE);
}
break;
case ANY:
enterOuterAlt(_localctx, 26);
{
setState(1986);
match(ANY);
setState(2002);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,201,_ctx) ) {
case 1:
{
setState(1987);
match(NODE);
}
break;
case 2:
{
setState(1988);
match(VERTEX);
}
break;
case 3:
{
setState(1989);
match(RELATIONSHIP);
}
break;
case 4:
{
setState(1990);
match(EDGE);
}
break;
case 5:
{
setState(1991);
match(MAP);
}
break;
case 6:
{
setState(1992);
match(PROPERTY);
setState(1993);
match(VALUE);
}
break;
case 7:
{
setState(1995);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==VALUE) {
{
setState(1994);
match(VALUE);
}
}
setState(1997);
match(LT);
setState(1998);
type();
setState(1999);
match(GT);
}
break;
case 8:
{
setState(2001);
match(VALUE);
}
break;
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeNullabilityContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public TerminalNode NULL() { return getToken(Cypher6Parser.NULL, 0); }
public TerminalNode EXCLAMATION_MARK() { return getToken(Cypher6Parser.EXCLAMATION_MARK, 0); }
public TypeNullabilityContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_typeNullability; }
}
public final TypeNullabilityContext typeNullability() throws RecognitionException {
TypeNullabilityContext _localctx = new TypeNullabilityContext(_ctx, getState());
enterRule(_localctx, 282, RULE_typeNullability);
try {
setState(2009);
_errHandler.sync(this);
switch (_input.LA(1)) {
case NOT:
enterOuterAlt(_localctx, 1);
{
setState(2006);
match(NOT);
setState(2007);
match(NULL);
}
break;
case EXCLAMATION_MARK:
enterOuterAlt(_localctx, 2);
{
setState(2008);
match(EXCLAMATION_MARK);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeListSuffixContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LIST() { return getToken(Cypher6Parser.LIST, 0); }
public TerminalNode ARRAY() { return getToken(Cypher6Parser.ARRAY, 0); }
public TypeNullabilityContext typeNullability() {
return getRuleContext(TypeNullabilityContext.class,0);
}
public TypeListSuffixContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_typeListSuffix; }
}
public final TypeListSuffixContext typeListSuffix() throws RecognitionException {
TypeListSuffixContext _localctx = new TypeListSuffixContext(_ctx, getState());
enterRule(_localctx, 284, RULE_typeListSuffix);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2011);
_la = _input.LA(1);
if ( !(_la==ARRAY || _la==LIST) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2013);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==EXCLAMATION_MARK || _la==NOT) {
{
setState(2012);
typeNullability();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public CreateCommandContext createCommand() {
return getRuleContext(CreateCommandContext.class,0);
}
public DropCommandContext dropCommand() {
return getRuleContext(DropCommandContext.class,0);
}
public AlterCommandContext alterCommand() {
return getRuleContext(AlterCommandContext.class,0);
}
public RenameCommandContext renameCommand() {
return getRuleContext(RenameCommandContext.class,0);
}
public DenyCommandContext denyCommand() {
return getRuleContext(DenyCommandContext.class,0);
}
public RevokeCommandContext revokeCommand() {
return getRuleContext(RevokeCommandContext.class,0);
}
public GrantCommandContext grantCommand() {
return getRuleContext(GrantCommandContext.class,0);
}
public StartDatabaseContext startDatabase() {
return getRuleContext(StartDatabaseContext.class,0);
}
public StopDatabaseContext stopDatabase() {
return getRuleContext(StopDatabaseContext.class,0);
}
public EnableServerCommandContext enableServerCommand() {
return getRuleContext(EnableServerCommandContext.class,0);
}
public AllocationCommandContext allocationCommand() {
return getRuleContext(AllocationCommandContext.class,0);
}
public ShowCommandContext showCommand() {
return getRuleContext(ShowCommandContext.class,0);
}
public TerminateCommandContext terminateCommand() {
return getRuleContext(TerminateCommandContext.class,0);
}
public UseClauseContext useClause() {
return getRuleContext(UseClauseContext.class,0);
}
public CommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_command; }
}
public final CommandContext command() throws RecognitionException {
CommandContext _localctx = new CommandContext(_ctx, getState());
enterRule(_localctx, 286, RULE_command);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2016);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==USE) {
{
setState(2015);
useClause();
}
}
setState(2031);
_errHandler.sync(this);
switch (_input.LA(1)) {
case CREATE:
{
setState(2018);
createCommand();
}
break;
case DROP:
{
setState(2019);
dropCommand();
}
break;
case ALTER:
{
setState(2020);
alterCommand();
}
break;
case RENAME:
{
setState(2021);
renameCommand();
}
break;
case DENY:
{
setState(2022);
denyCommand();
}
break;
case REVOKE:
{
setState(2023);
revokeCommand();
}
break;
case GRANT:
{
setState(2024);
grantCommand();
}
break;
case START:
{
setState(2025);
startDatabase();
}
break;
case STOP:
{
setState(2026);
stopDatabase();
}
break;
case ENABLE:
{
setState(2027);
enableServerCommand();
}
break;
case DEALLOCATE:
case DRYRUN:
case REALLOCATE:
{
setState(2028);
allocationCommand();
}
break;
case SHOW:
{
setState(2029);
showCommand();
}
break;
case TERMINATE:
{
setState(2030);
terminateCommand();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CREATE() { return getToken(Cypher6Parser.CREATE, 0); }
public CreateAliasContext createAlias() {
return getRuleContext(CreateAliasContext.class,0);
}
public CreateCompositeDatabaseContext createCompositeDatabase() {
return getRuleContext(CreateCompositeDatabaseContext.class,0);
}
public CreateConstraintContext createConstraint() {
return getRuleContext(CreateConstraintContext.class,0);
}
public CreateDatabaseContext createDatabase() {
return getRuleContext(CreateDatabaseContext.class,0);
}
public CreateIndexContext createIndex() {
return getRuleContext(CreateIndexContext.class,0);
}
public CreateRoleContext createRole() {
return getRuleContext(CreateRoleContext.class,0);
}
public CreateUserContext createUser() {
return getRuleContext(CreateUserContext.class,0);
}
public TerminalNode OR() { return getToken(Cypher6Parser.OR, 0); }
public TerminalNode REPLACE() { return getToken(Cypher6Parser.REPLACE, 0); }
public CreateCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createCommand; }
}
public final CreateCommandContext createCommand() throws RecognitionException {
CreateCommandContext _localctx = new CreateCommandContext(_ctx, getState());
enterRule(_localctx, 288, RULE_createCommand);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2033);
match(CREATE);
setState(2036);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==OR) {
{
setState(2034);
match(OR);
setState(2035);
match(REPLACE);
}
}
setState(2045);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALIAS:
{
setState(2038);
createAlias();
}
break;
case COMPOSITE:
{
setState(2039);
createCompositeDatabase();
}
break;
case CONSTRAINT:
{
setState(2040);
createConstraint();
}
break;
case DATABASE:
{
setState(2041);
createDatabase();
}
break;
case BTREE:
case FULLTEXT:
case INDEX:
case LOOKUP:
case POINT:
case RANGE:
case TEXT:
case VECTOR:
{
setState(2042);
createIndex();
}
break;
case ROLE:
{
setState(2043);
createRole();
}
break;
case USER:
{
setState(2044);
createUser();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DROP() { return getToken(Cypher6Parser.DROP, 0); }
public DropAliasContext dropAlias() {
return getRuleContext(DropAliasContext.class,0);
}
public DropConstraintContext dropConstraint() {
return getRuleContext(DropConstraintContext.class,0);
}
public DropDatabaseContext dropDatabase() {
return getRuleContext(DropDatabaseContext.class,0);
}
public DropIndexContext dropIndex() {
return getRuleContext(DropIndexContext.class,0);
}
public DropRoleContext dropRole() {
return getRuleContext(DropRoleContext.class,0);
}
public DropServerContext dropServer() {
return getRuleContext(DropServerContext.class,0);
}
public DropUserContext dropUser() {
return getRuleContext(DropUserContext.class,0);
}
public DropCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dropCommand; }
}
public final DropCommandContext dropCommand() throws RecognitionException {
DropCommandContext _localctx = new DropCommandContext(_ctx, getState());
enterRule(_localctx, 290, RULE_dropCommand);
try {
enterOuterAlt(_localctx, 1);
{
setState(2047);
match(DROP);
setState(2055);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALIAS:
{
setState(2048);
dropAlias();
}
break;
case CONSTRAINT:
{
setState(2049);
dropConstraint();
}
break;
case COMPOSITE:
case DATABASE:
{
setState(2050);
dropDatabase();
}
break;
case INDEX:
{
setState(2051);
dropIndex();
}
break;
case ROLE:
{
setState(2052);
dropRole();
}
break;
case SERVER:
{
setState(2053);
dropServer();
}
break;
case USER:
{
setState(2054);
dropUser();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SHOW() { return getToken(Cypher6Parser.SHOW, 0); }
public ShowAliasesContext showAliases() {
return getRuleContext(ShowAliasesContext.class,0);
}
public ShowConstraintCommandContext showConstraintCommand() {
return getRuleContext(ShowConstraintCommandContext.class,0);
}
public ShowCurrentUserContext showCurrentUser() {
return getRuleContext(ShowCurrentUserContext.class,0);
}
public ShowDatabaseContext showDatabase() {
return getRuleContext(ShowDatabaseContext.class,0);
}
public ShowFunctionsContext showFunctions() {
return getRuleContext(ShowFunctionsContext.class,0);
}
public ShowIndexCommandContext showIndexCommand() {
return getRuleContext(ShowIndexCommandContext.class,0);
}
public ShowPrivilegesContext showPrivileges() {
return getRuleContext(ShowPrivilegesContext.class,0);
}
public ShowProceduresContext showProcedures() {
return getRuleContext(ShowProceduresContext.class,0);
}
public ShowRolePrivilegesContext showRolePrivileges() {
return getRuleContext(ShowRolePrivilegesContext.class,0);
}
public ShowRolesContext showRoles() {
return getRuleContext(ShowRolesContext.class,0);
}
public ShowServersContext showServers() {
return getRuleContext(ShowServersContext.class,0);
}
public ShowSettingsContext showSettings() {
return getRuleContext(ShowSettingsContext.class,0);
}
public ShowSupportedPrivilegesContext showSupportedPrivileges() {
return getRuleContext(ShowSupportedPrivilegesContext.class,0);
}
public ShowTransactionsContext showTransactions() {
return getRuleContext(ShowTransactionsContext.class,0);
}
public ShowUserPrivilegesContext showUserPrivileges() {
return getRuleContext(ShowUserPrivilegesContext.class,0);
}
public ShowUsersContext showUsers() {
return getRuleContext(ShowUsersContext.class,0);
}
public ShowCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showCommand; }
}
public final ShowCommandContext showCommand() throws RecognitionException {
ShowCommandContext _localctx = new ShowCommandContext(_ctx, getState());
enterRule(_localctx, 292, RULE_showCommand);
try {
enterOuterAlt(_localctx, 1);
{
setState(2057);
match(SHOW);
setState(2074);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,210,_ctx) ) {
case 1:
{
setState(2058);
showAliases();
}
break;
case 2:
{
setState(2059);
showConstraintCommand();
}
break;
case 3:
{
setState(2060);
showCurrentUser();
}
break;
case 4:
{
setState(2061);
showDatabase();
}
break;
case 5:
{
setState(2062);
showFunctions();
}
break;
case 6:
{
setState(2063);
showIndexCommand();
}
break;
case 7:
{
setState(2064);
showPrivileges();
}
break;
case 8:
{
setState(2065);
showProcedures();
}
break;
case 9:
{
setState(2066);
showRolePrivileges();
}
break;
case 10:
{
setState(2067);
showRoles();
}
break;
case 11:
{
setState(2068);
showServers();
}
break;
case 12:
{
setState(2069);
showSettings();
}
break;
case 13:
{
setState(2070);
showSupportedPrivileges();
}
break;
case 14:
{
setState(2071);
showTransactions();
}
break;
case 15:
{
setState(2072);
showUserPrivileges();
}
break;
case 16:
{
setState(2073);
showUsers();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowCommandYieldContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public YieldClauseContext yieldClause() {
return getRuleContext(YieldClauseContext.class,0);
}
public ReturnClauseContext returnClause() {
return getRuleContext(ReturnClauseContext.class,0);
}
public WhereClauseContext whereClause() {
return getRuleContext(WhereClauseContext.class,0);
}
public ShowCommandYieldContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showCommandYield; }
}
public final ShowCommandYieldContext showCommandYield() throws RecognitionException {
ShowCommandYieldContext _localctx = new ShowCommandYieldContext(_ctx, getState());
enterRule(_localctx, 294, RULE_showCommandYield);
int _la;
try {
setState(2081);
_errHandler.sync(this);
switch (_input.LA(1)) {
case YIELD:
enterOuterAlt(_localctx, 1);
{
setState(2076);
yieldClause();
setState(2078);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==RETURN) {
{
setState(2077);
returnClause();
}
}
}
break;
case WHERE:
enterOuterAlt(_localctx, 2);
{
setState(2080);
whereClause();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class YieldItemContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List variable() {
return getRuleContexts(VariableContext.class);
}
public VariableContext variable(int i) {
return getRuleContext(VariableContext.class,i);
}
public TerminalNode AS() { return getToken(Cypher6Parser.AS, 0); }
public YieldItemContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_yieldItem; }
}
public final YieldItemContext yieldItem() throws RecognitionException {
YieldItemContext _localctx = new YieldItemContext(_ctx, getState());
enterRule(_localctx, 296, RULE_yieldItem);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2083);
variable();
setState(2086);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AS) {
{
setState(2084);
match(AS);
setState(2085);
variable();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class YieldSkipContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SignedIntegerLiteralContext signedIntegerLiteral() {
return getRuleContext(SignedIntegerLiteralContext.class,0);
}
public TerminalNode OFFSET() { return getToken(Cypher6Parser.OFFSET, 0); }
public TerminalNode SKIPROWS() { return getToken(Cypher6Parser.SKIPROWS, 0); }
public YieldSkipContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_yieldSkip; }
}
public final YieldSkipContext yieldSkip() throws RecognitionException {
YieldSkipContext _localctx = new YieldSkipContext(_ctx, getState());
enterRule(_localctx, 298, RULE_yieldSkip);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2088);
_la = _input.LA(1);
if ( !(_la==OFFSET || _la==SKIPROWS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2089);
signedIntegerLiteral();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class YieldLimitContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LIMITROWS() { return getToken(Cypher6Parser.LIMITROWS, 0); }
public SignedIntegerLiteralContext signedIntegerLiteral() {
return getRuleContext(SignedIntegerLiteralContext.class,0);
}
public YieldLimitContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_yieldLimit; }
}
public final YieldLimitContext yieldLimit() throws RecognitionException {
YieldLimitContext _localctx = new YieldLimitContext(_ctx, getState());
enterRule(_localctx, 300, RULE_yieldLimit);
try {
enterOuterAlt(_localctx, 1);
{
setState(2091);
match(LIMITROWS);
setState(2092);
signedIntegerLiteral();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class YieldClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode YIELD() { return getToken(Cypher6Parser.YIELD, 0); }
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public List yieldItem() {
return getRuleContexts(YieldItemContext.class);
}
public YieldItemContext yieldItem(int i) {
return getRuleContext(YieldItemContext.class,i);
}
public OrderByContext orderBy() {
return getRuleContext(OrderByContext.class,0);
}
public YieldSkipContext yieldSkip() {
return getRuleContext(YieldSkipContext.class,0);
}
public YieldLimitContext yieldLimit() {
return getRuleContext(YieldLimitContext.class,0);
}
public WhereClauseContext whereClause() {
return getRuleContext(WhereClauseContext.class,0);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public YieldClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_yieldClause; }
}
public final YieldClauseContext yieldClause() throws RecognitionException {
YieldClauseContext _localctx = new YieldClauseContext(_ctx, getState());
enterRule(_localctx, 302, RULE_yieldClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2094);
match(YIELD);
setState(2104);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(2095);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
{
setState(2096);
yieldItem();
setState(2101);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(2097);
match(COMMA);
setState(2098);
yieldItem();
}
}
setState(2103);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(2107);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==ORDER) {
{
setState(2106);
orderBy();
}
}
setState(2110);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==OFFSET || _la==SKIPROWS) {
{
setState(2109);
yieldSkip();
}
}
setState(2113);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LIMITROWS) {
{
setState(2112);
yieldLimit();
}
}
setState(2116);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE) {
{
setState(2115);
whereClause();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CommandOptionsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode OPTIONS() { return getToken(Cypher6Parser.OPTIONS, 0); }
public MapOrParameterContext mapOrParameter() {
return getRuleContext(MapOrParameterContext.class,0);
}
public CommandOptionsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_commandOptions; }
}
public final CommandOptionsContext commandOptions() throws RecognitionException {
CommandOptionsContext _localctx = new CommandOptionsContext(_ctx, getState());
enterRule(_localctx, 304, RULE_commandOptions);
try {
enterOuterAlt(_localctx, 1);
{
setState(2118);
match(OPTIONS);
setState(2119);
mapOrParameter();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TerminateCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode TERMINATE() { return getToken(Cypher6Parser.TERMINATE, 0); }
public TerminateTransactionsContext terminateTransactions() {
return getRuleContext(TerminateTransactionsContext.class,0);
}
public TerminateCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_terminateCommand; }
}
public final TerminateCommandContext terminateCommand() throws RecognitionException {
TerminateCommandContext _localctx = new TerminateCommandContext(_ctx, getState());
enterRule(_localctx, 306, RULE_terminateCommand);
try {
enterOuterAlt(_localctx, 1);
{
setState(2121);
match(TERMINATE);
setState(2122);
terminateTransactions();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ComposableCommandClausesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminateCommandContext terminateCommand() {
return getRuleContext(TerminateCommandContext.class,0);
}
public ComposableShowCommandClausesContext composableShowCommandClauses() {
return getRuleContext(ComposableShowCommandClausesContext.class,0);
}
public ComposableCommandClausesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_composableCommandClauses; }
}
public final ComposableCommandClausesContext composableCommandClauses() throws RecognitionException {
ComposableCommandClausesContext _localctx = new ComposableCommandClausesContext(_ctx, getState());
enterRule(_localctx, 308, RULE_composableCommandClauses);
try {
setState(2126);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TERMINATE:
enterOuterAlt(_localctx, 1);
{
setState(2124);
terminateCommand();
}
break;
case SHOW:
enterOuterAlt(_localctx, 2);
{
setState(2125);
composableShowCommandClauses();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ComposableShowCommandClausesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SHOW() { return getToken(Cypher6Parser.SHOW, 0); }
public ShowIndexCommandContext showIndexCommand() {
return getRuleContext(ShowIndexCommandContext.class,0);
}
public ShowConstraintCommandContext showConstraintCommand() {
return getRuleContext(ShowConstraintCommandContext.class,0);
}
public ShowFunctionsContext showFunctions() {
return getRuleContext(ShowFunctionsContext.class,0);
}
public ShowProceduresContext showProcedures() {
return getRuleContext(ShowProceduresContext.class,0);
}
public ShowSettingsContext showSettings() {
return getRuleContext(ShowSettingsContext.class,0);
}
public ShowTransactionsContext showTransactions() {
return getRuleContext(ShowTransactionsContext.class,0);
}
public ComposableShowCommandClausesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_composableShowCommandClauses; }
}
public final ComposableShowCommandClausesContext composableShowCommandClauses() throws RecognitionException {
ComposableShowCommandClausesContext _localctx = new ComposableShowCommandClausesContext(_ctx, getState());
enterRule(_localctx, 310, RULE_composableShowCommandClauses);
try {
enterOuterAlt(_localctx, 1);
{
setState(2128);
match(SHOW);
setState(2135);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,221,_ctx) ) {
case 1:
{
setState(2129);
showIndexCommand();
}
break;
case 2:
{
setState(2130);
showConstraintCommand();
}
break;
case 3:
{
setState(2131);
showFunctions();
}
break;
case 4:
{
setState(2132);
showProcedures();
}
break;
case 5:
{
setState(2133);
showSettings();
}
break;
case 6:
{
setState(2134);
showTransactions();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowIndexCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ShowIndexesEndContext showIndexesEnd() {
return getRuleContext(ShowIndexesEndContext.class,0);
}
public ShowIndexTypeContext showIndexType() {
return getRuleContext(ShowIndexTypeContext.class,0);
}
public ShowIndexCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showIndexCommand; }
}
public final ShowIndexCommandContext showIndexCommand() throws RecognitionException {
ShowIndexCommandContext _localctx = new ShowIndexCommandContext(_ctx, getState());
enterRule(_localctx, 312, RULE_showIndexCommand);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2138);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==ALL || _la==BTREE || _la==FULLTEXT || _la==LOOKUP || _la==POINT || _la==RANGE || _la==TEXT || _la==VECTOR) {
{
setState(2137);
showIndexType();
}
}
setState(2140);
showIndexesEnd();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowIndexTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ALL() { return getToken(Cypher6Parser.ALL, 0); }
public TerminalNode BTREE() { return getToken(Cypher6Parser.BTREE, 0); }
public TerminalNode FULLTEXT() { return getToken(Cypher6Parser.FULLTEXT, 0); }
public TerminalNode LOOKUP() { return getToken(Cypher6Parser.LOOKUP, 0); }
public TerminalNode POINT() { return getToken(Cypher6Parser.POINT, 0); }
public TerminalNode RANGE() { return getToken(Cypher6Parser.RANGE, 0); }
public TerminalNode TEXT() { return getToken(Cypher6Parser.TEXT, 0); }
public TerminalNode VECTOR() { return getToken(Cypher6Parser.VECTOR, 0); }
public ShowIndexTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showIndexType; }
}
public final ShowIndexTypeContext showIndexType() throws RecognitionException {
ShowIndexTypeContext _localctx = new ShowIndexTypeContext(_ctx, getState());
enterRule(_localctx, 314, RULE_showIndexType);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2142);
_la = _input.LA(1);
if ( !(_la==ALL || _la==BTREE || _la==FULLTEXT || _la==LOOKUP || _la==POINT || _la==RANGE || _la==TEXT || _la==VECTOR) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowIndexesEndContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public IndexTokenContext indexToken() {
return getRuleContext(IndexTokenContext.class,0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class,0);
}
public ComposableCommandClausesContext composableCommandClauses() {
return getRuleContext(ComposableCommandClausesContext.class,0);
}
public ShowIndexesEndContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showIndexesEnd; }
}
public final ShowIndexesEndContext showIndexesEnd() throws RecognitionException {
ShowIndexesEndContext _localctx = new ShowIndexesEndContext(_ctx, getState());
enterRule(_localctx, 316, RULE_showIndexesEnd);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2144);
indexToken();
setState(2146);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(2145);
showCommandYield();
}
}
setState(2149);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==SHOW || _la==TERMINATE) {
{
setState(2148);
composableCommandClauses();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ShowConstraintCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showConstraintCommand; }
public ShowConstraintCommandContext() { }
public void copyFrom(ShowConstraintCommandContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintUniqueContext extends ShowConstraintCommandContext {
public ShowConstraintsEndContext showConstraintsEnd() {
return getRuleContext(ShowConstraintsEndContext.class,0);
}
public TerminalNode UNIQUE() { return getToken(Cypher6Parser.UNIQUE, 0); }
public TerminalNode UNIQUENESS() { return getToken(Cypher6Parser.UNIQUENESS, 0); }
public ShowConstraintEntityContext showConstraintEntity() {
return getRuleContext(ShowConstraintEntityContext.class,0);
}
public TerminalNode PROPERTY() { return getToken(Cypher6Parser.PROPERTY, 0); }
public ShowConstraintUniqueContext(ShowConstraintCommandContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintExistContext extends ShowConstraintCommandContext {
public ConstraintExistTypeContext constraintExistType() {
return getRuleContext(ConstraintExistTypeContext.class,0);
}
public ShowConstraintsEndContext showConstraintsEnd() {
return getRuleContext(ShowConstraintsEndContext.class,0);
}
public ShowConstraintEntityContext showConstraintEntity() {
return getRuleContext(ShowConstraintEntityContext.class,0);
}
public ShowConstraintExistContext(ShowConstraintCommandContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintAllContext extends ShowConstraintCommandContext {
public ShowConstraintsEndContext showConstraintsEnd() {
return getRuleContext(ShowConstraintsEndContext.class,0);
}
public TerminalNode ALL() { return getToken(Cypher6Parser.ALL, 0); }
public ShowConstraintAllContext(ShowConstraintCommandContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintKeyContext extends ShowConstraintCommandContext {
public TerminalNode KEY() { return getToken(Cypher6Parser.KEY, 0); }
public ShowConstraintsEndContext showConstraintsEnd() {
return getRuleContext(ShowConstraintsEndContext.class,0);
}
public ShowConstraintEntityContext showConstraintEntity() {
return getRuleContext(ShowConstraintEntityContext.class,0);
}
public ShowConstraintKeyContext(ShowConstraintCommandContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintPropTypeContext extends ShowConstraintCommandContext {
public TerminalNode PROPERTY() { return getToken(Cypher6Parser.PROPERTY, 0); }
public TerminalNode TYPE() { return getToken(Cypher6Parser.TYPE, 0); }
public ShowConstraintsEndContext showConstraintsEnd() {
return getRuleContext(ShowConstraintsEndContext.class,0);
}
public ShowConstraintEntityContext showConstraintEntity() {
return getRuleContext(ShowConstraintEntityContext.class,0);
}
public ShowConstraintPropTypeContext(ShowConstraintCommandContext ctx) { copyFrom(ctx); }
}
public final ShowConstraintCommandContext showConstraintCommand() throws RecognitionException {
ShowConstraintCommandContext _localctx = new ShowConstraintCommandContext(_ctx, getState());
enterRule(_localctx, 318, RULE_showConstraintCommand);
int _la;
try {
setState(2180);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,231,_ctx) ) {
case 1:
_localctx = new ShowConstraintAllContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(2152);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==ALL) {
{
setState(2151);
match(ALL);
}
}
setState(2154);
showConstraintsEnd();
}
break;
case 2:
_localctx = new ShowConstraintExistContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(2156);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 170)) & ~0x3f) == 0 && ((1L << (_la - 170)) & 844424930131969L) != 0)) {
{
setState(2155);
showConstraintEntity();
}
}
setState(2158);
constraintExistType();
setState(2159);
showConstraintsEnd();
}
break;
case 3:
_localctx = new ShowConstraintKeyContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(2162);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 170)) & ~0x3f) == 0 && ((1L << (_la - 170)) & 844424930131969L) != 0)) {
{
setState(2161);
showConstraintEntity();
}
}
setState(2164);
match(KEY);
setState(2165);
showConstraintsEnd();
}
break;
case 4:
_localctx = new ShowConstraintPropTypeContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(2167);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 170)) & ~0x3f) == 0 && ((1L << (_la - 170)) & 844424930131969L) != 0)) {
{
setState(2166);
showConstraintEntity();
}
}
setState(2169);
match(PROPERTY);
setState(2170);
match(TYPE);
setState(2171);
showConstraintsEnd();
}
break;
case 5:
_localctx = new ShowConstraintUniqueContext(_localctx);
enterOuterAlt(_localctx, 5);
{
setState(2173);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 170)) & ~0x3f) == 0 && ((1L << (_la - 170)) & 844424930131969L) != 0)) {
{
setState(2172);
showConstraintEntity();
}
}
setState(2176);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==PROPERTY) {
{
setState(2175);
match(PROPERTY);
}
}
setState(2178);
_la = _input.LA(1);
if ( !(_la==UNIQUE || _la==UNIQUENESS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2179);
showConstraintsEnd();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintEntityContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ShowConstraintEntityContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showConstraintEntity; }
public ShowConstraintEntityContext() { }
public void copyFrom(ShowConstraintEntityContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class NodeEntityContext extends ShowConstraintEntityContext {
public TerminalNode NODE() { return getToken(Cypher6Parser.NODE, 0); }
public NodeEntityContext(ShowConstraintEntityContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class RelEntityContext extends ShowConstraintEntityContext {
public TerminalNode RELATIONSHIP() { return getToken(Cypher6Parser.RELATIONSHIP, 0); }
public TerminalNode REL() { return getToken(Cypher6Parser.REL, 0); }
public RelEntityContext(ShowConstraintEntityContext ctx) { copyFrom(ctx); }
}
public final ShowConstraintEntityContext showConstraintEntity() throws RecognitionException {
ShowConstraintEntityContext _localctx = new ShowConstraintEntityContext(_ctx, getState());
enterRule(_localctx, 320, RULE_showConstraintEntity);
int _la;
try {
setState(2184);
_errHandler.sync(this);
switch (_input.LA(1)) {
case NODE:
_localctx = new NodeEntityContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(2182);
match(NODE);
}
break;
case REL:
case RELATIONSHIP:
_localctx = new RelEntityContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(2183);
_la = _input.LA(1);
if ( !(_la==REL || _la==RELATIONSHIP) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintExistTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode EXISTENCE() { return getToken(Cypher6Parser.EXISTENCE, 0); }
public TerminalNode EXIST() { return getToken(Cypher6Parser.EXIST, 0); }
public TerminalNode PROPERTY() { return getToken(Cypher6Parser.PROPERTY, 0); }
public ConstraintExistTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_constraintExistType; }
}
public final ConstraintExistTypeContext constraintExistType() throws RecognitionException {
ConstraintExistTypeContext _localctx = new ConstraintExistTypeContext(_ctx, getState());
enterRule(_localctx, 322, RULE_constraintExistType);
try {
setState(2192);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,233,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(2186);
match(EXISTENCE);
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(2187);
match(EXIST);
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(2188);
match(PROPERTY);
setState(2189);
match(EXISTENCE);
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(2190);
match(PROPERTY);
setState(2191);
match(EXIST);
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintsEndContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ConstraintTokenContext constraintToken() {
return getRuleContext(ConstraintTokenContext.class,0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class,0);
}
public ComposableCommandClausesContext composableCommandClauses() {
return getRuleContext(ComposableCommandClausesContext.class,0);
}
public ShowConstraintsEndContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showConstraintsEnd; }
}
public final ShowConstraintsEndContext showConstraintsEnd() throws RecognitionException {
ShowConstraintsEndContext _localctx = new ShowConstraintsEndContext(_ctx, getState());
enterRule(_localctx, 324, RULE_showConstraintsEnd);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2194);
constraintToken();
setState(2196);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(2195);
showCommandYield();
}
}
setState(2199);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==SHOW || _la==TERMINATE) {
{
setState(2198);
composableCommandClauses();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowProceduresContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PROCEDURE() { return getToken(Cypher6Parser.PROCEDURE, 0); }
public TerminalNode PROCEDURES() { return getToken(Cypher6Parser.PROCEDURES, 0); }
public ExecutableByContext executableBy() {
return getRuleContext(ExecutableByContext.class,0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class,0);
}
public ComposableCommandClausesContext composableCommandClauses() {
return getRuleContext(ComposableCommandClausesContext.class,0);
}
public ShowProceduresContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showProcedures; }
}
public final ShowProceduresContext showProcedures() throws RecognitionException {
ShowProceduresContext _localctx = new ShowProceduresContext(_ctx, getState());
enterRule(_localctx, 326, RULE_showProcedures);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2201);
_la = _input.LA(1);
if ( !(_la==PROCEDURE || _la==PROCEDURES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2203);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==EXECUTABLE) {
{
setState(2202);
executableBy();
}
}
setState(2206);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(2205);
showCommandYield();
}
}
setState(2209);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==SHOW || _la==TERMINATE) {
{
setState(2208);
composableCommandClauses();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowFunctionsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public FunctionTokenContext functionToken() {
return getRuleContext(FunctionTokenContext.class,0);
}
public ShowFunctionsTypeContext showFunctionsType() {
return getRuleContext(ShowFunctionsTypeContext.class,0);
}
public ExecutableByContext executableBy() {
return getRuleContext(ExecutableByContext.class,0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class,0);
}
public ComposableCommandClausesContext composableCommandClauses() {
return getRuleContext(ComposableCommandClausesContext.class,0);
}
public ShowFunctionsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showFunctions; }
}
public final ShowFunctionsContext showFunctions() throws RecognitionException {
ShowFunctionsContext _localctx = new ShowFunctionsContext(_ctx, getState());
enterRule(_localctx, 328, RULE_showFunctions);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2212);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==ALL || _la==BUILT || _la==USER) {
{
setState(2211);
showFunctionsType();
}
}
setState(2214);
functionToken();
setState(2216);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==EXECUTABLE) {
{
setState(2215);
executableBy();
}
}
setState(2219);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(2218);
showCommandYield();
}
}
setState(2222);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==SHOW || _la==TERMINATE) {
{
setState(2221);
composableCommandClauses();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FunctionTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode FUNCTION() { return getToken(Cypher6Parser.FUNCTION, 0); }
public TerminalNode FUNCTIONS() { return getToken(Cypher6Parser.FUNCTIONS, 0); }
public FunctionTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_functionToken; }
}
public final FunctionTokenContext functionToken() throws RecognitionException {
FunctionTokenContext _localctx = new FunctionTokenContext(_ctx, getState());
enterRule(_localctx, 330, RULE_functionToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2224);
_la = _input.LA(1);
if ( !(_la==FUNCTION || _la==FUNCTIONS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExecutableByContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode EXECUTABLE() { return getToken(Cypher6Parser.EXECUTABLE, 0); }
public TerminalNode BY() { return getToken(Cypher6Parser.BY, 0); }
public TerminalNode CURRENT() { return getToken(Cypher6Parser.CURRENT, 0); }
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public ExecutableByContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_executableBy; }
}
public final ExecutableByContext executableBy() throws RecognitionException {
ExecutableByContext _localctx = new ExecutableByContext(_ctx, getState());
enterRule(_localctx, 332, RULE_executableBy);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2226);
match(EXECUTABLE);
setState(2233);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==BY) {
{
setState(2227);
match(BY);
setState(2231);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,243,_ctx) ) {
case 1:
{
setState(2228);
match(CURRENT);
setState(2229);
match(USER);
}
break;
case 2:
{
setState(2230);
symbolicNameString();
}
break;
}
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowFunctionsTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ALL() { return getToken(Cypher6Parser.ALL, 0); }
public TerminalNode BUILT() { return getToken(Cypher6Parser.BUILT, 0); }
public TerminalNode IN() { return getToken(Cypher6Parser.IN, 0); }
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public TerminalNode DEFINED() { return getToken(Cypher6Parser.DEFINED, 0); }
public ShowFunctionsTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showFunctionsType; }
}
public final ShowFunctionsTypeContext showFunctionsType() throws RecognitionException {
ShowFunctionsTypeContext _localctx = new ShowFunctionsTypeContext(_ctx, getState());
enterRule(_localctx, 334, RULE_showFunctionsType);
try {
setState(2240);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALL:
enterOuterAlt(_localctx, 1);
{
setState(2235);
match(ALL);
}
break;
case BUILT:
enterOuterAlt(_localctx, 2);
{
setState(2236);
match(BUILT);
setState(2237);
match(IN);
}
break;
case USER:
enterOuterAlt(_localctx, 3);
{
setState(2238);
match(USER);
setState(2239);
match(DEFINED);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowTransactionsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TransactionTokenContext transactionToken() {
return getRuleContext(TransactionTokenContext.class,0);
}
public NamesAndClausesContext namesAndClauses() {
return getRuleContext(NamesAndClausesContext.class,0);
}
public ShowTransactionsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showTransactions; }
}
public final ShowTransactionsContext showTransactions() throws RecognitionException {
ShowTransactionsContext _localctx = new ShowTransactionsContext(_ctx, getState());
enterRule(_localctx, 336, RULE_showTransactions);
try {
enterOuterAlt(_localctx, 1);
{
setState(2242);
transactionToken();
setState(2243);
namesAndClauses();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TerminateTransactionsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TransactionTokenContext transactionToken() {
return getRuleContext(TransactionTokenContext.class,0);
}
public NamesAndClausesContext namesAndClauses() {
return getRuleContext(NamesAndClausesContext.class,0);
}
public TerminateTransactionsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_terminateTransactions; }
}
public final TerminateTransactionsContext terminateTransactions() throws RecognitionException {
TerminateTransactionsContext _localctx = new TerminateTransactionsContext(_ctx, getState());
enterRule(_localctx, 338, RULE_terminateTransactions);
try {
enterOuterAlt(_localctx, 1);
{
setState(2245);
transactionToken();
setState(2246);
namesAndClauses();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowSettingsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SettingTokenContext settingToken() {
return getRuleContext(SettingTokenContext.class,0);
}
public NamesAndClausesContext namesAndClauses() {
return getRuleContext(NamesAndClausesContext.class,0);
}
public ShowSettingsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showSettings; }
}
public final ShowSettingsContext showSettings() throws RecognitionException {
ShowSettingsContext _localctx = new ShowSettingsContext(_ctx, getState());
enterRule(_localctx, 340, RULE_showSettings);
try {
enterOuterAlt(_localctx, 1);
{
setState(2248);
settingToken();
setState(2249);
namesAndClauses();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SettingTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SETTING() { return getToken(Cypher6Parser.SETTING, 0); }
public TerminalNode SETTINGS() { return getToken(Cypher6Parser.SETTINGS, 0); }
public SettingTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_settingToken; }
}
public final SettingTokenContext settingToken() throws RecognitionException {
SettingTokenContext _localctx = new SettingTokenContext(_ctx, getState());
enterRule(_localctx, 342, RULE_settingToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2251);
_la = _input.LA(1);
if ( !(_la==SETTING || _la==SETTINGS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NamesAndClausesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public StringsOrExpressionContext stringsOrExpression() {
return getRuleContext(StringsOrExpressionContext.class,0);
}
public ComposableCommandClausesContext composableCommandClauses() {
return getRuleContext(ComposableCommandClausesContext.class,0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class,0);
}
public NamesAndClausesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_namesAndClauses; }
}
public final NamesAndClausesContext namesAndClauses() throws RecognitionException {
NamesAndClausesContext _localctx = new NamesAndClausesContext(_ctx, getState());
enterRule(_localctx, 344, RULE_namesAndClauses);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2260);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,248,_ctx) ) {
case 1:
{
setState(2254);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(2253);
showCommandYield();
}
}
}
break;
case 2:
{
setState(2256);
stringsOrExpression();
setState(2258);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(2257);
showCommandYield();
}
}
}
break;
}
setState(2263);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==SHOW || _la==TERMINATE) {
{
setState(2262);
composableCommandClauses();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StringsOrExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public StringListContext stringList() {
return getRuleContext(StringListContext.class,0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public StringsOrExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_stringsOrExpression; }
}
public final StringsOrExpressionContext stringsOrExpression() throws RecognitionException {
StringsOrExpressionContext _localctx = new StringsOrExpressionContext(_ctx, getState());
enterRule(_localctx, 346, RULE_stringsOrExpression);
try {
setState(2267);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,250,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(2265);
stringList();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(2266);
expression();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CommandNodePatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public LabelTypeContext labelType() {
return getRuleContext(LabelTypeContext.class,0);
}
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public CommandNodePatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_commandNodePattern; }
}
public final CommandNodePatternContext commandNodePattern() throws RecognitionException {
CommandNodePatternContext _localctx = new CommandNodePatternContext(_ctx, getState());
enterRule(_localctx, 348, RULE_commandNodePattern);
try {
enterOuterAlt(_localctx, 1);
{
setState(2269);
match(LPAREN);
setState(2270);
variable();
setState(2271);
labelType();
setState(2272);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CommandRelPatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List LPAREN() { return getTokens(Cypher6Parser.LPAREN); }
public TerminalNode LPAREN(int i) {
return getToken(Cypher6Parser.LPAREN, i);
}
public List RPAREN() { return getTokens(Cypher6Parser.RPAREN); }
public TerminalNode RPAREN(int i) {
return getToken(Cypher6Parser.RPAREN, i);
}
public List arrowLine() {
return getRuleContexts(ArrowLineContext.class);
}
public ArrowLineContext arrowLine(int i) {
return getRuleContext(ArrowLineContext.class,i);
}
public TerminalNode LBRACKET() { return getToken(Cypher6Parser.LBRACKET, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public RelTypeContext relType() {
return getRuleContext(RelTypeContext.class,0);
}
public TerminalNode RBRACKET() { return getToken(Cypher6Parser.RBRACKET, 0); }
public LeftArrowContext leftArrow() {
return getRuleContext(LeftArrowContext.class,0);
}
public RightArrowContext rightArrow() {
return getRuleContext(RightArrowContext.class,0);
}
public CommandRelPatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_commandRelPattern; }
}
public final CommandRelPatternContext commandRelPattern() throws RecognitionException {
CommandRelPatternContext _localctx = new CommandRelPatternContext(_ctx, getState());
enterRule(_localctx, 350, RULE_commandRelPattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2274);
match(LPAREN);
setState(2275);
match(RPAREN);
setState(2277);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LT || _la==ARROW_LEFT_HEAD) {
{
setState(2276);
leftArrow();
}
}
setState(2279);
arrowLine();
setState(2280);
match(LBRACKET);
setState(2281);
variable();
setState(2282);
relType();
setState(2283);
match(RBRACKET);
setState(2284);
arrowLine();
setState(2286);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==GT || _la==ARROW_RIGHT_HEAD) {
{
setState(2285);
rightArrow();
}
}
setState(2288);
match(LPAREN);
setState(2289);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateConstraintContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CONSTRAINT() { return getToken(Cypher6Parser.CONSTRAINT, 0); }
public TerminalNode FOR() { return getToken(Cypher6Parser.FOR, 0); }
public ConstraintTypeContext constraintType() {
return getRuleContext(ConstraintTypeContext.class,0);
}
public CommandNodePatternContext commandNodePattern() {
return getRuleContext(CommandNodePatternContext.class,0);
}
public CommandRelPatternContext commandRelPattern() {
return getRuleContext(CommandRelPatternContext.class,0);
}
public SymbolicNameOrStringParameterContext symbolicNameOrStringParameter() {
return getRuleContext(SymbolicNameOrStringParameterContext.class,0);
}
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public CommandOptionsContext commandOptions() {
return getRuleContext(CommandOptionsContext.class,0);
}
public CreateConstraintContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createConstraint; }
}
public final CreateConstraintContext createConstraint() throws RecognitionException {
CreateConstraintContext _localctx = new CreateConstraintContext(_ctx, getState());
enterRule(_localctx, 352, RULE_createConstraint);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2291);
match(CONSTRAINT);
setState(2293);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,253,_ctx) ) {
case 1:
{
setState(2292);
symbolicNameOrStringParameter();
}
break;
}
setState(2298);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(2295);
match(IF);
setState(2296);
match(NOT);
setState(2297);
match(EXISTS);
}
}
setState(2300);
match(FOR);
setState(2303);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,255,_ctx) ) {
case 1:
{
setState(2301);
commandNodePattern();
}
break;
case 2:
{
setState(2302);
commandRelPattern();
}
break;
}
setState(2305);
constraintType();
setState(2307);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==OPTIONS) {
{
setState(2306);
commandOptions();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ConstraintTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_constraintType; }
public ConstraintTypeContext() { }
public void copyFrom(ConstraintTypeContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintTypedContext extends ConstraintTypeContext {
public TerminalNode REQUIRE() { return getToken(Cypher6Parser.REQUIRE, 0); }
public PropertyListContext propertyList() {
return getRuleContext(PropertyListContext.class,0);
}
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public TerminalNode COLONCOLON() { return getToken(Cypher6Parser.COLONCOLON, 0); }
public TerminalNode IS() { return getToken(Cypher6Parser.IS, 0); }
public TerminalNode TYPED() { return getToken(Cypher6Parser.TYPED, 0); }
public ConstraintTypedContext(ConstraintTypeContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintKeyContext extends ConstraintTypeContext {
public TerminalNode REQUIRE() { return getToken(Cypher6Parser.REQUIRE, 0); }
public PropertyListContext propertyList() {
return getRuleContext(PropertyListContext.class,0);
}
public TerminalNode IS() { return getToken(Cypher6Parser.IS, 0); }
public TerminalNode KEY() { return getToken(Cypher6Parser.KEY, 0); }
public TerminalNode NODE() { return getToken(Cypher6Parser.NODE, 0); }
public TerminalNode RELATIONSHIP() { return getToken(Cypher6Parser.RELATIONSHIP, 0); }
public TerminalNode REL() { return getToken(Cypher6Parser.REL, 0); }
public ConstraintKeyContext(ConstraintTypeContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintIsNotNullContext extends ConstraintTypeContext {
public TerminalNode REQUIRE() { return getToken(Cypher6Parser.REQUIRE, 0); }
public PropertyListContext propertyList() {
return getRuleContext(PropertyListContext.class,0);
}
public TerminalNode IS() { return getToken(Cypher6Parser.IS, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public TerminalNode NULL() { return getToken(Cypher6Parser.NULL, 0); }
public ConstraintIsNotNullContext(ConstraintTypeContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintIsUniqueContext extends ConstraintTypeContext {
public TerminalNode REQUIRE() { return getToken(Cypher6Parser.REQUIRE, 0); }
public PropertyListContext propertyList() {
return getRuleContext(PropertyListContext.class,0);
}
public TerminalNode IS() { return getToken(Cypher6Parser.IS, 0); }
public TerminalNode UNIQUE() { return getToken(Cypher6Parser.UNIQUE, 0); }
public TerminalNode NODE() { return getToken(Cypher6Parser.NODE, 0); }
public TerminalNode RELATIONSHIP() { return getToken(Cypher6Parser.RELATIONSHIP, 0); }
public TerminalNode REL() { return getToken(Cypher6Parser.REL, 0); }
public ConstraintIsUniqueContext(ConstraintTypeContext ctx) { copyFrom(ctx); }
}
public final ConstraintTypeContext constraintType() throws RecognitionException {
ConstraintTypeContext _localctx = new ConstraintTypeContext(_ctx, getState());
enterRule(_localctx, 354, RULE_constraintType);
int _la;
try {
setState(2340);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,260,_ctx) ) {
case 1:
_localctx = new ConstraintTypedContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(2309);
match(REQUIRE);
setState(2310);
propertyList();
setState(2314);
_errHandler.sync(this);
switch (_input.LA(1)) {
case COLONCOLON:
{
setState(2311);
match(COLONCOLON);
}
break;
case IS:
{
setState(2312);
match(IS);
setState(2313);
_la = _input.LA(1);
if ( !(_la==COLONCOLON || _la==TYPED) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(2316);
type();
}
break;
case 2:
_localctx = new ConstraintIsUniqueContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(2318);
match(REQUIRE);
setState(2319);
propertyList();
setState(2320);
match(IS);
setState(2322);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 170)) & ~0x3f) == 0 && ((1L << (_la - 170)) & 844424930131969L) != 0)) {
{
setState(2321);
_la = _input.LA(1);
if ( !(((((_la - 170)) & ~0x3f) == 0 && ((1L << (_la - 170)) & 844424930131969L) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(2324);
match(UNIQUE);
}
break;
case 3:
_localctx = new ConstraintKeyContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(2326);
match(REQUIRE);
setState(2327);
propertyList();
setState(2328);
match(IS);
setState(2330);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 170)) & ~0x3f) == 0 && ((1L << (_la - 170)) & 844424930131969L) != 0)) {
{
setState(2329);
_la = _input.LA(1);
if ( !(((((_la - 170)) & ~0x3f) == 0 && ((1L << (_la - 170)) & 844424930131969L) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(2332);
match(KEY);
}
break;
case 4:
_localctx = new ConstraintIsNotNullContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(2334);
match(REQUIRE);
setState(2335);
propertyList();
setState(2336);
match(IS);
setState(2337);
match(NOT);
setState(2338);
match(NULL);
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropConstraintContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CONSTRAINT() { return getToken(Cypher6Parser.CONSTRAINT, 0); }
public SymbolicNameOrStringParameterContext symbolicNameOrStringParameter() {
return getRuleContext(SymbolicNameOrStringParameterContext.class,0);
}
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public DropConstraintContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dropConstraint; }
}
public final DropConstraintContext dropConstraint() throws RecognitionException {
DropConstraintContext _localctx = new DropConstraintContext(_ctx, getState());
enterRule(_localctx, 356, RULE_dropConstraint);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2342);
match(CONSTRAINT);
setState(2343);
symbolicNameOrStringParameter();
setState(2346);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(2344);
match(IF);
setState(2345);
match(EXISTS);
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateIndexContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode BTREE() { return getToken(Cypher6Parser.BTREE, 0); }
public TerminalNode INDEX() { return getToken(Cypher6Parser.INDEX, 0); }
public CreateIndex_Context createIndex_() {
return getRuleContext(CreateIndex_Context.class,0);
}
public TerminalNode RANGE() { return getToken(Cypher6Parser.RANGE, 0); }
public TerminalNode TEXT() { return getToken(Cypher6Parser.TEXT, 0); }
public TerminalNode POINT() { return getToken(Cypher6Parser.POINT, 0); }
public TerminalNode VECTOR() { return getToken(Cypher6Parser.VECTOR, 0); }
public TerminalNode LOOKUP() { return getToken(Cypher6Parser.LOOKUP, 0); }
public CreateLookupIndexContext createLookupIndex() {
return getRuleContext(CreateLookupIndexContext.class,0);
}
public TerminalNode FULLTEXT() { return getToken(Cypher6Parser.FULLTEXT, 0); }
public CreateFulltextIndexContext createFulltextIndex() {
return getRuleContext(CreateFulltextIndexContext.class,0);
}
public CreateIndexContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createIndex; }
}
public final CreateIndexContext createIndex() throws RecognitionException {
CreateIndexContext _localctx = new CreateIndexContext(_ctx, getState());
enterRule(_localctx, 358, RULE_createIndex);
try {
setState(2371);
_errHandler.sync(this);
switch (_input.LA(1)) {
case BTREE:
enterOuterAlt(_localctx, 1);
{
setState(2348);
match(BTREE);
setState(2349);
match(INDEX);
setState(2350);
createIndex_();
}
break;
case RANGE:
enterOuterAlt(_localctx, 2);
{
setState(2351);
match(RANGE);
setState(2352);
match(INDEX);
setState(2353);
createIndex_();
}
break;
case TEXT:
enterOuterAlt(_localctx, 3);
{
setState(2354);
match(TEXT);
setState(2355);
match(INDEX);
setState(2356);
createIndex_();
}
break;
case POINT:
enterOuterAlt(_localctx, 4);
{
setState(2357);
match(POINT);
setState(2358);
match(INDEX);
setState(2359);
createIndex_();
}
break;
case VECTOR:
enterOuterAlt(_localctx, 5);
{
setState(2360);
match(VECTOR);
setState(2361);
match(INDEX);
setState(2362);
createIndex_();
}
break;
case LOOKUP:
enterOuterAlt(_localctx, 6);
{
setState(2363);
match(LOOKUP);
setState(2364);
match(INDEX);
setState(2365);
createLookupIndex();
}
break;
case FULLTEXT:
enterOuterAlt(_localctx, 7);
{
setState(2366);
match(FULLTEXT);
setState(2367);
match(INDEX);
setState(2368);
createFulltextIndex();
}
break;
case INDEX:
enterOuterAlt(_localctx, 8);
{
setState(2369);
match(INDEX);
setState(2370);
createIndex_();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateIndex_Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode FOR() { return getToken(Cypher6Parser.FOR, 0); }
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public PropertyListContext propertyList() {
return getRuleContext(PropertyListContext.class,0);
}
public CommandNodePatternContext commandNodePattern() {
return getRuleContext(CommandNodePatternContext.class,0);
}
public CommandRelPatternContext commandRelPattern() {
return getRuleContext(CommandRelPatternContext.class,0);
}
public SymbolicNameOrStringParameterContext symbolicNameOrStringParameter() {
return getRuleContext(SymbolicNameOrStringParameterContext.class,0);
}
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public CommandOptionsContext commandOptions() {
return getRuleContext(CommandOptionsContext.class,0);
}
public CreateIndex_Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createIndex_; }
}
public final CreateIndex_Context createIndex_() throws RecognitionException {
CreateIndex_Context _localctx = new CreateIndex_Context(_ctx, getState());
enterRule(_localctx, 360, RULE_createIndex_);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2374);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,263,_ctx) ) {
case 1:
{
setState(2373);
symbolicNameOrStringParameter();
}
break;
}
setState(2379);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(2376);
match(IF);
setState(2377);
match(NOT);
setState(2378);
match(EXISTS);
}
}
setState(2381);
match(FOR);
setState(2384);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,265,_ctx) ) {
case 1:
{
setState(2382);
commandNodePattern();
}
break;
case 2:
{
setState(2383);
commandRelPattern();
}
break;
}
setState(2386);
match(ON);
setState(2387);
propertyList();
setState(2389);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==OPTIONS) {
{
setState(2388);
commandOptions();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateFulltextIndexContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode FOR() { return getToken(Cypher6Parser.FOR, 0); }
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public TerminalNode EACH() { return getToken(Cypher6Parser.EACH, 0); }
public TerminalNode LBRACKET() { return getToken(Cypher6Parser.LBRACKET, 0); }
public EnclosedPropertyListContext enclosedPropertyList() {
return getRuleContext(EnclosedPropertyListContext.class,0);
}
public TerminalNode RBRACKET() { return getToken(Cypher6Parser.RBRACKET, 0); }
public FulltextNodePatternContext fulltextNodePattern() {
return getRuleContext(FulltextNodePatternContext.class,0);
}
public FulltextRelPatternContext fulltextRelPattern() {
return getRuleContext(FulltextRelPatternContext.class,0);
}
public SymbolicNameOrStringParameterContext symbolicNameOrStringParameter() {
return getRuleContext(SymbolicNameOrStringParameterContext.class,0);
}
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public CommandOptionsContext commandOptions() {
return getRuleContext(CommandOptionsContext.class,0);
}
public CreateFulltextIndexContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createFulltextIndex; }
}
public final CreateFulltextIndexContext createFulltextIndex() throws RecognitionException {
CreateFulltextIndexContext _localctx = new CreateFulltextIndexContext(_ctx, getState());
enterRule(_localctx, 362, RULE_createFulltextIndex);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2392);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,267,_ctx) ) {
case 1:
{
setState(2391);
symbolicNameOrStringParameter();
}
break;
}
setState(2397);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(2394);
match(IF);
setState(2395);
match(NOT);
setState(2396);
match(EXISTS);
}
}
setState(2399);
match(FOR);
setState(2402);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,269,_ctx) ) {
case 1:
{
setState(2400);
fulltextNodePattern();
}
break;
case 2:
{
setState(2401);
fulltextRelPattern();
}
break;
}
setState(2404);
match(ON);
setState(2405);
match(EACH);
setState(2406);
match(LBRACKET);
setState(2407);
enclosedPropertyList();
setState(2408);
match(RBRACKET);
setState(2410);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==OPTIONS) {
{
setState(2409);
commandOptions();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FulltextNodePatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public TerminalNode COLON() { return getToken(Cypher6Parser.COLON, 0); }
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class,i);
}
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public List BAR() { return getTokens(Cypher6Parser.BAR); }
public TerminalNode BAR(int i) {
return getToken(Cypher6Parser.BAR, i);
}
public FulltextNodePatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_fulltextNodePattern; }
}
public final FulltextNodePatternContext fulltextNodePattern() throws RecognitionException {
FulltextNodePatternContext _localctx = new FulltextNodePatternContext(_ctx, getState());
enterRule(_localctx, 364, RULE_fulltextNodePattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2412);
match(LPAREN);
setState(2413);
variable();
setState(2414);
match(COLON);
setState(2415);
symbolicNameString();
setState(2420);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==BAR) {
{
{
setState(2416);
match(BAR);
setState(2417);
symbolicNameString();
}
}
setState(2422);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2423);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FulltextRelPatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List LPAREN() { return getTokens(Cypher6Parser.LPAREN); }
public TerminalNode LPAREN(int i) {
return getToken(Cypher6Parser.LPAREN, i);
}
public List RPAREN() { return getTokens(Cypher6Parser.RPAREN); }
public TerminalNode RPAREN(int i) {
return getToken(Cypher6Parser.RPAREN, i);
}
public List arrowLine() {
return getRuleContexts(ArrowLineContext.class);
}
public ArrowLineContext arrowLine(int i) {
return getRuleContext(ArrowLineContext.class,i);
}
public TerminalNode LBRACKET() { return getToken(Cypher6Parser.LBRACKET, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public TerminalNode COLON() { return getToken(Cypher6Parser.COLON, 0); }
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class,i);
}
public TerminalNode RBRACKET() { return getToken(Cypher6Parser.RBRACKET, 0); }
public LeftArrowContext leftArrow() {
return getRuleContext(LeftArrowContext.class,0);
}
public List BAR() { return getTokens(Cypher6Parser.BAR); }
public TerminalNode BAR(int i) {
return getToken(Cypher6Parser.BAR, i);
}
public RightArrowContext rightArrow() {
return getRuleContext(RightArrowContext.class,0);
}
public FulltextRelPatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_fulltextRelPattern; }
}
public final FulltextRelPatternContext fulltextRelPattern() throws RecognitionException {
FulltextRelPatternContext _localctx = new FulltextRelPatternContext(_ctx, getState());
enterRule(_localctx, 366, RULE_fulltextRelPattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2425);
match(LPAREN);
setState(2426);
match(RPAREN);
setState(2428);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LT || _la==ARROW_LEFT_HEAD) {
{
setState(2427);
leftArrow();
}
}
setState(2430);
arrowLine();
setState(2431);
match(LBRACKET);
setState(2432);
variable();
setState(2433);
match(COLON);
setState(2434);
symbolicNameString();
setState(2439);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==BAR) {
{
{
setState(2435);
match(BAR);
setState(2436);
symbolicNameString();
}
}
setState(2441);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2442);
match(RBRACKET);
setState(2443);
arrowLine();
setState(2445);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==GT || _la==ARROW_RIGHT_HEAD) {
{
setState(2444);
rightArrow();
}
}
setState(2447);
match(LPAREN);
setState(2448);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateLookupIndexContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode FOR() { return getToken(Cypher6Parser.FOR, 0); }
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public LookupIndexNodePatternContext lookupIndexNodePattern() {
return getRuleContext(LookupIndexNodePatternContext.class,0);
}
public LookupIndexRelPatternContext lookupIndexRelPattern() {
return getRuleContext(LookupIndexRelPatternContext.class,0);
}
public SymbolicNameOrStringParameterContext symbolicNameOrStringParameter() {
return getRuleContext(SymbolicNameOrStringParameterContext.class,0);
}
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public CommandOptionsContext commandOptions() {
return getRuleContext(CommandOptionsContext.class,0);
}
public CreateLookupIndexContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createLookupIndex; }
}
public final CreateLookupIndexContext createLookupIndex() throws RecognitionException {
CreateLookupIndexContext _localctx = new CreateLookupIndexContext(_ctx, getState());
enterRule(_localctx, 368, RULE_createLookupIndex);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2451);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,275,_ctx) ) {
case 1:
{
setState(2450);
symbolicNameOrStringParameter();
}
break;
}
setState(2456);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(2453);
match(IF);
setState(2454);
match(NOT);
setState(2455);
match(EXISTS);
}
}
setState(2458);
match(FOR);
setState(2461);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,277,_ctx) ) {
case 1:
{
setState(2459);
lookupIndexNodePattern();
}
break;
case 2:
{
setState(2460);
lookupIndexRelPattern();
}
break;
}
setState(2463);
symbolicNameString();
setState(2464);
match(LPAREN);
setState(2465);
variable();
setState(2466);
match(RPAREN);
setState(2468);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==OPTIONS) {
{
setState(2467);
commandOptions();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LookupIndexNodePatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public TerminalNode EACH() { return getToken(Cypher6Parser.EACH, 0); }
public LookupIndexNodePatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_lookupIndexNodePattern; }
}
public final LookupIndexNodePatternContext lookupIndexNodePattern() throws RecognitionException {
LookupIndexNodePatternContext _localctx = new LookupIndexNodePatternContext(_ctx, getState());
enterRule(_localctx, 370, RULE_lookupIndexNodePattern);
try {
enterOuterAlt(_localctx, 1);
{
setState(2470);
match(LPAREN);
setState(2471);
variable();
setState(2472);
match(RPAREN);
setState(2473);
match(ON);
setState(2474);
match(EACH);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LookupIndexRelPatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List LPAREN() { return getTokens(Cypher6Parser.LPAREN); }
public TerminalNode LPAREN(int i) {
return getToken(Cypher6Parser.LPAREN, i);
}
public List RPAREN() { return getTokens(Cypher6Parser.RPAREN); }
public TerminalNode RPAREN(int i) {
return getToken(Cypher6Parser.RPAREN, i);
}
public List arrowLine() {
return getRuleContexts(ArrowLineContext.class);
}
public ArrowLineContext arrowLine(int i) {
return getRuleContext(ArrowLineContext.class,i);
}
public TerminalNode LBRACKET() { return getToken(Cypher6Parser.LBRACKET, 0); }
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public TerminalNode RBRACKET() { return getToken(Cypher6Parser.RBRACKET, 0); }
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public LeftArrowContext leftArrow() {
return getRuleContext(LeftArrowContext.class,0);
}
public RightArrowContext rightArrow() {
return getRuleContext(RightArrowContext.class,0);
}
public TerminalNode EACH() { return getToken(Cypher6Parser.EACH, 0); }
public LookupIndexRelPatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_lookupIndexRelPattern; }
}
public final LookupIndexRelPatternContext lookupIndexRelPattern() throws RecognitionException {
LookupIndexRelPatternContext _localctx = new LookupIndexRelPatternContext(_ctx, getState());
enterRule(_localctx, 372, RULE_lookupIndexRelPattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2476);
match(LPAREN);
setState(2477);
match(RPAREN);
setState(2479);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LT || _la==ARROW_LEFT_HEAD) {
{
setState(2478);
leftArrow();
}
}
setState(2481);
arrowLine();
setState(2482);
match(LBRACKET);
setState(2483);
variable();
setState(2484);
match(RBRACKET);
setState(2485);
arrowLine();
setState(2487);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==GT || _la==ARROW_RIGHT_HEAD) {
{
setState(2486);
rightArrow();
}
}
setState(2489);
match(LPAREN);
setState(2490);
match(RPAREN);
setState(2491);
match(ON);
setState(2493);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,281,_ctx) ) {
case 1:
{
setState(2492);
match(EACH);
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropIndexContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode INDEX() { return getToken(Cypher6Parser.INDEX, 0); }
public SymbolicNameOrStringParameterContext symbolicNameOrStringParameter() {
return getRuleContext(SymbolicNameOrStringParameterContext.class,0);
}
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public DropIndexContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dropIndex; }
}
public final DropIndexContext dropIndex() throws RecognitionException {
DropIndexContext _localctx = new DropIndexContext(_ctx, getState());
enterRule(_localctx, 374, RULE_dropIndex);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2495);
match(INDEX);
setState(2496);
symbolicNameOrStringParameter();
setState(2499);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(2497);
match(IF);
setState(2498);
match(EXISTS);
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PropertyListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public PropertyContext property() {
return getRuleContext(PropertyContext.class,0);
}
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public EnclosedPropertyListContext enclosedPropertyList() {
return getRuleContext(EnclosedPropertyListContext.class,0);
}
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public PropertyListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_propertyList; }
}
public final PropertyListContext propertyList() throws RecognitionException {
PropertyListContext _localctx = new PropertyListContext(_ctx, getState());
enterRule(_localctx, 376, RULE_propertyList);
try {
setState(2508);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 1);
{
setState(2501);
variable();
setState(2502);
property();
}
break;
case LPAREN:
enterOuterAlt(_localctx, 2);
{
setState(2504);
match(LPAREN);
setState(2505);
enclosedPropertyList();
setState(2506);
match(RPAREN);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class EnclosedPropertyListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List variable() {
return getRuleContexts(VariableContext.class);
}
public VariableContext variable(int i) {
return getRuleContext(VariableContext.class,i);
}
public List property() {
return getRuleContexts(PropertyContext.class);
}
public PropertyContext property(int i) {
return getRuleContext(PropertyContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public EnclosedPropertyListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_enclosedPropertyList; }
}
public final EnclosedPropertyListContext enclosedPropertyList() throws RecognitionException {
EnclosedPropertyListContext _localctx = new EnclosedPropertyListContext(_ctx, getState());
enterRule(_localctx, 378, RULE_enclosedPropertyList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2510);
variable();
setState(2511);
property();
setState(2518);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(2512);
match(COMMA);
setState(2513);
variable();
setState(2514);
property();
}
}
setState(2520);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ALTER() { return getToken(Cypher6Parser.ALTER, 0); }
public AlterAliasContext alterAlias() {
return getRuleContext(AlterAliasContext.class,0);
}
public AlterCurrentUserContext alterCurrentUser() {
return getRuleContext(AlterCurrentUserContext.class,0);
}
public AlterDatabaseContext alterDatabase() {
return getRuleContext(AlterDatabaseContext.class,0);
}
public AlterUserContext alterUser() {
return getRuleContext(AlterUserContext.class,0);
}
public AlterServerContext alterServer() {
return getRuleContext(AlterServerContext.class,0);
}
public AlterCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterCommand; }
}
public final AlterCommandContext alterCommand() throws RecognitionException {
AlterCommandContext _localctx = new AlterCommandContext(_ctx, getState());
enterRule(_localctx, 380, RULE_alterCommand);
try {
enterOuterAlt(_localctx, 1);
{
setState(2521);
match(ALTER);
setState(2527);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALIAS:
{
setState(2522);
alterAlias();
}
break;
case CURRENT:
{
setState(2523);
alterCurrentUser();
}
break;
case DATABASE:
{
setState(2524);
alterDatabase();
}
break;
case USER:
{
setState(2525);
alterUser();
}
break;
case SERVER:
{
setState(2526);
alterServer();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RenameCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode RENAME() { return getToken(Cypher6Parser.RENAME, 0); }
public RenameRoleContext renameRole() {
return getRuleContext(RenameRoleContext.class,0);
}
public RenameServerContext renameServer() {
return getRuleContext(RenameServerContext.class,0);
}
public RenameUserContext renameUser() {
return getRuleContext(RenameUserContext.class,0);
}
public RenameCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_renameCommand; }
}
public final RenameCommandContext renameCommand() throws RecognitionException {
RenameCommandContext _localctx = new RenameCommandContext(_ctx, getState());
enterRule(_localctx, 382, RULE_renameCommand);
try {
enterOuterAlt(_localctx, 1);
{
setState(2529);
match(RENAME);
setState(2533);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ROLE:
{
setState(2530);
renameRole();
}
break;
case SERVER:
{
setState(2531);
renameServer();
}
break;
case USER:
{
setState(2532);
renameUser();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GrantCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode GRANT() { return getToken(Cypher6Parser.GRANT, 0); }
public PrivilegeContext privilege() {
return getRuleContext(PrivilegeContext.class,0);
}
public TerminalNode TO() { return getToken(Cypher6Parser.TO, 0); }
public RoleNamesContext roleNames() {
return getRuleContext(RoleNamesContext.class,0);
}
public RoleTokenContext roleToken() {
return getRuleContext(RoleTokenContext.class,0);
}
public GrantRoleContext grantRole() {
return getRuleContext(GrantRoleContext.class,0);
}
public TerminalNode IMMUTABLE() { return getToken(Cypher6Parser.IMMUTABLE, 0); }
public GrantCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_grantCommand; }
}
public final GrantCommandContext grantCommand() throws RecognitionException {
GrantCommandContext _localctx = new GrantCommandContext(_ctx, getState());
enterRule(_localctx, 384, RULE_grantCommand);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2535);
match(GRANT);
setState(2546);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,288,_ctx) ) {
case 1:
{
setState(2537);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IMMUTABLE) {
{
setState(2536);
match(IMMUTABLE);
}
}
setState(2539);
privilege();
setState(2540);
match(TO);
setState(2541);
roleNames();
}
break;
case 2:
{
setState(2543);
roleToken();
setState(2544);
grantRole();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DenyCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DENY() { return getToken(Cypher6Parser.DENY, 0); }
public PrivilegeContext privilege() {
return getRuleContext(PrivilegeContext.class,0);
}
public TerminalNode TO() { return getToken(Cypher6Parser.TO, 0); }
public RoleNamesContext roleNames() {
return getRuleContext(RoleNamesContext.class,0);
}
public TerminalNode IMMUTABLE() { return getToken(Cypher6Parser.IMMUTABLE, 0); }
public DenyCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_denyCommand; }
}
public final DenyCommandContext denyCommand() throws RecognitionException {
DenyCommandContext _localctx = new DenyCommandContext(_ctx, getState());
enterRule(_localctx, 386, RULE_denyCommand);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2548);
match(DENY);
setState(2550);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IMMUTABLE) {
{
setState(2549);
match(IMMUTABLE);
}
}
setState(2552);
privilege();
setState(2553);
match(TO);
setState(2554);
roleNames();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RevokeCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode REVOKE() { return getToken(Cypher6Parser.REVOKE, 0); }
public PrivilegeContext privilege() {
return getRuleContext(PrivilegeContext.class,0);
}
public TerminalNode FROM() { return getToken(Cypher6Parser.FROM, 0); }
public RoleNamesContext roleNames() {
return getRuleContext(RoleNamesContext.class,0);
}
public RoleTokenContext roleToken() {
return getRuleContext(RoleTokenContext.class,0);
}
public RevokeRoleContext revokeRole() {
return getRuleContext(RevokeRoleContext.class,0);
}
public TerminalNode IMMUTABLE() { return getToken(Cypher6Parser.IMMUTABLE, 0); }
public TerminalNode DENY() { return getToken(Cypher6Parser.DENY, 0); }
public TerminalNode GRANT() { return getToken(Cypher6Parser.GRANT, 0); }
public RevokeCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_revokeCommand; }
}
public final RevokeCommandContext revokeCommand() throws RecognitionException {
RevokeCommandContext _localctx = new RevokeCommandContext(_ctx, getState());
enterRule(_localctx, 388, RULE_revokeCommand);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2556);
match(REVOKE);
setState(2570);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,292,_ctx) ) {
case 1:
{
setState(2558);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DENY || _la==GRANT) {
{
setState(2557);
_la = _input.LA(1);
if ( !(_la==DENY || _la==GRANT) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(2561);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IMMUTABLE) {
{
setState(2560);
match(IMMUTABLE);
}
}
setState(2563);
privilege();
setState(2564);
match(FROM);
setState(2565);
roleNames();
}
break;
case 2:
{
setState(2567);
roleToken();
setState(2568);
revokeRole();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UserNamesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicNameOrStringParameterListContext symbolicNameOrStringParameterList() {
return getRuleContext(SymbolicNameOrStringParameterListContext.class,0);
}
public UserNamesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_userNames; }
}
public final UserNamesContext userNames() throws RecognitionException {
UserNamesContext _localctx = new UserNamesContext(_ctx, getState());
enterRule(_localctx, 390, RULE_userNames);
try {
enterOuterAlt(_localctx, 1);
{
setState(2572);
symbolicNameOrStringParameterList();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RoleNamesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicNameOrStringParameterListContext symbolicNameOrStringParameterList() {
return getRuleContext(SymbolicNameOrStringParameterListContext.class,0);
}
public RoleNamesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_roleNames; }
}
public final RoleNamesContext roleNames() throws RecognitionException {
RoleNamesContext _localctx = new RoleNamesContext(_ctx, getState());
enterRule(_localctx, 392, RULE_roleNames);
try {
enterOuterAlt(_localctx, 1);
{
setState(2574);
symbolicNameOrStringParameterList();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RoleTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ROLES() { return getToken(Cypher6Parser.ROLES, 0); }
public TerminalNode ROLE() { return getToken(Cypher6Parser.ROLE, 0); }
public RoleTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_roleToken; }
}
public final RoleTokenContext roleToken() throws RecognitionException {
RoleTokenContext _localctx = new RoleTokenContext(_ctx, getState());
enterRule(_localctx, 394, RULE_roleToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2576);
_la = _input.LA(1);
if ( !(_la==ROLE || _la==ROLES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class EnableServerCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ENABLE() { return getToken(Cypher6Parser.ENABLE, 0); }
public TerminalNode SERVER() { return getToken(Cypher6Parser.SERVER, 0); }
public StringOrParameterContext stringOrParameter() {
return getRuleContext(StringOrParameterContext.class,0);
}
public CommandOptionsContext commandOptions() {
return getRuleContext(CommandOptionsContext.class,0);
}
public EnableServerCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_enableServerCommand; }
}
public final EnableServerCommandContext enableServerCommand() throws RecognitionException {
EnableServerCommandContext _localctx = new EnableServerCommandContext(_ctx, getState());
enterRule(_localctx, 396, RULE_enableServerCommand);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2578);
match(ENABLE);
setState(2579);
match(SERVER);
setState(2580);
stringOrParameter();
setState(2582);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==OPTIONS) {
{
setState(2581);
commandOptions();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterServerContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SERVER() { return getToken(Cypher6Parser.SERVER, 0); }
public StringOrParameterContext stringOrParameter() {
return getRuleContext(StringOrParameterContext.class,0);
}
public TerminalNode SET() { return getToken(Cypher6Parser.SET, 0); }
public CommandOptionsContext commandOptions() {
return getRuleContext(CommandOptionsContext.class,0);
}
public AlterServerContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterServer; }
}
public final AlterServerContext alterServer() throws RecognitionException {
AlterServerContext _localctx = new AlterServerContext(_ctx, getState());
enterRule(_localctx, 398, RULE_alterServer);
try {
enterOuterAlt(_localctx, 1);
{
setState(2584);
match(SERVER);
setState(2585);
stringOrParameter();
setState(2586);
match(SET);
setState(2587);
commandOptions();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RenameServerContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SERVER() { return getToken(Cypher6Parser.SERVER, 0); }
public List stringOrParameter() {
return getRuleContexts(StringOrParameterContext.class);
}
public StringOrParameterContext stringOrParameter(int i) {
return getRuleContext(StringOrParameterContext.class,i);
}
public TerminalNode TO() { return getToken(Cypher6Parser.TO, 0); }
public RenameServerContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_renameServer; }
}
public final RenameServerContext renameServer() throws RecognitionException {
RenameServerContext _localctx = new RenameServerContext(_ctx, getState());
enterRule(_localctx, 400, RULE_renameServer);
try {
enterOuterAlt(_localctx, 1);
{
setState(2589);
match(SERVER);
setState(2590);
stringOrParameter();
setState(2591);
match(TO);
setState(2592);
stringOrParameter();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropServerContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SERVER() { return getToken(Cypher6Parser.SERVER, 0); }
public StringOrParameterContext stringOrParameter() {
return getRuleContext(StringOrParameterContext.class,0);
}
public DropServerContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dropServer; }
}
public final DropServerContext dropServer() throws RecognitionException {
DropServerContext _localctx = new DropServerContext(_ctx, getState());
enterRule(_localctx, 402, RULE_dropServer);
try {
enterOuterAlt(_localctx, 1);
{
setState(2594);
match(SERVER);
setState(2595);
stringOrParameter();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowServersContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SERVER() { return getToken(Cypher6Parser.SERVER, 0); }
public TerminalNode SERVERS() { return getToken(Cypher6Parser.SERVERS, 0); }
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class,0);
}
public ShowServersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showServers; }
}
public final ShowServersContext showServers() throws RecognitionException {
ShowServersContext _localctx = new ShowServersContext(_ctx, getState());
enterRule(_localctx, 404, RULE_showServers);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2597);
_la = _input.LA(1);
if ( !(_la==SERVER || _la==SERVERS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2599);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(2598);
showCommandYield();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AllocationCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public DeallocateDatabaseFromServersContext deallocateDatabaseFromServers() {
return getRuleContext(DeallocateDatabaseFromServersContext.class,0);
}
public ReallocateDatabasesContext reallocateDatabases() {
return getRuleContext(ReallocateDatabasesContext.class,0);
}
public TerminalNode DRYRUN() { return getToken(Cypher6Parser.DRYRUN, 0); }
public AllocationCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_allocationCommand; }
}
public final AllocationCommandContext allocationCommand() throws RecognitionException {
AllocationCommandContext _localctx = new AllocationCommandContext(_ctx, getState());
enterRule(_localctx, 406, RULE_allocationCommand);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2602);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DRYRUN) {
{
setState(2601);
match(DRYRUN);
}
}
setState(2606);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DEALLOCATE:
{
setState(2604);
deallocateDatabaseFromServers();
}
break;
case REALLOCATE:
{
setState(2605);
reallocateDatabases();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DeallocateDatabaseFromServersContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DEALLOCATE() { return getToken(Cypher6Parser.DEALLOCATE, 0); }
public TerminalNode FROM() { return getToken(Cypher6Parser.FROM, 0); }
public List stringOrParameter() {
return getRuleContexts(StringOrParameterContext.class);
}
public StringOrParameterContext stringOrParameter(int i) {
return getRuleContext(StringOrParameterContext.class,i);
}
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public TerminalNode DATABASES() { return getToken(Cypher6Parser.DATABASES, 0); }
public TerminalNode SERVER() { return getToken(Cypher6Parser.SERVER, 0); }
public TerminalNode SERVERS() { return getToken(Cypher6Parser.SERVERS, 0); }
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public DeallocateDatabaseFromServersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_deallocateDatabaseFromServers; }
}
public final DeallocateDatabaseFromServersContext deallocateDatabaseFromServers() throws RecognitionException {
DeallocateDatabaseFromServersContext _localctx = new DeallocateDatabaseFromServersContext(_ctx, getState());
enterRule(_localctx, 408, RULE_deallocateDatabaseFromServers);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2608);
match(DEALLOCATE);
setState(2609);
_la = _input.LA(1);
if ( !(_la==DATABASE || _la==DATABASES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2610);
match(FROM);
setState(2611);
_la = _input.LA(1);
if ( !(_la==SERVER || _la==SERVERS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2612);
stringOrParameter();
setState(2617);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(2613);
match(COMMA);
setState(2614);
stringOrParameter();
}
}
setState(2619);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ReallocateDatabasesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode REALLOCATE() { return getToken(Cypher6Parser.REALLOCATE, 0); }
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public TerminalNode DATABASES() { return getToken(Cypher6Parser.DATABASES, 0); }
public ReallocateDatabasesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_reallocateDatabases; }
}
public final ReallocateDatabasesContext reallocateDatabases() throws RecognitionException {
ReallocateDatabasesContext _localctx = new ReallocateDatabasesContext(_ctx, getState());
enterRule(_localctx, 410, RULE_reallocateDatabases);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2620);
match(REALLOCATE);
setState(2621);
_la = _input.LA(1);
if ( !(_la==DATABASE || _la==DATABASES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateRoleContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ROLE() { return getToken(Cypher6Parser.ROLE, 0); }
public List commandNameExpression() {
return getRuleContexts(CommandNameExpressionContext.class);
}
public CommandNameExpressionContext commandNameExpression(int i) {
return getRuleContext(CommandNameExpressionContext.class,i);
}
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public TerminalNode AS() { return getToken(Cypher6Parser.AS, 0); }
public TerminalNode COPY() { return getToken(Cypher6Parser.COPY, 0); }
public TerminalNode OF() { return getToken(Cypher6Parser.OF, 0); }
public CreateRoleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createRole; }
}
public final CreateRoleContext createRole() throws RecognitionException {
CreateRoleContext _localctx = new CreateRoleContext(_ctx, getState());
enterRule(_localctx, 412, RULE_createRole);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2623);
match(ROLE);
setState(2624);
commandNameExpression();
setState(2628);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(2625);
match(IF);
setState(2626);
match(NOT);
setState(2627);
match(EXISTS);
}
}
setState(2634);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AS) {
{
setState(2630);
match(AS);
setState(2631);
match(COPY);
setState(2632);
match(OF);
setState(2633);
commandNameExpression();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropRoleContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ROLE() { return getToken(Cypher6Parser.ROLE, 0); }
public CommandNameExpressionContext commandNameExpression() {
return getRuleContext(CommandNameExpressionContext.class,0);
}
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public DropRoleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dropRole; }
}
public final DropRoleContext dropRole() throws RecognitionException {
DropRoleContext _localctx = new DropRoleContext(_ctx, getState());
enterRule(_localctx, 414, RULE_dropRole);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2636);
match(ROLE);
setState(2637);
commandNameExpression();
setState(2640);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(2638);
match(IF);
setState(2639);
match(EXISTS);
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RenameRoleContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ROLE() { return getToken(Cypher6Parser.ROLE, 0); }
public List commandNameExpression() {
return getRuleContexts(CommandNameExpressionContext.class);
}
public CommandNameExpressionContext commandNameExpression(int i) {
return getRuleContext(CommandNameExpressionContext.class,i);
}
public TerminalNode TO() { return getToken(Cypher6Parser.TO, 0); }
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public RenameRoleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_renameRole; }
}
public final RenameRoleContext renameRole() throws RecognitionException {
RenameRoleContext _localctx = new RenameRoleContext(_ctx, getState());
enterRule(_localctx, 416, RULE_renameRole);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2642);
match(ROLE);
setState(2643);
commandNameExpression();
setState(2646);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(2644);
match(IF);
setState(2645);
match(EXISTS);
}
}
setState(2648);
match(TO);
setState(2649);
commandNameExpression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowRolesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public RoleTokenContext roleToken() {
return getRuleContext(RoleTokenContext.class,0);
}
public TerminalNode WITH() { return getToken(Cypher6Parser.WITH, 0); }
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class,0);
}
public TerminalNode ALL() { return getToken(Cypher6Parser.ALL, 0); }
public TerminalNode POPULATED() { return getToken(Cypher6Parser.POPULATED, 0); }
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public TerminalNode USERS() { return getToken(Cypher6Parser.USERS, 0); }
public ShowRolesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showRoles; }
}
public final ShowRolesContext showRoles() throws RecognitionException {
ShowRolesContext _localctx = new ShowRolesContext(_ctx, getState());
enterRule(_localctx, 418, RULE_showRoles);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2652);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==ALL || _la==POPULATED) {
{
setState(2651);
_la = _input.LA(1);
if ( !(_la==ALL || _la==POPULATED) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(2654);
roleToken();
setState(2657);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WITH) {
{
setState(2655);
match(WITH);
setState(2656);
_la = _input.LA(1);
if ( !(_la==USER || _la==USERS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(2660);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(2659);
showCommandYield();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GrantRoleContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public RoleNamesContext roleNames() {
return getRuleContext(RoleNamesContext.class,0);
}
public TerminalNode TO() { return getToken(Cypher6Parser.TO, 0); }
public UserNamesContext userNames() {
return getRuleContext(UserNamesContext.class,0);
}
public GrantRoleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_grantRole; }
}
public final GrantRoleContext grantRole() throws RecognitionException {
GrantRoleContext _localctx = new GrantRoleContext(_ctx, getState());
enterRule(_localctx, 420, RULE_grantRole);
try {
enterOuterAlt(_localctx, 1);
{
setState(2662);
roleNames();
setState(2663);
match(TO);
setState(2664);
userNames();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RevokeRoleContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public RoleNamesContext roleNames() {
return getRuleContext(RoleNamesContext.class,0);
}
public TerminalNode FROM() { return getToken(Cypher6Parser.FROM, 0); }
public UserNamesContext userNames() {
return getRuleContext(UserNamesContext.class,0);
}
public RevokeRoleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_revokeRole; }
}
public final RevokeRoleContext revokeRole() throws RecognitionException {
RevokeRoleContext _localctx = new RevokeRoleContext(_ctx, getState());
enterRule(_localctx, 422, RULE_revokeRole);
try {
enterOuterAlt(_localctx, 1);
{
setState(2666);
roleNames();
setState(2667);
match(FROM);
setState(2668);
userNames();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateUserContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public CommandNameExpressionContext commandNameExpression() {
return getRuleContext(CommandNameExpressionContext.class,0);
}
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public List SET() { return getTokens(Cypher6Parser.SET); }
public TerminalNode SET(int i) {
return getToken(Cypher6Parser.SET, i);
}
public List password() {
return getRuleContexts(PasswordContext.class);
}
public PasswordContext password(int i) {
return getRuleContext(PasswordContext.class,i);
}
public List PASSWORD() { return getTokens(Cypher6Parser.PASSWORD); }
public TerminalNode PASSWORD(int i) {
return getToken(Cypher6Parser.PASSWORD, i);
}
public List passwordChangeRequired() {
return getRuleContexts(PasswordChangeRequiredContext.class);
}
public PasswordChangeRequiredContext passwordChangeRequired(int i) {
return getRuleContext(PasswordChangeRequiredContext.class,i);
}
public List userStatus() {
return getRuleContexts(UserStatusContext.class);
}
public UserStatusContext userStatus(int i) {
return getRuleContext(UserStatusContext.class,i);
}
public List homeDatabase() {
return getRuleContexts(HomeDatabaseContext.class);
}
public HomeDatabaseContext homeDatabase(int i) {
return getRuleContext(HomeDatabaseContext.class,i);
}
public List setAuthClause() {
return getRuleContexts(SetAuthClauseContext.class);
}
public SetAuthClauseContext setAuthClause(int i) {
return getRuleContext(SetAuthClauseContext.class,i);
}
public CreateUserContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createUser; }
}
public final CreateUserContext createUser() throws RecognitionException {
CreateUserContext _localctx = new CreateUserContext(_ctx, getState());
enterRule(_localctx, 424, RULE_createUser);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2670);
match(USER);
setState(2671);
commandNameExpression();
setState(2675);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(2672);
match(IF);
setState(2673);
match(NOT);
setState(2674);
match(EXISTS);
}
}
setState(2686);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(2677);
match(SET);
setState(2684);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,306,_ctx) ) {
case 1:
{
setState(2678);
password();
}
break;
case 2:
{
setState(2679);
match(PASSWORD);
setState(2680);
passwordChangeRequired();
}
break;
case 3:
{
setState(2681);
userStatus();
}
break;
case 4:
{
setState(2682);
homeDatabase();
}
break;
case 5:
{
setState(2683);
setAuthClause();
}
break;
}
}
}
setState(2688);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==SET );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropUserContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public CommandNameExpressionContext commandNameExpression() {
return getRuleContext(CommandNameExpressionContext.class,0);
}
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public DropUserContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dropUser; }
}
public final DropUserContext dropUser() throws RecognitionException {
DropUserContext _localctx = new DropUserContext(_ctx, getState());
enterRule(_localctx, 426, RULE_dropUser);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2690);
match(USER);
setState(2691);
commandNameExpression();
setState(2694);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(2692);
match(IF);
setState(2693);
match(EXISTS);
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RenameUserContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public List commandNameExpression() {
return getRuleContexts(CommandNameExpressionContext.class);
}
public CommandNameExpressionContext commandNameExpression(int i) {
return getRuleContext(CommandNameExpressionContext.class,i);
}
public TerminalNode TO() { return getToken(Cypher6Parser.TO, 0); }
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public RenameUserContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_renameUser; }
}
public final RenameUserContext renameUser() throws RecognitionException {
RenameUserContext _localctx = new RenameUserContext(_ctx, getState());
enterRule(_localctx, 428, RULE_renameUser);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2696);
match(USER);
setState(2697);
commandNameExpression();
setState(2700);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(2698);
match(IF);
setState(2699);
match(EXISTS);
}
}
setState(2702);
match(TO);
setState(2703);
commandNameExpression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterCurrentUserContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CURRENT() { return getToken(Cypher6Parser.CURRENT, 0); }
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public TerminalNode SET() { return getToken(Cypher6Parser.SET, 0); }
public TerminalNode PASSWORD() { return getToken(Cypher6Parser.PASSWORD, 0); }
public TerminalNode FROM() { return getToken(Cypher6Parser.FROM, 0); }
public List passwordExpression() {
return getRuleContexts(PasswordExpressionContext.class);
}
public PasswordExpressionContext passwordExpression(int i) {
return getRuleContext(PasswordExpressionContext.class,i);
}
public TerminalNode TO() { return getToken(Cypher6Parser.TO, 0); }
public AlterCurrentUserContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterCurrentUser; }
}
public final AlterCurrentUserContext alterCurrentUser() throws RecognitionException {
AlterCurrentUserContext _localctx = new AlterCurrentUserContext(_ctx, getState());
enterRule(_localctx, 430, RULE_alterCurrentUser);
try {
enterOuterAlt(_localctx, 1);
{
setState(2705);
match(CURRENT);
setState(2706);
match(USER);
setState(2707);
match(SET);
setState(2708);
match(PASSWORD);
setState(2709);
match(FROM);
setState(2710);
passwordExpression();
setState(2711);
match(TO);
setState(2712);
passwordExpression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterUserContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public CommandNameExpressionContext commandNameExpression() {
return getRuleContext(CommandNameExpressionContext.class,0);
}
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public List REMOVE() { return getTokens(Cypher6Parser.REMOVE); }
public TerminalNode REMOVE(int i) {
return getToken(Cypher6Parser.REMOVE, i);
}
public List SET() { return getTokens(Cypher6Parser.SET); }
public TerminalNode SET(int i) {
return getToken(Cypher6Parser.SET, i);
}
public List HOME() { return getTokens(Cypher6Parser.HOME); }
public TerminalNode HOME(int i) {
return getToken(Cypher6Parser.HOME, i);
}
public List DATABASE() { return getTokens(Cypher6Parser.DATABASE); }
public TerminalNode DATABASE(int i) {
return getToken(Cypher6Parser.DATABASE, i);
}
public List ALL() { return getTokens(Cypher6Parser.ALL); }
public TerminalNode ALL(int i) {
return getToken(Cypher6Parser.ALL, i);
}
public List AUTH() { return getTokens(Cypher6Parser.AUTH); }
public TerminalNode AUTH(int i) {
return getToken(Cypher6Parser.AUTH, i);
}
public List removeNamedProvider() {
return getRuleContexts(RemoveNamedProviderContext.class);
}
public RemoveNamedProviderContext removeNamedProvider(int i) {
return getRuleContext(RemoveNamedProviderContext.class,i);
}
public List password() {
return getRuleContexts(PasswordContext.class);
}
public PasswordContext password(int i) {
return getRuleContext(PasswordContext.class,i);
}
public List PASSWORD() { return getTokens(Cypher6Parser.PASSWORD); }
public TerminalNode PASSWORD(int i) {
return getToken(Cypher6Parser.PASSWORD, i);
}
public List passwordChangeRequired() {
return getRuleContexts(PasswordChangeRequiredContext.class);
}
public PasswordChangeRequiredContext passwordChangeRequired(int i) {
return getRuleContext(PasswordChangeRequiredContext.class,i);
}
public List userStatus() {
return getRuleContexts(UserStatusContext.class);
}
public UserStatusContext userStatus(int i) {
return getRuleContext(UserStatusContext.class,i);
}
public List homeDatabase() {
return getRuleContexts(HomeDatabaseContext.class);
}
public HomeDatabaseContext homeDatabase(int i) {
return getRuleContext(HomeDatabaseContext.class,i);
}
public List setAuthClause() {
return getRuleContexts(SetAuthClauseContext.class);
}
public SetAuthClauseContext setAuthClause(int i) {
return getRuleContext(SetAuthClauseContext.class,i);
}
public List PROVIDER() { return getTokens(Cypher6Parser.PROVIDER); }
public TerminalNode PROVIDER(int i) {
return getToken(Cypher6Parser.PROVIDER, i);
}
public List PROVIDERS() { return getTokens(Cypher6Parser.PROVIDERS); }
public TerminalNode PROVIDERS(int i) {
return getToken(Cypher6Parser.PROVIDERS, i);
}
public AlterUserContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterUser; }
}
public final AlterUserContext alterUser() throws RecognitionException {
AlterUserContext _localctx = new AlterUserContext(_ctx, getState());
enterRule(_localctx, 432, RULE_alterUser);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2714);
match(USER);
setState(2715);
commandNameExpression();
setState(2718);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(2716);
match(IF);
setState(2717);
match(EXISTS);
}
}
setState(2733);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==REMOVE) {
{
{
setState(2720);
match(REMOVE);
setState(2729);
_errHandler.sync(this);
switch (_input.LA(1)) {
case HOME:
{
setState(2721);
match(HOME);
setState(2722);
match(DATABASE);
}
break;
case ALL:
{
setState(2723);
match(ALL);
setState(2724);
match(AUTH);
setState(2726);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==PROVIDER || _la==PROVIDERS) {
{
setState(2725);
_la = _input.LA(1);
if ( !(_la==PROVIDER || _la==PROVIDERS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
}
break;
case AUTH:
{
setState(2728);
removeNamedProvider();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
setState(2735);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2747);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==SET) {
{
{
setState(2736);
match(SET);
setState(2743);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,314,_ctx) ) {
case 1:
{
setState(2737);
password();
}
break;
case 2:
{
setState(2738);
match(PASSWORD);
setState(2739);
passwordChangeRequired();
}
break;
case 3:
{
setState(2740);
userStatus();
}
break;
case 4:
{
setState(2741);
homeDatabase();
}
break;
case 5:
{
setState(2742);
setAuthClause();
}
break;
}
}
}
setState(2749);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RemoveNamedProviderContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode AUTH() { return getToken(Cypher6Parser.AUTH, 0); }
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public StringListLiteralContext stringListLiteral() {
return getRuleContext(StringListLiteralContext.class,0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class,0);
}
public TerminalNode PROVIDER() { return getToken(Cypher6Parser.PROVIDER, 0); }
public TerminalNode PROVIDERS() { return getToken(Cypher6Parser.PROVIDERS, 0); }
public RemoveNamedProviderContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_removeNamedProvider; }
}
public final RemoveNamedProviderContext removeNamedProvider() throws RecognitionException {
RemoveNamedProviderContext _localctx = new RemoveNamedProviderContext(_ctx, getState());
enterRule(_localctx, 434, RULE_removeNamedProvider);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2750);
match(AUTH);
setState(2752);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==PROVIDER || _la==PROVIDERS) {
{
setState(2751);
_la = _input.LA(1);
if ( !(_la==PROVIDER || _la==PROVIDERS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(2757);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STRING_LITERAL1:
case STRING_LITERAL2:
{
setState(2754);
stringLiteral();
}
break;
case LBRACKET:
{
setState(2755);
stringListLiteral();
}
break;
case DOLLAR:
{
setState(2756);
parameter("ANY");
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PasswordContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PASSWORD() { return getToken(Cypher6Parser.PASSWORD, 0); }
public PasswordExpressionContext passwordExpression() {
return getRuleContext(PasswordExpressionContext.class,0);
}
public PasswordChangeRequiredContext passwordChangeRequired() {
return getRuleContext(PasswordChangeRequiredContext.class,0);
}
public TerminalNode PLAINTEXT() { return getToken(Cypher6Parser.PLAINTEXT, 0); }
public TerminalNode ENCRYPTED() { return getToken(Cypher6Parser.ENCRYPTED, 0); }
public PasswordContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_password; }
}
public final PasswordContext password() throws RecognitionException {
PasswordContext _localctx = new PasswordContext(_ctx, getState());
enterRule(_localctx, 436, RULE_password);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2760);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==ENCRYPTED || _la==PLAINTEXT) {
{
setState(2759);
_la = _input.LA(1);
if ( !(_la==ENCRYPTED || _la==PLAINTEXT) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(2762);
match(PASSWORD);
setState(2763);
passwordExpression();
setState(2765);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==CHANGE) {
{
setState(2764);
passwordChangeRequired();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PasswordOnlyContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PASSWORD() { return getToken(Cypher6Parser.PASSWORD, 0); }
public PasswordExpressionContext passwordExpression() {
return getRuleContext(PasswordExpressionContext.class,0);
}
public TerminalNode PLAINTEXT() { return getToken(Cypher6Parser.PLAINTEXT, 0); }
public TerminalNode ENCRYPTED() { return getToken(Cypher6Parser.ENCRYPTED, 0); }
public PasswordOnlyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_passwordOnly; }
}
public final PasswordOnlyContext passwordOnly() throws RecognitionException {
PasswordOnlyContext _localctx = new PasswordOnlyContext(_ctx, getState());
enterRule(_localctx, 438, RULE_passwordOnly);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2768);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==ENCRYPTED || _la==PLAINTEXT) {
{
setState(2767);
_la = _input.LA(1);
if ( !(_la==ENCRYPTED || _la==PLAINTEXT) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(2770);
match(PASSWORD);
setState(2771);
passwordExpression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PasswordExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class,0);
}
public PasswordExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_passwordExpression; }
}
public final PasswordExpressionContext passwordExpression() throws RecognitionException {
PasswordExpressionContext _localctx = new PasswordExpressionContext(_ctx, getState());
enterRule(_localctx, 440, RULE_passwordExpression);
try {
setState(2775);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STRING_LITERAL1:
case STRING_LITERAL2:
enterOuterAlt(_localctx, 1);
{
setState(2773);
stringLiteral();
}
break;
case DOLLAR:
enterOuterAlt(_localctx, 2);
{
setState(2774);
parameter("STRING");
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PasswordChangeRequiredContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CHANGE() { return getToken(Cypher6Parser.CHANGE, 0); }
public TerminalNode REQUIRED() { return getToken(Cypher6Parser.REQUIRED, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public PasswordChangeRequiredContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_passwordChangeRequired; }
}
public final PasswordChangeRequiredContext passwordChangeRequired() throws RecognitionException {
PasswordChangeRequiredContext _localctx = new PasswordChangeRequiredContext(_ctx, getState());
enterRule(_localctx, 442, RULE_passwordChangeRequired);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2777);
match(CHANGE);
setState(2779);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==NOT) {
{
setState(2778);
match(NOT);
}
}
setState(2781);
match(REQUIRED);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UserStatusContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode STATUS() { return getToken(Cypher6Parser.STATUS, 0); }
public TerminalNode SUSPENDED() { return getToken(Cypher6Parser.SUSPENDED, 0); }
public TerminalNode ACTIVE() { return getToken(Cypher6Parser.ACTIVE, 0); }
public UserStatusContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_userStatus; }
}
public final UserStatusContext userStatus() throws RecognitionException {
UserStatusContext _localctx = new UserStatusContext(_ctx, getState());
enterRule(_localctx, 444, RULE_userStatus);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2783);
match(STATUS);
setState(2784);
_la = _input.LA(1);
if ( !(_la==ACTIVE || _la==SUSPENDED) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class HomeDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode HOME() { return getToken(Cypher6Parser.HOME, 0); }
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class,0);
}
public HomeDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_homeDatabase; }
}
public final HomeDatabaseContext homeDatabase() throws RecognitionException {
HomeDatabaseContext _localctx = new HomeDatabaseContext(_ctx, getState());
enterRule(_localctx, 446, RULE_homeDatabase);
try {
enterOuterAlt(_localctx, 1);
{
setState(2786);
match(HOME);
setState(2787);
match(DATABASE);
setState(2788);
symbolicAliasNameOrParameter();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SetAuthClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode AUTH() { return getToken(Cypher6Parser.AUTH, 0); }
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public TerminalNode LCURLY() { return getToken(Cypher6Parser.LCURLY, 0); }
public TerminalNode RCURLY() { return getToken(Cypher6Parser.RCURLY, 0); }
public TerminalNode PROVIDER() { return getToken(Cypher6Parser.PROVIDER, 0); }
public List SET() { return getTokens(Cypher6Parser.SET); }
public TerminalNode SET(int i) {
return getToken(Cypher6Parser.SET, i);
}
public List userAuthAttribute() {
return getRuleContexts(UserAuthAttributeContext.class);
}
public UserAuthAttributeContext userAuthAttribute(int i) {
return getRuleContext(UserAuthAttributeContext.class,i);
}
public SetAuthClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_setAuthClause; }
}
public final SetAuthClauseContext setAuthClause() throws RecognitionException {
SetAuthClauseContext _localctx = new SetAuthClauseContext(_ctx, getState());
enterRule(_localctx, 448, RULE_setAuthClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2790);
match(AUTH);
setState(2792);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==PROVIDER) {
{
setState(2791);
match(PROVIDER);
}
}
setState(2794);
stringLiteral();
setState(2795);
match(LCURLY);
setState(2798);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(2796);
match(SET);
{
setState(2797);
userAuthAttribute();
}
}
}
setState(2800);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==SET );
setState(2802);
match(RCURLY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UserAuthAttributeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ID() { return getToken(Cypher6Parser.ID, 0); }
public StringOrParameterExpressionContext stringOrParameterExpression() {
return getRuleContext(StringOrParameterExpressionContext.class,0);
}
public PasswordOnlyContext passwordOnly() {
return getRuleContext(PasswordOnlyContext.class,0);
}
public TerminalNode PASSWORD() { return getToken(Cypher6Parser.PASSWORD, 0); }
public PasswordChangeRequiredContext passwordChangeRequired() {
return getRuleContext(PasswordChangeRequiredContext.class,0);
}
public UserAuthAttributeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_userAuthAttribute; }
}
public final UserAuthAttributeContext userAuthAttribute() throws RecognitionException {
UserAuthAttributeContext _localctx = new UserAuthAttributeContext(_ctx, getState());
enterRule(_localctx, 450, RULE_userAuthAttribute);
try {
setState(2809);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,325,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(2804);
match(ID);
setState(2805);
stringOrParameterExpression();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(2806);
passwordOnly();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(2807);
match(PASSWORD);
setState(2808);
passwordChangeRequired();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowUsersContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public TerminalNode USERS() { return getToken(Cypher6Parser.USERS, 0); }
public TerminalNode WITH() { return getToken(Cypher6Parser.WITH, 0); }
public TerminalNode AUTH() { return getToken(Cypher6Parser.AUTH, 0); }
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class,0);
}
public ShowUsersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showUsers; }
}
public final ShowUsersContext showUsers() throws RecognitionException {
ShowUsersContext _localctx = new ShowUsersContext(_ctx, getState());
enterRule(_localctx, 452, RULE_showUsers);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2811);
_la = _input.LA(1);
if ( !(_la==USER || _la==USERS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2814);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WITH) {
{
setState(2812);
match(WITH);
setState(2813);
match(AUTH);
}
}
setState(2817);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(2816);
showCommandYield();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowCurrentUserContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CURRENT() { return getToken(Cypher6Parser.CURRENT, 0); }
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class,0);
}
public ShowCurrentUserContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showCurrentUser; }
}
public final ShowCurrentUserContext showCurrentUser() throws RecognitionException {
ShowCurrentUserContext _localctx = new ShowCurrentUserContext(_ctx, getState());
enterRule(_localctx, 454, RULE_showCurrentUser);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2819);
match(CURRENT);
setState(2820);
match(USER);
setState(2822);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(2821);
showCommandYield();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowSupportedPrivilegesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SUPPORTED() { return getToken(Cypher6Parser.SUPPORTED, 0); }
public PrivilegeTokenContext privilegeToken() {
return getRuleContext(PrivilegeTokenContext.class,0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class,0);
}
public ShowSupportedPrivilegesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showSupportedPrivileges; }
}
public final ShowSupportedPrivilegesContext showSupportedPrivileges() throws RecognitionException {
ShowSupportedPrivilegesContext _localctx = new ShowSupportedPrivilegesContext(_ctx, getState());
enterRule(_localctx, 456, RULE_showSupportedPrivileges);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2824);
match(SUPPORTED);
setState(2825);
privilegeToken();
setState(2827);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(2826);
showCommandYield();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowPrivilegesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public PrivilegeTokenContext privilegeToken() {
return getRuleContext(PrivilegeTokenContext.class,0);
}
public TerminalNode ALL() { return getToken(Cypher6Parser.ALL, 0); }
public PrivilegeAsCommandContext privilegeAsCommand() {
return getRuleContext(PrivilegeAsCommandContext.class,0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class,0);
}
public ShowPrivilegesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showPrivileges; }
}
public final ShowPrivilegesContext showPrivileges() throws RecognitionException {
ShowPrivilegesContext _localctx = new ShowPrivilegesContext(_ctx, getState());
enterRule(_localctx, 458, RULE_showPrivileges);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2830);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==ALL) {
{
setState(2829);
match(ALL);
}
}
setState(2832);
privilegeToken();
setState(2834);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AS) {
{
setState(2833);
privilegeAsCommand();
}
}
setState(2837);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(2836);
showCommandYield();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowRolePrivilegesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public RoleNamesContext roleNames() {
return getRuleContext(RoleNamesContext.class,0);
}
public PrivilegeTokenContext privilegeToken() {
return getRuleContext(PrivilegeTokenContext.class,0);
}
public TerminalNode ROLE() { return getToken(Cypher6Parser.ROLE, 0); }
public TerminalNode ROLES() { return getToken(Cypher6Parser.ROLES, 0); }
public PrivilegeAsCommandContext privilegeAsCommand() {
return getRuleContext(PrivilegeAsCommandContext.class,0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class,0);
}
public ShowRolePrivilegesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showRolePrivileges; }
}
public final ShowRolePrivilegesContext showRolePrivileges() throws RecognitionException {
ShowRolePrivilegesContext _localctx = new ShowRolePrivilegesContext(_ctx, getState());
enterRule(_localctx, 460, RULE_showRolePrivileges);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2839);
_la = _input.LA(1);
if ( !(_la==ROLE || _la==ROLES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2840);
roleNames();
setState(2841);
privilegeToken();
setState(2843);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AS) {
{
setState(2842);
privilegeAsCommand();
}
}
setState(2846);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(2845);
showCommandYield();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowUserPrivilegesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public PrivilegeTokenContext privilegeToken() {
return getRuleContext(PrivilegeTokenContext.class,0);
}
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public TerminalNode USERS() { return getToken(Cypher6Parser.USERS, 0); }
public UserNamesContext userNames() {
return getRuleContext(UserNamesContext.class,0);
}
public PrivilegeAsCommandContext privilegeAsCommand() {
return getRuleContext(PrivilegeAsCommandContext.class,0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class,0);
}
public ShowUserPrivilegesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showUserPrivileges; }
}
public final ShowUserPrivilegesContext showUserPrivileges() throws RecognitionException {
ShowUserPrivilegesContext _localctx = new ShowUserPrivilegesContext(_ctx, getState());
enterRule(_localctx, 462, RULE_showUserPrivileges);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2848);
_la = _input.LA(1);
if ( !(_la==USER || _la==USERS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2850);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,335,_ctx) ) {
case 1:
{
setState(2849);
userNames();
}
break;
}
setState(2852);
privilegeToken();
setState(2854);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AS) {
{
setState(2853);
privilegeAsCommand();
}
}
setState(2857);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(2856);
showCommandYield();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PrivilegeAsCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode AS() { return getToken(Cypher6Parser.AS, 0); }
public TerminalNode COMMAND() { return getToken(Cypher6Parser.COMMAND, 0); }
public TerminalNode COMMANDS() { return getToken(Cypher6Parser.COMMANDS, 0); }
public TerminalNode REVOKE() { return getToken(Cypher6Parser.REVOKE, 0); }
public PrivilegeAsCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_privilegeAsCommand; }
}
public final PrivilegeAsCommandContext privilegeAsCommand() throws RecognitionException {
PrivilegeAsCommandContext _localctx = new PrivilegeAsCommandContext(_ctx, getState());
enterRule(_localctx, 464, RULE_privilegeAsCommand);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2859);
match(AS);
setState(2861);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==REVOKE) {
{
setState(2860);
match(REVOKE);
}
}
setState(2863);
_la = _input.LA(1);
if ( !(_la==COMMAND || _la==COMMANDS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PrivilegeTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PRIVILEGE() { return getToken(Cypher6Parser.PRIVILEGE, 0); }
public TerminalNode PRIVILEGES() { return getToken(Cypher6Parser.PRIVILEGES, 0); }
public PrivilegeTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_privilegeToken; }
}
public final PrivilegeTokenContext privilegeToken() throws RecognitionException {
PrivilegeTokenContext _localctx = new PrivilegeTokenContext(_ctx, getState());
enterRule(_localctx, 466, RULE_privilegeToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2865);
_la = _input.LA(1);
if ( !(_la==PRIVILEGE || _la==PRIVILEGES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public AllPrivilegeContext allPrivilege() {
return getRuleContext(AllPrivilegeContext.class,0);
}
public CreatePrivilegeContext createPrivilege() {
return getRuleContext(CreatePrivilegeContext.class,0);
}
public DatabasePrivilegeContext databasePrivilege() {
return getRuleContext(DatabasePrivilegeContext.class,0);
}
public DbmsPrivilegeContext dbmsPrivilege() {
return getRuleContext(DbmsPrivilegeContext.class,0);
}
public DropPrivilegeContext dropPrivilege() {
return getRuleContext(DropPrivilegeContext.class,0);
}
public LoadPrivilegeContext loadPrivilege() {
return getRuleContext(LoadPrivilegeContext.class,0);
}
public QualifiedGraphPrivilegesContext qualifiedGraphPrivileges() {
return getRuleContext(QualifiedGraphPrivilegesContext.class,0);
}
public QualifiedGraphPrivilegesWithPropertyContext qualifiedGraphPrivilegesWithProperty() {
return getRuleContext(QualifiedGraphPrivilegesWithPropertyContext.class,0);
}
public RemovePrivilegeContext removePrivilege() {
return getRuleContext(RemovePrivilegeContext.class,0);
}
public SetPrivilegeContext setPrivilege() {
return getRuleContext(SetPrivilegeContext.class,0);
}
public ShowPrivilegeContext showPrivilege() {
return getRuleContext(ShowPrivilegeContext.class,0);
}
public WritePrivilegeContext writePrivilege() {
return getRuleContext(WritePrivilegeContext.class,0);
}
public PrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_privilege; }
}
public final PrivilegeContext privilege() throws RecognitionException {
PrivilegeContext _localctx = new PrivilegeContext(_ctx, getState());
enterRule(_localctx, 468, RULE_privilege);
try {
setState(2879);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALL:
enterOuterAlt(_localctx, 1);
{
setState(2867);
allPrivilege();
}
break;
case CREATE:
enterOuterAlt(_localctx, 2);
{
setState(2868);
createPrivilege();
}
break;
case ACCESS:
case CONSTRAINT:
case CONSTRAINTS:
case INDEX:
case INDEXES:
case NAME:
case START:
case STOP:
case TERMINATE:
case TRANSACTION:
enterOuterAlt(_localctx, 3);
{
setState(2869);
databasePrivilege();
}
break;
case ALIAS:
case ALTER:
case ASSIGN:
case COMPOSITE:
case DATABASE:
case EXECUTE:
case IMPERSONATE:
case PRIVILEGE:
case RENAME:
case ROLE:
case SERVER:
case USER:
enterOuterAlt(_localctx, 4);
{
setState(2870);
dbmsPrivilege();
}
break;
case DROP:
enterOuterAlt(_localctx, 5);
{
setState(2871);
dropPrivilege();
}
break;
case LOAD:
enterOuterAlt(_localctx, 6);
{
setState(2872);
loadPrivilege();
}
break;
case DELETE:
case MERGE:
enterOuterAlt(_localctx, 7);
{
setState(2873);
qualifiedGraphPrivileges();
}
break;
case MATCH:
case READ:
case TRAVERSE:
enterOuterAlt(_localctx, 8);
{
setState(2874);
qualifiedGraphPrivilegesWithProperty();
}
break;
case REMOVE:
enterOuterAlt(_localctx, 9);
{
setState(2875);
removePrivilege();
}
break;
case SET:
enterOuterAlt(_localctx, 10);
{
setState(2876);
setPrivilege();
}
break;
case SHOW:
enterOuterAlt(_localctx, 11);
{
setState(2877);
showPrivilege();
}
break;
case WRITE:
enterOuterAlt(_localctx, 12);
{
setState(2878);
writePrivilege();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AllPrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ALL() { return getToken(Cypher6Parser.ALL, 0); }
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public AllPrivilegeTargetContext allPrivilegeTarget() {
return getRuleContext(AllPrivilegeTargetContext.class,0);
}
public AllPrivilegeTypeContext allPrivilegeType() {
return getRuleContext(AllPrivilegeTypeContext.class,0);
}
public AllPrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_allPrivilege; }
}
public final AllPrivilegeContext allPrivilege() throws RecognitionException {
AllPrivilegeContext _localctx = new AllPrivilegeContext(_ctx, getState());
enterRule(_localctx, 470, RULE_allPrivilege);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2881);
match(ALL);
setState(2883);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 62)) & ~0x3f) == 0 && ((1L << (_la - 62)) & 36028797018963985L) != 0) || _la==PRIVILEGES) {
{
setState(2882);
allPrivilegeType();
}
}
setState(2885);
match(ON);
setState(2886);
allPrivilegeTarget();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AllPrivilegeTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PRIVILEGES() { return getToken(Cypher6Parser.PRIVILEGES, 0); }
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public TerminalNode GRAPH() { return getToken(Cypher6Parser.GRAPH, 0); }
public TerminalNode DBMS() { return getToken(Cypher6Parser.DBMS, 0); }
public AllPrivilegeTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_allPrivilegeType; }
}
public final AllPrivilegeTypeContext allPrivilegeType() throws RecognitionException {
AllPrivilegeTypeContext _localctx = new AllPrivilegeTypeContext(_ctx, getState());
enterRule(_localctx, 472, RULE_allPrivilegeType);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2889);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 62)) & ~0x3f) == 0 && ((1L << (_la - 62)) & 36028797018963985L) != 0)) {
{
setState(2888);
_la = _input.LA(1);
if ( !(((((_la - 62)) & ~0x3f) == 0 && ((1L << (_la - 62)) & 36028797018963985L) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(2891);
match(PRIVILEGES);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AllPrivilegeTargetContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public AllPrivilegeTargetContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_allPrivilegeTarget; }
public AllPrivilegeTargetContext() { }
public void copyFrom(AllPrivilegeTargetContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class DefaultTargetContext extends AllPrivilegeTargetContext {
public TerminalNode DEFAULT() { return getToken(Cypher6Parser.DEFAULT, 0); }
public TerminalNode HOME() { return getToken(Cypher6Parser.HOME, 0); }
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public TerminalNode GRAPH() { return getToken(Cypher6Parser.GRAPH, 0); }
public DefaultTargetContext(AllPrivilegeTargetContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class DatabaseVariableTargetContext extends AllPrivilegeTargetContext {
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public TerminalNode DATABASES() { return getToken(Cypher6Parser.DATABASES, 0); }
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public SymbolicAliasNameListContext symbolicAliasNameList() {
return getRuleContext(SymbolicAliasNameListContext.class,0);
}
public DatabaseVariableTargetContext(AllPrivilegeTargetContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class GraphVariableTargetContext extends AllPrivilegeTargetContext {
public TerminalNode GRAPH() { return getToken(Cypher6Parser.GRAPH, 0); }
public TerminalNode GRAPHS() { return getToken(Cypher6Parser.GRAPHS, 0); }
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public SymbolicAliasNameListContext symbolicAliasNameList() {
return getRuleContext(SymbolicAliasNameListContext.class,0);
}
public GraphVariableTargetContext(AllPrivilegeTargetContext ctx) { copyFrom(ctx); }
}
@SuppressWarnings("CheckReturnValue")
public static class DBMSTargetContext extends AllPrivilegeTargetContext {
public TerminalNode DBMS() { return getToken(Cypher6Parser.DBMS, 0); }
public DBMSTargetContext(AllPrivilegeTargetContext ctx) { copyFrom(ctx); }
}
public final AllPrivilegeTargetContext allPrivilegeTarget() throws RecognitionException {
AllPrivilegeTargetContext _localctx = new AllPrivilegeTargetContext(_ctx, getState());
enterRule(_localctx, 474, RULE_allPrivilegeTarget);
int _la;
try {
setState(2906);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DEFAULT:
case HOME:
_localctx = new DefaultTargetContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(2893);
_la = _input.LA(1);
if ( !(_la==DEFAULT || _la==HOME) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2894);
_la = _input.LA(1);
if ( !(_la==DATABASE || _la==GRAPH) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
case DATABASE:
case DATABASES:
_localctx = new DatabaseVariableTargetContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(2895);
_la = _input.LA(1);
if ( !(_la==DATABASE || _la==DATABASES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2898);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(2896);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
{
setState(2897);
symbolicAliasNameList();
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
case GRAPH:
case GRAPHS:
_localctx = new GraphVariableTargetContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(2900);
_la = _input.LA(1);
if ( !(_la==GRAPH || _la==GRAPHS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2903);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(2901);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
{
setState(2902);
symbolicAliasNameList();
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
case DBMS:
_localctx = new DBMSTargetContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(2905);
match(DBMS);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreatePrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CREATE() { return getToken(Cypher6Parser.CREATE, 0); }
public CreatePrivilegeForDatabaseContext createPrivilegeForDatabase() {
return getRuleContext(CreatePrivilegeForDatabaseContext.class,0);
}
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public DatabaseScopeContext databaseScope() {
return getRuleContext(DatabaseScopeContext.class,0);
}
public ActionForDBMSContext actionForDBMS() {
return getRuleContext(ActionForDBMSContext.class,0);
}
public TerminalNode DBMS() { return getToken(Cypher6Parser.DBMS, 0); }
public GraphScopeContext graphScope() {
return getRuleContext(GraphScopeContext.class,0);
}
public GraphQualifierContext graphQualifier() {
return getRuleContext(GraphQualifierContext.class,0);
}
public CreatePrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createPrivilege; }
}
public final CreatePrivilegeContext createPrivilege() throws RecognitionException {
CreatePrivilegeContext _localctx = new CreatePrivilegeContext(_ctx, getState());
enterRule(_localctx, 476, RULE_createPrivilege);
try {
enterOuterAlt(_localctx, 1);
{
setState(2908);
match(CREATE);
setState(2921);
_errHandler.sync(this);
switch (_input.LA(1)) {
case CONSTRAINT:
case CONSTRAINTS:
case INDEX:
case INDEXES:
case NEW:
{
setState(2909);
createPrivilegeForDatabase();
setState(2910);
match(ON);
setState(2911);
databaseScope();
}
break;
case ALIAS:
case COMPOSITE:
case DATABASE:
case ROLE:
case USER:
{
setState(2913);
actionForDBMS();
setState(2914);
match(ON);
setState(2915);
match(DBMS);
}
break;
case ON:
{
setState(2917);
match(ON);
setState(2918);
graphScope();
setState(2919);
graphQualifier();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreatePrivilegeForDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public IndexTokenContext indexToken() {
return getRuleContext(IndexTokenContext.class,0);
}
public ConstraintTokenContext constraintToken() {
return getRuleContext(ConstraintTokenContext.class,0);
}
public CreateNodePrivilegeTokenContext createNodePrivilegeToken() {
return getRuleContext(CreateNodePrivilegeTokenContext.class,0);
}
public CreateRelPrivilegeTokenContext createRelPrivilegeToken() {
return getRuleContext(CreateRelPrivilegeTokenContext.class,0);
}
public CreatePropertyPrivilegeTokenContext createPropertyPrivilegeToken() {
return getRuleContext(CreatePropertyPrivilegeTokenContext.class,0);
}
public CreatePrivilegeForDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createPrivilegeForDatabase; }
}
public final CreatePrivilegeForDatabaseContext createPrivilegeForDatabase() throws RecognitionException {
CreatePrivilegeForDatabaseContext _localctx = new CreatePrivilegeForDatabaseContext(_ctx, getState());
enterRule(_localctx, 478, RULE_createPrivilegeForDatabase);
try {
setState(2928);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,346,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(2923);
indexToken();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(2924);
constraintToken();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(2925);
createNodePrivilegeToken();
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(2926);
createRelPrivilegeToken();
}
break;
case 5:
enterOuterAlt(_localctx, 5);
{
setState(2927);
createPropertyPrivilegeToken();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateNodePrivilegeTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode NEW() { return getToken(Cypher6Parser.NEW, 0); }
public TerminalNode LABEL() { return getToken(Cypher6Parser.LABEL, 0); }
public TerminalNode LABELS() { return getToken(Cypher6Parser.LABELS, 0); }
public TerminalNode NODE() { return getToken(Cypher6Parser.NODE, 0); }
public CreateNodePrivilegeTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createNodePrivilegeToken; }
}
public final CreateNodePrivilegeTokenContext createNodePrivilegeToken() throws RecognitionException {
CreateNodePrivilegeTokenContext _localctx = new CreateNodePrivilegeTokenContext(_ctx, getState());
enterRule(_localctx, 480, RULE_createNodePrivilegeToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2930);
match(NEW);
setState(2932);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==NODE) {
{
setState(2931);
match(NODE);
}
}
setState(2934);
_la = _input.LA(1);
if ( !(_la==LABEL || _la==LABELS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateRelPrivilegeTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode NEW() { return getToken(Cypher6Parser.NEW, 0); }
public TerminalNode TYPE() { return getToken(Cypher6Parser.TYPE, 0); }
public TerminalNode TYPES() { return getToken(Cypher6Parser.TYPES, 0); }
public TerminalNode RELATIONSHIP() { return getToken(Cypher6Parser.RELATIONSHIP, 0); }
public CreateRelPrivilegeTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createRelPrivilegeToken; }
}
public final CreateRelPrivilegeTokenContext createRelPrivilegeToken() throws RecognitionException {
CreateRelPrivilegeTokenContext _localctx = new CreateRelPrivilegeTokenContext(_ctx, getState());
enterRule(_localctx, 482, RULE_createRelPrivilegeToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2936);
match(NEW);
setState(2938);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==RELATIONSHIP) {
{
setState(2937);
match(RELATIONSHIP);
}
}
setState(2940);
_la = _input.LA(1);
if ( !(_la==TYPE || _la==TYPES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreatePropertyPrivilegeTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode NEW() { return getToken(Cypher6Parser.NEW, 0); }
public TerminalNode NAME() { return getToken(Cypher6Parser.NAME, 0); }
public TerminalNode NAMES() { return getToken(Cypher6Parser.NAMES, 0); }
public TerminalNode PROPERTY() { return getToken(Cypher6Parser.PROPERTY, 0); }
public CreatePropertyPrivilegeTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createPropertyPrivilegeToken; }
}
public final CreatePropertyPrivilegeTokenContext createPropertyPrivilegeToken() throws RecognitionException {
CreatePropertyPrivilegeTokenContext _localctx = new CreatePropertyPrivilegeTokenContext(_ctx, getState());
enterRule(_localctx, 484, RULE_createPropertyPrivilegeToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2942);
match(NEW);
setState(2944);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==PROPERTY) {
{
setState(2943);
match(PROPERTY);
}
}
setState(2946);
_la = _input.LA(1);
if ( !(_la==NAME || _la==NAMES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ActionForDBMSContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ALIAS() { return getToken(Cypher6Parser.ALIAS, 0); }
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public TerminalNode COMPOSITE() { return getToken(Cypher6Parser.COMPOSITE, 0); }
public TerminalNode ROLE() { return getToken(Cypher6Parser.ROLE, 0); }
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public ActionForDBMSContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_actionForDBMS; }
}
public final ActionForDBMSContext actionForDBMS() throws RecognitionException {
ActionForDBMSContext _localctx = new ActionForDBMSContext(_ctx, getState());
enterRule(_localctx, 486, RULE_actionForDBMS);
int _la;
try {
setState(2955);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALIAS:
enterOuterAlt(_localctx, 1);
{
setState(2948);
match(ALIAS);
}
break;
case COMPOSITE:
case DATABASE:
enterOuterAlt(_localctx, 2);
{
setState(2950);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMPOSITE) {
{
setState(2949);
match(COMPOSITE);
}
}
setState(2952);
match(DATABASE);
}
break;
case ROLE:
enterOuterAlt(_localctx, 3);
{
setState(2953);
match(ROLE);
}
break;
case USER:
enterOuterAlt(_localctx, 4);
{
setState(2954);
match(USER);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropPrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DROP() { return getToken(Cypher6Parser.DROP, 0); }
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public DatabaseScopeContext databaseScope() {
return getRuleContext(DatabaseScopeContext.class,0);
}
public ActionForDBMSContext actionForDBMS() {
return getRuleContext(ActionForDBMSContext.class,0);
}
public TerminalNode DBMS() { return getToken(Cypher6Parser.DBMS, 0); }
public IndexTokenContext indexToken() {
return getRuleContext(IndexTokenContext.class,0);
}
public ConstraintTokenContext constraintToken() {
return getRuleContext(ConstraintTokenContext.class,0);
}
public DropPrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dropPrivilege; }
}
public final DropPrivilegeContext dropPrivilege() throws RecognitionException {
DropPrivilegeContext _localctx = new DropPrivilegeContext(_ctx, getState());
enterRule(_localctx, 488, RULE_dropPrivilege);
try {
enterOuterAlt(_localctx, 1);
{
setState(2957);
match(DROP);
setState(2969);
_errHandler.sync(this);
switch (_input.LA(1)) {
case CONSTRAINT:
case CONSTRAINTS:
case INDEX:
case INDEXES:
{
setState(2960);
_errHandler.sync(this);
switch (_input.LA(1)) {
case INDEX:
case INDEXES:
{
setState(2958);
indexToken();
}
break;
case CONSTRAINT:
case CONSTRAINTS:
{
setState(2959);
constraintToken();
}
break;
default:
throw new NoViableAltException(this);
}
setState(2962);
match(ON);
setState(2963);
databaseScope();
}
break;
case ALIAS:
case COMPOSITE:
case DATABASE:
case ROLE:
case USER:
{
setState(2965);
actionForDBMS();
setState(2966);
match(ON);
setState(2967);
match(DBMS);
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LoadPrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LOAD() { return getToken(Cypher6Parser.LOAD, 0); }
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public StringOrParameterContext stringOrParameter() {
return getRuleContext(StringOrParameterContext.class,0);
}
public TerminalNode ALL() { return getToken(Cypher6Parser.ALL, 0); }
public TerminalNode DATA() { return getToken(Cypher6Parser.DATA, 0); }
public TerminalNode URL() { return getToken(Cypher6Parser.URL, 0); }
public TerminalNode CIDR() { return getToken(Cypher6Parser.CIDR, 0); }
public LoadPrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_loadPrivilege; }
}
public final LoadPrivilegeContext loadPrivilege() throws RecognitionException {
LoadPrivilegeContext _localctx = new LoadPrivilegeContext(_ctx, getState());
enterRule(_localctx, 490, RULE_loadPrivilege);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2971);
match(LOAD);
setState(2972);
match(ON);
setState(2977);
_errHandler.sync(this);
switch (_input.LA(1)) {
case CIDR:
case URL:
{
setState(2973);
_la = _input.LA(1);
if ( !(_la==CIDR || _la==URL) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2974);
stringOrParameter();
}
break;
case ALL:
{
setState(2975);
match(ALL);
setState(2976);
match(DATA);
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowPrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SHOW() { return getToken(Cypher6Parser.SHOW, 0); }
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public DatabaseScopeContext databaseScope() {
return getRuleContext(DatabaseScopeContext.class,0);
}
public TerminalNode DBMS() { return getToken(Cypher6Parser.DBMS, 0); }
public IndexTokenContext indexToken() {
return getRuleContext(IndexTokenContext.class,0);
}
public ConstraintTokenContext constraintToken() {
return getRuleContext(ConstraintTokenContext.class,0);
}
public TransactionTokenContext transactionToken() {
return getRuleContext(TransactionTokenContext.class,0);
}
public TerminalNode ALIAS() { return getToken(Cypher6Parser.ALIAS, 0); }
public TerminalNode PRIVILEGE() { return getToken(Cypher6Parser.PRIVILEGE, 0); }
public TerminalNode ROLE() { return getToken(Cypher6Parser.ROLE, 0); }
public TerminalNode SERVER() { return getToken(Cypher6Parser.SERVER, 0); }
public TerminalNode SERVERS() { return getToken(Cypher6Parser.SERVERS, 0); }
public SettingTokenContext settingToken() {
return getRuleContext(SettingTokenContext.class,0);
}
public SettingQualifierContext settingQualifier() {
return getRuleContext(SettingQualifierContext.class,0);
}
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public UserQualifierContext userQualifier() {
return getRuleContext(UserQualifierContext.class,0);
}
public ShowPrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showPrivilege; }
}
public final ShowPrivilegeContext showPrivilege() throws RecognitionException {
ShowPrivilegeContext _localctx = new ShowPrivilegeContext(_ctx, getState());
enterRule(_localctx, 492, RULE_showPrivilege);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2979);
match(SHOW);
setState(3004);
_errHandler.sync(this);
switch (_input.LA(1)) {
case CONSTRAINT:
case CONSTRAINTS:
case INDEX:
case INDEXES:
case TRANSACTION:
case TRANSACTIONS:
{
setState(2986);
_errHandler.sync(this);
switch (_input.LA(1)) {
case INDEX:
case INDEXES:
{
setState(2980);
indexToken();
}
break;
case CONSTRAINT:
case CONSTRAINTS:
{
setState(2981);
constraintToken();
}
break;
case TRANSACTION:
case TRANSACTIONS:
{
setState(2982);
transactionToken();
setState(2984);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LPAREN) {
{
setState(2983);
userQualifier();
}
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(2988);
match(ON);
setState(2989);
databaseScope();
}
break;
case ALIAS:
case PRIVILEGE:
case ROLE:
case SERVER:
case SERVERS:
case SETTING:
case SETTINGS:
case USER:
{
setState(3000);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALIAS:
{
setState(2991);
match(ALIAS);
}
break;
case PRIVILEGE:
{
setState(2992);
match(PRIVILEGE);
}
break;
case ROLE:
{
setState(2993);
match(ROLE);
}
break;
case SERVER:
{
setState(2994);
match(SERVER);
}
break;
case SERVERS:
{
setState(2995);
match(SERVERS);
}
break;
case SETTING:
case SETTINGS:
{
setState(2996);
settingToken();
setState(2997);
settingQualifier();
}
break;
case USER:
{
setState(2999);
match(USER);
}
break;
default:
throw new NoViableAltException(this);
}
setState(3002);
match(ON);
setState(3003);
match(DBMS);
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SetPrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SET() { return getToken(Cypher6Parser.SET, 0); }
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public TerminalNode DBMS() { return getToken(Cypher6Parser.DBMS, 0); }
public TerminalNode LABEL() { return getToken(Cypher6Parser.LABEL, 0); }
public LabelsResourceContext labelsResource() {
return getRuleContext(LabelsResourceContext.class,0);
}
public GraphScopeContext graphScope() {
return getRuleContext(GraphScopeContext.class,0);
}
public TerminalNode PROPERTY() { return getToken(Cypher6Parser.PROPERTY, 0); }
public PropertiesResourceContext propertiesResource() {
return getRuleContext(PropertiesResourceContext.class,0);
}
public GraphQualifierContext graphQualifier() {
return getRuleContext(GraphQualifierContext.class,0);
}
public TerminalNode AUTH() { return getToken(Cypher6Parser.AUTH, 0); }
public PasswordTokenContext passwordToken() {
return getRuleContext(PasswordTokenContext.class,0);
}
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public TerminalNode ACCESS() { return getToken(Cypher6Parser.ACCESS, 0); }
public TerminalNode STATUS() { return getToken(Cypher6Parser.STATUS, 0); }
public TerminalNode HOME() { return getToken(Cypher6Parser.HOME, 0); }
public SetPrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_setPrivilege; }
}
public final SetPrivilegeContext setPrivilege() throws RecognitionException {
SetPrivilegeContext _localctx = new SetPrivilegeContext(_ctx, getState());
enterRule(_localctx, 494, RULE_setPrivilege);
try {
enterOuterAlt(_localctx, 1);
{
setState(3006);
match(SET);
setState(3034);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DATABASE:
case PASSWORD:
case PASSWORDS:
case USER:
{
setState(3016);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PASSWORD:
case PASSWORDS:
{
setState(3007);
passwordToken();
}
break;
case USER:
{
setState(3008);
match(USER);
setState(3012);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STATUS:
{
setState(3009);
match(STATUS);
}
break;
case HOME:
{
setState(3010);
match(HOME);
setState(3011);
match(DATABASE);
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
case DATABASE:
{
setState(3014);
match(DATABASE);
setState(3015);
match(ACCESS);
}
break;
default:
throw new NoViableAltException(this);
}
setState(3018);
match(ON);
setState(3019);
match(DBMS);
}
break;
case LABEL:
{
setState(3020);
match(LABEL);
setState(3021);
labelsResource();
setState(3022);
match(ON);
setState(3023);
graphScope();
}
break;
case PROPERTY:
{
setState(3025);
match(PROPERTY);
setState(3026);
propertiesResource();
setState(3027);
match(ON);
setState(3028);
graphScope();
setState(3029);
graphQualifier();
}
break;
case AUTH:
{
setState(3031);
match(AUTH);
setState(3032);
match(ON);
setState(3033);
match(DBMS);
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PasswordTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PASSWORD() { return getToken(Cypher6Parser.PASSWORD, 0); }
public TerminalNode PASSWORDS() { return getToken(Cypher6Parser.PASSWORDS, 0); }
public PasswordTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_passwordToken; }
}
public final PasswordTokenContext passwordToken() throws RecognitionException {
PasswordTokenContext _localctx = new PasswordTokenContext(_ctx, getState());
enterRule(_localctx, 496, RULE_passwordToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3036);
_la = _input.LA(1);
if ( !(_la==PASSWORD || _la==PASSWORDS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RemovePrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode REMOVE() { return getToken(Cypher6Parser.REMOVE, 0); }
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public TerminalNode DBMS() { return getToken(Cypher6Parser.DBMS, 0); }
public TerminalNode LABEL() { return getToken(Cypher6Parser.LABEL, 0); }
public LabelsResourceContext labelsResource() {
return getRuleContext(LabelsResourceContext.class,0);
}
public GraphScopeContext graphScope() {
return getRuleContext(GraphScopeContext.class,0);
}
public TerminalNode PRIVILEGE() { return getToken(Cypher6Parser.PRIVILEGE, 0); }
public TerminalNode ROLE() { return getToken(Cypher6Parser.ROLE, 0); }
public RemovePrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_removePrivilege; }
}
public final RemovePrivilegeContext removePrivilege() throws RecognitionException {
RemovePrivilegeContext _localctx = new RemovePrivilegeContext(_ctx, getState());
enterRule(_localctx, 498, RULE_removePrivilege);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3038);
match(REMOVE);
setState(3047);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PRIVILEGE:
case ROLE:
{
setState(3039);
_la = _input.LA(1);
if ( !(_la==PRIVILEGE || _la==ROLE) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3040);
match(ON);
setState(3041);
match(DBMS);
}
break;
case LABEL:
{
setState(3042);
match(LABEL);
setState(3043);
labelsResource();
setState(3044);
match(ON);
setState(3045);
graphScope();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class WritePrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode WRITE() { return getToken(Cypher6Parser.WRITE, 0); }
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public GraphScopeContext graphScope() {
return getRuleContext(GraphScopeContext.class,0);
}
public WritePrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_writePrivilege; }
}
public final WritePrivilegeContext writePrivilege() throws RecognitionException {
WritePrivilegeContext _localctx = new WritePrivilegeContext(_ctx, getState());
enterRule(_localctx, 500, RULE_writePrivilege);
try {
enterOuterAlt(_localctx, 1);
{
setState(3049);
match(WRITE);
setState(3050);
match(ON);
setState(3051);
graphScope();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DatabasePrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public DatabaseScopeContext databaseScope() {
return getRuleContext(DatabaseScopeContext.class,0);
}
public TerminalNode ACCESS() { return getToken(Cypher6Parser.ACCESS, 0); }
public TerminalNode START() { return getToken(Cypher6Parser.START, 0); }
public TerminalNode STOP() { return getToken(Cypher6Parser.STOP, 0); }
public IndexTokenContext indexToken() {
return getRuleContext(IndexTokenContext.class,0);
}
public ConstraintTokenContext constraintToken() {
return getRuleContext(ConstraintTokenContext.class,0);
}
public TerminalNode NAME() { return getToken(Cypher6Parser.NAME, 0); }
public TerminalNode TRANSACTION() { return getToken(Cypher6Parser.TRANSACTION, 0); }
public TerminalNode TERMINATE() { return getToken(Cypher6Parser.TERMINATE, 0); }
public TransactionTokenContext transactionToken() {
return getRuleContext(TransactionTokenContext.class,0);
}
public TerminalNode MANAGEMENT() { return getToken(Cypher6Parser.MANAGEMENT, 0); }
public UserQualifierContext userQualifier() {
return getRuleContext(UserQualifierContext.class,0);
}
public DatabasePrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_databasePrivilege; }
}
public final DatabasePrivilegeContext databasePrivilege() throws RecognitionException {
DatabasePrivilegeContext _localctx = new DatabasePrivilegeContext(_ctx, getState());
enterRule(_localctx, 502, RULE_databasePrivilege);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3075);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ACCESS:
{
setState(3053);
match(ACCESS);
}
break;
case START:
{
setState(3054);
match(START);
}
break;
case STOP:
{
setState(3055);
match(STOP);
}
break;
case CONSTRAINT:
case CONSTRAINTS:
case INDEX:
case INDEXES:
case NAME:
{
setState(3059);
_errHandler.sync(this);
switch (_input.LA(1)) {
case INDEX:
case INDEXES:
{
setState(3056);
indexToken();
}
break;
case CONSTRAINT:
case CONSTRAINTS:
{
setState(3057);
constraintToken();
}
break;
case NAME:
{
setState(3058);
match(NAME);
}
break;
default:
throw new NoViableAltException(this);
}
setState(3062);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==MANAGEMENT) {
{
setState(3061);
match(MANAGEMENT);
}
}
}
break;
case TERMINATE:
case TRANSACTION:
{
setState(3070);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TRANSACTION:
{
setState(3064);
match(TRANSACTION);
setState(3066);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==MANAGEMENT) {
{
setState(3065);
match(MANAGEMENT);
}
}
}
break;
case TERMINATE:
{
setState(3068);
match(TERMINATE);
setState(3069);
transactionToken();
}
break;
default:
throw new NoViableAltException(this);
}
setState(3073);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LPAREN) {
{
setState(3072);
userQualifier();
}
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(3077);
match(ON);
setState(3078);
databaseScope();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DbmsPrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public TerminalNode DBMS() { return getToken(Cypher6Parser.DBMS, 0); }
public TerminalNode ALTER() { return getToken(Cypher6Parser.ALTER, 0); }
public TerminalNode ASSIGN() { return getToken(Cypher6Parser.ASSIGN, 0); }
public TerminalNode MANAGEMENT() { return getToken(Cypher6Parser.MANAGEMENT, 0); }
public DbmsPrivilegeExecuteContext dbmsPrivilegeExecute() {
return getRuleContext(DbmsPrivilegeExecuteContext.class,0);
}
public TerminalNode RENAME() { return getToken(Cypher6Parser.RENAME, 0); }
public TerminalNode IMPERSONATE() { return getToken(Cypher6Parser.IMPERSONATE, 0); }
public TerminalNode ALIAS() { return getToken(Cypher6Parser.ALIAS, 0); }
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public TerminalNode PRIVILEGE() { return getToken(Cypher6Parser.PRIVILEGE, 0); }
public TerminalNode ROLE() { return getToken(Cypher6Parser.ROLE, 0); }
public TerminalNode SERVER() { return getToken(Cypher6Parser.SERVER, 0); }
public UserQualifierContext userQualifier() {
return getRuleContext(UserQualifierContext.class,0);
}
public TerminalNode COMPOSITE() { return getToken(Cypher6Parser.COMPOSITE, 0); }
public DbmsPrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dbmsPrivilege; }
}
public final DbmsPrivilegeContext dbmsPrivilege() throws RecognitionException {
DbmsPrivilegeContext _localctx = new DbmsPrivilegeContext(_ctx, getState());
enterRule(_localctx, 504, RULE_dbmsPrivilege);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3103);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALTER:
{
setState(3080);
match(ALTER);
setState(3081);
_la = _input.LA(1);
if ( !(_la==ALIAS || _la==DATABASE || _la==USER) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
case ASSIGN:
{
setState(3082);
match(ASSIGN);
setState(3083);
_la = _input.LA(1);
if ( !(_la==PRIVILEGE || _la==ROLE) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
case ALIAS:
case COMPOSITE:
case DATABASE:
case PRIVILEGE:
case ROLE:
case SERVER:
case USER:
{
setState(3093);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALIAS:
{
setState(3084);
match(ALIAS);
}
break;
case COMPOSITE:
case DATABASE:
{
setState(3086);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMPOSITE) {
{
setState(3085);
match(COMPOSITE);
}
}
setState(3088);
match(DATABASE);
}
break;
case PRIVILEGE:
{
setState(3089);
match(PRIVILEGE);
}
break;
case ROLE:
{
setState(3090);
match(ROLE);
}
break;
case SERVER:
{
setState(3091);
match(SERVER);
}
break;
case USER:
{
setState(3092);
match(USER);
}
break;
default:
throw new NoViableAltException(this);
}
setState(3095);
match(MANAGEMENT);
}
break;
case EXECUTE:
{
setState(3096);
dbmsPrivilegeExecute();
}
break;
case RENAME:
{
setState(3097);
match(RENAME);
setState(3098);
_la = _input.LA(1);
if ( !(_la==ROLE || _la==USER) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
case IMPERSONATE:
{
setState(3099);
match(IMPERSONATE);
setState(3101);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LPAREN) {
{
setState(3100);
userQualifier();
}
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(3105);
match(ON);
setState(3106);
match(DBMS);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DbmsPrivilegeExecuteContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode EXECUTE() { return getToken(Cypher6Parser.EXECUTE, 0); }
public AdminTokenContext adminToken() {
return getRuleContext(AdminTokenContext.class,0);
}
public TerminalNode PROCEDURES() { return getToken(Cypher6Parser.PROCEDURES, 0); }
public ProcedureTokenContext procedureToken() {
return getRuleContext(ProcedureTokenContext.class,0);
}
public ExecuteProcedureQualifierContext executeProcedureQualifier() {
return getRuleContext(ExecuteProcedureQualifierContext.class,0);
}
public FunctionTokenContext functionToken() {
return getRuleContext(FunctionTokenContext.class,0);
}
public ExecuteFunctionQualifierContext executeFunctionQualifier() {
return getRuleContext(ExecuteFunctionQualifierContext.class,0);
}
public TerminalNode BOOSTED() { return getToken(Cypher6Parser.BOOSTED, 0); }
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public TerminalNode DEFINED() { return getToken(Cypher6Parser.DEFINED, 0); }
public DbmsPrivilegeExecuteContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dbmsPrivilegeExecute; }
}
public final DbmsPrivilegeExecuteContext dbmsPrivilegeExecute() throws RecognitionException {
DbmsPrivilegeExecuteContext _localctx = new DbmsPrivilegeExecuteContext(_ctx, getState());
enterRule(_localctx, 506, RULE_dbmsPrivilegeExecute);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3108);
match(EXECUTE);
setState(3129);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ADMIN:
case ADMINISTRATOR:
{
setState(3109);
adminToken();
setState(3110);
match(PROCEDURES);
}
break;
case BOOSTED:
case FUNCTION:
case FUNCTIONS:
case PROCEDURE:
case PROCEDURES:
case USER:
{
setState(3113);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==BOOSTED) {
{
setState(3112);
match(BOOSTED);
}
}
setState(3127);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PROCEDURE:
case PROCEDURES:
{
setState(3115);
procedureToken();
setState(3116);
executeProcedureQualifier();
}
break;
case FUNCTION:
case FUNCTIONS:
case USER:
{
setState(3122);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==USER) {
{
setState(3118);
match(USER);
setState(3120);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DEFINED) {
{
setState(3119);
match(DEFINED);
}
}
}
}
setState(3124);
functionToken();
setState(3125);
executeFunctionQualifier();
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AdminTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ADMIN() { return getToken(Cypher6Parser.ADMIN, 0); }
public TerminalNode ADMINISTRATOR() { return getToken(Cypher6Parser.ADMINISTRATOR, 0); }
public AdminTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_adminToken; }
}
public final AdminTokenContext adminToken() throws RecognitionException {
AdminTokenContext _localctx = new AdminTokenContext(_ctx, getState());
enterRule(_localctx, 508, RULE_adminToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3131);
_la = _input.LA(1);
if ( !(_la==ADMIN || _la==ADMINISTRATOR) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ProcedureTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PROCEDURE() { return getToken(Cypher6Parser.PROCEDURE, 0); }
public TerminalNode PROCEDURES() { return getToken(Cypher6Parser.PROCEDURES, 0); }
public ProcedureTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_procedureToken; }
}
public final ProcedureTokenContext procedureToken() throws RecognitionException {
ProcedureTokenContext _localctx = new ProcedureTokenContext(_ctx, getState());
enterRule(_localctx, 510, RULE_procedureToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3133);
_la = _input.LA(1);
if ( !(_la==PROCEDURE || _la==PROCEDURES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class IndexTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode INDEX() { return getToken(Cypher6Parser.INDEX, 0); }
public TerminalNode INDEXES() { return getToken(Cypher6Parser.INDEXES, 0); }
public IndexTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_indexToken; }
}
public final IndexTokenContext indexToken() throws RecognitionException {
IndexTokenContext _localctx = new IndexTokenContext(_ctx, getState());
enterRule(_localctx, 512, RULE_indexToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3135);
_la = _input.LA(1);
if ( !(_la==INDEX || _la==INDEXES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CONSTRAINT() { return getToken(Cypher6Parser.CONSTRAINT, 0); }
public TerminalNode CONSTRAINTS() { return getToken(Cypher6Parser.CONSTRAINTS, 0); }
public ConstraintTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_constraintToken; }
}
public final ConstraintTokenContext constraintToken() throws RecognitionException {
ConstraintTokenContext _localctx = new ConstraintTokenContext(_ctx, getState());
enterRule(_localctx, 514, RULE_constraintToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3137);
_la = _input.LA(1);
if ( !(_la==CONSTRAINT || _la==CONSTRAINTS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TransactionTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode TRANSACTION() { return getToken(Cypher6Parser.TRANSACTION, 0); }
public TerminalNode TRANSACTIONS() { return getToken(Cypher6Parser.TRANSACTIONS, 0); }
public TransactionTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_transactionToken; }
}
public final TransactionTokenContext transactionToken() throws RecognitionException {
TransactionTokenContext _localctx = new TransactionTokenContext(_ctx, getState());
enterRule(_localctx, 516, RULE_transactionToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3139);
_la = _input.LA(1);
if ( !(_la==TRANSACTION || _la==TRANSACTIONS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UserQualifierContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public UserNamesContext userNames() {
return getRuleContext(UserNamesContext.class,0);
}
public UserQualifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_userQualifier; }
}
public final UserQualifierContext userQualifier() throws RecognitionException {
UserQualifierContext _localctx = new UserQualifierContext(_ctx, getState());
enterRule(_localctx, 518, RULE_userQualifier);
try {
enterOuterAlt(_localctx, 1);
{
setState(3141);
match(LPAREN);
setState(3144);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(3142);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
{
setState(3143);
userNames();
}
break;
default:
throw new NoViableAltException(this);
}
setState(3146);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExecuteFunctionQualifierContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public GlobsContext globs() {
return getRuleContext(GlobsContext.class,0);
}
public ExecuteFunctionQualifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_executeFunctionQualifier; }
}
public final ExecuteFunctionQualifierContext executeFunctionQualifier() throws RecognitionException {
ExecuteFunctionQualifierContext _localctx = new ExecuteFunctionQualifierContext(_ctx, getState());
enterRule(_localctx, 520, RULE_executeFunctionQualifier);
try {
enterOuterAlt(_localctx, 1);
{
setState(3148);
globs();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExecuteProcedureQualifierContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public GlobsContext globs() {
return getRuleContext(GlobsContext.class,0);
}
public ExecuteProcedureQualifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_executeProcedureQualifier; }
}
public final ExecuteProcedureQualifierContext executeProcedureQualifier() throws RecognitionException {
ExecuteProcedureQualifierContext _localctx = new ExecuteProcedureQualifierContext(_ctx, getState());
enterRule(_localctx, 522, RULE_executeProcedureQualifier);
try {
enterOuterAlt(_localctx, 1);
{
setState(3150);
globs();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SettingQualifierContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public GlobsContext globs() {
return getRuleContext(GlobsContext.class,0);
}
public SettingQualifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_settingQualifier; }
}
public final SettingQualifierContext settingQualifier() throws RecognitionException {
SettingQualifierContext _localctx = new SettingQualifierContext(_ctx, getState());
enterRule(_localctx, 524, RULE_settingQualifier);
try {
enterOuterAlt(_localctx, 1);
{
setState(3152);
globs();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GlobsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List glob() {
return getRuleContexts(GlobContext.class);
}
public GlobContext glob(int i) {
return getRuleContext(GlobContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public GlobsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_globs; }
}
public final GlobsContext globs() throws RecognitionException {
GlobsContext _localctx = new GlobsContext(_ctx, getState());
enterRule(_localctx, 526, RULE_globs);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3154);
glob();
setState(3159);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(3155);
match(COMMA);
setState(3156);
glob();
}
}
setState(3161);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GlobContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public EscapedSymbolicNameStringContext escapedSymbolicNameString() {
return getRuleContext(EscapedSymbolicNameStringContext.class,0);
}
public GlobRecursiveContext globRecursive() {
return getRuleContext(GlobRecursiveContext.class,0);
}
public GlobContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_glob; }
}
public final GlobContext glob() throws RecognitionException {
GlobContext _localctx = new GlobContext(_ctx, getState());
enterRule(_localctx, 528, RULE_glob);
try {
setState(3167);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
enterOuterAlt(_localctx, 1);
{
setState(3162);
escapedSymbolicNameString();
setState(3164);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,380,_ctx) ) {
case 1:
{
setState(3163);
globRecursive();
}
break;
}
}
break;
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DOT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case QUESTION:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMES:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 2);
{
setState(3166);
globRecursive();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GlobRecursiveContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public GlobPartContext globPart() {
return getRuleContext(GlobPartContext.class,0);
}
public GlobRecursiveContext globRecursive() {
return getRuleContext(GlobRecursiveContext.class,0);
}
public GlobRecursiveContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_globRecursive; }
}
public final GlobRecursiveContext globRecursive() throws RecognitionException {
GlobRecursiveContext _localctx = new GlobRecursiveContext(_ctx, getState());
enterRule(_localctx, 530, RULE_globRecursive);
try {
enterOuterAlt(_localctx, 1);
{
setState(3169);
globPart();
setState(3171);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,382,_ctx) ) {
case 1:
{
setState(3170);
globRecursive();
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GlobPartContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DOT() { return getToken(Cypher6Parser.DOT, 0); }
public EscapedSymbolicNameStringContext escapedSymbolicNameString() {
return getRuleContext(EscapedSymbolicNameStringContext.class,0);
}
public TerminalNode QUESTION() { return getToken(Cypher6Parser.QUESTION, 0); }
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public UnescapedSymbolicNameStringContext unescapedSymbolicNameString() {
return getRuleContext(UnescapedSymbolicNameStringContext.class,0);
}
public GlobPartContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_globPart; }
}
public final GlobPartContext globPart() throws RecognitionException {
GlobPartContext _localctx = new GlobPartContext(_ctx, getState());
enterRule(_localctx, 532, RULE_globPart);
int _la;
try {
setState(3180);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DOT:
enterOuterAlt(_localctx, 1);
{
setState(3173);
match(DOT);
setState(3175);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==ESCAPED_SYMBOLIC_NAME) {
{
setState(3174);
escapedSymbolicNameString();
}
}
}
break;
case QUESTION:
enterOuterAlt(_localctx, 2);
{
setState(3177);
match(QUESTION);
}
break;
case TIMES:
enterOuterAlt(_localctx, 3);
{
setState(3178);
match(TIMES);
}
break;
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 4);
{
setState(3179);
unescapedSymbolicNameString();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class QualifiedGraphPrivilegesWithPropertyContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public GraphScopeContext graphScope() {
return getRuleContext(GraphScopeContext.class,0);
}
public GraphQualifierContext graphQualifier() {
return getRuleContext(GraphQualifierContext.class,0);
}
public TerminalNode TRAVERSE() { return getToken(Cypher6Parser.TRAVERSE, 0); }
public PropertiesResourceContext propertiesResource() {
return getRuleContext(PropertiesResourceContext.class,0);
}
public TerminalNode READ() { return getToken(Cypher6Parser.READ, 0); }
public TerminalNode MATCH() { return getToken(Cypher6Parser.MATCH, 0); }
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public QualifiedGraphPrivilegesWithPropertyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_qualifiedGraphPrivilegesWithProperty; }
}
public final QualifiedGraphPrivilegesWithPropertyContext qualifiedGraphPrivilegesWithProperty() throws RecognitionException {
QualifiedGraphPrivilegesWithPropertyContext _localctx = new QualifiedGraphPrivilegesWithPropertyContext(_ctx, getState());
enterRule(_localctx, 534, RULE_qualifiedGraphPrivilegesWithProperty);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3185);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TRAVERSE:
{
setState(3182);
match(TRAVERSE);
}
break;
case MATCH:
case READ:
{
setState(3183);
_la = _input.LA(1);
if ( !(_la==MATCH || _la==READ) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3184);
propertiesResource();
}
break;
default:
throw new NoViableAltException(this);
}
setState(3187);
match(ON);
setState(3188);
graphScope();
setState(3189);
graphQualifier();
setState(3193);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==LPAREN) {
{
setState(3190);
match(LPAREN);
setState(3191);
match(TIMES);
setState(3192);
match(RPAREN);
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class QualifiedGraphPrivilegesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public GraphScopeContext graphScope() {
return getRuleContext(GraphScopeContext.class,0);
}
public GraphQualifierContext graphQualifier() {
return getRuleContext(GraphQualifierContext.class,0);
}
public TerminalNode DELETE() { return getToken(Cypher6Parser.DELETE, 0); }
public TerminalNode MERGE() { return getToken(Cypher6Parser.MERGE, 0); }
public PropertiesResourceContext propertiesResource() {
return getRuleContext(PropertiesResourceContext.class,0);
}
public QualifiedGraphPrivilegesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_qualifiedGraphPrivileges; }
}
public final QualifiedGraphPrivilegesContext qualifiedGraphPrivileges() throws RecognitionException {
QualifiedGraphPrivilegesContext _localctx = new QualifiedGraphPrivilegesContext(_ctx, getState());
enterRule(_localctx, 536, RULE_qualifiedGraphPrivileges);
try {
enterOuterAlt(_localctx, 1);
{
setState(3198);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DELETE:
{
setState(3195);
match(DELETE);
}
break;
case MERGE:
{
setState(3196);
match(MERGE);
setState(3197);
propertiesResource();
}
break;
default:
throw new NoViableAltException(this);
}
setState(3200);
match(ON);
setState(3201);
graphScope();
setState(3202);
graphQualifier();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelsResourceContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public NonEmptyStringListContext nonEmptyStringList() {
return getRuleContext(NonEmptyStringListContext.class,0);
}
public LabelsResourceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_labelsResource; }
}
public final LabelsResourceContext labelsResource() throws RecognitionException {
LabelsResourceContext _localctx = new LabelsResourceContext(_ctx, getState());
enterRule(_localctx, 538, RULE_labelsResource);
try {
setState(3206);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
enterOuterAlt(_localctx, 1);
{
setState(3204);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 2);
{
setState(3205);
nonEmptyStringList();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PropertiesResourceContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LCURLY() { return getToken(Cypher6Parser.LCURLY, 0); }
public TerminalNode RCURLY() { return getToken(Cypher6Parser.RCURLY, 0); }
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public NonEmptyStringListContext nonEmptyStringList() {
return getRuleContext(NonEmptyStringListContext.class,0);
}
public PropertiesResourceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_propertiesResource; }
}
public final PropertiesResourceContext propertiesResource() throws RecognitionException {
PropertiesResourceContext _localctx = new PropertiesResourceContext(_ctx, getState());
enterRule(_localctx, 540, RULE_propertiesResource);
try {
enterOuterAlt(_localctx, 1);
{
setState(3208);
match(LCURLY);
setState(3211);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(3209);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
{
setState(3210);
nonEmptyStringList();
}
break;
default:
throw new NoViableAltException(this);
}
setState(3213);
match(RCURLY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NonEmptyStringListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public NonEmptyStringListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_nonEmptyStringList; }
}
public final NonEmptyStringListContext nonEmptyStringList() throws RecognitionException {
NonEmptyStringListContext _localctx = new NonEmptyStringListContext(_ctx, getState());
enterRule(_localctx, 542, RULE_nonEmptyStringList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3215);
symbolicNameString();
setState(3220);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(3216);
match(COMMA);
setState(3217);
symbolicNameString();
}
}
setState(3222);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GraphQualifierContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public GraphQualifierTokenContext graphQualifierToken() {
return getRuleContext(GraphQualifierTokenContext.class,0);
}
public TerminalNode FOR() { return getToken(Cypher6Parser.FOR, 0); }
public TerminalNode LPAREN() { return getToken(Cypher6Parser.LPAREN, 0); }
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public NonEmptyStringListContext nonEmptyStringList() {
return getRuleContext(NonEmptyStringListContext.class,0);
}
public TerminalNode RPAREN() { return getToken(Cypher6Parser.RPAREN, 0); }
public TerminalNode WHERE() { return getToken(Cypher6Parser.WHERE, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class,0);
}
public TerminalNode COLON() { return getToken(Cypher6Parser.COLON, 0); }
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class,i);
}
public MapContext map() {
return getRuleContext(MapContext.class,0);
}
public List BAR() { return getTokens(Cypher6Parser.BAR); }
public TerminalNode BAR(int i) {
return getToken(Cypher6Parser.BAR, i);
}
public GraphQualifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_graphQualifier; }
}
public final GraphQualifierContext graphQualifier() throws RecognitionException {
GraphQualifierContext _localctx = new GraphQualifierContext(_ctx, getState());
enterRule(_localctx, 544, RULE_graphQualifier);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3256);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ELEMENT:
case ELEMENTS:
case NODE:
case NODES:
case RELATIONSHIP:
case RELATIONSHIPS:
{
setState(3223);
graphQualifierToken();
setState(3226);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(3224);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
{
setState(3225);
nonEmptyStringList();
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
case FOR:
{
setState(3228);
match(FOR);
setState(3229);
match(LPAREN);
setState(3231);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,392,_ctx) ) {
case 1:
{
setState(3230);
variable();
}
break;
}
setState(3242);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COLON) {
{
setState(3233);
match(COLON);
setState(3234);
symbolicNameString();
setState(3239);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==BAR) {
{
{
setState(3235);
match(BAR);
setState(3236);
symbolicNameString();
}
}
setState(3241);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(3254);
_errHandler.sync(this);
switch (_input.LA(1)) {
case RPAREN:
{
setState(3244);
match(RPAREN);
setState(3245);
match(WHERE);
setState(3246);
expression();
}
break;
case LCURLY:
case WHERE:
{
setState(3250);
_errHandler.sync(this);
switch (_input.LA(1)) {
case WHERE:
{
setState(3247);
match(WHERE);
setState(3248);
expression();
}
break;
case LCURLY:
{
setState(3249);
map();
}
break;
default:
throw new NoViableAltException(this);
}
setState(3252);
match(RPAREN);
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
case FROM:
case LPAREN:
case TO:
break;
default:
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GraphQualifierTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public RelTokenContext relToken() {
return getRuleContext(RelTokenContext.class,0);
}
public NodeTokenContext nodeToken() {
return getRuleContext(NodeTokenContext.class,0);
}
public ElementTokenContext elementToken() {
return getRuleContext(ElementTokenContext.class,0);
}
public GraphQualifierTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_graphQualifierToken; }
}
public final GraphQualifierTokenContext graphQualifierToken() throws RecognitionException {
GraphQualifierTokenContext _localctx = new GraphQualifierTokenContext(_ctx, getState());
enterRule(_localctx, 546, RULE_graphQualifierToken);
try {
setState(3261);
_errHandler.sync(this);
switch (_input.LA(1)) {
case RELATIONSHIP:
case RELATIONSHIPS:
enterOuterAlt(_localctx, 1);
{
setState(3258);
relToken();
}
break;
case NODE:
case NODES:
enterOuterAlt(_localctx, 2);
{
setState(3259);
nodeToken();
}
break;
case ELEMENT:
case ELEMENTS:
enterOuterAlt(_localctx, 3);
{
setState(3260);
elementToken();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RelTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode RELATIONSHIP() { return getToken(Cypher6Parser.RELATIONSHIP, 0); }
public TerminalNode RELATIONSHIPS() { return getToken(Cypher6Parser.RELATIONSHIPS, 0); }
public RelTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_relToken; }
}
public final RelTokenContext relToken() throws RecognitionException {
RelTokenContext _localctx = new RelTokenContext(_ctx, getState());
enterRule(_localctx, 548, RULE_relToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3263);
_la = _input.LA(1);
if ( !(_la==RELATIONSHIP || _la==RELATIONSHIPS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ElementTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ELEMENT() { return getToken(Cypher6Parser.ELEMENT, 0); }
public TerminalNode ELEMENTS() { return getToken(Cypher6Parser.ELEMENTS, 0); }
public ElementTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_elementToken; }
}
public final ElementTokenContext elementToken() throws RecognitionException {
ElementTokenContext _localctx = new ElementTokenContext(_ctx, getState());
enterRule(_localctx, 550, RULE_elementToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3265);
_la = _input.LA(1);
if ( !(_la==ELEMENT || _la==ELEMENTS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NodeTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode NODE() { return getToken(Cypher6Parser.NODE, 0); }
public TerminalNode NODES() { return getToken(Cypher6Parser.NODES, 0); }
public NodeTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_nodeToken; }
}
public final NodeTokenContext nodeToken() throws RecognitionException {
NodeTokenContext _localctx = new NodeTokenContext(_ctx, getState());
enterRule(_localctx, 552, RULE_nodeToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3267);
_la = _input.LA(1);
if ( !(_la==NODE || _la==NODES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DatabaseScopeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public TerminalNode DEFAULT() { return getToken(Cypher6Parser.DEFAULT, 0); }
public TerminalNode HOME() { return getToken(Cypher6Parser.HOME, 0); }
public TerminalNode DATABASES() { return getToken(Cypher6Parser.DATABASES, 0); }
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public SymbolicAliasNameListContext symbolicAliasNameList() {
return getRuleContext(SymbolicAliasNameListContext.class,0);
}
public DatabaseScopeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_databaseScope; }
}
public final DatabaseScopeContext databaseScope() throws RecognitionException {
DatabaseScopeContext _localctx = new DatabaseScopeContext(_ctx, getState());
enterRule(_localctx, 554, RULE_databaseScope);
int _la;
try {
setState(3276);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DEFAULT:
case HOME:
enterOuterAlt(_localctx, 1);
{
setState(3269);
_la = _input.LA(1);
if ( !(_la==DEFAULT || _la==HOME) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3270);
match(DATABASE);
}
break;
case DATABASE:
case DATABASES:
enterOuterAlt(_localctx, 2);
{
setState(3271);
_la = _input.LA(1);
if ( !(_la==DATABASE || _la==DATABASES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3274);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(3272);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
{
setState(3273);
symbolicAliasNameList();
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GraphScopeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode GRAPH() { return getToken(Cypher6Parser.GRAPH, 0); }
public TerminalNode DEFAULT() { return getToken(Cypher6Parser.DEFAULT, 0); }
public TerminalNode HOME() { return getToken(Cypher6Parser.HOME, 0); }
public TerminalNode GRAPHS() { return getToken(Cypher6Parser.GRAPHS, 0); }
public TerminalNode TIMES() { return getToken(Cypher6Parser.TIMES, 0); }
public SymbolicAliasNameListContext symbolicAliasNameList() {
return getRuleContext(SymbolicAliasNameListContext.class,0);
}
public GraphScopeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_graphScope; }
}
public final GraphScopeContext graphScope() throws RecognitionException {
GraphScopeContext _localctx = new GraphScopeContext(_ctx, getState());
enterRule(_localctx, 556, RULE_graphScope);
int _la;
try {
setState(3285);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DEFAULT:
case HOME:
enterOuterAlt(_localctx, 1);
{
setState(3278);
_la = _input.LA(1);
if ( !(_la==DEFAULT || _la==HOME) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3279);
match(GRAPH);
}
break;
case GRAPH:
case GRAPHS:
enterOuterAlt(_localctx, 2);
{
setState(3280);
_la = _input.LA(1);
if ( !(_la==GRAPH || _la==GRAPHS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3283);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(3281);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
{
setState(3282);
symbolicAliasNameList();
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateCompositeDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COMPOSITE() { return getToken(Cypher6Parser.COMPOSITE, 0); }
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class,0);
}
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public CommandOptionsContext commandOptions() {
return getRuleContext(CommandOptionsContext.class,0);
}
public WaitClauseContext waitClause() {
return getRuleContext(WaitClauseContext.class,0);
}
public CreateCompositeDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createCompositeDatabase; }
}
public final CreateCompositeDatabaseContext createCompositeDatabase() throws RecognitionException {
CreateCompositeDatabaseContext _localctx = new CreateCompositeDatabaseContext(_ctx, getState());
enterRule(_localctx, 558, RULE_createCompositeDatabase);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3287);
match(COMPOSITE);
setState(3288);
match(DATABASE);
setState(3289);
symbolicAliasNameOrParameter();
setState(3293);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(3290);
match(IF);
setState(3291);
match(NOT);
setState(3292);
match(EXISTS);
}
}
setState(3296);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==OPTIONS) {
{
setState(3295);
commandOptions();
}
}
setState(3299);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==NOWAIT || _la==WAIT) {
{
setState(3298);
waitClause();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class,0);
}
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public TerminalNode TOPOLOGY() { return getToken(Cypher6Parser.TOPOLOGY, 0); }
public CommandOptionsContext commandOptions() {
return getRuleContext(CommandOptionsContext.class,0);
}
public WaitClauseContext waitClause() {
return getRuleContext(WaitClauseContext.class,0);
}
public List primaryTopology() {
return getRuleContexts(PrimaryTopologyContext.class);
}
public PrimaryTopologyContext primaryTopology(int i) {
return getRuleContext(PrimaryTopologyContext.class,i);
}
public List secondaryTopology() {
return getRuleContexts(SecondaryTopologyContext.class);
}
public SecondaryTopologyContext secondaryTopology(int i) {
return getRuleContext(SecondaryTopologyContext.class,i);
}
public CreateDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createDatabase; }
}
public final CreateDatabaseContext createDatabase() throws RecognitionException {
CreateDatabaseContext _localctx = new CreateDatabaseContext(_ctx, getState());
enterRule(_localctx, 560, RULE_createDatabase);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3301);
match(DATABASE);
setState(3302);
symbolicAliasNameOrParameter();
setState(3306);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(3303);
match(IF);
setState(3304);
match(NOT);
setState(3305);
match(EXISTS);
}
}
setState(3315);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==TOPOLOGY) {
{
setState(3308);
match(TOPOLOGY);
setState(3311);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
setState(3311);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,407,_ctx) ) {
case 1:
{
setState(3309);
primaryTopology();
}
break;
case 2:
{
setState(3310);
secondaryTopology();
}
break;
}
}
setState(3313);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==UNSIGNED_DECIMAL_INTEGER );
}
}
setState(3318);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==OPTIONS) {
{
setState(3317);
commandOptions();
}
}
setState(3321);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==NOWAIT || _la==WAIT) {
{
setState(3320);
waitClause();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PrimaryTopologyContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode UNSIGNED_DECIMAL_INTEGER() { return getToken(Cypher6Parser.UNSIGNED_DECIMAL_INTEGER, 0); }
public PrimaryTokenContext primaryToken() {
return getRuleContext(PrimaryTokenContext.class,0);
}
public PrimaryTopologyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_primaryTopology; }
}
public final PrimaryTopologyContext primaryTopology() throws RecognitionException {
PrimaryTopologyContext _localctx = new PrimaryTopologyContext(_ctx, getState());
enterRule(_localctx, 562, RULE_primaryTopology);
try {
enterOuterAlt(_localctx, 1);
{
setState(3323);
match(UNSIGNED_DECIMAL_INTEGER);
setState(3324);
primaryToken();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PrimaryTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PRIMARY() { return getToken(Cypher6Parser.PRIMARY, 0); }
public TerminalNode PRIMARIES() { return getToken(Cypher6Parser.PRIMARIES, 0); }
public PrimaryTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_primaryToken; }
}
public final PrimaryTokenContext primaryToken() throws RecognitionException {
PrimaryTokenContext _localctx = new PrimaryTokenContext(_ctx, getState());
enterRule(_localctx, 564, RULE_primaryToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3326);
_la = _input.LA(1);
if ( !(_la==PRIMARY || _la==PRIMARIES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SecondaryTopologyContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode UNSIGNED_DECIMAL_INTEGER() { return getToken(Cypher6Parser.UNSIGNED_DECIMAL_INTEGER, 0); }
public SecondaryTokenContext secondaryToken() {
return getRuleContext(SecondaryTokenContext.class,0);
}
public SecondaryTopologyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_secondaryTopology; }
}
public final SecondaryTopologyContext secondaryTopology() throws RecognitionException {
SecondaryTopologyContext _localctx = new SecondaryTopologyContext(_ctx, getState());
enterRule(_localctx, 566, RULE_secondaryTopology);
try {
enterOuterAlt(_localctx, 1);
{
setState(3328);
match(UNSIGNED_DECIMAL_INTEGER);
setState(3329);
secondaryToken();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SecondaryTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SECONDARY() { return getToken(Cypher6Parser.SECONDARY, 0); }
public TerminalNode SECONDARIES() { return getToken(Cypher6Parser.SECONDARIES, 0); }
public SecondaryTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_secondaryToken; }
}
public final SecondaryTokenContext secondaryToken() throws RecognitionException {
SecondaryTokenContext _localctx = new SecondaryTokenContext(_ctx, getState());
enterRule(_localctx, 568, RULE_secondaryToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3331);
_la = _input.LA(1);
if ( !(_la==SECONDARY || _la==SECONDARIES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class,0);
}
public TerminalNode COMPOSITE() { return getToken(Cypher6Parser.COMPOSITE, 0); }
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public AliasActionContext aliasAction() {
return getRuleContext(AliasActionContext.class,0);
}
public TerminalNode DATA() { return getToken(Cypher6Parser.DATA, 0); }
public WaitClauseContext waitClause() {
return getRuleContext(WaitClauseContext.class,0);
}
public TerminalNode DUMP() { return getToken(Cypher6Parser.DUMP, 0); }
public TerminalNode DESTROY() { return getToken(Cypher6Parser.DESTROY, 0); }
public DropDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dropDatabase; }
}
public final DropDatabaseContext dropDatabase() throws RecognitionException {
DropDatabaseContext _localctx = new DropDatabaseContext(_ctx, getState());
enterRule(_localctx, 570, RULE_dropDatabase);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3334);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COMPOSITE) {
{
setState(3333);
match(COMPOSITE);
}
}
setState(3336);
match(DATABASE);
setState(3337);
symbolicAliasNameOrParameter();
setState(3340);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(3338);
match(IF);
setState(3339);
match(EXISTS);
}
}
setState(3343);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==CASCADE || _la==RESTRICT) {
{
setState(3342);
aliasAction();
}
}
setState(3347);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DESTROY || _la==DUMP) {
{
setState(3345);
_la = _input.LA(1);
if ( !(_la==DESTROY || _la==DUMP) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3346);
match(DATA);
}
}
setState(3350);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==NOWAIT || _la==WAIT) {
{
setState(3349);
waitClause();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AliasActionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode RESTRICT() { return getToken(Cypher6Parser.RESTRICT, 0); }
public TerminalNode CASCADE() { return getToken(Cypher6Parser.CASCADE, 0); }
public TerminalNode ALIAS() { return getToken(Cypher6Parser.ALIAS, 0); }
public TerminalNode ALIASES() { return getToken(Cypher6Parser.ALIASES, 0); }
public AliasActionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_aliasAction; }
}
public final AliasActionContext aliasAction() throws RecognitionException {
AliasActionContext _localctx = new AliasActionContext(_ctx, getState());
enterRule(_localctx, 572, RULE_aliasAction);
int _la;
try {
setState(3355);
_errHandler.sync(this);
switch (_input.LA(1)) {
case RESTRICT:
enterOuterAlt(_localctx, 1);
{
setState(3352);
match(RESTRICT);
}
break;
case CASCADE:
enterOuterAlt(_localctx, 2);
{
setState(3353);
match(CASCADE);
setState(3354);
_la = _input.LA(1);
if ( !(_la==ALIAS || _la==ALIASES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class,0);
}
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public WaitClauseContext waitClause() {
return getRuleContext(WaitClauseContext.class,0);
}
public List SET() { return getTokens(Cypher6Parser.SET); }
public TerminalNode SET(int i) {
return getToken(Cypher6Parser.SET, i);
}
public List REMOVE() { return getTokens(Cypher6Parser.REMOVE); }
public TerminalNode REMOVE(int i) {
return getToken(Cypher6Parser.REMOVE, i);
}
public List OPTION() { return getTokens(Cypher6Parser.OPTION); }
public TerminalNode OPTION(int i) {
return getToken(Cypher6Parser.OPTION, i);
}
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class,i);
}
public List alterDatabaseAccess() {
return getRuleContexts(AlterDatabaseAccessContext.class);
}
public AlterDatabaseAccessContext alterDatabaseAccess(int i) {
return getRuleContext(AlterDatabaseAccessContext.class,i);
}
public List alterDatabaseTopology() {
return getRuleContexts(AlterDatabaseTopologyContext.class);
}
public AlterDatabaseTopologyContext alterDatabaseTopology(int i) {
return getRuleContext(AlterDatabaseTopologyContext.class,i);
}
public List alterDatabaseOption() {
return getRuleContexts(AlterDatabaseOptionContext.class);
}
public AlterDatabaseOptionContext alterDatabaseOption(int i) {
return getRuleContext(AlterDatabaseOptionContext.class,i);
}
public AlterDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterDatabase; }
}
public final AlterDatabaseContext alterDatabase() throws RecognitionException {
AlterDatabaseContext _localctx = new AlterDatabaseContext(_ctx, getState());
enterRule(_localctx, 574, RULE_alterDatabase);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3357);
match(DATABASE);
setState(3358);
symbolicAliasNameOrParameter();
setState(3361);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(3359);
match(IF);
setState(3360);
match(EXISTS);
}
}
setState(3380);
_errHandler.sync(this);
switch (_input.LA(1)) {
case SET:
{
setState(3369);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(3363);
match(SET);
setState(3367);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ACCESS:
{
setState(3364);
alterDatabaseAccess();
}
break;
case TOPOLOGY:
{
setState(3365);
alterDatabaseTopology();
}
break;
case OPTION:
{
setState(3366);
alterDatabaseOption();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
setState(3371);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==SET );
}
break;
case REMOVE:
{
setState(3376);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(3373);
match(REMOVE);
setState(3374);
match(OPTION);
setState(3375);
symbolicNameString();
}
}
setState(3378);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==REMOVE );
}
break;
default:
throw new NoViableAltException(this);
}
setState(3383);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==NOWAIT || _la==WAIT) {
{
setState(3382);
waitClause();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterDatabaseAccessContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ACCESS() { return getToken(Cypher6Parser.ACCESS, 0); }
public TerminalNode READ() { return getToken(Cypher6Parser.READ, 0); }
public TerminalNode ONLY() { return getToken(Cypher6Parser.ONLY, 0); }
public TerminalNode WRITE() { return getToken(Cypher6Parser.WRITE, 0); }
public AlterDatabaseAccessContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterDatabaseAccess; }
}
public final AlterDatabaseAccessContext alterDatabaseAccess() throws RecognitionException {
AlterDatabaseAccessContext _localctx = new AlterDatabaseAccessContext(_ctx, getState());
enterRule(_localctx, 576, RULE_alterDatabaseAccess);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3385);
match(ACCESS);
setState(3386);
match(READ);
setState(3387);
_la = _input.LA(1);
if ( !(_la==ONLY || _la==WRITE) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterDatabaseTopologyContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode TOPOLOGY() { return getToken(Cypher6Parser.TOPOLOGY, 0); }
public List primaryTopology() {
return getRuleContexts(PrimaryTopologyContext.class);
}
public PrimaryTopologyContext primaryTopology(int i) {
return getRuleContext(PrimaryTopologyContext.class,i);
}
public List secondaryTopology() {
return getRuleContexts(SecondaryTopologyContext.class);
}
public SecondaryTopologyContext secondaryTopology(int i) {
return getRuleContext(SecondaryTopologyContext.class,i);
}
public AlterDatabaseTopologyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterDatabaseTopology; }
}
public final AlterDatabaseTopologyContext alterDatabaseTopology() throws RecognitionException {
AlterDatabaseTopologyContext _localctx = new AlterDatabaseTopologyContext(_ctx, getState());
enterRule(_localctx, 578, RULE_alterDatabaseTopology);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3389);
match(TOPOLOGY);
setState(3392);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
setState(3392);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,424,_ctx) ) {
case 1:
{
setState(3390);
primaryTopology();
}
break;
case 2:
{
setState(3391);
secondaryTopology();
}
break;
}
}
setState(3394);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==UNSIGNED_DECIMAL_INTEGER );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterDatabaseOptionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode OPTION() { return getToken(Cypher6Parser.OPTION, 0); }
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public AlterDatabaseOptionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterDatabaseOption; }
}
public final AlterDatabaseOptionContext alterDatabaseOption() throws RecognitionException {
AlterDatabaseOptionContext _localctx = new AlterDatabaseOptionContext(_ctx, getState());
enterRule(_localctx, 580, RULE_alterDatabaseOption);
try {
enterOuterAlt(_localctx, 1);
{
setState(3396);
match(OPTION);
setState(3397);
symbolicNameString();
setState(3398);
expression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StartDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode START() { return getToken(Cypher6Parser.START, 0); }
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class,0);
}
public WaitClauseContext waitClause() {
return getRuleContext(WaitClauseContext.class,0);
}
public StartDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_startDatabase; }
}
public final StartDatabaseContext startDatabase() throws RecognitionException {
StartDatabaseContext _localctx = new StartDatabaseContext(_ctx, getState());
enterRule(_localctx, 582, RULE_startDatabase);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3400);
match(START);
setState(3401);
match(DATABASE);
setState(3402);
symbolicAliasNameOrParameter();
setState(3404);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==NOWAIT || _la==WAIT) {
{
setState(3403);
waitClause();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StopDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode STOP() { return getToken(Cypher6Parser.STOP, 0); }
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class,0);
}
public WaitClauseContext waitClause() {
return getRuleContext(WaitClauseContext.class,0);
}
public StopDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_stopDatabase; }
}
public final StopDatabaseContext stopDatabase() throws RecognitionException {
StopDatabaseContext _localctx = new StopDatabaseContext(_ctx, getState());
enterRule(_localctx, 584, RULE_stopDatabase);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3406);
match(STOP);
setState(3407);
match(DATABASE);
setState(3408);
symbolicAliasNameOrParameter();
setState(3410);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==NOWAIT || _la==WAIT) {
{
setState(3409);
waitClause();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class WaitClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode WAIT() { return getToken(Cypher6Parser.WAIT, 0); }
public TerminalNode UNSIGNED_DECIMAL_INTEGER() { return getToken(Cypher6Parser.UNSIGNED_DECIMAL_INTEGER, 0); }
public SecondsTokenContext secondsToken() {
return getRuleContext(SecondsTokenContext.class,0);
}
public TerminalNode NOWAIT() { return getToken(Cypher6Parser.NOWAIT, 0); }
public WaitClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_waitClause; }
}
public final WaitClauseContext waitClause() throws RecognitionException {
WaitClauseContext _localctx = new WaitClauseContext(_ctx, getState());
enterRule(_localctx, 586, RULE_waitClause);
int _la;
try {
setState(3420);
_errHandler.sync(this);
switch (_input.LA(1)) {
case WAIT:
enterOuterAlt(_localctx, 1);
{
setState(3412);
match(WAIT);
setState(3417);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==UNSIGNED_DECIMAL_INTEGER) {
{
setState(3413);
match(UNSIGNED_DECIMAL_INTEGER);
setState(3415);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 236)) & ~0x3f) == 0 && ((1L << (_la - 236)) & 19L) != 0)) {
{
setState(3414);
secondsToken();
}
}
}
}
}
break;
case NOWAIT:
enterOuterAlt(_localctx, 2);
{
setState(3419);
match(NOWAIT);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SecondsTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SEC() { return getToken(Cypher6Parser.SEC, 0); }
public TerminalNode SECOND() { return getToken(Cypher6Parser.SECOND, 0); }
public TerminalNode SECONDS() { return getToken(Cypher6Parser.SECONDS, 0); }
public SecondsTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_secondsToken; }
}
public final SecondsTokenContext secondsToken() throws RecognitionException {
SecondsTokenContext _localctx = new SecondsTokenContext(_ctx, getState());
enterRule(_localctx, 588, RULE_secondsToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3422);
_la = _input.LA(1);
if ( !(((((_la - 236)) & ~0x3f) == 0 && ((1L << (_la - 236)) & 19L) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public TerminalNode DEFAULT() { return getToken(Cypher6Parser.DEFAULT, 0); }
public TerminalNode HOME() { return getToken(Cypher6Parser.HOME, 0); }
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class,0);
}
public TerminalNode DATABASES() { return getToken(Cypher6Parser.DATABASES, 0); }
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class,0);
}
public ShowDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showDatabase; }
}
public final ShowDatabaseContext showDatabase() throws RecognitionException {
ShowDatabaseContext _localctx = new ShowDatabaseContext(_ctx, getState());
enterRule(_localctx, 590, RULE_showDatabase);
int _la;
try {
setState(3436);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DEFAULT:
case HOME:
enterOuterAlt(_localctx, 1);
{
setState(3424);
_la = _input.LA(1);
if ( !(_la==DEFAULT || _la==HOME) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3425);
match(DATABASE);
setState(3427);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(3426);
showCommandYield();
}
}
}
break;
case DATABASE:
case DATABASES:
enterOuterAlt(_localctx, 2);
{
setState(3429);
_la = _input.LA(1);
if ( !(_la==DATABASE || _la==DATABASES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3431);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,432,_ctx) ) {
case 1:
{
setState(3430);
symbolicAliasNameOrParameter();
}
break;
}
setState(3434);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(3433);
showCommandYield();
}
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AliasNameContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class,0);
}
public AliasNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_aliasName; }
}
public final AliasNameContext aliasName() throws RecognitionException {
AliasNameContext _localctx = new AliasNameContext(_ctx, getState());
enterRule(_localctx, 592, RULE_aliasName);
try {
enterOuterAlt(_localctx, 1);
{
setState(3438);
symbolicAliasNameOrParameter();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DatabaseNameContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class,0);
}
public DatabaseNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_databaseName; }
}
public final DatabaseNameContext databaseName() throws RecognitionException {
DatabaseNameContext _localctx = new DatabaseNameContext(_ctx, getState());
enterRule(_localctx, 594, RULE_databaseName);
try {
enterOuterAlt(_localctx, 1);
{
setState(3440);
symbolicAliasNameOrParameter();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateAliasContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ALIAS() { return getToken(Cypher6Parser.ALIAS, 0); }
public AliasNameContext aliasName() {
return getRuleContext(AliasNameContext.class,0);
}
public TerminalNode FOR() { return getToken(Cypher6Parser.FOR, 0); }
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public DatabaseNameContext databaseName() {
return getRuleContext(DatabaseNameContext.class,0);
}
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public TerminalNode AT() { return getToken(Cypher6Parser.AT, 0); }
public StringOrParameterContext stringOrParameter() {
return getRuleContext(StringOrParameterContext.class,0);
}
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public CommandNameExpressionContext commandNameExpression() {
return getRuleContext(CommandNameExpressionContext.class,0);
}
public TerminalNode PASSWORD() { return getToken(Cypher6Parser.PASSWORD, 0); }
public PasswordExpressionContext passwordExpression() {
return getRuleContext(PasswordExpressionContext.class,0);
}
public TerminalNode PROPERTIES() { return getToken(Cypher6Parser.PROPERTIES, 0); }
public List mapOrParameter() {
return getRuleContexts(MapOrParameterContext.class);
}
public MapOrParameterContext mapOrParameter(int i) {
return getRuleContext(MapOrParameterContext.class,i);
}
public TerminalNode DRIVER() { return getToken(Cypher6Parser.DRIVER, 0); }
public CreateAliasContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_createAlias; }
}
public final CreateAliasContext createAlias() throws RecognitionException {
CreateAliasContext _localctx = new CreateAliasContext(_ctx, getState());
enterRule(_localctx, 596, RULE_createAlias);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3442);
match(ALIAS);
setState(3443);
aliasName();
setState(3447);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(3444);
match(IF);
setState(3445);
match(NOT);
setState(3446);
match(EXISTS);
}
}
setState(3449);
match(FOR);
setState(3450);
match(DATABASE);
setState(3451);
databaseName();
setState(3462);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AT) {
{
setState(3452);
match(AT);
setState(3453);
stringOrParameter();
setState(3454);
match(USER);
setState(3455);
commandNameExpression();
setState(3456);
match(PASSWORD);
setState(3457);
passwordExpression();
setState(3460);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DRIVER) {
{
setState(3458);
match(DRIVER);
setState(3459);
mapOrParameter();
}
}
}
}
setState(3466);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==PROPERTIES) {
{
setState(3464);
match(PROPERTIES);
setState(3465);
mapOrParameter();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropAliasContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ALIAS() { return getToken(Cypher6Parser.ALIAS, 0); }
public AliasNameContext aliasName() {
return getRuleContext(AliasNameContext.class,0);
}
public TerminalNode FOR() { return getToken(Cypher6Parser.FOR, 0); }
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public DropAliasContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dropAlias; }
}
public final DropAliasContext dropAlias() throws RecognitionException {
DropAliasContext _localctx = new DropAliasContext(_ctx, getState());
enterRule(_localctx, 598, RULE_dropAlias);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3468);
match(ALIAS);
setState(3469);
aliasName();
setState(3472);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(3470);
match(IF);
setState(3471);
match(EXISTS);
}
}
setState(3474);
match(FOR);
setState(3475);
match(DATABASE);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterAliasContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ALIAS() { return getToken(Cypher6Parser.ALIAS, 0); }
public AliasNameContext aliasName() {
return getRuleContext(AliasNameContext.class,0);
}
public TerminalNode SET() { return getToken(Cypher6Parser.SET, 0); }
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public List alterAliasTarget() {
return getRuleContexts(AlterAliasTargetContext.class);
}
public AlterAliasTargetContext alterAliasTarget(int i) {
return getRuleContext(AlterAliasTargetContext.class,i);
}
public List alterAliasUser() {
return getRuleContexts(AlterAliasUserContext.class);
}
public AlterAliasUserContext alterAliasUser(int i) {
return getRuleContext(AlterAliasUserContext.class,i);
}
public List alterAliasPassword() {
return getRuleContexts(AlterAliasPasswordContext.class);
}
public AlterAliasPasswordContext alterAliasPassword(int i) {
return getRuleContext(AlterAliasPasswordContext.class,i);
}
public List alterAliasDriver() {
return getRuleContexts(AlterAliasDriverContext.class);
}
public AlterAliasDriverContext alterAliasDriver(int i) {
return getRuleContext(AlterAliasDriverContext.class,i);
}
public List alterAliasProperties() {
return getRuleContexts(AlterAliasPropertiesContext.class);
}
public AlterAliasPropertiesContext alterAliasProperties(int i) {
return getRuleContext(AlterAliasPropertiesContext.class,i);
}
public AlterAliasContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterAlias; }
}
public final AlterAliasContext alterAlias() throws RecognitionException {
AlterAliasContext _localctx = new AlterAliasContext(_ctx, getState());
enterRule(_localctx, 600, RULE_alterAlias);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3477);
match(ALIAS);
setState(3478);
aliasName();
setState(3481);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IF) {
{
setState(3479);
match(IF);
setState(3480);
match(EXISTS);
}
}
setState(3483);
match(SET);
setState(3484);
match(DATABASE);
setState(3490);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
setState(3490);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TARGET:
{
setState(3485);
alterAliasTarget();
}
break;
case USER:
{
setState(3486);
alterAliasUser();
}
break;
case PASSWORD:
{
setState(3487);
alterAliasPassword();
}
break;
case DRIVER:
{
setState(3488);
alterAliasDriver();
}
break;
case PROPERTIES:
{
setState(3489);
alterAliasProperties();
}
break;
default:
throw new NoViableAltException(this);
}
}
setState(3492);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==DRIVER || _la==PASSWORD || _la==PROPERTIES || _la==TARGET || _la==USER );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterAliasTargetContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode TARGET() { return getToken(Cypher6Parser.TARGET, 0); }
public DatabaseNameContext databaseName() {
return getRuleContext(DatabaseNameContext.class,0);
}
public TerminalNode AT() { return getToken(Cypher6Parser.AT, 0); }
public StringOrParameterContext stringOrParameter() {
return getRuleContext(StringOrParameterContext.class,0);
}
public AlterAliasTargetContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterAliasTarget; }
}
public final AlterAliasTargetContext alterAliasTarget() throws RecognitionException {
AlterAliasTargetContext _localctx = new AlterAliasTargetContext(_ctx, getState());
enterRule(_localctx, 602, RULE_alterAliasTarget);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3494);
match(TARGET);
setState(3495);
databaseName();
setState(3498);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AT) {
{
setState(3496);
match(AT);
setState(3497);
stringOrParameter();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterAliasUserContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public CommandNameExpressionContext commandNameExpression() {
return getRuleContext(CommandNameExpressionContext.class,0);
}
public AlterAliasUserContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterAliasUser; }
}
public final AlterAliasUserContext alterAliasUser() throws RecognitionException {
AlterAliasUserContext _localctx = new AlterAliasUserContext(_ctx, getState());
enterRule(_localctx, 604, RULE_alterAliasUser);
try {
enterOuterAlt(_localctx, 1);
{
setState(3500);
match(USER);
setState(3501);
commandNameExpression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterAliasPasswordContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PASSWORD() { return getToken(Cypher6Parser.PASSWORD, 0); }
public PasswordExpressionContext passwordExpression() {
return getRuleContext(PasswordExpressionContext.class,0);
}
public AlterAliasPasswordContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterAliasPassword; }
}
public final AlterAliasPasswordContext alterAliasPassword() throws RecognitionException {
AlterAliasPasswordContext _localctx = new AlterAliasPasswordContext(_ctx, getState());
enterRule(_localctx, 606, RULE_alterAliasPassword);
try {
enterOuterAlt(_localctx, 1);
{
setState(3503);
match(PASSWORD);
setState(3504);
passwordExpression();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterAliasDriverContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DRIVER() { return getToken(Cypher6Parser.DRIVER, 0); }
public MapOrParameterContext mapOrParameter() {
return getRuleContext(MapOrParameterContext.class,0);
}
public AlterAliasDriverContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterAliasDriver; }
}
public final AlterAliasDriverContext alterAliasDriver() throws RecognitionException {
AlterAliasDriverContext _localctx = new AlterAliasDriverContext(_ctx, getState());
enterRule(_localctx, 608, RULE_alterAliasDriver);
try {
enterOuterAlt(_localctx, 1);
{
setState(3506);
match(DRIVER);
setState(3507);
mapOrParameter();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterAliasPropertiesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PROPERTIES() { return getToken(Cypher6Parser.PROPERTIES, 0); }
public MapOrParameterContext mapOrParameter() {
return getRuleContext(MapOrParameterContext.class,0);
}
public AlterAliasPropertiesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_alterAliasProperties; }
}
public final AlterAliasPropertiesContext alterAliasProperties() throws RecognitionException {
AlterAliasPropertiesContext _localctx = new AlterAliasPropertiesContext(_ctx, getState());
enterRule(_localctx, 610, RULE_alterAliasProperties);
try {
enterOuterAlt(_localctx, 1);
{
setState(3509);
match(PROPERTIES);
setState(3510);
mapOrParameter();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowAliasesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode FOR() { return getToken(Cypher6Parser.FOR, 0); }
public TerminalNode ALIAS() { return getToken(Cypher6Parser.ALIAS, 0); }
public TerminalNode ALIASES() { return getToken(Cypher6Parser.ALIASES, 0); }
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public TerminalNode DATABASES() { return getToken(Cypher6Parser.DATABASES, 0); }
public AliasNameContext aliasName() {
return getRuleContext(AliasNameContext.class,0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class,0);
}
public ShowAliasesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_showAliases; }
}
public final ShowAliasesContext showAliases() throws RecognitionException {
ShowAliasesContext _localctx = new ShowAliasesContext(_ctx, getState());
enterRule(_localctx, 612, RULE_showAliases);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3512);
_la = _input.LA(1);
if ( !(_la==ALIAS || _la==ALIASES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3514);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,444,_ctx) ) {
case 1:
{
setState(3513);
aliasName();
}
break;
}
setState(3516);
match(FOR);
setState(3517);
_la = _input.LA(1);
if ( !(_la==DATABASE || _la==DATABASES) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3519);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==WHERE || _la==YIELD) {
{
setState(3518);
showCommandYield();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SymbolicNameOrStringParameterContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class,0);
}
public SymbolicNameOrStringParameterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_symbolicNameOrStringParameter; }
}
public final SymbolicNameOrStringParameterContext symbolicNameOrStringParameter() throws RecognitionException {
SymbolicNameOrStringParameterContext _localctx = new SymbolicNameOrStringParameterContext(_ctx, getState());
enterRule(_localctx, 614, RULE_symbolicNameOrStringParameter);
try {
setState(3523);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 1);
{
setState(3521);
symbolicNameString();
}
break;
case DOLLAR:
enterOuterAlt(_localctx, 2);
{
setState(3522);
parameter("STRING");
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CommandNameExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class,0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class,0);
}
public CommandNameExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_commandNameExpression; }
}
public final CommandNameExpressionContext commandNameExpression() throws RecognitionException {
CommandNameExpressionContext _localctx = new CommandNameExpressionContext(_ctx, getState());
enterRule(_localctx, 616, RULE_commandNameExpression);
try {
setState(3527);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 1);
{
setState(3525);
symbolicNameString();
}
break;
case DOLLAR:
enterOuterAlt(_localctx, 2);
{
setState(3526);
parameter("STRING");
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SymbolicNameOrStringParameterListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List commandNameExpression() {
return getRuleContexts(CommandNameExpressionContext.class);
}
public CommandNameExpressionContext commandNameExpression(int i) {
return getRuleContext(CommandNameExpressionContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public SymbolicNameOrStringParameterListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_symbolicNameOrStringParameterList; }
}
public final SymbolicNameOrStringParameterListContext symbolicNameOrStringParameterList() throws RecognitionException {
SymbolicNameOrStringParameterListContext _localctx = new SymbolicNameOrStringParameterListContext(_ctx, getState());
enterRule(_localctx, 618, RULE_symbolicNameOrStringParameterList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3529);
commandNameExpression();
setState(3534);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(3530);
match(COMMA);
setState(3531);
commandNameExpression();
}
}
setState(3536);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SymbolicAliasNameListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List symbolicAliasNameOrParameter() {
return getRuleContexts(SymbolicAliasNameOrParameterContext.class);
}
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter(int i) {
return getRuleContext(SymbolicAliasNameOrParameterContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public SymbolicAliasNameListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_symbolicAliasNameList; }
}
public final SymbolicAliasNameListContext symbolicAliasNameList() throws RecognitionException {
SymbolicAliasNameListContext _localctx = new SymbolicAliasNameListContext(_ctx, getState());
enterRule(_localctx, 620, RULE_symbolicAliasNameList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3537);
symbolicAliasNameOrParameter();
setState(3542);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(3538);
match(COMMA);
setState(3539);
symbolicAliasNameOrParameter();
}
}
setState(3544);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SymbolicAliasNameOrParameterContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicAliasNameContext symbolicAliasName() {
return getRuleContext(SymbolicAliasNameContext.class,0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class,0);
}
public SymbolicAliasNameOrParameterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_symbolicAliasNameOrParameter; }
}
public final SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() throws RecognitionException {
SymbolicAliasNameOrParameterContext _localctx = new SymbolicAliasNameOrParameterContext(_ctx, getState());
enterRule(_localctx, 622, RULE_symbolicAliasNameOrParameter);
try {
setState(3547);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 1);
{
setState(3545);
symbolicAliasName();
}
break;
case DOLLAR:
enterOuterAlt(_localctx, 2);
{
setState(3546);
parameter("STRING");
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SymbolicAliasNameContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class,i);
}
public List DOT() { return getTokens(Cypher6Parser.DOT); }
public TerminalNode DOT(int i) {
return getToken(Cypher6Parser.DOT, i);
}
public SymbolicAliasNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_symbolicAliasName; }
}
public final SymbolicAliasNameContext symbolicAliasName() throws RecognitionException {
SymbolicAliasNameContext _localctx = new SymbolicAliasNameContext(_ctx, getState());
enterRule(_localctx, 624, RULE_symbolicAliasName);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3549);
symbolicNameString();
setState(3554);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==DOT) {
{
{
setState(3550);
match(DOT);
setState(3551);
symbolicNameString();
}
}
setState(3556);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StringListLiteralContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LBRACKET() { return getToken(Cypher6Parser.LBRACKET, 0); }
public TerminalNode RBRACKET() { return getToken(Cypher6Parser.RBRACKET, 0); }
public List stringLiteral() {
return getRuleContexts(StringLiteralContext.class);
}
public StringLiteralContext stringLiteral(int i) {
return getRuleContext(StringLiteralContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public StringListLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_stringListLiteral; }
}
public final StringListLiteralContext stringListLiteral() throws RecognitionException {
StringListLiteralContext _localctx = new StringListLiteralContext(_ctx, getState());
enterRule(_localctx, 626, RULE_stringListLiteral);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3557);
match(LBRACKET);
setState(3566);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==STRING_LITERAL1 || _la==STRING_LITERAL2) {
{
setState(3558);
stringLiteral();
setState(3563);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(3559);
match(COMMA);
setState(3560);
stringLiteral();
}
}
setState(3565);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(3568);
match(RBRACKET);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StringListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List stringLiteral() {
return getRuleContexts(StringLiteralContext.class);
}
public StringLiteralContext stringLiteral(int i) {
return getRuleContext(StringLiteralContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public StringListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_stringList; }
}
public final StringListContext stringList() throws RecognitionException {
StringListContext _localctx = new StringListContext(_ctx, getState());
enterRule(_localctx, 628, RULE_stringList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3570);
stringLiteral();
setState(3573);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(3571);
match(COMMA);
setState(3572);
stringLiteral();
}
}
setState(3575);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==COMMA );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StringLiteralContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode STRING_LITERAL1() { return getToken(Cypher6Parser.STRING_LITERAL1, 0); }
public TerminalNode STRING_LITERAL2() { return getToken(Cypher6Parser.STRING_LITERAL2, 0); }
public StringLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_stringLiteral; }
}
public final StringLiteralContext stringLiteral() throws RecognitionException {
StringLiteralContext _localctx = new StringLiteralContext(_ctx, getState());
enterRule(_localctx, 630, RULE_stringLiteral);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3577);
_la = _input.LA(1);
if ( !(_la==STRING_LITERAL1 || _la==STRING_LITERAL2) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StringOrParameterExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class,0);
}
public StringOrParameterExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_stringOrParameterExpression; }
}
public final StringOrParameterExpressionContext stringOrParameterExpression() throws RecognitionException {
StringOrParameterExpressionContext _localctx = new StringOrParameterExpressionContext(_ctx, getState());
enterRule(_localctx, 632, RULE_stringOrParameterExpression);
try {
setState(3581);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STRING_LITERAL1:
case STRING_LITERAL2:
enterOuterAlt(_localctx, 1);
{
setState(3579);
stringLiteral();
}
break;
case DOLLAR:
enterOuterAlt(_localctx, 2);
{
setState(3580);
parameter("STRING");
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StringOrParameterContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class,0);
}
public StringOrParameterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_stringOrParameter; }
}
public final StringOrParameterContext stringOrParameter() throws RecognitionException {
StringOrParameterContext _localctx = new StringOrParameterContext(_ctx, getState());
enterRule(_localctx, 634, RULE_stringOrParameter);
try {
setState(3585);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STRING_LITERAL1:
case STRING_LITERAL2:
enterOuterAlt(_localctx, 1);
{
setState(3583);
stringLiteral();
}
break;
case DOLLAR:
enterOuterAlt(_localctx, 2);
{
setState(3584);
parameter("STRING");
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MapOrParameterContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public MapContext map() {
return getRuleContext(MapContext.class,0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class,0);
}
public MapOrParameterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_mapOrParameter; }
}
public final MapOrParameterContext mapOrParameter() throws RecognitionException {
MapOrParameterContext _localctx = new MapOrParameterContext(_ctx, getState());
enterRule(_localctx, 636, RULE_mapOrParameter);
try {
setState(3589);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LCURLY:
enterOuterAlt(_localctx, 1);
{
setState(3587);
map();
}
break;
case DOLLAR:
enterOuterAlt(_localctx, 2);
{
setState(3588);
parameter("MAP");
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MapContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LCURLY() { return getToken(Cypher6Parser.LCURLY, 0); }
public TerminalNode RCURLY() { return getToken(Cypher6Parser.RCURLY, 0); }
public List propertyKeyName() {
return getRuleContexts(PropertyKeyNameContext.class);
}
public PropertyKeyNameContext propertyKeyName(int i) {
return getRuleContext(PropertyKeyNameContext.class,i);
}
public List COLON() { return getTokens(Cypher6Parser.COLON); }
public TerminalNode COLON(int i) {
return getToken(Cypher6Parser.COLON, i);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public List COMMA() { return getTokens(Cypher6Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(Cypher6Parser.COMMA, i);
}
public MapContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_map; }
}
public final MapContext map() throws RecognitionException {
MapContext _localctx = new MapContext(_ctx, getState());
enterRule(_localctx, 638, RULE_map);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3591);
match(LCURLY);
setState(3605);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -246291141493760L) != 0) || ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -146366996479975425L) != 0) || ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -16156712961L) != 0) || ((((_la - 192)) & ~0x3f) == 0 && ((1L << (_la - 192)) & -1130297988612173L) != 0) || ((((_la - 256)) & ~0x3f) == 0 && ((1L << (_la - 256)) & 281474976709631L) != 0)) {
{
setState(3592);
propertyKeyName();
setState(3593);
match(COLON);
setState(3594);
expression();
setState(3602);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(3595);
match(COMMA);
setState(3596);
propertyKeyName();
setState(3597);
match(COLON);
setState(3598);
expression();
}
}
setState(3604);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(3607);
match(RCURLY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SymbolicNameStringContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public EscapedSymbolicNameStringContext escapedSymbolicNameString() {
return getRuleContext(EscapedSymbolicNameStringContext.class,0);
}
public UnescapedSymbolicNameStringContext unescapedSymbolicNameString() {
return getRuleContext(UnescapedSymbolicNameStringContext.class,0);
}
public SymbolicNameStringContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_symbolicNameString; }
}
public final SymbolicNameStringContext symbolicNameString() throws RecognitionException {
SymbolicNameStringContext _localctx = new SymbolicNameStringContext(_ctx, getState());
enterRule(_localctx, 640, RULE_symbolicNameString);
try {
setState(3611);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
enterOuterAlt(_localctx, 1);
{
setState(3609);
escapedSymbolicNameString();
}
break;
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 2);
{
setState(3610);
unescapedSymbolicNameString();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class EscapedSymbolicNameStringContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ESCAPED_SYMBOLIC_NAME() { return getToken(Cypher6Parser.ESCAPED_SYMBOLIC_NAME, 0); }
public EscapedSymbolicNameStringContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_escapedSymbolicNameString; }
}
public final EscapedSymbolicNameStringContext escapedSymbolicNameString() throws RecognitionException {
EscapedSymbolicNameStringContext _localctx = new EscapedSymbolicNameStringContext(_ctx, getState());
enterRule(_localctx, 642, RULE_escapedSymbolicNameString);
try {
enterOuterAlt(_localctx, 1);
{
setState(3613);
match(ESCAPED_SYMBOLIC_NAME);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UnescapedSymbolicNameStringContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public UnescapedLabelSymbolicNameStringContext unescapedLabelSymbolicNameString() {
return getRuleContext(UnescapedLabelSymbolicNameStringContext.class,0);
}
public TerminalNode NOT() { return getToken(Cypher6Parser.NOT, 0); }
public TerminalNode NULL() { return getToken(Cypher6Parser.NULL, 0); }
public TerminalNode TYPED() { return getToken(Cypher6Parser.TYPED, 0); }
public TerminalNode NORMALIZED() { return getToken(Cypher6Parser.NORMALIZED, 0); }
public TerminalNode NFC() { return getToken(Cypher6Parser.NFC, 0); }
public TerminalNode NFD() { return getToken(Cypher6Parser.NFD, 0); }
public TerminalNode NFKC() { return getToken(Cypher6Parser.NFKC, 0); }
public TerminalNode NFKD() { return getToken(Cypher6Parser.NFKD, 0); }
public UnescapedSymbolicNameStringContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_unescapedSymbolicNameString; }
}
public final UnescapedSymbolicNameStringContext unescapedSymbolicNameString() throws RecognitionException {
UnescapedSymbolicNameStringContext _localctx = new UnescapedSymbolicNameStringContext(_ctx, getState());
enterRule(_localctx, 644, RULE_unescapedSymbolicNameString);
try {
setState(3624);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NOTHING:
case NOWAIT:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 1);
{
setState(3615);
unescapedLabelSymbolicNameString();
}
break;
case NOT:
enterOuterAlt(_localctx, 2);
{
setState(3616);
match(NOT);
}
break;
case NULL:
enterOuterAlt(_localctx, 3);
{
setState(3617);
match(NULL);
}
break;
case TYPED:
enterOuterAlt(_localctx, 4);
{
setState(3618);
match(TYPED);
}
break;
case NORMALIZED:
enterOuterAlt(_localctx, 5);
{
setState(3619);
match(NORMALIZED);
}
break;
case NFC:
enterOuterAlt(_localctx, 6);
{
setState(3620);
match(NFC);
}
break;
case NFD:
enterOuterAlt(_localctx, 7);
{
setState(3621);
match(NFD);
}
break;
case NFKC:
enterOuterAlt(_localctx, 8);
{
setState(3622);
match(NFKC);
}
break;
case NFKD:
enterOuterAlt(_localctx, 9);
{
setState(3623);
match(NFKD);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SymbolicLabelNameStringContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public EscapedSymbolicNameStringContext escapedSymbolicNameString() {
return getRuleContext(EscapedSymbolicNameStringContext.class,0);
}
public UnescapedLabelSymbolicNameStringContext unescapedLabelSymbolicNameString() {
return getRuleContext(UnescapedLabelSymbolicNameStringContext.class,0);
}
public SymbolicLabelNameStringContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_symbolicLabelNameString; }
}
public final SymbolicLabelNameStringContext symbolicLabelNameString() throws RecognitionException {
SymbolicLabelNameStringContext _localctx = new SymbolicLabelNameStringContext(_ctx, getState());
enterRule(_localctx, 646, RULE_symbolicLabelNameString);
try {
setState(3628);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
enterOuterAlt(_localctx, 1);
{
setState(3626);
escapedSymbolicNameString();
}
break;
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASCENDING:
case ASSIGN:
case AT:
case AUTH:
case BINDINGS:
case BOOL:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASCADE:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESCENDING:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case GROUPS:
case HEADERS:
case HOME:
case ID:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INF:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NOTHING:
case NOWAIT:
case OF:
case OFFSET:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case PASSWORD:
case PASSWORDS:
case PATH:
case PATHS:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIMARIES:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case PROVIDER:
case PROVIDERS:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RESTRICT:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDARY:
case SECONDARIES:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SETTINGS:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VARCHAR:
case VECTOR:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONE:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 2);
{
setState(3627);
unescapedLabelSymbolicNameString();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UnescapedLabelSymbolicNameStringContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode IDENTIFIER() { return getToken(Cypher6Parser.IDENTIFIER, 0); }
public TerminalNode ACCESS() { return getToken(Cypher6Parser.ACCESS, 0); }
public TerminalNode ACTIVE() { return getToken(Cypher6Parser.ACTIVE, 0); }
public TerminalNode ADMIN() { return getToken(Cypher6Parser.ADMIN, 0); }
public TerminalNode ADMINISTRATOR() { return getToken(Cypher6Parser.ADMINISTRATOR, 0); }
public TerminalNode ALIAS() { return getToken(Cypher6Parser.ALIAS, 0); }
public TerminalNode ALIASES() { return getToken(Cypher6Parser.ALIASES, 0); }
public TerminalNode ALL_SHORTEST_PATHS() { return getToken(Cypher6Parser.ALL_SHORTEST_PATHS, 0); }
public TerminalNode ALL() { return getToken(Cypher6Parser.ALL, 0); }
public TerminalNode ALTER() { return getToken(Cypher6Parser.ALTER, 0); }
public TerminalNode AND() { return getToken(Cypher6Parser.AND, 0); }
public TerminalNode ANY() { return getToken(Cypher6Parser.ANY, 0); }
public TerminalNode ARRAY() { return getToken(Cypher6Parser.ARRAY, 0); }
public TerminalNode AS() { return getToken(Cypher6Parser.AS, 0); }
public TerminalNode ASC() { return getToken(Cypher6Parser.ASC, 0); }
public TerminalNode ASCENDING() { return getToken(Cypher6Parser.ASCENDING, 0); }
public TerminalNode ASSIGN() { return getToken(Cypher6Parser.ASSIGN, 0); }
public TerminalNode AT() { return getToken(Cypher6Parser.AT, 0); }
public TerminalNode AUTH() { return getToken(Cypher6Parser.AUTH, 0); }
public TerminalNode BINDINGS() { return getToken(Cypher6Parser.BINDINGS, 0); }
public TerminalNode BOOL() { return getToken(Cypher6Parser.BOOL, 0); }
public TerminalNode BOOLEAN() { return getToken(Cypher6Parser.BOOLEAN, 0); }
public TerminalNode BOOSTED() { return getToken(Cypher6Parser.BOOSTED, 0); }
public TerminalNode BOTH() { return getToken(Cypher6Parser.BOTH, 0); }
public TerminalNode BREAK() { return getToken(Cypher6Parser.BREAK, 0); }
public TerminalNode BTREE() { return getToken(Cypher6Parser.BTREE, 0); }
public TerminalNode BUILT() { return getToken(Cypher6Parser.BUILT, 0); }
public TerminalNode BY() { return getToken(Cypher6Parser.BY, 0); }
public TerminalNode CALL() { return getToken(Cypher6Parser.CALL, 0); }
public TerminalNode CASCADE() { return getToken(Cypher6Parser.CASCADE, 0); }
public TerminalNode CASE() { return getToken(Cypher6Parser.CASE, 0); }
public TerminalNode CHANGE() { return getToken(Cypher6Parser.CHANGE, 0); }
public TerminalNode CIDR() { return getToken(Cypher6Parser.CIDR, 0); }
public TerminalNode COLLECT() { return getToken(Cypher6Parser.COLLECT, 0); }
public TerminalNode COMMAND() { return getToken(Cypher6Parser.COMMAND, 0); }
public TerminalNode COMMANDS() { return getToken(Cypher6Parser.COMMANDS, 0); }
public TerminalNode COMPOSITE() { return getToken(Cypher6Parser.COMPOSITE, 0); }
public TerminalNode CONCURRENT() { return getToken(Cypher6Parser.CONCURRENT, 0); }
public TerminalNode CONSTRAINT() { return getToken(Cypher6Parser.CONSTRAINT, 0); }
public TerminalNode CONSTRAINTS() { return getToken(Cypher6Parser.CONSTRAINTS, 0); }
public TerminalNode CONTAINS() { return getToken(Cypher6Parser.CONTAINS, 0); }
public TerminalNode CONTINUE() { return getToken(Cypher6Parser.CONTINUE, 0); }
public TerminalNode COPY() { return getToken(Cypher6Parser.COPY, 0); }
public TerminalNode COUNT() { return getToken(Cypher6Parser.COUNT, 0); }
public TerminalNode CREATE() { return getToken(Cypher6Parser.CREATE, 0); }
public TerminalNode CSV() { return getToken(Cypher6Parser.CSV, 0); }
public TerminalNode CURRENT() { return getToken(Cypher6Parser.CURRENT, 0); }
public TerminalNode DATA() { return getToken(Cypher6Parser.DATA, 0); }
public TerminalNode DATABASE() { return getToken(Cypher6Parser.DATABASE, 0); }
public TerminalNode DATABASES() { return getToken(Cypher6Parser.DATABASES, 0); }
public TerminalNode DATE() { return getToken(Cypher6Parser.DATE, 0); }
public TerminalNode DATETIME() { return getToken(Cypher6Parser.DATETIME, 0); }
public TerminalNode DBMS() { return getToken(Cypher6Parser.DBMS, 0); }
public TerminalNode DEALLOCATE() { return getToken(Cypher6Parser.DEALLOCATE, 0); }
public TerminalNode DEFAULT() { return getToken(Cypher6Parser.DEFAULT, 0); }
public TerminalNode DEFINED() { return getToken(Cypher6Parser.DEFINED, 0); }
public TerminalNode DELETE() { return getToken(Cypher6Parser.DELETE, 0); }
public TerminalNode DENY() { return getToken(Cypher6Parser.DENY, 0); }
public TerminalNode DESC() { return getToken(Cypher6Parser.DESC, 0); }
public TerminalNode DESCENDING() { return getToken(Cypher6Parser.DESCENDING, 0); }
public TerminalNode DESTROY() { return getToken(Cypher6Parser.DESTROY, 0); }
public TerminalNode DETACH() { return getToken(Cypher6Parser.DETACH, 0); }
public TerminalNode DIFFERENT() { return getToken(Cypher6Parser.DIFFERENT, 0); }
public TerminalNode DISTINCT() { return getToken(Cypher6Parser.DISTINCT, 0); }
public TerminalNode DRIVER() { return getToken(Cypher6Parser.DRIVER, 0); }
public TerminalNode DROP() { return getToken(Cypher6Parser.DROP, 0); }
public TerminalNode DRYRUN() { return getToken(Cypher6Parser.DRYRUN, 0); }
public TerminalNode DUMP() { return getToken(Cypher6Parser.DUMP, 0); }
public TerminalNode DURATION() { return getToken(Cypher6Parser.DURATION, 0); }
public TerminalNode EACH() { return getToken(Cypher6Parser.EACH, 0); }
public TerminalNode EDGE() { return getToken(Cypher6Parser.EDGE, 0); }
public TerminalNode ELEMENT() { return getToken(Cypher6Parser.ELEMENT, 0); }
public TerminalNode ELEMENTS() { return getToken(Cypher6Parser.ELEMENTS, 0); }
public TerminalNode ELSE() { return getToken(Cypher6Parser.ELSE, 0); }
public TerminalNode ENABLE() { return getToken(Cypher6Parser.ENABLE, 0); }
public TerminalNode ENCRYPTED() { return getToken(Cypher6Parser.ENCRYPTED, 0); }
public TerminalNode END() { return getToken(Cypher6Parser.END, 0); }
public TerminalNode ENDS() { return getToken(Cypher6Parser.ENDS, 0); }
public TerminalNode ERROR() { return getToken(Cypher6Parser.ERROR, 0); }
public TerminalNode EXECUTABLE() { return getToken(Cypher6Parser.EXECUTABLE, 0); }
public TerminalNode EXECUTE() { return getToken(Cypher6Parser.EXECUTE, 0); }
public TerminalNode EXIST() { return getToken(Cypher6Parser.EXIST, 0); }
public TerminalNode EXISTENCE() { return getToken(Cypher6Parser.EXISTENCE, 0); }
public TerminalNode EXISTS() { return getToken(Cypher6Parser.EXISTS, 0); }
public TerminalNode FAIL() { return getToken(Cypher6Parser.FAIL, 0); }
public TerminalNode FALSE() { return getToken(Cypher6Parser.FALSE, 0); }
public TerminalNode FIELDTERMINATOR() { return getToken(Cypher6Parser.FIELDTERMINATOR, 0); }
public TerminalNode FINISH() { return getToken(Cypher6Parser.FINISH, 0); }
public TerminalNode FLOAT() { return getToken(Cypher6Parser.FLOAT, 0); }
public TerminalNode FOREACH() { return getToken(Cypher6Parser.FOREACH, 0); }
public TerminalNode FOR() { return getToken(Cypher6Parser.FOR, 0); }
public TerminalNode FROM() { return getToken(Cypher6Parser.FROM, 0); }
public TerminalNode FULLTEXT() { return getToken(Cypher6Parser.FULLTEXT, 0); }
public TerminalNode FUNCTION() { return getToken(Cypher6Parser.FUNCTION, 0); }
public TerminalNode FUNCTIONS() { return getToken(Cypher6Parser.FUNCTIONS, 0); }
public TerminalNode GRANT() { return getToken(Cypher6Parser.GRANT, 0); }
public TerminalNode GRAPH() { return getToken(Cypher6Parser.GRAPH, 0); }
public TerminalNode GRAPHS() { return getToken(Cypher6Parser.GRAPHS, 0); }
public TerminalNode GROUP() { return getToken(Cypher6Parser.GROUP, 0); }
public TerminalNode GROUPS() { return getToken(Cypher6Parser.GROUPS, 0); }
public TerminalNode HEADERS() { return getToken(Cypher6Parser.HEADERS, 0); }
public TerminalNode HOME() { return getToken(Cypher6Parser.HOME, 0); }
public TerminalNode ID() { return getToken(Cypher6Parser.ID, 0); }
public TerminalNode IF() { return getToken(Cypher6Parser.IF, 0); }
public TerminalNode IMMUTABLE() { return getToken(Cypher6Parser.IMMUTABLE, 0); }
public TerminalNode IMPERSONATE() { return getToken(Cypher6Parser.IMPERSONATE, 0); }
public TerminalNode IN() { return getToken(Cypher6Parser.IN, 0); }
public TerminalNode INDEX() { return getToken(Cypher6Parser.INDEX, 0); }
public TerminalNode INDEXES() { return getToken(Cypher6Parser.INDEXES, 0); }
public TerminalNode INF() { return getToken(Cypher6Parser.INF, 0); }
public TerminalNode INFINITY() { return getToken(Cypher6Parser.INFINITY, 0); }
public TerminalNode INSERT() { return getToken(Cypher6Parser.INSERT, 0); }
public TerminalNode INT() { return getToken(Cypher6Parser.INT, 0); }
public TerminalNode INTEGER() { return getToken(Cypher6Parser.INTEGER, 0); }
public TerminalNode IS() { return getToken(Cypher6Parser.IS, 0); }
public TerminalNode JOIN() { return getToken(Cypher6Parser.JOIN, 0); }
public TerminalNode KEY() { return getToken(Cypher6Parser.KEY, 0); }
public TerminalNode LABEL() { return getToken(Cypher6Parser.LABEL, 0); }
public TerminalNode LABELS() { return getToken(Cypher6Parser.LABELS, 0); }
public TerminalNode LEADING() { return getToken(Cypher6Parser.LEADING, 0); }
public TerminalNode LIMITROWS() { return getToken(Cypher6Parser.LIMITROWS, 0); }
public TerminalNode LIST() { return getToken(Cypher6Parser.LIST, 0); }
public TerminalNode LOAD() { return getToken(Cypher6Parser.LOAD, 0); }
public TerminalNode LOCAL() { return getToken(Cypher6Parser.LOCAL, 0); }
public TerminalNode LOOKUP() { return getToken(Cypher6Parser.LOOKUP, 0); }
public TerminalNode MATCH() { return getToken(Cypher6Parser.MATCH, 0); }
public TerminalNode MANAGEMENT() { return getToken(Cypher6Parser.MANAGEMENT, 0); }
public TerminalNode MAP() { return getToken(Cypher6Parser.MAP, 0); }
public TerminalNode MERGE() { return getToken(Cypher6Parser.MERGE, 0); }
public TerminalNode NAME() { return getToken(Cypher6Parser.NAME, 0); }
public TerminalNode NAMES() { return getToken(Cypher6Parser.NAMES, 0); }
public TerminalNode NAN() { return getToken(Cypher6Parser.NAN, 0); }
public TerminalNode NEW() { return getToken(Cypher6Parser.NEW, 0); }
public TerminalNode NODE() { return getToken(Cypher6Parser.NODE, 0); }
public TerminalNode NODETACH() { return getToken(Cypher6Parser.NODETACH, 0); }
public TerminalNode NODES() { return getToken(Cypher6Parser.NODES, 0); }
public TerminalNode NONE() { return getToken(Cypher6Parser.NONE, 0); }
public TerminalNode NORMALIZE() { return getToken(Cypher6Parser.NORMALIZE, 0); }
public TerminalNode NOTHING() { return getToken(Cypher6Parser.NOTHING, 0); }
public TerminalNode NOWAIT() { return getToken(Cypher6Parser.NOWAIT, 0); }
public TerminalNode OF() { return getToken(Cypher6Parser.OF, 0); }
public TerminalNode OFFSET() { return getToken(Cypher6Parser.OFFSET, 0); }
public TerminalNode ON() { return getToken(Cypher6Parser.ON, 0); }
public TerminalNode ONLY() { return getToken(Cypher6Parser.ONLY, 0); }
public TerminalNode OPTIONAL() { return getToken(Cypher6Parser.OPTIONAL, 0); }
public TerminalNode OPTIONS() { return getToken(Cypher6Parser.OPTIONS, 0); }
public TerminalNode OPTION() { return getToken(Cypher6Parser.OPTION, 0); }
public TerminalNode OR() { return getToken(Cypher6Parser.OR, 0); }
public TerminalNode ORDER() { return getToken(Cypher6Parser.ORDER, 0); }
public TerminalNode PASSWORD() { return getToken(Cypher6Parser.PASSWORD, 0); }
public TerminalNode PASSWORDS() { return getToken(Cypher6Parser.PASSWORDS, 0); }
public TerminalNode PATH() { return getToken(Cypher6Parser.PATH, 0); }
public TerminalNode PATHS() { return getToken(Cypher6Parser.PATHS, 0); }
public TerminalNode PLAINTEXT() { return getToken(Cypher6Parser.PLAINTEXT, 0); }
public TerminalNode POINT() { return getToken(Cypher6Parser.POINT, 0); }
public TerminalNode POPULATED() { return getToken(Cypher6Parser.POPULATED, 0); }
public TerminalNode PRIMARY() { return getToken(Cypher6Parser.PRIMARY, 0); }
public TerminalNode PRIMARIES() { return getToken(Cypher6Parser.PRIMARIES, 0); }
public TerminalNode PRIVILEGE() { return getToken(Cypher6Parser.PRIVILEGE, 0); }
public TerminalNode PRIVILEGES() { return getToken(Cypher6Parser.PRIVILEGES, 0); }
public TerminalNode PROCEDURE() { return getToken(Cypher6Parser.PROCEDURE, 0); }
public TerminalNode PROCEDURES() { return getToken(Cypher6Parser.PROCEDURES, 0); }
public TerminalNode PROPERTIES() { return getToken(Cypher6Parser.PROPERTIES, 0); }
public TerminalNode PROPERTY() { return getToken(Cypher6Parser.PROPERTY, 0); }
public TerminalNode PROVIDER() { return getToken(Cypher6Parser.PROVIDER, 0); }
public TerminalNode PROVIDERS() { return getToken(Cypher6Parser.PROVIDERS, 0); }
public TerminalNode RANGE() { return getToken(Cypher6Parser.RANGE, 0); }
public TerminalNode READ() { return getToken(Cypher6Parser.READ, 0); }
public TerminalNode REALLOCATE() { return getToken(Cypher6Parser.REALLOCATE, 0); }
public TerminalNode REDUCE() { return getToken(Cypher6Parser.REDUCE, 0); }
public TerminalNode REL() { return getToken(Cypher6Parser.REL, 0); }
public TerminalNode RELATIONSHIP() { return getToken(Cypher6Parser.RELATIONSHIP, 0); }
public TerminalNode RELATIONSHIPS() { return getToken(Cypher6Parser.RELATIONSHIPS, 0); }
public TerminalNode REMOVE() { return getToken(Cypher6Parser.REMOVE, 0); }
public TerminalNode RENAME() { return getToken(Cypher6Parser.RENAME, 0); }
public TerminalNode REPEATABLE() { return getToken(Cypher6Parser.REPEATABLE, 0); }
public TerminalNode REPLACE() { return getToken(Cypher6Parser.REPLACE, 0); }
public TerminalNode REPORT() { return getToken(Cypher6Parser.REPORT, 0); }
public TerminalNode REQUIRE() { return getToken(Cypher6Parser.REQUIRE, 0); }
public TerminalNode REQUIRED() { return getToken(Cypher6Parser.REQUIRED, 0); }
public TerminalNode RESTRICT() { return getToken(Cypher6Parser.RESTRICT, 0); }
public TerminalNode RETURN() { return getToken(Cypher6Parser.RETURN, 0); }
public TerminalNode REVOKE() { return getToken(Cypher6Parser.REVOKE, 0); }
public TerminalNode ROLE() { return getToken(Cypher6Parser.ROLE, 0); }
public TerminalNode ROLES() { return getToken(Cypher6Parser.ROLES, 0); }
public TerminalNode ROW() { return getToken(Cypher6Parser.ROW, 0); }
public TerminalNode ROWS() { return getToken(Cypher6Parser.ROWS, 0); }
public TerminalNode SCAN() { return getToken(Cypher6Parser.SCAN, 0); }
public TerminalNode SECONDARY() { return getToken(Cypher6Parser.SECONDARY, 0); }
public TerminalNode SECONDARIES() { return getToken(Cypher6Parser.SECONDARIES, 0); }
public TerminalNode SEC() { return getToken(Cypher6Parser.SEC, 0); }
public TerminalNode SECOND() { return getToken(Cypher6Parser.SECOND, 0); }
public TerminalNode SECONDS() { return getToken(Cypher6Parser.SECONDS, 0); }
public TerminalNode SEEK() { return getToken(Cypher6Parser.SEEK, 0); }
public TerminalNode SERVER() { return getToken(Cypher6Parser.SERVER, 0); }
public TerminalNode SERVERS() { return getToken(Cypher6Parser.SERVERS, 0); }
public TerminalNode SET() { return getToken(Cypher6Parser.SET, 0); }
public TerminalNode SETTING() { return getToken(Cypher6Parser.SETTING, 0); }
public TerminalNode SETTINGS() { return getToken(Cypher6Parser.SETTINGS, 0); }
public TerminalNode SHORTEST() { return getToken(Cypher6Parser.SHORTEST, 0); }
public TerminalNode SHORTEST_PATH() { return getToken(Cypher6Parser.SHORTEST_PATH, 0); }
public TerminalNode SHOW() { return getToken(Cypher6Parser.SHOW, 0); }
public TerminalNode SIGNED() { return getToken(Cypher6Parser.SIGNED, 0); }
public TerminalNode SINGLE() { return getToken(Cypher6Parser.SINGLE, 0); }
public TerminalNode SKIPROWS() { return getToken(Cypher6Parser.SKIPROWS, 0); }
public TerminalNode START() { return getToken(Cypher6Parser.START, 0); }
public TerminalNode STARTS() { return getToken(Cypher6Parser.STARTS, 0); }
public TerminalNode STATUS() { return getToken(Cypher6Parser.STATUS, 0); }
public TerminalNode STOP() { return getToken(Cypher6Parser.STOP, 0); }
public TerminalNode VARCHAR() { return getToken(Cypher6Parser.VARCHAR, 0); }
public TerminalNode STRING() { return getToken(Cypher6Parser.STRING, 0); }
public TerminalNode SUPPORTED() { return getToken(Cypher6Parser.SUPPORTED, 0); }
public TerminalNode SUSPENDED() { return getToken(Cypher6Parser.SUSPENDED, 0); }
public TerminalNode TARGET() { return getToken(Cypher6Parser.TARGET, 0); }
public TerminalNode TERMINATE() { return getToken(Cypher6Parser.TERMINATE, 0); }
public TerminalNode TEXT() { return getToken(Cypher6Parser.TEXT, 0); }
public TerminalNode THEN() { return getToken(Cypher6Parser.THEN, 0); }
public TerminalNode TIME() { return getToken(Cypher6Parser.TIME, 0); }
public TerminalNode TIMESTAMP() { return getToken(Cypher6Parser.TIMESTAMP, 0); }
public TerminalNode TIMEZONE() { return getToken(Cypher6Parser.TIMEZONE, 0); }
public TerminalNode TO() { return getToken(Cypher6Parser.TO, 0); }
public TerminalNode TOPOLOGY() { return getToken(Cypher6Parser.TOPOLOGY, 0); }
public TerminalNode TRAILING() { return getToken(Cypher6Parser.TRAILING, 0); }
public TerminalNode TRANSACTION() { return getToken(Cypher6Parser.TRANSACTION, 0); }
public TerminalNode TRANSACTIONS() { return getToken(Cypher6Parser.TRANSACTIONS, 0); }
public TerminalNode TRAVERSE() { return getToken(Cypher6Parser.TRAVERSE, 0); }
public TerminalNode TRIM() { return getToken(Cypher6Parser.TRIM, 0); }
public TerminalNode TRUE() { return getToken(Cypher6Parser.TRUE, 0); }
public TerminalNode TYPE() { return getToken(Cypher6Parser.TYPE, 0); }
public TerminalNode TYPES() { return getToken(Cypher6Parser.TYPES, 0); }
public TerminalNode UNION() { return getToken(Cypher6Parser.UNION, 0); }
public TerminalNode UNIQUE() { return getToken(Cypher6Parser.UNIQUE, 0); }
public TerminalNode UNIQUENESS() { return getToken(Cypher6Parser.UNIQUENESS, 0); }
public TerminalNode UNWIND() { return getToken(Cypher6Parser.UNWIND, 0); }
public TerminalNode URL() { return getToken(Cypher6Parser.URL, 0); }
public TerminalNode USE() { return getToken(Cypher6Parser.USE, 0); }
public TerminalNode USER() { return getToken(Cypher6Parser.USER, 0); }
public TerminalNode USERS() { return getToken(Cypher6Parser.USERS, 0); }
public TerminalNode USING() { return getToken(Cypher6Parser.USING, 0); }
public TerminalNode VALUE() { return getToken(Cypher6Parser.VALUE, 0); }
public TerminalNode VECTOR() { return getToken(Cypher6Parser.VECTOR, 0); }
public TerminalNode VERTEX() { return getToken(Cypher6Parser.VERTEX, 0); }
public TerminalNode WAIT() { return getToken(Cypher6Parser.WAIT, 0); }
public TerminalNode WHEN() { return getToken(Cypher6Parser.WHEN, 0); }
public TerminalNode WHERE() { return getToken(Cypher6Parser.WHERE, 0); }
public TerminalNode WITH() { return getToken(Cypher6Parser.WITH, 0); }
public TerminalNode WITHOUT() { return getToken(Cypher6Parser.WITHOUT, 0); }
public TerminalNode WRITE() { return getToken(Cypher6Parser.WRITE, 0); }
public TerminalNode XOR() { return getToken(Cypher6Parser.XOR, 0); }
public TerminalNode YIELD() { return getToken(Cypher6Parser.YIELD, 0); }
public TerminalNode ZONE() { return getToken(Cypher6Parser.ZONE, 0); }
public TerminalNode ZONED() { return getToken(Cypher6Parser.ZONED, 0); }
public UnescapedLabelSymbolicNameStringContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_unescapedLabelSymbolicNameString; }
}
public final UnescapedLabelSymbolicNameStringContext unescapedLabelSymbolicNameString() throws RecognitionException {
UnescapedLabelSymbolicNameStringContext _localctx = new UnescapedLabelSymbolicNameStringContext(_ctx, getState());
enterRule(_localctx, 648, RULE_unescapedLabelSymbolicNameString);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3630);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & -246291141494784L) != 0) || ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -146366996479975425L) != 0) || ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -2676090019766273L) != 0) || ((((_la - 192)) & ~0x3f) == 0 && ((1L << (_la - 192)) & -1130297988612173L) != 0) || ((((_la - 256)) & ~0x3f) == 0 && ((1L << (_la - 256)) & 281474972515327L) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class EndOfFileContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode EOF() { return getToken(Cypher6Parser.EOF, 0); }
public EndOfFileContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_endOfFile; }
}
public final EndOfFileContext endOfFile() throws RecognitionException {
EndOfFileContext _localctx = new EndOfFileContext(_ctx, getState());
enterRule(_localctx, 650, RULE_endOfFile);
try {
enterOuterAlt(_localctx, 1);
{
setState(3632);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
private static final String _serializedATNSegment0 =
"\u0004\u0001\u0134\u0e33\u0002\u0000\u0007\u0000\u0002\u0001\u0007\u0001"+
"\u0002\u0002\u0007\u0002\u0002\u0003\u0007\u0003\u0002\u0004\u0007\u0004"+
"\u0002\u0005\u0007\u0005\u0002\u0006\u0007\u0006\u0002\u0007\u0007\u0007"+
"\u0002\b\u0007\b\u0002\t\u0007\t\u0002\n\u0007\n\u0002\u000b\u0007\u000b"+
"\u0002\f\u0007\f\u0002\r\u0007\r\u0002\u000e\u0007\u000e\u0002\u000f\u0007"+
"\u000f\u0002\u0010\u0007\u0010\u0002\u0011\u0007\u0011\u0002\u0012\u0007"+
"\u0012\u0002\u0013\u0007\u0013\u0002\u0014\u0007\u0014\u0002\u0015\u0007"+
"\u0015\u0002\u0016\u0007\u0016\u0002\u0017\u0007\u0017\u0002\u0018\u0007"+
"\u0018\u0002\u0019\u0007\u0019\u0002\u001a\u0007\u001a\u0002\u001b\u0007"+
"\u001b\u0002\u001c\u0007\u001c\u0002\u001d\u0007\u001d\u0002\u001e\u0007"+
"\u001e\u0002\u001f\u0007\u001f\u0002 \u0007 \u0002!\u0007!\u0002\"\u0007"+
"\"\u0002#\u0007#\u0002$\u0007$\u0002%\u0007%\u0002&\u0007&\u0002\'\u0007"+
"\'\u0002(\u0007(\u0002)\u0007)\u0002*\u0007*\u0002+\u0007+\u0002,\u0007"+
",\u0002-\u0007-\u0002.\u0007.\u0002/\u0007/\u00020\u00070\u00021\u0007"+
"1\u00022\u00072\u00023\u00073\u00024\u00074\u00025\u00075\u00026\u0007"+
"6\u00027\u00077\u00028\u00078\u00029\u00079\u0002:\u0007:\u0002;\u0007"+
";\u0002<\u0007<\u0002=\u0007=\u0002>\u0007>\u0002?\u0007?\u0002@\u0007"+
"@\u0002A\u0007A\u0002B\u0007B\u0002C\u0007C\u0002D\u0007D\u0002E\u0007"+
"E\u0002F\u0007F\u0002G\u0007G\u0002H\u0007H\u0002I\u0007I\u0002J\u0007"+
"J\u0002K\u0007K\u0002L\u0007L\u0002M\u0007M\u0002N\u0007N\u0002O\u0007"+
"O\u0002P\u0007P\u0002Q\u0007Q\u0002R\u0007R\u0002S\u0007S\u0002T\u0007"+
"T\u0002U\u0007U\u0002V\u0007V\u0002W\u0007W\u0002X\u0007X\u0002Y\u0007"+
"Y\u0002Z\u0007Z\u0002[\u0007[\u0002\\\u0007\\\u0002]\u0007]\u0002^\u0007"+
"^\u0002_\u0007_\u0002`\u0007`\u0002a\u0007a\u0002b\u0007b\u0002c\u0007"+
"c\u0002d\u0007d\u0002e\u0007e\u0002f\u0007f\u0002g\u0007g\u0002h\u0007"+
"h\u0002i\u0007i\u0002j\u0007j\u0002k\u0007k\u0002l\u0007l\u0002m\u0007"+
"m\u0002n\u0007n\u0002o\u0007o\u0002p\u0007p\u0002q\u0007q\u0002r\u0007"+
"r\u0002s\u0007s\u0002t\u0007t\u0002u\u0007u\u0002v\u0007v\u0002w\u0007"+
"w\u0002x\u0007x\u0002y\u0007y\u0002z\u0007z\u0002{\u0007{\u0002|\u0007"+
"|\u0002}\u0007}\u0002~\u0007~\u0002\u007f\u0007\u007f\u0002\u0080\u0007"+
"\u0080\u0002\u0081\u0007\u0081\u0002\u0082\u0007\u0082\u0002\u0083\u0007"+
"\u0083\u0002\u0084\u0007\u0084\u0002\u0085\u0007\u0085\u0002\u0086\u0007"+
"\u0086\u0002\u0087\u0007\u0087\u0002\u0088\u0007\u0088\u0002\u0089\u0007"+
"\u0089\u0002\u008a\u0007\u008a\u0002\u008b\u0007\u008b\u0002\u008c\u0007"+
"\u008c\u0002\u008d\u0007\u008d\u0002\u008e\u0007\u008e\u0002\u008f\u0007"+
"\u008f\u0002\u0090\u0007\u0090\u0002\u0091\u0007\u0091\u0002\u0092\u0007"+
"\u0092\u0002\u0093\u0007\u0093\u0002\u0094\u0007\u0094\u0002\u0095\u0007"+
"\u0095\u0002\u0096\u0007\u0096\u0002\u0097\u0007\u0097\u0002\u0098\u0007"+
"\u0098\u0002\u0099\u0007\u0099\u0002\u009a\u0007\u009a\u0002\u009b\u0007"+
"\u009b\u0002\u009c\u0007\u009c\u0002\u009d\u0007\u009d\u0002\u009e\u0007"+
"\u009e\u0002\u009f\u0007\u009f\u0002\u00a0\u0007\u00a0\u0002\u00a1\u0007"+
"\u00a1\u0002\u00a2\u0007\u00a2\u0002\u00a3\u0007\u00a3\u0002\u00a4\u0007"+
"\u00a4\u0002\u00a5\u0007\u00a5\u0002\u00a6\u0007\u00a6\u0002\u00a7\u0007"+
"\u00a7\u0002\u00a8\u0007\u00a8\u0002\u00a9\u0007\u00a9\u0002\u00aa\u0007"+
"\u00aa\u0002\u00ab\u0007\u00ab\u0002\u00ac\u0007\u00ac\u0002\u00ad\u0007"+
"\u00ad\u0002\u00ae\u0007\u00ae\u0002\u00af\u0007\u00af\u0002\u00b0\u0007"+
"\u00b0\u0002\u00b1\u0007\u00b1\u0002\u00b2\u0007\u00b2\u0002\u00b3\u0007"+
"\u00b3\u0002\u00b4\u0007\u00b4\u0002\u00b5\u0007\u00b5\u0002\u00b6\u0007"+
"\u00b6\u0002\u00b7\u0007\u00b7\u0002\u00b8\u0007\u00b8\u0002\u00b9\u0007"+
"\u00b9\u0002\u00ba\u0007\u00ba\u0002\u00bb\u0007\u00bb\u0002\u00bc\u0007"+
"\u00bc\u0002\u00bd\u0007\u00bd\u0002\u00be\u0007\u00be\u0002\u00bf\u0007"+
"\u00bf\u0002\u00c0\u0007\u00c0\u0002\u00c1\u0007\u00c1\u0002\u00c2\u0007"+
"\u00c2\u0002\u00c3\u0007\u00c3\u0002\u00c4\u0007\u00c4\u0002\u00c5\u0007"+
"\u00c5\u0002\u00c6\u0007\u00c6\u0002\u00c7\u0007\u00c7\u0002\u00c8\u0007"+
"\u00c8\u0002\u00c9\u0007\u00c9\u0002\u00ca\u0007\u00ca\u0002\u00cb\u0007"+
"\u00cb\u0002\u00cc\u0007\u00cc\u0002\u00cd\u0007\u00cd\u0002\u00ce\u0007"+
"\u00ce\u0002\u00cf\u0007\u00cf\u0002\u00d0\u0007\u00d0\u0002\u00d1\u0007"+
"\u00d1\u0002\u00d2\u0007\u00d2\u0002\u00d3\u0007\u00d3\u0002\u00d4\u0007"+
"\u00d4\u0002\u00d5\u0007\u00d5\u0002\u00d6\u0007\u00d6\u0002\u00d7\u0007"+
"\u00d7\u0002\u00d8\u0007\u00d8\u0002\u00d9\u0007\u00d9\u0002\u00da\u0007"+
"\u00da\u0002\u00db\u0007\u00db\u0002\u00dc\u0007\u00dc\u0002\u00dd\u0007"+
"\u00dd\u0002\u00de\u0007\u00de\u0002\u00df\u0007\u00df\u0002\u00e0\u0007"+
"\u00e0\u0002\u00e1\u0007\u00e1\u0002\u00e2\u0007\u00e2\u0002\u00e3\u0007"+
"\u00e3\u0002\u00e4\u0007\u00e4\u0002\u00e5\u0007\u00e5\u0002\u00e6\u0007"+
"\u00e6\u0002\u00e7\u0007\u00e7\u0002\u00e8\u0007\u00e8\u0002\u00e9\u0007"+
"\u00e9\u0002\u00ea\u0007\u00ea\u0002\u00eb\u0007\u00eb\u0002\u00ec\u0007"+
"\u00ec\u0002\u00ed\u0007\u00ed\u0002\u00ee\u0007\u00ee\u0002\u00ef\u0007"+
"\u00ef\u0002\u00f0\u0007\u00f0\u0002\u00f1\u0007\u00f1\u0002\u00f2\u0007"+
"\u00f2\u0002\u00f3\u0007\u00f3\u0002\u00f4\u0007\u00f4\u0002\u00f5\u0007"+
"\u00f5\u0002\u00f6\u0007\u00f6\u0002\u00f7\u0007\u00f7\u0002\u00f8\u0007"+
"\u00f8\u0002\u00f9\u0007\u00f9\u0002\u00fa\u0007\u00fa\u0002\u00fb\u0007"+
"\u00fb\u0002\u00fc\u0007\u00fc\u0002\u00fd\u0007\u00fd\u0002\u00fe\u0007"+
"\u00fe\u0002\u00ff\u0007\u00ff\u0002\u0100\u0007\u0100\u0002\u0101\u0007"+
"\u0101\u0002\u0102\u0007\u0102\u0002\u0103\u0007\u0103\u0002\u0104\u0007"+
"\u0104\u0002\u0105\u0007\u0105\u0002\u0106\u0007\u0106\u0002\u0107\u0007"+
"\u0107\u0002\u0108\u0007\u0108\u0002\u0109\u0007\u0109\u0002\u010a\u0007"+
"\u010a\u0002\u010b\u0007\u010b\u0002\u010c\u0007\u010c\u0002\u010d\u0007"+
"\u010d\u0002\u010e\u0007\u010e\u0002\u010f\u0007\u010f\u0002\u0110\u0007"+
"\u0110\u0002\u0111\u0007\u0111\u0002\u0112\u0007\u0112\u0002\u0113\u0007"+
"\u0113\u0002\u0114\u0007\u0114\u0002\u0115\u0007\u0115\u0002\u0116\u0007"+
"\u0116\u0002\u0117\u0007\u0117\u0002\u0118\u0007\u0118\u0002\u0119\u0007"+
"\u0119\u0002\u011a\u0007\u011a\u0002\u011b\u0007\u011b\u0002\u011c\u0007"+
"\u011c\u0002\u011d\u0007\u011d\u0002\u011e\u0007\u011e\u0002\u011f\u0007"+
"\u011f\u0002\u0120\u0007\u0120\u0002\u0121\u0007\u0121\u0002\u0122\u0007"+
"\u0122\u0002\u0123\u0007\u0123\u0002\u0124\u0007\u0124\u0002\u0125\u0007"+
"\u0125\u0002\u0126\u0007\u0126\u0002\u0127\u0007\u0127\u0002\u0128\u0007"+
"\u0128\u0002\u0129\u0007\u0129\u0002\u012a\u0007\u012a\u0002\u012b\u0007"+
"\u012b\u0002\u012c\u0007\u012c\u0002\u012d\u0007\u012d\u0002\u012e\u0007"+
"\u012e\u0002\u012f\u0007\u012f\u0002\u0130\u0007\u0130\u0002\u0131\u0007"+
"\u0131\u0002\u0132\u0007\u0132\u0002\u0133\u0007\u0133\u0002\u0134\u0007"+
"\u0134\u0002\u0135\u0007\u0135\u0002\u0136\u0007\u0136\u0002\u0137\u0007"+
"\u0137\u0002\u0138\u0007\u0138\u0002\u0139\u0007\u0139\u0002\u013a\u0007"+
"\u013a\u0002\u013b\u0007\u013b\u0002\u013c\u0007\u013c\u0002\u013d\u0007"+
"\u013d\u0002\u013e\u0007\u013e\u0002\u013f\u0007\u013f\u0002\u0140\u0007"+
"\u0140\u0002\u0141\u0007\u0141\u0002\u0142\u0007\u0142\u0002\u0143\u0007"+
"\u0143\u0002\u0144\u0007\u0144\u0002\u0145\u0007\u0145\u0001\u0000\u0001"+
"\u0000\u0001\u0000\u0005\u0000\u0290\b\u0000\n\u0000\f\u0000\u0293\t\u0000"+
"\u0001\u0000\u0003\u0000\u0296\b\u0000\u0001\u0000\u0001\u0000\u0001\u0001"+
"\u0001\u0001\u0003\u0001\u029c\b\u0001\u0001\u0002\u0001\u0002\u0001\u0002"+
"\u0003\u0002\u02a1\b\u0002\u0001\u0002\u0005\u0002\u02a4\b\u0002\n\u0002"+
"\f\u0002\u02a7\t\u0002\u0001\u0003\u0004\u0003\u02aa\b\u0003\u000b\u0003"+
"\f\u0003\u02ab\u0001\u0004\u0001\u0004\u0001\u0004\u0001\u0004\u0001\u0004"+
"\u0001\u0004\u0001\u0004\u0001\u0004\u0001\u0004\u0001\u0004\u0001\u0004"+
"\u0001\u0004\u0001\u0004\u0001\u0004\u0001\u0004\u0001\u0004\u0001\u0004"+
"\u0003\u0004\u02bf\b\u0004\u0001\u0005\u0001\u0005\u0003\u0005\u02c3\b"+
"\u0005\u0001\u0005\u0001\u0005\u0001\u0006\u0001\u0006\u0001\u0006\u0001"+
"\u0006\u0001\u0006\u0001\u0006\u0003\u0006\u02cd\b\u0006\u0001\u0007\u0001"+
"\u0007\u0001\b\u0001\b\u0001\b\u0001\t\u0003\t\u02d5\b\t\u0001\t\u0001"+
"\t\u0003\t\u02d9\b\t\u0001\t\u0003\t\u02dc\b\t\u0001\t\u0003\t\u02df\b"+
"\t\u0001\n\u0001\n\u0001\n\u0003\n\u02e4\b\n\u0001\u000b\u0001\u000b\u0003"+
"\u000b\u02e8\b\u000b\u0001\u000b\u0001\u000b\u0005\u000b\u02ec\b\u000b"+
"\n\u000b\f\u000b\u02ef\t\u000b\u0001\f\u0001\f\u0001\f\u0003\f\u02f4\b"+
"\f\u0001\r\u0001\r\u0001\u000e\u0001\u000e\u0001\u000f\u0001\u000f\u0001"+
"\u000f\u0001\u000f\u0001\u000f\u0005\u000f\u02ff\b\u000f\n\u000f\f\u000f"+
"\u0302\t\u000f\u0001\u0010\u0001\u0010\u0001\u0010\u0001\u0011\u0001\u0011"+
"\u0001\u0011\u0001\u0012\u0001\u0012\u0001\u0012\u0001\u0013\u0001\u0013"+
"\u0001\u0013\u0003\u0013\u0310\b\u0013\u0001\u0014\u0001\u0014\u0001\u0014"+
"\u0001\u0015\u0001\u0015\u0001\u0015\u0001\u0016\u0001\u0016\u0001\u0016"+
"\u0001\u0016\u0005\u0016\u031c\b\u0016\n\u0016\f\u0016\u031f\t\u0016\u0001"+
"\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001"+
"\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001"+
"\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001"+
"\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0003\u0017\u0337\b\u0017\u0001"+
"\u0018\u0001\u0018\u0001\u0018\u0001\u0018\u0005\u0018\u033d\b\u0018\n"+
"\u0018\f\u0018\u0340\t\u0018\u0001\u0019\u0001\u0019\u0001\u0019\u0001"+
"\u0019\u0001\u0019\u0001\u0019\u0001\u0019\u0001\u0019\u0003\u0019\u034a"+
"\b\u0019\u0001\u001a\u0003\u001a\u034d\b\u001a\u0001\u001a\u0001\u001a"+
"\u0001\u001a\u0001\u001a\u0005\u001a\u0353\b\u001a\n\u001a\f\u001a\u0356"+
"\t\u001a\u0001\u001b\u0003\u001b\u0359\b\u001b\u0001\u001b\u0001\u001b"+
"\u0003\u001b\u035d\b\u001b\u0001\u001b\u0001\u001b\u0005\u001b\u0361\b"+
"\u001b\n\u001b\f\u001b\u0364\t\u001b\u0001\u001b\u0003\u001b\u0367\b\u001b"+
"\u0001\u001c\u0001\u001c\u0001\u001c\u0003\u001c\u036c\b\u001c\u0001\u001c"+
"\u0003\u001c\u036f\b\u001c\u0001\u001c\u0001\u001c\u0001\u001c\u0003\u001c"+
"\u0374\b\u001c\u0001\u001c\u0003\u001c\u0377\b\u001c\u0003\u001c\u0379"+
"\b\u001c\u0001\u001d\u0001\u001d\u0001\u001d\u0001\u001d\u0001\u001d\u0001"+
"\u001d\u0001\u001d\u0001\u001d\u0001\u001d\u0001\u001d\u0003\u001d\u0385"+
"\b\u001d\u0001\u001d\u0003\u001d\u0388\b\u001d\u0001\u001d\u0001\u001d"+
"\u0001\u001d\u0001\u001d\u0001\u001d\u0001\u001d\u0001\u001d\u0001\u001d"+
"\u0001\u001d\u0001\u001d\u0001\u001d\u0001\u001d\u0001\u001d\u0003\u001d"+
"\u0397\b\u001d\u0001\u001e\u0001\u001e\u0001\u001e\u0005\u001e\u039c\b"+
"\u001e\n\u001e\f\u001e\u039f\t\u001e\u0001\u001f\u0001\u001f\u0001\u001f"+
"\u0001\u001f\u0001 \u0001 \u0001 \u0001 \u0001 \u0001!\u0003!\u03ab\b"+
"!\u0001!\u0001!\u0001!\u0001!\u0001!\u0001!\u0005!\u03b3\b!\n!\f!\u03b6"+
"\t!\u0003!\u03b8\b!\u0001!\u0003!\u03bb\b!\u0001!\u0001!\u0001!\u0001"+
"!\u0001!\u0005!\u03c2\b!\n!\f!\u03c5\t!\u0001!\u0003!\u03c8\b!\u0003!"+
"\u03ca\b!\u0003!\u03cc\b!\u0001\"\u0001\"\u0001\"\u0001#\u0001#\u0001"+
"$\u0001$\u0001$\u0003$\u03d6\b$\u0001%\u0001%\u0001%\u0001%\u0003%\u03dc"+
"\b%\u0001%\u0001%\u0001%\u0001%\u0001%\u0001%\u0003%\u03e4\b%\u0001&\u0001"+
"&\u0001&\u0001&\u0001&\u0001&\u0001&\u0004&\u03ed\b&\u000b&\f&\u03ee\u0001"+
"&\u0001&\u0001\'\u0003\'\u03f4\b\'\u0001\'\u0001\'\u0003\'\u03f8\b\'\u0001"+
"\'\u0001\'\u0001\'\u0001\'\u0003\'\u03fe\b\'\u0001(\u0001(\u0001(\u0001"+
"(\u0001(\u0005(\u0405\b(\n(\f(\u0408\t(\u0003(\u040a\b(\u0001(\u0001("+
"\u0001)\u0001)\u0003)\u0410\b)\u0001)\u0003)\u0413\b)\u0001)\u0001)\u0001"+
")\u0001)\u0005)\u0419\b)\n)\f)\u041c\t)\u0001*\u0001*\u0001*\u0001*\u0001"+
"+\u0001+\u0001+\u0001+\u0001,\u0001,\u0001,\u0001,\u0001,\u0001-\u0001"+
"-\u0003-\u042d\b-\u0001-\u0003-\u0430\b-\u0001-\u0001-\u0003-\u0434\b"+
"-\u0001-\u0003-\u0437\b-\u0001.\u0001.\u0001.\u0005.\u043c\b.\n.\f.\u043f"+
"\t.\u0001/\u0001/\u0001/\u0005/\u0444\b/\n/\f/\u0447\t/\u00010\u00010"+
"\u00010\u00030\u044c\b0\u00010\u00030\u044f\b0\u00010\u00010\u00011\u0001"+
"1\u00011\u00031\u0456\b1\u00011\u00011\u00011\u00011\u00051\u045c\b1\n"+
"1\f1\u045f\t1\u00012\u00012\u00012\u00012\u00012\u00032\u0466\b2\u0001"+
"2\u00012\u00032\u046a\b2\u00012\u00012\u00012\u00032\u046f\b2\u00013\u0001"+
"3\u00033\u0473\b3\u00014\u00014\u00014\u00014\u00014\u00015\u00015\u0001"+
"5\u00035\u047d\b5\u00015\u00015\u00055\u0481\b5\n5\f5\u0484\t5\u00015"+
"\u00045\u0487\b5\u000b5\f5\u0488\u00016\u00016\u00016\u00036\u048e\b6"+
"\u00016\u00016\u00016\u00036\u0493\b6\u00016\u00016\u00036\u0497\b6\u0001"+
"6\u00036\u049a\b6\u00016\u00016\u00036\u049e\b6\u00016\u00016\u00036\u04a2"+
"\b6\u00016\u00036\u04a5\b6\u00016\u00016\u00016\u00016\u00036\u04ab\b"+
"6\u00036\u04ad\b6\u00017\u00017\u00018\u00018\u00019\u00019\u00019\u0001"+
"9\u00049\u04b7\b9\u000b9\f9\u04b8\u0001:\u0001:\u0003:\u04bd\b:\u0001"+
":\u0003:\u04c0\b:\u0001:\u0003:\u04c3\b:\u0001:\u0001:\u0003:\u04c7\b"+
":\u0001:\u0001:\u0001;\u0001;\u0003;\u04cd\b;\u0001;\u0003;\u04d0\b;\u0001"+
";\u0003;\u04d3\b;\u0001;\u0001;\u0001<\u0001<\u0001<\u0001<\u0003<\u04db"+
"\b<\u0001<\u0001<\u0003<\u04df\b<\u0001=\u0001=\u0004=\u04e3\b=\u000b"+
"=\f=\u04e4\u0001>\u0001>\u0001>\u0003>\u04ea\b>\u0001>\u0001>\u0005>\u04ee"+
"\b>\n>\f>\u04f1\t>\u0001?\u0001?\u0001?\u0001?\u0001?\u0001@\u0001@\u0001"+
"@\u0001A\u0001A\u0001A\u0001B\u0001B\u0001B\u0001C\u0001C\u0001C\u0001"+
"D\u0001D\u0003D\u0506\bD\u0001E\u0003E\u0509\bE\u0001E\u0001E\u0001E\u0003"+
"E\u050e\bE\u0001E\u0003E\u0511\bE\u0001E\u0003E\u0514\bE\u0001E\u0003"+
"E\u0517\bE\u0001E\u0001E\u0003E\u051b\bE\u0001E\u0003E\u051e\bE\u0001"+
"E\u0001E\u0003E\u0522\bE\u0001F\u0003F\u0525\bF\u0001F\u0001F\u0001F\u0003"+
"F\u052a\bF\u0001F\u0001F\u0003F\u052e\bF\u0001F\u0001F\u0001F\u0003F\u0533"+
"\bF\u0001G\u0001G\u0001H\u0001H\u0001I\u0001I\u0001J\u0001J\u0003J\u053d"+
"\bJ\u0001J\u0001J\u0003J\u0541\bJ\u0001J\u0003J\u0544\bJ\u0001K\u0001"+
"K\u0001K\u0001K\u0003K\u054a\bK\u0001L\u0001L\u0001L\u0003L\u054f\bL\u0001"+
"L\u0005L\u0552\bL\nL\fL\u0555\tL\u0001M\u0001M\u0001M\u0003M\u055a\bM"+
"\u0001M\u0005M\u055d\bM\nM\fM\u0560\tM\u0001N\u0001N\u0001N\u0005N\u0565"+
"\bN\nN\fN\u0568\tN\u0001O\u0001O\u0001O\u0005O\u056d\bO\nO\fO\u0570\t"+
"O\u0001P\u0005P\u0573\bP\nP\fP\u0576\tP\u0001P\u0001P\u0001Q\u0005Q\u057b"+
"\bQ\nQ\fQ\u057e\tQ\u0001Q\u0001Q\u0001R\u0001R\u0001R\u0001R\u0001R\u0001"+
"R\u0003R\u0588\bR\u0001S\u0001S\u0001S\u0001S\u0001S\u0001S\u0003S\u0590"+
"\bS\u0001T\u0001T\u0001T\u0001T\u0005T\u0596\bT\nT\fT\u0599\tT\u0001U"+
"\u0001U\u0001U\u0001V\u0001V\u0001V\u0005V\u05a1\bV\nV\fV\u05a4\tV\u0001"+
"W\u0001W\u0001W\u0005W\u05a9\bW\nW\fW\u05ac\tW\u0001X\u0001X\u0001X\u0005"+
"X\u05b1\bX\nX\fX\u05b4\tX\u0001Y\u0005Y\u05b7\bY\nY\fY\u05ba\tY\u0001"+
"Y\u0001Y\u0001Z\u0001Z\u0001Z\u0005Z\u05c1\bZ\nZ\fZ\u05c4\tZ\u0001[\u0001"+
"[\u0003[\u05c8\b[\u0001\\\u0001\\\u0001\\\u0001\\\u0001\\\u0001\\\u0001"+
"\\\u0003\\\u05d1\b\\\u0001\\\u0001\\\u0001\\\u0003\\\u05d6\b\\\u0001\\"+
"\u0001\\\u0001\\\u0003\\\u05db\b\\\u0001\\\u0001\\\u0003\\\u05df\b\\\u0001"+
"\\\u0001\\\u0001\\\u0003\\\u05e4\b\\\u0001\\\u0003\\\u05e7\b\\\u0001\\"+
"\u0003\\\u05ea\b\\\u0001]\u0001]\u0001^\u0001^\u0001^\u0005^\u05f1\b^"+
"\n^\f^\u05f4\t^\u0001_\u0001_\u0001_\u0005_\u05f9\b_\n_\f_\u05fc\t_\u0001"+
"`\u0001`\u0001`\u0005`\u0601\b`\n`\f`\u0604\t`\u0001a\u0001a\u0001a\u0003"+
"a\u0609\ba\u0001b\u0001b\u0005b\u060d\bb\nb\fb\u0610\tb\u0001c\u0001c"+
"\u0001c\u0001c\u0001c\u0001c\u0001c\u0001c\u0003c\u061a\bc\u0001c\u0001"+
"c\u0003c\u061e\bc\u0001c\u0003c\u0621\bc\u0001d\u0001d\u0001d\u0001e\u0001"+
"e\u0001e\u0001e\u0001f\u0001f\u0004f\u062c\bf\u000bf\ff\u062d\u0001g\u0001"+
"g\u0001g\u0001h\u0001h\u0001h\u0001h\u0001h\u0001h\u0001h\u0001h\u0001"+
"h\u0001h\u0001h\u0001h\u0001h\u0001h\u0001h\u0001h\u0001h\u0001h\u0001"+
"h\u0001h\u0001h\u0003h\u0648\bh\u0001i\u0001i\u0001i\u0001i\u0001i\u0001"+
"i\u0001i\u0001i\u0001i\u0003i\u0653\bi\u0001j\u0001j\u0004j\u0657\bj\u000b"+
"j\fj\u0658\u0001j\u0001j\u0003j\u065d\bj\u0001j\u0001j\u0001k\u0001k\u0001"+
"k\u0001k\u0001k\u0001l\u0001l\u0001l\u0004l\u0669\bl\u000bl\fl\u066a\u0001"+
"l\u0001l\u0003l\u066f\bl\u0001l\u0001l\u0001m\u0001m\u0001m\u0001m\u0005"+
"m\u0677\bm\nm\fm\u067a\tm\u0001m\u0001m\u0001m\u0001n\u0001n\u0001n\u0001"+
"n\u0001n\u0003n\u0684\bn\u0001n\u0001n\u0001n\u0003n\u0689\bn\u0001n\u0001"+
"n\u0001n\u0003n\u068e\bn\u0001n\u0001n\u0003n\u0692\bn\u0001n\u0001n\u0001"+
"n\u0003n\u0697\bn\u0001n\u0003n\u069a\bn\u0001n\u0001n\u0001n\u0001n\u0003"+
"n\u06a0\bn\u0001o\u0001o\u0001o\u0001o\u0001o\u0001o\u0003o\u06a8\bo\u0001"+
"o\u0001o\u0001o\u0001o\u0003o\u06ae\bo\u0003o\u06b0\bo\u0001o\u0001o\u0001"+
"p\u0001p\u0001p\u0001p\u0003p\u06b8\bp\u0001p\u0001p\u0001p\u0003p\u06bd"+
"\bp\u0001p\u0001p\u0001p\u0001p\u0001q\u0001q\u0001q\u0001q\u0001q\u0001"+
"q\u0001q\u0001q\u0001q\u0001q\u0001q\u0001q\u0001q\u0001r\u0001r\u0001"+
"r\u0001r\u0001r\u0001r\u0001r\u0003r\u06d7\br\u0001r\u0001r\u0001s\u0001"+
"s\u0001s\u0001s\u0001s\u0003s\u06e0\bs\u0001s\u0001s\u0001t\u0001t\u0001"+
"t\u0003t\u06e7\bt\u0001t\u0003t\u06ea\bt\u0001t\u0003t\u06ed\bt\u0001"+
"t\u0001t\u0001t\u0001u\u0001u\u0001v\u0001v\u0001w\u0001w\u0001w\u0001"+
"w\u0001x\u0001x\u0001x\u0001x\u0001x\u0005x\u06ff\bx\nx\fx\u0702\tx\u0003"+
"x\u0704\bx\u0001x\u0001x\u0001y\u0001y\u0001y\u0001y\u0001y\u0001y\u0001"+
"y\u0001y\u0003y\u0710\by\u0001z\u0001z\u0001z\u0001z\u0001z\u0001{\u0001"+
"{\u0001{\u0001{\u0003{\u071b\b{\u0001{\u0001{\u0003{\u071f\b{\u0003{\u0721"+
"\b{\u0001{\u0001{\u0001|\u0001|\u0001|\u0001|\u0003|\u0729\b|\u0001|\u0001"+
"|\u0003|\u072d\b|\u0003|\u072f\b|\u0001|\u0001|\u0001}\u0001}\u0001}\u0001"+
"}\u0001}\u0001~\u0003~\u0739\b~\u0001~\u0001~\u0001\u007f\u0003\u007f"+
"\u073e\b\u007f\u0001\u007f\u0001\u007f\u0001\u0080\u0001\u0080\u0001\u0080"+
"\u0001\u0080\u0005\u0080\u0746\b\u0080\n\u0080\f\u0080\u0749\t\u0080\u0003"+
"\u0080\u074b\b\u0080\u0001\u0080\u0001\u0080\u0001\u0081\u0001\u0081\u0001"+
"\u0082\u0001\u0082\u0001\u0082\u0001\u0083\u0001\u0083\u0001\u0083\u0001"+
"\u0083\u0003\u0083\u0758\b\u0083\u0001\u0084\u0001\u0084\u0001\u0084\u0003"+
"\u0084\u075d\b\u0084\u0001\u0084\u0001\u0084\u0001\u0084\u0005\u0084\u0762"+
"\b\u0084\n\u0084\f\u0084\u0765\t\u0084\u0003\u0084\u0767\b\u0084\u0001"+
"\u0084\u0001\u0084\u0001\u0085\u0001\u0085\u0001\u0086\u0001\u0086\u0001"+
"\u0086\u0001\u0087\u0001\u0087\u0001\u0087\u0005\u0087\u0773\b\u0087\n"+
"\u0087\f\u0087\u0776\t\u0087\u0001\u0088\u0001\u0088\u0001\u0089\u0001"+
"\u0089\u0001\u0089\u0005\u0089\u077d\b\u0089\n\u0089\f\u0089\u0780\t\u0089"+
"\u0001\u008a\u0001\u008a\u0001\u008a\u0005\u008a\u0785\b\u008a\n\u008a"+
"\f\u008a\u0788\t\u008a\u0001\u008b\u0001\u008b\u0003\u008b\u078c\b\u008b"+
"\u0001\u008b\u0005\u008b\u078f\b\u008b\n\u008b\f\u008b\u0792\t\u008b\u0001"+
"\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001"+
"\u008c\u0001\u008c\u0003\u008c\u079c\b\u008c\u0001\u008c\u0001\u008c\u0001"+
"\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001"+
"\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0003\u008c\u07aa\b\u008c\u0001"+
"\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0003\u008c\u07b1"+
"\b\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001"+
"\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001"+
"\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001"+
"\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001\u008c\u0001"+
"\u008c\u0001\u008c\u0003\u008c\u07cc\b\u008c\u0001\u008c\u0001\u008c\u0001"+
"\u008c\u0001\u008c\u0001\u008c\u0003\u008c\u07d3\b\u008c\u0003\u008c\u07d5"+
"\b\u008c\u0001\u008d\u0001\u008d\u0001\u008d\u0003\u008d\u07da\b\u008d"+
"\u0001\u008e\u0001\u008e\u0003\u008e\u07de\b\u008e\u0001\u008f\u0003\u008f"+
"\u07e1\b\u008f\u0001\u008f\u0001\u008f\u0001\u008f\u0001\u008f\u0001\u008f"+
"\u0001\u008f\u0001\u008f\u0001\u008f\u0001\u008f\u0001\u008f\u0001\u008f"+
"\u0001\u008f\u0001\u008f\u0003\u008f\u07f0\b\u008f\u0001\u0090\u0001\u0090"+
"\u0001\u0090\u0003\u0090\u07f5\b\u0090\u0001\u0090\u0001\u0090\u0001\u0090"+
"\u0001\u0090\u0001\u0090\u0001\u0090\u0001\u0090\u0003\u0090\u07fe\b\u0090"+
"\u0001\u0091\u0001\u0091\u0001\u0091\u0001\u0091\u0001\u0091\u0001\u0091"+
"\u0001\u0091\u0001\u0091\u0003\u0091\u0808\b\u0091\u0001\u0092\u0001\u0092"+
"\u0001\u0092\u0001\u0092\u0001\u0092\u0001\u0092\u0001\u0092\u0001\u0092"+
"\u0001\u0092\u0001\u0092\u0001\u0092\u0001\u0092\u0001\u0092\u0001\u0092"+
"\u0001\u0092\u0001\u0092\u0001\u0092\u0003\u0092\u081b\b\u0092\u0001\u0093"+
"\u0001\u0093\u0003\u0093\u081f\b\u0093\u0001\u0093\u0003\u0093\u0822\b"+
"\u0093\u0001\u0094\u0001\u0094\u0001\u0094\u0003\u0094\u0827\b\u0094\u0001"+
"\u0095\u0001\u0095\u0001\u0095\u0001\u0096\u0001\u0096\u0001\u0096\u0001"+
"\u0097\u0001\u0097\u0001\u0097\u0001\u0097\u0001\u0097\u0005\u0097\u0834"+
"\b\u0097\n\u0097\f\u0097\u0837\t\u0097\u0003\u0097\u0839\b\u0097\u0001"+
"\u0097\u0003\u0097\u083c\b\u0097\u0001\u0097\u0003\u0097\u083f\b\u0097"+
"\u0001\u0097\u0003\u0097\u0842\b\u0097\u0001\u0097\u0003\u0097\u0845\b"+
"\u0097\u0001\u0098\u0001\u0098\u0001\u0098\u0001\u0099\u0001\u0099\u0001"+
"\u0099\u0001\u009a\u0001\u009a\u0003\u009a\u084f\b\u009a\u0001\u009b\u0001"+
"\u009b\u0001\u009b\u0001\u009b\u0001\u009b\u0001\u009b\u0001\u009b\u0003"+
"\u009b\u0858\b\u009b\u0001\u009c\u0003\u009c\u085b\b\u009c\u0001\u009c"+
"\u0001\u009c\u0001\u009d\u0001\u009d\u0001\u009e\u0001\u009e\u0003\u009e"+
"\u0863\b\u009e\u0001\u009e\u0003\u009e\u0866\b\u009e\u0001\u009f\u0003"+
"\u009f\u0869\b\u009f\u0001\u009f\u0001\u009f\u0003\u009f\u086d\b\u009f"+
"\u0001\u009f\u0001\u009f\u0001\u009f\u0001\u009f\u0003\u009f\u0873\b\u009f"+
"\u0001\u009f\u0001\u009f\u0001\u009f\u0003\u009f\u0878\b\u009f\u0001\u009f"+
"\u0001\u009f\u0001\u009f\u0001\u009f\u0003\u009f\u087e\b\u009f\u0001\u009f"+
"\u0003\u009f\u0881\b\u009f\u0001\u009f\u0001\u009f\u0003\u009f\u0885\b"+
"\u009f\u0001\u00a0\u0001\u00a0\u0003\u00a0\u0889\b\u00a0\u0001\u00a1\u0001"+
"\u00a1\u0001\u00a1\u0001\u00a1\u0001\u00a1\u0001\u00a1\u0003\u00a1\u0891"+
"\b\u00a1\u0001\u00a2\u0001\u00a2\u0003\u00a2\u0895\b\u00a2\u0001\u00a2"+
"\u0003\u00a2\u0898\b\u00a2\u0001\u00a3\u0001\u00a3\u0003\u00a3\u089c\b"+
"\u00a3\u0001\u00a3\u0003\u00a3\u089f\b\u00a3\u0001\u00a3\u0003\u00a3\u08a2"+
"\b\u00a3\u0001\u00a4\u0003\u00a4\u08a5\b\u00a4\u0001\u00a4\u0001\u00a4"+
"\u0003\u00a4\u08a9\b\u00a4\u0001\u00a4\u0003\u00a4\u08ac\b\u00a4\u0001"+
"\u00a4\u0003\u00a4\u08af\b\u00a4\u0001\u00a5\u0001\u00a5\u0001\u00a6\u0001"+
"\u00a6\u0001\u00a6\u0001\u00a6\u0001\u00a6\u0003\u00a6\u08b8\b\u00a6\u0003"+
"\u00a6\u08ba\b\u00a6\u0001\u00a7\u0001\u00a7\u0001\u00a7\u0001\u00a7\u0001"+
"\u00a7\u0003\u00a7\u08c1\b\u00a7\u0001\u00a8\u0001\u00a8\u0001\u00a8\u0001"+
"\u00a9\u0001\u00a9\u0001\u00a9\u0001\u00aa\u0001\u00aa\u0001\u00aa\u0001"+
"\u00ab\u0001\u00ab\u0001\u00ac\u0003\u00ac\u08cf\b\u00ac\u0001\u00ac\u0001"+
"\u00ac\u0003\u00ac\u08d3\b\u00ac\u0003\u00ac\u08d5\b\u00ac\u0001\u00ac"+
"\u0003\u00ac\u08d8\b\u00ac\u0001\u00ad\u0001\u00ad\u0003\u00ad\u08dc\b"+
"\u00ad\u0001\u00ae\u0001\u00ae\u0001\u00ae\u0001\u00ae\u0001\u00ae\u0001"+
"\u00af\u0001\u00af\u0001\u00af\u0003\u00af\u08e6\b\u00af\u0001\u00af\u0001"+
"\u00af\u0001\u00af\u0001\u00af\u0001\u00af\u0001\u00af\u0001\u00af\u0003"+
"\u00af\u08ef\b\u00af\u0001\u00af\u0001\u00af\u0001\u00af\u0001\u00b0\u0001"+
"\u00b0\u0003\u00b0\u08f6\b\u00b0\u0001\u00b0\u0001\u00b0\u0001\u00b0\u0003"+
"\u00b0\u08fb\b\u00b0\u0001\u00b0\u0001\u00b0\u0001\u00b0\u0003\u00b0\u0900"+
"\b\u00b0\u0001\u00b0\u0001\u00b0\u0003\u00b0\u0904\b\u00b0\u0001\u00b1"+
"\u0001\u00b1\u0001\u00b1\u0001\u00b1\u0001\u00b1\u0003\u00b1\u090b\b\u00b1"+
"\u0001\u00b1\u0001\u00b1\u0001\u00b1\u0001\u00b1\u0001\u00b1\u0001\u00b1"+
"\u0003\u00b1\u0913\b\u00b1\u0001\u00b1\u0001\u00b1\u0001\u00b1\u0001\u00b1"+
"\u0001\u00b1\u0001\u00b1\u0003\u00b1\u091b\b\u00b1\u0001\u00b1\u0001\u00b1"+
"\u0001\u00b1\u0001\u00b1\u0001\u00b1\u0001\u00b1\u0001\u00b1\u0001\u00b1"+
"\u0003\u00b1\u0925\b\u00b1\u0001\u00b2\u0001\u00b2\u0001\u00b2\u0001\u00b2"+
"\u0003\u00b2\u092b\b\u00b2\u0001\u00b3\u0001\u00b3\u0001\u00b3\u0001\u00b3"+
"\u0001\u00b3\u0001\u00b3\u0001\u00b3\u0001\u00b3\u0001\u00b3\u0001\u00b3"+
"\u0001\u00b3\u0001\u00b3\u0001\u00b3\u0001\u00b3\u0001\u00b3\u0001\u00b3"+
"\u0001\u00b3\u0001\u00b3\u0001\u00b3\u0001\u00b3\u0001\u00b3\u0001\u00b3"+
"\u0001\u00b3\u0003\u00b3\u0944\b\u00b3\u0001\u00b4\u0003\u00b4\u0947\b"+
"\u00b4\u0001\u00b4\u0001\u00b4\u0001\u00b4\u0003\u00b4\u094c\b\u00b4\u0001"+
"\u00b4\u0001\u00b4\u0001\u00b4\u0003\u00b4\u0951\b\u00b4\u0001\u00b4\u0001"+
"\u00b4\u0001\u00b4\u0003\u00b4\u0956\b\u00b4\u0001\u00b5\u0003\u00b5\u0959"+
"\b\u00b5\u0001\u00b5\u0001\u00b5\u0001\u00b5\u0003\u00b5\u095e\b\u00b5"+
"\u0001\u00b5\u0001\u00b5\u0001\u00b5\u0003\u00b5\u0963\b\u00b5\u0001\u00b5"+
"\u0001\u00b5\u0001\u00b5\u0001\u00b5\u0001\u00b5\u0001\u00b5\u0003\u00b5"+
"\u096b\b\u00b5\u0001\u00b6\u0001\u00b6\u0001\u00b6\u0001\u00b6\u0001\u00b6"+
"\u0001\u00b6\u0005\u00b6\u0973\b\u00b6\n\u00b6\f\u00b6\u0976\t\u00b6\u0001"+
"\u00b6\u0001\u00b6\u0001\u00b7\u0001\u00b7\u0001\u00b7\u0003\u00b7\u097d"+
"\b\u00b7\u0001\u00b7\u0001\u00b7\u0001\u00b7\u0001\u00b7\u0001\u00b7\u0001"+
"\u00b7\u0001\u00b7\u0005\u00b7\u0986\b\u00b7\n\u00b7\f\u00b7\u0989\t\u00b7"+
"\u0001\u00b7\u0001\u00b7\u0001\u00b7\u0003\u00b7\u098e\b\u00b7\u0001\u00b7"+
"\u0001\u00b7\u0001\u00b7\u0001\u00b8\u0003\u00b8\u0994\b\u00b8\u0001\u00b8"+
"\u0001\u00b8\u0001\u00b8\u0003\u00b8\u0999\b\u00b8\u0001\u00b8\u0001\u00b8"+
"\u0001\u00b8\u0003\u00b8\u099e\b\u00b8\u0001\u00b8\u0001\u00b8\u0001\u00b8"+
"\u0001\u00b8\u0001\u00b8\u0003\u00b8\u09a5\b\u00b8\u0001\u00b9\u0001\u00b9"+
"\u0001\u00b9\u0001\u00b9\u0001\u00b9\u0001\u00b9\u0001\u00ba\u0001\u00ba"+
"\u0001\u00ba\u0003\u00ba\u09b0\b\u00ba\u0001\u00ba\u0001\u00ba\u0001\u00ba"+
"\u0001\u00ba\u0001\u00ba\u0001\u00ba\u0003\u00ba\u09b8\b\u00ba\u0001\u00ba"+
"\u0001\u00ba\u0001\u00ba\u0001\u00ba\u0003\u00ba\u09be\b\u00ba\u0001\u00bb"+
"\u0001\u00bb\u0001\u00bb\u0001\u00bb\u0003\u00bb\u09c4\b\u00bb\u0001\u00bc"+
"\u0001\u00bc\u0001\u00bc\u0001\u00bc\u0001\u00bc\u0001\u00bc\u0001\u00bc"+
"\u0003\u00bc\u09cd\b\u00bc\u0001\u00bd\u0001\u00bd\u0001\u00bd\u0001\u00bd"+
"\u0001\u00bd\u0001\u00bd\u0005\u00bd\u09d5\b\u00bd\n\u00bd\f\u00bd\u09d8"+
"\t\u00bd\u0001\u00be\u0001\u00be\u0001\u00be\u0001\u00be\u0001\u00be\u0001"+
"\u00be\u0003\u00be\u09e0\b\u00be\u0001\u00bf\u0001\u00bf\u0001\u00bf\u0001"+
"\u00bf\u0003\u00bf\u09e6\b\u00bf\u0001\u00c0\u0001\u00c0\u0003\u00c0\u09ea"+
"\b\u00c0\u0001\u00c0\u0001\u00c0\u0001\u00c0\u0001\u00c0\u0001\u00c0\u0001"+
"\u00c0\u0001\u00c0\u0003\u00c0\u09f3\b\u00c0\u0001\u00c1\u0001\u00c1\u0003"+
"\u00c1\u09f7\b\u00c1\u0001\u00c1\u0001\u00c1\u0001\u00c1\u0001\u00c1\u0001"+
"\u00c2\u0001\u00c2\u0003\u00c2\u09ff\b\u00c2\u0001\u00c2\u0003\u00c2\u0a02"+
"\b\u00c2\u0001\u00c2\u0001\u00c2\u0001\u00c2\u0001\u00c2\u0001\u00c2\u0001"+
"\u00c2\u0001\u00c2\u0003\u00c2\u0a0b\b\u00c2\u0001\u00c3\u0001\u00c3\u0001"+
"\u00c4\u0001\u00c4\u0001\u00c5\u0001\u00c5\u0001\u00c6\u0001\u00c6\u0001"+
"\u00c6\u0001\u00c6\u0003\u00c6\u0a17\b\u00c6\u0001\u00c7\u0001\u00c7\u0001"+
"\u00c7\u0001\u00c7\u0001\u00c7\u0001\u00c8\u0001\u00c8\u0001\u00c8\u0001"+
"\u00c8\u0001\u00c8\u0001\u00c9\u0001\u00c9\u0001\u00c9\u0001\u00ca\u0001"+
"\u00ca\u0003\u00ca\u0a28\b\u00ca\u0001\u00cb\u0003\u00cb\u0a2b\b\u00cb"+
"\u0001\u00cb\u0001\u00cb\u0003\u00cb\u0a2f\b\u00cb\u0001\u00cc\u0001\u00cc"+
"\u0001\u00cc\u0001\u00cc\u0001\u00cc\u0001\u00cc\u0001\u00cc\u0005\u00cc"+
"\u0a38\b\u00cc\n\u00cc\f\u00cc\u0a3b\t\u00cc\u0001\u00cd\u0001\u00cd\u0001"+
"\u00cd\u0001\u00ce\u0001\u00ce\u0001\u00ce\u0001\u00ce\u0001\u00ce\u0003"+
"\u00ce\u0a45\b\u00ce\u0001\u00ce\u0001\u00ce\u0001\u00ce\u0001\u00ce\u0003"+
"\u00ce\u0a4b\b\u00ce\u0001\u00cf\u0001\u00cf\u0001\u00cf\u0001\u00cf\u0003"+
"\u00cf\u0a51\b\u00cf\u0001\u00d0\u0001\u00d0\u0001\u00d0\u0001\u00d0\u0003"+
"\u00d0\u0a57\b\u00d0\u0001\u00d0\u0001\u00d0\u0001\u00d0\u0001\u00d1\u0003"+
"\u00d1\u0a5d\b\u00d1\u0001\u00d1\u0001\u00d1\u0001\u00d1\u0003\u00d1\u0a62"+
"\b\u00d1\u0001\u00d1\u0003\u00d1\u0a65\b\u00d1\u0001\u00d2\u0001\u00d2"+
"\u0001\u00d2\u0001\u00d2\u0001\u00d3\u0001\u00d3\u0001\u00d3\u0001\u00d3"+
"\u0001\u00d4\u0001\u00d4\u0001\u00d4\u0001\u00d4\u0001\u00d4\u0003\u00d4"+
"\u0a74\b\u00d4\u0001\u00d4\u0001\u00d4\u0001\u00d4\u0001\u00d4\u0001\u00d4"+
"\u0001\u00d4\u0001\u00d4\u0003\u00d4\u0a7d\b\u00d4\u0004\u00d4\u0a7f\b"+
"\u00d4\u000b\u00d4\f\u00d4\u0a80\u0001\u00d5\u0001\u00d5\u0001\u00d5\u0001"+
"\u00d5\u0003\u00d5\u0a87\b\u00d5\u0001\u00d6\u0001\u00d6\u0001\u00d6\u0001"+
"\u00d6\u0003\u00d6\u0a8d\b\u00d6\u0001\u00d6\u0001\u00d6\u0001\u00d6\u0001"+
"\u00d7\u0001\u00d7\u0001\u00d7\u0001\u00d7\u0001\u00d7\u0001\u00d7\u0001"+
"\u00d7\u0001\u00d7\u0001\u00d7\u0001\u00d8\u0001\u00d8\u0001\u00d8\u0001"+
"\u00d8\u0003\u00d8\u0a9f\b\u00d8\u0001\u00d8\u0001\u00d8\u0001\u00d8\u0001"+
"\u00d8\u0001\u00d8\u0001\u00d8\u0003\u00d8\u0aa7\b\u00d8\u0001\u00d8\u0003"+
"\u00d8\u0aaa\b\u00d8\u0005\u00d8\u0aac\b\u00d8\n\u00d8\f\u00d8\u0aaf\t"+
"\u00d8\u0001\u00d8\u0001\u00d8\u0001\u00d8\u0001\u00d8\u0001\u00d8\u0001"+
"\u00d8\u0001\u00d8\u0003\u00d8\u0ab8\b\u00d8\u0005\u00d8\u0aba\b\u00d8"+
"\n\u00d8\f\u00d8\u0abd\t\u00d8\u0001\u00d9\u0001\u00d9\u0003\u00d9\u0ac1"+
"\b\u00d9\u0001\u00d9\u0001\u00d9\u0001\u00d9\u0003\u00d9\u0ac6\b\u00d9"+
"\u0001\u00da\u0003\u00da\u0ac9\b\u00da\u0001\u00da\u0001\u00da\u0001\u00da"+
"\u0003\u00da\u0ace\b\u00da\u0001\u00db\u0003\u00db\u0ad1\b\u00db\u0001"+
"\u00db\u0001\u00db\u0001\u00db\u0001\u00dc\u0001\u00dc\u0003\u00dc\u0ad8"+
"\b\u00dc\u0001\u00dd\u0001\u00dd\u0003\u00dd\u0adc\b\u00dd\u0001\u00dd"+
"\u0001\u00dd\u0001\u00de\u0001\u00de\u0001\u00de\u0001\u00df\u0001\u00df"+
"\u0001\u00df\u0001\u00df\u0001\u00e0\u0001\u00e0\u0003\u00e0\u0ae9\b\u00e0"+
"\u0001\u00e0\u0001\u00e0\u0001\u00e0\u0001\u00e0\u0004\u00e0\u0aef\b\u00e0"+
"\u000b\u00e0\f\u00e0\u0af0\u0001\u00e0\u0001\u00e0\u0001\u00e1\u0001\u00e1"+
"\u0001\u00e1\u0001\u00e1\u0001\u00e1\u0003\u00e1\u0afa\b\u00e1\u0001\u00e2"+
"\u0001\u00e2\u0001\u00e2\u0003\u00e2\u0aff\b\u00e2\u0001\u00e2\u0003\u00e2"+
"\u0b02\b\u00e2\u0001\u00e3\u0001\u00e3\u0001\u00e3\u0003\u00e3\u0b07\b"+
"\u00e3\u0001\u00e4\u0001\u00e4\u0001\u00e4\u0003\u00e4\u0b0c\b\u00e4\u0001"+
"\u00e5\u0003\u00e5\u0b0f\b\u00e5\u0001\u00e5\u0001\u00e5\u0003\u00e5\u0b13"+
"\b\u00e5\u0001\u00e5\u0003\u00e5\u0b16\b\u00e5\u0001\u00e6\u0001\u00e6"+
"\u0001\u00e6\u0001\u00e6\u0003\u00e6\u0b1c\b\u00e6\u0001\u00e6\u0003\u00e6"+
"\u0b1f\b\u00e6\u0001\u00e7\u0001\u00e7\u0003\u00e7\u0b23\b\u00e7\u0001"+
"\u00e7\u0001\u00e7\u0003\u00e7\u0b27\b\u00e7\u0001\u00e7\u0003\u00e7\u0b2a"+
"\b\u00e7\u0001\u00e8\u0001\u00e8\u0003\u00e8\u0b2e\b\u00e8\u0001\u00e8"+
"\u0001\u00e8\u0001\u00e9\u0001\u00e9\u0001\u00ea\u0001\u00ea\u0001\u00ea"+
"\u0001\u00ea\u0001\u00ea\u0001\u00ea\u0001\u00ea\u0001\u00ea\u0001\u00ea"+
"\u0001\u00ea\u0001\u00ea\u0001\u00ea\u0003\u00ea\u0b40\b\u00ea\u0001\u00eb"+
"\u0001\u00eb\u0003\u00eb\u0b44\b\u00eb\u0001\u00eb\u0001\u00eb\u0001\u00eb"+
"\u0001\u00ec\u0003\u00ec\u0b4a\b\u00ec\u0001\u00ec\u0001\u00ec\u0001\u00ed"+
"\u0001\u00ed\u0001\u00ed\u0001\u00ed\u0001\u00ed\u0003\u00ed\u0b53\b\u00ed"+
"\u0001\u00ed\u0001\u00ed\u0001\u00ed\u0003\u00ed\u0b58\b\u00ed\u0001\u00ed"+
"\u0003\u00ed\u0b5b\b\u00ed\u0001\u00ee\u0001\u00ee\u0001\u00ee\u0001\u00ee"+
"\u0001\u00ee\u0001\u00ee\u0001\u00ee\u0001\u00ee\u0001\u00ee\u0001\u00ee"+
"\u0001\u00ee\u0001\u00ee\u0001\u00ee\u0003\u00ee\u0b6a\b\u00ee\u0001\u00ef"+
"\u0001\u00ef\u0001\u00ef\u0001\u00ef\u0001\u00ef\u0003\u00ef\u0b71\b\u00ef"+
"\u0001\u00f0\u0001\u00f0\u0003\u00f0\u0b75\b\u00f0\u0001\u00f0\u0001\u00f0"+
"\u0001\u00f1\u0001\u00f1\u0003\u00f1\u0b7b\b\u00f1\u0001\u00f1\u0001\u00f1"+
"\u0001\u00f2\u0001\u00f2\u0003\u00f2\u0b81\b\u00f2\u0001\u00f2\u0001\u00f2"+
"\u0001\u00f3\u0001\u00f3\u0003\u00f3\u0b87\b\u00f3\u0001\u00f3\u0001\u00f3"+
"\u0001\u00f3\u0003\u00f3\u0b8c\b\u00f3\u0001\u00f4\u0001\u00f4\u0001\u00f4"+
"\u0003\u00f4\u0b91\b\u00f4\u0001\u00f4\u0001\u00f4\u0001\u00f4\u0001\u00f4"+
"\u0001\u00f4\u0001\u00f4\u0001\u00f4\u0003\u00f4\u0b9a\b\u00f4\u0001\u00f5"+
"\u0001\u00f5\u0001\u00f5\u0001\u00f5\u0001\u00f5\u0001\u00f5\u0003\u00f5"+
"\u0ba2\b\u00f5\u0001\u00f6\u0001\u00f6\u0001\u00f6\u0001\u00f6\u0001\u00f6"+
"\u0003\u00f6\u0ba9\b\u00f6\u0003\u00f6\u0bab\b\u00f6\u0001\u00f6\u0001"+
"\u00f6\u0001\u00f6\u0001\u00f6\u0001\u00f6\u0001\u00f6\u0001\u00f6\u0001"+
"\u00f6\u0001\u00f6\u0001\u00f6\u0001\u00f6\u0001\u00f6\u0003\u00f6\u0bb9"+
"\b\u00f6\u0001\u00f6\u0001\u00f6\u0003\u00f6\u0bbd\b\u00f6\u0001\u00f7"+
"\u0001\u00f7\u0001\u00f7\u0001\u00f7\u0001\u00f7\u0001\u00f7\u0003\u00f7"+
"\u0bc5\b\u00f7\u0001\u00f7\u0001\u00f7\u0003\u00f7\u0bc9\b\u00f7\u0001"+
"\u00f7\u0001\u00f7\u0001\u00f7\u0001\u00f7\u0001\u00f7\u0001\u00f7\u0001"+
"\u00f7\u0001\u00f7\u0001\u00f7\u0001\u00f7\u0001\u00f7\u0001\u00f7\u0001"+
"\u00f7\u0001\u00f7\u0001\u00f7\u0001\u00f7\u0003\u00f7\u0bdb\b\u00f7\u0001"+
"\u00f8\u0001\u00f8\u0001\u00f9\u0001\u00f9\u0001\u00f9\u0001\u00f9\u0001"+
"\u00f9\u0001\u00f9\u0001\u00f9\u0001\u00f9\u0001\u00f9\u0003\u00f9\u0be8"+
"\b\u00f9\u0001\u00fa\u0001\u00fa\u0001\u00fa\u0001\u00fa\u0001\u00fb\u0001"+
"\u00fb\u0001\u00fb\u0001\u00fb\u0001\u00fb\u0001\u00fb\u0003\u00fb\u0bf4"+
"\b\u00fb\u0001\u00fb\u0003\u00fb\u0bf7\b\u00fb\u0001\u00fb\u0001\u00fb"+
"\u0003\u00fb\u0bfb\b\u00fb\u0001\u00fb\u0001\u00fb\u0003\u00fb\u0bff\b"+
"\u00fb\u0001\u00fb\u0003\u00fb\u0c02\b\u00fb\u0003\u00fb\u0c04\b\u00fb"+
"\u0001\u00fb\u0001\u00fb\u0001\u00fb\u0001\u00fc\u0001\u00fc\u0001\u00fc"+
"\u0001\u00fc\u0001\u00fc\u0001\u00fc\u0003\u00fc\u0c0f\b\u00fc\u0001\u00fc"+
"\u0001\u00fc\u0001\u00fc\u0001\u00fc\u0001\u00fc\u0003\u00fc\u0c16\b\u00fc"+
"\u0001\u00fc\u0001\u00fc\u0001\u00fc\u0001\u00fc\u0001\u00fc\u0001\u00fc"+
"\u0003\u00fc\u0c1e\b\u00fc\u0003\u00fc\u0c20\b\u00fc\u0001\u00fc\u0001"+
"\u00fc\u0001\u00fc\u0001\u00fd\u0001\u00fd\u0001\u00fd\u0001\u00fd\u0001"+
"\u00fd\u0003\u00fd\u0c2a\b\u00fd\u0001\u00fd\u0001\u00fd\u0001\u00fd\u0001"+
"\u00fd\u0001\u00fd\u0003\u00fd\u0c31\b\u00fd\u0003\u00fd\u0c33\b\u00fd"+
"\u0001\u00fd\u0001\u00fd\u0001\u00fd\u0003\u00fd\u0c38\b\u00fd\u0003\u00fd"+
"\u0c3a\b\u00fd\u0001\u00fe\u0001\u00fe\u0001\u00ff\u0001\u00ff\u0001\u0100"+
"\u0001\u0100\u0001\u0101\u0001\u0101\u0001\u0102\u0001\u0102\u0001\u0103"+
"\u0001\u0103\u0001\u0103\u0003\u0103\u0c49\b\u0103\u0001\u0103\u0001\u0103"+
"\u0001\u0104\u0001\u0104\u0001\u0105\u0001\u0105\u0001\u0106\u0001\u0106"+
"\u0001\u0107\u0001\u0107\u0001\u0107\u0005\u0107\u0c56\b\u0107\n\u0107"+
"\f\u0107\u0c59\t\u0107\u0001\u0108\u0001\u0108\u0003\u0108\u0c5d\b\u0108"+
"\u0001\u0108\u0003\u0108\u0c60\b\u0108\u0001\u0109\u0001\u0109\u0003\u0109"+
"\u0c64\b\u0109\u0001\u010a\u0001\u010a\u0003\u010a\u0c68\b\u010a\u0001"+
"\u010a\u0001\u010a\u0001\u010a\u0003\u010a\u0c6d\b\u010a\u0001\u010b\u0001"+
"\u010b\u0001\u010b\u0003\u010b\u0c72\b\u010b\u0001\u010b\u0001\u010b\u0001"+
"\u010b\u0001\u010b\u0001\u010b\u0001\u010b\u0003\u010b\u0c7a\b\u010b\u0001"+
"\u010c\u0001\u010c\u0001\u010c\u0003\u010c\u0c7f\b\u010c\u0001\u010c\u0001"+
"\u010c\u0001\u010c\u0001\u010c\u0001\u010d\u0001\u010d\u0003\u010d\u0c87"+
"\b\u010d\u0001\u010e\u0001\u010e\u0001\u010e\u0003\u010e\u0c8c\b\u010e"+
"\u0001\u010e\u0001\u010e\u0001\u010f\u0001\u010f\u0001\u010f\u0005\u010f"+
"\u0c93\b\u010f\n\u010f\f\u010f\u0c96\t\u010f\u0001\u0110\u0001\u0110\u0001"+
"\u0110\u0003\u0110\u0c9b\b\u0110\u0001\u0110\u0001\u0110\u0001\u0110\u0003"+
"\u0110\u0ca0\b\u0110\u0001\u0110\u0001\u0110\u0001\u0110\u0001\u0110\u0005"+
"\u0110\u0ca6\b\u0110\n\u0110\f\u0110\u0ca9\t\u0110\u0003\u0110\u0cab\b"+
"\u0110\u0001\u0110\u0001\u0110\u0001\u0110\u0001\u0110\u0001\u0110\u0001"+
"\u0110\u0003\u0110\u0cb3\b\u0110\u0001\u0110\u0001\u0110\u0003\u0110\u0cb7"+
"\b\u0110\u0003\u0110\u0cb9\b\u0110\u0001\u0111\u0001\u0111\u0001\u0111"+
"\u0003\u0111\u0cbe\b\u0111\u0001\u0112\u0001\u0112\u0001\u0113\u0001\u0113"+
"\u0001\u0114\u0001\u0114\u0001\u0115\u0001\u0115\u0001\u0115\u0001\u0115"+
"\u0001\u0115\u0003\u0115\u0ccb\b\u0115\u0003\u0115\u0ccd\b\u0115\u0001"+
"\u0116\u0001\u0116\u0001\u0116\u0001\u0116\u0001\u0116\u0003\u0116\u0cd4"+
"\b\u0116\u0003\u0116\u0cd6\b\u0116\u0001\u0117\u0001\u0117\u0001\u0117"+
"\u0001\u0117\u0001\u0117\u0001\u0117\u0003\u0117\u0cde\b\u0117\u0001\u0117"+
"\u0003\u0117\u0ce1\b\u0117\u0001\u0117\u0003\u0117\u0ce4\b\u0117\u0001"+
"\u0118\u0001\u0118\u0001\u0118\u0001\u0118\u0001\u0118\u0003\u0118\u0ceb"+
"\b\u0118\u0001\u0118\u0001\u0118\u0001\u0118\u0004\u0118\u0cf0\b\u0118"+
"\u000b\u0118\f\u0118\u0cf1\u0003\u0118\u0cf4\b\u0118\u0001\u0118\u0003"+
"\u0118\u0cf7\b\u0118\u0001\u0118\u0003\u0118\u0cfa\b\u0118\u0001\u0119"+
"\u0001\u0119\u0001\u0119\u0001\u011a\u0001\u011a\u0001\u011b\u0001\u011b"+
"\u0001\u011b\u0001\u011c\u0001\u011c\u0001\u011d\u0003\u011d\u0d07\b\u011d"+
"\u0001\u011d\u0001\u011d\u0001\u011d\u0001\u011d\u0003\u011d\u0d0d\b\u011d"+
"\u0001\u011d\u0003\u011d\u0d10\b\u011d\u0001\u011d\u0001\u011d\u0003\u011d"+
"\u0d14\b\u011d\u0001\u011d\u0003\u011d\u0d17\b\u011d\u0001\u011e\u0001"+
"\u011e\u0001\u011e\u0003\u011e\u0d1c\b\u011e\u0001\u011f\u0001\u011f\u0001"+
"\u011f\u0001\u011f\u0003\u011f\u0d22\b\u011f\u0001\u011f\u0001\u011f\u0001"+
"\u011f\u0001\u011f\u0003\u011f\u0d28\b\u011f\u0004\u011f\u0d2a\b\u011f"+
"\u000b\u011f\f\u011f\u0d2b\u0001\u011f\u0001\u011f\u0001\u011f\u0004\u011f"+
"\u0d31\b\u011f\u000b\u011f\f\u011f\u0d32\u0003\u011f\u0d35\b\u011f\u0001"+
"\u011f\u0003\u011f\u0d38\b\u011f\u0001\u0120\u0001\u0120\u0001\u0120\u0001"+
"\u0120\u0001\u0121\u0001\u0121\u0001\u0121\u0004\u0121\u0d41\b\u0121\u000b"+
"\u0121\f\u0121\u0d42\u0001\u0122\u0001\u0122\u0001\u0122\u0001\u0122\u0001"+
"\u0123\u0001\u0123\u0001\u0123\u0001\u0123\u0003\u0123\u0d4d\b\u0123\u0001"+
"\u0124\u0001\u0124\u0001\u0124\u0001\u0124\u0003\u0124\u0d53\b\u0124\u0001"+
"\u0125\u0001\u0125\u0001\u0125\u0003\u0125\u0d58\b\u0125\u0003\u0125\u0d5a"+
"\b\u0125\u0001\u0125\u0003\u0125\u0d5d\b\u0125\u0001\u0126\u0001\u0126"+
"\u0001\u0127\u0001\u0127\u0001\u0127\u0003\u0127\u0d64\b\u0127\u0001\u0127"+
"\u0001\u0127\u0003\u0127\u0d68\b\u0127\u0001\u0127\u0003\u0127\u0d6b\b"+
"\u0127\u0003\u0127\u0d6d\b\u0127\u0001\u0128\u0001\u0128\u0001\u0129\u0001"+
"\u0129\u0001\u012a\u0001\u012a\u0001\u012a\u0001\u012a\u0001\u012a\u0003"+
"\u012a\u0d78\b\u012a\u0001\u012a\u0001\u012a\u0001\u012a\u0001\u012a\u0001"+
"\u012a\u0001\u012a\u0001\u012a\u0001\u012a\u0001\u012a\u0001\u012a\u0001"+
"\u012a\u0003\u012a\u0d85\b\u012a\u0003\u012a\u0d87\b\u012a\u0001\u012a"+
"\u0001\u012a\u0003\u012a\u0d8b\b\u012a\u0001\u012b\u0001\u012b\u0001\u012b"+
"\u0001\u012b\u0003\u012b\u0d91\b\u012b\u0001\u012b\u0001\u012b\u0001\u012b"+
"\u0001\u012c\u0001\u012c\u0001\u012c\u0001\u012c\u0003\u012c\u0d9a\b\u012c"+
"\u0001\u012c\u0001\u012c\u0001\u012c\u0001\u012c\u0001\u012c\u0001\u012c"+
"\u0001\u012c\u0004\u012c\u0da3\b\u012c\u000b\u012c\f\u012c\u0da4\u0001"+
"\u012d\u0001\u012d\u0001\u012d\u0001\u012d\u0003\u012d\u0dab\b\u012d\u0001"+
"\u012e\u0001\u012e\u0001\u012e\u0001\u012f\u0001\u012f\u0001\u012f\u0001"+
"\u0130\u0001\u0130\u0001\u0130\u0001\u0131\u0001\u0131\u0001\u0131\u0001"+
"\u0132\u0001\u0132\u0003\u0132\u0dbb\b\u0132\u0001\u0132\u0001\u0132\u0001"+
"\u0132\u0003\u0132\u0dc0\b\u0132\u0001\u0133\u0001\u0133\u0003\u0133\u0dc4"+
"\b\u0133\u0001\u0134\u0001\u0134\u0003\u0134\u0dc8\b\u0134\u0001\u0135"+
"\u0001\u0135\u0001\u0135\u0005\u0135\u0dcd\b\u0135\n\u0135\f\u0135\u0dd0"+
"\t\u0135\u0001\u0136\u0001\u0136\u0001\u0136\u0005\u0136\u0dd5\b\u0136"+
"\n\u0136\f\u0136\u0dd8\t\u0136\u0001\u0137\u0001\u0137\u0003\u0137\u0ddc"+
"\b\u0137\u0001\u0138\u0001\u0138\u0001\u0138\u0005\u0138\u0de1\b\u0138"+
"\n\u0138\f\u0138\u0de4\t\u0138\u0001\u0139\u0001\u0139\u0001\u0139\u0001"+
"\u0139\u0005\u0139\u0dea\b\u0139\n\u0139\f\u0139\u0ded\t\u0139\u0003\u0139"+
"\u0def\b\u0139\u0001\u0139\u0001\u0139\u0001\u013a\u0001\u013a\u0001\u013a"+
"\u0004\u013a\u0df6\b\u013a\u000b\u013a\f\u013a\u0df7\u0001\u013b\u0001"+
"\u013b\u0001\u013c\u0001\u013c\u0003\u013c\u0dfe\b\u013c\u0001\u013d\u0001"+
"\u013d\u0003\u013d\u0e02\b\u013d\u0001\u013e\u0001\u013e\u0003\u013e\u0e06"+
"\b\u013e\u0001\u013f\u0001\u013f\u0001\u013f\u0001\u013f\u0001\u013f\u0001"+
"\u013f\u0001\u013f\u0001\u013f\u0001\u013f\u0005\u013f\u0e11\b\u013f\n"+
"\u013f\f\u013f\u0e14\t\u013f\u0003\u013f\u0e16\b\u013f\u0001\u013f\u0001"+
"\u013f\u0001\u0140\u0001\u0140\u0003\u0140\u0e1c\b\u0140\u0001\u0141\u0001"+
"\u0141\u0001\u0142\u0001\u0142\u0001\u0142\u0001\u0142\u0001\u0142\u0001"+
"\u0142\u0001\u0142\u0001\u0142\u0001\u0142\u0003\u0142\u0e29\b\u0142\u0001"+
"\u0143\u0001\u0143\u0003\u0143\u0e2d\b\u0143\u0001\u0144\u0001\u0144\u0001"+
"\u0145\u0001\u0145\u0001\u0145\u0000\u0000\u0146\u0000\u0002\u0004\u0006"+
"\b\n\f\u000e\u0010\u0012\u0014\u0016\u0018\u001a\u001c\u001e \"$&(*,."+
"02468:<>@BDFHJLNPRTVXZ\\^`bdfhjlnprtvxz|~\u0080\u0082\u0084\u0086\u0088"+
"\u008a\u008c\u008e\u0090\u0092\u0094\u0096\u0098\u009a\u009c\u009e\u00a0"+
"\u00a2\u00a4\u00a6\u00a8\u00aa\u00ac\u00ae\u00b0\u00b2\u00b4\u00b6\u00b8"+
"\u00ba\u00bc\u00be\u00c0\u00c2\u00c4\u00c6\u00c8\u00ca\u00cc\u00ce\u00d0"+
"\u00d2\u00d4\u00d6\u00d8\u00da\u00dc\u00de\u00e0\u00e2\u00e4\u00e6\u00e8"+
"\u00ea\u00ec\u00ee\u00f0\u00f2\u00f4\u00f6\u00f8\u00fa\u00fc\u00fe\u0100"+
"\u0102\u0104\u0106\u0108\u010a\u010c\u010e\u0110\u0112\u0114\u0116\u0118"+
"\u011a\u011c\u011e\u0120\u0122\u0124\u0126\u0128\u012a\u012c\u012e\u0130"+
"\u0132\u0134\u0136\u0138\u013a\u013c\u013e\u0140\u0142\u0144\u0146\u0148"+
"\u014a\u014c\u014e\u0150\u0152\u0154\u0156\u0158\u015a\u015c\u015e\u0160"+
"\u0162\u0164\u0166\u0168\u016a\u016c\u016e\u0170\u0172\u0174\u0176\u0178"+
"\u017a\u017c\u017e\u0180\u0182\u0184\u0186\u0188\u018a\u018c\u018e\u0190"+
"\u0192\u0194\u0196\u0198\u019a\u019c\u019e\u01a0\u01a2\u01a4\u01a6\u01a8"+
"\u01aa\u01ac\u01ae\u01b0\u01b2\u01b4\u01b6\u01b8\u01ba\u01bc\u01be\u01c0"+
"\u01c2\u01c4\u01c6\u01c8\u01ca\u01cc\u01ce\u01d0\u01d2\u01d4\u01d6\u01d8"+
"\u01da\u01dc\u01de\u01e0\u01e2\u01e4\u01e6\u01e8\u01ea\u01ec\u01ee\u01f0"+
"\u01f2\u01f4\u01f6\u01f8\u01fa\u01fc\u01fe\u0200\u0202\u0204\u0206\u0208"+
"\u020a\u020c\u020e\u0210\u0212\u0214\u0216\u0218\u021a\u021c\u021e\u0220"+
"\u0222\u0224\u0226\u0228\u022a\u022c\u022e\u0230\u0232\u0234\u0236\u0238"+
"\u023a\u023c\u023e\u0240\u0242\u0244\u0246\u0248\u024a\u024c\u024e\u0250"+
"\u0252\u0254\u0256\u0258\u025a\u025c\u025e\u0260\u0262\u0264\u0266\u0268"+
"\u026a\u026c\u026e\u0270\u0272\u0274\u0276\u0278\u027a\u027c\u027e\u0280"+
"\u0282\u0284\u0286\u0288\u028a\u0000J\u0002\u0000\u0012\u0012NN\u0001"+
"\u0000\u0018\u0019\u0001\u0000HI\u0002\u0000\u00b5\u00b5\u00fd\u00fd\u0002"+
"\u0000KK\u00ab\u00ab\u0002\u0000::\u009c\u009c\u0001\u0000\u00e8\u00e9"+
"\u0003\u0000##88hh\u0002\u0000\u0011\u0011\u00f8\u00f8\u0001\u0000wx\u0001"+
"\u0000\u00bf\u00c0\u0002\u0000\u0099\u0099\u0132\u0132\u0002\u0000\u009e"+
"\u009e\u0131\u0131\u0002\u0000yy\u0133\u0133\u0002\u0000--\u008d\u008d"+
"\u0002\u0000--\u0088\u0088\u0006\u0000aassyy\u0091\u0091\u0099\u0099\u00a0"+
"\u00a1\u0002\u0000..\u0116\u0116\u0001\u0000\u00a5\u00a8\u0003\u0000R"+
"R\u009e\u009e\u00c2\u00c2\u0003\u0000OO\u009f\u009f\u010a\u010a\u0002"+
"\u0000\u009e\u009e\u00c2\u00c2\u0004\u0000\u0012\u0012\u0015\u0015\u00ad"+
"\u00ad\u00fc\u00fc\u0003\u0000\"\"\u0092\u0092\u010f\u010f\u0001\u0000"+
"\u0004\u0007\u0002\u0000AA\u0109\u0109\u0001\u0000\u0128\u0129\u0002\u0000"+
"\u0016\u0016\u0094\u0094\b\u0000\u0012\u0012$$pp\u0097\u0097\u00c4\u00c4"+
"\u00d2\u00d2\u0107\u0107\u0123\u0123\u0001\u0000\u0119\u011a\u0001\u0000"+
"\u00da\u00db\u0001\u0000\u00cb\u00cc\u0001\u0000qr\u0001\u0000\u00f6\u00f7"+
"\u0002\u0000\u00aa\u00aa\u00da\u00db\u0002\u0000GGtt\u0001\u0000\u00e6"+
"\u00e7\u0001\u0000\u00f3\u00f4\u0001\u0000>?\u0002\u0000\u0012\u0012\u00c5"+
"\u00c5\u0001\u0000\u011e\u011f\u0001\u0000\u00cf\u00d0\u0002\u0000^^\u00c1"+
"\u00c1\u0002\u0000\f\f\u0104\u0104\u0001\u000001\u0001\u0000\u00c9\u00ca"+
"\u0003\u0000>>BBuu\u0002\u0000DD{{\u0002\u0000>>uu\u0001\u0000uv\u0001"+
"\u0000\u008b\u008c\u0002\u0000\u0115\u0115\u0117\u0117\u0001\u0000\u00a2"+
"\u00a3\u0002\u0000++\u011c\u011c\u0001\u0000\u00bd\u00be\u0002\u0000\u00c9"+
"\u00c9\u00e6\u00e6\u0003\u0000\u000f\u000f>>\u011e\u011e\u0002\u0000\u00e6"+
"\u00e6\u011e\u011e\u0001\u0000\r\u000e\u0001\u0000\u0081\u0082\u0001\u0000"+
"45\u0001\u0000\u0110\u0111\u0002\u0000\u009c\u009c\u00d5\u00d5\u0001\u0000"+
"\u00db\u00dc\u0001\u0000[\\\u0002\u0000\u00aa\u00aa\u00ac\u00ac\u0001"+
"\u0000\u00c7\u00c8\u0001\u0000\u00ee\u00ef\u0002\u0000JJVV\u0001\u0000"+
"\u000f\u0010\u0002\u0000\u00b7\u00b7\u012a\u012a\u0002\u0000\u00ec\u00ed"+
"\u00f0\u00f0\u0001\u0000\b\t\u0017\u0000\u000b\u001c\u001e,0LNNS`brtx"+
"z\u008c\u0092\u0097\u009a\u009d\u00a2\u00a4\u00a9\u00ae\u00b1\u00b2\u00b4"+
"\u00c1\u00c4\u00c5\u00c7\u00d0\u00d2\u00d2\u00d5\u00d8\u00da\u00e9\u00eb"+
"\u00f1\u00f3\u0109\u010b\u0115\u0117\u012f\u0fa3\u0000\u028c\u0001\u0000"+
"\u0000\u0000\u0002\u029b\u0001\u0000\u0000\u0000\u0004\u029d\u0001\u0000"+
"\u0000\u0000\u0006\u02a9\u0001\u0000\u0000\u0000\b\u02be\u0001\u0000\u0000"+
"\u0000\n\u02c0\u0001\u0000\u0000\u0000\f\u02cc\u0001\u0000\u0000\u0000"+
"\u000e\u02ce\u0001\u0000\u0000\u0000\u0010\u02d0\u0001\u0000\u0000\u0000"+
"\u0012\u02d4\u0001\u0000\u0000\u0000\u0014\u02e0\u0001\u0000\u0000\u0000"+
"\u0016\u02e7\u0001\u0000\u0000\u0000\u0018\u02f0\u0001\u0000\u0000\u0000"+
"\u001a\u02f5\u0001\u0000\u0000\u0000\u001c\u02f7\u0001\u0000\u0000\u0000"+
"\u001e\u02f9\u0001\u0000\u0000\u0000 \u0303\u0001\u0000\u0000\u0000\""+
"\u0306\u0001\u0000\u0000\u0000$\u0309\u0001\u0000\u0000\u0000&\u030c\u0001"+
"\u0000\u0000\u0000(\u0311\u0001\u0000\u0000\u0000*\u0314\u0001\u0000\u0000"+
"\u0000,\u0317\u0001\u0000\u0000\u0000.\u0336\u0001\u0000\u0000\u00000"+
"\u0338\u0001\u0000\u0000\u00002\u0349\u0001\u0000\u0000\u00004\u034c\u0001"+
"\u0000\u0000\u00006\u0358\u0001\u0000\u0000\u00008\u0378\u0001\u0000\u0000"+
"\u0000:\u037a\u0001\u0000\u0000\u0000<\u0398\u0001\u0000\u0000\u0000>"+
"\u03a0\u0001\u0000\u0000\u0000@\u03a4\u0001\u0000\u0000\u0000B\u03aa\u0001"+
"\u0000\u0000\u0000D\u03cd\u0001\u0000\u0000\u0000F\u03d0\u0001\u0000\u0000"+
"\u0000H\u03d2\u0001\u0000\u0000\u0000J\u03d7\u0001\u0000\u0000\u0000L"+
"\u03e5\u0001\u0000\u0000\u0000N\u03f3\u0001\u0000\u0000\u0000P\u03ff\u0001"+
"\u0000\u0000\u0000R\u040d\u0001\u0000\u0000\u0000T\u041d\u0001\u0000\u0000"+
"\u0000V\u0421\u0001\u0000\u0000\u0000X\u0425\u0001\u0000\u0000\u0000Z"+
"\u0436\u0001\u0000\u0000\u0000\\\u0438\u0001\u0000\u0000\u0000^\u0440"+
"\u0001\u0000\u0000\u0000`\u044b\u0001\u0000\u0000\u0000b\u0455\u0001\u0000"+
"\u0000\u0000d\u046e\u0001\u0000\u0000\u0000f\u0472\u0001\u0000\u0000\u0000"+
"h\u0474\u0001\u0000\u0000\u0000j\u0486\u0001\u0000\u0000\u0000l\u04ac"+
"\u0001\u0000\u0000\u0000n\u04ae\u0001\u0000\u0000\u0000p\u04b0\u0001\u0000"+
"\u0000\u0000r\u04b2\u0001\u0000\u0000\u0000t\u04ba\u0001\u0000\u0000\u0000"+
"v\u04ca\u0001\u0000\u0000\u0000x\u04d6\u0001\u0000\u0000\u0000z\u04e2"+
"\u0001\u0000\u0000\u0000|\u04e6\u0001\u0000\u0000\u0000~\u04f2\u0001\u0000"+
"\u0000\u0000\u0080\u04f7\u0001\u0000\u0000\u0000\u0082\u04fa\u0001\u0000"+
"\u0000\u0000\u0084\u04fd\u0001\u0000\u0000\u0000\u0086\u0500\u0001\u0000"+
"\u0000\u0000\u0088\u0505\u0001\u0000\u0000\u0000\u008a\u0508\u0001\u0000"+
"\u0000\u0000\u008c\u0524\u0001\u0000\u0000\u0000\u008e\u0534\u0001\u0000"+
"\u0000\u0000\u0090\u0536\u0001\u0000\u0000\u0000\u0092\u0538\u0001\u0000"+
"\u0000\u0000\u0094\u053a\u0001\u0000\u0000\u0000\u0096\u0549\u0001\u0000"+
"\u0000\u0000\u0098\u054b\u0001\u0000\u0000\u0000\u009a\u0556\u0001\u0000"+
"\u0000\u0000\u009c\u0561\u0001\u0000\u0000\u0000\u009e\u0569\u0001\u0000"+
"\u0000\u0000\u00a0\u0574\u0001\u0000\u0000\u0000\u00a2\u057c\u0001\u0000"+
"\u0000\u0000\u00a4\u0587\u0001\u0000\u0000\u0000\u00a6\u058f\u0001\u0000"+
"\u0000\u0000\u00a8\u0591\u0001\u0000\u0000\u0000\u00aa\u059a\u0001\u0000"+
"\u0000\u0000\u00ac\u059d\u0001\u0000\u0000\u0000\u00ae\u05a5\u0001\u0000"+
"\u0000\u0000\u00b0\u05ad\u0001\u0000\u0000\u0000\u00b2\u05b8\u0001\u0000"+
"\u0000\u0000\u00b4\u05bd\u0001\u0000\u0000\u0000\u00b6\u05c5\u0001\u0000"+
"\u0000\u0000\u00b8\u05e9\u0001\u0000\u0000\u0000\u00ba\u05eb\u0001\u0000"+
"\u0000\u0000\u00bc\u05ed\u0001\u0000\u0000\u0000\u00be\u05f5\u0001\u0000"+
"\u0000\u0000\u00c0\u05fd\u0001\u0000\u0000\u0000\u00c2\u0608\u0001\u0000"+
"\u0000\u0000\u00c4\u060a\u0001\u0000\u0000\u0000\u00c6\u0620\u0001\u0000"+
"\u0000\u0000\u00c8\u0622\u0001\u0000\u0000\u0000\u00ca\u0625\u0001\u0000"+
"\u0000\u0000\u00cc\u0629\u0001\u0000\u0000\u0000\u00ce\u062f\u0001\u0000"+
"\u0000\u0000\u00d0\u0647\u0001\u0000\u0000\u0000\u00d2\u0652\u0001\u0000"+
"\u0000\u0000\u00d4\u0654\u0001\u0000\u0000\u0000\u00d6\u0660\u0001\u0000"+
"\u0000\u0000\u00d8\u0665\u0001\u0000\u0000\u0000\u00da\u0672\u0001\u0000"+
"\u0000\u0000\u00dc\u069f\u0001\u0000\u0000\u0000\u00de\u06a1\u0001\u0000"+
"\u0000\u0000\u00e0\u06b3\u0001\u0000\u0000\u0000\u00e2\u06c2\u0001\u0000"+
"\u0000\u0000\u00e4\u06cf\u0001\u0000\u0000\u0000\u00e6\u06da\u0001\u0000"+
"\u0000\u0000\u00e8\u06e3\u0001\u0000\u0000\u0000\u00ea\u06f1\u0001\u0000"+
"\u0000\u0000\u00ec\u06f3\u0001\u0000\u0000\u0000\u00ee\u06f5\u0001\u0000"+
"\u0000\u0000\u00f0\u06f9\u0001\u0000\u0000\u0000\u00f2\u070f\u0001\u0000"+
"\u0000\u0000\u00f4\u0711\u0001\u0000\u0000\u0000\u00f6\u0716\u0001\u0000"+
"\u0000\u0000\u00f8\u0724\u0001\u0000\u0000\u0000\u00fa\u0732\u0001\u0000"+
"\u0000\u0000\u00fc\u0738\u0001\u0000\u0000\u0000\u00fe\u073d\u0001\u0000"+
"\u0000\u0000\u0100\u0741\u0001\u0000\u0000\u0000\u0102\u074e\u0001\u0000"+
"\u0000\u0000\u0104\u0750\u0001\u0000\u0000\u0000\u0106\u0757\u0001\u0000"+
"\u0000\u0000\u0108\u0759\u0001\u0000\u0000\u0000\u010a\u076a\u0001\u0000"+
"\u0000\u0000\u010c\u076c\u0001\u0000\u0000\u0000\u010e\u0774\u0001\u0000"+
"\u0000\u0000\u0110\u0777\u0001\u0000\u0000\u0000\u0112\u0779\u0001\u0000"+
"\u0000\u0000\u0114\u0781\u0001\u0000\u0000\u0000\u0116\u0789\u0001\u0000"+
"\u0000\u0000\u0118\u07d4\u0001\u0000\u0000\u0000\u011a\u07d9\u0001\u0000"+
"\u0000\u0000\u011c\u07db\u0001\u0000\u0000\u0000\u011e\u07e0\u0001\u0000"+
"\u0000\u0000\u0120\u07f1\u0001\u0000\u0000\u0000\u0122\u07ff\u0001\u0000"+
"\u0000\u0000\u0124\u0809\u0001\u0000\u0000\u0000\u0126\u0821\u0001\u0000"+
"\u0000\u0000\u0128\u0823\u0001\u0000\u0000\u0000\u012a\u0828\u0001\u0000"+
"\u0000\u0000\u012c\u082b\u0001\u0000\u0000\u0000\u012e\u082e\u0001\u0000"+
"\u0000\u0000\u0130\u0846\u0001\u0000\u0000\u0000\u0132\u0849\u0001\u0000"+
"\u0000\u0000\u0134\u084e\u0001\u0000\u0000\u0000\u0136\u0850\u0001\u0000"+
"\u0000\u0000\u0138\u085a\u0001\u0000\u0000\u0000\u013a\u085e\u0001\u0000"+
"\u0000\u0000\u013c\u0860\u0001\u0000\u0000\u0000\u013e\u0884\u0001\u0000"+
"\u0000\u0000\u0140\u0888\u0001\u0000\u0000\u0000\u0142\u0890\u0001\u0000"+
"\u0000\u0000\u0144\u0892\u0001\u0000\u0000\u0000\u0146\u0899\u0001\u0000"+
"\u0000\u0000\u0148\u08a4\u0001\u0000\u0000\u0000\u014a\u08b0\u0001\u0000"+
"\u0000\u0000\u014c\u08b2\u0001\u0000\u0000\u0000\u014e\u08c0\u0001\u0000"+
"\u0000\u0000\u0150\u08c2\u0001\u0000\u0000\u0000\u0152\u08c5\u0001\u0000"+
"\u0000\u0000\u0154\u08c8\u0001\u0000\u0000\u0000\u0156\u08cb\u0001\u0000"+
"\u0000\u0000\u0158\u08d4\u0001\u0000\u0000\u0000\u015a\u08db\u0001\u0000"+
"\u0000\u0000\u015c\u08dd\u0001\u0000\u0000\u0000\u015e\u08e2\u0001\u0000"+
"\u0000\u0000\u0160\u08f3\u0001\u0000\u0000\u0000\u0162\u0924\u0001\u0000"+
"\u0000\u0000\u0164\u0926\u0001\u0000\u0000\u0000\u0166\u0943\u0001\u0000"+
"\u0000\u0000\u0168\u0946\u0001\u0000\u0000\u0000\u016a\u0958\u0001\u0000"+
"\u0000\u0000\u016c\u096c\u0001\u0000\u0000\u0000\u016e\u0979\u0001\u0000"+
"\u0000\u0000\u0170\u0993\u0001\u0000\u0000\u0000\u0172\u09a6\u0001\u0000"+
"\u0000\u0000\u0174\u09ac\u0001\u0000\u0000\u0000\u0176\u09bf\u0001\u0000"+
"\u0000\u0000\u0178\u09cc\u0001\u0000\u0000\u0000\u017a\u09ce\u0001\u0000"+
"\u0000\u0000\u017c\u09d9\u0001\u0000\u0000\u0000\u017e\u09e1\u0001\u0000"+
"\u0000\u0000\u0180\u09e7\u0001\u0000\u0000\u0000\u0182\u09f4\u0001\u0000"+
"\u0000\u0000\u0184\u09fc\u0001\u0000\u0000\u0000\u0186\u0a0c\u0001\u0000"+
"\u0000\u0000\u0188\u0a0e\u0001\u0000\u0000\u0000\u018a\u0a10\u0001\u0000"+
"\u0000\u0000\u018c\u0a12\u0001\u0000\u0000\u0000\u018e\u0a18\u0001\u0000"+
"\u0000\u0000\u0190\u0a1d\u0001\u0000\u0000\u0000\u0192\u0a22\u0001\u0000"+
"\u0000\u0000\u0194\u0a25\u0001\u0000\u0000\u0000\u0196\u0a2a\u0001\u0000"+
"\u0000\u0000\u0198\u0a30\u0001\u0000\u0000\u0000\u019a\u0a3c\u0001\u0000"+
"\u0000\u0000\u019c\u0a3f\u0001\u0000\u0000\u0000\u019e\u0a4c\u0001\u0000"+
"\u0000\u0000\u01a0\u0a52\u0001\u0000\u0000\u0000\u01a2\u0a5c\u0001\u0000"+
"\u0000\u0000\u01a4\u0a66\u0001\u0000\u0000\u0000\u01a6\u0a6a\u0001\u0000"+
"\u0000\u0000\u01a8\u0a6e\u0001\u0000\u0000\u0000\u01aa\u0a82\u0001\u0000"+
"\u0000\u0000\u01ac\u0a88\u0001\u0000\u0000\u0000\u01ae\u0a91\u0001\u0000"+
"\u0000\u0000\u01b0\u0a9a\u0001\u0000\u0000\u0000\u01b2\u0abe\u0001\u0000"+
"\u0000\u0000\u01b4\u0ac8\u0001\u0000\u0000\u0000\u01b6\u0ad0\u0001\u0000"+
"\u0000\u0000\u01b8\u0ad7\u0001\u0000\u0000\u0000\u01ba\u0ad9\u0001\u0000"+
"\u0000\u0000\u01bc\u0adf\u0001\u0000\u0000\u0000\u01be\u0ae2\u0001\u0000"+
"\u0000\u0000\u01c0\u0ae6\u0001\u0000\u0000\u0000\u01c2\u0af9\u0001\u0000"+
"\u0000\u0000\u01c4\u0afb\u0001\u0000\u0000\u0000\u01c6\u0b03\u0001\u0000"+
"\u0000\u0000\u01c8\u0b08\u0001\u0000\u0000\u0000\u01ca\u0b0e\u0001\u0000"+
"\u0000\u0000\u01cc\u0b17\u0001\u0000\u0000\u0000\u01ce\u0b20\u0001\u0000"+
"\u0000\u0000\u01d0\u0b2b\u0001\u0000\u0000\u0000\u01d2\u0b31\u0001\u0000"+
"\u0000\u0000\u01d4\u0b3f\u0001\u0000\u0000\u0000\u01d6\u0b41\u0001\u0000"+
"\u0000\u0000\u01d8\u0b49\u0001\u0000\u0000\u0000\u01da\u0b5a\u0001\u0000"+
"\u0000\u0000\u01dc\u0b5c\u0001\u0000\u0000\u0000\u01de\u0b70\u0001\u0000"+
"\u0000\u0000\u01e0\u0b72\u0001\u0000\u0000\u0000\u01e2\u0b78\u0001\u0000"+
"\u0000\u0000\u01e4\u0b7e\u0001\u0000\u0000\u0000\u01e6\u0b8b\u0001\u0000"+
"\u0000\u0000\u01e8\u0b8d\u0001\u0000\u0000\u0000\u01ea\u0b9b\u0001\u0000"+
"\u0000\u0000\u01ec\u0ba3\u0001\u0000\u0000\u0000\u01ee\u0bbe\u0001\u0000"+
"\u0000\u0000\u01f0\u0bdc\u0001\u0000\u0000\u0000\u01f2\u0bde\u0001\u0000"+
"\u0000\u0000\u01f4\u0be9\u0001\u0000\u0000\u0000\u01f6\u0c03\u0001\u0000"+
"\u0000\u0000\u01f8\u0c1f\u0001\u0000\u0000\u0000\u01fa\u0c24\u0001\u0000"+
"\u0000\u0000\u01fc\u0c3b\u0001\u0000\u0000\u0000\u01fe\u0c3d\u0001\u0000"+
"\u0000\u0000\u0200\u0c3f\u0001\u0000\u0000\u0000\u0202\u0c41\u0001\u0000"+
"\u0000\u0000\u0204\u0c43\u0001\u0000\u0000\u0000\u0206\u0c45\u0001\u0000"+
"\u0000\u0000\u0208\u0c4c\u0001\u0000\u0000\u0000\u020a\u0c4e\u0001\u0000"+
"\u0000\u0000\u020c\u0c50\u0001\u0000\u0000\u0000\u020e\u0c52\u0001\u0000"+
"\u0000\u0000\u0210\u0c5f\u0001\u0000\u0000\u0000\u0212\u0c61\u0001\u0000"+
"\u0000\u0000\u0214\u0c6c\u0001\u0000\u0000\u0000\u0216\u0c71\u0001\u0000"+
"\u0000\u0000\u0218\u0c7e\u0001\u0000\u0000\u0000\u021a\u0c86\u0001\u0000"+
"\u0000\u0000\u021c\u0c88\u0001\u0000\u0000\u0000\u021e\u0c8f\u0001\u0000"+
"\u0000\u0000\u0220\u0cb8\u0001\u0000\u0000\u0000\u0222\u0cbd\u0001\u0000"+
"\u0000\u0000\u0224\u0cbf\u0001\u0000\u0000\u0000\u0226\u0cc1\u0001\u0000"+
"\u0000\u0000\u0228\u0cc3\u0001\u0000\u0000\u0000\u022a\u0ccc\u0001\u0000"+
"\u0000\u0000\u022c\u0cd5\u0001\u0000\u0000\u0000\u022e\u0cd7\u0001\u0000"+
"\u0000\u0000\u0230\u0ce5\u0001\u0000\u0000\u0000\u0232\u0cfb\u0001\u0000"+
"\u0000\u0000\u0234\u0cfe\u0001\u0000\u0000\u0000\u0236\u0d00\u0001\u0000"+
"\u0000\u0000\u0238\u0d03\u0001\u0000\u0000\u0000\u023a\u0d06\u0001\u0000"+
"\u0000\u0000\u023c\u0d1b\u0001\u0000\u0000\u0000\u023e\u0d1d\u0001\u0000"+
"\u0000\u0000\u0240\u0d39\u0001\u0000\u0000\u0000\u0242\u0d3d\u0001\u0000"+
"\u0000\u0000\u0244\u0d44\u0001\u0000\u0000\u0000\u0246\u0d48\u0001\u0000"+
"\u0000\u0000\u0248\u0d4e\u0001\u0000\u0000\u0000\u024a\u0d5c\u0001\u0000"+
"\u0000\u0000\u024c\u0d5e\u0001\u0000\u0000\u0000\u024e\u0d6c\u0001\u0000"+
"\u0000\u0000\u0250\u0d6e\u0001\u0000\u0000\u0000\u0252\u0d70\u0001\u0000"+
"\u0000\u0000\u0254\u0d72\u0001\u0000\u0000\u0000\u0256\u0d8c\u0001\u0000"+
"\u0000\u0000\u0258\u0d95\u0001\u0000\u0000\u0000\u025a\u0da6\u0001\u0000"+
"\u0000\u0000\u025c\u0dac\u0001\u0000\u0000\u0000\u025e\u0daf\u0001\u0000"+
"\u0000\u0000\u0260\u0db2\u0001\u0000\u0000\u0000\u0262\u0db5\u0001\u0000"+
"\u0000\u0000\u0264\u0db8\u0001\u0000\u0000\u0000\u0266\u0dc3\u0001\u0000"+
"\u0000\u0000\u0268\u0dc7\u0001\u0000\u0000\u0000\u026a\u0dc9\u0001\u0000"+
"\u0000\u0000\u026c\u0dd1\u0001\u0000\u0000\u0000\u026e\u0ddb\u0001\u0000"+
"\u0000\u0000\u0270\u0ddd\u0001\u0000\u0000\u0000\u0272\u0de5\u0001\u0000"+
"\u0000\u0000\u0274\u0df2\u0001\u0000\u0000\u0000\u0276\u0df9\u0001\u0000"+
"\u0000\u0000\u0278\u0dfd\u0001\u0000\u0000\u0000\u027a\u0e01\u0001\u0000"+
"\u0000\u0000\u027c\u0e05\u0001\u0000\u0000\u0000\u027e\u0e07\u0001\u0000"+
"\u0000\u0000\u0280\u0e1b\u0001\u0000\u0000\u0000\u0282\u0e1d\u0001\u0000"+
"\u0000\u0000\u0284\u0e28\u0001\u0000\u0000\u0000\u0286\u0e2c\u0001\u0000"+
"\u0000\u0000\u0288\u0e2e\u0001\u0000\u0000\u0000\u028a\u0e30\u0001\u0000"+
"\u0000\u0000\u028c\u0291\u0003\u0002\u0001\u0000\u028d\u028e\u0005\u00f2"+
"\u0000\u0000\u028e\u0290\u0003\u0002\u0001\u0000\u028f\u028d\u0001\u0000"+
"\u0000\u0000\u0290\u0293\u0001\u0000\u0000\u0000\u0291\u028f\u0001\u0000"+
"\u0000\u0000\u0291\u0292\u0001\u0000\u0000\u0000\u0292\u0295\u0001\u0000"+
"\u0000\u0000\u0293\u0291\u0001\u0000\u0000\u0000\u0294\u0296\u0005\u00f2"+
"\u0000\u0000\u0295\u0294\u0001\u0000\u0000\u0000\u0295\u0296\u0001\u0000"+
"\u0000\u0000\u0296\u0297\u0001\u0000\u0000\u0000\u0297\u0298\u0005\u0000"+
"\u0000\u0001\u0298\u0001\u0001\u0000\u0000\u0000\u0299\u029c\u0003\u011e"+
"\u008f\u0000\u029a\u029c\u0003\u0004\u0002\u0000\u029b\u0299\u0001\u0000"+
"\u0000\u0000\u029b\u029a\u0001\u0000\u0000\u0000\u029c\u0003\u0001\u0000"+
"\u0000\u0000\u029d\u02a5\u0003\u0006\u0003\u0000\u029e\u02a0\u0005\u0118"+
"\u0000\u0000\u029f\u02a1\u0007\u0000\u0000\u0000\u02a0\u029f\u0001\u0000"+
"\u0000\u0000\u02a0\u02a1\u0001\u0000\u0000\u0000\u02a1\u02a2\u0001\u0000"+
"\u0000\u0000\u02a2\u02a4\u0003\u0006\u0003\u0000\u02a3\u029e\u0001\u0000"+
"\u0000\u0000\u02a4\u02a7\u0001\u0000\u0000\u0000\u02a5\u02a3\u0001\u0000"+
"\u0000\u0000\u02a5\u02a6\u0001\u0000\u0000\u0000\u02a6\u0005\u0001\u0000"+
"\u0000\u0000\u02a7\u02a5\u0001\u0000\u0000\u0000\u02a8\u02aa\u0003\b\u0004"+
"\u0000\u02a9\u02a8\u0001\u0000\u0000\u0000\u02aa\u02ab\u0001\u0000\u0000"+
"\u0000\u02ab\u02a9\u0001\u0000\u0000\u0000\u02ab\u02ac\u0001\u0000\u0000"+
"\u0000\u02ac\u0007\u0001\u0000\u0000\u0000\u02ad\u02bf\u0003\n\u0005\u0000"+
"\u02ae\u02bf\u0003\u000e\u0007\u0000\u02af\u02bf\u0003\u0010\b\u0000\u02b0"+
"\u02bf\u0003(\u0014\u0000\u02b1\u02bf\u0003*\u0015\u0000\u02b2\u02bf\u0003"+
"4\u001a\u0000\u02b3\u02bf\u0003,\u0016\u0000\u02b4\u02bf\u00030\u0018"+
"\u0000\u02b5\u02bf\u00036\u001b\u0000\u02b6\u02bf\u0003<\u001e\u0000\u02b7"+
"\u02bf\u0003&\u0013\u0000\u02b8\u02bf\u0003@ \u0000\u02b9\u02bf\u0003"+
"B!\u0000\u02ba\u02bf\u0003N\'\u0000\u02bb\u02bf\u0003J%\u0000\u02bc\u02bf"+
"\u0003L&\u0000\u02bd\u02bf\u0003Z-\u0000\u02be\u02ad\u0001\u0000\u0000"+
"\u0000\u02be\u02ae\u0001\u0000\u0000\u0000\u02be\u02af\u0001\u0000\u0000"+
"\u0000\u02be\u02b0\u0001\u0000\u0000\u0000\u02be\u02b1\u0001\u0000\u0000"+
"\u0000\u02be\u02b2\u0001\u0000\u0000\u0000\u02be\u02b3\u0001\u0000\u0000"+
"\u0000\u02be\u02b4\u0001\u0000\u0000\u0000\u02be\u02b5\u0001\u0000\u0000"+
"\u0000\u02be\u02b6\u0001\u0000\u0000\u0000\u02be\u02b7\u0001\u0000\u0000"+
"\u0000\u02be\u02b8\u0001\u0000\u0000\u0000\u02be\u02b9\u0001\u0000\u0000"+
"\u0000\u02be\u02ba\u0001\u0000\u0000\u0000\u02be\u02bb\u0001\u0000\u0000"+
"\u0000\u02be\u02bc\u0001\u0000\u0000\u0000\u02be\u02bd\u0001\u0000\u0000"+
"\u0000\u02bf\t\u0001\u0000\u0000\u0000\u02c0\u02c2\u0005\u011d\u0000\u0000"+
"\u02c1\u02c3\u0005u\u0000\u0000\u02c2\u02c1\u0001\u0000\u0000\u0000\u02c2"+
"\u02c3\u0001\u0000\u0000\u0000\u02c3\u02c4\u0001\u0000\u0000\u0000\u02c4"+
"\u02c5\u0003\f\u0006\u0000\u02c5\u000b\u0001\u0000\u0000\u0000\u02c6\u02c7"+
"\u0005\u0098\u0000\u0000\u02c7\u02c8\u0003\f\u0006\u0000\u02c8\u02c9\u0005"+
"\u00ea\u0000\u0000\u02c9\u02cd\u0001\u0000\u0000\u0000\u02ca\u02cd\u0003"+
"\u0108\u0084\u0000\u02cb\u02cd\u0003\u0270\u0138\u0000\u02cc\u02c6\u0001"+
"\u0000\u0000\u0000\u02cc\u02ca\u0001\u0000\u0000\u0000\u02cc\u02cb\u0001"+
"\u0000\u0000\u0000\u02cd\r\u0001\u0000\u0000\u0000\u02ce\u02cf\u0005k"+
"\u0000\u0000\u02cf\u000f\u0001\u0000\u0000\u0000\u02d0\u02d1\u0005\u00e4"+
"\u0000\u0000\u02d1\u02d2\u0003\u0012\t\u0000\u02d2\u0011\u0001\u0000\u0000"+
"\u0000\u02d3\u02d5\u0005N\u0000\u0000\u02d4\u02d3\u0001\u0000\u0000\u0000"+
"\u02d4\u02d5\u0001\u0000\u0000\u0000\u02d5\u02d6\u0001\u0000\u0000\u0000"+
"\u02d6\u02d8\u0003\u0016\u000b\u0000\u02d7\u02d9\u0003\u001e\u000f\u0000"+
"\u02d8\u02d7\u0001\u0000\u0000\u0000\u02d8\u02d9\u0001\u0000\u0000\u0000"+
"\u02d9\u02db\u0001\u0000\u0000\u0000\u02da\u02dc\u0003 \u0010\u0000\u02db"+
"\u02da\u0001\u0000\u0000\u0000\u02db\u02dc\u0001\u0000\u0000\u0000\u02dc"+
"\u02de\u0001\u0000\u0000\u0000\u02dd\u02df\u0003\"\u0011\u0000\u02de\u02dd"+
"\u0001\u0000\u0000\u0000\u02de\u02df\u0001\u0000\u0000\u0000\u02df\u0013"+
"\u0001\u0000\u0000\u0000\u02e0\u02e3\u0003\u00acV\u0000\u02e1\u02e2\u0005"+
"\u0017\u0000\u0000\u02e2\u02e4\u0003\u0110\u0088\u0000\u02e3\u02e1\u0001"+
"\u0000\u0000\u0000\u02e3\u02e4\u0001\u0000\u0000\u0000\u02e4\u0015\u0001"+
"\u0000\u0000\u0000\u02e5\u02e8\u0005\u010a\u0000\u0000\u02e6\u02e8\u0003"+
"\u0014\n\u0000\u02e7\u02e5\u0001\u0000\u0000\u0000\u02e7\u02e6\u0001\u0000"+
"\u0000\u0000\u02e8\u02ed\u0001\u0000\u0000\u0000\u02e9\u02ea\u0005/\u0000"+
"\u0000\u02ea\u02ec\u0003\u0014\n\u0000\u02eb\u02e9\u0001\u0000\u0000\u0000"+
"\u02ec\u02ef\u0001\u0000\u0000\u0000\u02ed\u02eb\u0001\u0000\u0000\u0000"+
"\u02ed\u02ee\u0001\u0000\u0000\u0000\u02ee\u0017\u0001\u0000\u0000\u0000"+
"\u02ef\u02ed\u0001\u0000\u0000\u0000\u02f0\u02f3\u0003\u00acV\u0000\u02f1"+
"\u02f4\u0003\u001a\r\u0000\u02f2\u02f4\u0003\u001c\u000e\u0000\u02f3\u02f1"+
"\u0001\u0000\u0000\u0000\u02f3\u02f2\u0001\u0000\u0000\u0000\u02f3\u02f4"+
"\u0001\u0000\u0000\u0000\u02f4\u0019\u0001\u0000\u0000\u0000\u02f5\u02f6"+
"\u0007\u0001\u0000\u0000\u02f6\u001b\u0001\u0000\u0000\u0000\u02f7\u02f8"+
"\u0007\u0002\u0000\u0000\u02f8\u001d\u0001\u0000\u0000\u0000\u02f9\u02fa"+
"\u0005\u00bc\u0000\u0000\u02fa\u02fb\u0005&\u0000\u0000\u02fb\u0300\u0003"+
"\u0018\f\u0000\u02fc\u02fd\u0005/\u0000\u0000\u02fd\u02ff\u0003\u0018"+
"\f\u0000\u02fe\u02fc\u0001\u0000\u0000\u0000\u02ff\u0302\u0001\u0000\u0000"+
"\u0000\u0300\u02fe\u0001\u0000\u0000\u0000\u0300\u0301\u0001\u0000\u0000"+
"\u0000\u0301\u001f\u0001\u0000\u0000\u0000\u0302\u0300\u0001\u0000\u0000"+
"\u0000\u0303\u0304\u0007\u0003\u0000\u0000\u0304\u0305\u0003\u00acV\u0000"+
"\u0305!\u0001\u0000\u0000\u0000\u0306\u0307\u0005\u0093\u0000\u0000\u0307"+
"\u0308\u0003\u00acV\u0000\u0308#\u0001\u0000\u0000\u0000\u0309\u030a\u0005"+
"\u0127\u0000\u0000\u030a\u030b\u0003\u00acV\u0000\u030b%\u0001\u0000\u0000"+
"\u0000\u030c\u030d\u0005\u0128\u0000\u0000\u030d\u030f\u0003\u0012\t\u0000"+
"\u030e\u0310\u0003$\u0012\u0000\u030f\u030e\u0001\u0000\u0000\u0000\u030f"+
"\u0310\u0001\u0000\u0000\u0000\u0310\'\u0001\u0000\u0000\u0000\u0311\u0312"+
"\u0005:\u0000\u0000\u0312\u0313\u0003\\.\u0000\u0313)\u0001\u0000\u0000"+
"\u0000\u0314\u0315\u0005\u0085\u0000\u0000\u0315\u0316\u0003^/\u0000\u0316"+
"+\u0001\u0000\u0000\u0000\u0317\u0318\u0005\u00f5\u0000\u0000\u0318\u031d"+
"\u0003.\u0017\u0000\u0319\u031a\u0005/\u0000\u0000\u031a\u031c\u0003."+
"\u0017\u0000\u031b\u0319\u0001\u0000\u0000\u0000\u031c\u031f\u0001\u0000"+
"\u0000\u0000\u031d\u031b\u0001\u0000\u0000\u0000\u031d\u031e\u0001\u0000"+
"\u0000\u0000\u031e-\u0001\u0000\u0000\u0000\u031f\u031d\u0001\u0000\u0000"+
"\u0000\u0320\u0321\u0003\u00ccf\u0000\u0321\u0322\u0005a\u0000\u0000\u0322"+
"\u0323\u0003\u00acV\u0000\u0323\u0337\u0001\u0000\u0000\u0000\u0324\u0325"+
"\u0003\u00ceg\u0000\u0325\u0326\u0005a\u0000\u0000\u0326\u0327\u0003\u00ac"+
"V\u0000\u0327\u0337\u0001\u0000\u0000\u0000\u0328\u0329\u0003\u0110\u0088"+
"\u0000\u0329\u032a\u0005a\u0000\u0000\u032a\u032b\u0003\u00acV\u0000\u032b"+
"\u0337\u0001\u0000\u0000\u0000\u032c\u032d\u0003\u0110\u0088\u0000\u032d"+
"\u032e\u0005\u00c3\u0000\u0000\u032e\u032f\u0003\u00acV\u0000\u032f\u0337"+
"\u0001\u0000\u0000\u0000\u0330\u0331\u0003\u0110\u0088\u0000\u0331\u0332"+
"\u0003z=\u0000\u0332\u0337\u0001\u0000\u0000\u0000\u0333\u0334\u0003\u0110"+
"\u0088\u0000\u0334\u0335\u0003|>\u0000\u0335\u0337\u0001\u0000\u0000\u0000"+
"\u0336\u0320\u0001\u0000\u0000\u0000\u0336\u0324\u0001\u0000\u0000\u0000"+
"\u0336\u0328\u0001\u0000\u0000\u0000\u0336\u032c\u0001\u0000\u0000\u0000"+
"\u0336\u0330\u0001\u0000\u0000\u0000\u0336\u0333\u0001\u0000\u0000\u0000"+
"\u0337/\u0001\u0000\u0000\u0000\u0338\u0339\u0005\u00dd\u0000\u0000\u0339"+
"\u033e\u00032\u0019\u0000\u033a\u033b\u0005/\u0000\u0000\u033b\u033d\u0003"+
"2\u0019\u0000\u033c\u033a\u0001\u0000\u0000\u0000\u033d\u0340\u0001\u0000"+
"\u0000\u0000\u033e\u033c\u0001\u0000\u0000\u0000\u033e\u033f\u0001\u0000"+
"\u0000\u0000\u033f1\u0001\u0000\u0000\u0000\u0340\u033e\u0001\u0000\u0000"+
"\u0000\u0341\u034a\u0003\u00ccf\u0000\u0342\u034a\u0003\u00ceg\u0000\u0343"+
"\u0344\u0003\u0110\u0088\u0000\u0344\u0345\u0003z=\u0000\u0345\u034a\u0001"+
"\u0000\u0000\u0000\u0346\u0347\u0003\u0110\u0088\u0000\u0347\u0348\u0003"+
"|>\u0000\u0348\u034a\u0001\u0000\u0000\u0000\u0349\u0341\u0001\u0000\u0000"+
"\u0000\u0349\u0342\u0001\u0000\u0000\u0000\u0349\u0343\u0001\u0000\u0000"+
"\u0000\u0349\u0346\u0001\u0000\u0000\u0000\u034a3\u0001\u0000\u0000\u0000"+
"\u034b\u034d\u0007\u0004\u0000\u0000\u034c\u034b\u0001\u0000\u0000\u0000"+
"\u034c\u034d\u0001\u0000\u0000\u0000\u034d\u034e\u0001\u0000\u0000\u0000"+
"\u034e\u034f\u0005F\u0000\u0000\u034f\u0354\u0003\u00acV\u0000\u0350\u0351"+
"\u0005/\u0000\u0000\u0351\u0353\u0003\u00acV\u0000\u0352\u0350\u0001\u0000"+
"\u0000\u0000\u0353\u0356\u0001\u0000\u0000\u0000\u0354\u0352\u0001\u0000"+
"\u0000\u0000\u0354\u0355\u0001\u0000\u0000\u0000\u03555\u0001\u0000\u0000"+
"\u0000\u0356\u0354\u0001\u0000\u0000\u0000\u0357\u0359\u0005\u00b8\u0000"+
"\u0000\u0358\u0357\u0001\u0000\u0000\u0000\u0358\u0359\u0001\u0000\u0000"+
"\u0000\u0359\u035a\u0001\u0000\u0000\u0000\u035a\u035c\u0005\u009c\u0000"+
"\u0000\u035b\u035d\u00038\u001c\u0000\u035c\u035b\u0001\u0000\u0000\u0000"+
"\u035c\u035d\u0001\u0000\u0000\u0000\u035d\u035e\u0001\u0000\u0000\u0000"+
"\u035e\u0362\u0003\\.\u0000\u035f\u0361\u0003:\u001d\u0000\u0360\u035f"+
"\u0001\u0000\u0000\u0000\u0361\u0364\u0001\u0000\u0000\u0000\u0362\u0360"+
"\u0001\u0000\u0000\u0000\u0362\u0363\u0001\u0000\u0000\u0000\u0363\u0366"+
"\u0001\u0000\u0000\u0000\u0364\u0362\u0001\u0000\u0000\u0000\u0365\u0367"+
"\u0003$\u0012\u0000\u0366\u0365\u0001\u0000\u0000\u0000\u0366\u0367\u0001"+
"\u0000\u0000\u0000\u03677\u0001\u0000\u0000\u0000\u0368\u036e\u0005\u00de"+
"\u0000\u0000\u0369\u036b\u0005[\u0000\u0000\u036a\u036c\u0005\u001e\u0000"+
"\u0000\u036b\u036a\u0001\u0000\u0000\u0000\u036b\u036c\u0001\u0000\u0000"+
"\u0000\u036c\u036f\u0001\u0000\u0000\u0000\u036d\u036f\u0005\\\u0000\u0000"+
"\u036e\u0369\u0001\u0000\u0000\u0000\u036e\u036d\u0001\u0000\u0000\u0000"+
"\u036f\u0379\u0001\u0000\u0000\u0000\u0370\u0376\u0005L\u0000\u0000\u0371"+
"\u0373\u0005\u00db\u0000\u0000\u0372\u0374\u0005\u001e\u0000\u0000\u0373"+
"\u0372\u0001\u0000\u0000\u0000\u0373\u0374\u0001\u0000\u0000\u0000\u0374"+
"\u0377\u0001\u0000\u0000\u0000\u0375\u0377\u0005\u00dc\u0000\u0000\u0376"+
"\u0371\u0001\u0000\u0000\u0000\u0376\u0375\u0001\u0000\u0000\u0000\u0377"+
"\u0379\u0001\u0000\u0000\u0000\u0378\u0368\u0001\u0000\u0000\u0000\u0378"+
"\u0370\u0001\u0000\u0000\u0000\u03799\u0001\u0000\u0000\u0000\u037a\u0396"+
"\u0005\u0120\u0000\u0000\u037b\u0385\u0005\u0081\u0000\u0000\u037c\u037d"+
"\u0005$\u0000\u0000\u037d\u0385\u0005\u0081\u0000\u0000\u037e\u037f\u0005"+
"\u0107\u0000\u0000\u037f\u0385\u0005\u0081\u0000\u0000\u0380\u0381\u0005"+
"\u00d2\u0000\u0000\u0381\u0385\u0005\u0081\u0000\u0000\u0382\u0383\u0005"+
"\u00c4\u0000\u0000\u0383\u0385\u0005\u0081\u0000\u0000\u0384\u037b\u0001"+
"\u0000\u0000\u0000\u0384\u037c\u0001\u0000\u0000\u0000\u0384\u037e\u0001"+
"\u0000\u0000\u0000\u0384\u0380\u0001\u0000\u0000\u0000\u0384\u0382\u0001"+
"\u0000\u0000\u0000\u0385\u0387\u0001\u0000\u0000\u0000\u0386\u0388\u0005"+
"\u00f1\u0000\u0000\u0387\u0386\u0001\u0000\u0000\u0000\u0387\u0388\u0001"+
"\u0000\u0000\u0000\u0388\u0389\u0001\u0000\u0000\u0000\u0389\u038a\u0003"+
"\u0110\u0088\u0000\u038a\u038b\u0003\u0086C\u0000\u038b\u038c\u0005\u0098"+
"\u0000\u0000\u038c\u038d\u0003\u0112\u0089\u0000\u038d\u038e\u0005\u00ea"+
"\u0000\u0000\u038e\u0397\u0001\u0000\u0000\u0000\u038f\u0390\u0005\u0089"+
"\u0000\u0000\u0390\u0391\u0005\u00b6\u0000\u0000\u0391\u0397\u0003\u0112"+
"\u0089\u0000\u0392\u0393\u0005\u00eb\u0000\u0000\u0393\u0394\u0003\u0110"+
"\u0088\u0000\u0394\u0395\u0003\u0086C\u0000\u0395\u0397\u0001\u0000\u0000"+
"\u0000\u0396\u0384\u0001\u0000\u0000\u0000\u0396\u038f\u0001\u0000\u0000"+
"\u0000\u0396\u0392\u0001\u0000\u0000\u0000\u0397;\u0001\u0000\u0000\u0000"+
"\u0398\u0399\u0005\u009d\u0000\u0000\u0399\u039d\u0003`0\u0000\u039a\u039c"+
"\u0003>\u001f\u0000\u039b\u039a\u0001\u0000\u0000\u0000\u039c\u039f\u0001"+
"\u0000\u0000\u0000\u039d\u039b\u0001\u0000\u0000\u0000\u039d\u039e\u0001"+
"\u0000\u0000\u0000\u039e=\u0001\u0000\u0000\u0000\u039f\u039d\u0001\u0000"+
"\u0000\u0000\u03a0\u03a1\u0005\u00b6\u0000\u0000\u03a1\u03a2\u0007\u0005"+
"\u0000\u0000\u03a2\u03a3\u0003,\u0016\u0000\u03a3?\u0001\u0000\u0000\u0000"+
"\u03a4\u03a5\u0005\u011b\u0000\u0000\u03a5\u03a6\u0003\u00acV\u0000\u03a6"+
"\u03a7\u0005\u0017\u0000\u0000\u03a7\u03a8\u0003\u0110\u0088\u0000\u03a8"+
"A\u0001\u0000\u0000\u0000\u03a9\u03ab\u0005\u00b8\u0000\u0000\u03aa\u03a9"+
"\u0001\u0000\u0000\u0000\u03aa\u03ab\u0001\u0000\u0000\u0000\u03ab\u03ac"+
"\u0001\u0000\u0000\u0000\u03ac\u03ad\u0005\'\u0000\u0000\u03ad\u03ba\u0003"+
"D\"\u0000\u03ae\u03b7\u0005\u0098\u0000\u0000\u03af\u03b4\u0003F#\u0000"+
"\u03b0\u03b1\u0005/\u0000\u0000\u03b1\u03b3\u0003F#\u0000\u03b2\u03b0"+
"\u0001\u0000\u0000\u0000\u03b3\u03b6\u0001\u0000\u0000\u0000\u03b4\u03b2"+
"\u0001\u0000\u0000\u0000\u03b4\u03b5\u0001\u0000\u0000\u0000\u03b5\u03b8"+
"\u0001\u0000\u0000\u0000\u03b6\u03b4\u0001\u0000\u0000\u0000\u03b7\u03af"+
"\u0001\u0000\u0000\u0000\u03b7\u03b8\u0001\u0000\u0000\u0000\u03b8\u03b9"+
"\u0001\u0000\u0000\u0000\u03b9\u03bb\u0005\u00ea\u0000\u0000\u03ba\u03ae"+
"\u0001\u0000\u0000\u0000\u03ba\u03bb\u0001\u0000\u0000\u0000\u03bb\u03cb"+
"\u0001\u0000\u0000\u0000\u03bc\u03c9\u0005\u012c\u0000\u0000\u03bd\u03ca"+
"\u0005\u010a\u0000\u0000\u03be\u03c3\u0003H$\u0000\u03bf\u03c0\u0005/"+
"\u0000\u0000\u03c0\u03c2\u0003H$\u0000\u03c1\u03bf\u0001\u0000\u0000\u0000"+
"\u03c2\u03c5\u0001\u0000\u0000\u0000\u03c3\u03c1\u0001\u0000\u0000\u0000"+
"\u03c3\u03c4\u0001\u0000\u0000\u0000\u03c4\u03c7\u0001\u0000\u0000\u0000"+
"\u03c5\u03c3\u0001\u0000\u0000\u0000\u03c6\u03c8\u0003$\u0012\u0000\u03c7"+
"\u03c6\u0001\u0000\u0000\u0000\u03c7\u03c8\u0001\u0000\u0000\u0000\u03c8"+
"\u03ca\u0001\u0000\u0000\u0000\u03c9\u03bd\u0001\u0000\u0000\u0000\u03c9"+
"\u03be\u0001\u0000\u0000\u0000\u03ca\u03cc\u0001\u0000\u0000\u0000\u03cb"+
"\u03bc\u0001\u0000\u0000\u0000\u03cb\u03cc\u0001\u0000\u0000\u0000\u03cc"+
"C\u0001\u0000\u0000\u0000\u03cd\u03ce\u0003\u010e\u0087\u0000\u03ce\u03cf"+
"\u0003\u0280\u0140\u0000\u03cfE\u0001\u0000\u0000\u0000\u03d0\u03d1\u0003"+
"\u00acV\u0000\u03d1G\u0001\u0000\u0000\u0000\u03d2\u03d5\u0003\u0280\u0140"+
"\u0000\u03d3\u03d4\u0005\u0017\u0000\u0000\u03d4\u03d6\u0003\u0110\u0088"+
"\u0000\u03d5\u03d3\u0001\u0000\u0000\u0000\u03d5\u03d6\u0001\u0000\u0000"+
"\u0000\u03d6I\u0001\u0000\u0000\u0000\u03d7\u03d8\u0005\u0095\u0000\u0000"+
"\u03d8\u03db\u0005;\u0000\u0000\u03d9\u03da\u0005\u0128\u0000\u0000\u03da"+
"\u03dc\u0005z\u0000\u0000\u03db\u03d9\u0001\u0000\u0000\u0000\u03db\u03dc"+
"\u0001\u0000\u0000\u0000\u03dc\u03dd\u0001\u0000\u0000\u0000\u03dd\u03de"+
"\u0005o\u0000\u0000\u03de\u03df\u0003\u00acV\u0000\u03df\u03e0\u0005\u0017"+
"\u0000\u0000\u03e0\u03e3\u0003\u0110\u0088\u0000\u03e1\u03e2\u0005j\u0000"+
"\u0000\u03e2\u03e4\u0003\u0276\u013b\u0000\u03e3\u03e1\u0001\u0000\u0000"+
"\u0000\u03e3\u03e4\u0001\u0000\u0000\u0000\u03e4K\u0001\u0000\u0000\u0000"+
"\u03e5\u03e6\u0005n\u0000\u0000\u03e6\u03e7\u0005\u0098\u0000\u0000\u03e7"+
"\u03e8\u0003\u0110\u0088\u0000\u03e8\u03e9\u0005\u0080\u0000\u0000\u03e9"+
"\u03ea\u0003\u00acV\u0000\u03ea\u03ec\u0005\u001d\u0000\u0000\u03eb\u03ed"+
"\u0003\b\u0004\u0000\u03ec\u03eb\u0001\u0000\u0000\u0000\u03ed\u03ee\u0001"+
"\u0000\u0000\u0000\u03ee\u03ec\u0001\u0000\u0000\u0000\u03ee\u03ef\u0001"+
"\u0000\u0000\u0000\u03ef\u03f0\u0001\u0000\u0000\u0000\u03f0\u03f1\u0005"+
"\u00ea\u0000\u0000\u03f1M\u0001\u0000\u0000\u0000\u03f2\u03f4\u0005\u00b8"+
"\u0000\u0000\u03f3\u03f2\u0001\u0000\u0000\u0000\u03f3\u03f4\u0001\u0000"+
"\u0000\u0000\u03f4\u03f5\u0001\u0000\u0000\u0000\u03f5\u03f7\u0005\'\u0000"+
"\u0000\u03f6\u03f8\u0003P(\u0000\u03f7\u03f6\u0001\u0000\u0000\u0000\u03f7"+
"\u03f8\u0001\u0000\u0000\u0000\u03f8\u03f9\u0001\u0000\u0000\u0000\u03f9"+
"\u03fa\u0005\u0090\u0000\u0000\u03fa\u03fb\u0003\u0004\u0002\u0000\u03fb"+
"\u03fd\u0005\u00d4\u0000\u0000\u03fc\u03fe\u0003R)\u0000\u03fd\u03fc\u0001"+
"\u0000\u0000\u0000\u03fd\u03fe\u0001\u0000\u0000\u0000\u03feO\u0001\u0000"+
"\u0000\u0000\u03ff\u0409\u0005\u0098\u0000\u0000\u0400\u040a\u0005\u010a"+
"\u0000\u0000\u0401\u0406\u0003\u0110\u0088\u0000\u0402\u0403\u0005/\u0000"+
"\u0000\u0403\u0405\u0003\u0110\u0088\u0000\u0404\u0402\u0001\u0000\u0000"+
"\u0000\u0405\u0408\u0001\u0000\u0000\u0000\u0406\u0404\u0001\u0000\u0000"+
"\u0000\u0406\u0407\u0001\u0000\u0000\u0000\u0407\u040a\u0001\u0000\u0000"+
"\u0000\u0408\u0406\u0001\u0000\u0000\u0000\u0409\u0400\u0001\u0000\u0000"+
"\u0000\u0409\u0401\u0001\u0000\u0000\u0000\u0409\u040a\u0001\u0000\u0000"+
"\u0000\u040a\u040b\u0001\u0000\u0000\u0000\u040b\u040c\u0005\u00ea\u0000"+
"\u0000\u040cQ\u0001\u0000\u0000\u0000\u040d\u0412\u0005\u0080\u0000\u0000"+
"\u040e\u0410\u0003\u00acV\u0000\u040f\u040e\u0001\u0000\u0000\u0000\u040f"+
"\u0410\u0001\u0000\u0000\u0000\u0410\u0411\u0001\u0000\u0000\u0000\u0411"+
"\u0413\u00053\u0000\u0000\u0412\u040f\u0001\u0000\u0000\u0000\u0412\u0413"+
"\u0001\u0000\u0000\u0000\u0413\u0414\u0001\u0000\u0000\u0000\u0414\u041a"+
"\u0005\u0111\u0000\u0000\u0415\u0419\u0003T*\u0000\u0416\u0419\u0003V"+
"+\u0000\u0417\u0419\u0003X,\u0000\u0418\u0415\u0001\u0000\u0000\u0000"+
"\u0418\u0416\u0001\u0000\u0000\u0000\u0418\u0417\u0001\u0000\u0000\u0000"+
"\u0419\u041c\u0001\u0000\u0000\u0000\u041a\u0418\u0001\u0000\u0000\u0000"+
"\u041a\u041b\u0001\u0000\u0000\u0000\u041bS\u0001\u0000\u0000\u0000\u041c"+
"\u041a\u0001\u0000\u0000\u0000\u041d\u041e\u0005\u00b4\u0000\u0000\u041e"+
"\u041f\u0003\u00acV\u0000\u041f\u0420\u0007\u0006\u0000\u0000\u0420U\u0001"+
"\u0000\u0000\u0000\u0421\u0422\u0005\u00b6\u0000\u0000\u0422\u0423\u0005"+
"g\u0000\u0000\u0423\u0424\u0007\u0007\u0000\u0000\u0424W\u0001\u0000\u0000"+
"\u0000\u0425\u0426\u0005\u00e0\u0000\u0000\u0426\u0427\u0005\u0100\u0000"+
"\u0000\u0427\u0428\u0005\u0017\u0000\u0000\u0428\u0429\u0003\u0110\u0088"+
"\u0000\u0429Y\u0001\u0000\u0000\u0000\u042a\u042c\u0003\u001e\u000f\u0000"+
"\u042b\u042d\u0003 \u0010\u0000\u042c\u042b\u0001\u0000\u0000\u0000\u042c"+
"\u042d\u0001\u0000\u0000\u0000\u042d\u042f\u0001\u0000\u0000\u0000\u042e"+
"\u0430\u0003\"\u0011\u0000\u042f\u042e\u0001\u0000\u0000\u0000\u042f\u0430"+
"\u0001\u0000\u0000\u0000\u0430\u0437\u0001\u0000\u0000\u0000\u0431\u0433"+
"\u0003 \u0010\u0000\u0432\u0434\u0003\"\u0011\u0000\u0433\u0432\u0001"+
"\u0000\u0000\u0000\u0433\u0434\u0001\u0000\u0000\u0000\u0434\u0437\u0001"+
"\u0000\u0000\u0000\u0435\u0437\u0003\"\u0011\u0000\u0436\u042a\u0001\u0000"+
"\u0000\u0000\u0436\u0431\u0001\u0000\u0000\u0000\u0436\u0435\u0001\u0000"+
"\u0000\u0000\u0437[\u0001\u0000\u0000\u0000\u0438\u043d\u0003`0\u0000"+
"\u0439\u043a\u0005/\u0000\u0000\u043a\u043c\u0003`0\u0000\u043b\u0439"+
"\u0001\u0000\u0000\u0000\u043c\u043f\u0001\u0000\u0000\u0000\u043d\u043b"+
"\u0001\u0000\u0000\u0000\u043d\u043e\u0001\u0000\u0000\u0000\u043e]\u0001"+
"\u0000\u0000\u0000\u043f\u043d\u0001\u0000\u0000\u0000\u0440\u0445\u0003"+
"b1\u0000\u0441\u0442\u0005/\u0000\u0000\u0442\u0444\u0003b1\u0000\u0443"+
"\u0441\u0001\u0000\u0000\u0000\u0444\u0447\u0001\u0000\u0000\u0000\u0445"+
"\u0443\u0001\u0000\u0000\u0000\u0445\u0446\u0001\u0000\u0000\u0000\u0446"+
"_\u0001\u0000\u0000\u0000\u0447\u0445\u0001\u0000\u0000\u0000\u0448\u0449"+
"\u0003\u0110\u0088\u0000\u0449\u044a\u0005a\u0000\u0000\u044a\u044c\u0001"+
"\u0000\u0000\u0000\u044b\u0448\u0001\u0000\u0000\u0000\u044b\u044c\u0001"+
"\u0000\u0000\u0000\u044c\u044e\u0001\u0000\u0000\u0000\u044d\u044f\u0003"+
"l6\u0000\u044e\u044d\u0001\u0000\u0000\u0000\u044e\u044f\u0001\u0000\u0000"+
"\u0000\u044f\u0450\u0001\u0000\u0000\u0000\u0450\u0451\u0003f3\u0000\u0451"+
"a\u0001\u0000\u0000\u0000\u0452\u0453\u0003\u0280\u0140\u0000\u0453\u0454"+
"\u0005a\u0000\u0000\u0454\u0456\u0001\u0000\u0000\u0000\u0455\u0452\u0001"+
"\u0000\u0000\u0000\u0455\u0456\u0001\u0000\u0000\u0000\u0456\u0457\u0001"+
"\u0000\u0000\u0000\u0457\u045d\u0003v;\u0000\u0458\u0459\u0003\u008cF"+
"\u0000\u0459\u045a\u0003v;\u0000\u045a\u045c\u0001\u0000\u0000\u0000\u045b"+
"\u0458\u0001\u0000\u0000\u0000\u045c\u045f\u0001\u0000\u0000\u0000\u045d"+
"\u045b\u0001\u0000\u0000\u0000\u045d\u045e\u0001\u0000\u0000\u0000\u045e"+
"c\u0001\u0000\u0000\u0000\u045f\u045d\u0001\u0000\u0000\u0000\u0460\u0461"+
"\u0005\u0090\u0000\u0000\u0461\u0462\u0005\u0005\u0000\u0000\u0462\u046f"+
"\u0005\u00d4\u0000\u0000\u0463\u0465\u0005\u0090\u0000\u0000\u0464\u0466"+
"\u0005\u0005\u0000\u0000\u0465\u0464\u0001\u0000\u0000\u0000\u0465\u0466"+
"\u0001\u0000\u0000\u0000\u0466\u0467\u0001\u0000\u0000\u0000\u0467\u0469"+
"\u0005/\u0000\u0000\u0468\u046a\u0005\u0005\u0000\u0000\u0469\u0468\u0001"+
"\u0000\u0000\u0000\u0469\u046a\u0001\u0000\u0000\u0000\u046a\u046b\u0001"+
"\u0000\u0000\u0000\u046b\u046f\u0005\u00d4\u0000\u0000\u046c\u046f\u0005"+
"\u00c2\u0000\u0000\u046d\u046f\u0005\u010a\u0000\u0000\u046e\u0460\u0001"+
"\u0000\u0000\u0000\u046e\u0463\u0001\u0000\u0000\u0000\u046e\u046c\u0001"+
"\u0000\u0000\u0000\u046e\u046d\u0001\u0000\u0000\u0000\u046fe\u0001\u0000"+
"\u0000\u0000\u0470\u0473\u0003h4\u0000\u0471\u0473\u0003j5\u0000\u0472"+
"\u0470\u0001\u0000\u0000\u0000\u0472\u0471\u0001\u0000\u0000\u0000\u0473"+
"g\u0001\u0000\u0000\u0000\u0474\u0475\u0007\b\u0000\u0000\u0475\u0476"+
"\u0005\u0098\u0000\u0000\u0476\u0477\u0003j5\u0000\u0477\u0478\u0005\u00ea"+
"\u0000\u0000\u0478i\u0001\u0000\u0000\u0000\u0479\u0482\u0003t:\u0000"+
"\u047a\u047c\u0003\u008aE\u0000\u047b\u047d\u0003d2\u0000\u047c\u047b"+
"\u0001\u0000\u0000\u0000\u047c\u047d\u0001\u0000\u0000\u0000\u047d\u047e"+
"\u0001\u0000\u0000\u0000\u047e\u047f\u0003t:\u0000\u047f\u0481\u0001\u0000"+
"\u0000\u0000\u0480\u047a\u0001\u0000\u0000\u0000\u0481\u0484\u0001\u0000"+
"\u0000\u0000\u0482\u0480\u0001\u0000\u0000\u0000\u0482\u0483\u0001\u0000"+
"\u0000\u0000\u0483\u0487\u0001\u0000\u0000\u0000\u0484\u0482\u0001\u0000"+
"\u0000\u0000\u0485\u0487\u0003x<\u0000\u0486\u0479\u0001\u0000\u0000\u0000"+
"\u0486\u0485\u0001\u0000\u0000\u0000\u0487\u0488\u0001\u0000\u0000\u0000"+
"\u0488\u0486\u0001\u0000\u0000\u0000\u0488\u0489\u0001\u0000\u0000\u0000"+
"\u0489k\u0001\u0000\u0000\u0000\u048a\u048b\u0005\u0015\u0000\u0000\u048b"+
"\u048d\u0005\u00f9\u0000\u0000\u048c\u048e\u0003p8\u0000\u048d\u048c\u0001"+
"\u0000\u0000\u0000\u048d\u048e\u0001\u0000\u0000\u0000\u048e\u04ad\u0001"+
"\u0000\u0000\u0000\u048f\u0490\u0005\u0012\u0000\u0000\u0490\u0492\u0005"+
"\u00f9\u0000\u0000\u0491\u0493\u0003p8\u0000\u0492\u0491\u0001\u0000\u0000"+
"\u0000\u0492\u0493\u0001\u0000\u0000\u0000\u0493\u04ad\u0001\u0000\u0000"+
"\u0000\u0494\u0496\u0005\u0015\u0000\u0000\u0495\u0497\u0005\u0005\u0000"+
"\u0000\u0496\u0495\u0001\u0000\u0000\u0000\u0496\u0497\u0001\u0000\u0000"+
"\u0000\u0497\u0499\u0001\u0000\u0000\u0000\u0498\u049a\u0003p8\u0000\u0499"+
"\u0498\u0001\u0000\u0000\u0000\u0499\u049a\u0001\u0000\u0000\u0000\u049a"+
"\u04ad\u0001\u0000\u0000\u0000\u049b\u049d\u0005\u0012\u0000\u0000\u049c"+
"\u049e\u0003p8\u0000\u049d\u049c\u0001\u0000\u0000\u0000\u049d\u049e\u0001"+
"\u0000\u0000\u0000\u049e\u04ad\u0001\u0000\u0000\u0000\u049f\u04a1\u0005"+
"\u00f9\u0000\u0000\u04a0\u04a2\u0005\u0005\u0000\u0000\u04a1\u04a0\u0001"+
"\u0000\u0000\u0000\u04a1\u04a2\u0001\u0000\u0000\u0000\u04a2\u04a4\u0001"+
"\u0000\u0000\u0000\u04a3\u04a5\u0003p8\u0000\u04a4\u04a3\u0001\u0000\u0000"+
"\u0000\u04a4\u04a5\u0001\u0000\u0000\u0000\u04a5\u04a6\u0001\u0000\u0000"+
"\u0000\u04a6\u04ad\u0003n7\u0000\u04a7\u04a8\u0005\u00f9\u0000\u0000\u04a8"+
"\u04aa\u0005\u0005\u0000\u0000\u04a9\u04ab\u0003p8\u0000\u04aa\u04a9\u0001"+
"\u0000\u0000\u0000\u04aa\u04ab\u0001\u0000\u0000\u0000\u04ab\u04ad\u0001"+
"\u0000\u0000\u0000\u04ac\u048a\u0001\u0000\u0000\u0000\u04ac\u048f\u0001"+
"\u0000\u0000\u0000\u04ac\u0494\u0001\u0000\u0000\u0000\u04ac\u049b\u0001"+
"\u0000\u0000\u0000\u04ac\u049f\u0001\u0000\u0000\u0000\u04ac\u04a7\u0001"+
"\u0000\u0000\u0000\u04adm\u0001\u0000\u0000\u0000\u04ae\u04af\u0007\t"+
"\u0000\u0000\u04afo\u0001\u0000\u0000\u0000\u04b0\u04b1\u0007\n\u0000"+
"\u0000\u04b1q\u0001\u0000\u0000\u0000\u04b2\u04b6\u0003t:\u0000\u04b3"+
"\u04b4\u0003\u008aE\u0000\u04b4\u04b5\u0003t:\u0000\u04b5\u04b7\u0001"+
"\u0000\u0000\u0000\u04b6\u04b3\u0001\u0000\u0000\u0000\u04b7\u04b8\u0001"+
"\u0000\u0000\u0000\u04b8\u04b6\u0001\u0000\u0000\u0000\u04b8\u04b9\u0001"+
"\u0000\u0000\u0000\u04b9s\u0001\u0000\u0000\u0000\u04ba\u04bc\u0005\u0098"+
"\u0000\u0000\u04bb\u04bd\u0003\u0110\u0088\u0000\u04bc\u04bb\u0001\u0000"+
"\u0000\u0000\u04bc\u04bd\u0001\u0000\u0000\u0000\u04bd\u04bf\u0001\u0000"+
"\u0000\u0000\u04be\u04c0\u0003\u0096K\u0000\u04bf\u04be\u0001\u0000\u0000"+
"\u0000\u04bf\u04c0\u0001\u0000\u0000\u0000\u04c0\u04c2\u0001\u0000\u0000"+
"\u0000\u04c1\u04c3\u0003\u0088D\u0000\u04c2\u04c1\u0001\u0000\u0000\u0000"+
"\u04c2\u04c3\u0001\u0000\u0000\u0000\u04c3\u04c6\u0001\u0000\u0000\u0000"+
"\u04c4\u04c5\u0005\u0127\u0000\u0000\u04c5\u04c7\u0003\u00acV\u0000\u04c6"+
"\u04c4\u0001\u0000\u0000\u0000\u04c6\u04c7\u0001\u0000\u0000\u0000\u04c7"+
"\u04c8\u0001\u0000\u0000\u0000\u04c8\u04c9\u0005\u00ea\u0000\u0000\u04c9"+
"u\u0001\u0000\u0000\u0000\u04ca\u04cc\u0005\u0098\u0000\u0000\u04cb\u04cd"+
"\u0003\u0110\u0088\u0000\u04cc\u04cb\u0001\u0000\u0000\u0000\u04cc\u04cd"+
"\u0001\u0000\u0000\u0000\u04cd\u04cf\u0001\u0000\u0000\u0000\u04ce\u04d0"+
"\u0003\u00a8T\u0000\u04cf\u04ce\u0001\u0000\u0000\u0000\u04cf\u04d0\u0001"+
"\u0000\u0000\u0000\u04d0\u04d2\u0001\u0000\u0000\u0000\u04d1\u04d3\u0003"+
"\u027e\u013f\u0000\u04d2\u04d1\u0001\u0000\u0000\u0000\u04d2\u04d3\u0001"+
"\u0000\u0000\u0000\u04d3\u04d4\u0001\u0000\u0000\u0000\u04d4\u04d5\u0005"+
"\u00ea\u0000\u0000\u04d5w\u0001\u0000\u0000\u0000\u04d6\u04d7\u0005\u0098"+
"\u0000\u0000\u04d7\u04da\u0003`0\u0000\u04d8\u04d9\u0005\u0127\u0000\u0000"+
"\u04d9\u04db\u0003\u00acV\u0000\u04da\u04d8\u0001\u0000\u0000\u0000\u04da"+
"\u04db\u0001\u0000\u0000\u0000\u04db\u04dc\u0001\u0000\u0000\u0000\u04dc"+
"\u04de\u0005\u00ea\u0000\u0000\u04dd\u04df\u0003d2\u0000\u04de\u04dd\u0001"+
"\u0000\u0000\u0000\u04de\u04df\u0001\u0000\u0000\u0000\u04dfy\u0001\u0000"+
"\u0000\u0000\u04e0\u04e3\u0003\u0082A\u0000\u04e1\u04e3\u0003\u0080@\u0000"+
"\u04e2\u04e0\u0001\u0000\u0000\u0000\u04e2\u04e1\u0001\u0000\u0000\u0000"+
"\u04e3\u04e4\u0001\u0000\u0000\u0000\u04e4\u04e2\u0001\u0000\u0000\u0000"+
"\u04e4\u04e5\u0001\u0000\u0000\u0000\u04e5{\u0001\u0000\u0000\u0000\u04e6"+
"\u04e9\u0005\u0088\u0000\u0000\u04e7\u04ea\u0003\u0280\u0140\u0000\u04e8"+
"\u04ea\u0003~?\u0000\u04e9\u04e7\u0001\u0000\u0000\u0000\u04e9\u04e8\u0001"+
"\u0000\u0000\u0000\u04ea\u04ef\u0001\u0000\u0000\u0000\u04eb\u04ee\u0003"+
"\u0082A\u0000\u04ec\u04ee\u0003\u0080@\u0000\u04ed\u04eb\u0001\u0000\u0000"+
"\u0000\u04ed\u04ec\u0001\u0000\u0000\u0000\u04ee\u04f1\u0001\u0000\u0000"+
"\u0000\u04ef\u04ed\u0001\u0000\u0000\u0000\u04ef\u04f0\u0001\u0000\u0000"+
"\u0000\u04f0}\u0001\u0000\u0000\u0000\u04f1\u04ef\u0001\u0000\u0000\u0000"+
"\u04f2\u04f3\u0005M\u0000\u0000\u04f3\u04f4\u0005\u0098\u0000\u0000\u04f4"+
"\u04f5\u0003\u00acV\u0000\u04f5\u04f6\u0005\u00ea\u0000\u0000\u04f6\u007f"+
"\u0001\u0000\u0000\u0000\u04f7\u04f8\u0005-\u0000\u0000\u04f8\u04f9\u0003"+
"~?\u0000\u04f9\u0081\u0001\u0000\u0000\u0000\u04fa\u04fb\u0005-\u0000"+
"\u0000\u04fb\u04fc\u0003\u0280\u0140\u0000\u04fc\u0083\u0001\u0000\u0000"+
"\u0000\u04fd\u04fe\u0005-\u0000\u0000\u04fe\u04ff\u0003\u0280\u0140\u0000"+
"\u04ff\u0085\u0001\u0000\u0000\u0000\u0500\u0501\u0005-\u0000\u0000\u0501"+
"\u0502\u0003\u0280\u0140\u0000\u0502\u0087\u0001\u0000\u0000\u0000\u0503"+
"\u0506\u0003\u027e\u013f\u0000\u0504\u0506\u0003\u0104\u0082\u0000\u0505"+
"\u0503\u0001\u0000\u0000\u0000\u0505\u0504\u0001\u0000\u0000\u0000\u0506"+
"\u0089\u0001\u0000\u0000\u0000\u0507\u0509\u0003\u008eG\u0000\u0508\u0507"+
"\u0001\u0000\u0000\u0000\u0508\u0509\u0001\u0000\u0000\u0000\u0509\u050a"+
"\u0001\u0000\u0000\u0000\u050a\u051d\u0003\u0090H\u0000\u050b\u050d\u0005"+
"\u008f\u0000\u0000\u050c\u050e\u0003\u0110\u0088\u0000\u050d\u050c\u0001"+
"\u0000\u0000\u0000\u050d\u050e\u0001\u0000\u0000\u0000\u050e\u0510\u0001"+
"\u0000\u0000\u0000\u050f\u0511\u0003\u0096K\u0000\u0510\u050f\u0001\u0000"+
"\u0000\u0000\u0510\u0511\u0001\u0000\u0000\u0000\u0511\u0513\u0001\u0000"+
"\u0000\u0000\u0512\u0514\u0003\u0094J\u0000\u0513\u0512\u0001\u0000\u0000"+
"\u0000\u0513\u0514\u0001\u0000\u0000\u0000\u0514\u0516\u0001\u0000\u0000"+
"\u0000\u0515\u0517\u0003\u0088D\u0000\u0516\u0515\u0001\u0000\u0000\u0000"+
"\u0516\u0517\u0001\u0000\u0000\u0000\u0517\u051a\u0001\u0000\u0000\u0000"+
"\u0518\u0519\u0005\u0127\u0000\u0000\u0519\u051b\u0003\u00acV\u0000\u051a"+
"\u0518\u0001\u0000\u0000\u0000\u051a\u051b\u0001\u0000\u0000\u0000\u051b"+
"\u051c\u0001\u0000\u0000\u0000\u051c\u051e\u0005\u00d3\u0000\u0000\u051d"+
"\u050b\u0001\u0000\u0000\u0000\u051d\u051e\u0001\u0000\u0000\u0000\u051e"+
"\u051f\u0001\u0000\u0000\u0000\u051f\u0521\u0003\u0090H\u0000\u0520\u0522"+
"\u0003\u0092I\u0000\u0521\u0520\u0001\u0000\u0000\u0000\u0521\u0522\u0001"+
"\u0000\u0000\u0000\u0522\u008b\u0001\u0000\u0000\u0000\u0523\u0525\u0003"+
"\u008eG\u0000\u0524\u0523\u0001\u0000\u0000\u0000\u0524\u0525\u0001\u0000"+
"\u0000\u0000\u0525\u0526\u0001\u0000\u0000\u0000\u0526\u0527\u0003\u0090"+
"H\u0000\u0527\u0529\u0005\u008f\u0000\u0000\u0528\u052a\u0003\u0110\u0088"+
"\u0000\u0529\u0528\u0001\u0000\u0000\u0000\u0529\u052a\u0001\u0000\u0000"+
"\u0000\u052a\u052b\u0001\u0000\u0000\u0000\u052b\u052d\u0003\u00aaU\u0000"+
"\u052c\u052e\u0003\u027e\u013f\u0000\u052d\u052c\u0001\u0000\u0000\u0000"+
"\u052d\u052e\u0001\u0000\u0000\u0000\u052e\u052f\u0001\u0000\u0000\u0000"+
"\u052f\u0530\u0005\u00d3\u0000\u0000\u0530\u0532\u0003\u0090H\u0000\u0531"+
"\u0533\u0003\u0092I\u0000\u0532\u0531\u0001\u0000\u0000\u0000\u0532\u0533"+
"\u0001\u0000\u0000\u0000\u0533\u008d\u0001\u0000\u0000\u0000\u0534\u0535"+
"\u0007\u000b\u0000\u0000\u0535\u008f\u0001\u0000\u0000\u0000\u0536\u0537"+
"\u0007\f\u0000\u0000\u0537\u0091\u0001\u0000\u0000\u0000\u0538\u0539\u0007"+
"\r\u0000\u0000\u0539\u0093\u0001\u0000\u0000\u0000\u053a\u0543\u0005\u010a"+
"\u0000\u0000\u053b\u053d\u0005\u0005\u0000\u0000\u053c\u053b\u0001\u0000"+
"\u0000\u0000\u053c\u053d\u0001\u0000\u0000\u0000\u053d\u053e\u0001\u0000"+
"\u0000\u0000\u053e\u0540\u0005Q\u0000\u0000\u053f\u0541\u0005\u0005\u0000"+
"\u0000\u0540\u053f\u0001\u0000\u0000\u0000\u0540\u0541\u0001\u0000\u0000"+
"\u0000\u0541\u0544\u0001\u0000\u0000\u0000\u0542\u0544\u0005\u0005\u0000"+
"\u0000\u0543\u053c\u0001\u0000\u0000\u0000\u0543\u0542\u0001\u0000\u0000"+
"\u0000\u0543\u0544\u0001\u0000\u0000\u0000\u0544\u0095\u0001\u0000\u0000"+
"\u0000\u0545\u0546\u0005-\u0000\u0000\u0546\u054a\u0003\u0098L\u0000\u0547"+
"\u0548\u0005\u0088\u0000\u0000\u0548\u054a\u0003\u009aM\u0000\u0549\u0545"+
"\u0001\u0000\u0000\u0000\u0549\u0547\u0001\u0000\u0000\u0000\u054a\u0097"+
"\u0001\u0000\u0000\u0000\u054b\u0553\u0003\u009cN\u0000\u054c\u054e\u0005"+
"\u001d\u0000\u0000\u054d\u054f\u0005-\u0000\u0000\u054e\u054d\u0001\u0000"+
"\u0000\u0000\u054e\u054f\u0001\u0000\u0000\u0000\u054f\u0550\u0001\u0000"+
"\u0000\u0000\u0550\u0552\u0003\u009cN\u0000\u0551\u054c\u0001\u0000\u0000"+
"\u0000\u0552\u0555\u0001\u0000\u0000\u0000\u0553\u0551\u0001\u0000\u0000"+
"\u0000\u0553\u0554\u0001\u0000\u0000\u0000\u0554\u0099\u0001\u0000\u0000"+
"\u0000\u0555\u0553\u0001\u0000\u0000\u0000\u0556\u055e\u0003\u009eO\u0000"+
"\u0557\u0559\u0005\u001d\u0000\u0000\u0558\u055a\u0005-\u0000\u0000\u0559"+
"\u0558\u0001\u0000\u0000\u0000\u0559\u055a\u0001\u0000\u0000\u0000\u055a"+
"\u055b\u0001\u0000\u0000\u0000\u055b\u055d\u0003\u009eO\u0000\u055c\u0557"+
"\u0001\u0000\u0000\u0000\u055d\u0560\u0001\u0000\u0000\u0000\u055e\u055c"+
"\u0001\u0000\u0000\u0000\u055e\u055f\u0001\u0000\u0000\u0000\u055f\u009b"+
"\u0001\u0000\u0000\u0000\u0560\u055e\u0001\u0000\u0000\u0000\u0561\u0566"+
"\u0003\u00a0P\u0000\u0562\u0563\u0007\u000e\u0000\u0000\u0563\u0565\u0003"+
"\u00a0P\u0000\u0564\u0562\u0001\u0000\u0000\u0000\u0565\u0568\u0001\u0000"+
"\u0000\u0000\u0566\u0564\u0001\u0000\u0000\u0000\u0566\u0567\u0001\u0000"+
"\u0000\u0000\u0567\u009d\u0001\u0000\u0000\u0000\u0568\u0566\u0001\u0000"+
"\u0000\u0000\u0569\u056e\u0003\u00a2Q\u0000\u056a\u056b\u0007\u000e\u0000"+
"\u0000\u056b\u056d\u0003\u00a2Q\u0000\u056c\u056a\u0001\u0000\u0000\u0000"+
"\u056d\u0570\u0001\u0000\u0000\u0000\u056e\u056c\u0001\u0000\u0000\u0000"+
"\u056e\u056f\u0001\u0000\u0000\u0000\u056f\u009f\u0001\u0000\u0000\u0000"+
"\u0570\u056e\u0001\u0000\u0000\u0000\u0571\u0573\u0005\u008e\u0000\u0000"+
"\u0572\u0571\u0001\u0000\u0000\u0000\u0573\u0576\u0001\u0000\u0000\u0000"+
"\u0574\u0572\u0001\u0000\u0000\u0000\u0574\u0575\u0001\u0000\u0000\u0000"+
"\u0575\u0577\u0001\u0000\u0000\u0000\u0576\u0574\u0001\u0000\u0000\u0000"+
"\u0577\u0578\u0003\u00a4R\u0000\u0578\u00a1\u0001\u0000\u0000\u0000\u0579"+
"\u057b\u0005\u008e\u0000\u0000\u057a\u0579\u0001\u0000\u0000\u0000\u057b"+
"\u057e\u0001\u0000\u0000\u0000\u057c\u057a\u0001\u0000\u0000\u0000\u057c"+
"\u057d\u0001\u0000\u0000\u0000\u057d\u057f\u0001\u0000\u0000\u0000\u057e"+
"\u057c\u0001\u0000\u0000\u0000\u057f\u0580\u0003\u00a6S\u0000\u0580\u00a3"+
"\u0001\u0000\u0000\u0000\u0581\u0582\u0005\u0098\u0000\u0000\u0582\u0583"+
"\u0003\u0098L\u0000\u0583\u0584\u0005\u00ea\u0000\u0000\u0584\u0588\u0001"+
"\u0000\u0000\u0000\u0585\u0588\u0005\u009f\u0000\u0000\u0586\u0588\u0003"+
"\u0280\u0140\u0000\u0587\u0581\u0001\u0000\u0000\u0000\u0587\u0585\u0001"+
"\u0000\u0000\u0000\u0587\u0586\u0001\u0000\u0000\u0000\u0588\u00a5\u0001"+
"\u0000\u0000\u0000\u0589\u058a\u0005\u0098\u0000\u0000\u058a\u058b\u0003"+
"\u009aM\u0000\u058b\u058c\u0005\u00ea\u0000\u0000\u058c\u0590\u0001\u0000"+
"\u0000\u0000\u058d\u0590\u0005\u009f\u0000\u0000\u058e\u0590\u0003\u0286"+
"\u0143\u0000\u058f\u0589\u0001\u0000\u0000\u0000\u058f\u058d\u0001\u0000"+
"\u0000\u0000\u058f\u058e\u0001\u0000\u0000\u0000\u0590\u00a7\u0001\u0000"+
"\u0000\u0000\u0591\u0592\u0007\u000f\u0000\u0000\u0592\u0597\u0003\u0280"+
"\u0140\u0000\u0593\u0594\u0007\u000e\u0000\u0000\u0594\u0596\u0003\u0280"+
"\u0140\u0000\u0595\u0593\u0001\u0000\u0000\u0000\u0596\u0599\u0001\u0000"+
"\u0000\u0000\u0597\u0595\u0001\u0000\u0000\u0000\u0597\u0598\u0001\u0000"+
"\u0000\u0000\u0598\u00a9\u0001\u0000\u0000\u0000\u0599\u0597\u0001\u0000"+
"\u0000\u0000\u059a\u059b\u0007\u000f\u0000\u0000\u059b\u059c\u0003\u0280"+
"\u0140\u0000\u059c\u00ab\u0001\u0000\u0000\u0000\u059d\u05a2\u0003\u00ae"+
"W\u0000\u059e\u059f\u0005\u00bb\u0000\u0000\u059f\u05a1\u0003\u00aeW\u0000"+
"\u05a0\u059e\u0001\u0000\u0000\u0000\u05a1\u05a4\u0001\u0000\u0000\u0000"+
"\u05a2\u05a0\u0001\u0000\u0000\u0000\u05a2\u05a3\u0001\u0000\u0000\u0000"+
"\u05a3\u00ad\u0001\u0000\u0000\u0000\u05a4\u05a2\u0001\u0000\u0000\u0000"+
"\u05a5\u05aa\u0003\u00b0X\u0000\u05a6\u05a7\u0005\u012b\u0000\u0000\u05a7"+
"\u05a9\u0003\u00b0X\u0000\u05a8\u05a6\u0001\u0000\u0000\u0000\u05a9\u05ac"+
"\u0001\u0000\u0000\u0000\u05aa\u05a8\u0001\u0000\u0000\u0000\u05aa\u05ab"+
"\u0001\u0000\u0000\u0000\u05ab\u00af\u0001\u0000\u0000\u0000\u05ac\u05aa"+
"\u0001\u0000\u0000\u0000\u05ad\u05b2\u0003\u00b2Y\u0000\u05ae\u05af\u0005"+
"\u0014\u0000\u0000\u05af\u05b1\u0003\u00b2Y\u0000\u05b0\u05ae\u0001\u0000"+
"\u0000\u0000\u05b1\u05b4\u0001\u0000\u0000\u0000\u05b2\u05b0\u0001\u0000"+
"\u0000\u0000\u05b2\u05b3\u0001\u0000\u0000\u0000\u05b3\u00b1\u0001\u0000"+
"\u0000\u0000\u05b4\u05b2\u0001\u0000\u0000\u0000\u05b5\u05b7\u0005\u00b0"+
"\u0000\u0000\u05b6\u05b5\u0001\u0000\u0000\u0000\u05b7\u05ba\u0001\u0000"+
"\u0000\u0000\u05b8\u05b6\u0001\u0000\u0000\u0000\u05b8\u05b9\u0001\u0000"+
"\u0000\u0000\u05b9\u05bb\u0001\u0000\u0000\u0000\u05ba\u05b8\u0001\u0000"+
"\u0000\u0000\u05bb\u05bc\u0003\u00b4Z\u0000\u05bc\u00b3\u0001\u0000\u0000"+
"\u0000\u05bd\u05c2\u0003\u00b6[\u0000\u05be\u05bf\u0007\u0010\u0000\u0000"+
"\u05bf\u05c1\u0003\u00b6[\u0000\u05c0\u05be\u0001\u0000\u0000\u0000\u05c1"+
"\u05c4\u0001\u0000\u0000\u0000\u05c2\u05c0\u0001\u0000\u0000\u0000\u05c2"+
"\u05c3\u0001\u0000\u0000\u0000\u05c3\u00b5\u0001\u0000\u0000\u0000\u05c4"+
"\u05c2\u0001\u0000\u0000\u0000\u05c5\u05c7\u0003\u00bc^\u0000\u05c6\u05c8"+
"\u0003\u00b8\\\u0000\u05c7\u05c6\u0001\u0000\u0000\u0000\u05c7\u05c8\u0001"+
"\u0000\u0000\u0000\u05c8\u00b7\u0001\u0000\u0000\u0000\u05c9\u05d1\u0005"+
"\u00d9\u0000\u0000\u05ca\u05cb\u0005\u00ff\u0000\u0000\u05cb\u05d1\u0005"+
"\u0128\u0000\u0000\u05cc\u05cd\u0005`\u0000\u0000\u05cd\u05d1\u0005\u0128"+
"\u0000\u0000\u05ce\u05d1\u00056\u0000\u0000\u05cf\u05d1\u0005\u0080\u0000"+
"\u0000\u05d0\u05c9\u0001\u0000\u0000\u0000\u05d0\u05ca\u0001\u0000\u0000"+
"\u0000\u05d0\u05cc\u0001\u0000\u0000\u0000\u05d0\u05ce\u0001\u0000\u0000"+
"\u0000\u05d0\u05cf\u0001\u0000\u0000\u0000\u05d1\u05d2\u0001\u0000\u0000"+
"\u0000\u05d2\u05ea\u0003\u00bc^\u0000\u05d3\u05d5\u0005\u0088\u0000\u0000"+
"\u05d4\u05d6\u0005\u00b0\u0000\u0000\u05d5\u05d4\u0001\u0000\u0000\u0000"+
"\u05d5\u05d6\u0001\u0000\u0000\u0000\u05d6\u05d7\u0001\u0000\u0000\u0000"+
"\u05d7\u05ea\u0005\u00b3\u0000\u0000\u05d8\u05da\u0005\u0088\u0000\u0000"+
"\u05d9\u05db\u0005\u00b0\u0000\u0000\u05da\u05d9\u0001\u0000\u0000\u0000"+
"\u05da\u05db\u0001\u0000\u0000\u0000\u05db\u05dc\u0001\u0000\u0000\u0000"+
"\u05dc\u05df\u0007\u0011\u0000\u0000\u05dd\u05df\u0005.\u0000\u0000\u05de"+
"\u05d8\u0001\u0000\u0000\u0000\u05de\u05dd\u0001\u0000\u0000\u0000\u05df"+
"\u05e0\u0001\u0000\u0000\u0000\u05e0\u05ea\u0003\u0114\u008a\u0000\u05e1"+
"\u05e3\u0005\u0088\u0000\u0000\u05e2\u05e4\u0005\u00b0\u0000\u0000\u05e3"+
"\u05e2\u0001\u0000\u0000\u0000\u05e3\u05e4\u0001\u0000\u0000\u0000\u05e4"+
"\u05e6\u0001\u0000\u0000\u0000\u05e5\u05e7\u0003\u00ba]\u0000\u05e6\u05e5"+
"\u0001\u0000\u0000\u0000\u05e6\u05e7\u0001\u0000\u0000\u0000\u05e7\u05e8"+
"\u0001\u0000\u0000\u0000\u05e8\u05ea\u0005\u00af\u0000\u0000\u05e9\u05d0"+
"\u0001\u0000\u0000\u0000\u05e9\u05d3\u0001\u0000\u0000\u0000\u05e9\u05de"+
"\u0001\u0000\u0000\u0000\u05e9\u05e1\u0001\u0000\u0000\u0000\u05ea\u00b9"+
"\u0001\u0000\u0000\u0000\u05eb\u05ec\u0007\u0012\u0000\u0000\u05ec\u00bb"+
"\u0001\u0000\u0000\u0000\u05ed\u05f2\u0003\u00be_\u0000\u05ee\u05ef\u0007"+
"\u0013\u0000\u0000\u05ef\u05f1\u0003\u00be_\u0000\u05f0\u05ee\u0001\u0000"+
"\u0000\u0000\u05f1\u05f4\u0001\u0000\u0000\u0000\u05f2\u05f0\u0001\u0000"+
"\u0000\u0000\u05f2\u05f3\u0001\u0000\u0000\u0000\u05f3\u00bd\u0001\u0000"+
"\u0000\u0000\u05f4\u05f2\u0001\u0000\u0000\u0000\u05f5\u05fa\u0003\u00c0"+
"`\u0000\u05f6\u05f7\u0007\u0014\u0000\u0000\u05f7\u05f9\u0003\u00c0`\u0000"+
"\u05f8\u05f6\u0001\u0000\u0000\u0000\u05f9\u05fc\u0001\u0000\u0000\u0000"+
"\u05fa\u05f8\u0001\u0000\u0000\u0000\u05fa\u05fb\u0001\u0000\u0000\u0000"+
"\u05fb\u00bf\u0001\u0000\u0000\u0000\u05fc\u05fa\u0001\u0000\u0000\u0000"+
"\u05fd\u0602\u0003\u00c2a\u0000\u05fe\u05ff\u0005\u00c6\u0000\u0000\u05ff"+
"\u0601\u0003\u00c2a\u0000\u0600\u05fe\u0001\u0000\u0000\u0000\u0601\u0604"+
"\u0001\u0000\u0000\u0000\u0602\u0600\u0001\u0000\u0000\u0000\u0602\u0603"+
"\u0001\u0000\u0000\u0000\u0603\u00c1\u0001\u0000\u0000\u0000\u0604\u0602"+
"\u0001\u0000\u0000\u0000\u0605\u0609\u0003\u00c4b\u0000\u0606\u0607\u0007"+
"\u0015\u0000\u0000\u0607\u0609\u0003\u00c4b\u0000\u0608\u0605\u0001\u0000"+
"\u0000\u0000\u0608\u0606\u0001\u0000\u0000\u0000\u0609\u00c3\u0001\u0000"+
"\u0000\u0000\u060a\u060e\u0003\u00d0h\u0000\u060b\u060d\u0003\u00c6c\u0000"+
"\u060c\u060b\u0001\u0000\u0000\u0000\u060d\u0610\u0001\u0000\u0000\u0000"+
"\u060e\u060c\u0001\u0000\u0000\u0000\u060e\u060f\u0001\u0000\u0000\u0000"+
"\u060f\u00c5\u0001\u0000\u0000\u0000\u0610\u060e\u0001\u0000\u0000\u0000"+
"\u0611\u0621\u0003\u00c8d\u0000\u0612\u0621\u0003\u0096K\u0000\u0613\u0614"+
"\u0005\u008f\u0000\u0000\u0614\u0615\u0003\u00acV\u0000\u0615\u0616\u0005"+
"\u00d3\u0000\u0000\u0616\u0621\u0001\u0000\u0000\u0000\u0617\u0619\u0005"+
"\u008f\u0000\u0000\u0618\u061a\u0003\u00acV\u0000\u0619\u0618\u0001\u0000"+
"\u0000\u0000\u0619\u061a\u0001\u0000\u0000\u0000\u061a\u061b\u0001\u0000"+
"\u0000\u0000\u061b\u061d\u0005Q\u0000\u0000\u061c\u061e\u0003\u00acV\u0000"+
"\u061d\u061c\u0001\u0000\u0000\u0000\u061d\u061e\u0001\u0000\u0000\u0000"+
"\u061e\u061f\u0001\u0000\u0000\u0000\u061f\u0621\u0005\u00d3\u0000\u0000"+
"\u0620\u0611\u0001\u0000\u0000\u0000\u0620\u0612\u0001\u0000\u0000\u0000"+
"\u0620\u0613\u0001\u0000\u0000\u0000\u0620\u0617\u0001\u0000\u0000\u0000"+
"\u0621\u00c7\u0001\u0000\u0000\u0000\u0622\u0623\u0005P\u0000\u0000\u0623"+
"\u0624\u0003\u0102\u0081\u0000\u0624\u00c9\u0001\u0000\u0000\u0000\u0625"+
"\u0626\u0005\u008f\u0000\u0000\u0626\u0627\u0003\u00acV\u0000\u0627\u0628"+
"\u0005\u00d3\u0000\u0000\u0628\u00cb\u0001\u0000\u0000\u0000\u0629\u062b"+
"\u0003\u00d0h\u0000\u062a\u062c\u0003\u00c8d\u0000\u062b\u062a\u0001\u0000"+
"\u0000\u0000\u062c\u062d\u0001\u0000\u0000\u0000\u062d\u062b\u0001\u0000"+
"\u0000\u0000\u062d\u062e\u0001\u0000\u0000\u0000\u062e\u00cd\u0001\u0000"+
"\u0000\u0000\u062f\u0630\u0003\u00d0h\u0000\u0630\u0631\u0003\u00cae\u0000"+
"\u0631\u00cf\u0001\u0000\u0000\u0000\u0632\u0648\u0003\u00d2i\u0000\u0633"+
"\u0648\u0003\u0104\u0082\u0000\u0634\u0648\u0003\u00d4j\u0000\u0635\u0648"+
"\u0003\u00d8l\u0000\u0636\u0648\u0003\u00f4z\u0000\u0637\u0648\u0003\u00f6"+
"{\u0000\u0638\u0648\u0003\u00f8|\u0000\u0639\u0648\u0003\u00fa}\u0000"+
"\u063a\u0648\u0003\u00f0x\u0000\u063b\u0648\u0003\u00deo\u0000\u063c\u0648"+
"\u0003\u0100\u0080\u0000\u063d\u0648\u0003\u00e0p\u0000\u063e\u0648\u0003"+
"\u00e2q\u0000\u063f\u0648\u0003\u00e4r\u0000\u0640\u0648\u0003\u00e6s"+
"\u0000\u0641\u0648\u0003\u00e8t\u0000\u0642\u0648\u0003\u00eau\u0000\u0643"+
"\u0648\u0003\u00ecv\u0000\u0644\u0648\u0003\u00eew\u0000\u0645\u0648\u0003"+
"\u0108\u0084\u0000\u0646\u0648\u0003\u0110\u0088\u0000\u0647\u0632\u0001"+
"\u0000\u0000\u0000\u0647\u0633\u0001\u0000\u0000\u0000\u0647\u0634\u0001"+
"\u0000\u0000\u0000\u0647\u0635\u0001\u0000\u0000\u0000\u0647\u0636\u0001"+
"\u0000\u0000\u0000\u0647\u0637\u0001\u0000\u0000\u0000\u0647\u0638\u0001"+
"\u0000\u0000\u0000\u0647\u0639\u0001\u0000\u0000\u0000\u0647\u063a\u0001"+
"\u0000\u0000\u0000\u0647\u063b\u0001\u0000\u0000\u0000\u0647\u063c\u0001"+
"\u0000\u0000\u0000\u0647\u063d\u0001\u0000\u0000\u0000\u0647\u063e\u0001"+
"\u0000\u0000\u0000\u0647\u063f\u0001\u0000\u0000\u0000\u0647\u0640\u0001"+
"\u0000\u0000\u0000\u0647\u0641\u0001\u0000\u0000\u0000\u0647\u0642\u0001"+
"\u0000\u0000\u0000\u0647\u0643\u0001\u0000\u0000\u0000\u0647\u0644\u0001"+
"\u0000\u0000\u0000\u0647\u0645\u0001\u0000\u0000\u0000\u0647\u0646\u0001"+
"\u0000\u0000\u0000\u0648\u00d1\u0001\u0000\u0000\u0000\u0649\u0653\u0003"+
"\u00fc~\u0000\u064a\u0653\u0003\u0276\u013b\u0000\u064b\u0653\u0003\u027e"+
"\u013f\u0000\u064c\u0653\u0005\u0114\u0000\u0000\u064d\u0653\u0005i\u0000"+
"\u0000\u064e\u0653\u0005\u0083\u0000\u0000\u064f\u0653\u0005\u0084\u0000"+
"\u0000\u0650\u0653\u0005\u00a4\u0000\u0000\u0651\u0653\u0005\u00b3\u0000"+
"\u0000\u0652\u0649\u0001\u0000\u0000\u0000\u0652\u064a\u0001\u0000\u0000"+
"\u0000\u0652\u064b\u0001\u0000\u0000\u0000\u0652\u064c\u0001\u0000\u0000"+
"\u0000\u0652\u064d\u0001\u0000\u0000\u0000\u0652\u064e\u0001\u0000\u0000"+
"\u0000\u0652\u064f\u0001\u0000\u0000\u0000\u0652\u0650\u0001\u0000\u0000"+
"\u0000\u0652\u0651\u0001\u0000\u0000\u0000\u0653\u00d3\u0001\u0000\u0000"+
"\u0000\u0654\u0656\u0005)\u0000\u0000\u0655\u0657\u0003\u00d6k\u0000\u0656"+
"\u0655\u0001\u0000\u0000\u0000\u0657\u0658\u0001\u0000\u0000\u0000\u0658"+
"\u0656\u0001\u0000\u0000\u0000\u0658\u0659\u0001\u0000\u0000\u0000\u0659"+
"\u065c\u0001\u0000\u0000\u0000\u065a\u065b\u0005]\u0000\u0000\u065b\u065d"+
"\u0003\u00acV\u0000\u065c\u065a\u0001\u0000\u0000\u0000\u065c\u065d\u0001"+
"\u0000\u0000\u0000\u065d\u065e\u0001\u0000\u0000\u0000\u065e\u065f\u0005"+
"_\u0000\u0000\u065f\u00d5\u0001\u0000\u0000\u0000\u0660\u0661\u0005\u0126"+
"\u0000\u0000\u0661\u0662\u0003\u00acV\u0000\u0662\u0663\u0005\u0108\u0000"+
"\u0000\u0663\u0664\u0003\u00acV\u0000\u0664\u00d7\u0001\u0000\u0000\u0000"+
"\u0665\u0666\u0005)\u0000\u0000\u0666\u0668\u0003\u00acV\u0000\u0667\u0669"+
"\u0003\u00dam\u0000\u0668\u0667\u0001\u0000\u0000\u0000\u0669\u066a\u0001"+
"\u0000\u0000\u0000\u066a\u0668\u0001\u0000\u0000\u0000\u066a\u066b\u0001"+
"\u0000\u0000\u0000\u066b\u066e\u0001\u0000\u0000\u0000\u066c\u066d\u0005"+
"]\u0000\u0000\u066d\u066f\u0003\u00acV\u0000\u066e\u066c\u0001\u0000\u0000"+
"\u0000\u066e\u066f\u0001\u0000\u0000\u0000\u066f\u0670\u0001\u0000\u0000"+
"\u0000\u0670\u0671\u0005_\u0000\u0000\u0671\u00d9\u0001\u0000\u0000\u0000"+
"\u0672\u0673\u0005\u0126\u0000\u0000\u0673\u0678\u0003\u00dcn\u0000\u0674"+
"\u0675\u0005/\u0000\u0000\u0675\u0677\u0003\u00dcn\u0000\u0676\u0674\u0001"+
"\u0000\u0000\u0000\u0677\u067a\u0001\u0000\u0000\u0000\u0678\u0676\u0001"+
"\u0000\u0000\u0000\u0678\u0679\u0001\u0000\u0000\u0000\u0679\u067b\u0001"+
"\u0000\u0000\u0000\u067a\u0678\u0001\u0000\u0000\u0000\u067b\u067c\u0005"+
"\u0108\u0000\u0000\u067c\u067d\u0003\u00acV\u0000\u067d\u00db\u0001\u0000"+
"\u0000\u0000\u067e\u0684\u0005\u00d9\u0000\u0000\u067f\u0680\u0005\u00ff"+
"\u0000\u0000\u0680\u0684\u0005\u0128\u0000\u0000\u0681\u0682\u0005`\u0000"+
"\u0000\u0682\u0684\u0005\u0128\u0000\u0000\u0683\u067e\u0001\u0000\u0000"+
"\u0000\u0683\u067f\u0001\u0000\u0000\u0000\u0683\u0681\u0001\u0000\u0000"+
"\u0000\u0684\u0685\u0001\u0000\u0000\u0000\u0685\u06a0\u0003\u00bc^\u0000"+
"\u0686\u0688\u0005\u0088\u0000\u0000\u0687\u0689\u0005\u00b0\u0000\u0000"+
"\u0688\u0687\u0001\u0000\u0000\u0000\u0688\u0689\u0001\u0000\u0000\u0000"+
"\u0689\u068a\u0001\u0000\u0000\u0000\u068a\u06a0\u0005\u00b3\u0000\u0000"+
"\u068b\u068d\u0005\u0088\u0000\u0000\u068c\u068e\u0005\u00b0\u0000\u0000"+
"\u068d\u068c\u0001\u0000\u0000\u0000\u068d\u068e\u0001\u0000\u0000\u0000"+
"\u068e\u068f\u0001\u0000\u0000\u0000\u068f\u0692\u0005\u0116\u0000\u0000"+
"\u0690\u0692\u0005.\u0000\u0000\u0691\u068b\u0001\u0000\u0000\u0000\u0691"+
"\u0690\u0001\u0000\u0000\u0000\u0692\u0693\u0001\u0000\u0000\u0000\u0693"+
"\u06a0\u0003\u0114\u008a\u0000\u0694\u0696\u0005\u0088\u0000\u0000\u0695"+
"\u0697\u0005\u00b0\u0000\u0000\u0696\u0695\u0001\u0000\u0000\u0000\u0696"+
"\u0697\u0001\u0000\u0000\u0000\u0697\u0699\u0001\u0000\u0000\u0000\u0698"+
"\u069a\u0003\u00ba]\u0000\u0699\u0698\u0001\u0000\u0000\u0000\u0699\u069a"+
"\u0001\u0000\u0000\u0000\u069a\u069b\u0001\u0000\u0000\u0000\u069b\u06a0"+
"\u0005\u00af\u0000\u0000\u069c\u069d\u0007\u0010\u0000\u0000\u069d\u06a0"+
"\u0003\u00b6[\u0000\u069e\u06a0\u0003\u00acV\u0000\u069f\u0683\u0001\u0000"+
"\u0000\u0000\u069f\u0686\u0001\u0000\u0000\u0000\u069f\u0691\u0001\u0000"+
"\u0000\u0000\u069f\u0694\u0001\u0000\u0000\u0000\u069f\u069c\u0001\u0000"+
"\u0000\u0000\u069f\u069e\u0001\u0000\u0000\u0000\u06a0\u00dd\u0001\u0000"+
"\u0000\u0000\u06a1\u06a2\u0005\u008f\u0000\u0000\u06a2\u06a3\u0003\u0110"+
"\u0088\u0000\u06a3\u06a4\u0005\u0080\u0000\u0000\u06a4\u06af\u0003\u00ac"+
"V\u0000\u06a5\u06a6\u0005\u0127\u0000\u0000\u06a6\u06a8\u0003\u00acV\u0000"+
"\u06a7\u06a5\u0001\u0000\u0000\u0000\u06a7\u06a8\u0001\u0000\u0000\u0000"+
"\u06a8\u06a9\u0001\u0000\u0000\u0000\u06a9\u06aa\u0005\u001d\u0000\u0000"+
"\u06aa\u06b0\u0003\u00acV\u0000\u06ab\u06ac\u0005\u0127\u0000\u0000\u06ac"+
"\u06ae\u0003\u00acV\u0000\u06ad\u06ab\u0001\u0000\u0000\u0000\u06ad\u06ae"+
"\u0001\u0000\u0000\u0000\u06ae\u06b0\u0001\u0000\u0000\u0000\u06af\u06a7"+
"\u0001\u0000\u0000\u0000\u06af\u06ad\u0001\u0000\u0000\u0000\u06b0\u06b1"+
"\u0001\u0000\u0000\u0000\u06b1\u06b2\u0005\u00d3\u0000\u0000\u06b2\u00df"+
"\u0001\u0000\u0000\u0000\u06b3\u06b7\u0005\u008f\u0000\u0000\u06b4\u06b5"+
"\u0003\u0110\u0088\u0000\u06b5\u06b6\u0005a\u0000\u0000\u06b6\u06b8\u0001"+
"\u0000\u0000\u0000\u06b7\u06b4\u0001\u0000\u0000\u0000\u06b7\u06b8\u0001"+
"\u0000\u0000\u0000\u06b8\u06b9\u0001\u0000\u0000\u0000\u06b9\u06bc\u0003"+
"r9\u0000\u06ba\u06bb\u0005\u0127\u0000\u0000\u06bb\u06bd\u0003\u00acV"+
"\u0000\u06bc\u06ba\u0001\u0000\u0000\u0000\u06bc\u06bd\u0001\u0000\u0000"+
"\u0000\u06bd\u06be\u0001\u0000\u0000\u0000\u06be\u06bf\u0005\u001d\u0000"+
"\u0000\u06bf\u06c0\u0003\u00acV\u0000\u06c0\u06c1\u0005\u00d3\u0000\u0000"+
"\u06c1\u00e1\u0001\u0000\u0000\u0000\u06c2\u06c3\u0005\u00d7\u0000\u0000"+
"\u06c3\u06c4\u0005\u0098\u0000\u0000\u06c4\u06c5\u0003\u0110\u0088\u0000"+
"\u06c5\u06c6\u0005a\u0000\u0000\u06c6\u06c7\u0003\u00acV\u0000\u06c7\u06c8"+
"\u0005/\u0000\u0000\u06c8\u06c9\u0003\u0110\u0088\u0000\u06c9\u06ca\u0005"+
"\u0080\u0000\u0000\u06ca\u06cb\u0003\u00acV\u0000\u06cb\u06cc\u0005\u001d"+
"\u0000\u0000\u06cc\u06cd\u0003\u00acV\u0000\u06cd\u06ce\u0005\u00ea\u0000"+
"\u0000\u06ce\u00e3\u0001\u0000\u0000\u0000\u06cf\u06d0\u0007\u0016\u0000"+
"\u0000\u06d0\u06d1\u0005\u0098\u0000\u0000\u06d1\u06d2\u0003\u0110\u0088"+
"\u0000\u06d2\u06d3\u0005\u0080\u0000\u0000\u06d3\u06d6\u0003\u00acV\u0000"+
"\u06d4\u06d5\u0005\u0127\u0000\u0000\u06d5\u06d7\u0003\u00acV\u0000\u06d6"+
"\u06d4\u0001\u0000\u0000\u0000\u06d6\u06d7\u0001\u0000\u0000\u0000\u06d7"+
"\u06d8\u0001\u0000\u0000\u0000\u06d8\u06d9\u0005\u00ea\u0000\u0000\u06d9"+
"\u00e5\u0001\u0000\u0000\u0000\u06da\u06db\u0005\u00ae\u0000\u0000\u06db"+
"\u06dc\u0005\u0098\u0000\u0000\u06dc\u06df\u0003\u00acV\u0000\u06dd\u06de"+
"\u0005/\u0000\u0000\u06de\u06e0\u0003\u00ba]\u0000\u06df\u06dd\u0001\u0000"+
"\u0000\u0000\u06df\u06e0\u0001\u0000\u0000\u0000\u06e0\u06e1\u0001\u0000"+
"\u0000\u0000\u06e1\u06e2\u0005\u00ea\u0000\u0000\u06e2\u00e7\u0001\u0000"+
"\u0000\u0000\u06e3\u06e4\u0005\u0113\u0000\u0000\u06e4\u06ec\u0005\u0098"+
"\u0000\u0000\u06e5\u06e7\u0007\u0017\u0000\u0000\u06e6\u06e5\u0001\u0000"+
"\u0000\u0000\u06e6\u06e7\u0001\u0000\u0000\u0000\u06e7\u06e9\u0001\u0000"+
"\u0000\u0000\u06e8\u06ea\u0003\u00acV\u0000\u06e9\u06e8\u0001\u0000\u0000"+
"\u0000\u06e9\u06ea\u0001\u0000\u0000\u0000\u06ea\u06eb\u0001\u0000\u0000"+
"\u0000\u06eb\u06ed\u0005o\u0000\u0000\u06ec\u06e6\u0001\u0000\u0000\u0000"+
"\u06ec\u06ed\u0001\u0000\u0000\u0000\u06ed\u06ee\u0001\u0000\u0000\u0000"+
"\u06ee\u06ef\u0003\u00acV\u0000\u06ef\u06f0\u0005\u00ea\u0000\u0000\u06f0"+
"\u00e9\u0001\u0000\u0000\u0000\u06f1\u06f2\u0003r9\u0000\u06f2\u00eb\u0001"+
"\u0000\u0000\u0000\u06f3\u06f4\u0003h4\u0000\u06f4\u00ed\u0001\u0000\u0000"+
"\u0000\u06f5\u06f6\u0005\u0098\u0000\u0000\u06f6\u06f7\u0003\u00acV\u0000"+
"\u06f7\u06f8\u0005\u00ea\u0000\u0000\u06f8\u00ef\u0001\u0000\u0000\u0000"+
"\u06f9\u06fa\u0003\u0110\u0088\u0000\u06fa\u0703\u0005\u0090\u0000\u0000"+
"\u06fb\u0700\u0003\u00f2y\u0000\u06fc\u06fd\u0005/\u0000\u0000\u06fd\u06ff"+
"\u0003\u00f2y\u0000\u06fe\u06fc\u0001\u0000\u0000\u0000\u06ff\u0702\u0001"+
"\u0000\u0000\u0000\u0700\u06fe\u0001\u0000\u0000\u0000\u0700\u0701\u0001"+
"\u0000\u0000\u0000\u0701\u0704\u0001\u0000\u0000\u0000\u0702\u0700\u0001"+
"\u0000\u0000\u0000\u0703\u06fb\u0001\u0000\u0000\u0000\u0703\u0704\u0001"+
"\u0000\u0000\u0000\u0704\u0705\u0001\u0000\u0000\u0000\u0705\u0706\u0005"+
"\u00d4\u0000\u0000\u0706\u00f1\u0001\u0000\u0000\u0000\u0707\u0708\u0003"+
"\u0102\u0081\u0000\u0708\u0709\u0005-\u0000\u0000\u0709\u070a\u0003\u00ac"+
"V\u0000\u070a\u0710\u0001\u0000\u0000\u0000\u070b\u0710\u0003\u00c8d\u0000"+
"\u070c\u0710\u0003\u0110\u0088\u0000\u070d\u070e\u0005P\u0000\u0000\u070e"+
"\u0710\u0005\u010a\u0000\u0000\u070f\u0707\u0001\u0000\u0000\u0000\u070f"+
"\u070b\u0001\u0000\u0000\u0000\u070f\u070c\u0001\u0000\u0000\u0000\u070f"+
"\u070d\u0001\u0000\u0000\u0000\u0710\u00f3\u0001\u0000\u0000\u0000\u0711"+
"\u0712\u00059\u0000\u0000\u0712\u0713\u0005\u0098\u0000\u0000\u0713\u0714"+
"\u0005\u010a\u0000\u0000\u0714\u0715\u0005\u00ea\u0000\u0000\u0715\u00f5"+
"\u0001\u0000\u0000\u0000\u0716\u0717\u0005f\u0000\u0000\u0717\u0720\u0005"+
"\u0090\u0000\u0000\u0718\u0721\u0003\u0004\u0002\u0000\u0719\u071b\u0003"+
"8\u001c\u0000\u071a\u0719\u0001\u0000\u0000\u0000\u071a\u071b\u0001\u0000"+
"\u0000\u0000\u071b\u071c\u0001\u0000\u0000\u0000\u071c\u071e\u0003\\."+
"\u0000\u071d\u071f\u0003$\u0012\u0000\u071e\u071d\u0001\u0000\u0000\u0000"+
"\u071e\u071f\u0001\u0000\u0000\u0000\u071f\u0721\u0001\u0000\u0000\u0000"+
"\u0720\u0718\u0001\u0000\u0000\u0000\u0720\u071a\u0001\u0000\u0000\u0000"+
"\u0721\u0722\u0001\u0000\u0000\u0000\u0722\u0723\u0005\u00d4\u0000\u0000"+
"\u0723\u00f7\u0001\u0000\u0000\u0000\u0724\u0725\u00059\u0000\u0000\u0725"+
"\u072e\u0005\u0090\u0000\u0000\u0726\u072f\u0003\u0004\u0002\u0000\u0727"+
"\u0729\u00038\u001c\u0000\u0728\u0727\u0001\u0000\u0000\u0000\u0728\u0729"+
"\u0001\u0000\u0000\u0000\u0729\u072a\u0001\u0000\u0000\u0000\u072a\u072c"+
"\u0003\\.\u0000\u072b\u072d\u0003$\u0012\u0000\u072c\u072b\u0001\u0000"+
"\u0000\u0000\u072c\u072d\u0001\u0000\u0000\u0000\u072d\u072f\u0001\u0000"+
"\u0000\u0000\u072e\u0726\u0001\u0000\u0000\u0000\u072e\u0728\u0001\u0000"+
"\u0000\u0000\u072f\u0730\u0001\u0000\u0000\u0000\u0730\u0731\u0005\u00d4"+
"\u0000\u0000\u0731\u00f9\u0001\u0000\u0000\u0000\u0732\u0733\u0005,\u0000"+
"\u0000\u0733\u0734\u0005\u0090\u0000\u0000\u0734\u0735\u0003\u0004\u0002"+
"\u0000\u0735\u0736\u0005\u00d4\u0000\u0000\u0736\u00fb\u0001\u0000\u0000"+
"\u0000\u0737\u0739\u0005\u009e\u0000\u0000\u0738\u0737\u0001\u0000\u0000"+
"\u0000\u0738\u0739\u0001\u0000\u0000\u0000\u0739\u073a\u0001\u0000\u0000"+
"\u0000\u073a\u073b\u0007\u0018\u0000\u0000\u073b\u00fd\u0001\u0000\u0000"+
"\u0000\u073c\u073e\u0005\u009e\u0000\u0000\u073d\u073c\u0001\u0000\u0000"+
"\u0000\u073d\u073e\u0001\u0000\u0000\u0000\u073e\u073f\u0001\u0000\u0000"+
"\u0000\u073f\u0740\u0005\u0005\u0000\u0000\u0740\u00ff\u0001\u0000\u0000"+
"\u0000\u0741\u074a\u0005\u008f\u0000\u0000\u0742\u0747\u0003\u00acV\u0000"+
"\u0743\u0744\u0005/\u0000\u0000\u0744\u0746\u0003\u00acV\u0000\u0745\u0743"+
"\u0001\u0000\u0000\u0000\u0746\u0749\u0001\u0000\u0000\u0000\u0747\u0745"+
"\u0001\u0000\u0000\u0000\u0747\u0748\u0001\u0000\u0000\u0000\u0748\u074b"+
"\u0001\u0000\u0000\u0000\u0749\u0747\u0001\u0000\u0000\u0000\u074a\u0742"+
"\u0001\u0000\u0000\u0000\u074a\u074b\u0001\u0000\u0000\u0000\u074b\u074c"+
"\u0001\u0000\u0000\u0000\u074c\u074d\u0005\u00d3\u0000\u0000\u074d\u0101"+
"\u0001\u0000\u0000\u0000\u074e\u074f\u0003\u0280\u0140\u0000\u074f\u0103"+
"\u0001\u0000\u0000\u0000\u0750\u0751\u0005M\u0000\u0000\u0751\u0752\u0003"+
"\u0106\u0083\u0000\u0752\u0105\u0001\u0000\u0000\u0000\u0753\u0758\u0003"+
"\u0280\u0140\u0000\u0754\u0758\u0005\u0005\u0000\u0000\u0755\u0758\u0005"+
"\u0007\u0000\u0000\u0756\u0758\u0005\u0130\u0000\u0000\u0757\u0753\u0001"+
"\u0000\u0000\u0000\u0757\u0754\u0001\u0000\u0000\u0000\u0757\u0755\u0001"+
"\u0000\u0000\u0000\u0757\u0756\u0001\u0000\u0000\u0000\u0758\u0107\u0001"+
"\u0000\u0000\u0000\u0759\u075a\u0003\u010c\u0086\u0000\u075a\u075c\u0005"+
"\u0098\u0000\u0000\u075b\u075d\u0007\u0000\u0000\u0000\u075c\u075b\u0001"+
"\u0000\u0000\u0000\u075c\u075d\u0001\u0000\u0000\u0000\u075d\u0766\u0001"+
"\u0000\u0000\u0000\u075e\u0763\u0003\u010a\u0085\u0000\u075f\u0760\u0005"+
"/\u0000\u0000\u0760\u0762\u0003\u010a\u0085\u0000\u0761\u075f\u0001\u0000"+
"\u0000\u0000\u0762\u0765\u0001\u0000\u0000\u0000\u0763\u0761\u0001\u0000"+
"\u0000\u0000\u0763\u0764\u0001\u0000\u0000\u0000\u0764\u0767\u0001\u0000"+
"\u0000\u0000\u0765\u0763\u0001\u0000\u0000\u0000\u0766\u075e\u0001\u0000"+
"\u0000\u0000\u0766\u0767\u0001\u0000\u0000\u0000\u0767\u0768\u0001\u0000"+
"\u0000\u0000\u0768\u0769\u0005\u00ea\u0000\u0000\u0769\u0109\u0001\u0000"+
"\u0000\u0000\u076a\u076b\u0003\u00acV\u0000\u076b\u010b\u0001\u0000\u0000"+
"\u0000\u076c\u076d\u0003\u010e\u0087\u0000\u076d\u076e\u0003\u0280\u0140"+
"\u0000\u076e\u010d\u0001\u0000\u0000\u0000\u076f\u0770\u0003\u0280\u0140"+
"\u0000\u0770\u0771\u0005P\u0000\u0000\u0771\u0773\u0001\u0000\u0000\u0000"+
"\u0772\u076f\u0001\u0000\u0000\u0000\u0773\u0776\u0001\u0000\u0000\u0000"+
"\u0774\u0772\u0001\u0000\u0000\u0000\u0774\u0775\u0001\u0000\u0000\u0000"+
"\u0775\u010f\u0001\u0000\u0000\u0000\u0776\u0774\u0001\u0000\u0000\u0000"+
"\u0777\u0778\u0003\u0280\u0140\u0000\u0778\u0111\u0001\u0000\u0000\u0000"+
"\u0779\u077e\u0003\u0280\u0140\u0000\u077a\u077b\u0005/\u0000\u0000\u077b"+
"\u077d\u0003\u0280\u0140\u0000\u077c\u077a\u0001\u0000\u0000\u0000\u077d"+
"\u0780\u0001\u0000\u0000\u0000\u077e\u077c\u0001\u0000\u0000\u0000\u077e"+
"\u077f\u0001\u0000\u0000\u0000\u077f\u0113\u0001\u0000\u0000\u0000\u0780"+
"\u077e\u0001\u0000\u0000\u0000\u0781\u0786\u0003\u0116\u008b\u0000\u0782"+
"\u0783\u0005\u001d\u0000\u0000\u0783\u0785\u0003\u0116\u008b\u0000\u0784"+
"\u0782\u0001\u0000\u0000\u0000\u0785\u0788\u0001\u0000\u0000\u0000\u0786"+
"\u0784\u0001\u0000\u0000\u0000\u0786\u0787\u0001\u0000\u0000\u0000\u0787"+
"\u0115\u0001\u0000\u0000\u0000\u0788\u0786\u0001\u0000\u0000\u0000\u0789"+
"\u078b\u0003\u0118\u008c\u0000\u078a\u078c\u0003\u011a\u008d\u0000\u078b"+
"\u078a\u0001\u0000\u0000\u0000\u078b\u078c\u0001\u0000\u0000\u0000\u078c"+
"\u0790\u0001\u0000\u0000\u0000\u078d\u078f\u0003\u011c\u008e\u0000\u078e"+
"\u078d\u0001\u0000\u0000\u0000\u078f\u0792\u0001\u0000\u0000\u0000\u0790"+
"\u078e\u0001\u0000\u0000\u0000\u0790\u0791\u0001\u0000\u0000\u0000\u0791"+
"\u0117\u0001\u0000\u0000\u0000\u0792\u0790\u0001\u0000\u0000\u0000\u0793"+
"\u07d5\u0005\u00b1\u0000\u0000\u0794\u07d5\u0005\u00b3\u0000\u0000\u0795"+
"\u07d5\u0005\u001f\u0000\u0000\u0796\u07d5\u0005 \u0000\u0000\u0797\u07d5"+
"\u0005\u0122\u0000\u0000\u0798\u07d5\u0005\u0102\u0000\u0000\u0799\u07d5"+
"\u0005\u0086\u0000\u0000\u079a\u079c\u0005\u00fb\u0000\u0000\u079b\u079a"+
"\u0001\u0000\u0000\u0000\u079b\u079c\u0001\u0000\u0000\u0000\u079c\u079d"+
"\u0001\u0000\u0000\u0000\u079d\u07d5\u0005\u0087\u0000\u0000\u079e\u07d5"+
"\u0005l\u0000\u0000\u079f\u07d5\u0005@\u0000\u0000\u07a0\u07a1\u0005\u0096"+
"\u0000\u0000\u07a1\u07d5\u0007\u0019\u0000\u0000\u07a2\u07a3\u0005\u012e"+
"\u0000\u0000\u07a3\u07d5\u0007\u0019\u0000\u0000\u07a4\u07a5\u0005\u0109"+
"\u0000\u0000\u07a5\u07a9\u0007\u001a\u0000\u0000\u07a6\u07aa\u0005\u010c"+
"\u0000\u0000\u07a7\u07a8\u0005\u0109\u0000\u0000\u07a8\u07aa\u0005\u012d"+
"\u0000\u0000\u07a9\u07a6\u0001\u0000\u0000\u0000\u07a9\u07a7\u0001\u0000"+
"\u0000\u0000\u07aa\u07d5\u0001\u0000\u0000\u0000\u07ab\u07ac\u0005\u010b"+
"\u0000\u0000\u07ac\u07b0\u0007\u001a\u0000\u0000\u07ad\u07b1\u0005\u010c"+
"\u0000\u0000\u07ae\u07af\u0005\u0109\u0000\u0000\u07af\u07b1\u0005\u012d"+
"\u0000\u0000\u07b0\u07ad\u0001\u0000\u0000\u0000\u07b0\u07ae\u0001\u0000"+
"\u0000\u0000\u07b1\u07d5\u0001\u0000\u0000\u0000\u07b2\u07d5\u0005W\u0000"+
"\u0000\u07b3\u07d5\u0005\u00c4\u0000\u0000\u07b4\u07d5\u0005\u00aa\u0000"+
"\u0000\u07b5\u07d5\u0005\u0124\u0000\u0000\u07b6\u07d5\u0005\u00db\u0000"+
"\u0000\u07b7\u07d5\u0005Y\u0000\u0000\u07b8\u07d5\u0005\u009b\u0000\u0000"+
"\u07b9\u07ba\u0007\u001b\u0000\u0000\u07ba\u07bb\u0005\u0099\u0000\u0000"+
"\u07bb\u07bc\u0003\u0114\u008a\u0000\u07bc\u07bd\u0005y\u0000\u0000\u07bd"+
"\u07d5\u0001\u0000\u0000\u0000\u07be\u07d5\u0005\u00bf\u0000\u0000\u07bf"+
"\u07d5\u0005\u00c0\u0000\u0000\u07c0\u07c1\u0005\u00ce\u0000\u0000\u07c1"+
"\u07d5\u0005\u0121\u0000\u0000\u07c2\u07d2\u0005\u0015\u0000\u0000\u07c3"+
"\u07d3\u0005\u00aa\u0000\u0000\u07c4\u07d3\u0005\u0124\u0000\u0000\u07c5"+
"\u07d3\u0005\u00db\u0000\u0000\u07c6\u07d3\u0005Y\u0000\u0000\u07c7\u07d3"+
"\u0005\u009b\u0000\u0000\u07c8\u07c9\u0005\u00ce\u0000\u0000\u07c9\u07d3"+
"\u0005\u0121\u0000\u0000\u07ca\u07cc\u0005\u0121\u0000\u0000\u07cb\u07ca"+
"\u0001\u0000\u0000\u0000\u07cb\u07cc\u0001\u0000\u0000\u0000\u07cc\u07cd"+
"\u0001\u0000\u0000\u0000\u07cd\u07ce\u0005\u0099\u0000\u0000\u07ce\u07cf"+
"\u0003\u0114\u008a\u0000\u07cf\u07d0\u0005y\u0000\u0000\u07d0\u07d3\u0001"+
"\u0000\u0000\u0000\u07d1\u07d3\u0005\u0121\u0000\u0000\u07d2\u07c3\u0001"+
"\u0000\u0000\u0000\u07d2\u07c4\u0001\u0000\u0000\u0000\u07d2\u07c5\u0001"+
"\u0000\u0000\u0000\u07d2\u07c6\u0001\u0000\u0000\u0000\u07d2\u07c7\u0001"+
"\u0000\u0000\u0000\u07d2\u07c8\u0001\u0000\u0000\u0000\u07d2\u07cb\u0001"+
"\u0000\u0000\u0000\u07d2\u07d1\u0001\u0000\u0000\u0000\u07d2\u07d3\u0001"+
"\u0000\u0000\u0000\u07d3\u07d5\u0001\u0000\u0000\u0000\u07d4\u0793\u0001"+
"\u0000\u0000\u0000\u07d4\u0794\u0001\u0000\u0000\u0000\u07d4\u0795\u0001"+
"\u0000\u0000\u0000\u07d4\u0796\u0001\u0000\u0000\u0000\u07d4\u0797\u0001"+
"\u0000\u0000\u0000\u07d4\u0798\u0001\u0000\u0000\u0000\u07d4\u0799\u0001"+
"\u0000\u0000\u0000\u07d4\u079b\u0001\u0000\u0000\u0000\u07d4\u079e\u0001"+
"\u0000\u0000\u0000\u07d4\u079f\u0001\u0000\u0000\u0000\u07d4\u07a0\u0001"+
"\u0000\u0000\u0000\u07d4\u07a2\u0001\u0000\u0000\u0000\u07d4\u07a4\u0001"+
"\u0000\u0000\u0000\u07d4\u07ab\u0001\u0000\u0000\u0000\u07d4\u07b2\u0001"+
"\u0000\u0000\u0000\u07d4\u07b3\u0001\u0000\u0000\u0000\u07d4\u07b4\u0001"+
"\u0000\u0000\u0000\u07d4\u07b5\u0001\u0000\u0000\u0000\u07d4\u07b6\u0001"+
"\u0000\u0000\u0000\u07d4\u07b7\u0001\u0000\u0000\u0000\u07d4\u07b8\u0001"+
"\u0000\u0000\u0000\u07d4\u07b9\u0001\u0000\u0000\u0000\u07d4\u07be\u0001"+
"\u0000\u0000\u0000\u07d4\u07bf\u0001\u0000\u0000\u0000\u07d4\u07c0\u0001"+
"\u0000\u0000\u0000\u07d4\u07c2\u0001\u0000\u0000\u0000\u07d5\u0119\u0001"+
"\u0000\u0000\u0000\u07d6\u07d7\u0005\u00b0\u0000\u0000\u07d7\u07da\u0005"+
"\u00b3\u0000\u0000\u07d8\u07da\u0005\u008e\u0000\u0000\u07d9\u07d6\u0001"+
"\u0000\u0000\u0000\u07d9\u07d8\u0001\u0000\u0000\u0000\u07da\u011b\u0001"+
"\u0000\u0000\u0000\u07db\u07dd\u0007\u001b\u0000\u0000\u07dc\u07de\u0003"+
"\u011a\u008d\u0000\u07dd\u07dc\u0001\u0000\u0000\u0000\u07dd\u07de\u0001"+
"\u0000\u0000\u0000\u07de\u011d\u0001\u0000\u0000\u0000\u07df\u07e1\u0003"+
"\n\u0005\u0000\u07e0\u07df\u0001\u0000\u0000\u0000\u07e0\u07e1\u0001\u0000"+
"\u0000\u0000\u07e1\u07ef\u0001\u0000\u0000\u0000\u07e2\u07f0\u0003\u0120"+
"\u0090\u0000\u07e3\u07f0\u0003\u0122\u0091\u0000\u07e4\u07f0\u0003\u017c"+
"\u00be\u0000\u07e5\u07f0\u0003\u017e\u00bf\u0000\u07e6\u07f0\u0003\u0182"+
"\u00c1\u0000\u07e7\u07f0\u0003\u0184\u00c2\u0000\u07e8\u07f0\u0003\u0180"+
"\u00c0\u0000\u07e9\u07f0\u0003\u0246\u0123\u0000\u07ea\u07f0\u0003\u0248"+
"\u0124\u0000\u07eb\u07f0\u0003\u018c\u00c6\u0000\u07ec\u07f0\u0003\u0196"+
"\u00cb\u0000\u07ed\u07f0\u0003\u0124\u0092\u0000\u07ee\u07f0\u0003\u0132"+
"\u0099\u0000\u07ef\u07e2\u0001\u0000\u0000\u0000\u07ef\u07e3\u0001\u0000"+
"\u0000\u0000\u07ef\u07e4\u0001\u0000\u0000\u0000\u07ef\u07e5\u0001\u0000"+
"\u0000\u0000\u07ef\u07e6\u0001\u0000\u0000\u0000\u07ef\u07e7\u0001\u0000"+
"\u0000\u0000\u07ef\u07e8\u0001\u0000\u0000\u0000\u07ef\u07e9\u0001\u0000"+
"\u0000\u0000\u07ef\u07ea\u0001\u0000\u0000\u0000\u07ef\u07eb\u0001\u0000"+
"\u0000\u0000\u07ef\u07ec\u0001\u0000\u0000\u0000\u07ef\u07ed\u0001\u0000"+
"\u0000\u0000\u07ef\u07ee\u0001\u0000\u0000\u0000\u07f0\u011f\u0001\u0000"+
"\u0000\u0000\u07f1\u07f4\u0005:\u0000\u0000\u07f2\u07f3\u0005\u00bb\u0000"+
"\u0000\u07f3\u07f5\u0005\u00df\u0000\u0000\u07f4\u07f2\u0001\u0000\u0000"+
"\u0000\u07f4\u07f5\u0001\u0000\u0000\u0000\u07f5\u07fd\u0001\u0000\u0000"+
"\u0000\u07f6\u07fe\u0003\u0254\u012a\u0000\u07f7\u07fe\u0003\u022e\u0117"+
"\u0000\u07f8\u07fe\u0003\u0160\u00b0\u0000\u07f9\u07fe\u0003\u0230\u0118"+
"\u0000\u07fa\u07fe\u0003\u0166\u00b3\u0000\u07fb\u07fe\u0003\u019c\u00ce"+
"\u0000\u07fc\u07fe\u0003\u01a8\u00d4\u0000\u07fd\u07f6\u0001\u0000\u0000"+
"\u0000\u07fd\u07f7\u0001\u0000\u0000\u0000\u07fd\u07f8\u0001\u0000\u0000"+
"\u0000\u07fd\u07f9\u0001\u0000\u0000\u0000\u07fd\u07fa\u0001\u0000\u0000"+
"\u0000\u07fd\u07fb\u0001\u0000\u0000\u0000\u07fd\u07fc\u0001\u0000\u0000"+
"\u0000\u07fe\u0121\u0001\u0000\u0000\u0000\u07ff\u0807\u0005T\u0000\u0000"+
"\u0800\u0808\u0003\u0256\u012b\u0000\u0801\u0808\u0003\u0164\u00b2\u0000"+
"\u0802\u0808\u0003\u023a\u011d\u0000\u0803\u0808\u0003\u0176\u00bb\u0000"+
"\u0804\u0808\u0003\u019e\u00cf\u0000\u0805\u0808\u0003\u0192\u00c9\u0000"+
"\u0806\u0808\u0003\u01aa\u00d5\u0000\u0807\u0800\u0001\u0000\u0000\u0000"+
"\u0807\u0801\u0001\u0000\u0000\u0000\u0807\u0802\u0001\u0000\u0000\u0000"+
"\u0807\u0803\u0001\u0000\u0000\u0000\u0807\u0804\u0001\u0000\u0000\u0000"+
"\u0807\u0805\u0001\u0000\u0000\u0000\u0807\u0806\u0001\u0000\u0000\u0000"+
"\u0808\u0123\u0001\u0000\u0000\u0000\u0809\u081a\u0005\u00fa\u0000\u0000"+
"\u080a\u081b\u0003\u0264\u0132\u0000\u080b\u081b\u0003\u013e\u009f\u0000"+
"\u080c\u081b\u0003\u01c6\u00e3\u0000\u080d\u081b\u0003\u024e\u0127\u0000"+
"\u080e\u081b\u0003\u0148\u00a4\u0000\u080f\u081b\u0003\u0138\u009c\u0000"+
"\u0810\u081b\u0003\u01ca\u00e5\u0000\u0811\u081b\u0003\u0146\u00a3\u0000"+
"\u0812\u081b\u0003\u01cc\u00e6\u0000\u0813\u081b\u0003\u01a2\u00d1\u0000"+
"\u0814\u081b\u0003\u0194\u00ca\u0000\u0815\u081b\u0003\u0154\u00aa\u0000"+
"\u0816\u081b\u0003\u01c8\u00e4\u0000\u0817\u081b\u0003\u0150\u00a8\u0000"+
"\u0818\u081b\u0003\u01ce\u00e7\u0000\u0819\u081b\u0003\u01c4\u00e2\u0000"+
"\u081a\u080a\u0001\u0000\u0000\u0000\u081a\u080b\u0001\u0000\u0000\u0000"+
"\u081a\u080c\u0001\u0000\u0000\u0000\u081a\u080d\u0001\u0000\u0000\u0000"+
"\u081a\u080e\u0001\u0000\u0000\u0000\u081a\u080f\u0001\u0000\u0000\u0000"+
"\u081a\u0810\u0001\u0000\u0000\u0000\u081a\u0811\u0001\u0000\u0000\u0000"+
"\u081a\u0812\u0001\u0000\u0000\u0000\u081a\u0813\u0001\u0000\u0000\u0000"+
"\u081a\u0814\u0001\u0000\u0000\u0000\u081a\u0815\u0001\u0000\u0000\u0000"+
"\u081a\u0816\u0001\u0000\u0000\u0000\u081a\u0817\u0001\u0000\u0000\u0000"+
"\u081a\u0818\u0001\u0000\u0000\u0000\u081a\u0819\u0001\u0000\u0000\u0000"+
"\u081b\u0125\u0001\u0000\u0000\u0000\u081c\u081e\u0003\u012e\u0097\u0000"+
"\u081d\u081f\u0003\u0010\b\u0000\u081e\u081d\u0001\u0000\u0000\u0000\u081e"+
"\u081f\u0001\u0000\u0000\u0000\u081f\u0822\u0001\u0000\u0000\u0000\u0820"+
"\u0822\u0003$\u0012\u0000\u0821\u081c\u0001\u0000\u0000\u0000\u0821\u0820"+
"\u0001\u0000\u0000\u0000\u0822\u0127\u0001\u0000\u0000\u0000\u0823\u0826"+
"\u0003\u0110\u0088\u0000\u0824\u0825\u0005\u0017\u0000\u0000\u0825\u0827"+
"\u0003\u0110\u0088\u0000\u0826\u0824\u0001\u0000\u0000\u0000\u0826\u0827"+
"\u0001\u0000\u0000\u0000\u0827\u0129\u0001\u0000\u0000\u0000\u0828\u0829"+
"\u0007\u0003\u0000\u0000\u0829\u082a\u0003\u00fe\u007f\u0000\u082a\u012b"+
"\u0001\u0000\u0000\u0000\u082b\u082c\u0005\u0093\u0000\u0000\u082c\u082d"+
"\u0003\u00fe\u007f\u0000\u082d\u012d\u0001\u0000\u0000\u0000\u082e\u0838"+
"\u0005\u012c\u0000\u0000\u082f\u0839\u0005\u010a\u0000\u0000\u0830\u0835"+
"\u0003\u0128\u0094\u0000\u0831\u0832\u0005/\u0000\u0000\u0832\u0834\u0003"+
"\u0128\u0094\u0000\u0833\u0831\u0001\u0000\u0000\u0000\u0834\u0837\u0001"+
"\u0000\u0000\u0000\u0835\u0833\u0001\u0000\u0000\u0000\u0835\u0836\u0001"+
"\u0000\u0000\u0000\u0836\u0839\u0001\u0000\u0000\u0000\u0837\u0835\u0001"+
"\u0000\u0000\u0000\u0838\u082f\u0001\u0000\u0000\u0000\u0838\u0830\u0001"+
"\u0000\u0000\u0000\u0839\u083b\u0001\u0000\u0000\u0000\u083a\u083c\u0003"+
"\u001e\u000f\u0000\u083b\u083a\u0001\u0000\u0000\u0000\u083b\u083c\u0001"+
"\u0000\u0000\u0000\u083c\u083e\u0001\u0000\u0000\u0000\u083d\u083f\u0003"+
"\u012a\u0095\u0000\u083e\u083d\u0001\u0000\u0000\u0000\u083e\u083f\u0001"+
"\u0000\u0000\u0000\u083f\u0841\u0001\u0000\u0000\u0000\u0840\u0842\u0003"+
"\u012c\u0096\u0000\u0841\u0840\u0001\u0000\u0000\u0000\u0841\u0842\u0001"+
"\u0000\u0000\u0000\u0842\u0844\u0001\u0000\u0000\u0000\u0843\u0845\u0003"+
"$\u0012\u0000\u0844\u0843\u0001\u0000\u0000\u0000\u0844\u0845\u0001\u0000"+
"\u0000\u0000\u0845\u012f\u0001\u0000\u0000\u0000\u0846\u0847\u0005\u00b9"+
"\u0000\u0000\u0847\u0848\u0003\u027c\u013e\u0000\u0848\u0131\u0001\u0000"+
"\u0000\u0000\u0849\u084a\u0005\u0106\u0000\u0000\u084a\u084b\u0003\u0152"+
"\u00a9\u0000\u084b\u0133\u0001\u0000\u0000\u0000\u084c\u084f\u0003\u0132"+
"\u0099\u0000\u084d\u084f\u0003\u0136\u009b\u0000\u084e\u084c\u0001\u0000"+
"\u0000\u0000\u084e\u084d\u0001\u0000\u0000\u0000\u084f\u0135\u0001\u0000"+
"\u0000\u0000\u0850\u0857\u0005\u00fa\u0000\u0000\u0851\u0858\u0003\u0138"+
"\u009c\u0000\u0852\u0858\u0003\u013e\u009f\u0000\u0853\u0858\u0003\u0148"+
"\u00a4\u0000\u0854\u0858\u0003\u0146\u00a3\u0000\u0855\u0858\u0003\u0154"+
"\u00aa\u0000\u0856\u0858\u0003\u0150\u00a8\u0000\u0857\u0851\u0001\u0000"+
"\u0000\u0000\u0857\u0852\u0001\u0000\u0000\u0000\u0857\u0853\u0001\u0000"+
"\u0000\u0000\u0857\u0854\u0001\u0000\u0000\u0000\u0857\u0855\u0001\u0000"+
"\u0000\u0000\u0857\u0856\u0001\u0000\u0000\u0000\u0858\u0137\u0001\u0000"+
"\u0000\u0000\u0859\u085b\u0003\u013a\u009d\u0000\u085a\u0859\u0001\u0000"+
"\u0000\u0000\u085a\u085b\u0001\u0000\u0000\u0000\u085b\u085c\u0001\u0000"+
"\u0000\u0000\u085c\u085d\u0003\u013c\u009e\u0000\u085d\u0139\u0001\u0000"+
"\u0000\u0000\u085e\u085f\u0007\u001c\u0000\u0000\u085f\u013b\u0001\u0000"+
"\u0000\u0000\u0860\u0862\u0003\u0200\u0100\u0000\u0861\u0863\u0003\u0126"+
"\u0093\u0000\u0862\u0861\u0001\u0000\u0000\u0000\u0862\u0863\u0001\u0000"+
"\u0000\u0000\u0863\u0865\u0001\u0000\u0000\u0000\u0864\u0866\u0003\u0134"+
"\u009a\u0000\u0865\u0864\u0001\u0000\u0000\u0000\u0865\u0866\u0001\u0000"+
"\u0000\u0000\u0866\u013d\u0001\u0000\u0000\u0000\u0867\u0869\u0005\u0012"+
"\u0000\u0000\u0868\u0867\u0001\u0000\u0000\u0000\u0868\u0869\u0001\u0000"+
"\u0000\u0000\u0869\u086a\u0001\u0000\u0000\u0000\u086a\u0885\u0003\u0144"+
"\u00a2\u0000\u086b\u086d\u0003\u0140\u00a0\u0000\u086c\u086b\u0001\u0000"+
"\u0000\u0000\u086c\u086d\u0001\u0000\u0000\u0000\u086d\u086e";
private static final String _serializedATNSegment1 =
"\u0001\u0000\u0000\u0000\u086e\u086f\u0003\u0142\u00a1\u0000\u086f\u0870"+
"\u0003\u0144\u00a2\u0000\u0870\u0885\u0001\u0000\u0000\u0000\u0871\u0873"+
"\u0003\u0140\u00a0\u0000\u0872\u0871\u0001\u0000\u0000\u0000\u0872\u0873"+
"\u0001\u0000\u0000\u0000\u0873\u0874\u0001\u0000\u0000\u0000\u0874\u0875"+
"\u0005\u008a\u0000\u0000\u0875\u0885\u0003\u0144\u00a2\u0000\u0876\u0878"+
"\u0003\u0140\u00a0\u0000\u0877\u0876\u0001\u0000\u0000\u0000\u0877\u0878"+
"\u0001\u0000\u0000\u0000\u0878\u0879\u0001\u0000\u0000\u0000\u0879\u087a"+
"\u0005\u00ce\u0000\u0000\u087a\u087b\u0005\u0115\u0000\u0000\u087b\u0885"+
"\u0003\u0144\u00a2\u0000\u087c\u087e\u0003\u0140\u00a0\u0000\u087d\u087c"+
"\u0001\u0000\u0000\u0000\u087d\u087e\u0001\u0000\u0000\u0000\u087e\u0880"+
"\u0001\u0000\u0000\u0000\u087f\u0881\u0005\u00ce\u0000\u0000\u0880\u087f"+
"\u0001\u0000\u0000\u0000\u0880\u0881\u0001\u0000\u0000\u0000\u0881\u0882"+
"\u0001\u0000\u0000\u0000\u0882\u0883\u0007\u001d\u0000\u0000\u0883\u0885"+
"\u0003\u0144\u00a2\u0000\u0884\u0868\u0001\u0000\u0000\u0000\u0884\u086c"+
"\u0001\u0000\u0000\u0000\u0884\u0872\u0001\u0000\u0000\u0000\u0884\u0877"+
"\u0001\u0000\u0000\u0000\u0884\u087d\u0001\u0000\u0000\u0000\u0885\u013f"+
"\u0001\u0000\u0000\u0000\u0886\u0889\u0005\u00aa\u0000\u0000\u0887\u0889"+
"\u0007\u001e\u0000\u0000\u0888\u0886\u0001\u0000\u0000\u0000\u0888\u0887"+
"\u0001\u0000\u0000\u0000\u0889\u0141\u0001\u0000\u0000\u0000\u088a\u0891"+
"\u0005e\u0000\u0000\u088b\u0891\u0005d\u0000\u0000\u088c\u088d\u0005\u00ce"+
"\u0000\u0000\u088d\u0891\u0005e\u0000\u0000\u088e\u088f\u0005\u00ce\u0000"+
"\u0000\u088f\u0891\u0005d\u0000\u0000\u0890\u088a\u0001\u0000\u0000\u0000"+
"\u0890\u088b\u0001\u0000\u0000\u0000\u0890\u088c\u0001\u0000\u0000\u0000"+
"\u0890\u088e\u0001\u0000\u0000\u0000\u0891\u0143\u0001\u0000\u0000\u0000"+
"\u0892\u0894\u0003\u0202\u0101\u0000\u0893\u0895\u0003\u0126\u0093\u0000"+
"\u0894\u0893\u0001\u0000\u0000\u0000\u0894\u0895\u0001\u0000\u0000\u0000"+
"\u0895\u0897\u0001\u0000\u0000\u0000\u0896\u0898\u0003\u0134\u009a\u0000"+
"\u0897\u0896\u0001\u0000\u0000\u0000\u0897\u0898\u0001\u0000\u0000\u0000"+
"\u0898\u0145\u0001\u0000\u0000\u0000\u0899\u089b\u0007\u001f\u0000\u0000"+
"\u089a\u089c\u0003\u014c\u00a6\u0000\u089b\u089a\u0001\u0000\u0000\u0000"+
"\u089b\u089c\u0001\u0000\u0000\u0000\u089c\u089e\u0001\u0000\u0000\u0000"+
"\u089d\u089f\u0003\u0126\u0093\u0000\u089e\u089d\u0001\u0000\u0000\u0000"+
"\u089e\u089f\u0001\u0000\u0000\u0000\u089f\u08a1\u0001\u0000\u0000\u0000"+
"\u08a0\u08a2\u0003\u0134\u009a\u0000\u08a1\u08a0\u0001\u0000\u0000\u0000"+
"\u08a1\u08a2\u0001\u0000\u0000\u0000\u08a2\u0147\u0001\u0000\u0000\u0000"+
"\u08a3\u08a5\u0003\u014e\u00a7\u0000\u08a4\u08a3\u0001\u0000\u0000\u0000"+
"\u08a4\u08a5\u0001\u0000\u0000\u0000\u08a5\u08a6\u0001\u0000\u0000\u0000"+
"\u08a6\u08a8\u0003\u014a\u00a5\u0000\u08a7\u08a9\u0003\u014c\u00a6\u0000"+
"\u08a8\u08a7\u0001\u0000\u0000\u0000\u08a8\u08a9\u0001\u0000\u0000\u0000"+
"\u08a9\u08ab\u0001\u0000\u0000\u0000\u08aa\u08ac\u0003\u0126\u0093\u0000"+
"\u08ab\u08aa\u0001\u0000\u0000\u0000\u08ab\u08ac\u0001\u0000\u0000\u0000"+
"\u08ac\u08ae\u0001\u0000\u0000\u0000\u08ad\u08af\u0003\u0134\u009a\u0000"+
"\u08ae\u08ad\u0001\u0000\u0000\u0000\u08ae\u08af\u0001\u0000\u0000\u0000"+
"\u08af\u0149\u0001\u0000\u0000\u0000\u08b0\u08b1\u0007 \u0000\u0000\u08b1"+
"\u014b\u0001\u0000\u0000\u0000\u08b2\u08b9\u0005b\u0000\u0000\u08b3\u08b7"+
"\u0005&\u0000\u0000\u08b4\u08b5\u0005<\u0000\u0000\u08b5\u08b8\u0005\u011e"+
"\u0000\u0000\u08b6\u08b8\u0003\u0280\u0140\u0000\u08b7\u08b4\u0001\u0000"+
"\u0000\u0000\u08b7\u08b6\u0001\u0000\u0000\u0000\u08b8\u08ba\u0001\u0000"+
"\u0000\u0000\u08b9\u08b3\u0001\u0000\u0000\u0000\u08b9\u08ba\u0001\u0000"+
"\u0000\u0000\u08ba\u014d\u0001\u0000\u0000\u0000\u08bb\u08c1\u0005\u0012"+
"\u0000\u0000\u08bc\u08bd\u0005%\u0000\u0000\u08bd\u08c1\u0005\u0080\u0000"+
"\u0000\u08be\u08bf\u0005\u011e\u0000\u0000\u08bf\u08c1\u0005E\u0000\u0000"+
"\u08c0\u08bb\u0001\u0000\u0000\u0000\u08c0\u08bc\u0001\u0000\u0000\u0000"+
"\u08c0\u08be\u0001\u0000\u0000\u0000\u08c1\u014f\u0001\u0000\u0000\u0000"+
"\u08c2\u08c3\u0003\u0204\u0102\u0000\u08c3\u08c4\u0003\u0158\u00ac\u0000"+
"\u08c4\u0151\u0001\u0000\u0000\u0000\u08c5\u08c6\u0003\u0204\u0102\u0000"+
"\u08c6\u08c7\u0003\u0158\u00ac\u0000\u08c7\u0153\u0001\u0000\u0000\u0000"+
"\u08c8\u08c9\u0003\u0156\u00ab\u0000\u08c9\u08ca\u0003\u0158\u00ac\u0000"+
"\u08ca\u0155\u0001\u0000\u0000\u0000\u08cb\u08cc\u0007!\u0000\u0000\u08cc"+
"\u0157\u0001\u0000\u0000\u0000\u08cd\u08cf\u0003\u0126\u0093\u0000\u08ce"+
"\u08cd\u0001\u0000\u0000\u0000\u08ce\u08cf\u0001\u0000\u0000\u0000\u08cf"+
"\u08d5\u0001\u0000\u0000\u0000\u08d0\u08d2\u0003\u015a\u00ad\u0000\u08d1"+
"\u08d3\u0003\u0126\u0093\u0000\u08d2\u08d1\u0001\u0000\u0000\u0000\u08d2"+
"\u08d3\u0001\u0000\u0000\u0000\u08d3\u08d5\u0001\u0000\u0000\u0000\u08d4"+
"\u08ce\u0001\u0000\u0000\u0000\u08d4\u08d0\u0001\u0000\u0000\u0000\u08d5"+
"\u08d7\u0001\u0000\u0000\u0000\u08d6\u08d8\u0003\u0134\u009a\u0000\u08d7"+
"\u08d6\u0001\u0000\u0000\u0000\u08d7\u08d8\u0001\u0000\u0000\u0000\u08d8"+
"\u0159\u0001\u0000\u0000\u0000\u08d9\u08dc\u0003\u0274\u013a\u0000\u08da"+
"\u08dc\u0003\u00acV\u0000\u08db\u08d9\u0001\u0000\u0000\u0000\u08db\u08da"+
"\u0001\u0000\u0000\u0000\u08dc\u015b\u0001\u0000\u0000\u0000\u08dd\u08de"+
"\u0005\u0098\u0000\u0000\u08de\u08df\u0003\u0110\u0088\u0000\u08df\u08e0"+
"\u0003\u0082A\u0000\u08e0\u08e1\u0005\u00ea\u0000\u0000\u08e1\u015d\u0001"+
"\u0000\u0000\u0000\u08e2\u08e3\u0005\u0098\u0000\u0000\u08e3\u08e5\u0005"+
"\u00ea\u0000\u0000\u08e4\u08e6\u0003\u008eG\u0000\u08e5\u08e4\u0001\u0000"+
"\u0000\u0000\u08e5\u08e6\u0001\u0000\u0000\u0000\u08e6\u08e7\u0001\u0000"+
"\u0000\u0000\u08e7\u08e8\u0003\u0090H\u0000\u08e8\u08e9\u0005\u008f\u0000"+
"\u0000\u08e9\u08ea\u0003\u0110\u0088\u0000\u08ea\u08eb\u0003\u0084B\u0000"+
"\u08eb\u08ec\u0005\u00d3\u0000\u0000\u08ec\u08ee\u0003\u0090H\u0000\u08ed"+
"\u08ef\u0003\u0092I\u0000\u08ee\u08ed\u0001\u0000\u0000\u0000\u08ee\u08ef"+
"\u0001\u0000\u0000\u0000\u08ef\u08f0\u0001\u0000\u0000\u0000\u08f0\u08f1"+
"\u0005\u0098\u0000\u0000\u08f1\u08f2\u0005\u00ea\u0000\u0000\u08f2\u015f"+
"\u0001\u0000\u0000\u0000\u08f3\u08f5\u00054\u0000\u0000\u08f4\u08f6\u0003"+
"\u0266\u0133\u0000\u08f5\u08f4\u0001\u0000\u0000\u0000\u08f5\u08f6\u0001"+
"\u0000\u0000\u0000\u08f6\u08fa\u0001\u0000\u0000\u0000\u08f7\u08f8\u0005"+
"}\u0000\u0000\u08f8\u08f9\u0005\u00b0\u0000\u0000\u08f9\u08fb\u0005f\u0000"+
"\u0000\u08fa\u08f7\u0001\u0000\u0000\u0000\u08fa\u08fb\u0001\u0000\u0000"+
"\u0000\u08fb\u08fc\u0001\u0000\u0000\u0000\u08fc\u08ff\u0005m\u0000\u0000"+
"\u08fd\u0900\u0003\u015c\u00ae\u0000\u08fe\u0900\u0003\u015e\u00af\u0000"+
"\u08ff\u08fd\u0001\u0000\u0000\u0000\u08ff\u08fe\u0001\u0000\u0000\u0000"+
"\u0900\u0901\u0001\u0000\u0000\u0000\u0901\u0903\u0003\u0162\u00b1\u0000"+
"\u0902\u0904\u0003\u0130\u0098\u0000\u0903\u0902\u0001\u0000\u0000\u0000"+
"\u0903\u0904\u0001\u0000\u0000\u0000\u0904\u0161\u0001\u0000\u0000\u0000"+
"\u0905\u0906\u0005\u00e1\u0000\u0000\u0906\u090a\u0003\u0178\u00bc\u0000"+
"\u0907\u090b\u0005.\u0000\u0000\u0908\u0909\u0005\u0088\u0000\u0000\u0909"+
"\u090b\u0007\u0011\u0000\u0000\u090a\u0907\u0001\u0000\u0000\u0000\u090a"+
"\u0908\u0001\u0000\u0000\u0000\u090b\u090c\u0001\u0000\u0000\u0000\u090c"+
"\u090d\u0003\u0114\u008a\u0000\u090d\u0925\u0001\u0000\u0000\u0000\u090e"+
"\u090f\u0005\u00e1\u0000\u0000\u090f\u0910\u0003\u0178\u00bc\u0000\u0910"+
"\u0912\u0005\u0088\u0000\u0000\u0911\u0913\u0007\"\u0000\u0000\u0912\u0911"+
"\u0001\u0000\u0000\u0000\u0912\u0913\u0001\u0000\u0000\u0000\u0913\u0914"+
"\u0001\u0000\u0000\u0000\u0914\u0915\u0005\u0119\u0000\u0000\u0915\u0925"+
"\u0001\u0000\u0000\u0000\u0916\u0917\u0005\u00e1\u0000\u0000\u0917\u0918"+
"\u0003\u0178\u00bc\u0000\u0918\u091a\u0005\u0088\u0000\u0000\u0919\u091b"+
"\u0007\"\u0000\u0000\u091a\u0919\u0001\u0000\u0000\u0000\u091a\u091b\u0001"+
"\u0000\u0000\u0000\u091b\u091c\u0001\u0000\u0000\u0000\u091c\u091d\u0005"+
"\u008a\u0000\u0000\u091d\u0925\u0001\u0000\u0000\u0000\u091e\u091f\u0005"+
"\u00e1\u0000\u0000\u091f\u0920\u0003\u0178\u00bc\u0000\u0920\u0921\u0005"+
"\u0088\u0000\u0000\u0921\u0922\u0005\u00b0\u0000\u0000\u0922\u0923\u0005"+
"\u00b3\u0000\u0000\u0923\u0925\u0001\u0000\u0000\u0000\u0924\u0905\u0001"+
"\u0000\u0000\u0000\u0924\u090e\u0001\u0000\u0000\u0000\u0924\u0916\u0001"+
"\u0000\u0000\u0000\u0924\u091e\u0001\u0000\u0000\u0000\u0925\u0163\u0001"+
"\u0000\u0000\u0000\u0926\u0927\u00054\u0000\u0000\u0927\u092a\u0003\u0266"+
"\u0133\u0000\u0928\u0929\u0005}\u0000\u0000\u0929\u092b\u0005f\u0000\u0000"+
"\u092a\u0928\u0001\u0000\u0000\u0000\u092a\u092b\u0001\u0000\u0000\u0000"+
"\u092b\u0165\u0001\u0000\u0000\u0000\u092c\u092d\u0005$\u0000\u0000\u092d"+
"\u092e\u0005\u0081\u0000\u0000\u092e\u0944\u0003\u0168\u00b4\u0000\u092f"+
"\u0930\u0005\u00d2\u0000\u0000\u0930\u0931\u0005\u0081\u0000\u0000\u0931"+
"\u0944\u0003\u0168\u00b4\u0000\u0932\u0933\u0005\u0107\u0000\u0000\u0933"+
"\u0934\u0005\u0081\u0000\u0000\u0934\u0944\u0003\u0168\u00b4\u0000\u0935"+
"\u0936\u0005\u00c4\u0000\u0000\u0936\u0937\u0005\u0081\u0000\u0000\u0937"+
"\u0944\u0003\u0168\u00b4\u0000\u0938\u0939\u0005\u0123\u0000\u0000\u0939"+
"\u093a\u0005\u0081\u0000\u0000\u093a\u0944\u0003\u0168\u00b4\u0000\u093b"+
"\u093c\u0005\u0097\u0000\u0000\u093c\u093d\u0005\u0081\u0000\u0000\u093d"+
"\u0944\u0003\u0170\u00b8\u0000\u093e\u093f\u0005p\u0000\u0000\u093f\u0940"+
"\u0005\u0081\u0000\u0000\u0940\u0944\u0003\u016a\u00b5\u0000\u0941\u0942"+
"\u0005\u0081\u0000\u0000\u0942\u0944\u0003\u0168\u00b4\u0000\u0943\u092c"+
"\u0001\u0000\u0000\u0000\u0943\u092f\u0001\u0000\u0000\u0000\u0943\u0932"+
"\u0001\u0000\u0000\u0000\u0943\u0935\u0001\u0000\u0000\u0000\u0943\u0938"+
"\u0001\u0000\u0000\u0000\u0943\u093b\u0001\u0000\u0000\u0000\u0943\u093e"+
"\u0001\u0000\u0000\u0000\u0943\u0941\u0001\u0000\u0000\u0000\u0944\u0167"+
"\u0001\u0000\u0000\u0000\u0945\u0947\u0003\u0266\u0133\u0000\u0946\u0945"+
"\u0001\u0000\u0000\u0000\u0946\u0947\u0001\u0000\u0000\u0000\u0947\u094b"+
"\u0001\u0000\u0000\u0000\u0948\u0949\u0005}\u0000\u0000\u0949\u094a\u0005"+
"\u00b0\u0000\u0000\u094a\u094c\u0005f\u0000\u0000\u094b\u0948\u0001\u0000"+
"\u0000\u0000\u094b\u094c\u0001\u0000\u0000\u0000\u094c\u094d\u0001\u0000"+
"\u0000\u0000\u094d\u0950\u0005m\u0000\u0000\u094e\u0951\u0003\u015c\u00ae"+
"\u0000\u094f\u0951\u0003\u015e\u00af\u0000\u0950\u094e\u0001\u0000\u0000"+
"\u0000\u0950\u094f\u0001\u0000\u0000\u0000\u0951\u0952\u0001\u0000\u0000"+
"\u0000\u0952\u0953\u0005\u00b6\u0000\u0000\u0953\u0955\u0003\u0178\u00bc"+
"\u0000\u0954\u0956\u0003\u0130\u0098\u0000\u0955\u0954\u0001\u0000\u0000"+
"\u0000\u0955\u0956\u0001\u0000\u0000\u0000\u0956\u0169\u0001\u0000\u0000"+
"\u0000\u0957\u0959\u0003\u0266\u0133\u0000\u0958\u0957\u0001\u0000\u0000"+
"\u0000\u0958\u0959\u0001\u0000\u0000\u0000\u0959\u095d\u0001\u0000\u0000"+
"\u0000\u095a\u095b\u0005}\u0000\u0000\u095b\u095c\u0005\u00b0\u0000\u0000"+
"\u095c\u095e\u0005f\u0000\u0000\u095d\u095a\u0001\u0000\u0000\u0000\u095d"+
"\u095e\u0001\u0000\u0000\u0000\u095e\u095f\u0001\u0000\u0000\u0000\u095f"+
"\u0962\u0005m\u0000\u0000\u0960\u0963\u0003\u016c\u00b6\u0000\u0961\u0963"+
"\u0003\u016e\u00b7\u0000\u0962\u0960\u0001\u0000\u0000\u0000\u0962\u0961"+
"\u0001\u0000\u0000\u0000\u0963\u0964\u0001\u0000\u0000\u0000\u0964\u0965"+
"\u0005\u00b6\u0000\u0000\u0965\u0966\u0005X\u0000\u0000\u0966\u0967\u0005"+
"\u008f\u0000\u0000\u0967\u0968\u0003\u017a\u00bd\u0000\u0968\u096a\u0005"+
"\u00d3\u0000\u0000\u0969\u096b\u0003\u0130\u0098\u0000\u096a\u0969\u0001"+
"\u0000\u0000\u0000\u096a\u096b\u0001\u0000\u0000\u0000\u096b\u016b\u0001"+
"\u0000\u0000\u0000\u096c\u096d\u0005\u0098\u0000\u0000\u096d\u096e\u0003"+
"\u0110\u0088\u0000\u096e\u096f\u0005-\u0000\u0000\u096f\u0974\u0003\u0280"+
"\u0140\u0000\u0970\u0971\u0005\u001d\u0000\u0000\u0971\u0973\u0003\u0280"+
"\u0140\u0000\u0972\u0970\u0001\u0000\u0000\u0000\u0973\u0976\u0001\u0000"+
"\u0000\u0000\u0974\u0972\u0001\u0000\u0000\u0000\u0974\u0975\u0001\u0000"+
"\u0000\u0000\u0975\u0977\u0001\u0000\u0000\u0000\u0976\u0974\u0001\u0000"+
"\u0000\u0000\u0977\u0978\u0005\u00ea\u0000\u0000\u0978\u016d\u0001\u0000"+
"\u0000\u0000\u0979\u097a\u0005\u0098\u0000\u0000\u097a\u097c\u0005\u00ea"+
"\u0000\u0000\u097b\u097d\u0003\u008eG\u0000\u097c\u097b\u0001\u0000\u0000"+
"\u0000\u097c\u097d\u0001\u0000\u0000\u0000\u097d\u097e\u0001\u0000\u0000"+
"\u0000\u097e\u097f\u0003\u0090H\u0000\u097f\u0980\u0005\u008f\u0000\u0000"+
"\u0980\u0981\u0003\u0110\u0088\u0000\u0981\u0982\u0005-\u0000\u0000\u0982"+
"\u0987\u0003\u0280\u0140\u0000\u0983\u0984\u0005\u001d\u0000\u0000\u0984"+
"\u0986\u0003\u0280\u0140\u0000\u0985\u0983\u0001\u0000\u0000\u0000\u0986"+
"\u0989\u0001\u0000\u0000\u0000\u0987\u0985\u0001\u0000\u0000\u0000\u0987"+
"\u0988\u0001\u0000\u0000\u0000\u0988\u098a\u0001\u0000\u0000\u0000\u0989"+
"\u0987\u0001\u0000\u0000\u0000\u098a\u098b\u0005\u00d3\u0000\u0000\u098b"+
"\u098d\u0003\u0090H\u0000\u098c\u098e\u0003\u0092I\u0000\u098d\u098c\u0001"+
"\u0000\u0000\u0000\u098d\u098e\u0001\u0000\u0000\u0000\u098e\u098f\u0001"+
"\u0000\u0000\u0000\u098f\u0990\u0005\u0098\u0000\u0000\u0990\u0991\u0005"+
"\u00ea\u0000\u0000\u0991\u016f\u0001\u0000\u0000\u0000\u0992\u0994\u0003"+
"\u0266\u0133\u0000\u0993\u0992\u0001\u0000\u0000\u0000\u0993\u0994\u0001"+
"\u0000\u0000\u0000\u0994\u0998\u0001\u0000\u0000\u0000\u0995\u0996\u0005"+
"}\u0000\u0000\u0996\u0997\u0005\u00b0\u0000\u0000\u0997\u0999\u0005f\u0000"+
"\u0000\u0998\u0995\u0001\u0000\u0000\u0000\u0998\u0999\u0001\u0000\u0000"+
"\u0000\u0999\u099a\u0001\u0000\u0000\u0000\u099a\u099d\u0005m\u0000\u0000"+
"\u099b\u099e\u0003\u0172\u00b9\u0000\u099c\u099e\u0003\u0174\u00ba\u0000"+
"\u099d\u099b\u0001\u0000\u0000\u0000\u099d\u099c\u0001\u0000\u0000\u0000"+
"\u099e\u099f\u0001\u0000\u0000\u0000\u099f\u09a0\u0003\u0280\u0140\u0000"+
"\u09a0\u09a1\u0005\u0098\u0000\u0000\u09a1\u09a2\u0003\u0110\u0088\u0000"+
"\u09a2\u09a4\u0005\u00ea\u0000\u0000\u09a3\u09a5\u0003\u0130\u0098\u0000"+
"\u09a4\u09a3\u0001\u0000\u0000\u0000\u09a4\u09a5\u0001\u0000\u0000\u0000"+
"\u09a5\u0171\u0001\u0000\u0000\u0000\u09a6\u09a7\u0005\u0098\u0000\u0000"+
"\u09a7\u09a8\u0003\u0110\u0088\u0000\u09a8\u09a9\u0005\u00ea\u0000\u0000"+
"\u09a9\u09aa\u0005\u00b6\u0000\u0000\u09aa\u09ab\u0005X\u0000\u0000\u09ab"+
"\u0173\u0001\u0000\u0000\u0000\u09ac\u09ad\u0005\u0098\u0000\u0000\u09ad"+
"\u09af\u0005\u00ea\u0000\u0000\u09ae\u09b0\u0003\u008eG\u0000\u09af\u09ae"+
"\u0001\u0000\u0000\u0000\u09af\u09b0\u0001\u0000\u0000\u0000\u09b0\u09b1"+
"\u0001\u0000\u0000\u0000\u09b1\u09b2\u0003\u0090H\u0000\u09b2\u09b3\u0005"+
"\u008f\u0000\u0000\u09b3\u09b4\u0003\u0110\u0088\u0000\u09b4\u09b5\u0005"+
"\u00d3\u0000\u0000\u09b5\u09b7\u0003\u0090H\u0000\u09b6\u09b8\u0003\u0092"+
"I\u0000\u09b7\u09b6\u0001\u0000\u0000\u0000\u09b7\u09b8\u0001\u0000\u0000"+
"\u0000\u09b8\u09b9\u0001\u0000\u0000\u0000\u09b9\u09ba\u0005\u0098\u0000"+
"\u0000\u09ba\u09bb\u0005\u00ea\u0000\u0000\u09bb\u09bd\u0005\u00b6\u0000"+
"\u0000\u09bc\u09be\u0005X\u0000\u0000\u09bd\u09bc\u0001\u0000\u0000\u0000"+
"\u09bd\u09be\u0001\u0000\u0000\u0000\u09be\u0175\u0001\u0000\u0000\u0000"+
"\u09bf\u09c0\u0005\u0081\u0000\u0000\u09c0\u09c3\u0003\u0266\u0133\u0000"+
"\u09c1\u09c2\u0005}\u0000\u0000\u09c2\u09c4\u0005f\u0000\u0000\u09c3\u09c1"+
"\u0001\u0000\u0000\u0000\u09c3\u09c4\u0001\u0000\u0000\u0000\u09c4\u0177"+
"\u0001\u0000\u0000\u0000\u09c5\u09c6\u0003\u0110\u0088\u0000\u09c6\u09c7"+
"\u0003\u00c8d\u0000\u09c7\u09cd\u0001\u0000\u0000\u0000\u09c8\u09c9\u0005"+
"\u0098\u0000\u0000\u09c9\u09ca\u0003\u017a\u00bd\u0000\u09ca\u09cb\u0005"+
"\u00ea\u0000\u0000\u09cb\u09cd\u0001\u0000\u0000\u0000\u09cc\u09c5\u0001"+
"\u0000\u0000\u0000\u09cc\u09c8\u0001\u0000\u0000\u0000\u09cd\u0179\u0001"+
"\u0000\u0000\u0000\u09ce\u09cf\u0003\u0110\u0088\u0000\u09cf\u09d6\u0003"+
"\u00c8d\u0000\u09d0\u09d1\u0005/\u0000\u0000\u09d1\u09d2\u0003\u0110\u0088"+
"\u0000\u09d2\u09d3\u0003\u00c8d\u0000\u09d3\u09d5\u0001\u0000\u0000\u0000"+
"\u09d4\u09d0\u0001\u0000\u0000\u0000\u09d5\u09d8\u0001\u0000\u0000\u0000"+
"\u09d6\u09d4\u0001\u0000\u0000\u0000\u09d6\u09d7\u0001\u0000\u0000\u0000"+
"\u09d7\u017b\u0001\u0000\u0000\u0000\u09d8\u09d6\u0001\u0000\u0000\u0000"+
"\u09d9\u09df\u0005\u0013\u0000\u0000\u09da\u09e0\u0003\u0258\u012c\u0000"+
"\u09db\u09e0\u0003\u01ae\u00d7\u0000\u09dc\u09e0\u0003\u023e\u011f\u0000"+
"\u09dd\u09e0\u0003\u01b0\u00d8\u0000\u09de\u09e0\u0003\u018e\u00c7\u0000"+
"\u09df\u09da\u0001\u0000\u0000\u0000\u09df\u09db\u0001\u0000\u0000\u0000"+
"\u09df\u09dc\u0001\u0000\u0000\u0000\u09df\u09dd\u0001\u0000\u0000\u0000"+
"\u09df\u09de\u0001\u0000\u0000\u0000\u09e0\u017d\u0001\u0000\u0000\u0000"+
"\u09e1\u09e5\u0005\u00d8\u0000\u0000\u09e2\u09e6\u0003\u01a0\u00d0\u0000"+
"\u09e3\u09e6\u0003\u0190\u00c8\u0000\u09e4\u09e6\u0003\u01ac\u00d6\u0000"+
"\u09e5\u09e2\u0001\u0000\u0000\u0000\u09e5\u09e3\u0001\u0000\u0000\u0000"+
"\u09e5\u09e4\u0001\u0000\u0000\u0000\u09e6\u017f\u0001\u0000\u0000\u0000"+
"\u09e7\u09f2\u0005t\u0000\u0000\u09e8\u09ea\u0005\u007f\u0000\u0000\u09e9"+
"\u09e8\u0001\u0000\u0000\u0000\u09e9\u09ea\u0001\u0000\u0000\u0000\u09ea"+
"\u09eb\u0001\u0000\u0000\u0000\u09eb\u09ec\u0003\u01d4\u00ea\u0000\u09ec"+
"\u09ed\u0005\u010d\u0000\u0000\u09ed\u09ee\u0003\u0188\u00c4\u0000\u09ee"+
"\u09f3\u0001\u0000\u0000\u0000\u09ef\u09f0\u0003\u018a\u00c5\u0000\u09f0"+
"\u09f1\u0003\u01a4\u00d2\u0000\u09f1\u09f3\u0001\u0000\u0000\u0000\u09f2"+
"\u09e9\u0001\u0000\u0000\u0000\u09f2\u09ef\u0001\u0000\u0000\u0000\u09f3"+
"\u0181\u0001\u0000\u0000\u0000\u09f4\u09f6\u0005G\u0000\u0000\u09f5\u09f7"+
"\u0005\u007f\u0000\u0000\u09f6\u09f5\u0001\u0000\u0000\u0000\u09f6\u09f7"+
"\u0001\u0000\u0000\u0000\u09f7\u09f8\u0001\u0000\u0000\u0000\u09f8\u09f9"+
"\u0003\u01d4\u00ea\u0000\u09f9\u09fa\u0005\u010d\u0000\u0000\u09fa\u09fb"+
"\u0003\u0188\u00c4\u0000\u09fb\u0183\u0001\u0000\u0000\u0000\u09fc\u0a0a"+
"\u0005\u00e5\u0000\u0000\u09fd\u09ff\u0007#\u0000\u0000\u09fe\u09fd\u0001"+
"\u0000\u0000\u0000\u09fe\u09ff\u0001\u0000\u0000\u0000\u09ff\u0a01\u0001"+
"\u0000\u0000\u0000\u0a00\u0a02\u0005\u007f\u0000\u0000\u0a01\u0a00\u0001"+
"\u0000\u0000\u0000\u0a01\u0a02\u0001\u0000\u0000\u0000\u0a02\u0a03\u0001"+
"\u0000\u0000\u0000\u0a03\u0a04\u0003\u01d4\u00ea\u0000\u0a04\u0a05\u0005"+
"o\u0000\u0000\u0a05\u0a06\u0003\u0188\u00c4\u0000\u0a06\u0a0b\u0001\u0000"+
"\u0000\u0000\u0a07\u0a08\u0003\u018a\u00c5\u0000\u0a08\u0a09\u0003\u01a6"+
"\u00d3\u0000\u0a09\u0a0b\u0001\u0000\u0000\u0000\u0a0a\u09fe\u0001\u0000"+
"\u0000\u0000\u0a0a\u0a07\u0001\u0000\u0000\u0000\u0a0b\u0185\u0001\u0000"+
"\u0000\u0000\u0a0c\u0a0d\u0003\u026a\u0135\u0000\u0a0d\u0187\u0001\u0000"+
"\u0000\u0000\u0a0e\u0a0f\u0003\u026a\u0135\u0000\u0a0f\u0189\u0001\u0000"+
"\u0000\u0000\u0a10\u0a11\u0007$\u0000\u0000\u0a11\u018b\u0001\u0000\u0000"+
"\u0000\u0a12\u0a13\u0005Z\u0000\u0000\u0a13\u0a14\u0005\u00f3\u0000\u0000"+
"\u0a14\u0a16\u0003\u027a\u013d\u0000\u0a15\u0a17\u0003\u0130\u0098\u0000"+
"\u0a16\u0a15\u0001\u0000\u0000\u0000\u0a16\u0a17\u0001\u0000\u0000\u0000"+
"\u0a17\u018d\u0001\u0000\u0000\u0000\u0a18\u0a19\u0005\u00f3\u0000\u0000"+
"\u0a19\u0a1a\u0003\u027a\u013d\u0000\u0a1a\u0a1b\u0005\u00f5\u0000\u0000"+
"\u0a1b\u0a1c\u0003\u0130\u0098\u0000\u0a1c\u018f\u0001\u0000\u0000\u0000"+
"\u0a1d\u0a1e\u0005\u00f3\u0000\u0000\u0a1e\u0a1f\u0003\u027a\u013d\u0000"+
"\u0a1f\u0a20\u0005\u010d\u0000\u0000\u0a20\u0a21\u0003\u027a\u013d\u0000"+
"\u0a21\u0191\u0001\u0000\u0000\u0000\u0a22\u0a23\u0005\u00f3\u0000\u0000"+
"\u0a23\u0a24\u0003\u027a\u013d\u0000\u0a24\u0193\u0001\u0000\u0000\u0000"+
"\u0a25\u0a27\u0007%\u0000\u0000\u0a26\u0a28\u0003\u0126\u0093\u0000\u0a27"+
"\u0a26\u0001\u0000\u0000\u0000\u0a27\u0a28\u0001\u0000\u0000\u0000\u0a28"+
"\u0195\u0001\u0000\u0000\u0000\u0a29\u0a2b\u0005U\u0000\u0000\u0a2a\u0a29"+
"\u0001\u0000\u0000\u0000\u0a2a\u0a2b\u0001\u0000\u0000\u0000\u0a2b\u0a2e"+
"\u0001\u0000\u0000\u0000\u0a2c\u0a2f\u0003\u0198\u00cc\u0000\u0a2d\u0a2f"+
"\u0003\u019a\u00cd\u0000\u0a2e\u0a2c\u0001\u0000\u0000\u0000\u0a2e\u0a2d"+
"\u0001\u0000\u0000\u0000\u0a2f\u0197\u0001\u0000\u0000\u0000\u0a30\u0a31"+
"\u0005C\u0000\u0000\u0a31\u0a32\u0007&\u0000\u0000\u0a32\u0a33\u0005o"+
"\u0000\u0000\u0a33\u0a34\u0007%\u0000\u0000\u0a34\u0a39\u0003\u027a\u013d"+
"\u0000\u0a35\u0a36\u0005/\u0000\u0000\u0a36\u0a38\u0003\u027a\u013d\u0000"+
"\u0a37\u0a35\u0001\u0000\u0000\u0000\u0a38\u0a3b\u0001\u0000\u0000\u0000"+
"\u0a39\u0a37\u0001\u0000\u0000\u0000\u0a39\u0a3a\u0001\u0000\u0000\u0000"+
"\u0a3a\u0199\u0001\u0000\u0000\u0000\u0a3b\u0a39\u0001\u0000\u0000\u0000"+
"\u0a3c\u0a3d\u0005\u00d6\u0000\u0000\u0a3d\u0a3e\u0007&\u0000\u0000\u0a3e"+
"\u019b\u0001\u0000\u0000\u0000\u0a3f\u0a40\u0005\u00e6\u0000\u0000\u0a40"+
"\u0a44\u0003\u0268\u0134\u0000\u0a41\u0a42\u0005}\u0000\u0000\u0a42\u0a43"+
"\u0005\u00b0\u0000\u0000\u0a43\u0a45\u0005f\u0000\u0000\u0a44\u0a41\u0001"+
"\u0000\u0000\u0000\u0a44\u0a45\u0001\u0000\u0000\u0000\u0a45\u0a4a\u0001"+
"\u0000\u0000\u0000\u0a46\u0a47\u0005\u0017\u0000\u0000\u0a47\u0a48\u0005"+
"7\u0000\u0000\u0a48\u0a49\u0005\u00b4\u0000\u0000\u0a49\u0a4b\u0003\u0268"+
"\u0134\u0000\u0a4a\u0a46\u0001\u0000\u0000\u0000\u0a4a\u0a4b\u0001\u0000"+
"\u0000\u0000\u0a4b\u019d\u0001\u0000\u0000\u0000\u0a4c\u0a4d\u0005\u00e6"+
"\u0000\u0000\u0a4d\u0a50\u0003\u0268\u0134\u0000\u0a4e\u0a4f\u0005}\u0000"+
"\u0000\u0a4f\u0a51\u0005f\u0000\u0000\u0a50\u0a4e\u0001\u0000\u0000\u0000"+
"\u0a50\u0a51\u0001\u0000\u0000\u0000\u0a51\u019f\u0001\u0000\u0000\u0000"+
"\u0a52\u0a53\u0005\u00e6\u0000\u0000\u0a53\u0a56\u0003\u0268\u0134\u0000"+
"\u0a54\u0a55\u0005}\u0000\u0000\u0a55\u0a57\u0005f\u0000\u0000\u0a56\u0a54"+
"\u0001\u0000\u0000\u0000\u0a56\u0a57\u0001\u0000\u0000\u0000\u0a57\u0a58"+
"\u0001\u0000\u0000\u0000\u0a58\u0a59\u0005\u010d\u0000\u0000\u0a59\u0a5a"+
"\u0003\u0268\u0134\u0000\u0a5a\u01a1\u0001\u0000\u0000\u0000\u0a5b\u0a5d"+
"\u0007\'\u0000\u0000\u0a5c\u0a5b\u0001\u0000\u0000\u0000\u0a5c\u0a5d\u0001"+
"\u0000\u0000\u0000\u0a5d\u0a5e\u0001\u0000\u0000\u0000\u0a5e\u0a61\u0003"+
"\u018a\u00c5\u0000\u0a5f\u0a60\u0005\u0128\u0000\u0000\u0a60\u0a62\u0007"+
"(\u0000\u0000\u0a61\u0a5f\u0001\u0000\u0000\u0000\u0a61\u0a62\u0001\u0000"+
"\u0000\u0000\u0a62\u0a64\u0001\u0000\u0000\u0000\u0a63\u0a65\u0003\u0126"+
"\u0093\u0000\u0a64\u0a63\u0001\u0000\u0000\u0000\u0a64\u0a65\u0001\u0000"+
"\u0000\u0000\u0a65\u01a3\u0001\u0000\u0000\u0000\u0a66\u0a67\u0003\u0188"+
"\u00c4\u0000\u0a67\u0a68\u0005\u010d\u0000\u0000\u0a68\u0a69\u0003\u0186"+
"\u00c3\u0000\u0a69\u01a5\u0001\u0000\u0000\u0000\u0a6a\u0a6b\u0003\u0188"+
"\u00c4\u0000\u0a6b\u0a6c\u0005o\u0000\u0000\u0a6c\u0a6d\u0003\u0186\u00c3"+
"\u0000\u0a6d\u01a7\u0001\u0000\u0000\u0000\u0a6e\u0a6f\u0005\u011e\u0000"+
"\u0000\u0a6f\u0a73\u0003\u0268\u0134\u0000\u0a70\u0a71\u0005}\u0000\u0000"+
"\u0a71\u0a72\u0005\u00b0\u0000\u0000\u0a72\u0a74\u0005f\u0000\u0000\u0a73"+
"\u0a70\u0001\u0000\u0000\u0000\u0a73\u0a74\u0001\u0000\u0000\u0000\u0a74"+
"\u0a7e\u0001\u0000\u0000\u0000\u0a75\u0a7c\u0005\u00f5\u0000\u0000\u0a76"+
"\u0a7d\u0003\u01b4\u00da\u0000\u0a77\u0a78\u0005\u00bd\u0000\u0000\u0a78"+
"\u0a7d\u0003\u01ba\u00dd\u0000\u0a79\u0a7d\u0003\u01bc\u00de\u0000\u0a7a"+
"\u0a7d\u0003\u01be\u00df\u0000\u0a7b\u0a7d\u0003\u01c0\u00e0\u0000\u0a7c"+
"\u0a76\u0001\u0000\u0000\u0000\u0a7c\u0a77\u0001\u0000\u0000\u0000\u0a7c"+
"\u0a79\u0001\u0000\u0000\u0000\u0a7c\u0a7a\u0001\u0000\u0000\u0000\u0a7c"+
"\u0a7b\u0001\u0000\u0000\u0000\u0a7d\u0a7f\u0001\u0000\u0000\u0000\u0a7e"+
"\u0a75\u0001\u0000\u0000\u0000\u0a7f\u0a80\u0001\u0000\u0000\u0000\u0a80"+
"\u0a7e\u0001\u0000\u0000\u0000\u0a80\u0a81\u0001\u0000\u0000\u0000\u0a81"+
"\u01a9\u0001\u0000\u0000\u0000\u0a82\u0a83\u0005\u011e\u0000\u0000\u0a83"+
"\u0a86\u0003\u0268\u0134\u0000\u0a84\u0a85\u0005}\u0000\u0000\u0a85\u0a87"+
"\u0005f\u0000\u0000\u0a86\u0a84\u0001\u0000\u0000\u0000\u0a86\u0a87\u0001"+
"\u0000\u0000\u0000\u0a87\u01ab\u0001\u0000\u0000\u0000\u0a88\u0a89\u0005"+
"\u011e\u0000\u0000\u0a89\u0a8c\u0003\u0268\u0134\u0000\u0a8a\u0a8b\u0005"+
"}\u0000\u0000\u0a8b\u0a8d\u0005f\u0000\u0000\u0a8c\u0a8a\u0001\u0000\u0000"+
"\u0000\u0a8c\u0a8d\u0001\u0000\u0000\u0000\u0a8d\u0a8e\u0001\u0000\u0000"+
"\u0000\u0a8e\u0a8f\u0005\u010d\u0000\u0000\u0a8f\u0a90\u0003\u0268\u0134"+
"\u0000\u0a90\u01ad\u0001\u0000\u0000\u0000\u0a91\u0a92\u0005<\u0000\u0000"+
"\u0a92\u0a93\u0005\u011e\u0000\u0000\u0a93\u0a94\u0005\u00f5\u0000\u0000"+
"\u0a94\u0a95\u0005\u00bd\u0000\u0000\u0a95\u0a96\u0005o\u0000\u0000\u0a96"+
"\u0a97\u0003\u01b8\u00dc\u0000\u0a97\u0a98\u0005\u010d\u0000\u0000\u0a98"+
"\u0a99\u0003\u01b8\u00dc\u0000\u0a99\u01af\u0001\u0000\u0000\u0000\u0a9a"+
"\u0a9b\u0005\u011e\u0000\u0000\u0a9b\u0a9e\u0003\u0268\u0134\u0000\u0a9c"+
"\u0a9d\u0005}\u0000\u0000\u0a9d\u0a9f\u0005f\u0000\u0000\u0a9e\u0a9c\u0001"+
"\u0000\u0000\u0000\u0a9e\u0a9f\u0001\u0000\u0000\u0000\u0a9f\u0aad\u0001"+
"\u0000\u0000\u0000\u0aa0\u0aa9\u0005\u00dd\u0000\u0000\u0aa1\u0aa2\u0005"+
"{\u0000\u0000\u0aa2\u0aaa\u0005>\u0000\u0000\u0aa3\u0aa4\u0005\u0012\u0000"+
"\u0000\u0aa4\u0aa6\u0005\u001c\u0000\u0000\u0aa5\u0aa7\u0007)\u0000\u0000"+
"\u0aa6\u0aa5\u0001\u0000\u0000\u0000\u0aa6\u0aa7\u0001\u0000\u0000\u0000"+
"\u0aa7\u0aaa\u0001\u0000\u0000\u0000\u0aa8\u0aaa\u0003\u01b2\u00d9\u0000"+
"\u0aa9\u0aa1\u0001\u0000\u0000\u0000\u0aa9\u0aa3\u0001\u0000\u0000\u0000"+
"\u0aa9\u0aa8\u0001\u0000\u0000\u0000\u0aaa\u0aac\u0001\u0000\u0000\u0000"+
"\u0aab\u0aa0\u0001\u0000\u0000\u0000\u0aac\u0aaf\u0001\u0000\u0000\u0000"+
"\u0aad\u0aab\u0001\u0000\u0000\u0000\u0aad\u0aae\u0001\u0000\u0000\u0000"+
"\u0aae\u0abb\u0001\u0000\u0000\u0000\u0aaf\u0aad\u0001\u0000\u0000\u0000"+
"\u0ab0\u0ab7\u0005\u00f5\u0000\u0000\u0ab1\u0ab8\u0003\u01b4\u00da\u0000"+
"\u0ab2\u0ab3\u0005\u00bd\u0000\u0000\u0ab3\u0ab8\u0003\u01ba\u00dd\u0000"+
"\u0ab4\u0ab8\u0003\u01bc\u00de\u0000\u0ab5\u0ab8\u0003\u01be\u00df\u0000"+
"\u0ab6\u0ab8\u0003\u01c0\u00e0\u0000\u0ab7\u0ab1\u0001\u0000\u0000\u0000"+
"\u0ab7\u0ab2\u0001\u0000\u0000\u0000\u0ab7\u0ab4\u0001\u0000\u0000\u0000"+
"\u0ab7\u0ab5\u0001\u0000\u0000\u0000\u0ab7\u0ab6\u0001\u0000\u0000\u0000"+
"\u0ab8\u0aba\u0001\u0000\u0000\u0000\u0ab9\u0ab0\u0001\u0000\u0000\u0000"+
"\u0aba\u0abd\u0001\u0000\u0000\u0000\u0abb\u0ab9\u0001\u0000\u0000\u0000"+
"\u0abb\u0abc\u0001\u0000\u0000\u0000\u0abc\u01b1\u0001\u0000\u0000\u0000"+
"\u0abd\u0abb\u0001\u0000\u0000\u0000\u0abe\u0ac0\u0005\u001c\u0000\u0000"+
"\u0abf\u0ac1\u0007)\u0000\u0000\u0ac0\u0abf\u0001\u0000\u0000\u0000\u0ac0"+
"\u0ac1\u0001\u0000\u0000\u0000\u0ac1\u0ac5\u0001\u0000\u0000\u0000\u0ac2"+
"\u0ac6\u0003\u0276\u013b\u0000\u0ac3\u0ac6\u0003\u0272\u0139\u0000\u0ac4"+
"\u0ac6\u0003\u0104\u0082\u0000\u0ac5\u0ac2\u0001\u0000\u0000\u0000\u0ac5"+
"\u0ac3\u0001\u0000\u0000\u0000\u0ac5\u0ac4\u0001\u0000\u0000\u0000\u0ac6"+
"\u01b3\u0001\u0000\u0000\u0000\u0ac7\u0ac9\u0007*\u0000\u0000\u0ac8\u0ac7"+
"\u0001\u0000\u0000\u0000\u0ac8\u0ac9\u0001\u0000\u0000\u0000\u0ac9\u0aca"+
"\u0001\u0000\u0000\u0000\u0aca\u0acb\u0005\u00bd\u0000\u0000\u0acb\u0acd"+
"\u0003\u01b8\u00dc\u0000\u0acc\u0ace\u0003\u01ba\u00dd\u0000\u0acd\u0acc"+
"\u0001\u0000\u0000\u0000\u0acd\u0ace\u0001\u0000\u0000\u0000\u0ace\u01b5"+
"\u0001\u0000\u0000\u0000\u0acf\u0ad1\u0007*\u0000\u0000\u0ad0\u0acf\u0001"+
"\u0000\u0000\u0000\u0ad0\u0ad1\u0001\u0000\u0000\u0000\u0ad1\u0ad2\u0001"+
"\u0000\u0000\u0000\u0ad2\u0ad3\u0005\u00bd\u0000\u0000\u0ad3\u0ad4\u0003"+
"\u01b8\u00dc\u0000\u0ad4\u01b7\u0001\u0000\u0000\u0000\u0ad5\u0ad8\u0003"+
"\u0276\u013b\u0000\u0ad6\u0ad8\u0003\u0104\u0082\u0000\u0ad7\u0ad5\u0001"+
"\u0000\u0000\u0000\u0ad7\u0ad6\u0001\u0000\u0000\u0000\u0ad8\u01b9\u0001"+
"\u0000\u0000\u0000\u0ad9\u0adb\u0005*\u0000\u0000\u0ada\u0adc\u0005\u00b0"+
"\u0000\u0000\u0adb\u0ada\u0001\u0000\u0000\u0000\u0adb\u0adc\u0001\u0000"+
"\u0000\u0000\u0adc\u0add\u0001\u0000\u0000\u0000\u0add\u0ade\u0005\u00e2"+
"\u0000\u0000\u0ade\u01bb\u0001\u0000\u0000\u0000\u0adf\u0ae0\u0005\u0100"+
"\u0000\u0000\u0ae0\u0ae1\u0007+\u0000\u0000\u0ae1\u01bd\u0001\u0000\u0000"+
"\u0000\u0ae2\u0ae3\u0005{\u0000\u0000\u0ae3\u0ae4\u0005>\u0000\u0000\u0ae4"+
"\u0ae5\u0003\u026e\u0137\u0000\u0ae5\u01bf\u0001\u0000\u0000\u0000\u0ae6"+
"\u0ae8\u0005\u001c\u0000\u0000\u0ae7\u0ae9\u0005\u00cf\u0000\u0000\u0ae8"+
"\u0ae7\u0001\u0000\u0000\u0000\u0ae8\u0ae9\u0001\u0000\u0000\u0000\u0ae9"+
"\u0aea\u0001\u0000\u0000\u0000\u0aea\u0aeb\u0003\u0276\u013b\u0000\u0aeb"+
"\u0aee\u0005\u0090\u0000\u0000\u0aec\u0aed\u0005\u00f5\u0000\u0000\u0aed"+
"\u0aef\u0003\u01c2\u00e1\u0000\u0aee\u0aec\u0001\u0000\u0000\u0000\u0aef"+
"\u0af0\u0001\u0000\u0000\u0000\u0af0\u0aee\u0001\u0000\u0000\u0000\u0af0"+
"\u0af1\u0001\u0000\u0000\u0000\u0af1\u0af2\u0001\u0000\u0000\u0000\u0af2"+
"\u0af3\u0005\u00d4\u0000\u0000\u0af3\u01c1\u0001\u0000\u0000\u0000\u0af4"+
"\u0af5\u0005|\u0000\u0000\u0af5\u0afa\u0003\u0278\u013c\u0000\u0af6\u0afa"+
"\u0003\u01b6\u00db\u0000\u0af7\u0af8\u0005\u00bd\u0000\u0000\u0af8\u0afa"+
"\u0003\u01ba\u00dd\u0000\u0af9\u0af4\u0001\u0000\u0000\u0000\u0af9\u0af6"+
"\u0001\u0000\u0000\u0000\u0af9\u0af7\u0001\u0000\u0000\u0000\u0afa\u01c3"+
"\u0001\u0000\u0000\u0000\u0afb\u0afe\u0007(\u0000\u0000\u0afc\u0afd\u0005"+
"\u0128\u0000\u0000\u0afd\u0aff\u0005\u001c\u0000\u0000\u0afe\u0afc\u0001"+
"\u0000\u0000\u0000\u0afe\u0aff\u0001\u0000\u0000\u0000\u0aff\u0b01\u0001"+
"\u0000\u0000\u0000\u0b00\u0b02\u0003\u0126\u0093\u0000\u0b01\u0b00\u0001"+
"\u0000\u0000\u0000\u0b01\u0b02\u0001\u0000\u0000\u0000\u0b02\u01c5\u0001"+
"\u0000\u0000\u0000\u0b03\u0b04\u0005<\u0000\u0000\u0b04\u0b06\u0005\u011e"+
"\u0000\u0000\u0b05\u0b07\u0003\u0126\u0093\u0000\u0b06\u0b05\u0001\u0000"+
"\u0000\u0000\u0b06\u0b07\u0001\u0000\u0000\u0000\u0b07\u01c7\u0001\u0000"+
"\u0000\u0000\u0b08\u0b09\u0005\u0103\u0000\u0000\u0b09\u0b0b\u0003\u01d2"+
"\u00e9\u0000\u0b0a\u0b0c\u0003\u0126\u0093\u0000\u0b0b\u0b0a\u0001\u0000"+
"\u0000\u0000\u0b0b\u0b0c\u0001\u0000\u0000\u0000\u0b0c\u01c9\u0001\u0000"+
"\u0000\u0000\u0b0d\u0b0f\u0005\u0012\u0000\u0000\u0b0e\u0b0d\u0001\u0000"+
"\u0000\u0000\u0b0e\u0b0f\u0001\u0000\u0000\u0000\u0b0f\u0b10\u0001\u0000"+
"\u0000\u0000\u0b10\u0b12\u0003\u01d2\u00e9\u0000\u0b11\u0b13\u0003\u01d0"+
"\u00e8\u0000\u0b12\u0b11\u0001\u0000\u0000\u0000\u0b12\u0b13\u0001\u0000"+
"\u0000\u0000\u0b13\u0b15\u0001\u0000\u0000\u0000\u0b14\u0b16\u0003\u0126"+
"\u0093\u0000\u0b15\u0b14\u0001\u0000\u0000\u0000\u0b15\u0b16\u0001\u0000"+
"\u0000\u0000\u0b16\u01cb\u0001\u0000\u0000\u0000\u0b17\u0b18\u0007$\u0000"+
"\u0000\u0b18\u0b19\u0003\u0188\u00c4\u0000\u0b19\u0b1b\u0003\u01d2\u00e9"+
"\u0000\u0b1a\u0b1c\u0003\u01d0\u00e8\u0000\u0b1b\u0b1a\u0001\u0000\u0000"+
"\u0000\u0b1b\u0b1c\u0001\u0000\u0000\u0000\u0b1c\u0b1e\u0001\u0000\u0000"+
"\u0000\u0b1d\u0b1f\u0003\u0126\u0093\u0000\u0b1e\u0b1d\u0001\u0000\u0000"+
"\u0000\u0b1e\u0b1f\u0001\u0000\u0000\u0000\u0b1f\u01cd\u0001\u0000\u0000"+
"\u0000\u0b20\u0b22\u0007(\u0000\u0000\u0b21\u0b23\u0003\u0186\u00c3\u0000"+
"\u0b22\u0b21\u0001\u0000\u0000\u0000\u0b22\u0b23\u0001\u0000\u0000\u0000"+
"\u0b23\u0b24\u0001\u0000\u0000\u0000\u0b24\u0b26\u0003\u01d2\u00e9\u0000"+
"\u0b25\u0b27\u0003\u01d0\u00e8\u0000\u0b26\u0b25\u0001\u0000\u0000\u0000"+
"\u0b26\u0b27\u0001\u0000\u0000\u0000\u0b27\u0b29\u0001\u0000\u0000\u0000"+
"\u0b28\u0b2a\u0003\u0126\u0093\u0000\u0b29\u0b28\u0001\u0000\u0000\u0000"+
"\u0b29\u0b2a\u0001\u0000\u0000\u0000\u0b2a\u01cf\u0001\u0000\u0000\u0000"+
"\u0b2b\u0b2d\u0005\u0017\u0000\u0000\u0b2c\u0b2e\u0005\u00e5\u0000\u0000"+
"\u0b2d\u0b2c\u0001\u0000\u0000\u0000\u0b2d\u0b2e\u0001\u0000\u0000\u0000"+
"\u0b2e\u0b2f\u0001\u0000\u0000\u0000\u0b2f\u0b30\u0007,\u0000\u0000\u0b30"+
"\u01d1\u0001\u0000\u0000\u0000\u0b31\u0b32\u0007-\u0000\u0000\u0b32\u01d3"+
"\u0001\u0000\u0000\u0000\u0b33\u0b40\u0003\u01d6\u00eb\u0000\u0b34\u0b40"+
"\u0003\u01dc\u00ee\u0000\u0b35\u0b40\u0003\u01f6\u00fb\u0000\u0b36\u0b40"+
"\u0003\u01f8\u00fc\u0000\u0b37\u0b40\u0003\u01e8\u00f4\u0000\u0b38\u0b40"+
"\u0003\u01ea\u00f5\u0000\u0b39\u0b40\u0003\u0218\u010c\u0000\u0b3a\u0b40"+
"\u0003\u0216\u010b\u0000\u0b3b\u0b40\u0003\u01f2\u00f9\u0000\u0b3c\u0b40"+
"\u0003\u01ee\u00f7\u0000\u0b3d\u0b40\u0003\u01ec\u00f6\u0000\u0b3e\u0b40"+
"\u0003\u01f4\u00fa\u0000\u0b3f\u0b33\u0001\u0000\u0000\u0000\u0b3f\u0b34"+
"\u0001\u0000\u0000\u0000\u0b3f\u0b35\u0001\u0000\u0000\u0000\u0b3f\u0b36"+
"\u0001\u0000\u0000\u0000\u0b3f\u0b37\u0001\u0000\u0000\u0000\u0b3f\u0b38"+
"\u0001\u0000\u0000\u0000\u0b3f\u0b39\u0001\u0000\u0000\u0000\u0b3f\u0b3a"+
"\u0001\u0000\u0000\u0000\u0b3f\u0b3b\u0001\u0000\u0000\u0000\u0b3f\u0b3c"+
"\u0001\u0000\u0000\u0000\u0b3f\u0b3d\u0001\u0000\u0000\u0000\u0b3f\u0b3e"+
"\u0001\u0000\u0000\u0000\u0b40\u01d5\u0001\u0000\u0000\u0000\u0b41\u0b43"+
"\u0005\u0012\u0000\u0000\u0b42\u0b44\u0003\u01d8\u00ec\u0000\u0b43\u0b42"+
"\u0001\u0000\u0000\u0000\u0b43\u0b44\u0001\u0000\u0000\u0000\u0b44\u0b45"+
"\u0001\u0000\u0000\u0000\u0b45\u0b46\u0005\u00b6\u0000\u0000\u0b46\u0b47"+
"\u0003\u01da\u00ed\u0000\u0b47\u01d7\u0001\u0000\u0000\u0000\u0b48\u0b4a"+
"\u0007.\u0000\u0000\u0b49\u0b48\u0001\u0000\u0000\u0000\u0b49\u0b4a\u0001"+
"\u0000\u0000\u0000\u0b4a\u0b4b\u0001\u0000\u0000\u0000\u0b4b\u0b4c\u0005"+
"\u00ca\u0000\u0000\u0b4c\u01d9\u0001\u0000\u0000\u0000\u0b4d\u0b4e\u0007"+
"/\u0000\u0000\u0b4e\u0b5b\u00070\u0000\u0000\u0b4f\u0b52\u0007&\u0000"+
"\u0000\u0b50\u0b53\u0005\u010a\u0000\u0000\u0b51\u0b53\u0003\u026c\u0136"+
"\u0000\u0b52\u0b50\u0001\u0000\u0000\u0000\u0b52\u0b51\u0001\u0000\u0000"+
"\u0000\u0b53\u0b5b\u0001\u0000\u0000\u0000\u0b54\u0b57\u00071\u0000\u0000"+
"\u0b55\u0b58\u0005\u010a\u0000\u0000\u0b56\u0b58\u0003\u026c\u0136\u0000"+
"\u0b57\u0b55\u0001\u0000\u0000\u0000\u0b57\u0b56\u0001\u0000\u0000\u0000"+
"\u0b58\u0b5b\u0001\u0000\u0000\u0000\u0b59\u0b5b\u0005B\u0000\u0000\u0b5a"+
"\u0b4d\u0001\u0000\u0000\u0000\u0b5a\u0b4f\u0001\u0000\u0000\u0000\u0b5a"+
"\u0b54\u0001\u0000\u0000\u0000\u0b5a\u0b59\u0001\u0000\u0000\u0000\u0b5b"+
"\u01db\u0001\u0000\u0000\u0000\u0b5c\u0b69\u0005:\u0000\u0000\u0b5d\u0b5e"+
"\u0003\u01de\u00ef\u0000\u0b5e\u0b5f\u0005\u00b6\u0000\u0000\u0b5f\u0b60"+
"\u0003\u022a\u0115\u0000\u0b60\u0b6a\u0001\u0000\u0000\u0000\u0b61\u0b62"+
"\u0003\u01e6\u00f3\u0000\u0b62\u0b63\u0005\u00b6\u0000\u0000\u0b63\u0b64"+
"\u0005B\u0000\u0000\u0b64\u0b6a\u0001\u0000\u0000\u0000\u0b65\u0b66\u0005"+
"\u00b6\u0000\u0000\u0b66\u0b67\u0003\u022c\u0116\u0000\u0b67\u0b68\u0003"+
"\u0220\u0110\u0000\u0b68\u0b6a\u0001\u0000\u0000\u0000\u0b69\u0b5d\u0001"+
"\u0000\u0000\u0000\u0b69\u0b61\u0001\u0000\u0000\u0000\u0b69\u0b65\u0001"+
"\u0000\u0000\u0000\u0b6a\u01dd\u0001\u0000\u0000\u0000\u0b6b\u0b71\u0003"+
"\u0200\u0100\u0000\u0b6c\u0b71\u0003\u0202\u0101\u0000\u0b6d\u0b71\u0003"+
"\u01e0\u00f0\u0000\u0b6e\u0b71\u0003\u01e2\u00f1\u0000\u0b6f\u0b71\u0003"+
"\u01e4\u00f2\u0000\u0b70\u0b6b\u0001\u0000\u0000\u0000\u0b70\u0b6c\u0001"+
"\u0000\u0000\u0000\u0b70\u0b6d\u0001\u0000\u0000\u0000\u0b70\u0b6e\u0001"+
"\u0000\u0000\u0000\u0b70\u0b6f\u0001\u0000\u0000\u0000\u0b71\u01df\u0001"+
"\u0000\u0000\u0000\u0b72\u0b74\u0005\u00a9\u0000\u0000\u0b73\u0b75\u0005"+
"\u00aa\u0000\u0000\u0b74\u0b73\u0001\u0000\u0000\u0000\u0b74\u0b75\u0001"+
"\u0000\u0000\u0000\u0b75\u0b76\u0001\u0000\u0000\u0000\u0b76\u0b77\u0007"+
"2\u0000\u0000\u0b77\u01e1\u0001\u0000\u0000\u0000\u0b78\u0b7a\u0005\u00a9"+
"\u0000\u0000\u0b79\u0b7b\u0005\u00db\u0000\u0000\u0b7a\u0b79\u0001\u0000"+
"\u0000\u0000\u0b7a\u0b7b\u0001\u0000\u0000\u0000\u0b7b\u0b7c\u0001\u0000"+
"\u0000\u0000\u0b7c\u0b7d\u00073\u0000\u0000\u0b7d\u01e3\u0001\u0000\u0000"+
"\u0000\u0b7e\u0b80\u0005\u00a9\u0000\u0000\u0b7f\u0b81\u0005\u00ce\u0000"+
"\u0000\u0b80\u0b7f\u0001\u0000\u0000\u0000\u0b80\u0b81\u0001\u0000\u0000"+
"\u0000\u0b81\u0b82\u0001\u0000\u0000\u0000\u0b82\u0b83\u00074\u0000\u0000"+
"\u0b83\u01e5\u0001\u0000\u0000\u0000\u0b84\u0b8c\u0005\u000f\u0000\u0000"+
"\u0b85\u0b87\u00052\u0000\u0000\u0b86\u0b85\u0001\u0000\u0000\u0000\u0b86"+
"\u0b87\u0001\u0000\u0000\u0000\u0b87\u0b88\u0001\u0000\u0000\u0000\u0b88"+
"\u0b8c\u0005>\u0000\u0000\u0b89\u0b8c\u0005\u00e6\u0000\u0000\u0b8a\u0b8c"+
"\u0005\u011e\u0000\u0000\u0b8b\u0b84\u0001\u0000\u0000\u0000\u0b8b\u0b86"+
"\u0001\u0000\u0000\u0000\u0b8b\u0b89\u0001\u0000\u0000\u0000\u0b8b\u0b8a"+
"\u0001\u0000\u0000\u0000\u0b8c\u01e7\u0001\u0000\u0000\u0000\u0b8d\u0b99"+
"\u0005T\u0000\u0000\u0b8e\u0b91\u0003\u0200\u0100\u0000\u0b8f\u0b91\u0003"+
"\u0202\u0101\u0000\u0b90\u0b8e\u0001\u0000\u0000\u0000\u0b90\u0b8f\u0001"+
"\u0000\u0000\u0000\u0b91\u0b92\u0001\u0000\u0000\u0000\u0b92\u0b93\u0005"+
"\u00b6\u0000\u0000\u0b93\u0b94\u0003\u022a\u0115\u0000\u0b94\u0b9a\u0001"+
"\u0000\u0000\u0000\u0b95\u0b96\u0003\u01e6\u00f3\u0000\u0b96\u0b97\u0005"+
"\u00b6\u0000\u0000\u0b97\u0b98\u0005B\u0000\u0000\u0b98\u0b9a\u0001\u0000"+
"\u0000\u0000\u0b99\u0b90\u0001\u0000\u0000\u0000\u0b99\u0b95\u0001\u0000"+
"\u0000\u0000\u0b9a\u01e9\u0001\u0000\u0000\u0000\u0b9b\u0b9c\u0005\u0095"+
"\u0000\u0000\u0b9c\u0ba1\u0005\u00b6\u0000\u0000\u0b9d\u0b9e\u00075\u0000"+
"\u0000\u0b9e\u0ba2\u0003\u027a\u013d\u0000\u0b9f\u0ba0\u0005\u0012\u0000"+
"\u0000\u0ba0\u0ba2\u0005=\u0000\u0000\u0ba1\u0b9d\u0001\u0000\u0000\u0000"+
"\u0ba1\u0b9f\u0001\u0000\u0000\u0000\u0ba2\u01eb\u0001\u0000\u0000\u0000"+
"\u0ba3\u0bbc\u0005\u00fa\u0000\u0000\u0ba4\u0bab\u0003\u0200\u0100\u0000"+
"\u0ba5\u0bab\u0003\u0202\u0101\u0000\u0ba6\u0ba8\u0003\u0204\u0102\u0000"+
"\u0ba7\u0ba9\u0003\u0206\u0103\u0000\u0ba8\u0ba7\u0001\u0000\u0000\u0000"+
"\u0ba8\u0ba9\u0001\u0000\u0000\u0000\u0ba9\u0bab\u0001\u0000\u0000\u0000"+
"\u0baa\u0ba4\u0001\u0000\u0000\u0000\u0baa\u0ba5\u0001\u0000\u0000\u0000"+
"\u0baa\u0ba6\u0001\u0000\u0000\u0000\u0bab\u0bac\u0001\u0000\u0000\u0000"+
"\u0bac\u0bad\u0005\u00b6\u0000\u0000\u0bad\u0bae\u0003\u022a\u0115\u0000"+
"\u0bae\u0bbd\u0001\u0000\u0000\u0000\u0baf\u0bb9\u0005\u000f\u0000\u0000"+
"\u0bb0\u0bb9\u0005\u00c9\u0000\u0000\u0bb1\u0bb9\u0005\u00e6\u0000\u0000"+
"\u0bb2\u0bb9\u0005\u00f3\u0000\u0000\u0bb3\u0bb9\u0005\u00f4\u0000\u0000"+
"\u0bb4\u0bb5\u0003\u0156\u00ab\u0000\u0bb5\u0bb6\u0003\u020c\u0106\u0000"+
"\u0bb6\u0bb9\u0001\u0000\u0000\u0000\u0bb7\u0bb9\u0005\u011e\u0000\u0000"+
"\u0bb8\u0baf\u0001\u0000\u0000\u0000\u0bb8\u0bb0\u0001\u0000\u0000\u0000"+
"\u0bb8\u0bb1\u0001\u0000\u0000\u0000\u0bb8\u0bb2\u0001\u0000\u0000\u0000"+
"\u0bb8\u0bb3\u0001\u0000\u0000\u0000\u0bb8\u0bb4\u0001\u0000\u0000\u0000"+
"\u0bb8\u0bb7\u0001\u0000\u0000\u0000\u0bb9\u0bba\u0001\u0000\u0000\u0000"+
"\u0bba\u0bbb\u0005\u00b6\u0000\u0000\u0bbb\u0bbd\u0005B\u0000\u0000\u0bbc"+
"\u0baa\u0001\u0000\u0000\u0000\u0bbc\u0bb8\u0001\u0000\u0000\u0000\u0bbd"+
"\u01ed\u0001\u0000\u0000\u0000\u0bbe\u0bda\u0005\u00f5\u0000\u0000\u0bbf"+
"\u0bc9\u0003\u01f0\u00f8\u0000\u0bc0\u0bc4\u0005\u011e\u0000\u0000\u0bc1"+
"\u0bc5\u0005\u0100\u0000\u0000\u0bc2\u0bc3\u0005{\u0000\u0000\u0bc3\u0bc5"+
"\u0005>\u0000\u0000\u0bc4\u0bc1\u0001\u0000\u0000\u0000\u0bc4\u0bc2\u0001"+
"\u0000\u0000\u0000\u0bc5\u0bc9\u0001\u0000\u0000\u0000\u0bc6\u0bc7\u0005"+
">\u0000\u0000\u0bc7\u0bc9\u0005\u000b\u0000\u0000\u0bc8\u0bbf\u0001\u0000"+
"\u0000\u0000\u0bc8\u0bc0\u0001\u0000\u0000\u0000\u0bc8\u0bc6\u0001\u0000"+
"\u0000\u0000\u0bc9\u0bca\u0001\u0000\u0000\u0000\u0bca\u0bcb\u0005\u00b6"+
"\u0000\u0000\u0bcb\u0bdb\u0005B\u0000\u0000\u0bcc\u0bcd\u0005\u008b\u0000"+
"\u0000\u0bcd\u0bce\u0003\u021a\u010d\u0000\u0bce\u0bcf\u0005\u00b6\u0000"+
"\u0000\u0bcf\u0bd0\u0003\u022c\u0116\u0000\u0bd0\u0bdb\u0001\u0000\u0000"+
"\u0000\u0bd1\u0bd2\u0005\u00ce\u0000\u0000\u0bd2\u0bd3\u0003\u021c\u010e"+
"\u0000\u0bd3\u0bd4\u0005\u00b6\u0000\u0000\u0bd4\u0bd5\u0003\u022c\u0116"+
"\u0000\u0bd5\u0bd6\u0003\u0220\u0110\u0000\u0bd6\u0bdb\u0001\u0000\u0000"+
"\u0000\u0bd7\u0bd8\u0005\u001c\u0000\u0000\u0bd8\u0bd9\u0005\u00b6\u0000"+
"\u0000\u0bd9\u0bdb\u0005B\u0000\u0000\u0bda\u0bc8\u0001\u0000\u0000\u0000"+
"\u0bda\u0bcc\u0001\u0000\u0000\u0000\u0bda\u0bd1\u0001\u0000\u0000\u0000"+
"\u0bda\u0bd7\u0001\u0000\u0000\u0000\u0bdb\u01ef\u0001\u0000\u0000\u0000"+
"\u0bdc\u0bdd\u00076\u0000\u0000\u0bdd\u01f1\u0001\u0000\u0000\u0000\u0bde"+
"\u0be7\u0005\u00dd\u0000\u0000\u0bdf\u0be0\u00077\u0000\u0000\u0be0\u0be1"+
"\u0005\u00b6\u0000\u0000\u0be1\u0be8\u0005B\u0000\u0000\u0be2\u0be3\u0005"+
"\u008b\u0000\u0000\u0be3\u0be4\u0003\u021a\u010d\u0000\u0be4\u0be5\u0005"+
"\u00b6\u0000\u0000\u0be5\u0be6\u0003\u022c\u0116\u0000\u0be6\u0be8\u0001"+
"\u0000\u0000\u0000\u0be7\u0bdf\u0001\u0000\u0000\u0000\u0be7\u0be2\u0001"+
"\u0000\u0000\u0000\u0be8\u01f3\u0001\u0000\u0000\u0000\u0be9\u0bea\u0005"+
"\u012a\u0000\u0000\u0bea\u0beb\u0005\u00b6\u0000\u0000\u0beb\u0bec\u0003"+
"\u022c\u0116\u0000\u0bec\u01f5\u0001\u0000\u0000\u0000\u0bed\u0c04\u0005"+
"\u000b\u0000\u0000\u0bee\u0c04\u0005\u00fe\u0000\u0000\u0bef\u0c04\u0005"+
"\u0101\u0000\u0000\u0bf0\u0bf4\u0003\u0200\u0100\u0000\u0bf1\u0bf4\u0003"+
"\u0202\u0101\u0000\u0bf2\u0bf4\u0005\u00a2\u0000\u0000\u0bf3\u0bf0\u0001"+
"\u0000\u0000\u0000\u0bf3\u0bf1\u0001\u0000\u0000\u0000\u0bf3\u0bf2\u0001"+
"\u0000\u0000\u0000\u0bf4\u0bf6\u0001\u0000\u0000\u0000\u0bf5\u0bf7\u0005"+
"\u009a\u0000\u0000\u0bf6\u0bf5\u0001\u0000\u0000\u0000\u0bf6\u0bf7\u0001"+
"\u0000\u0000\u0000\u0bf7\u0c04\u0001\u0000\u0000\u0000\u0bf8\u0bfa\u0005"+
"\u0110\u0000\u0000\u0bf9\u0bfb\u0005\u009a\u0000\u0000\u0bfa\u0bf9\u0001"+
"\u0000\u0000\u0000\u0bfa\u0bfb\u0001\u0000\u0000\u0000\u0bfb\u0bff\u0001"+
"\u0000\u0000\u0000\u0bfc\u0bfd\u0005\u0106\u0000\u0000\u0bfd\u0bff\u0003"+
"\u0204\u0102\u0000\u0bfe\u0bf8\u0001\u0000\u0000\u0000\u0bfe\u0bfc\u0001"+
"\u0000\u0000\u0000\u0bff\u0c01\u0001\u0000\u0000\u0000\u0c00\u0c02\u0003"+
"\u0206\u0103\u0000\u0c01\u0c00\u0001\u0000\u0000\u0000\u0c01\u0c02\u0001"+
"\u0000\u0000\u0000\u0c02\u0c04\u0001\u0000\u0000\u0000\u0c03\u0bed\u0001"+
"\u0000\u0000\u0000\u0c03\u0bee\u0001\u0000\u0000\u0000\u0c03\u0bef\u0001"+
"\u0000\u0000\u0000\u0c03\u0bf3\u0001\u0000\u0000\u0000\u0c03\u0bfe\u0001"+
"\u0000\u0000\u0000\u0c04\u0c05\u0001\u0000\u0000\u0000\u0c05\u0c06\u0005"+
"\u00b6\u0000\u0000\u0c06\u0c07\u0003\u022a\u0115\u0000\u0c07\u01f7\u0001"+
"\u0000\u0000\u0000\u0c08\u0c09\u0005\u0013\u0000\u0000\u0c09\u0c20\u0007"+
"8\u0000\u0000\u0c0a\u0c0b\u0005\u001a\u0000\u0000\u0c0b\u0c20\u00077\u0000"+
"\u0000\u0c0c\u0c16\u0005\u000f\u0000\u0000\u0c0d\u0c0f\u00052\u0000\u0000"+
"\u0c0e\u0c0d\u0001\u0000\u0000\u0000\u0c0e\u0c0f\u0001\u0000\u0000\u0000"+
"\u0c0f\u0c10\u0001\u0000\u0000\u0000\u0c10\u0c16\u0005>\u0000\u0000\u0c11"+
"\u0c16\u0005\u00c9\u0000\u0000\u0c12\u0c16\u0005\u00e6\u0000\u0000\u0c13"+
"\u0c16\u0005\u00f3\u0000\u0000\u0c14\u0c16\u0005\u011e\u0000\u0000\u0c15"+
"\u0c0c\u0001\u0000\u0000\u0000\u0c15\u0c0e\u0001\u0000\u0000\u0000\u0c15"+
"\u0c11\u0001\u0000\u0000\u0000\u0c15\u0c12\u0001\u0000\u0000\u0000\u0c15"+
"\u0c13\u0001\u0000\u0000\u0000\u0c15\u0c14\u0001\u0000\u0000\u0000\u0c16"+
"\u0c17\u0001\u0000\u0000\u0000\u0c17\u0c20\u0005\u009a\u0000\u0000\u0c18"+
"\u0c20\u0003\u01fa\u00fd\u0000\u0c19\u0c1a\u0005\u00d8\u0000\u0000\u0c1a"+
"\u0c20\u00079\u0000\u0000\u0c1b\u0c1d\u0005~\u0000\u0000\u0c1c\u0c1e\u0003"+
"\u0206\u0103\u0000\u0c1d\u0c1c\u0001\u0000\u0000\u0000\u0c1d\u0c1e\u0001"+
"\u0000\u0000\u0000\u0c1e\u0c20\u0001\u0000\u0000\u0000\u0c1f\u0c08\u0001"+
"\u0000\u0000\u0000\u0c1f\u0c0a\u0001\u0000\u0000\u0000\u0c1f\u0c15\u0001"+
"\u0000\u0000\u0000\u0c1f\u0c18\u0001\u0000\u0000\u0000\u0c1f\u0c19\u0001"+
"\u0000\u0000\u0000\u0c1f\u0c1b\u0001\u0000\u0000\u0000\u0c20\u0c21\u0001"+
"\u0000\u0000\u0000\u0c21\u0c22\u0005\u00b6\u0000\u0000\u0c22\u0c23\u0005"+
"B\u0000\u0000\u0c23\u01f9\u0001\u0000\u0000\u0000\u0c24\u0c39\u0005c\u0000"+
"\u0000\u0c25\u0c26\u0003\u01fc\u00fe\u0000\u0c26\u0c27\u0005\u00cc\u0000"+
"\u0000\u0c27\u0c3a\u0001\u0000\u0000\u0000\u0c28\u0c2a\u0005!\u0000\u0000"+
"\u0c29\u0c28\u0001\u0000\u0000\u0000\u0c29\u0c2a\u0001\u0000\u0000\u0000"+
"\u0c2a\u0c37\u0001\u0000\u0000\u0000\u0c2b\u0c2c\u0003\u01fe\u00ff\u0000"+
"\u0c2c\u0c2d\u0003\u020a\u0105\u0000\u0c2d\u0c38\u0001\u0000\u0000\u0000"+
"\u0c2e\u0c30\u0005\u011e\u0000\u0000\u0c2f\u0c31\u0005E\u0000\u0000\u0c30"+
"\u0c2f\u0001\u0000\u0000\u0000\u0c30\u0c31\u0001\u0000\u0000\u0000\u0c31"+
"\u0c33\u0001\u0000\u0000\u0000\u0c32\u0c2e\u0001\u0000\u0000\u0000\u0c32"+
"\u0c33\u0001\u0000\u0000\u0000\u0c33\u0c34\u0001\u0000\u0000\u0000\u0c34"+
"\u0c35\u0003\u014a\u00a5\u0000\u0c35\u0c36\u0003\u0208\u0104\u0000\u0c36"+
"\u0c38\u0001\u0000\u0000\u0000\u0c37\u0c2b\u0001\u0000\u0000\u0000\u0c37"+
"\u0c32\u0001\u0000\u0000\u0000\u0c38\u0c3a\u0001\u0000\u0000\u0000\u0c39"+
"\u0c25\u0001\u0000\u0000\u0000\u0c39\u0c29\u0001\u0000\u0000\u0000\u0c3a"+
"\u01fb\u0001\u0000\u0000\u0000\u0c3b\u0c3c\u0007:\u0000\u0000\u0c3c\u01fd"+
"\u0001\u0000\u0000\u0000\u0c3d\u0c3e\u0007\u001f\u0000\u0000\u0c3e\u01ff"+
"\u0001\u0000\u0000\u0000\u0c3f\u0c40\u0007;\u0000\u0000\u0c40\u0201\u0001"+
"\u0000\u0000\u0000\u0c41\u0c42\u0007<\u0000\u0000\u0c42\u0203\u0001\u0000"+
"\u0000\u0000\u0c43\u0c44\u0007=\u0000\u0000\u0c44\u0205\u0001\u0000\u0000"+
"\u0000\u0c45\u0c48\u0005\u0098\u0000\u0000\u0c46\u0c49\u0005\u010a\u0000"+
"\u0000\u0c47\u0c49\u0003\u0186\u00c3\u0000\u0c48\u0c46\u0001\u0000\u0000"+
"\u0000\u0c48\u0c47\u0001\u0000\u0000\u0000\u0c49\u0c4a\u0001\u0000\u0000"+
"\u0000\u0c4a\u0c4b\u0005\u00ea\u0000\u0000\u0c4b\u0207\u0001\u0000\u0000"+
"\u0000\u0c4c\u0c4d\u0003\u020e\u0107\u0000\u0c4d\u0209\u0001\u0000\u0000"+
"\u0000\u0c4e\u0c4f\u0003\u020e\u0107\u0000\u0c4f\u020b\u0001\u0000\u0000"+
"\u0000\u0c50\u0c51\u0003\u020e\u0107\u0000\u0c51\u020d\u0001\u0000\u0000"+
"\u0000\u0c52\u0c57\u0003\u0210\u0108\u0000\u0c53\u0c54\u0005/\u0000\u0000"+
"\u0c54\u0c56\u0003\u0210\u0108\u0000\u0c55\u0c53\u0001\u0000\u0000\u0000"+
"\u0c56\u0c59\u0001\u0000\u0000\u0000\u0c57\u0c55\u0001\u0000\u0000\u0000"+
"\u0c57\u0c58\u0001\u0000\u0000\u0000\u0c58\u020f\u0001\u0000\u0000\u0000"+
"\u0c59\u0c57\u0001\u0000\u0000\u0000\u0c5a\u0c5c\u0003\u0282\u0141\u0000"+
"\u0c5b\u0c5d\u0003\u0212\u0109\u0000\u0c5c\u0c5b\u0001\u0000\u0000\u0000"+
"\u0c5c\u0c5d\u0001\u0000\u0000\u0000\u0c5d\u0c60\u0001\u0000\u0000\u0000"+
"\u0c5e\u0c60\u0003\u0212\u0109\u0000\u0c5f\u0c5a\u0001\u0000\u0000\u0000"+
"\u0c5f\u0c5e\u0001\u0000\u0000\u0000\u0c60\u0211\u0001\u0000\u0000\u0000"+
"\u0c61\u0c63\u0003\u0214\u010a\u0000\u0c62\u0c64\u0003\u0212\u0109\u0000"+
"\u0c63\u0c62\u0001\u0000\u0000\u0000\u0c63\u0c64\u0001\u0000\u0000\u0000"+
"\u0c64\u0213\u0001\u0000\u0000\u0000\u0c65\u0c67\u0005P\u0000\u0000\u0c66"+
"\u0c68\u0003\u0282\u0141\u0000\u0c67\u0c66\u0001\u0000\u0000\u0000\u0c67"+
"\u0c68\u0001\u0000\u0000\u0000\u0c68\u0c6d\u0001\u0000\u0000\u0000\u0c69"+
"\u0c6d\u0005\u00d1\u0000\u0000\u0c6a\u0c6d\u0005\u010a\u0000\u0000\u0c6b"+
"\u0c6d\u0003\u0284\u0142\u0000\u0c6c\u0c65\u0001\u0000\u0000\u0000\u0c6c"+
"\u0c69\u0001\u0000\u0000\u0000\u0c6c\u0c6a\u0001\u0000\u0000\u0000\u0c6c"+
"\u0c6b\u0001\u0000\u0000\u0000\u0c6d\u0215\u0001\u0000\u0000\u0000\u0c6e"+
"\u0c72\u0005\u0112\u0000\u0000\u0c6f\u0c70\u0007>\u0000\u0000\u0c70\u0c72"+
"\u0003\u021c\u010e\u0000\u0c71\u0c6e\u0001\u0000\u0000\u0000\u0c71\u0c6f"+
"\u0001\u0000\u0000\u0000\u0c72\u0c73\u0001\u0000\u0000\u0000\u0c73\u0c74"+
"\u0005\u00b6\u0000\u0000\u0c74\u0c75\u0003\u022c\u0116\u0000\u0c75\u0c79"+
"\u0003\u0220\u0110\u0000\u0c76\u0c77\u0005\u0098\u0000\u0000\u0c77\u0c78"+
"\u0005\u010a\u0000\u0000\u0c78\u0c7a\u0005\u00ea\u0000\u0000\u0c79\u0c76"+
"\u0001\u0000\u0000\u0000\u0c79\u0c7a\u0001\u0000\u0000\u0000\u0c7a\u0217"+
"\u0001\u0000\u0000\u0000\u0c7b\u0c7f\u0005F\u0000\u0000\u0c7c\u0c7d\u0005"+
"\u009d\u0000\u0000\u0c7d\u0c7f\u0003\u021c\u010e\u0000\u0c7e\u0c7b\u0001"+
"\u0000\u0000\u0000\u0c7e\u0c7c\u0001\u0000\u0000\u0000\u0c7f\u0c80\u0001"+
"\u0000\u0000\u0000\u0c80\u0c81\u0005\u00b6\u0000\u0000\u0c81\u0c82\u0003"+
"\u022c\u0116\u0000\u0c82\u0c83\u0003\u0220\u0110\u0000\u0c83\u0219\u0001"+
"\u0000\u0000\u0000\u0c84\u0c87\u0005\u010a\u0000\u0000\u0c85\u0c87\u0003"+
"\u021e\u010f\u0000\u0c86\u0c84\u0001\u0000\u0000\u0000\u0c86\u0c85\u0001"+
"\u0000\u0000\u0000\u0c87\u021b\u0001\u0000\u0000\u0000\u0c88\u0c8b\u0005"+
"\u0090\u0000\u0000\u0c89\u0c8c\u0005\u010a\u0000\u0000\u0c8a\u0c8c\u0003"+
"\u021e\u010f\u0000\u0c8b\u0c89\u0001\u0000\u0000\u0000\u0c8b\u0c8a\u0001"+
"\u0000\u0000\u0000\u0c8c\u0c8d\u0001\u0000\u0000\u0000\u0c8d\u0c8e\u0005"+
"\u00d4\u0000\u0000\u0c8e\u021d\u0001\u0000\u0000\u0000\u0c8f\u0c94\u0003"+
"\u0280\u0140\u0000\u0c90\u0c91\u0005/\u0000\u0000\u0c91\u0c93\u0003\u0280"+
"\u0140\u0000\u0c92\u0c90\u0001\u0000\u0000\u0000\u0c93\u0c96\u0001\u0000"+
"\u0000\u0000\u0c94\u0c92\u0001\u0000\u0000\u0000\u0c94\u0c95\u0001\u0000"+
"\u0000\u0000\u0c95\u021f\u0001\u0000\u0000\u0000\u0c96\u0c94\u0001\u0000"+
"\u0000\u0000\u0c97\u0c9a\u0003\u0222\u0111\u0000\u0c98\u0c9b\u0005\u010a"+
"\u0000\u0000\u0c99\u0c9b\u0003\u021e\u010f\u0000\u0c9a\u0c98\u0001\u0000"+
"\u0000\u0000\u0c9a\u0c99\u0001\u0000\u0000\u0000\u0c9b\u0cb9\u0001\u0000"+
"\u0000\u0000\u0c9c\u0c9d\u0005m\u0000\u0000\u0c9d\u0c9f\u0005\u0098\u0000"+
"\u0000\u0c9e\u0ca0\u0003\u0110\u0088\u0000\u0c9f\u0c9e\u0001\u0000\u0000"+
"\u0000\u0c9f\u0ca0\u0001\u0000\u0000\u0000\u0ca0\u0caa\u0001\u0000\u0000"+
"\u0000\u0ca1\u0ca2\u0005-\u0000\u0000\u0ca2\u0ca7\u0003\u0280\u0140\u0000"+
"\u0ca3\u0ca4\u0005\u001d\u0000\u0000\u0ca4\u0ca6\u0003\u0280\u0140\u0000"+
"\u0ca5\u0ca3\u0001\u0000\u0000\u0000\u0ca6\u0ca9\u0001\u0000\u0000\u0000"+
"\u0ca7\u0ca5\u0001\u0000\u0000\u0000\u0ca7\u0ca8\u0001\u0000\u0000\u0000"+
"\u0ca8\u0cab\u0001\u0000\u0000\u0000\u0ca9\u0ca7\u0001\u0000\u0000\u0000"+
"\u0caa\u0ca1\u0001\u0000\u0000\u0000\u0caa\u0cab\u0001\u0000\u0000\u0000"+
"\u0cab\u0cb6\u0001\u0000\u0000\u0000\u0cac\u0cad\u0005\u00ea\u0000\u0000"+
"\u0cad\u0cae\u0005\u0127\u0000\u0000\u0cae\u0cb7\u0003\u00acV\u0000\u0caf"+
"\u0cb0\u0005\u0127\u0000\u0000\u0cb0\u0cb3\u0003\u00acV\u0000\u0cb1\u0cb3"+
"\u0003\u027e\u013f\u0000\u0cb2\u0caf\u0001\u0000\u0000\u0000\u0cb2\u0cb1"+
"\u0001\u0000\u0000\u0000\u0cb3\u0cb4\u0001\u0000\u0000\u0000\u0cb4\u0cb5"+
"\u0005\u00ea\u0000\u0000\u0cb5\u0cb7\u0001\u0000\u0000\u0000\u0cb6\u0cac"+
"\u0001\u0000\u0000\u0000\u0cb6\u0cb2\u0001\u0000\u0000\u0000\u0cb7\u0cb9"+
"\u0001\u0000\u0000\u0000\u0cb8\u0c97\u0001\u0000\u0000\u0000\u0cb8\u0c9c"+
"\u0001\u0000\u0000\u0000\u0cb8\u0cb9\u0001\u0000\u0000\u0000\u0cb9\u0221"+
"\u0001\u0000\u0000\u0000\u0cba\u0cbe\u0003\u0224\u0112\u0000\u0cbb\u0cbe"+
"\u0003\u0228\u0114\u0000\u0cbc\u0cbe\u0003\u0226\u0113\u0000\u0cbd\u0cba"+
"\u0001\u0000\u0000\u0000\u0cbd\u0cbb\u0001\u0000\u0000\u0000\u0cbd\u0cbc"+
"\u0001\u0000\u0000\u0000\u0cbe\u0223\u0001\u0000\u0000\u0000\u0cbf\u0cc0"+
"\u0007?\u0000\u0000\u0cc0\u0225\u0001\u0000\u0000\u0000\u0cc1\u0cc2\u0007"+
"@\u0000\u0000\u0cc2\u0227\u0001\u0000\u0000\u0000\u0cc3\u0cc4\u0007A\u0000"+
"\u0000\u0cc4\u0229\u0001\u0000\u0000\u0000\u0cc5\u0cc6\u0007/\u0000\u0000"+
"\u0cc6\u0ccd\u0005>\u0000\u0000\u0cc7\u0cca\u0007&\u0000\u0000\u0cc8\u0ccb"+
"\u0005\u010a\u0000\u0000\u0cc9\u0ccb\u0003\u026c\u0136\u0000\u0cca\u0cc8"+
"\u0001\u0000\u0000\u0000\u0cca\u0cc9\u0001\u0000\u0000\u0000\u0ccb\u0ccd"+
"\u0001\u0000\u0000\u0000\u0ccc\u0cc5\u0001\u0000\u0000\u0000\u0ccc\u0cc7"+
"\u0001\u0000\u0000\u0000\u0ccd\u022b\u0001\u0000\u0000\u0000\u0cce\u0ccf"+
"\u0007/\u0000\u0000\u0ccf\u0cd6\u0005u\u0000\u0000\u0cd0\u0cd3\u00071"+
"\u0000\u0000\u0cd1\u0cd4\u0005\u010a\u0000\u0000\u0cd2\u0cd4\u0003\u026c"+
"\u0136\u0000\u0cd3\u0cd1\u0001\u0000\u0000\u0000\u0cd3\u0cd2\u0001\u0000"+
"\u0000\u0000\u0cd4\u0cd6\u0001\u0000\u0000\u0000\u0cd5\u0cce\u0001\u0000"+
"\u0000\u0000\u0cd5\u0cd0\u0001\u0000\u0000\u0000\u0cd6\u022d\u0001\u0000"+
"\u0000\u0000\u0cd7\u0cd8\u00052\u0000\u0000\u0cd8\u0cd9\u0005>\u0000\u0000"+
"\u0cd9\u0cdd\u0003\u026e\u0137\u0000\u0cda\u0cdb\u0005}\u0000\u0000\u0cdb"+
"\u0cdc\u0005\u00b0\u0000\u0000\u0cdc\u0cde\u0005f\u0000\u0000\u0cdd\u0cda"+
"\u0001\u0000\u0000\u0000\u0cdd\u0cde\u0001\u0000\u0000\u0000\u0cde\u0ce0"+
"\u0001\u0000\u0000\u0000\u0cdf\u0ce1\u0003\u0130\u0098\u0000\u0ce0\u0cdf"+
"\u0001\u0000\u0000\u0000\u0ce0\u0ce1\u0001\u0000\u0000\u0000\u0ce1\u0ce3"+
"\u0001\u0000\u0000\u0000\u0ce2\u0ce4\u0003\u024a\u0125\u0000\u0ce3\u0ce2"+
"\u0001\u0000\u0000\u0000\u0ce3\u0ce4\u0001\u0000\u0000\u0000\u0ce4\u022f"+
"\u0001\u0000\u0000\u0000\u0ce5\u0ce6\u0005>\u0000\u0000\u0ce6\u0cea\u0003"+
"\u026e\u0137\u0000\u0ce7\u0ce8\u0005}\u0000\u0000\u0ce8\u0ce9\u0005\u00b0"+
"\u0000\u0000\u0ce9\u0ceb\u0005f\u0000\u0000\u0cea\u0ce7\u0001\u0000\u0000"+
"\u0000\u0cea\u0ceb\u0001\u0000\u0000\u0000\u0ceb\u0cf3\u0001\u0000\u0000"+
"\u0000\u0cec\u0cef\u0005\u010e\u0000\u0000\u0ced\u0cf0\u0003\u0232\u0119"+
"\u0000\u0cee\u0cf0\u0003\u0236\u011b\u0000\u0cef\u0ced\u0001\u0000\u0000"+
"\u0000\u0cef\u0cee\u0001\u0000\u0000\u0000\u0cf0\u0cf1\u0001\u0000\u0000"+
"\u0000\u0cf1\u0cef\u0001\u0000\u0000\u0000\u0cf1\u0cf2\u0001\u0000\u0000"+
"\u0000\u0cf2\u0cf4\u0001\u0000\u0000\u0000\u0cf3\u0cec\u0001\u0000\u0000"+
"\u0000\u0cf3\u0cf4\u0001\u0000\u0000\u0000\u0cf4\u0cf6\u0001\u0000\u0000"+
"\u0000\u0cf5\u0cf7\u0003\u0130\u0098\u0000\u0cf6\u0cf5\u0001\u0000\u0000"+
"\u0000\u0cf6\u0cf7\u0001\u0000\u0000\u0000\u0cf7\u0cf9\u0001\u0000\u0000"+
"\u0000\u0cf8\u0cfa\u0003\u024a\u0125\u0000\u0cf9\u0cf8\u0001\u0000\u0000"+
"\u0000\u0cf9\u0cfa\u0001\u0000\u0000\u0000\u0cfa\u0231\u0001\u0000\u0000"+
"\u0000\u0cfb\u0cfc\u0005\u0005\u0000\u0000\u0cfc\u0cfd\u0003\u0234\u011a"+
"\u0000\u0cfd\u0233\u0001\u0000\u0000\u0000\u0cfe\u0cff\u0007B\u0000\u0000"+
"\u0cff\u0235\u0001\u0000\u0000\u0000\u0d00\u0d01\u0005\u0005\u0000\u0000"+
"\u0d01\u0d02\u0003\u0238\u011c\u0000\u0d02\u0237\u0001\u0000\u0000\u0000"+
"\u0d03\u0d04\u0007C\u0000\u0000\u0d04\u0239\u0001\u0000\u0000\u0000\u0d05"+
"\u0d07\u00052\u0000\u0000\u0d06\u0d05\u0001\u0000\u0000\u0000\u0d06\u0d07"+
"\u0001\u0000\u0000\u0000\u0d07\u0d08\u0001\u0000\u0000\u0000\u0d08\u0d09"+
"\u0005>\u0000\u0000\u0d09\u0d0c\u0003\u026e\u0137\u0000\u0d0a\u0d0b\u0005"+
"}\u0000\u0000\u0d0b\u0d0d\u0005f\u0000\u0000\u0d0c\u0d0a\u0001\u0000\u0000"+
"\u0000\u0d0c\u0d0d\u0001\u0000\u0000\u0000\u0d0d\u0d0f\u0001\u0000\u0000"+
"\u0000\u0d0e\u0d10\u0003\u023c\u011e\u0000\u0d0f\u0d0e\u0001\u0000\u0000"+
"\u0000\u0d0f\u0d10\u0001\u0000\u0000\u0000\u0d10\u0d13\u0001\u0000\u0000"+
"\u0000\u0d11\u0d12\u0007D\u0000\u0000\u0d12\u0d14\u0005=\u0000\u0000\u0d13"+
"\u0d11\u0001\u0000\u0000\u0000\u0d13\u0d14\u0001\u0000\u0000\u0000\u0d14"+
"\u0d16\u0001\u0000\u0000\u0000\u0d15\u0d17\u0003\u024a\u0125\u0000\u0d16"+
"\u0d15\u0001\u0000\u0000\u0000\u0d16\u0d17\u0001\u0000\u0000\u0000\u0d17"+
"\u023b\u0001\u0000\u0000\u0000\u0d18\u0d1c\u0005\u00e3\u0000\u0000\u0d19"+
"\u0d1a\u0005(\u0000\u0000\u0d1a\u0d1c\u0007E\u0000\u0000\u0d1b\u0d18\u0001"+
"\u0000\u0000\u0000\u0d1b\u0d19\u0001\u0000\u0000\u0000\u0d1c\u023d\u0001"+
"\u0000\u0000\u0000\u0d1d\u0d1e\u0005>\u0000\u0000\u0d1e\u0d21\u0003\u026e"+
"\u0137\u0000\u0d1f\u0d20\u0005}\u0000\u0000\u0d20\u0d22\u0005f\u0000\u0000"+
"\u0d21\u0d1f\u0001\u0000\u0000\u0000\u0d21\u0d22\u0001\u0000\u0000\u0000"+
"\u0d22\u0d34\u0001\u0000\u0000\u0000\u0d23\u0d27\u0005\u00f5\u0000\u0000"+
"\u0d24\u0d28\u0003\u0240\u0120\u0000\u0d25\u0d28\u0003\u0242\u0121\u0000"+
"\u0d26\u0d28\u0003\u0244\u0122\u0000\u0d27\u0d24\u0001\u0000\u0000\u0000"+
"\u0d27\u0d25\u0001\u0000\u0000\u0000\u0d27\u0d26\u0001\u0000\u0000\u0000"+
"\u0d28\u0d2a\u0001\u0000\u0000\u0000\u0d29\u0d23\u0001\u0000\u0000\u0000"+
"\u0d2a\u0d2b\u0001\u0000\u0000\u0000\u0d2b\u0d29\u0001\u0000\u0000\u0000"+
"\u0d2b\u0d2c\u0001\u0000\u0000\u0000\u0d2c\u0d35\u0001\u0000\u0000\u0000"+
"\u0d2d\u0d2e\u0005\u00dd\u0000\u0000\u0d2e\u0d2f\u0005\u00ba\u0000\u0000"+
"\u0d2f\u0d31\u0003\u0280\u0140\u0000\u0d30\u0d2d\u0001\u0000\u0000\u0000"+
"\u0d31\u0d32\u0001\u0000\u0000\u0000\u0d32\u0d30\u0001\u0000\u0000\u0000"+
"\u0d32\u0d33\u0001\u0000\u0000\u0000\u0d33\u0d35\u0001\u0000\u0000\u0000"+
"\u0d34\u0d29\u0001\u0000\u0000\u0000\u0d34\u0d30\u0001\u0000\u0000\u0000"+
"\u0d35\u0d37\u0001\u0000\u0000\u0000\u0d36\u0d38\u0003\u024a\u0125\u0000"+
"\u0d37\u0d36\u0001\u0000\u0000\u0000\u0d37\u0d38\u0001\u0000\u0000\u0000"+
"\u0d38\u023f\u0001\u0000\u0000\u0000\u0d39\u0d3a\u0005\u000b\u0000\u0000"+
"\u0d3a\u0d3b\u0005\u00d5\u0000\u0000\u0d3b\u0d3c\u0007F\u0000\u0000\u0d3c"+
"\u0241\u0001\u0000\u0000\u0000\u0d3d\u0d40\u0005\u010e\u0000\u0000\u0d3e"+
"\u0d41\u0003\u0232\u0119\u0000\u0d3f\u0d41\u0003\u0236\u011b\u0000\u0d40"+
"\u0d3e\u0001\u0000\u0000\u0000\u0d40\u0d3f\u0001\u0000\u0000\u0000\u0d41"+
"\u0d42\u0001\u0000\u0000\u0000\u0d42\u0d40\u0001\u0000\u0000\u0000\u0d42"+
"\u0d43\u0001\u0000\u0000\u0000\u0d43\u0243\u0001\u0000\u0000\u0000\u0d44"+
"\u0d45\u0005\u00ba\u0000\u0000\u0d45\u0d46\u0003\u0280\u0140\u0000\u0d46"+
"\u0d47\u0003\u00acV\u0000\u0d47\u0245\u0001\u0000\u0000\u0000\u0d48\u0d49"+
"\u0005\u00fe\u0000\u0000\u0d49\u0d4a\u0005>\u0000\u0000\u0d4a\u0d4c\u0003"+
"\u026e\u0137\u0000\u0d4b\u0d4d\u0003\u024a\u0125\u0000\u0d4c\u0d4b\u0001"+
"\u0000\u0000\u0000\u0d4c\u0d4d\u0001\u0000\u0000\u0000\u0d4d\u0247\u0001"+
"\u0000\u0000\u0000\u0d4e\u0d4f\u0005\u0101\u0000\u0000\u0d4f\u0d50\u0005"+
">\u0000\u0000\u0d50\u0d52\u0003\u026e\u0137\u0000\u0d51\u0d53\u0003\u024a"+
"\u0125\u0000\u0d52\u0d51\u0001\u0000\u0000\u0000\u0d52\u0d53\u0001\u0000"+
"\u0000\u0000\u0d53\u0249\u0001\u0000\u0000\u0000\u0d54\u0d59\u0005\u0125"+
"\u0000\u0000\u0d55\u0d57\u0005\u0005\u0000\u0000\u0d56\u0d58\u0003\u024c"+
"\u0126\u0000\u0d57\u0d56\u0001\u0000\u0000\u0000\u0d57\u0d58\u0001\u0000"+
"\u0000\u0000\u0d58\u0d5a\u0001\u0000\u0000\u0000\u0d59\u0d55\u0001\u0000"+
"\u0000\u0000\u0d59\u0d5a\u0001\u0000\u0000\u0000\u0d5a\u0d5d\u0001\u0000"+
"\u0000\u0000\u0d5b\u0d5d\u0005\u00b2\u0000\u0000\u0d5c\u0d54\u0001\u0000"+
"\u0000\u0000\u0d5c\u0d5b\u0001\u0000\u0000\u0000\u0d5d\u024b\u0001\u0000"+
"\u0000\u0000\u0d5e\u0d5f\u0007G\u0000\u0000\u0d5f\u024d\u0001\u0000\u0000"+
"\u0000\u0d60\u0d61\u0007/\u0000\u0000\u0d61\u0d63\u0005>\u0000\u0000\u0d62"+
"\u0d64\u0003\u0126\u0093\u0000\u0d63\u0d62\u0001\u0000\u0000\u0000\u0d63"+
"\u0d64\u0001\u0000\u0000\u0000\u0d64\u0d6d\u0001\u0000\u0000\u0000\u0d65"+
"\u0d67\u0007&\u0000\u0000\u0d66\u0d68\u0003\u026e\u0137\u0000\u0d67\u0d66"+
"\u0001\u0000\u0000\u0000\u0d67\u0d68\u0001\u0000\u0000\u0000\u0d68\u0d6a"+
"\u0001\u0000\u0000\u0000\u0d69\u0d6b\u0003\u0126\u0093\u0000\u0d6a\u0d69"+
"\u0001\u0000\u0000\u0000\u0d6a\u0d6b\u0001\u0000\u0000\u0000\u0d6b\u0d6d"+
"\u0001\u0000\u0000\u0000\u0d6c\u0d60\u0001\u0000\u0000\u0000\u0d6c\u0d65"+
"\u0001\u0000\u0000\u0000\u0d6d\u024f\u0001\u0000\u0000\u0000\u0d6e\u0d6f"+
"\u0003\u026e\u0137\u0000\u0d6f\u0251\u0001\u0000\u0000\u0000\u0d70\u0d71"+
"\u0003\u026e\u0137\u0000\u0d71\u0253\u0001\u0000\u0000\u0000\u0d72\u0d73"+
"\u0005\u000f\u0000\u0000\u0d73\u0d77\u0003\u0250\u0128\u0000\u0d74\u0d75"+
"\u0005}\u0000\u0000\u0d75\u0d76\u0005\u00b0\u0000\u0000\u0d76\u0d78\u0005"+
"f\u0000\u0000\u0d77\u0d74\u0001\u0000\u0000\u0000\u0d77\u0d78\u0001\u0000"+
"\u0000\u0000\u0d78\u0d79\u0001\u0000\u0000\u0000\u0d79\u0d7a\u0005m\u0000"+
"\u0000\u0d7a\u0d7b\u0005>\u0000\u0000\u0d7b\u0d86\u0003\u0252\u0129\u0000"+
"\u0d7c\u0d7d\u0005\u001b\u0000\u0000\u0d7d\u0d7e\u0003\u027a\u013d\u0000"+
"\u0d7e\u0d7f\u0005\u011e\u0000\u0000\u0d7f\u0d80\u0003\u0268\u0134\u0000"+
"\u0d80\u0d81\u0005\u00bd\u0000\u0000\u0d81\u0d84\u0003\u01b8\u00dc\u0000"+
"\u0d82\u0d83\u0005S\u0000\u0000\u0d83\u0d85\u0003\u027c\u013e\u0000\u0d84"+
"\u0d82\u0001\u0000\u0000\u0000\u0d84\u0d85\u0001\u0000\u0000\u0000\u0d85"+
"\u0d87\u0001\u0000\u0000\u0000\u0d86\u0d7c\u0001\u0000\u0000\u0000\u0d86"+
"\u0d87\u0001\u0000\u0000\u0000\u0d87\u0d8a\u0001\u0000\u0000\u0000\u0d88"+
"\u0d89\u0005\u00cd\u0000\u0000\u0d89\u0d8b\u0003\u027c\u013e\u0000\u0d8a"+
"\u0d88\u0001\u0000\u0000\u0000\u0d8a\u0d8b\u0001\u0000\u0000\u0000\u0d8b"+
"\u0255\u0001\u0000\u0000\u0000\u0d8c\u0d8d\u0005\u000f\u0000\u0000\u0d8d"+
"\u0d90\u0003\u0250\u0128\u0000\u0d8e\u0d8f\u0005}\u0000\u0000\u0d8f\u0d91"+
"\u0005f\u0000\u0000\u0d90\u0d8e\u0001\u0000\u0000\u0000\u0d90\u0d91\u0001"+
"\u0000\u0000\u0000\u0d91\u0d92\u0001\u0000\u0000\u0000\u0d92\u0d93\u0005"+
"m\u0000\u0000\u0d93\u0d94\u0005>\u0000\u0000\u0d94\u0257\u0001\u0000\u0000"+
"\u0000\u0d95\u0d96\u0005\u000f\u0000\u0000\u0d96\u0d99\u0003\u0250\u0128"+
"\u0000\u0d97\u0d98\u0005}\u0000\u0000\u0d98\u0d9a\u0005f\u0000\u0000\u0d99"+
"\u0d97\u0001\u0000\u0000\u0000\u0d99\u0d9a\u0001\u0000\u0000\u0000\u0d9a"+
"\u0d9b\u0001\u0000\u0000\u0000\u0d9b\u0d9c\u0005\u00f5\u0000\u0000\u0d9c"+
"\u0da2\u0005>\u0000\u0000\u0d9d\u0da3\u0003\u025a\u012d\u0000\u0d9e\u0da3"+
"\u0003\u025c\u012e\u0000\u0d9f\u0da3\u0003\u025e\u012f\u0000\u0da0\u0da3"+
"\u0003\u0260\u0130\u0000\u0da1\u0da3\u0003\u0262\u0131\u0000\u0da2\u0d9d"+
"\u0001\u0000\u0000\u0000\u0da2\u0d9e\u0001\u0000\u0000\u0000\u0da2\u0d9f"+
"\u0001\u0000\u0000\u0000\u0da2\u0da0\u0001\u0000\u0000\u0000\u0da2\u0da1"+
"\u0001\u0000\u0000\u0000\u0da3\u0da4\u0001\u0000\u0000\u0000\u0da4\u0da2"+
"\u0001\u0000\u0000\u0000\u0da4\u0da5\u0001\u0000\u0000\u0000\u0da5\u0259"+
"\u0001\u0000\u0000\u0000\u0da6\u0da7\u0005\u0105\u0000\u0000\u0da7\u0daa"+
"\u0003\u0252\u0129\u0000\u0da8\u0da9\u0005\u001b\u0000\u0000\u0da9\u0dab"+
"\u0003\u027a\u013d\u0000\u0daa\u0da8\u0001\u0000\u0000\u0000\u0daa\u0dab"+
"\u0001\u0000\u0000\u0000\u0dab\u025b\u0001\u0000\u0000\u0000\u0dac\u0dad"+
"\u0005\u011e\u0000\u0000\u0dad\u0dae\u0003\u0268\u0134\u0000\u0dae\u025d"+
"\u0001\u0000\u0000\u0000\u0daf\u0db0\u0005\u00bd\u0000\u0000\u0db0\u0db1"+
"\u0003\u01b8\u00dc\u0000\u0db1\u025f\u0001\u0000\u0000\u0000\u0db2\u0db3"+
"\u0005S\u0000\u0000\u0db3\u0db4\u0003\u027c\u013e\u0000\u0db4\u0261\u0001"+
"\u0000\u0000\u0000\u0db5\u0db6\u0005\u00cd\u0000\u0000\u0db6\u0db7\u0003"+
"\u027c\u013e\u0000\u0db7\u0263\u0001\u0000\u0000\u0000\u0db8\u0dba\u0007"+
"E\u0000\u0000\u0db9\u0dbb\u0003\u0250\u0128\u0000\u0dba\u0db9\u0001\u0000"+
"\u0000\u0000\u0dba\u0dbb\u0001\u0000\u0000\u0000\u0dbb\u0dbc\u0001\u0000"+
"\u0000\u0000\u0dbc\u0dbd\u0005m\u0000\u0000\u0dbd\u0dbf\u0007&\u0000\u0000"+
"\u0dbe\u0dc0\u0003\u0126\u0093\u0000\u0dbf\u0dbe\u0001\u0000\u0000\u0000"+
"\u0dbf\u0dc0\u0001\u0000\u0000\u0000\u0dc0\u0265\u0001\u0000\u0000\u0000"+
"\u0dc1\u0dc4\u0003\u0280\u0140\u0000\u0dc2\u0dc4\u0003\u0104\u0082\u0000"+
"\u0dc3\u0dc1\u0001\u0000\u0000\u0000\u0dc3\u0dc2\u0001\u0000\u0000\u0000"+
"\u0dc4\u0267\u0001\u0000\u0000\u0000\u0dc5\u0dc8\u0003\u0280\u0140\u0000"+
"\u0dc6\u0dc8\u0003\u0104\u0082\u0000\u0dc7\u0dc5\u0001\u0000\u0000\u0000"+
"\u0dc7\u0dc6\u0001\u0000\u0000\u0000\u0dc8\u0269\u0001\u0000\u0000\u0000"+
"\u0dc9\u0dce\u0003\u0268\u0134\u0000\u0dca\u0dcb\u0005/\u0000\u0000\u0dcb"+
"\u0dcd\u0003\u0268\u0134\u0000\u0dcc\u0dca\u0001\u0000\u0000\u0000\u0dcd"+
"\u0dd0\u0001\u0000\u0000\u0000\u0dce\u0dcc\u0001\u0000\u0000\u0000\u0dce"+
"\u0dcf\u0001\u0000\u0000\u0000\u0dcf\u026b\u0001\u0000\u0000\u0000\u0dd0"+
"\u0dce\u0001\u0000\u0000\u0000\u0dd1\u0dd6\u0003\u026e\u0137\u0000\u0dd2"+
"\u0dd3\u0005/\u0000\u0000\u0dd3\u0dd5\u0003\u026e\u0137\u0000\u0dd4\u0dd2"+
"\u0001\u0000\u0000\u0000\u0dd5\u0dd8\u0001\u0000\u0000\u0000\u0dd6\u0dd4"+
"\u0001\u0000\u0000\u0000\u0dd6\u0dd7\u0001\u0000\u0000\u0000\u0dd7\u026d"+
"\u0001\u0000\u0000\u0000\u0dd8\u0dd6\u0001\u0000\u0000\u0000\u0dd9\u0ddc"+
"\u0003\u0270\u0138\u0000\u0dda\u0ddc\u0003\u0104\u0082\u0000\u0ddb\u0dd9"+
"\u0001\u0000\u0000\u0000\u0ddb\u0dda\u0001\u0000\u0000\u0000\u0ddc\u026f"+
"\u0001\u0000\u0000\u0000\u0ddd\u0de2\u0003\u0280\u0140\u0000\u0dde\u0ddf"+
"\u0005P\u0000\u0000\u0ddf\u0de1\u0003\u0280\u0140\u0000\u0de0\u0dde\u0001"+
"\u0000\u0000\u0000\u0de1\u0de4\u0001\u0000\u0000\u0000\u0de2\u0de0\u0001"+
"\u0000\u0000\u0000\u0de2\u0de3\u0001\u0000\u0000\u0000\u0de3\u0271\u0001"+
"\u0000\u0000\u0000\u0de4\u0de2\u0001\u0000\u0000\u0000\u0de5\u0dee\u0005"+
"\u008f\u0000\u0000\u0de6\u0deb\u0003\u0276\u013b\u0000\u0de7\u0de8\u0005"+
"/\u0000\u0000\u0de8\u0dea\u0003\u0276\u013b\u0000\u0de9\u0de7\u0001\u0000"+
"\u0000\u0000\u0dea\u0ded\u0001\u0000\u0000\u0000\u0deb\u0de9\u0001\u0000"+
"\u0000\u0000\u0deb\u0dec\u0001\u0000\u0000\u0000\u0dec\u0def\u0001\u0000"+
"\u0000\u0000\u0ded\u0deb\u0001\u0000\u0000\u0000\u0dee\u0de6\u0001\u0000"+
"\u0000\u0000\u0dee\u0def\u0001\u0000\u0000\u0000\u0def\u0df0\u0001\u0000"+
"\u0000\u0000\u0df0\u0df1\u0005\u00d3\u0000\u0000\u0df1\u0273\u0001\u0000"+
"\u0000\u0000\u0df2\u0df5\u0003\u0276\u013b\u0000\u0df3\u0df4\u0005/\u0000"+
"\u0000\u0df4\u0df6\u0003\u0276\u013b\u0000\u0df5\u0df3\u0001\u0000\u0000"+
"\u0000\u0df6\u0df7\u0001\u0000\u0000\u0000\u0df7\u0df5\u0001\u0000\u0000"+
"\u0000\u0df7\u0df8\u0001\u0000\u0000\u0000\u0df8\u0275\u0001\u0000\u0000"+
"\u0000\u0df9\u0dfa\u0007H\u0000\u0000\u0dfa\u0277\u0001\u0000\u0000\u0000"+
"\u0dfb\u0dfe\u0003\u0276\u013b\u0000\u0dfc\u0dfe\u0003\u0104\u0082\u0000"+
"\u0dfd\u0dfb\u0001\u0000\u0000\u0000\u0dfd\u0dfc\u0001\u0000\u0000\u0000"+
"\u0dfe\u0279\u0001\u0000\u0000\u0000\u0dff\u0e02\u0003\u0276\u013b\u0000"+
"\u0e00\u0e02\u0003\u0104\u0082\u0000\u0e01\u0dff\u0001\u0000\u0000\u0000"+
"\u0e01\u0e00\u0001\u0000\u0000\u0000\u0e02\u027b\u0001\u0000\u0000\u0000"+
"\u0e03\u0e06\u0003\u027e\u013f\u0000\u0e04\u0e06\u0003\u0104\u0082\u0000"+
"\u0e05\u0e03\u0001\u0000\u0000\u0000\u0e05\u0e04\u0001\u0000\u0000\u0000"+
"\u0e06\u027d\u0001\u0000\u0000\u0000\u0e07\u0e15\u0005\u0090\u0000\u0000"+
"\u0e08\u0e09\u0003\u0102\u0081\u0000\u0e09\u0e0a\u0005-\u0000\u0000\u0e0a"+
"\u0e12\u0003\u00acV\u0000\u0e0b\u0e0c\u0005/\u0000\u0000\u0e0c\u0e0d\u0003"+
"\u0102\u0081\u0000\u0e0d\u0e0e\u0005-\u0000\u0000\u0e0e\u0e0f\u0003\u00ac"+
"V\u0000\u0e0f\u0e11\u0001\u0000\u0000\u0000\u0e10\u0e0b\u0001\u0000\u0000"+
"\u0000\u0e11\u0e14\u0001\u0000\u0000\u0000\u0e12\u0e10\u0001\u0000\u0000"+
"\u0000\u0e12\u0e13\u0001\u0000\u0000\u0000\u0e13\u0e16\u0001\u0000\u0000"+
"\u0000\u0e14\u0e12\u0001\u0000\u0000\u0000\u0e15\u0e08\u0001\u0000\u0000"+
"\u0000\u0e15\u0e16\u0001\u0000\u0000\u0000\u0e16\u0e17\u0001\u0000\u0000"+
"\u0000\u0e17\u0e18\u0005\u00d4\u0000\u0000\u0e18\u027f\u0001\u0000\u0000"+
"\u0000\u0e19\u0e1c\u0003\u0282\u0141\u0000\u0e1a\u0e1c\u0003\u0284\u0142"+
"\u0000\u0e1b\u0e19\u0001\u0000\u0000\u0000\u0e1b\u0e1a\u0001\u0000\u0000"+
"\u0000\u0e1c\u0281\u0001\u0000\u0000\u0000\u0e1d\u0e1e\u0005\n\u0000\u0000"+
"\u0e1e\u0283\u0001\u0000\u0000\u0000\u0e1f\u0e29\u0003\u0288\u0144\u0000"+
"\u0e20\u0e29\u0005\u00b0\u0000\u0000\u0e21\u0e29\u0005\u00b3\u0000\u0000"+
"\u0e22\u0e29\u0005\u0116\u0000\u0000\u0e23\u0e29\u0005\u00af\u0000\u0000"+
"\u0e24\u0e29\u0005\u00a5\u0000\u0000\u0e25\u0e29\u0005\u00a6\u0000\u0000"+
"\u0e26\u0e29\u0005\u00a7\u0000\u0000\u0e27\u0e29\u0005\u00a8\u0000\u0000"+
"\u0e28\u0e1f\u0001\u0000\u0000\u0000\u0e28\u0e20\u0001\u0000\u0000\u0000"+
"\u0e28\u0e21\u0001\u0000\u0000\u0000\u0e28\u0e22\u0001\u0000\u0000\u0000"+
"\u0e28\u0e23\u0001\u0000\u0000\u0000\u0e28\u0e24\u0001\u0000\u0000\u0000"+
"\u0e28\u0e25\u0001\u0000\u0000\u0000\u0e28\u0e26\u0001\u0000\u0000\u0000"+
"\u0e28\u0e27\u0001\u0000\u0000\u0000\u0e29\u0285\u0001\u0000\u0000\u0000"+
"\u0e2a\u0e2d\u0003\u0282\u0141\u0000\u0e2b\u0e2d\u0003\u0288\u0144\u0000"+
"\u0e2c\u0e2a\u0001\u0000\u0000\u0000\u0e2c\u0e2b\u0001\u0000\u0000\u0000"+
"\u0e2d\u0287\u0001\u0000\u0000\u0000\u0e2e\u0e2f\u0007I\u0000\u0000\u0e2f"+
"\u0289\u0001\u0000\u0000\u0000\u0e30\u0e31\u0005\u0000\u0000\u0001\u0e31"+
"\u028b\u0001\u0000\u0000\u0000\u01cf\u0291\u0295\u029b\u02a0\u02a5\u02ab"+
"\u02be\u02c2\u02cc\u02d4\u02d8\u02db\u02de\u02e3\u02e7\u02ed\u02f3\u0300"+
"\u030f\u031d\u0336\u033e\u0349\u034c\u0354\u0358\u035c\u0362\u0366\u036b"+
"\u036e\u0373\u0376\u0378\u0384\u0387\u0396\u039d\u03aa\u03b4\u03b7\u03ba"+
"\u03c3\u03c7\u03c9\u03cb\u03d5\u03db\u03e3\u03ee\u03f3\u03f7\u03fd\u0406"+
"\u0409\u040f\u0412\u0418\u041a\u042c\u042f\u0433\u0436\u043d\u0445\u044b"+
"\u044e\u0455\u045d\u0465\u0469\u046e\u0472\u047c\u0482\u0486\u0488\u048d"+
"\u0492\u0496\u0499\u049d\u04a1\u04a4\u04aa\u04ac\u04b8\u04bc\u04bf\u04c2"+
"\u04c6\u04cc\u04cf\u04d2\u04da\u04de\u04e2\u04e4\u04e9\u04ed\u04ef\u0505"+
"\u0508\u050d\u0510\u0513\u0516\u051a\u051d\u0521\u0524\u0529\u052d\u0532"+
"\u053c\u0540\u0543\u0549\u054e\u0553\u0559\u055e\u0566\u056e\u0574\u057c"+
"\u0587\u058f\u0597\u05a2\u05aa\u05b2\u05b8\u05c2\u05c7\u05d0\u05d5\u05da"+
"\u05de\u05e3\u05e6\u05e9\u05f2\u05fa\u0602\u0608\u060e\u0619\u061d\u0620"+
"\u062d\u0647\u0652\u0658\u065c\u066a\u066e\u0678\u0683\u0688\u068d\u0691"+
"\u0696\u0699\u069f\u06a7\u06ad\u06af\u06b7\u06bc\u06d6\u06df\u06e6\u06e9"+
"\u06ec\u0700\u0703\u070f\u071a\u071e\u0720\u0728\u072c\u072e\u0738\u073d"+
"\u0747\u074a\u0757\u075c\u0763\u0766\u0774\u077e\u0786\u078b\u0790\u079b"+
"\u07a9\u07b0\u07cb\u07d2\u07d4\u07d9\u07dd\u07e0\u07ef\u07f4\u07fd\u0807"+
"\u081a\u081e\u0821\u0826\u0835\u0838\u083b\u083e\u0841\u0844\u084e\u0857"+
"\u085a\u0862\u0865\u0868\u086c\u0872\u0877\u087d\u0880\u0884\u0888\u0890"+
"\u0894\u0897\u089b\u089e\u08a1\u08a4\u08a8\u08ab\u08ae\u08b7\u08b9\u08c0"+
"\u08ce\u08d2\u08d4\u08d7\u08db\u08e5\u08ee\u08f5\u08fa\u08ff\u0903\u090a"+
"\u0912\u091a\u0924\u092a\u0943\u0946\u094b\u0950\u0955\u0958\u095d\u0962"+
"\u096a\u0974\u097c\u0987\u098d\u0993\u0998\u099d\u09a4\u09af\u09b7\u09bd"+
"\u09c3\u09cc\u09d6\u09df\u09e5\u09e9\u09f2\u09f6\u09fe\u0a01\u0a0a\u0a16"+
"\u0a27\u0a2a\u0a2e\u0a39\u0a44\u0a4a\u0a50\u0a56\u0a5c\u0a61\u0a64\u0a73"+
"\u0a7c\u0a80\u0a86\u0a8c\u0a9e\u0aa6\u0aa9\u0aad\u0ab7\u0abb\u0ac0\u0ac5"+
"\u0ac8\u0acd\u0ad0\u0ad7\u0adb\u0ae8\u0af0\u0af9\u0afe\u0b01\u0b06\u0b0b"+
"\u0b0e\u0b12\u0b15\u0b1b\u0b1e\u0b22\u0b26\u0b29\u0b2d\u0b3f\u0b43\u0b49"+
"\u0b52\u0b57\u0b5a\u0b69\u0b70\u0b74\u0b7a\u0b80\u0b86\u0b8b\u0b90\u0b99"+
"\u0ba1\u0ba8\u0baa\u0bb8\u0bbc\u0bc4\u0bc8\u0bda\u0be7\u0bf3\u0bf6\u0bfa"+
"\u0bfe\u0c01\u0c03\u0c0e\u0c15\u0c1d\u0c1f\u0c29\u0c30\u0c32\u0c37\u0c39"+
"\u0c48\u0c57\u0c5c\u0c5f\u0c63\u0c67\u0c6c\u0c71\u0c79\u0c7e\u0c86\u0c8b"+
"\u0c94\u0c9a\u0c9f\u0ca7\u0caa\u0cb2\u0cb6\u0cb8\u0cbd\u0cca\u0ccc\u0cd3"+
"\u0cd5\u0cdd\u0ce0\u0ce3\u0cea\u0cef\u0cf1\u0cf3\u0cf6\u0cf9\u0d06\u0d0c"+
"\u0d0f\u0d13\u0d16\u0d1b\u0d21\u0d27\u0d2b\u0d32\u0d34\u0d37\u0d40\u0d42"+
"\u0d4c\u0d52\u0d57\u0d59\u0d5c\u0d63\u0d67\u0d6a\u0d6c\u0d77\u0d84\u0d86"+
"\u0d8a\u0d90\u0d99\u0da2\u0da4\u0daa\u0dba\u0dbf\u0dc3\u0dc7\u0dce\u0dd6"+
"\u0ddb\u0de2\u0deb\u0dee\u0df7\u0dfd\u0e01\u0e05\u0e12\u0e15\u0e1b\u0e28"+
"\u0e2c";
public static final String _serializedATN = Utils.join(
new String[] {
_serializedATNSegment0,
_serializedATNSegment1
},
""
);
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy