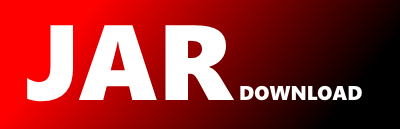
org.neo4j.packstream.struct.StructRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neo4j-bolt Show documentation
Show all versions of neo4j-bolt Show documentation
The core of Neo4j Bolt Protocol, this contains the state machine for Bolt sessions.
/*
* Copyright (c) "Neo4j"
* Neo4j Sweden AB [https://neo4j.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.packstream.struct;
import java.util.Map;
import java.util.Optional;
public interface StructRegistry {
/**
* Creates a new empty struct registry factory.
*
* @param a context object.
* @param a struct type.
* @return a registry factory.
*/
static Builder builder() {
return ImmutableStructRegistry.emptyBuilder();
}
/**
* Retrieves an empty struct registry which will reject any struct tags or payloads given to it.
*
* @param a context object.
* @param an arbitrary struct type.
* @return a struct registry.
*/
static StructRegistry empty() {
return EmptyStructRegistry.getInstance();
}
/**
* Returns a builder which mimics the configuration of this registry.
*
* @param a context type.
* @return a registry builder.
*/
Builder builderOf();
/**
* Retrieves the registered struct reader for a given header.
*
* @param header a struct header.
* @return a struct reader or, if none of the registered readers matches, an empty optional.
*/
Optional extends StructReader super CTX, ? extends S>> getReader(StructHeader header);
/**
* Retrieves the registered struct writer for a given payload object.
*
* @param payload a struct payload.
* @param a struct POJO type.
* @return a struct writer or, if none of the registered writers matches, an empty optional.
*/
Optional extends StructWriter super CTX, ? super O>> getWriter(O payload);
/**
* Provides a factory for arbitrary immutable registry instances.
*
* @param a context type.
* @param a struct base type.
*/
interface Builder {
/**
* Creates a new registry using a snapshot of the configuration present within this builder.
*
* @return a struct registry.
*/
StructRegistry build();
/**
* Registers a new reader with this builder using its self-identified tag.
*
* @param reader a reader.
* @return a reference to this builder.
*/
default Builder register(StructReader super CTX, ? extends S> reader) {
return this.register(reader.getTag(), reader);
}
/**
* Removes a previously registered reader.
* @param reader a reader.
* @return a reference to this builder.
*/
default Builder unregister(StructReader super CTX, ? extends S> reader) {
return this.unregisterReader(reader.getTag());
}
/**
* Removes a previously registered writer.
* @param writer a writer.
* @return a reference to this builder.
*/
default Builder unregister(StructWriter super CTX, ? extends S> writer) {
return this.unregisterWriter(writer.getType());
}
/**
* Registers a new reader for a given specific tag.
*
* @param tag a structure tag.
* @param reader a reader implementation.
* @return a reference to this builder.
*/
Builder register(short tag, StructReader super CTX, ? extends S> reader);
/**
* Removes a previously registered reader for a given specific tag.
*
* When no reader with the given tag has previously been registered, this method acts as a noop.
*
* @param tag a structure tag.
* @return a reference to this builder.
*/
StructRegistry.Builder unregisterReader(short tag);
/**
* Removes a previously registered writer for a given specific type.
*
* When no writer with the given tag has previously been registered, this method acts as a noop.
*
* @param type a specific value type.
* @param a value type.
* @return a reference to this builder.
*/
StructRegistry.Builder unregisterWriter(Class type);
/**
* Registers a set of readers.
*
* @param readers a reader implementation.
* @return a reference to this builder.
*/
default Builder registerReaders(Map> readers) {
readers.forEach(this::register);
return this;
}
/**
* Registers a new writer with this builder using its self-identified return type.
*
* @param writer a writer implementation.
* @param a value type.
* @return a reference to this builder.
*/
default Builder register(StructWriter super CTX, T> writer) {
return this.register(writer.getType(), writer);
}
/**
* Registers a writer for a given base type.
*
* @param type a specific value type.
* @param writer a writer implementation.
* @param a value type.
* @return a reference to this builder.
*/
Builder register(Class type, StructWriter super CTX, ? super T> writer);
/**
* Registers a set of writers.
*
* @param writers a map of writers.
* @return a reference to this builder.
*/
@SuppressWarnings({"unchecked", "rawtypes"})
default Builder registerWriters(Map, StructWriter super CTX, ? super S>> writers) {
writers.forEach((type, writer) -> this.register((Class) type, writer));
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy