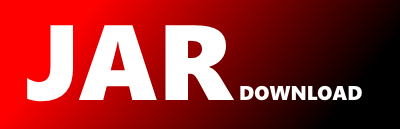
org.neo4j.cypher.internal.parser.CypherParser Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) "Neo4j"
* Neo4j Sweden AB [https://neo4j.com]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated from org/neo4j/cypher/internal/parser/CypherParser.g4 by ANTLR 4.13.1
package org.neo4j.cypher.internal.parser;
import java.util.List;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast", "CheckReturnValue"})
public class CypherParser extends Parser {
static {
RuntimeMetaData.checkVersion("4.13.1", RuntimeMetaData.VERSION);
}
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache = new PredictionContextCache();
public static final int SPACE = 1,
SINGLE_LINE_COMMENT = 2,
MULTI_LINE_COMMENT = 3,
DECIMAL_DOUBLE = 4,
UNSIGNED_DECIMAL_INTEGER = 5,
UNSIGNED_HEX_INTEGER = 6,
UNSIGNED_OCTAL_INTEGER = 7,
STRING_LITERAL1 = 8,
STRING_LITERAL2 = 9,
ESCAPED_SYMBOLIC_NAME = 10,
ACCESS = 11,
ACTIVE = 12,
ADMIN = 13,
ADMINISTRATOR = 14,
ALIAS = 15,
ALIASES = 16,
ALL_SHORTEST_PATHS = 17,
ALL = 18,
ALTER = 19,
AND = 20,
ANY = 21,
ARRAY = 22,
AS = 23,
ASC = 24,
ASSERT = 25,
ASSIGN = 26,
AT = 27,
BAR = 28,
BINDINGS = 29,
BOOLEAN = 30,
BOOSTED = 31,
BOTH = 32,
BREAK = 33,
BRIEF = 34,
BTREE = 35,
BUILT = 36,
BY = 37,
CALL = 38,
CASE = 39,
CHANGE = 40,
CIDR = 41,
COLLECT = 42,
COLON = 43,
COLONCOLON = 44,
COMMA = 45,
COMMAND = 46,
COMMANDS = 47,
COMMIT = 48,
COMPOSITE = 49,
CONCURRENT = 50,
CONSTRAINT = 51,
CONSTRAINTS = 52,
CONTAINS = 53,
COPY = 54,
CONTINUE = 55,
COUNT = 56,
CREATE = 57,
CSV = 58,
CURRENT = 59,
DATA = 60,
DATABASE = 61,
DATABASES = 62,
DATE = 63,
DATETIME = 64,
DBMS = 65,
DEALLOCATE = 66,
DEFAULT = 67,
DEFINED = 68,
DELETE = 69,
DENY = 70,
DESC = 71,
DESTROY = 72,
DETACH = 73,
DIFFERENT = 74,
DOLLAR = 75,
DISTINCT = 76,
DIVIDE = 77,
DOT = 78,
DOTDOT = 79,
DOUBLEBAR = 80,
DRIVER = 81,
DROP = 82,
DRYRUN = 83,
DUMP = 84,
DURATION = 85,
EACH = 86,
EDGE = 87,
ENABLE = 88,
ELEMENT = 89,
ELEMENTS = 90,
ELSE = 91,
ENCRYPTED = 92,
END = 93,
ENDS = 94,
EQ = 95,
EXECUTABLE = 96,
EXECUTE = 97,
EXIST = 98,
EXISTENCE = 99,
EXISTS = 100,
ERROR = 101,
FAIL = 102,
FALSE = 103,
FIELDTERMINATOR = 104,
FINISH = 105,
FLOAT = 106,
FOR = 107,
FOREACH = 108,
FROM = 109,
FULLTEXT = 110,
FUNCTIONS = 111,
GE = 112,
GRANT = 113,
GRAPH = 114,
GRAPHS = 115,
GROUP = 116,
GT = 117,
HEADERS = 118,
HOME = 119,
IF = 120,
IMPERSONATE = 121,
IMMUTABLE = 122,
IN = 123,
INDEX = 124,
INDEXES = 125,
INFINITY = 126,
INSERT = 127,
INT = 128,
INTEGER = 129,
IS = 130,
JOIN = 131,
KEY = 132,
LABEL = 133,
LABELS = 134,
AMPERSAND = 135,
EXCLAMATION_MARK = 136,
LBRACKET = 137,
LCURLY = 138,
LE = 139,
LEADING = 140,
LIMITROWS = 141,
LIST = 142,
LOAD = 143,
LOCAL = 144,
LOOKUP = 145,
LPAREN = 146,
LT = 147,
MANAGEMENT = 148,
MAP = 149,
MATCH = 150,
MERGE = 151,
MINUS = 152,
PERCENT = 153,
INVALID_NEQ = 154,
NEQ = 155,
NAME = 156,
NAMES = 157,
NAN = 158,
NFC = 159,
NFD = 160,
NFKC = 161,
NFKD = 162,
NEW = 163,
NODE = 164,
NODETACH = 165,
NODES = 166,
NONE = 167,
NORMALIZE = 168,
NORMALIZED = 169,
NOT = 170,
NOTHING = 171,
NOWAIT = 172,
NULL = 173,
OF = 174,
ON = 175,
ONLY = 176,
OPTIONAL = 177,
OPTIONS = 178,
OPTION = 179,
OR = 180,
ORDER = 181,
OUTPUT = 182,
PASSWORD = 183,
PASSWORDS = 184,
PATH = 185,
PERIODIC = 186,
PLAINTEXT = 187,
PLUS = 188,
PLUSEQUAL = 189,
POINT = 190,
POPULATED = 191,
POW = 192,
PRIMARY = 193,
PRIVILEGE = 194,
PRIVILEGES = 195,
PROCEDURE = 196,
PROCEDURES = 197,
PROPERTIES = 198,
PROPERTY = 199,
QUESTION = 200,
RANGE = 201,
RBRACKET = 202,
RCURLY = 203,
READ = 204,
REALLOCATE = 205,
REDUCE = 206,
RENAME = 207,
REGEQ = 208,
REL = 209,
RELATIONSHIP = 210,
RELATIONSHIPS = 211,
REMOVE = 212,
REPEATABLE = 213,
REPLACE = 214,
REPORT = 215,
REQUIRE = 216,
REQUIRED = 217,
RETURN = 218,
REVOKE = 219,
ROLE = 220,
ROLES = 221,
ROW = 222,
ROWS = 223,
RPAREN = 224,
SCAN = 225,
SECONDARY = 226,
SECONDS = 227,
SEEK = 228,
SEMICOLON = 229,
SERVER = 230,
SERVERS = 231,
SET = 232,
SETTING = 233,
SHORTEST_PATH = 234,
SHORTEST = 235,
SHOW = 236,
SIGNED = 237,
SINGLE = 238,
SKIPROWS = 239,
START = 240,
STARTS = 241,
STATUS = 242,
STOP = 243,
STRING = 244,
SUPPORTED = 245,
SUSPENDED = 246,
TARGET = 247,
TERMINATE = 248,
TEXT = 249,
THEN = 250,
TIME = 251,
TIMES = 252,
TIMESTAMP = 253,
TIMEZONE = 254,
TO = 255,
TOPOLOGY = 256,
TRAILING = 257,
TRANSACTION = 258,
TRANSACTIONS = 259,
TRAVERSE = 260,
TRIM = 261,
TRUE = 262,
TYPE = 263,
TYPED = 264,
TYPES = 265,
UNION = 266,
UNIQUE = 267,
UNIQUENESS = 268,
UNWIND = 269,
URL = 270,
USE = 271,
USER = 272,
USERS = 273,
USING = 274,
VALUE = 275,
VECTOR = 276,
VERBOSE = 277,
VERTEX = 278,
WAIT = 279,
WHEN = 280,
WHERE = 281,
WITH = 282,
WITHOUT = 283,
WRITE = 284,
XOR = 285,
YIELD = 286,
ZONED = 287,
IDENTIFIER = 288,
ARROW_LINE = 289,
ARROW_LEFT_HEAD = 290,
ARROW_RIGHT_HEAD = 291,
ErrorChar = 292;
public static final int RULE_statements = 0,
RULE_statement = 1,
RULE_periodicCommitQueryHintFailure = 2,
RULE_regularQuery = 3,
RULE_singleQuery = 4,
RULE_clause = 5,
RULE_useClause = 6,
RULE_graphReference = 7,
RULE_finishClause = 8,
RULE_returnClause = 9,
RULE_returnBody = 10,
RULE_returnItem = 11,
RULE_returnItems = 12,
RULE_orderItem = 13,
RULE_orderBy = 14,
RULE_skip = 15,
RULE_limit = 16,
RULE_whereClause = 17,
RULE_withClause = 18,
RULE_createClause = 19,
RULE_insertClause = 20,
RULE_setClause = 21,
RULE_setItem = 22,
RULE_removeClause = 23,
RULE_removeItem = 24,
RULE_deleteClause = 25,
RULE_matchClause = 26,
RULE_matchMode = 27,
RULE_hint = 28,
RULE_mergeClause = 29,
RULE_mergeAction = 30,
RULE_unwindClause = 31,
RULE_callClause = 32,
RULE_procedureResultItem = 33,
RULE_loadCSVClause = 34,
RULE_foreachClause = 35,
RULE_subqueryClause = 36,
RULE_subqueryInTransactionsParameters = 37,
RULE_subqueryInTransactionsBatchParameters = 38,
RULE_subqueryInTransactionsErrorParameters = 39,
RULE_subqueryInTransactionsReportParameters = 40,
RULE_patternList = 41,
RULE_insertPatternList = 42,
RULE_pattern = 43,
RULE_insertPattern = 44,
RULE_quantifier = 45,
RULE_anonymousPattern = 46,
RULE_shortestPathPattern = 47,
RULE_patternElement = 48,
RULE_selector = 49,
RULE_pathPatternNonEmpty = 50,
RULE_nodePattern = 51,
RULE_insertNodePattern = 52,
RULE_parenthesizedPath = 53,
RULE_nodeLabels = 54,
RULE_nodeLabelsIs = 55,
RULE_labelType = 56,
RULE_relType = 57,
RULE_labelOrRelType = 58,
RULE_properties = 59,
RULE_relationshipPattern = 60,
RULE_insertRelationshipPattern = 61,
RULE_leftArrow = 62,
RULE_arrowLine = 63,
RULE_rightArrow = 64,
RULE_pathLength = 65,
RULE_labelExpression = 66,
RULE_labelExpression4 = 67,
RULE_labelExpression4Is = 68,
RULE_labelExpression3 = 69,
RULE_labelExpression3Is = 70,
RULE_labelExpression2 = 71,
RULE_labelExpression2Is = 72,
RULE_labelExpression1 = 73,
RULE_labelExpression1Is = 74,
RULE_insertNodeLabelExpression = 75,
RULE_insertRelationshipLabelExpression = 76,
RULE_expression = 77,
RULE_expression11 = 78,
RULE_expression10 = 79,
RULE_expression9 = 80,
RULE_expression8 = 81,
RULE_expression7 = 82,
RULE_comparisonExpression6 = 83,
RULE_normalForm = 84,
RULE_expression6 = 85,
RULE_expression5 = 86,
RULE_expression4 = 87,
RULE_expression3 = 88,
RULE_expression2 = 89,
RULE_postFix = 90,
RULE_property = 91,
RULE_propertyExpression = 92,
RULE_expression1 = 93,
RULE_literal = 94,
RULE_caseExpression = 95,
RULE_caseAlternative = 96,
RULE_extendedCaseExpression = 97,
RULE_extendedCaseAlternative = 98,
RULE_extendedWhen = 99,
RULE_listComprehension = 100,
RULE_patternComprehension = 101,
RULE_reduceExpression = 102,
RULE_listItemsPredicate = 103,
RULE_normalizeFunction = 104,
RULE_trimFunction = 105,
RULE_patternExpression = 106,
RULE_shortestPathExpression = 107,
RULE_parenthesizedExpression = 108,
RULE_mapProjection = 109,
RULE_mapProjectionElement = 110,
RULE_countStar = 111,
RULE_existsExpression = 112,
RULE_countExpression = 113,
RULE_collectExpression = 114,
RULE_numberLiteral = 115,
RULE_signedIntegerLiteral = 116,
RULE_listLiteral = 117,
RULE_propertyKeyName = 118,
RULE_parameter = 119,
RULE_parameterName = 120,
RULE_functionInvocation = 121,
RULE_functionArgument = 122,
RULE_functionName = 123,
RULE_namespace = 124,
RULE_variable = 125,
RULE_nonEmptyNameList = 126,
RULE_command = 127,
RULE_createCommand = 128,
RULE_dropCommand = 129,
RULE_alterCommand = 130,
RULE_renameCommand = 131,
RULE_showCommand = 132,
RULE_showCommandYield = 133,
RULE_yieldItem = 134,
RULE_yieldSkip = 135,
RULE_yieldLimit = 136,
RULE_yieldClause = 137,
RULE_showBriefAndYield = 138,
RULE_showIndexCommand = 139,
RULE_showIndexesAllowBrief = 140,
RULE_showIndexesNoBrief = 141,
RULE_showConstraintCommand = 142,
RULE_constraintAllowYieldType = 143,
RULE_constraintExistType = 144,
RULE_constraintBriefAndYieldType = 145,
RULE_showConstraintsAllowBriefAndYield = 146,
RULE_showConstraintsAllowBrief = 147,
RULE_showConstraintsAllowYield = 148,
RULE_showProcedures = 149,
RULE_showFunctions = 150,
RULE_executableBy = 151,
RULE_showFunctionsType = 152,
RULE_showTransactions = 153,
RULE_terminateCommand = 154,
RULE_terminateTransactions = 155,
RULE_showSettings = 156,
RULE_namesAndClauses = 157,
RULE_composableCommandClauses = 158,
RULE_composableShowCommandClauses = 159,
RULE_stringsOrExpression = 160,
RULE_type = 161,
RULE_typePart = 162,
RULE_typeName = 163,
RULE_typeNullability = 164,
RULE_typeListSuffix = 165,
RULE_commandNodePattern = 166,
RULE_commandRelPattern = 167,
RULE_createConstraint = 168,
RULE_constraintType = 169,
RULE_dropConstraint = 170,
RULE_createIndex = 171,
RULE_oldCreateIndex = 172,
RULE_createIndex_ = 173,
RULE_createFulltextIndex = 174,
RULE_fulltextNodePattern = 175,
RULE_fulltextRelPattern = 176,
RULE_createLookupIndex = 177,
RULE_lookupIndexNodePattern = 178,
RULE_lookupIndexRelPattern = 179,
RULE_dropIndex = 180,
RULE_propertyList = 181,
RULE_enableServerCommand = 182,
RULE_alterServer = 183,
RULE_renameServer = 184,
RULE_dropServer = 185,
RULE_showServers = 186,
RULE_allocationCommand = 187,
RULE_deallocateDatabaseFromServers = 188,
RULE_reallocateDatabases = 189,
RULE_createRole = 190,
RULE_dropRole = 191,
RULE_renameRole = 192,
RULE_showRoles = 193,
RULE_roleToken = 194,
RULE_createUser = 195,
RULE_dropUser = 196,
RULE_renameUser = 197,
RULE_alterCurrentUser = 198,
RULE_alterUser = 199,
RULE_password = 200,
RULE_passwordExpression = 201,
RULE_passwordChangeRequired = 202,
RULE_userStatus = 203,
RULE_homeDatabase = 204,
RULE_showUsers = 205,
RULE_showCurrentUser = 206,
RULE_showPrivileges = 207,
RULE_showSupportedPrivileges = 208,
RULE_showRolePrivileges = 209,
RULE_showUserPrivileges = 210,
RULE_privilegeAsCommand = 211,
RULE_privilegeToken = 212,
RULE_grantCommand = 213,
RULE_grantRole = 214,
RULE_denyCommand = 215,
RULE_revokeCommand = 216,
RULE_revokeRole = 217,
RULE_privilege = 218,
RULE_allPrivilege = 219,
RULE_allPrivilegeType = 220,
RULE_allPrivilegeTarget = 221,
RULE_createPrivilege = 222,
RULE_createPrivilegeForDatabase = 223,
RULE_createNodePrivilegeToken = 224,
RULE_createRelPrivilegeToken = 225,
RULE_createPropertyPrivilegeToken = 226,
RULE_actionForDBMS = 227,
RULE_dropPrivilege = 228,
RULE_loadPrivilege = 229,
RULE_showPrivilege = 230,
RULE_setPrivilege = 231,
RULE_passwordToken = 232,
RULE_removePrivilege = 233,
RULE_writePrivilege = 234,
RULE_databasePrivilege = 235,
RULE_dbmsPrivilege = 236,
RULE_dbmsPrivilegeExecute = 237,
RULE_adminToken = 238,
RULE_procedureToken = 239,
RULE_indexToken = 240,
RULE_constraintToken = 241,
RULE_transactionToken = 242,
RULE_userQualifier = 243,
RULE_executeFunctionQualifier = 244,
RULE_executeProcedureQualifier = 245,
RULE_settingQualifier = 246,
RULE_globs = 247,
RULE_qualifiedGraphPrivilegesWithProperty = 248,
RULE_qualifiedGraphPrivileges = 249,
RULE_labelsResource = 250,
RULE_propertiesResource = 251,
RULE_nonEmptyStringList = 252,
RULE_graphQualifier = 253,
RULE_graphQualifierToken = 254,
RULE_relToken = 255,
RULE_elementToken = 256,
RULE_nodeToken = 257,
RULE_createCompositeDatabase = 258,
RULE_createDatabase = 259,
RULE_primaryTopology = 260,
RULE_secondaryTopology = 261,
RULE_dropDatabase = 262,
RULE_alterDatabase = 263,
RULE_alterDatabaseAccess = 264,
RULE_alterDatabaseTopology = 265,
RULE_alterDatabaseOption = 266,
RULE_startDatabase = 267,
RULE_stopDatabase = 268,
RULE_waitClause = 269,
RULE_showDatabase = 270,
RULE_databaseScope = 271,
RULE_graphScope = 272,
RULE_commandOptions = 273,
RULE_commandNameExpression = 274,
RULE_symbolicNameOrStringParameter = 275,
RULE_createAlias = 276,
RULE_dropAlias = 277,
RULE_alterAlias = 278,
RULE_alterAliasTarget = 279,
RULE_alterAliasUser = 280,
RULE_alterAliasPassword = 281,
RULE_alterAliasDriver = 282,
RULE_alterAliasProperties = 283,
RULE_showAliases = 284,
RULE_symbolicAliasNameList = 285,
RULE_symbolicAliasNameOrParameter = 286,
RULE_symbolicAliasName = 287,
RULE_symbolicNameOrStringParameterList = 288,
RULE_glob = 289,
RULE_globRecursive = 290,
RULE_globPart = 291,
RULE_stringList = 292,
RULE_stringLiteral = 293,
RULE_stringOrParameter = 294,
RULE_mapOrParameter = 295,
RULE_map = 296,
RULE_symbolicNameString = 297,
RULE_escapedSymbolicNameString = 298,
RULE_unescapedSymbolicNameString = 299,
RULE_symbolicLabelNameString = 300,
RULE_unescapedLabelSymbolicNameString = 301,
RULE_endOfFile = 302;
private static String[] makeRuleNames() {
return new String[] {
"statements",
"statement",
"periodicCommitQueryHintFailure",
"regularQuery",
"singleQuery",
"clause",
"useClause",
"graphReference",
"finishClause",
"returnClause",
"returnBody",
"returnItem",
"returnItems",
"orderItem",
"orderBy",
"skip",
"limit",
"whereClause",
"withClause",
"createClause",
"insertClause",
"setClause",
"setItem",
"removeClause",
"removeItem",
"deleteClause",
"matchClause",
"matchMode",
"hint",
"mergeClause",
"mergeAction",
"unwindClause",
"callClause",
"procedureResultItem",
"loadCSVClause",
"foreachClause",
"subqueryClause",
"subqueryInTransactionsParameters",
"subqueryInTransactionsBatchParameters",
"subqueryInTransactionsErrorParameters",
"subqueryInTransactionsReportParameters",
"patternList",
"insertPatternList",
"pattern",
"insertPattern",
"quantifier",
"anonymousPattern",
"shortestPathPattern",
"patternElement",
"selector",
"pathPatternNonEmpty",
"nodePattern",
"insertNodePattern",
"parenthesizedPath",
"nodeLabels",
"nodeLabelsIs",
"labelType",
"relType",
"labelOrRelType",
"properties",
"relationshipPattern",
"insertRelationshipPattern",
"leftArrow",
"arrowLine",
"rightArrow",
"pathLength",
"labelExpression",
"labelExpression4",
"labelExpression4Is",
"labelExpression3",
"labelExpression3Is",
"labelExpression2",
"labelExpression2Is",
"labelExpression1",
"labelExpression1Is",
"insertNodeLabelExpression",
"insertRelationshipLabelExpression",
"expression",
"expression11",
"expression10",
"expression9",
"expression8",
"expression7",
"comparisonExpression6",
"normalForm",
"expression6",
"expression5",
"expression4",
"expression3",
"expression2",
"postFix",
"property",
"propertyExpression",
"expression1",
"literal",
"caseExpression",
"caseAlternative",
"extendedCaseExpression",
"extendedCaseAlternative",
"extendedWhen",
"listComprehension",
"patternComprehension",
"reduceExpression",
"listItemsPredicate",
"normalizeFunction",
"trimFunction",
"patternExpression",
"shortestPathExpression",
"parenthesizedExpression",
"mapProjection",
"mapProjectionElement",
"countStar",
"existsExpression",
"countExpression",
"collectExpression",
"numberLiteral",
"signedIntegerLiteral",
"listLiteral",
"propertyKeyName",
"parameter",
"parameterName",
"functionInvocation",
"functionArgument",
"functionName",
"namespace",
"variable",
"nonEmptyNameList",
"command",
"createCommand",
"dropCommand",
"alterCommand",
"renameCommand",
"showCommand",
"showCommandYield",
"yieldItem",
"yieldSkip",
"yieldLimit",
"yieldClause",
"showBriefAndYield",
"showIndexCommand",
"showIndexesAllowBrief",
"showIndexesNoBrief",
"showConstraintCommand",
"constraintAllowYieldType",
"constraintExistType",
"constraintBriefAndYieldType",
"showConstraintsAllowBriefAndYield",
"showConstraintsAllowBrief",
"showConstraintsAllowYield",
"showProcedures",
"showFunctions",
"executableBy",
"showFunctionsType",
"showTransactions",
"terminateCommand",
"terminateTransactions",
"showSettings",
"namesAndClauses",
"composableCommandClauses",
"composableShowCommandClauses",
"stringsOrExpression",
"type",
"typePart",
"typeName",
"typeNullability",
"typeListSuffix",
"commandNodePattern",
"commandRelPattern",
"createConstraint",
"constraintType",
"dropConstraint",
"createIndex",
"oldCreateIndex",
"createIndex_",
"createFulltextIndex",
"fulltextNodePattern",
"fulltextRelPattern",
"createLookupIndex",
"lookupIndexNodePattern",
"lookupIndexRelPattern",
"dropIndex",
"propertyList",
"enableServerCommand",
"alterServer",
"renameServer",
"dropServer",
"showServers",
"allocationCommand",
"deallocateDatabaseFromServers",
"reallocateDatabases",
"createRole",
"dropRole",
"renameRole",
"showRoles",
"roleToken",
"createUser",
"dropUser",
"renameUser",
"alterCurrentUser",
"alterUser",
"password",
"passwordExpression",
"passwordChangeRequired",
"userStatus",
"homeDatabase",
"showUsers",
"showCurrentUser",
"showPrivileges",
"showSupportedPrivileges",
"showRolePrivileges",
"showUserPrivileges",
"privilegeAsCommand",
"privilegeToken",
"grantCommand",
"grantRole",
"denyCommand",
"revokeCommand",
"revokeRole",
"privilege",
"allPrivilege",
"allPrivilegeType",
"allPrivilegeTarget",
"createPrivilege",
"createPrivilegeForDatabase",
"createNodePrivilegeToken",
"createRelPrivilegeToken",
"createPropertyPrivilegeToken",
"actionForDBMS",
"dropPrivilege",
"loadPrivilege",
"showPrivilege",
"setPrivilege",
"passwordToken",
"removePrivilege",
"writePrivilege",
"databasePrivilege",
"dbmsPrivilege",
"dbmsPrivilegeExecute",
"adminToken",
"procedureToken",
"indexToken",
"constraintToken",
"transactionToken",
"userQualifier",
"executeFunctionQualifier",
"executeProcedureQualifier",
"settingQualifier",
"globs",
"qualifiedGraphPrivilegesWithProperty",
"qualifiedGraphPrivileges",
"labelsResource",
"propertiesResource",
"nonEmptyStringList",
"graphQualifier",
"graphQualifierToken",
"relToken",
"elementToken",
"nodeToken",
"createCompositeDatabase",
"createDatabase",
"primaryTopology",
"secondaryTopology",
"dropDatabase",
"alterDatabase",
"alterDatabaseAccess",
"alterDatabaseTopology",
"alterDatabaseOption",
"startDatabase",
"stopDatabase",
"waitClause",
"showDatabase",
"databaseScope",
"graphScope",
"commandOptions",
"commandNameExpression",
"symbolicNameOrStringParameter",
"createAlias",
"dropAlias",
"alterAlias",
"alterAliasTarget",
"alterAliasUser",
"alterAliasPassword",
"alterAliasDriver",
"alterAliasProperties",
"showAliases",
"symbolicAliasNameList",
"symbolicAliasNameOrParameter",
"symbolicAliasName",
"symbolicNameOrStringParameterList",
"glob",
"globRecursive",
"globPart",
"stringList",
"stringLiteral",
"stringOrParameter",
"mapOrParameter",
"map",
"symbolicNameString",
"escapedSymbolicNameString",
"unescapedSymbolicNameString",
"symbolicLabelNameString",
"unescapedLabelSymbolicNameString",
"endOfFile"
};
}
public static final String[] ruleNames = makeRuleNames();
private static String[] makeLiteralNames() {
return new String[] {
null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, "'|'", null, null, null, null, null, null, null,
null, null, null, null, null, null, null, "':'", "'::'", "','", null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, "'$'", null, "'/'", "'.'", "'..'", "'||'", null, null, null, null, null, null, null,
null, null, null, null, null, null, null, "'='", null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, "'>='", null, null, null, null, "'>'", null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null, "'&'", "'!'", "'['", "'{'", "'<='",
null, null, null, null, null, null, "'('", "'<'", null, null, null, null, "'-'", "'%'", "'!='", "'<>'",
null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null, null, null, "'+'", "'+='", null,
null, "'^'", null, null, null, null, null, null, null, "'?'", null, "']'", "'}'", null, null, null, null,
"'=~'", null, null, null, null, null, null, null, null, null, null, null, null, null, null, null, "')'",
null, null, null, null, "';'", null, null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, "'*'"
};
}
private static final String[] _LITERAL_NAMES = makeLiteralNames();
private static String[] makeSymbolicNames() {
return new String[] {
null,
"SPACE",
"SINGLE_LINE_COMMENT",
"MULTI_LINE_COMMENT",
"DECIMAL_DOUBLE",
"UNSIGNED_DECIMAL_INTEGER",
"UNSIGNED_HEX_INTEGER",
"UNSIGNED_OCTAL_INTEGER",
"STRING_LITERAL1",
"STRING_LITERAL2",
"ESCAPED_SYMBOLIC_NAME",
"ACCESS",
"ACTIVE",
"ADMIN",
"ADMINISTRATOR",
"ALIAS",
"ALIASES",
"ALL_SHORTEST_PATHS",
"ALL",
"ALTER",
"AND",
"ANY",
"ARRAY",
"AS",
"ASC",
"ASSERT",
"ASSIGN",
"AT",
"BAR",
"BINDINGS",
"BOOLEAN",
"BOOSTED",
"BOTH",
"BREAK",
"BRIEF",
"BTREE",
"BUILT",
"BY",
"CALL",
"CASE",
"CHANGE",
"CIDR",
"COLLECT",
"COLON",
"COLONCOLON",
"COMMA",
"COMMAND",
"COMMANDS",
"COMMIT",
"COMPOSITE",
"CONCURRENT",
"CONSTRAINT",
"CONSTRAINTS",
"CONTAINS",
"COPY",
"CONTINUE",
"COUNT",
"CREATE",
"CSV",
"CURRENT",
"DATA",
"DATABASE",
"DATABASES",
"DATE",
"DATETIME",
"DBMS",
"DEALLOCATE",
"DEFAULT",
"DEFINED",
"DELETE",
"DENY",
"DESC",
"DESTROY",
"DETACH",
"DIFFERENT",
"DOLLAR",
"DISTINCT",
"DIVIDE",
"DOT",
"DOTDOT",
"DOUBLEBAR",
"DRIVER",
"DROP",
"DRYRUN",
"DUMP",
"DURATION",
"EACH",
"EDGE",
"ENABLE",
"ELEMENT",
"ELEMENTS",
"ELSE",
"ENCRYPTED",
"END",
"ENDS",
"EQ",
"EXECUTABLE",
"EXECUTE",
"EXIST",
"EXISTENCE",
"EXISTS",
"ERROR",
"FAIL",
"FALSE",
"FIELDTERMINATOR",
"FINISH",
"FLOAT",
"FOR",
"FOREACH",
"FROM",
"FULLTEXT",
"FUNCTIONS",
"GE",
"GRANT",
"GRAPH",
"GRAPHS",
"GROUP",
"GT",
"HEADERS",
"HOME",
"IF",
"IMPERSONATE",
"IMMUTABLE",
"IN",
"INDEX",
"INDEXES",
"INFINITY",
"INSERT",
"INT",
"INTEGER",
"IS",
"JOIN",
"KEY",
"LABEL",
"LABELS",
"AMPERSAND",
"EXCLAMATION_MARK",
"LBRACKET",
"LCURLY",
"LE",
"LEADING",
"LIMITROWS",
"LIST",
"LOAD",
"LOCAL",
"LOOKUP",
"LPAREN",
"LT",
"MANAGEMENT",
"MAP",
"MATCH",
"MERGE",
"MINUS",
"PERCENT",
"INVALID_NEQ",
"NEQ",
"NAME",
"NAMES",
"NAN",
"NFC",
"NFD",
"NFKC",
"NFKD",
"NEW",
"NODE",
"NODETACH",
"NODES",
"NONE",
"NORMALIZE",
"NORMALIZED",
"NOT",
"NOTHING",
"NOWAIT",
"NULL",
"OF",
"ON",
"ONLY",
"OPTIONAL",
"OPTIONS",
"OPTION",
"OR",
"ORDER",
"OUTPUT",
"PASSWORD",
"PASSWORDS",
"PATH",
"PERIODIC",
"PLAINTEXT",
"PLUS",
"PLUSEQUAL",
"POINT",
"POPULATED",
"POW",
"PRIMARY",
"PRIVILEGE",
"PRIVILEGES",
"PROCEDURE",
"PROCEDURES",
"PROPERTIES",
"PROPERTY",
"QUESTION",
"RANGE",
"RBRACKET",
"RCURLY",
"READ",
"REALLOCATE",
"REDUCE",
"RENAME",
"REGEQ",
"REL",
"RELATIONSHIP",
"RELATIONSHIPS",
"REMOVE",
"REPEATABLE",
"REPLACE",
"REPORT",
"REQUIRE",
"REQUIRED",
"RETURN",
"REVOKE",
"ROLE",
"ROLES",
"ROW",
"ROWS",
"RPAREN",
"SCAN",
"SECONDARY",
"SECONDS",
"SEEK",
"SEMICOLON",
"SERVER",
"SERVERS",
"SET",
"SETTING",
"SHORTEST_PATH",
"SHORTEST",
"SHOW",
"SIGNED",
"SINGLE",
"SKIPROWS",
"START",
"STARTS",
"STATUS",
"STOP",
"STRING",
"SUPPORTED",
"SUSPENDED",
"TARGET",
"TERMINATE",
"TEXT",
"THEN",
"TIME",
"TIMES",
"TIMESTAMP",
"TIMEZONE",
"TO",
"TOPOLOGY",
"TRAILING",
"TRANSACTION",
"TRANSACTIONS",
"TRAVERSE",
"TRIM",
"TRUE",
"TYPE",
"TYPED",
"TYPES",
"UNION",
"UNIQUE",
"UNIQUENESS",
"UNWIND",
"URL",
"USE",
"USER",
"USERS",
"USING",
"VALUE",
"VECTOR",
"VERBOSE",
"VERTEX",
"WAIT",
"WHEN",
"WHERE",
"WITH",
"WITHOUT",
"WRITE",
"XOR",
"YIELD",
"ZONED",
"IDENTIFIER",
"ARROW_LINE",
"ARROW_LEFT_HEAD",
"ARROW_RIGHT_HEAD",
"ErrorChar"
};
}
private static final String[] _SYMBOLIC_NAMES = makeSymbolicNames();
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() {
return "CypherParser.g4";
}
@Override
public String[] getRuleNames() {
return ruleNames;
}
@Override
public String getSerializedATN() {
return _serializedATN;
}
@Override
public ATN getATN() {
return _ATN;
}
public CypherParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this, _ATN, _decisionToDFA, _sharedContextCache);
}
@SuppressWarnings("CheckReturnValue")
public static class StatementsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List statement() {
return getRuleContexts(StatementContext.class);
}
public StatementContext statement(int i) {
return getRuleContext(StatementContext.class, i);
}
public TerminalNode EOF() {
return getToken(CypherParser.EOF, 0);
}
public List SEMICOLON() {
return getTokens(CypherParser.SEMICOLON);
}
public TerminalNode SEMICOLON(int i) {
return getToken(CypherParser.SEMICOLON, i);
}
public StatementsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_statements;
}
}
public final StatementsContext statements() throws RecognitionException {
StatementsContext _localctx = new StatementsContext(_ctx, getState());
enterRule(_localctx, 0, RULE_statements);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(606);
statement();
setState(611);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 0, _ctx);
while (_alt != 2 && _alt != org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER) {
if (_alt == 1) {
{
{
setState(607);
match(SEMICOLON);
setState(608);
statement();
}
}
}
setState(613);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 0, _ctx);
}
setState(615);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == SEMICOLON) {
{
setState(614);
match(SEMICOLON);
}
}
setState(617);
match(EOF);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StatementContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public CommandContext command() {
return getRuleContext(CommandContext.class, 0);
}
public RegularQueryContext regularQuery() {
return getRuleContext(RegularQueryContext.class, 0);
}
public PeriodicCommitQueryHintFailureContext periodicCommitQueryHintFailure() {
return getRuleContext(PeriodicCommitQueryHintFailureContext.class, 0);
}
public StatementContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_statement;
}
}
public final StatementContext statement() throws RecognitionException {
StatementContext _localctx = new StatementContext(_ctx, getState());
enterRule(_localctx, 2, RULE_statement);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(620);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == USING) {
{
setState(619);
periodicCommitQueryHintFailure();
}
}
setState(624);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 3, _ctx)) {
case 1:
{
setState(622);
command();
}
break;
case 2:
{
setState(623);
regularQuery();
}
break;
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PeriodicCommitQueryHintFailureContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USING() {
return getToken(CypherParser.USING, 0);
}
public TerminalNode PERIODIC() {
return getToken(CypherParser.PERIODIC, 0);
}
public TerminalNode COMMIT() {
return getToken(CypherParser.COMMIT, 0);
}
public TerminalNode UNSIGNED_DECIMAL_INTEGER() {
return getToken(CypherParser.UNSIGNED_DECIMAL_INTEGER, 0);
}
public PeriodicCommitQueryHintFailureContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_periodicCommitQueryHintFailure;
}
}
public final PeriodicCommitQueryHintFailureContext periodicCommitQueryHintFailure() throws RecognitionException {
PeriodicCommitQueryHintFailureContext _localctx = new PeriodicCommitQueryHintFailureContext(_ctx, getState());
enterRule(_localctx, 4, RULE_periodicCommitQueryHintFailure);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(626);
match(USING);
setState(627);
match(PERIODIC);
setState(628);
match(COMMIT);
setState(630);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == UNSIGNED_DECIMAL_INTEGER) {
{
setState(629);
match(UNSIGNED_DECIMAL_INTEGER);
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RegularQueryContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List singleQuery() {
return getRuleContexts(SingleQueryContext.class);
}
public SingleQueryContext singleQuery(int i) {
return getRuleContext(SingleQueryContext.class, i);
}
public List UNION() {
return getTokens(CypherParser.UNION);
}
public TerminalNode UNION(int i) {
return getToken(CypherParser.UNION, i);
}
public List ALL() {
return getTokens(CypherParser.ALL);
}
public TerminalNode ALL(int i) {
return getToken(CypherParser.ALL, i);
}
public List DISTINCT() {
return getTokens(CypherParser.DISTINCT);
}
public TerminalNode DISTINCT(int i) {
return getToken(CypherParser.DISTINCT, i);
}
public RegularQueryContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_regularQuery;
}
}
public final RegularQueryContext regularQuery() throws RecognitionException {
RegularQueryContext _localctx = new RegularQueryContext(_ctx, getState());
enterRule(_localctx, 6, RULE_regularQuery);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(632);
singleQuery();
setState(640);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == UNION) {
{
{
setState(633);
match(UNION);
setState(635);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == ALL || _la == DISTINCT) {
{
setState(634);
_la = _input.LA(1);
if (!(_la == ALL || _la == DISTINCT)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(637);
singleQuery();
}
}
setState(642);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SingleQueryContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List clause() {
return getRuleContexts(ClauseContext.class);
}
public ClauseContext clause(int i) {
return getRuleContext(ClauseContext.class, i);
}
public SingleQueryContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_singleQuery;
}
}
public final SingleQueryContext singleQuery() throws RecognitionException {
SingleQueryContext _localctx = new SingleQueryContext(_ctx, getState());
enterRule(_localctx, 8, RULE_singleQuery);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(644);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(643);
clause();
}
}
setState(646);
_errHandler.sync(this);
_la = _input.LA(1);
} while (((((_la - 38)) & ~0x3f) == 0 && ((1L << (_la - 38)) & 36507746305L) != 0)
|| ((((_la - 105)) & ~0x3f) == 0 && ((1L << (_la - 105)) & 1153027332605214729L) != 0)
|| ((((_la - 177)) & ~0x3f) == 0 && ((1L << (_la - 177)) & 36031030401957889L) != 0)
|| ((((_la - 269)) & ~0x3f) == 0 && ((1L << (_la - 269)) & 8197L) != 0));
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public UseClauseContext useClause() {
return getRuleContext(UseClauseContext.class, 0);
}
public FinishClauseContext finishClause() {
return getRuleContext(FinishClauseContext.class, 0);
}
public ReturnClauseContext returnClause() {
return getRuleContext(ReturnClauseContext.class, 0);
}
public CreateClauseContext createClause() {
return getRuleContext(CreateClauseContext.class, 0);
}
public InsertClauseContext insertClause() {
return getRuleContext(InsertClauseContext.class, 0);
}
public DeleteClauseContext deleteClause() {
return getRuleContext(DeleteClauseContext.class, 0);
}
public SetClauseContext setClause() {
return getRuleContext(SetClauseContext.class, 0);
}
public RemoveClauseContext removeClause() {
return getRuleContext(RemoveClauseContext.class, 0);
}
public MatchClauseContext matchClause() {
return getRuleContext(MatchClauseContext.class, 0);
}
public MergeClauseContext mergeClause() {
return getRuleContext(MergeClauseContext.class, 0);
}
public WithClauseContext withClause() {
return getRuleContext(WithClauseContext.class, 0);
}
public UnwindClauseContext unwindClause() {
return getRuleContext(UnwindClauseContext.class, 0);
}
public CallClauseContext callClause() {
return getRuleContext(CallClauseContext.class, 0);
}
public SubqueryClauseContext subqueryClause() {
return getRuleContext(SubqueryClauseContext.class, 0);
}
public LoadCSVClauseContext loadCSVClause() {
return getRuleContext(LoadCSVClauseContext.class, 0);
}
public ForeachClauseContext foreachClause() {
return getRuleContext(ForeachClauseContext.class, 0);
}
public ClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_clause;
}
}
public final ClauseContext clause() throws RecognitionException {
ClauseContext _localctx = new ClauseContext(_ctx, getState());
enterRule(_localctx, 10, RULE_clause);
try {
setState(664);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 8, _ctx)) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(648);
useClause();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(649);
finishClause();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(650);
returnClause();
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(651);
createClause();
}
break;
case 5:
enterOuterAlt(_localctx, 5);
{
setState(652);
insertClause();
}
break;
case 6:
enterOuterAlt(_localctx, 6);
{
setState(653);
deleteClause();
}
break;
case 7:
enterOuterAlt(_localctx, 7);
{
setState(654);
setClause();
}
break;
case 8:
enterOuterAlt(_localctx, 8);
{
setState(655);
removeClause();
}
break;
case 9:
enterOuterAlt(_localctx, 9);
{
setState(656);
matchClause();
}
break;
case 10:
enterOuterAlt(_localctx, 10);
{
setState(657);
mergeClause();
}
break;
case 11:
enterOuterAlt(_localctx, 11);
{
setState(658);
withClause();
}
break;
case 12:
enterOuterAlt(_localctx, 12);
{
setState(659);
unwindClause();
}
break;
case 13:
enterOuterAlt(_localctx, 13);
{
setState(660);
callClause();
}
break;
case 14:
enterOuterAlt(_localctx, 14);
{
setState(661);
subqueryClause();
}
break;
case 15:
enterOuterAlt(_localctx, 15);
{
setState(662);
loadCSVClause();
}
break;
case 16:
enterOuterAlt(_localctx, 16);
{
setState(663);
foreachClause();
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UseClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USE() {
return getToken(CypherParser.USE, 0);
}
public GraphReferenceContext graphReference() {
return getRuleContext(GraphReferenceContext.class, 0);
}
public TerminalNode GRAPH() {
return getToken(CypherParser.GRAPH, 0);
}
public UseClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_useClause;
}
}
public final UseClauseContext useClause() throws RecognitionException {
UseClauseContext _localctx = new UseClauseContext(_ctx, getState());
enterRule(_localctx, 12, RULE_useClause);
try {
enterOuterAlt(_localctx, 1);
{
setState(666);
match(USE);
setState(668);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 9, _ctx)) {
case 1:
{
setState(667);
match(GRAPH);
}
break;
}
setState(670);
graphReference();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GraphReferenceContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public GraphReferenceContext graphReference() {
return getRuleContext(GraphReferenceContext.class, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public FunctionInvocationContext functionInvocation() {
return getRuleContext(FunctionInvocationContext.class, 0);
}
public SymbolicAliasNameContext symbolicAliasName() {
return getRuleContext(SymbolicAliasNameContext.class, 0);
}
public GraphReferenceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_graphReference;
}
}
public final GraphReferenceContext graphReference() throws RecognitionException {
GraphReferenceContext _localctx = new GraphReferenceContext(_ctx, getState());
enterRule(_localctx, 14, RULE_graphReference);
try {
setState(678);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 10, _ctx)) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(672);
match(LPAREN);
setState(673);
graphReference();
setState(674);
match(RPAREN);
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(676);
functionInvocation();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(677);
symbolicAliasName();
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FinishClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode FINISH() {
return getToken(CypherParser.FINISH, 0);
}
public FinishClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_finishClause;
}
}
public final FinishClauseContext finishClause() throws RecognitionException {
FinishClauseContext _localctx = new FinishClauseContext(_ctx, getState());
enterRule(_localctx, 16, RULE_finishClause);
try {
enterOuterAlt(_localctx, 1);
{
setState(680);
match(FINISH);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ReturnClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode RETURN() {
return getToken(CypherParser.RETURN, 0);
}
public ReturnBodyContext returnBody() {
return getRuleContext(ReturnBodyContext.class, 0);
}
public ReturnClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_returnClause;
}
}
public final ReturnClauseContext returnClause() throws RecognitionException {
ReturnClauseContext _localctx = new ReturnClauseContext(_ctx, getState());
enterRule(_localctx, 18, RULE_returnClause);
try {
enterOuterAlt(_localctx, 1);
{
setState(682);
match(RETURN);
setState(683);
returnBody();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ReturnBodyContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ReturnItemsContext returnItems() {
return getRuleContext(ReturnItemsContext.class, 0);
}
public TerminalNode DISTINCT() {
return getToken(CypherParser.DISTINCT, 0);
}
public OrderByContext orderBy() {
return getRuleContext(OrderByContext.class, 0);
}
public SkipContext skip() {
return getRuleContext(SkipContext.class, 0);
}
public LimitContext limit() {
return getRuleContext(LimitContext.class, 0);
}
public ReturnBodyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_returnBody;
}
}
public final ReturnBodyContext returnBody() throws RecognitionException {
ReturnBodyContext _localctx = new ReturnBodyContext(_ctx, getState());
enterRule(_localctx, 20, RULE_returnBody);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(686);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 11, _ctx)) {
case 1:
{
setState(685);
match(DISTINCT);
}
break;
}
setState(688);
returnItems();
setState(690);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == ORDER) {
{
setState(689);
orderBy();
}
}
setState(693);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == SKIPROWS) {
{
setState(692);
skip();
}
}
setState(696);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == LIMITROWS) {
{
setState(695);
limit();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ReturnItemContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public TerminalNode AS() {
return getToken(CypherParser.AS, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public ReturnItemContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_returnItem;
}
}
public final ReturnItemContext returnItem() throws RecognitionException {
ReturnItemContext _localctx = new ReturnItemContext(_ctx, getState());
enterRule(_localctx, 22, RULE_returnItem);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(698);
expression();
setState(701);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == AS) {
{
setState(699);
match(AS);
setState(700);
variable();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ReturnItemsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public List returnItem() {
return getRuleContexts(ReturnItemContext.class);
}
public ReturnItemContext returnItem(int i) {
return getRuleContext(ReturnItemContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public ReturnItemsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_returnItems;
}
}
public final ReturnItemsContext returnItems() throws RecognitionException {
ReturnItemsContext _localctx = new ReturnItemsContext(_ctx, getState());
enterRule(_localctx, 24, RULE_returnItems);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(705);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(703);
match(TIMES);
}
break;
case DECIMAL_DOUBLE:
case UNSIGNED_DECIMAL_INTEGER:
case UNSIGNED_HEX_INTEGER:
case UNSIGNED_OCTAL_INTEGER:
case STRING_LITERAL1:
case STRING_LITERAL2:
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LBRACKET:
case LCURLY:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case LPAREN:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case MINUS:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case PLUS:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
{
setState(704);
returnItem();
}
break;
default:
throw new NoViableAltException(this);
}
setState(711);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(707);
match(COMMA);
setState(708);
returnItem();
}
}
setState(713);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class OrderItemContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public TerminalNode ASC() {
return getToken(CypherParser.ASC, 0);
}
public TerminalNode DESC() {
return getToken(CypherParser.DESC, 0);
}
public OrderItemContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_orderItem;
}
}
public final OrderItemContext orderItem() throws RecognitionException {
OrderItemContext _localctx = new OrderItemContext(_ctx, getState());
enterRule(_localctx, 26, RULE_orderItem);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(714);
expression();
setState(716);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == ASC || _la == DESC) {
{
setState(715);
_la = _input.LA(1);
if (!(_la == ASC || _la == DESC)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class OrderByContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ORDER() {
return getToken(CypherParser.ORDER, 0);
}
public TerminalNode BY() {
return getToken(CypherParser.BY, 0);
}
public List orderItem() {
return getRuleContexts(OrderItemContext.class);
}
public OrderItemContext orderItem(int i) {
return getRuleContext(OrderItemContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public OrderByContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_orderBy;
}
}
public final OrderByContext orderBy() throws RecognitionException {
OrderByContext _localctx = new OrderByContext(_ctx, getState());
enterRule(_localctx, 28, RULE_orderBy);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(718);
match(ORDER);
setState(719);
match(BY);
setState(720);
orderItem();
setState(725);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(721);
match(COMMA);
setState(722);
orderItem();
}
}
setState(727);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SkipContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SKIPROWS() {
return getToken(CypherParser.SKIPROWS, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public SkipContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_skip;
}
}
public final SkipContext skip() throws RecognitionException {
SkipContext _localctx = new SkipContext(_ctx, getState());
enterRule(_localctx, 30, RULE_skip);
try {
enterOuterAlt(_localctx, 1);
{
setState(728);
match(SKIPROWS);
setState(729);
expression();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LimitContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LIMITROWS() {
return getToken(CypherParser.LIMITROWS, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public LimitContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_limit;
}
}
public final LimitContext limit() throws RecognitionException {
LimitContext _localctx = new LimitContext(_ctx, getState());
enterRule(_localctx, 32, RULE_limit);
try {
enterOuterAlt(_localctx, 1);
{
setState(731);
match(LIMITROWS);
setState(732);
expression();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class WhereClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode WHERE() {
return getToken(CypherParser.WHERE, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public WhereClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_whereClause;
}
}
public final WhereClauseContext whereClause() throws RecognitionException {
WhereClauseContext _localctx = new WhereClauseContext(_ctx, getState());
enterRule(_localctx, 34, RULE_whereClause);
try {
enterOuterAlt(_localctx, 1);
{
setState(734);
match(WHERE);
setState(735);
expression();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class WithClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode WITH() {
return getToken(CypherParser.WITH, 0);
}
public ReturnBodyContext returnBody() {
return getRuleContext(ReturnBodyContext.class, 0);
}
public WhereClauseContext whereClause() {
return getRuleContext(WhereClauseContext.class, 0);
}
public WithClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_withClause;
}
}
public final WithClauseContext withClause() throws RecognitionException {
WithClauseContext _localctx = new WithClauseContext(_ctx, getState());
enterRule(_localctx, 36, RULE_withClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(737);
match(WITH);
setState(738);
returnBody();
setState(740);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE) {
{
setState(739);
whereClause();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CREATE() {
return getToken(CypherParser.CREATE, 0);
}
public PatternListContext patternList() {
return getRuleContext(PatternListContext.class, 0);
}
public CreateClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createClause;
}
}
public final CreateClauseContext createClause() throws RecognitionException {
CreateClauseContext _localctx = new CreateClauseContext(_ctx, getState());
enterRule(_localctx, 38, RULE_createClause);
try {
enterOuterAlt(_localctx, 1);
{
setState(742);
match(CREATE);
setState(743);
patternList();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InsertClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode INSERT() {
return getToken(CypherParser.INSERT, 0);
}
public InsertPatternListContext insertPatternList() {
return getRuleContext(InsertPatternListContext.class, 0);
}
public InsertClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_insertClause;
}
}
public final InsertClauseContext insertClause() throws RecognitionException {
InsertClauseContext _localctx = new InsertClauseContext(_ctx, getState());
enterRule(_localctx, 40, RULE_insertClause);
try {
enterOuterAlt(_localctx, 1);
{
setState(745);
match(INSERT);
setState(746);
insertPatternList();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SetClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SET() {
return getToken(CypherParser.SET, 0);
}
public List setItem() {
return getRuleContexts(SetItemContext.class);
}
public SetItemContext setItem(int i) {
return getRuleContext(SetItemContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public SetClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_setClause;
}
}
public final SetClauseContext setClause() throws RecognitionException {
SetClauseContext _localctx = new SetClauseContext(_ctx, getState());
enterRule(_localctx, 42, RULE_setClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(748);
match(SET);
setState(749);
setItem();
setState(754);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(750);
match(COMMA);
setState(751);
setItem();
}
}
setState(756);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SetItemContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SetItemContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_setItem;
}
public SetItemContext() {}
public void copyFrom(SetItemContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class SetPropContext extends SetItemContext {
public PropertyExpressionContext propertyExpression() {
return getRuleContext(PropertyExpressionContext.class, 0);
}
public TerminalNode EQ() {
return getToken(CypherParser.EQ, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public SetPropContext(SetItemContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class AddPropContext extends SetItemContext {
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public TerminalNode PLUSEQUAL() {
return getToken(CypherParser.PLUSEQUAL, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public AddPropContext(SetItemContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class SetPropsContext extends SetItemContext {
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public TerminalNode EQ() {
return getToken(CypherParser.EQ, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public SetPropsContext(SetItemContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class SetLabelsContext extends SetItemContext {
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public NodeLabelsContext nodeLabels() {
return getRuleContext(NodeLabelsContext.class, 0);
}
public SetLabelsContext(SetItemContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class SetLabelsIsContext extends SetItemContext {
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public NodeLabelsIsContext nodeLabelsIs() {
return getRuleContext(NodeLabelsIsContext.class, 0);
}
public SetLabelsIsContext(SetItemContext ctx) {
copyFrom(ctx);
}
}
public final SetItemContext setItem() throws RecognitionException {
SetItemContext _localctx = new SetItemContext(_ctx, getState());
enterRule(_localctx, 44, RULE_setItem);
try {
setState(775);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 22, _ctx)) {
case 1:
_localctx = new SetPropContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(757);
propertyExpression();
setState(758);
match(EQ);
setState(759);
expression();
}
break;
case 2:
_localctx = new SetPropsContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(761);
variable();
setState(762);
match(EQ);
setState(763);
expression();
}
break;
case 3:
_localctx = new AddPropContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(765);
variable();
setState(766);
match(PLUSEQUAL);
setState(767);
expression();
}
break;
case 4:
_localctx = new SetLabelsContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(769);
variable();
setState(770);
nodeLabels();
}
break;
case 5:
_localctx = new SetLabelsIsContext(_localctx);
enterOuterAlt(_localctx, 5);
{
setState(772);
variable();
setState(773);
nodeLabelsIs();
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RemoveClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode REMOVE() {
return getToken(CypherParser.REMOVE, 0);
}
public List removeItem() {
return getRuleContexts(RemoveItemContext.class);
}
public RemoveItemContext removeItem(int i) {
return getRuleContext(RemoveItemContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public RemoveClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_removeClause;
}
}
public final RemoveClauseContext removeClause() throws RecognitionException {
RemoveClauseContext _localctx = new RemoveClauseContext(_ctx, getState());
enterRule(_localctx, 46, RULE_removeClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(777);
match(REMOVE);
setState(778);
removeItem();
setState(783);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(779);
match(COMMA);
setState(780);
removeItem();
}
}
setState(785);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RemoveItemContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public RemoveItemContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_removeItem;
}
public RemoveItemContext() {}
public void copyFrom(RemoveItemContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class RemoveLabelsIsContext extends RemoveItemContext {
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public NodeLabelsIsContext nodeLabelsIs() {
return getRuleContext(NodeLabelsIsContext.class, 0);
}
public RemoveLabelsIsContext(RemoveItemContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class RemoveLabelsContext extends RemoveItemContext {
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public NodeLabelsContext nodeLabels() {
return getRuleContext(NodeLabelsContext.class, 0);
}
public RemoveLabelsContext(RemoveItemContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class RemovePropContext extends RemoveItemContext {
public PropertyExpressionContext propertyExpression() {
return getRuleContext(PropertyExpressionContext.class, 0);
}
public RemovePropContext(RemoveItemContext ctx) {
copyFrom(ctx);
}
}
public final RemoveItemContext removeItem() throws RecognitionException {
RemoveItemContext _localctx = new RemoveItemContext(_ctx, getState());
enterRule(_localctx, 48, RULE_removeItem);
try {
setState(793);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 24, _ctx)) {
case 1:
_localctx = new RemovePropContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(786);
propertyExpression();
}
break;
case 2:
_localctx = new RemoveLabelsContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(787);
variable();
setState(788);
nodeLabels();
}
break;
case 3:
_localctx = new RemoveLabelsIsContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(790);
variable();
setState(791);
nodeLabelsIs();
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DeleteClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DELETE() {
return getToken(CypherParser.DELETE, 0);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public TerminalNode DETACH() {
return getToken(CypherParser.DETACH, 0);
}
public TerminalNode NODETACH() {
return getToken(CypherParser.NODETACH, 0);
}
public DeleteClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_deleteClause;
}
}
public final DeleteClauseContext deleteClause() throws RecognitionException {
DeleteClauseContext _localctx = new DeleteClauseContext(_ctx, getState());
enterRule(_localctx, 50, RULE_deleteClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(796);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == DETACH || _la == NODETACH) {
{
setState(795);
_la = _input.LA(1);
if (!(_la == DETACH || _la == NODETACH)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(798);
match(DELETE);
setState(799);
expression();
setState(804);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(800);
match(COMMA);
setState(801);
expression();
}
}
setState(806);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MatchClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode MATCH() {
return getToken(CypherParser.MATCH, 0);
}
public PatternListContext patternList() {
return getRuleContext(PatternListContext.class, 0);
}
public TerminalNode OPTIONAL() {
return getToken(CypherParser.OPTIONAL, 0);
}
public MatchModeContext matchMode() {
return getRuleContext(MatchModeContext.class, 0);
}
public List hint() {
return getRuleContexts(HintContext.class);
}
public HintContext hint(int i) {
return getRuleContext(HintContext.class, i);
}
public WhereClauseContext whereClause() {
return getRuleContext(WhereClauseContext.class, 0);
}
public MatchClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_matchClause;
}
}
public final MatchClauseContext matchClause() throws RecognitionException {
MatchClauseContext _localctx = new MatchClauseContext(_ctx, getState());
enterRule(_localctx, 52, RULE_matchClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(808);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == OPTIONAL) {
{
setState(807);
match(OPTIONAL);
}
}
setState(810);
match(MATCH);
setState(812);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 28, _ctx)) {
case 1:
{
setState(811);
matchMode();
}
break;
}
setState(814);
patternList();
setState(818);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == USING) {
{
{
setState(815);
hint();
}
}
setState(820);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(822);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE) {
{
setState(821);
whereClause();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MatchModeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode REPEATABLE() {
return getToken(CypherParser.REPEATABLE, 0);
}
public TerminalNode ELEMENT() {
return getToken(CypherParser.ELEMENT, 0);
}
public TerminalNode ELEMENTS() {
return getToken(CypherParser.ELEMENTS, 0);
}
public TerminalNode BINDINGS() {
return getToken(CypherParser.BINDINGS, 0);
}
public TerminalNode DIFFERENT() {
return getToken(CypherParser.DIFFERENT, 0);
}
public TerminalNode RELATIONSHIP() {
return getToken(CypherParser.RELATIONSHIP, 0);
}
public TerminalNode RELATIONSHIPS() {
return getToken(CypherParser.RELATIONSHIPS, 0);
}
public MatchModeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_matchMode;
}
}
public final MatchModeContext matchMode() throws RecognitionException {
MatchModeContext _localctx = new MatchModeContext(_ctx, getState());
enterRule(_localctx, 54, RULE_matchMode);
try {
setState(840);
_errHandler.sync(this);
switch (_input.LA(1)) {
case REPEATABLE:
enterOuterAlt(_localctx, 1);
{
setState(824);
match(REPEATABLE);
setState(830);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ELEMENT:
{
setState(825);
match(ELEMENT);
setState(827);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 31, _ctx)) {
case 1:
{
setState(826);
match(BINDINGS);
}
break;
}
}
break;
case ELEMENTS:
{
setState(829);
match(ELEMENTS);
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
case DIFFERENT:
enterOuterAlt(_localctx, 2);
{
setState(832);
match(DIFFERENT);
setState(838);
_errHandler.sync(this);
switch (_input.LA(1)) {
case RELATIONSHIP:
{
setState(833);
match(RELATIONSHIP);
setState(835);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 33, _ctx)) {
case 1:
{
setState(834);
match(BINDINGS);
}
break;
}
}
break;
case RELATIONSHIPS:
{
setState(837);
match(RELATIONSHIPS);
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class HintContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USING() {
return getToken(CypherParser.USING, 0);
}
public TerminalNode JOIN() {
return getToken(CypherParser.JOIN, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public NonEmptyNameListContext nonEmptyNameList() {
return getRuleContext(NonEmptyNameListContext.class, 0);
}
public TerminalNode SCAN() {
return getToken(CypherParser.SCAN, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public LabelOrRelTypeContext labelOrRelType() {
return getRuleContext(LabelOrRelTypeContext.class, 0);
}
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public TerminalNode INDEX() {
return getToken(CypherParser.INDEX, 0);
}
public TerminalNode BTREE() {
return getToken(CypherParser.BTREE, 0);
}
public TerminalNode TEXT() {
return getToken(CypherParser.TEXT, 0);
}
public TerminalNode RANGE() {
return getToken(CypherParser.RANGE, 0);
}
public TerminalNode POINT() {
return getToken(CypherParser.POINT, 0);
}
public TerminalNode SEEK() {
return getToken(CypherParser.SEEK, 0);
}
public HintContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_hint;
}
}
public final HintContext hint() throws RecognitionException {
HintContext _localctx = new HintContext(_ctx, getState());
enterRule(_localctx, 56, RULE_hint);
try {
enterOuterAlt(_localctx, 1);
{
setState(842);
match(USING);
setState(870);
_errHandler.sync(this);
switch (_input.LA(1)) {
case BTREE:
case INDEX:
case POINT:
case RANGE:
case TEXT:
{
{
setState(852);
_errHandler.sync(this);
switch (_input.LA(1)) {
case INDEX:
{
setState(843);
match(INDEX);
}
break;
case BTREE:
{
setState(844);
match(BTREE);
setState(845);
match(INDEX);
}
break;
case TEXT:
{
setState(846);
match(TEXT);
setState(847);
match(INDEX);
}
break;
case RANGE:
{
setState(848);
match(RANGE);
setState(849);
match(INDEX);
}
break;
case POINT:
{
setState(850);
match(POINT);
setState(851);
match(INDEX);
}
break;
default:
throw new NoViableAltException(this);
}
setState(855);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 37, _ctx)) {
case 1:
{
setState(854);
match(SEEK);
}
break;
}
setState(857);
variable();
setState(858);
labelOrRelType();
setState(859);
match(LPAREN);
setState(860);
nonEmptyNameList();
setState(861);
match(RPAREN);
}
}
break;
case JOIN:
{
setState(863);
match(JOIN);
setState(864);
match(ON);
setState(865);
nonEmptyNameList();
}
break;
case SCAN:
{
setState(866);
match(SCAN);
setState(867);
variable();
setState(868);
labelOrRelType();
}
break;
default:
throw new NoViableAltException(this);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MergeClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode MERGE() {
return getToken(CypherParser.MERGE, 0);
}
public PatternContext pattern() {
return getRuleContext(PatternContext.class, 0);
}
public List mergeAction() {
return getRuleContexts(MergeActionContext.class);
}
public MergeActionContext mergeAction(int i) {
return getRuleContext(MergeActionContext.class, i);
}
public MergeClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_mergeClause;
}
}
public final MergeClauseContext mergeClause() throws RecognitionException {
MergeClauseContext _localctx = new MergeClauseContext(_ctx, getState());
enterRule(_localctx, 58, RULE_mergeClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(872);
match(MERGE);
setState(873);
pattern();
setState(877);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == ON) {
{
{
setState(874);
mergeAction();
}
}
setState(879);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MergeActionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public SetClauseContext setClause() {
return getRuleContext(SetClauseContext.class, 0);
}
public TerminalNode MATCH() {
return getToken(CypherParser.MATCH, 0);
}
public TerminalNode CREATE() {
return getToken(CypherParser.CREATE, 0);
}
public MergeActionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_mergeAction;
}
}
public final MergeActionContext mergeAction() throws RecognitionException {
MergeActionContext _localctx = new MergeActionContext(_ctx, getState());
enterRule(_localctx, 60, RULE_mergeAction);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(880);
match(ON);
setState(881);
_la = _input.LA(1);
if (!(_la == CREATE || _la == MATCH)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(882);
setClause();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UnwindClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode UNWIND() {
return getToken(CypherParser.UNWIND, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public TerminalNode AS() {
return getToken(CypherParser.AS, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public UnwindClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_unwindClause;
}
}
public final UnwindClauseContext unwindClause() throws RecognitionException {
UnwindClauseContext _localctx = new UnwindClauseContext(_ctx, getState());
enterRule(_localctx, 62, RULE_unwindClause);
try {
enterOuterAlt(_localctx, 1);
{
setState(884);
match(UNWIND);
setState(885);
expression();
setState(886);
match(AS);
setState(887);
variable();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CallClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CALL() {
return getToken(CypherParser.CALL, 0);
}
public NamespaceContext namespace() {
return getRuleContext(NamespaceContext.class, 0);
}
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public TerminalNode YIELD() {
return getToken(CypherParser.YIELD, 0);
}
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public List procedureResultItem() {
return getRuleContexts(ProcedureResultItemContext.class);
}
public ProcedureResultItemContext procedureResultItem(int i) {
return getRuleContext(ProcedureResultItemContext.class, i);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public WhereClauseContext whereClause() {
return getRuleContext(WhereClauseContext.class, 0);
}
public CallClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_callClause;
}
}
public final CallClauseContext callClause() throws RecognitionException {
CallClauseContext _localctx = new CallClauseContext(_ctx, getState());
enterRule(_localctx, 64, RULE_callClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(889);
match(CALL);
setState(890);
namespace();
setState(891);
symbolicNameString();
setState(904);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == LPAREN) {
{
setState(892);
match(LPAREN);
setState(901);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -61572919590928L) != 0)
|| ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -9288676379058177L) != 0)
|| ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -2305843009449101697L) != 0)
|| ((((_la - 193)) & ~0x3f) == 0 && ((1L << (_la - 193)) & -576460823170418305L) != 0)
|| ((((_la - 257)) & ~0x3f) == 0 && ((1L << (_la - 257)) & 4294967295L) != 0)) {
{
setState(893);
expression();
setState(898);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(894);
match(COMMA);
setState(895);
expression();
}
}
setState(900);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(903);
match(RPAREN);
}
}
setState(921);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == YIELD) {
{
setState(906);
match(YIELD);
setState(919);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(907);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
{
setState(908);
procedureResultItem();
setState(913);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(909);
match(COMMA);
setState(910);
procedureResultItem();
}
}
setState(915);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(917);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE) {
{
setState(916);
whereClause();
}
}
}
break;
default:
throw new NoViableAltException(this);
}
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ProcedureResultItemContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public TerminalNode AS() {
return getToken(CypherParser.AS, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public ProcedureResultItemContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_procedureResultItem;
}
}
public final ProcedureResultItemContext procedureResultItem() throws RecognitionException {
ProcedureResultItemContext _localctx = new ProcedureResultItemContext(_ctx, getState());
enterRule(_localctx, 66, RULE_procedureResultItem);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(923);
symbolicNameString();
setState(926);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == AS) {
{
setState(924);
match(AS);
setState(925);
variable();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LoadCSVClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LOAD() {
return getToken(CypherParser.LOAD, 0);
}
public TerminalNode CSV() {
return getToken(CypherParser.CSV, 0);
}
public TerminalNode FROM() {
return getToken(CypherParser.FROM, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public TerminalNode AS() {
return getToken(CypherParser.AS, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public TerminalNode WITH() {
return getToken(CypherParser.WITH, 0);
}
public TerminalNode HEADERS() {
return getToken(CypherParser.HEADERS, 0);
}
public TerminalNode FIELDTERMINATOR() {
return getToken(CypherParser.FIELDTERMINATOR, 0);
}
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class, 0);
}
public LoadCSVClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_loadCSVClause;
}
}
public final LoadCSVClauseContext loadCSVClause() throws RecognitionException {
LoadCSVClauseContext _localctx = new LoadCSVClauseContext(_ctx, getState());
enterRule(_localctx, 68, RULE_loadCSVClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(928);
match(LOAD);
setState(929);
match(CSV);
setState(932);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WITH) {
{
setState(930);
match(WITH);
setState(931);
match(HEADERS);
}
}
setState(934);
match(FROM);
setState(935);
expression();
setState(936);
match(AS);
setState(937);
variable();
setState(940);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == FIELDTERMINATOR) {
{
setState(938);
match(FIELDTERMINATOR);
setState(939);
stringLiteral();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ForeachClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode FOREACH() {
return getToken(CypherParser.FOREACH, 0);
}
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public TerminalNode IN() {
return getToken(CypherParser.IN, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public TerminalNode BAR() {
return getToken(CypherParser.BAR, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public List clause() {
return getRuleContexts(ClauseContext.class);
}
public ClauseContext clause(int i) {
return getRuleContext(ClauseContext.class, i);
}
public ForeachClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_foreachClause;
}
}
public final ForeachClauseContext foreachClause() throws RecognitionException {
ForeachClauseContext _localctx = new ForeachClauseContext(_ctx, getState());
enterRule(_localctx, 70, RULE_foreachClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(942);
match(FOREACH);
setState(943);
match(LPAREN);
setState(944);
variable();
setState(945);
match(IN);
setState(946);
expression();
setState(947);
match(BAR);
setState(949);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(948);
clause();
}
}
setState(951);
_errHandler.sync(this);
_la = _input.LA(1);
} while (((((_la - 38)) & ~0x3f) == 0 && ((1L << (_la - 38)) & 36507746305L) != 0)
|| ((((_la - 105)) & ~0x3f) == 0 && ((1L << (_la - 105)) & 1153027332605214729L) != 0)
|| ((((_la - 177)) & ~0x3f) == 0 && ((1L << (_la - 177)) & 36031030401957889L) != 0)
|| ((((_la - 269)) & ~0x3f) == 0 && ((1L << (_la - 269)) & 8197L) != 0));
setState(953);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SubqueryClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CALL() {
return getToken(CypherParser.CALL, 0);
}
public TerminalNode LCURLY() {
return getToken(CypherParser.LCURLY, 0);
}
public RegularQueryContext regularQuery() {
return getRuleContext(RegularQueryContext.class, 0);
}
public TerminalNode RCURLY() {
return getToken(CypherParser.RCURLY, 0);
}
public SubqueryInTransactionsParametersContext subqueryInTransactionsParameters() {
return getRuleContext(SubqueryInTransactionsParametersContext.class, 0);
}
public SubqueryClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_subqueryClause;
}
}
public final SubqueryClauseContext subqueryClause() throws RecognitionException {
SubqueryClauseContext _localctx = new SubqueryClauseContext(_ctx, getState());
enterRule(_localctx, 72, RULE_subqueryClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(955);
match(CALL);
setState(956);
match(LCURLY);
setState(957);
regularQuery();
setState(958);
match(RCURLY);
setState(960);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IN) {
{
setState(959);
subqueryInTransactionsParameters();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SubqueryInTransactionsParametersContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode IN() {
return getToken(CypherParser.IN, 0);
}
public TerminalNode TRANSACTIONS() {
return getToken(CypherParser.TRANSACTIONS, 0);
}
public TerminalNode CONCURRENT() {
return getToken(CypherParser.CONCURRENT, 0);
}
public List subqueryInTransactionsBatchParameters() {
return getRuleContexts(SubqueryInTransactionsBatchParametersContext.class);
}
public SubqueryInTransactionsBatchParametersContext subqueryInTransactionsBatchParameters(int i) {
return getRuleContext(SubqueryInTransactionsBatchParametersContext.class, i);
}
public List subqueryInTransactionsErrorParameters() {
return getRuleContexts(SubqueryInTransactionsErrorParametersContext.class);
}
public SubqueryInTransactionsErrorParametersContext subqueryInTransactionsErrorParameters(int i) {
return getRuleContext(SubqueryInTransactionsErrorParametersContext.class, i);
}
public List subqueryInTransactionsReportParameters() {
return getRuleContexts(SubqueryInTransactionsReportParametersContext.class);
}
public SubqueryInTransactionsReportParametersContext subqueryInTransactionsReportParameters(int i) {
return getRuleContext(SubqueryInTransactionsReportParametersContext.class, i);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public SubqueryInTransactionsParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_subqueryInTransactionsParameters;
}
}
public final SubqueryInTransactionsParametersContext subqueryInTransactionsParameters()
throws RecognitionException {
SubqueryInTransactionsParametersContext _localctx =
new SubqueryInTransactionsParametersContext(_ctx, getState());
enterRule(_localctx, 74, RULE_subqueryInTransactionsParameters);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(962);
match(IN);
setState(967);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 53, _ctx)) {
case 1:
{
setState(964);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 52, _ctx)) {
case 1:
{
setState(963);
expression();
}
break;
}
setState(966);
match(CONCURRENT);
}
break;
}
setState(969);
match(TRANSACTIONS);
setState(975);
_errHandler.sync(this);
_la = _input.LA(1);
while (((((_la - 174)) & ~0x3f) == 0 && ((1L << (_la - 174)) & 2199023255555L) != 0)) {
{
setState(973);
_errHandler.sync(this);
switch (_input.LA(1)) {
case OF:
{
setState(970);
subqueryInTransactionsBatchParameters();
}
break;
case ON:
{
setState(971);
subqueryInTransactionsErrorParameters();
}
break;
case REPORT:
{
setState(972);
subqueryInTransactionsReportParameters();
}
break;
default:
throw new NoViableAltException(this);
}
}
setState(977);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SubqueryInTransactionsBatchParametersContext
extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode OF() {
return getToken(CypherParser.OF, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public TerminalNode ROW() {
return getToken(CypherParser.ROW, 0);
}
public TerminalNode ROWS() {
return getToken(CypherParser.ROWS, 0);
}
public SubqueryInTransactionsBatchParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_subqueryInTransactionsBatchParameters;
}
}
public final SubqueryInTransactionsBatchParametersContext subqueryInTransactionsBatchParameters()
throws RecognitionException {
SubqueryInTransactionsBatchParametersContext _localctx =
new SubqueryInTransactionsBatchParametersContext(_ctx, getState());
enterRule(_localctx, 76, RULE_subqueryInTransactionsBatchParameters);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(978);
match(OF);
setState(979);
expression();
setState(980);
_la = _input.LA(1);
if (!(_la == ROW || _la == ROWS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SubqueryInTransactionsErrorParametersContext
extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public TerminalNode ERROR() {
return getToken(CypherParser.ERROR, 0);
}
public TerminalNode CONTINUE() {
return getToken(CypherParser.CONTINUE, 0);
}
public TerminalNode BREAK() {
return getToken(CypherParser.BREAK, 0);
}
public TerminalNode FAIL() {
return getToken(CypherParser.FAIL, 0);
}
public SubqueryInTransactionsErrorParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_subqueryInTransactionsErrorParameters;
}
}
public final SubqueryInTransactionsErrorParametersContext subqueryInTransactionsErrorParameters()
throws RecognitionException {
SubqueryInTransactionsErrorParametersContext _localctx =
new SubqueryInTransactionsErrorParametersContext(_ctx, getState());
enterRule(_localctx, 78, RULE_subqueryInTransactionsErrorParameters);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(982);
match(ON);
setState(983);
match(ERROR);
setState(984);
_la = _input.LA(1);
if (!(_la == BREAK || _la == CONTINUE || _la == FAIL)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SubqueryInTransactionsReportParametersContext
extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode REPORT() {
return getToken(CypherParser.REPORT, 0);
}
public TerminalNode STATUS() {
return getToken(CypherParser.STATUS, 0);
}
public TerminalNode AS() {
return getToken(CypherParser.AS, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public SubqueryInTransactionsReportParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_subqueryInTransactionsReportParameters;
}
}
public final SubqueryInTransactionsReportParametersContext subqueryInTransactionsReportParameters()
throws RecognitionException {
SubqueryInTransactionsReportParametersContext _localctx =
new SubqueryInTransactionsReportParametersContext(_ctx, getState());
enterRule(_localctx, 80, RULE_subqueryInTransactionsReportParameters);
try {
enterOuterAlt(_localctx, 1);
{
setState(986);
match(REPORT);
setState(987);
match(STATUS);
setState(988);
match(AS);
setState(989);
variable();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PatternListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List pattern() {
return getRuleContexts(PatternContext.class);
}
public PatternContext pattern(int i) {
return getRuleContext(PatternContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public PatternListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_patternList;
}
}
public final PatternListContext patternList() throws RecognitionException {
PatternListContext _localctx = new PatternListContext(_ctx, getState());
enterRule(_localctx, 82, RULE_patternList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(991);
pattern();
setState(996);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(992);
match(COMMA);
setState(993);
pattern();
}
}
setState(998);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InsertPatternListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List insertPattern() {
return getRuleContexts(InsertPatternContext.class);
}
public InsertPatternContext insertPattern(int i) {
return getRuleContext(InsertPatternContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public InsertPatternListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_insertPatternList;
}
}
public final InsertPatternListContext insertPatternList() throws RecognitionException {
InsertPatternListContext _localctx = new InsertPatternListContext(_ctx, getState());
enterRule(_localctx, 84, RULE_insertPatternList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(999);
insertPattern();
setState(1004);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(1000);
match(COMMA);
setState(1001);
insertPattern();
}
}
setState(1006);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public AnonymousPatternContext anonymousPattern() {
return getRuleContext(AnonymousPatternContext.class, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public TerminalNode EQ() {
return getToken(CypherParser.EQ, 0);
}
public SelectorContext selector() {
return getRuleContext(SelectorContext.class, 0);
}
public PatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_pattern;
}
}
public final PatternContext pattern() throws RecognitionException {
PatternContext _localctx = new PatternContext(_ctx, getState());
enterRule(_localctx, 86, RULE_pattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1010);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 58, _ctx)) {
case 1:
{
setState(1007);
variable();
setState(1008);
match(EQ);
}
break;
}
setState(1013);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == ALL || _la == ANY || _la == SHORTEST) {
{
setState(1012);
selector();
}
}
setState(1015);
anonymousPattern();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InsertPatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List insertNodePattern() {
return getRuleContexts(InsertNodePatternContext.class);
}
public InsertNodePatternContext insertNodePattern(int i) {
return getRuleContext(InsertNodePatternContext.class, i);
}
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public TerminalNode EQ() {
return getToken(CypherParser.EQ, 0);
}
public List insertRelationshipPattern() {
return getRuleContexts(InsertRelationshipPatternContext.class);
}
public InsertRelationshipPatternContext insertRelationshipPattern(int i) {
return getRuleContext(InsertRelationshipPatternContext.class, i);
}
public InsertPatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_insertPattern;
}
}
public final InsertPatternContext insertPattern() throws RecognitionException {
InsertPatternContext _localctx = new InsertPatternContext(_ctx, getState());
enterRule(_localctx, 88, RULE_insertPattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1020);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -61572919591936L) != 0)
|| ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -9288676379060225L) != 0)
|| ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -3458764514072989569L) != 0)
|| ((((_la - 193)) & ~0x3f) == 0 && ((1L << (_la - 193)) & -576460823170418305L) != 0)
|| ((((_la - 257)) & ~0x3f) == 0 && ((1L << (_la - 257)) & 4294967295L) != 0)) {
{
setState(1017);
symbolicNameString();
setState(1018);
match(EQ);
}
}
setState(1022);
insertNodePattern();
setState(1028);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == LT || _la == MINUS || _la == ARROW_LINE || _la == ARROW_LEFT_HEAD) {
{
{
setState(1023);
insertRelationshipPattern();
setState(1024);
insertNodePattern();
}
}
setState(1030);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class QuantifierContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public Token from;
public Token to;
public TerminalNode LCURLY() {
return getToken(CypherParser.LCURLY, 0);
}
public List UNSIGNED_DECIMAL_INTEGER() {
return getTokens(CypherParser.UNSIGNED_DECIMAL_INTEGER);
}
public TerminalNode UNSIGNED_DECIMAL_INTEGER(int i) {
return getToken(CypherParser.UNSIGNED_DECIMAL_INTEGER, i);
}
public TerminalNode RCURLY() {
return getToken(CypherParser.RCURLY, 0);
}
public TerminalNode COMMA() {
return getToken(CypherParser.COMMA, 0);
}
public TerminalNode PLUS() {
return getToken(CypherParser.PLUS, 0);
}
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public QuantifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_quantifier;
}
}
public final QuantifierContext quantifier() throws RecognitionException {
QuantifierContext _localctx = new QuantifierContext(_ctx, getState());
enterRule(_localctx, 90, RULE_quantifier);
int _la;
try {
setState(1045);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 64, _ctx)) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(1031);
match(LCURLY);
setState(1032);
match(UNSIGNED_DECIMAL_INTEGER);
setState(1033);
match(RCURLY);
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(1034);
match(LCURLY);
setState(1036);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == UNSIGNED_DECIMAL_INTEGER) {
{
setState(1035);
((QuantifierContext) _localctx).from = match(UNSIGNED_DECIMAL_INTEGER);
}
}
setState(1038);
match(COMMA);
setState(1040);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == UNSIGNED_DECIMAL_INTEGER) {
{
setState(1039);
((QuantifierContext) _localctx).to = match(UNSIGNED_DECIMAL_INTEGER);
}
}
setState(1042);
match(RCURLY);
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(1043);
match(PLUS);
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(1044);
match(TIMES);
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AnonymousPatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ShortestPathPatternContext shortestPathPattern() {
return getRuleContext(ShortestPathPatternContext.class, 0);
}
public PatternElementContext patternElement() {
return getRuleContext(PatternElementContext.class, 0);
}
public AnonymousPatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_anonymousPattern;
}
}
public final AnonymousPatternContext anonymousPattern() throws RecognitionException {
AnonymousPatternContext _localctx = new AnonymousPatternContext(_ctx, getState());
enterRule(_localctx, 92, RULE_anonymousPattern);
try {
setState(1049);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALL_SHORTEST_PATHS:
case SHORTEST_PATH:
enterOuterAlt(_localctx, 1);
{
setState(1047);
shortestPathPattern();
}
break;
case LPAREN:
enterOuterAlt(_localctx, 2);
{
setState(1048);
patternElement();
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShortestPathPatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public PatternElementContext patternElement() {
return getRuleContext(PatternElementContext.class, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public TerminalNode SHORTEST_PATH() {
return getToken(CypherParser.SHORTEST_PATH, 0);
}
public TerminalNode ALL_SHORTEST_PATHS() {
return getToken(CypherParser.ALL_SHORTEST_PATHS, 0);
}
public ShortestPathPatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_shortestPathPattern;
}
}
public final ShortestPathPatternContext shortestPathPattern() throws RecognitionException {
ShortestPathPatternContext _localctx = new ShortestPathPatternContext(_ctx, getState());
enterRule(_localctx, 94, RULE_shortestPathPattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1051);
_la = _input.LA(1);
if (!(_la == ALL_SHORTEST_PATHS || _la == SHORTEST_PATH)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1052);
match(LPAREN);
setState(1053);
patternElement();
setState(1054);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PatternElementContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List nodePattern() {
return getRuleContexts(NodePatternContext.class);
}
public NodePatternContext nodePattern(int i) {
return getRuleContext(NodePatternContext.class, i);
}
public List parenthesizedPath() {
return getRuleContexts(ParenthesizedPathContext.class);
}
public ParenthesizedPathContext parenthesizedPath(int i) {
return getRuleContext(ParenthesizedPathContext.class, i);
}
public List relationshipPattern() {
return getRuleContexts(RelationshipPatternContext.class);
}
public RelationshipPatternContext relationshipPattern(int i) {
return getRuleContext(RelationshipPatternContext.class, i);
}
public List quantifier() {
return getRuleContexts(QuantifierContext.class);
}
public QuantifierContext quantifier(int i) {
return getRuleContext(QuantifierContext.class, i);
}
public PatternElementContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_patternElement;
}
}
public final PatternElementContext patternElement() throws RecognitionException {
PatternElementContext _localctx = new PatternElementContext(_ctx, getState());
enterRule(_localctx, 96, RULE_patternElement);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1069);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
setState(1069);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 68, _ctx)) {
case 1:
{
setState(1056);
nodePattern();
setState(1065);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == LT || _la == MINUS || _la == ARROW_LINE || _la == ARROW_LEFT_HEAD) {
{
{
setState(1057);
relationshipPattern();
setState(1059);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == LCURLY || _la == PLUS || _la == TIMES) {
{
setState(1058);
quantifier();
}
}
setState(1061);
nodePattern();
}
}
setState(1067);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
break;
case 2:
{
setState(1068);
parenthesizedPath();
}
break;
}
}
setState(1071);
_errHandler.sync(this);
_la = _input.LA(1);
} while (_la == LPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SelectorContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SelectorContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_selector;
}
public SelectorContext() {}
public void copyFrom(SelectorContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class AllShortestPathContext extends SelectorContext {
public TerminalNode ALL() {
return getToken(CypherParser.ALL, 0);
}
public TerminalNode SHORTEST() {
return getToken(CypherParser.SHORTEST, 0);
}
public TerminalNode PATH() {
return getToken(CypherParser.PATH, 0);
}
public AllShortestPathContext(SelectorContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class AnyPathContext extends SelectorContext {
public TerminalNode ANY() {
return getToken(CypherParser.ANY, 0);
}
public TerminalNode UNSIGNED_DECIMAL_INTEGER() {
return getToken(CypherParser.UNSIGNED_DECIMAL_INTEGER, 0);
}
public TerminalNode PATH() {
return getToken(CypherParser.PATH, 0);
}
public AnyPathContext(SelectorContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ShortestGroupContext extends SelectorContext {
public TerminalNode SHORTEST() {
return getToken(CypherParser.SHORTEST, 0);
}
public TerminalNode GROUP() {
return getToken(CypherParser.GROUP, 0);
}
public TerminalNode UNSIGNED_DECIMAL_INTEGER() {
return getToken(CypherParser.UNSIGNED_DECIMAL_INTEGER, 0);
}
public TerminalNode PATH() {
return getToken(CypherParser.PATH, 0);
}
public ShortestGroupContext(SelectorContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class AnyShortestPathContext extends SelectorContext {
public TerminalNode ANY() {
return getToken(CypherParser.ANY, 0);
}
public TerminalNode SHORTEST() {
return getToken(CypherParser.SHORTEST, 0);
}
public TerminalNode PATH() {
return getToken(CypherParser.PATH, 0);
}
public TerminalNode UNSIGNED_DECIMAL_INTEGER() {
return getToken(CypherParser.UNSIGNED_DECIMAL_INTEGER, 0);
}
public AnyShortestPathContext(SelectorContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class AllPathContext extends SelectorContext {
public TerminalNode ALL() {
return getToken(CypherParser.ALL, 0);
}
public TerminalNode PATH() {
return getToken(CypherParser.PATH, 0);
}
public AllPathContext(SelectorContext ctx) {
copyFrom(ctx);
}
}
public final SelectorContext selector() throws RecognitionException {
SelectorContext _localctx = new SelectorContext(_ctx, getState());
enterRule(_localctx, 98, RULE_selector);
int _la;
try {
setState(1107);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 78, _ctx)) {
case 1:
_localctx = new AnyShortestPathContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(1073);
match(ANY);
setState(1074);
match(SHORTEST);
setState(1076);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == PATH) {
{
setState(1075);
match(PATH);
}
}
}
break;
case 2:
_localctx = new AllShortestPathContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(1078);
match(ALL);
setState(1079);
match(SHORTEST);
setState(1081);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == PATH) {
{
setState(1080);
match(PATH);
}
}
}
break;
case 3:
_localctx = new AnyPathContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(1083);
match(ANY);
setState(1085);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == UNSIGNED_DECIMAL_INTEGER) {
{
setState(1084);
match(UNSIGNED_DECIMAL_INTEGER);
}
}
setState(1088);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == PATH) {
{
setState(1087);
match(PATH);
}
}
}
break;
case 4:
_localctx = new AllPathContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(1090);
match(ALL);
setState(1092);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == PATH) {
{
setState(1091);
match(PATH);
}
}
}
break;
case 5:
_localctx = new ShortestGroupContext(_localctx);
enterOuterAlt(_localctx, 5);
{
setState(1094);
match(SHORTEST);
setState(1096);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == UNSIGNED_DECIMAL_INTEGER) {
{
setState(1095);
match(UNSIGNED_DECIMAL_INTEGER);
}
}
setState(1099);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == PATH) {
{
setState(1098);
match(PATH);
}
}
setState(1101);
match(GROUP);
}
break;
case 6:
_localctx = new AnyShortestPathContext(_localctx);
enterOuterAlt(_localctx, 6);
{
setState(1102);
match(SHORTEST);
setState(1103);
match(UNSIGNED_DECIMAL_INTEGER);
setState(1105);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == PATH) {
{
setState(1104);
match(PATH);
}
}
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PathPatternNonEmptyContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List nodePattern() {
return getRuleContexts(NodePatternContext.class);
}
public NodePatternContext nodePattern(int i) {
return getRuleContext(NodePatternContext.class, i);
}
public List relationshipPattern() {
return getRuleContexts(RelationshipPatternContext.class);
}
public RelationshipPatternContext relationshipPattern(int i) {
return getRuleContext(RelationshipPatternContext.class, i);
}
public PathPatternNonEmptyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_pathPatternNonEmpty;
}
}
public final PathPatternNonEmptyContext pathPatternNonEmpty() throws RecognitionException {
PathPatternNonEmptyContext _localctx = new PathPatternNonEmptyContext(_ctx, getState());
enterRule(_localctx, 100, RULE_pathPatternNonEmpty);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1109);
nodePattern();
setState(1113);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(1110);
relationshipPattern();
setState(1111);
nodePattern();
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(1115);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 79, _ctx);
} while (_alt != 2 && _alt != org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NodePatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public LabelExpressionContext labelExpression() {
return getRuleContext(LabelExpressionContext.class, 0);
}
public PropertiesContext properties() {
return getRuleContext(PropertiesContext.class, 0);
}
public TerminalNode WHERE() {
return getToken(CypherParser.WHERE, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public NodePatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_nodePattern;
}
}
public final NodePatternContext nodePattern() throws RecognitionException {
NodePatternContext _localctx = new NodePatternContext(_ctx, getState());
enterRule(_localctx, 102, RULE_nodePattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1117);
match(LPAREN);
setState(1119);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 80, _ctx)) {
case 1:
{
setState(1118);
variable();
}
break;
}
setState(1122);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == COLON || _la == IS) {
{
setState(1121);
labelExpression();
}
}
setState(1125);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == DOLLAR || _la == LCURLY) {
{
setState(1124);
properties();
}
}
setState(1129);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE) {
{
setState(1127);
match(WHERE);
setState(1128);
expression();
}
}
setState(1131);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InsertNodePatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public InsertNodeLabelExpressionContext insertNodeLabelExpression() {
return getRuleContext(InsertNodeLabelExpressionContext.class, 0);
}
public PropertiesContext properties() {
return getRuleContext(PropertiesContext.class, 0);
}
public InsertNodePatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_insertNodePattern;
}
}
public final InsertNodePatternContext insertNodePattern() throws RecognitionException {
InsertNodePatternContext _localctx = new InsertNodePatternContext(_ctx, getState());
enterRule(_localctx, 104, RULE_insertNodePattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1133);
match(LPAREN);
setState(1135);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 84, _ctx)) {
case 1:
{
setState(1134);
variable();
}
break;
}
setState(1138);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == COLON || _la == IS) {
{
setState(1137);
insertNodeLabelExpression();
}
}
setState(1141);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == DOLLAR || _la == LCURLY) {
{
setState(1140);
properties();
}
}
setState(1143);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParenthesizedPathContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public PatternContext pattern() {
return getRuleContext(PatternContext.class, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public TerminalNode WHERE() {
return getToken(CypherParser.WHERE, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public QuantifierContext quantifier() {
return getRuleContext(QuantifierContext.class, 0);
}
public ParenthesizedPathContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_parenthesizedPath;
}
}
public final ParenthesizedPathContext parenthesizedPath() throws RecognitionException {
ParenthesizedPathContext _localctx = new ParenthesizedPathContext(_ctx, getState());
enterRule(_localctx, 106, RULE_parenthesizedPath);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1145);
match(LPAREN);
setState(1146);
pattern();
setState(1149);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE) {
{
setState(1147);
match(WHERE);
setState(1148);
expression();
}
}
setState(1151);
match(RPAREN);
setState(1153);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == LCURLY || _la == PLUS || _la == TIMES) {
{
setState(1152);
quantifier();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NodeLabelsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List labelType() {
return getRuleContexts(LabelTypeContext.class);
}
public LabelTypeContext labelType(int i) {
return getRuleContext(LabelTypeContext.class, i);
}
public NodeLabelsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_nodeLabels;
}
}
public final NodeLabelsContext nodeLabels() throws RecognitionException {
NodeLabelsContext _localctx = new NodeLabelsContext(_ctx, getState());
enterRule(_localctx, 108, RULE_nodeLabels);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1156);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(1155);
labelType();
}
}
setState(1158);
_errHandler.sync(this);
_la = _input.LA(1);
} while (_la == COLON);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NodeLabelsIsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode IS() {
return getToken(CypherParser.IS, 0);
}
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public List labelType() {
return getRuleContexts(LabelTypeContext.class);
}
public LabelTypeContext labelType(int i) {
return getRuleContext(LabelTypeContext.class, i);
}
public NodeLabelsIsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_nodeLabelsIs;
}
}
public final NodeLabelsIsContext nodeLabelsIs() throws RecognitionException {
NodeLabelsIsContext _localctx = new NodeLabelsIsContext(_ctx, getState());
enterRule(_localctx, 110, RULE_nodeLabelsIs);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1160);
match(IS);
setState(1161);
symbolicNameString();
setState(1165);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COLON) {
{
{
setState(1162);
labelType();
}
}
setState(1167);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COLON() {
return getToken(CypherParser.COLON, 0);
}
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public LabelTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_labelType;
}
}
public final LabelTypeContext labelType() throws RecognitionException {
LabelTypeContext _localctx = new LabelTypeContext(_ctx, getState());
enterRule(_localctx, 112, RULE_labelType);
try {
enterOuterAlt(_localctx, 1);
{
setState(1168);
match(COLON);
setState(1169);
symbolicNameString();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RelTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COLON() {
return getToken(CypherParser.COLON, 0);
}
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public RelTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_relType;
}
}
public final RelTypeContext relType() throws RecognitionException {
RelTypeContext _localctx = new RelTypeContext(_ctx, getState());
enterRule(_localctx, 114, RULE_relType);
try {
enterOuterAlt(_localctx, 1);
{
setState(1171);
match(COLON);
setState(1172);
symbolicNameString();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelOrRelTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COLON() {
return getToken(CypherParser.COLON, 0);
}
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public LabelOrRelTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_labelOrRelType;
}
}
public final LabelOrRelTypeContext labelOrRelType() throws RecognitionException {
LabelOrRelTypeContext _localctx = new LabelOrRelTypeContext(_ctx, getState());
enterRule(_localctx, 116, RULE_labelOrRelType);
try {
enterOuterAlt(_localctx, 1);
{
setState(1174);
match(COLON);
setState(1175);
symbolicNameString();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PropertiesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public MapContext map() {
return getRuleContext(MapContext.class, 0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class, 0);
}
public PropertiesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_properties;
}
}
public final PropertiesContext properties() throws RecognitionException {
PropertiesContext _localctx = new PropertiesContext(_ctx, getState());
enterRule(_localctx, 118, RULE_properties);
try {
setState(1179);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LCURLY:
enterOuterAlt(_localctx, 1);
{
setState(1177);
map();
}
break;
case DOLLAR:
enterOuterAlt(_localctx, 2);
{
setState(1178);
parameter("ANY");
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RelationshipPatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List arrowLine() {
return getRuleContexts(ArrowLineContext.class);
}
public ArrowLineContext arrowLine(int i) {
return getRuleContext(ArrowLineContext.class, i);
}
public LeftArrowContext leftArrow() {
return getRuleContext(LeftArrowContext.class, 0);
}
public TerminalNode LBRACKET() {
return getToken(CypherParser.LBRACKET, 0);
}
public TerminalNode RBRACKET() {
return getToken(CypherParser.RBRACKET, 0);
}
public RightArrowContext rightArrow() {
return getRuleContext(RightArrowContext.class, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public LabelExpressionContext labelExpression() {
return getRuleContext(LabelExpressionContext.class, 0);
}
public PathLengthContext pathLength() {
return getRuleContext(PathLengthContext.class, 0);
}
public PropertiesContext properties() {
return getRuleContext(PropertiesContext.class, 0);
}
public TerminalNode WHERE() {
return getToken(CypherParser.WHERE, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public RelationshipPatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_relationshipPattern;
}
}
public final RelationshipPatternContext relationshipPattern() throws RecognitionException {
RelationshipPatternContext _localctx = new RelationshipPatternContext(_ctx, getState());
enterRule(_localctx, 120, RULE_relationshipPattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1182);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == LT || _la == ARROW_LEFT_HEAD) {
{
setState(1181);
leftArrow();
}
}
setState(1184);
arrowLine();
setState(1203);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == LBRACKET) {
{
setState(1185);
match(LBRACKET);
setState(1187);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 93, _ctx)) {
case 1:
{
setState(1186);
variable();
}
break;
}
setState(1190);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == COLON || _la == IS) {
{
setState(1189);
labelExpression();
}
}
setState(1193);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == TIMES) {
{
setState(1192);
pathLength();
}
}
setState(1196);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == DOLLAR || _la == LCURLY) {
{
setState(1195);
properties();
}
}
setState(1200);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE) {
{
setState(1198);
match(WHERE);
setState(1199);
expression();
}
}
setState(1202);
match(RBRACKET);
}
}
setState(1205);
arrowLine();
setState(1207);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == GT || _la == ARROW_RIGHT_HEAD) {
{
setState(1206);
rightArrow();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InsertRelationshipPatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List arrowLine() {
return getRuleContexts(ArrowLineContext.class);
}
public ArrowLineContext arrowLine(int i) {
return getRuleContext(ArrowLineContext.class, i);
}
public TerminalNode LBRACKET() {
return getToken(CypherParser.LBRACKET, 0);
}
public InsertRelationshipLabelExpressionContext insertRelationshipLabelExpression() {
return getRuleContext(InsertRelationshipLabelExpressionContext.class, 0);
}
public TerminalNode RBRACKET() {
return getToken(CypherParser.RBRACKET, 0);
}
public LeftArrowContext leftArrow() {
return getRuleContext(LeftArrowContext.class, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public PropertiesContext properties() {
return getRuleContext(PropertiesContext.class, 0);
}
public RightArrowContext rightArrow() {
return getRuleContext(RightArrowContext.class, 0);
}
public InsertRelationshipPatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_insertRelationshipPattern;
}
}
public final InsertRelationshipPatternContext insertRelationshipPattern() throws RecognitionException {
InsertRelationshipPatternContext _localctx = new InsertRelationshipPatternContext(_ctx, getState());
enterRule(_localctx, 122, RULE_insertRelationshipPattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1210);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == LT || _la == ARROW_LEFT_HEAD) {
{
setState(1209);
leftArrow();
}
}
setState(1212);
arrowLine();
setState(1213);
match(LBRACKET);
setState(1215);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 101, _ctx)) {
case 1:
{
setState(1214);
variable();
}
break;
}
setState(1217);
insertRelationshipLabelExpression();
setState(1219);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == DOLLAR || _la == LCURLY) {
{
setState(1218);
properties();
}
}
setState(1221);
match(RBRACKET);
setState(1222);
arrowLine();
setState(1224);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == GT || _la == ARROW_RIGHT_HEAD) {
{
setState(1223);
rightArrow();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LeftArrowContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LT() {
return getToken(CypherParser.LT, 0);
}
public TerminalNode ARROW_LEFT_HEAD() {
return getToken(CypherParser.ARROW_LEFT_HEAD, 0);
}
public LeftArrowContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_leftArrow;
}
}
public final LeftArrowContext leftArrow() throws RecognitionException {
LeftArrowContext _localctx = new LeftArrowContext(_ctx, getState());
enterRule(_localctx, 124, RULE_leftArrow);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1226);
_la = _input.LA(1);
if (!(_la == LT || _la == ARROW_LEFT_HEAD)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ArrowLineContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ARROW_LINE() {
return getToken(CypherParser.ARROW_LINE, 0);
}
public TerminalNode MINUS() {
return getToken(CypherParser.MINUS, 0);
}
public ArrowLineContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_arrowLine;
}
}
public final ArrowLineContext arrowLine() throws RecognitionException {
ArrowLineContext _localctx = new ArrowLineContext(_ctx, getState());
enterRule(_localctx, 126, RULE_arrowLine);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1228);
_la = _input.LA(1);
if (!(_la == MINUS || _la == ARROW_LINE)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RightArrowContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode GT() {
return getToken(CypherParser.GT, 0);
}
public TerminalNode ARROW_RIGHT_HEAD() {
return getToken(CypherParser.ARROW_RIGHT_HEAD, 0);
}
public RightArrowContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_rightArrow;
}
}
public final RightArrowContext rightArrow() throws RecognitionException {
RightArrowContext _localctx = new RightArrowContext(_ctx, getState());
enterRule(_localctx, 128, RULE_rightArrow);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1230);
_la = _input.LA(1);
if (!(_la == GT || _la == ARROW_RIGHT_HEAD)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PathLengthContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public Token from;
public Token to;
public Token single;
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public TerminalNode DOTDOT() {
return getToken(CypherParser.DOTDOT, 0);
}
public List UNSIGNED_DECIMAL_INTEGER() {
return getTokens(CypherParser.UNSIGNED_DECIMAL_INTEGER);
}
public TerminalNode UNSIGNED_DECIMAL_INTEGER(int i) {
return getToken(CypherParser.UNSIGNED_DECIMAL_INTEGER, i);
}
public PathLengthContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_pathLength;
}
}
public final PathLengthContext pathLength() throws RecognitionException {
PathLengthContext _localctx = new PathLengthContext(_ctx, getState());
enterRule(_localctx, 130, RULE_pathLength);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1232);
match(TIMES);
setState(1241);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 106, _ctx)) {
case 1:
{
setState(1234);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == UNSIGNED_DECIMAL_INTEGER) {
{
setState(1233);
((PathLengthContext) _localctx).from = match(UNSIGNED_DECIMAL_INTEGER);
}
}
setState(1236);
match(DOTDOT);
setState(1238);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == UNSIGNED_DECIMAL_INTEGER) {
{
setState(1237);
((PathLengthContext) _localctx).to = match(UNSIGNED_DECIMAL_INTEGER);
}
}
}
break;
case 2:
{
setState(1240);
((PathLengthContext) _localctx).single = match(UNSIGNED_DECIMAL_INTEGER);
}
break;
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COLON() {
return getToken(CypherParser.COLON, 0);
}
public LabelExpression4Context labelExpression4() {
return getRuleContext(LabelExpression4Context.class, 0);
}
public TerminalNode IS() {
return getToken(CypherParser.IS, 0);
}
public LabelExpression4IsContext labelExpression4Is() {
return getRuleContext(LabelExpression4IsContext.class, 0);
}
public LabelExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_labelExpression;
}
}
public final LabelExpressionContext labelExpression() throws RecognitionException {
LabelExpressionContext _localctx = new LabelExpressionContext(_ctx, getState());
enterRule(_localctx, 132, RULE_labelExpression);
try {
setState(1247);
_errHandler.sync(this);
switch (_input.LA(1)) {
case COLON:
enterOuterAlt(_localctx, 1);
{
setState(1243);
match(COLON);
setState(1244);
labelExpression4();
}
break;
case IS:
enterOuterAlt(_localctx, 2);
{
setState(1245);
match(IS);
setState(1246);
labelExpression4Is();
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpression4Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List labelExpression3() {
return getRuleContexts(LabelExpression3Context.class);
}
public LabelExpression3Context labelExpression3(int i) {
return getRuleContext(LabelExpression3Context.class, i);
}
public List BAR() {
return getTokens(CypherParser.BAR);
}
public TerminalNode BAR(int i) {
return getToken(CypherParser.BAR, i);
}
public List COLON() {
return getTokens(CypherParser.COLON);
}
public TerminalNode COLON(int i) {
return getToken(CypherParser.COLON, i);
}
public LabelExpression4Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_labelExpression4;
}
}
public final LabelExpression4Context labelExpression4() throws RecognitionException {
LabelExpression4Context _localctx = new LabelExpression4Context(_ctx, getState());
enterRule(_localctx, 134, RULE_labelExpression4);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1249);
labelExpression3();
setState(1257);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 109, _ctx);
while (_alt != 2 && _alt != org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER) {
if (_alt == 1) {
{
{
setState(1250);
match(BAR);
setState(1252);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == COLON) {
{
setState(1251);
match(COLON);
}
}
setState(1254);
labelExpression3();
}
}
}
setState(1259);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 109, _ctx);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpression4IsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List labelExpression3Is() {
return getRuleContexts(LabelExpression3IsContext.class);
}
public LabelExpression3IsContext labelExpression3Is(int i) {
return getRuleContext(LabelExpression3IsContext.class, i);
}
public List BAR() {
return getTokens(CypherParser.BAR);
}
public TerminalNode BAR(int i) {
return getToken(CypherParser.BAR, i);
}
public List COLON() {
return getTokens(CypherParser.COLON);
}
public TerminalNode COLON(int i) {
return getToken(CypherParser.COLON, i);
}
public LabelExpression4IsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_labelExpression4Is;
}
}
public final LabelExpression4IsContext labelExpression4Is() throws RecognitionException {
LabelExpression4IsContext _localctx = new LabelExpression4IsContext(_ctx, getState());
enterRule(_localctx, 136, RULE_labelExpression4Is);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1260);
labelExpression3Is();
setState(1268);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 111, _ctx);
while (_alt != 2 && _alt != org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER) {
if (_alt == 1) {
{
{
setState(1261);
match(BAR);
setState(1263);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == COLON) {
{
setState(1262);
match(COLON);
}
}
setState(1265);
labelExpression3Is();
}
}
}
setState(1270);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 111, _ctx);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpression3Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List labelExpression2() {
return getRuleContexts(LabelExpression2Context.class);
}
public LabelExpression2Context labelExpression2(int i) {
return getRuleContext(LabelExpression2Context.class, i);
}
public List AMPERSAND() {
return getTokens(CypherParser.AMPERSAND);
}
public TerminalNode AMPERSAND(int i) {
return getToken(CypherParser.AMPERSAND, i);
}
public List COLON() {
return getTokens(CypherParser.COLON);
}
public TerminalNode COLON(int i) {
return getToken(CypherParser.COLON, i);
}
public LabelExpression3Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_labelExpression3;
}
}
public final LabelExpression3Context labelExpression3() throws RecognitionException {
LabelExpression3Context _localctx = new LabelExpression3Context(_ctx, getState());
enterRule(_localctx, 138, RULE_labelExpression3);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1271);
labelExpression2();
setState(1276);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 112, _ctx);
while (_alt != 2 && _alt != org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER) {
if (_alt == 1) {
{
{
setState(1272);
_la = _input.LA(1);
if (!(_la == COLON || _la == AMPERSAND)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1273);
labelExpression2();
}
}
}
setState(1278);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 112, _ctx);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpression3IsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List labelExpression2Is() {
return getRuleContexts(LabelExpression2IsContext.class);
}
public LabelExpression2IsContext labelExpression2Is(int i) {
return getRuleContext(LabelExpression2IsContext.class, i);
}
public List AMPERSAND() {
return getTokens(CypherParser.AMPERSAND);
}
public TerminalNode AMPERSAND(int i) {
return getToken(CypherParser.AMPERSAND, i);
}
public List COLON() {
return getTokens(CypherParser.COLON);
}
public TerminalNode COLON(int i) {
return getToken(CypherParser.COLON, i);
}
public LabelExpression3IsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_labelExpression3Is;
}
}
public final LabelExpression3IsContext labelExpression3Is() throws RecognitionException {
LabelExpression3IsContext _localctx = new LabelExpression3IsContext(_ctx, getState());
enterRule(_localctx, 140, RULE_labelExpression3Is);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1279);
labelExpression2Is();
setState(1284);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 113, _ctx);
while (_alt != 2 && _alt != org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER) {
if (_alt == 1) {
{
{
setState(1280);
_la = _input.LA(1);
if (!(_la == COLON || _la == AMPERSAND)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1281);
labelExpression2Is();
}
}
}
setState(1286);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 113, _ctx);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpression2Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public LabelExpression1Context labelExpression1() {
return getRuleContext(LabelExpression1Context.class, 0);
}
public List EXCLAMATION_MARK() {
return getTokens(CypherParser.EXCLAMATION_MARK);
}
public TerminalNode EXCLAMATION_MARK(int i) {
return getToken(CypherParser.EXCLAMATION_MARK, i);
}
public LabelExpression2Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_labelExpression2;
}
}
public final LabelExpression2Context labelExpression2() throws RecognitionException {
LabelExpression2Context _localctx = new LabelExpression2Context(_ctx, getState());
enterRule(_localctx, 142, RULE_labelExpression2);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1290);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == EXCLAMATION_MARK) {
{
{
setState(1287);
match(EXCLAMATION_MARK);
}
}
setState(1292);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1293);
labelExpression1();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpression2IsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public LabelExpression1IsContext labelExpression1Is() {
return getRuleContext(LabelExpression1IsContext.class, 0);
}
public List EXCLAMATION_MARK() {
return getTokens(CypherParser.EXCLAMATION_MARK);
}
public TerminalNode EXCLAMATION_MARK(int i) {
return getToken(CypherParser.EXCLAMATION_MARK, i);
}
public LabelExpression2IsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_labelExpression2Is;
}
}
public final LabelExpression2IsContext labelExpression2Is() throws RecognitionException {
LabelExpression2IsContext _localctx = new LabelExpression2IsContext(_ctx, getState());
enterRule(_localctx, 144, RULE_labelExpression2Is);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1298);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == EXCLAMATION_MARK) {
{
{
setState(1295);
match(EXCLAMATION_MARK);
}
}
setState(1300);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1301);
labelExpression1Is();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpression1Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public LabelExpression1Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_labelExpression1;
}
public LabelExpression1Context() {}
public void copyFrom(LabelExpression1Context ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class AnyLabelContext extends LabelExpression1Context {
public TerminalNode PERCENT() {
return getToken(CypherParser.PERCENT, 0);
}
public AnyLabelContext(LabelExpression1Context ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class LabelNameContext extends LabelExpression1Context {
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public LabelNameContext(LabelExpression1Context ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ParenthesizedLabelExpressionContext extends LabelExpression1Context {
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public LabelExpression4Context labelExpression4() {
return getRuleContext(LabelExpression4Context.class, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public ParenthesizedLabelExpressionContext(LabelExpression1Context ctx) {
copyFrom(ctx);
}
}
public final LabelExpression1Context labelExpression1() throws RecognitionException {
LabelExpression1Context _localctx = new LabelExpression1Context(_ctx, getState());
enterRule(_localctx, 146, RULE_labelExpression1);
try {
setState(1309);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LPAREN:
_localctx = new ParenthesizedLabelExpressionContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(1303);
match(LPAREN);
setState(1304);
labelExpression4();
setState(1305);
match(RPAREN);
}
break;
case PERCENT:
_localctx = new AnyLabelContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(1307);
match(PERCENT);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
_localctx = new LabelNameContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(1308);
symbolicNameString();
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelExpression1IsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public LabelExpression1IsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_labelExpression1Is;
}
public LabelExpression1IsContext() {}
public void copyFrom(LabelExpression1IsContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ParenthesizedLabelExpressionIsContext extends LabelExpression1IsContext {
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public LabelExpression4IsContext labelExpression4Is() {
return getRuleContext(LabelExpression4IsContext.class, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public ParenthesizedLabelExpressionIsContext(LabelExpression1IsContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class AnyLabelIsContext extends LabelExpression1IsContext {
public TerminalNode PERCENT() {
return getToken(CypherParser.PERCENT, 0);
}
public AnyLabelIsContext(LabelExpression1IsContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class LabelNameIsContext extends LabelExpression1IsContext {
public SymbolicLabelNameStringContext symbolicLabelNameString() {
return getRuleContext(SymbolicLabelNameStringContext.class, 0);
}
public LabelNameIsContext(LabelExpression1IsContext ctx) {
copyFrom(ctx);
}
}
public final LabelExpression1IsContext labelExpression1Is() throws RecognitionException {
LabelExpression1IsContext _localctx = new LabelExpression1IsContext(_ctx, getState());
enterRule(_localctx, 148, RULE_labelExpression1Is);
try {
setState(1317);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LPAREN:
_localctx = new ParenthesizedLabelExpressionIsContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(1311);
match(LPAREN);
setState(1312);
labelExpression4Is();
setState(1313);
match(RPAREN);
}
break;
case PERCENT:
_localctx = new AnyLabelIsContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(1315);
match(PERCENT);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NOTHING:
case NOWAIT:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
_localctx = new LabelNameIsContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(1316);
symbolicLabelNameString();
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InsertNodeLabelExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class, i);
}
public List COLON() {
return getTokens(CypherParser.COLON);
}
public TerminalNode COLON(int i) {
return getToken(CypherParser.COLON, i);
}
public TerminalNode IS() {
return getToken(CypherParser.IS, 0);
}
public List AMPERSAND() {
return getTokens(CypherParser.AMPERSAND);
}
public TerminalNode AMPERSAND(int i) {
return getToken(CypherParser.AMPERSAND, i);
}
public InsertNodeLabelExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_insertNodeLabelExpression;
}
}
public final InsertNodeLabelExpressionContext insertNodeLabelExpression() throws RecognitionException {
InsertNodeLabelExpressionContext _localctx = new InsertNodeLabelExpressionContext(_ctx, getState());
enterRule(_localctx, 150, RULE_insertNodeLabelExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1319);
_la = _input.LA(1);
if (!(_la == COLON || _la == IS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1320);
symbolicNameString();
setState(1325);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COLON || _la == AMPERSAND) {
{
{
setState(1321);
_la = _input.LA(1);
if (!(_la == COLON || _la == AMPERSAND)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1322);
symbolicNameString();
}
}
setState(1327);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InsertRelationshipLabelExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public TerminalNode COLON() {
return getToken(CypherParser.COLON, 0);
}
public TerminalNode IS() {
return getToken(CypherParser.IS, 0);
}
public InsertRelationshipLabelExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_insertRelationshipLabelExpression;
}
}
public final InsertRelationshipLabelExpressionContext insertRelationshipLabelExpression()
throws RecognitionException {
InsertRelationshipLabelExpressionContext _localctx =
new InsertRelationshipLabelExpressionContext(_ctx, getState());
enterRule(_localctx, 152, RULE_insertRelationshipLabelExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1328);
_la = _input.LA(1);
if (!(_la == COLON || _la == IS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1329);
symbolicNameString();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List expression11() {
return getRuleContexts(Expression11Context.class);
}
public Expression11Context expression11(int i) {
return getRuleContext(Expression11Context.class, i);
}
public List OR() {
return getTokens(CypherParser.OR);
}
public TerminalNode OR(int i) {
return getToken(CypherParser.OR, i);
}
public ExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_expression;
}
}
public final ExpressionContext expression() throws RecognitionException {
ExpressionContext _localctx = new ExpressionContext(_ctx, getState());
enterRule(_localctx, 154, RULE_expression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1331);
expression11();
setState(1336);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == OR) {
{
{
setState(1332);
match(OR);
setState(1333);
expression11();
}
}
setState(1338);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression11Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List expression10() {
return getRuleContexts(Expression10Context.class);
}
public Expression10Context expression10(int i) {
return getRuleContext(Expression10Context.class, i);
}
public List XOR() {
return getTokens(CypherParser.XOR);
}
public TerminalNode XOR(int i) {
return getToken(CypherParser.XOR, i);
}
public Expression11Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_expression11;
}
}
public final Expression11Context expression11() throws RecognitionException {
Expression11Context _localctx = new Expression11Context(_ctx, getState());
enterRule(_localctx, 156, RULE_expression11);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1339);
expression10();
setState(1344);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == XOR) {
{
{
setState(1340);
match(XOR);
setState(1341);
expression10();
}
}
setState(1346);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression10Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List expression9() {
return getRuleContexts(Expression9Context.class);
}
public Expression9Context expression9(int i) {
return getRuleContext(Expression9Context.class, i);
}
public List AND() {
return getTokens(CypherParser.AND);
}
public TerminalNode AND(int i) {
return getToken(CypherParser.AND, i);
}
public Expression10Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_expression10;
}
}
public final Expression10Context expression10() throws RecognitionException {
Expression10Context _localctx = new Expression10Context(_ctx, getState());
enterRule(_localctx, 158, RULE_expression10);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1347);
expression9();
setState(1352);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == AND) {
{
{
setState(1348);
match(AND);
setState(1349);
expression9();
}
}
setState(1354);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression9Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public Expression8Context expression8() {
return getRuleContext(Expression8Context.class, 0);
}
public List NOT() {
return getTokens(CypherParser.NOT);
}
public TerminalNode NOT(int i) {
return getToken(CypherParser.NOT, i);
}
public Expression9Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_expression9;
}
}
public final Expression9Context expression9() throws RecognitionException {
Expression9Context _localctx = new Expression9Context(_ctx, getState());
enterRule(_localctx, 160, RULE_expression9);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1358);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 122, _ctx);
while (_alt != 2 && _alt != org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER) {
if (_alt == 1) {
{
{
setState(1355);
match(NOT);
}
}
}
setState(1360);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 122, _ctx);
}
setState(1361);
expression8();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression8Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List expression7() {
return getRuleContexts(Expression7Context.class);
}
public Expression7Context expression7(int i) {
return getRuleContext(Expression7Context.class, i);
}
public List EQ() {
return getTokens(CypherParser.EQ);
}
public TerminalNode EQ(int i) {
return getToken(CypherParser.EQ, i);
}
public List INVALID_NEQ() {
return getTokens(CypherParser.INVALID_NEQ);
}
public TerminalNode INVALID_NEQ(int i) {
return getToken(CypherParser.INVALID_NEQ, i);
}
public List NEQ() {
return getTokens(CypherParser.NEQ);
}
public TerminalNode NEQ(int i) {
return getToken(CypherParser.NEQ, i);
}
public List LE() {
return getTokens(CypherParser.LE);
}
public TerminalNode LE(int i) {
return getToken(CypherParser.LE, i);
}
public List GE() {
return getTokens(CypherParser.GE);
}
public TerminalNode GE(int i) {
return getToken(CypherParser.GE, i);
}
public List LT() {
return getTokens(CypherParser.LT);
}
public TerminalNode LT(int i) {
return getToken(CypherParser.LT, i);
}
public List GT() {
return getTokens(CypherParser.GT);
}
public TerminalNode GT(int i) {
return getToken(CypherParser.GT, i);
}
public Expression8Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_expression8;
}
}
public final Expression8Context expression8() throws RecognitionException {
Expression8Context _localctx = new Expression8Context(_ctx, getState());
enterRule(_localctx, 162, RULE_expression8);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1363);
expression7();
setState(1368);
_errHandler.sync(this);
_la = _input.LA(1);
while (((((_la - 95)) & ~0x3f) == 0 && ((1L << (_la - 95)) & 1733903448728010753L) != 0)) {
{
{
setState(1364);
_la = _input.LA(1);
if (!(((((_la - 95)) & ~0x3f) == 0 && ((1L << (_la - 95)) & 1733903448728010753L) != 0))) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1365);
expression7();
}
}
setState(1370);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression7Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public Expression6Context expression6() {
return getRuleContext(Expression6Context.class, 0);
}
public ComparisonExpression6Context comparisonExpression6() {
return getRuleContext(ComparisonExpression6Context.class, 0);
}
public Expression7Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_expression7;
}
}
public final Expression7Context expression7() throws RecognitionException {
Expression7Context _localctx = new Expression7Context(_ctx, getState());
enterRule(_localctx, 164, RULE_expression7);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1371);
expression6();
setState(1373);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == COLONCOLON
|| _la == CONTAINS
|| ((((_la - 94)) & ~0x3f) == 0 && ((1L << (_la - 94)) & 69256347649L) != 0)
|| _la == REGEQ
|| _la == STARTS) {
{
setState(1372);
comparisonExpression6();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ComparisonExpression6Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ComparisonExpression6Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_comparisonExpression6;
}
public ComparisonExpression6Context() {}
public void copyFrom(ComparisonExpression6Context ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class TypeComparisonContext extends ComparisonExpression6Context {
public TypeContext type() {
return getRuleContext(TypeContext.class, 0);
}
public TerminalNode IS() {
return getToken(CypherParser.IS, 0);
}
public TerminalNode COLONCOLON() {
return getToken(CypherParser.COLONCOLON, 0);
}
public TerminalNode TYPED() {
return getToken(CypherParser.TYPED, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public TypeComparisonContext(ComparisonExpression6Context ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class StringAndListComparisonContext extends ComparisonExpression6Context {
public Expression6Context expression6() {
return getRuleContext(Expression6Context.class, 0);
}
public TerminalNode REGEQ() {
return getToken(CypherParser.REGEQ, 0);
}
public TerminalNode STARTS() {
return getToken(CypherParser.STARTS, 0);
}
public TerminalNode WITH() {
return getToken(CypherParser.WITH, 0);
}
public TerminalNode ENDS() {
return getToken(CypherParser.ENDS, 0);
}
public TerminalNode CONTAINS() {
return getToken(CypherParser.CONTAINS, 0);
}
public TerminalNode IN() {
return getToken(CypherParser.IN, 0);
}
public StringAndListComparisonContext(ComparisonExpression6Context ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class NormalFormComparisonContext extends ComparisonExpression6Context {
public TerminalNode IS() {
return getToken(CypherParser.IS, 0);
}
public TerminalNode NORMALIZED() {
return getToken(CypherParser.NORMALIZED, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public NormalFormContext normalForm() {
return getRuleContext(NormalFormContext.class, 0);
}
public NormalFormComparisonContext(ComparisonExpression6Context ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class NullComparisonContext extends ComparisonExpression6Context {
public TerminalNode IS() {
return getToken(CypherParser.IS, 0);
}
public TerminalNode NULL() {
return getToken(CypherParser.NULL, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public NullComparisonContext(ComparisonExpression6Context ctx) {
copyFrom(ctx);
}
}
public final ComparisonExpression6Context comparisonExpression6() throws RecognitionException {
ComparisonExpression6Context _localctx = new ComparisonExpression6Context(_ctx, getState());
enterRule(_localctx, 166, RULE_comparisonExpression6);
int _la;
try {
setState(1407);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 131, _ctx)) {
case 1:
_localctx = new StringAndListComparisonContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(1382);
_errHandler.sync(this);
switch (_input.LA(1)) {
case REGEQ:
{
setState(1375);
match(REGEQ);
}
break;
case STARTS:
{
setState(1376);
match(STARTS);
setState(1377);
match(WITH);
}
break;
case ENDS:
{
setState(1378);
match(ENDS);
setState(1379);
match(WITH);
}
break;
case CONTAINS:
{
setState(1380);
match(CONTAINS);
}
break;
case IN:
{
setState(1381);
match(IN);
}
break;
default:
throw new NoViableAltException(this);
}
setState(1384);
expression6();
}
break;
case 2:
_localctx = new NullComparisonContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(1385);
match(IS);
setState(1387);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == NOT) {
{
setState(1386);
match(NOT);
}
}
setState(1389);
match(NULL);
}
break;
case 3:
_localctx = new TypeComparisonContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(1396);
_errHandler.sync(this);
switch (_input.LA(1)) {
case IS:
{
setState(1390);
match(IS);
setState(1392);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == NOT) {
{
setState(1391);
match(NOT);
}
}
setState(1394);
_la = _input.LA(1);
if (!(_la == COLONCOLON || _la == TYPED)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
case COLONCOLON:
{
setState(1395);
match(COLONCOLON);
}
break;
default:
throw new NoViableAltException(this);
}
setState(1398);
type();
}
break;
case 4:
_localctx = new NormalFormComparisonContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(1399);
match(IS);
setState(1401);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == NOT) {
{
setState(1400);
match(NOT);
}
}
setState(1404);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 159)) & ~0x3f) == 0 && ((1L << (_la - 159)) & 15L) != 0)) {
{
setState(1403);
normalForm();
}
}
setState(1406);
match(NORMALIZED);
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NormalFormContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode NFC() {
return getToken(CypherParser.NFC, 0);
}
public TerminalNode NFD() {
return getToken(CypherParser.NFD, 0);
}
public TerminalNode NFKC() {
return getToken(CypherParser.NFKC, 0);
}
public TerminalNode NFKD() {
return getToken(CypherParser.NFKD, 0);
}
public NormalFormContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_normalForm;
}
}
public final NormalFormContext normalForm() throws RecognitionException {
NormalFormContext _localctx = new NormalFormContext(_ctx, getState());
enterRule(_localctx, 168, RULE_normalForm);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1409);
_la = _input.LA(1);
if (!(((((_la - 159)) & ~0x3f) == 0 && ((1L << (_la - 159)) & 15L) != 0))) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression6Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List expression5() {
return getRuleContexts(Expression5Context.class);
}
public Expression5Context expression5(int i) {
return getRuleContext(Expression5Context.class, i);
}
public List PLUS() {
return getTokens(CypherParser.PLUS);
}
public TerminalNode PLUS(int i) {
return getToken(CypherParser.PLUS, i);
}
public List MINUS() {
return getTokens(CypherParser.MINUS);
}
public TerminalNode MINUS(int i) {
return getToken(CypherParser.MINUS, i);
}
public List DOUBLEBAR() {
return getTokens(CypherParser.DOUBLEBAR);
}
public TerminalNode DOUBLEBAR(int i) {
return getToken(CypherParser.DOUBLEBAR, i);
}
public Expression6Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_expression6;
}
}
public final Expression6Context expression6() throws RecognitionException {
Expression6Context _localctx = new Expression6Context(_ctx, getState());
enterRule(_localctx, 170, RULE_expression6);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1411);
expression5();
setState(1416);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == DOUBLEBAR || _la == MINUS || _la == PLUS) {
{
{
setState(1412);
_la = _input.LA(1);
if (!(_la == DOUBLEBAR || _la == MINUS || _la == PLUS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1413);
expression5();
}
}
setState(1418);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression5Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List expression4() {
return getRuleContexts(Expression4Context.class);
}
public Expression4Context expression4(int i) {
return getRuleContext(Expression4Context.class, i);
}
public List TIMES() {
return getTokens(CypherParser.TIMES);
}
public TerminalNode TIMES(int i) {
return getToken(CypherParser.TIMES, i);
}
public List DIVIDE() {
return getTokens(CypherParser.DIVIDE);
}
public TerminalNode DIVIDE(int i) {
return getToken(CypherParser.DIVIDE, i);
}
public List PERCENT() {
return getTokens(CypherParser.PERCENT);
}
public TerminalNode PERCENT(int i) {
return getToken(CypherParser.PERCENT, i);
}
public Expression5Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_expression5;
}
}
public final Expression5Context expression5() throws RecognitionException {
Expression5Context _localctx = new Expression5Context(_ctx, getState());
enterRule(_localctx, 172, RULE_expression5);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1419);
expression4();
setState(1424);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == DIVIDE || _la == PERCENT || _la == TIMES) {
{
{
setState(1420);
_la = _input.LA(1);
if (!(_la == DIVIDE || _la == PERCENT || _la == TIMES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1421);
expression4();
}
}
setState(1426);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression4Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List expression3() {
return getRuleContexts(Expression3Context.class);
}
public Expression3Context expression3(int i) {
return getRuleContext(Expression3Context.class, i);
}
public List POW() {
return getTokens(CypherParser.POW);
}
public TerminalNode POW(int i) {
return getToken(CypherParser.POW, i);
}
public Expression4Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_expression4;
}
}
public final Expression4Context expression4() throws RecognitionException {
Expression4Context _localctx = new Expression4Context(_ctx, getState());
enterRule(_localctx, 174, RULE_expression4);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1427);
expression3();
setState(1432);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == POW) {
{
{
setState(1428);
match(POW);
setState(1429);
expression3();
}
}
setState(1434);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression3Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public Expression2Context expression2() {
return getRuleContext(Expression2Context.class, 0);
}
public TerminalNode PLUS() {
return getToken(CypherParser.PLUS, 0);
}
public TerminalNode MINUS() {
return getToken(CypherParser.MINUS, 0);
}
public Expression3Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_expression3;
}
}
public final Expression3Context expression3() throws RecognitionException {
Expression3Context _localctx = new Expression3Context(_ctx, getState());
enterRule(_localctx, 176, RULE_expression3);
int _la;
try {
setState(1438);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 135, _ctx)) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(1435);
expression2();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(1436);
_la = _input.LA(1);
if (!(_la == MINUS || _la == PLUS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1437);
expression2();
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression2Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public Expression1Context expression1() {
return getRuleContext(Expression1Context.class, 0);
}
public List postFix() {
return getRuleContexts(PostFixContext.class);
}
public PostFixContext postFix(int i) {
return getRuleContext(PostFixContext.class, i);
}
public Expression2Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_expression2;
}
}
public final Expression2Context expression2() throws RecognitionException {
Expression2Context _localctx = new Expression2Context(_ctx, getState());
enterRule(_localctx, 178, RULE_expression2);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1440);
expression1();
setState(1444);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 136, _ctx);
while (_alt != 2 && _alt != org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER) {
if (_alt == 1) {
{
{
setState(1441);
postFix();
}
}
}
setState(1446);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 136, _ctx);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PostFixContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public PostFixContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_postFix;
}
public PostFixContext() {}
public void copyFrom(PostFixContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class IndexPostfixContext extends PostFixContext {
public TerminalNode LBRACKET() {
return getToken(CypherParser.LBRACKET, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public TerminalNode RBRACKET() {
return getToken(CypherParser.RBRACKET, 0);
}
public IndexPostfixContext(PostFixContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class PropertyPostfixContext extends PostFixContext {
public PropertyContext property() {
return getRuleContext(PropertyContext.class, 0);
}
public PropertyPostfixContext(PostFixContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class LabelPostfixContext extends PostFixContext {
public LabelExpressionContext labelExpression() {
return getRuleContext(LabelExpressionContext.class, 0);
}
public LabelPostfixContext(PostFixContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class RangePostfixContext extends PostFixContext {
public ExpressionContext fromExp;
public ExpressionContext toExp;
public TerminalNode LBRACKET() {
return getToken(CypherParser.LBRACKET, 0);
}
public TerminalNode DOTDOT() {
return getToken(CypherParser.DOTDOT, 0);
}
public TerminalNode RBRACKET() {
return getToken(CypherParser.RBRACKET, 0);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class, i);
}
public RangePostfixContext(PostFixContext ctx) {
copyFrom(ctx);
}
}
public final PostFixContext postFix() throws RecognitionException {
PostFixContext _localctx = new PostFixContext(_ctx, getState());
enterRule(_localctx, 180, RULE_postFix);
int _la;
try {
setState(1462);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 139, _ctx)) {
case 1:
_localctx = new PropertyPostfixContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(1447);
property();
}
break;
case 2:
_localctx = new LabelPostfixContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(1448);
labelExpression();
}
break;
case 3:
_localctx = new IndexPostfixContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(1449);
match(LBRACKET);
setState(1450);
expression();
setState(1451);
match(RBRACKET);
}
break;
case 4:
_localctx = new RangePostfixContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(1453);
match(LBRACKET);
setState(1455);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -61572919590928L) != 0)
|| ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -9288676379058177L) != 0)
|| ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -2305843009449101697L) != 0)
|| ((((_la - 193)) & ~0x3f) == 0 && ((1L << (_la - 193)) & -576460823170418305L) != 0)
|| ((((_la - 257)) & ~0x3f) == 0 && ((1L << (_la - 257)) & 4294967295L) != 0)) {
{
setState(1454);
((RangePostfixContext) _localctx).fromExp = expression();
}
}
setState(1457);
match(DOTDOT);
setState(1459);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -61572919590928L) != 0)
|| ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -9288676379058177L) != 0)
|| ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -2305843009449101697L) != 0)
|| ((((_la - 193)) & ~0x3f) == 0 && ((1L << (_la - 193)) & -576460823170418305L) != 0)
|| ((((_la - 257)) & ~0x3f) == 0 && ((1L << (_la - 257)) & 4294967295L) != 0)) {
{
setState(1458);
((RangePostfixContext) _localctx).toExp = expression();
}
}
setState(1461);
match(RBRACKET);
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PropertyContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DOT() {
return getToken(CypherParser.DOT, 0);
}
public PropertyKeyNameContext propertyKeyName() {
return getRuleContext(PropertyKeyNameContext.class, 0);
}
public PropertyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_property;
}
}
public final PropertyContext property() throws RecognitionException {
PropertyContext _localctx = new PropertyContext(_ctx, getState());
enterRule(_localctx, 182, RULE_property);
try {
enterOuterAlt(_localctx, 1);
{
setState(1464);
match(DOT);
setState(1465);
propertyKeyName();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PropertyExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public Expression1Context expression1() {
return getRuleContext(Expression1Context.class, 0);
}
public List property() {
return getRuleContexts(PropertyContext.class);
}
public PropertyContext property(int i) {
return getRuleContext(PropertyContext.class, i);
}
public PropertyExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_propertyExpression;
}
}
public final PropertyExpressionContext propertyExpression() throws RecognitionException {
PropertyExpressionContext _localctx = new PropertyExpressionContext(_ctx, getState());
enterRule(_localctx, 184, RULE_propertyExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1467);
expression1();
setState(1469);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(1468);
property();
}
}
setState(1471);
_errHandler.sync(this);
_la = _input.LA(1);
} while (_la == DOT);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class Expression1Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public LiteralContext literal() {
return getRuleContext(LiteralContext.class, 0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class, 0);
}
public CaseExpressionContext caseExpression() {
return getRuleContext(CaseExpressionContext.class, 0);
}
public ExtendedCaseExpressionContext extendedCaseExpression() {
return getRuleContext(ExtendedCaseExpressionContext.class, 0);
}
public CountStarContext countStar() {
return getRuleContext(CountStarContext.class, 0);
}
public ExistsExpressionContext existsExpression() {
return getRuleContext(ExistsExpressionContext.class, 0);
}
public CountExpressionContext countExpression() {
return getRuleContext(CountExpressionContext.class, 0);
}
public CollectExpressionContext collectExpression() {
return getRuleContext(CollectExpressionContext.class, 0);
}
public MapProjectionContext mapProjection() {
return getRuleContext(MapProjectionContext.class, 0);
}
public ListComprehensionContext listComprehension() {
return getRuleContext(ListComprehensionContext.class, 0);
}
public ListLiteralContext listLiteral() {
return getRuleContext(ListLiteralContext.class, 0);
}
public PatternComprehensionContext patternComprehension() {
return getRuleContext(PatternComprehensionContext.class, 0);
}
public ReduceExpressionContext reduceExpression() {
return getRuleContext(ReduceExpressionContext.class, 0);
}
public ListItemsPredicateContext listItemsPredicate() {
return getRuleContext(ListItemsPredicateContext.class, 0);
}
public NormalizeFunctionContext normalizeFunction() {
return getRuleContext(NormalizeFunctionContext.class, 0);
}
public TrimFunctionContext trimFunction() {
return getRuleContext(TrimFunctionContext.class, 0);
}
public PatternExpressionContext patternExpression() {
return getRuleContext(PatternExpressionContext.class, 0);
}
public ShortestPathExpressionContext shortestPathExpression() {
return getRuleContext(ShortestPathExpressionContext.class, 0);
}
public ParenthesizedExpressionContext parenthesizedExpression() {
return getRuleContext(ParenthesizedExpressionContext.class, 0);
}
public FunctionInvocationContext functionInvocation() {
return getRuleContext(FunctionInvocationContext.class, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public Expression1Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_expression1;
}
}
public final Expression1Context expression1() throws RecognitionException {
Expression1Context _localctx = new Expression1Context(_ctx, getState());
enterRule(_localctx, 186, RULE_expression1);
try {
setState(1494);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 141, _ctx)) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(1473);
literal();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(1474);
parameter("ANY");
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(1475);
caseExpression();
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(1476);
extendedCaseExpression();
}
break;
case 5:
enterOuterAlt(_localctx, 5);
{
setState(1477);
countStar();
}
break;
case 6:
enterOuterAlt(_localctx, 6);
{
setState(1478);
existsExpression();
}
break;
case 7:
enterOuterAlt(_localctx, 7);
{
setState(1479);
countExpression();
}
break;
case 8:
enterOuterAlt(_localctx, 8);
{
setState(1480);
collectExpression();
}
break;
case 9:
enterOuterAlt(_localctx, 9);
{
setState(1481);
mapProjection();
}
break;
case 10:
enterOuterAlt(_localctx, 10);
{
setState(1482);
listComprehension();
}
break;
case 11:
enterOuterAlt(_localctx, 11);
{
setState(1483);
listLiteral();
}
break;
case 12:
enterOuterAlt(_localctx, 12);
{
setState(1484);
patternComprehension();
}
break;
case 13:
enterOuterAlt(_localctx, 13);
{
setState(1485);
reduceExpression();
}
break;
case 14:
enterOuterAlt(_localctx, 14);
{
setState(1486);
listItemsPredicate();
}
break;
case 15:
enterOuterAlt(_localctx, 15);
{
setState(1487);
normalizeFunction();
}
break;
case 16:
enterOuterAlt(_localctx, 16);
{
setState(1488);
trimFunction();
}
break;
case 17:
enterOuterAlt(_localctx, 17);
{
setState(1489);
patternExpression();
}
break;
case 18:
enterOuterAlt(_localctx, 18);
{
setState(1490);
shortestPathExpression();
}
break;
case 19:
enterOuterAlt(_localctx, 19);
{
setState(1491);
parenthesizedExpression();
}
break;
case 20:
enterOuterAlt(_localctx, 20);
{
setState(1492);
functionInvocation();
}
break;
case 21:
enterOuterAlt(_localctx, 21);
{
setState(1493);
variable();
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LiteralContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public LiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_literal;
}
public LiteralContext() {}
public void copyFrom(LiteralContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class NummericLiteralContext extends LiteralContext {
public NumberLiteralContext numberLiteral() {
return getRuleContext(NumberLiteralContext.class, 0);
}
public NummericLiteralContext(LiteralContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class BooleanLiteralContext extends LiteralContext {
public TerminalNode TRUE() {
return getToken(CypherParser.TRUE, 0);
}
public TerminalNode FALSE() {
return getToken(CypherParser.FALSE, 0);
}
public BooleanLiteralContext(LiteralContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class KeywordLiteralContext extends LiteralContext {
public TerminalNode INFINITY() {
return getToken(CypherParser.INFINITY, 0);
}
public TerminalNode NAN() {
return getToken(CypherParser.NAN, 0);
}
public TerminalNode NULL() {
return getToken(CypherParser.NULL, 0);
}
public KeywordLiteralContext(LiteralContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class OtherLiteralContext extends LiteralContext {
public MapContext map() {
return getRuleContext(MapContext.class, 0);
}
public OtherLiteralContext(LiteralContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class StringsLiteralContext extends LiteralContext {
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class, 0);
}
public StringsLiteralContext(LiteralContext ctx) {
copyFrom(ctx);
}
}
public final LiteralContext literal() throws RecognitionException {
LiteralContext _localctx = new LiteralContext(_ctx, getState());
enterRule(_localctx, 188, RULE_literal);
try {
setState(1504);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DECIMAL_DOUBLE:
case UNSIGNED_DECIMAL_INTEGER:
case UNSIGNED_HEX_INTEGER:
case UNSIGNED_OCTAL_INTEGER:
case MINUS:
_localctx = new NummericLiteralContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(1496);
numberLiteral();
}
break;
case STRING_LITERAL1:
case STRING_LITERAL2:
_localctx = new StringsLiteralContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(1497);
stringLiteral();
}
break;
case LCURLY:
_localctx = new OtherLiteralContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(1498);
map();
}
break;
case TRUE:
_localctx = new BooleanLiteralContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(1499);
match(TRUE);
}
break;
case FALSE:
_localctx = new BooleanLiteralContext(_localctx);
enterOuterAlt(_localctx, 5);
{
setState(1500);
match(FALSE);
}
break;
case INFINITY:
_localctx = new KeywordLiteralContext(_localctx);
enterOuterAlt(_localctx, 6);
{
setState(1501);
match(INFINITY);
}
break;
case NAN:
_localctx = new KeywordLiteralContext(_localctx);
enterOuterAlt(_localctx, 7);
{
setState(1502);
match(NAN);
}
break;
case NULL:
_localctx = new KeywordLiteralContext(_localctx);
enterOuterAlt(_localctx, 8);
{
setState(1503);
match(NULL);
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CaseExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CASE() {
return getToken(CypherParser.CASE, 0);
}
public TerminalNode END() {
return getToken(CypherParser.END, 0);
}
public List caseAlternative() {
return getRuleContexts(CaseAlternativeContext.class);
}
public CaseAlternativeContext caseAlternative(int i) {
return getRuleContext(CaseAlternativeContext.class, i);
}
public TerminalNode ELSE() {
return getToken(CypherParser.ELSE, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public CaseExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_caseExpression;
}
}
public final CaseExpressionContext caseExpression() throws RecognitionException {
CaseExpressionContext _localctx = new CaseExpressionContext(_ctx, getState());
enterRule(_localctx, 190, RULE_caseExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1506);
match(CASE);
setState(1508);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(1507);
caseAlternative();
}
}
setState(1510);
_errHandler.sync(this);
_la = _input.LA(1);
} while (_la == WHEN);
setState(1514);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == ELSE) {
{
setState(1512);
match(ELSE);
setState(1513);
expression();
}
}
setState(1516);
match(END);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CaseAlternativeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode WHEN() {
return getToken(CypherParser.WHEN, 0);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class, i);
}
public TerminalNode THEN() {
return getToken(CypherParser.THEN, 0);
}
public CaseAlternativeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_caseAlternative;
}
}
public final CaseAlternativeContext caseAlternative() throws RecognitionException {
CaseAlternativeContext _localctx = new CaseAlternativeContext(_ctx, getState());
enterRule(_localctx, 192, RULE_caseAlternative);
try {
enterOuterAlt(_localctx, 1);
{
setState(1518);
match(WHEN);
setState(1519);
expression();
setState(1520);
match(THEN);
setState(1521);
expression();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExtendedCaseExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext elseExp;
public TerminalNode CASE() {
return getToken(CypherParser.CASE, 0);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class, i);
}
public TerminalNode END() {
return getToken(CypherParser.END, 0);
}
public List extendedCaseAlternative() {
return getRuleContexts(ExtendedCaseAlternativeContext.class);
}
public ExtendedCaseAlternativeContext extendedCaseAlternative(int i) {
return getRuleContext(ExtendedCaseAlternativeContext.class, i);
}
public TerminalNode ELSE() {
return getToken(CypherParser.ELSE, 0);
}
public ExtendedCaseExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_extendedCaseExpression;
}
}
public final ExtendedCaseExpressionContext extendedCaseExpression() throws RecognitionException {
ExtendedCaseExpressionContext _localctx = new ExtendedCaseExpressionContext(_ctx, getState());
enterRule(_localctx, 194, RULE_extendedCaseExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1523);
match(CASE);
setState(1524);
expression();
setState(1526);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(1525);
extendedCaseAlternative();
}
}
setState(1528);
_errHandler.sync(this);
_la = _input.LA(1);
} while (_la == WHEN);
setState(1532);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == ELSE) {
{
setState(1530);
match(ELSE);
setState(1531);
((ExtendedCaseExpressionContext) _localctx).elseExp = expression();
}
}
setState(1534);
match(END);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExtendedCaseAlternativeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode WHEN() {
return getToken(CypherParser.WHEN, 0);
}
public List extendedWhen() {
return getRuleContexts(ExtendedWhenContext.class);
}
public ExtendedWhenContext extendedWhen(int i) {
return getRuleContext(ExtendedWhenContext.class, i);
}
public TerminalNode THEN() {
return getToken(CypherParser.THEN, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public ExtendedCaseAlternativeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_extendedCaseAlternative;
}
}
public final ExtendedCaseAlternativeContext extendedCaseAlternative() throws RecognitionException {
ExtendedCaseAlternativeContext _localctx = new ExtendedCaseAlternativeContext(_ctx, getState());
enterRule(_localctx, 196, RULE_extendedCaseAlternative);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1536);
match(WHEN);
setState(1537);
extendedWhen();
setState(1542);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(1538);
match(COMMA);
setState(1539);
extendedWhen();
}
}
setState(1544);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(1545);
match(THEN);
setState(1546);
expression();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExtendedWhenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExtendedWhenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_extendedWhen;
}
public ExtendedWhenContext() {}
public void copyFrom(ExtendedWhenContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class WhenStringOrListContext extends ExtendedWhenContext {
public Expression6Context expression6() {
return getRuleContext(Expression6Context.class, 0);
}
public TerminalNode REGEQ() {
return getToken(CypherParser.REGEQ, 0);
}
public TerminalNode STARTS() {
return getToken(CypherParser.STARTS, 0);
}
public TerminalNode WITH() {
return getToken(CypherParser.WITH, 0);
}
public TerminalNode ENDS() {
return getToken(CypherParser.ENDS, 0);
}
public WhenStringOrListContext(ExtendedWhenContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class WhenTypeContext extends ExtendedWhenContext {
public TypeContext type() {
return getRuleContext(TypeContext.class, 0);
}
public TerminalNode IS() {
return getToken(CypherParser.IS, 0);
}
public TerminalNode TYPED() {
return getToken(CypherParser.TYPED, 0);
}
public TerminalNode COLONCOLON() {
return getToken(CypherParser.COLONCOLON, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public WhenTypeContext(ExtendedWhenContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class WhenFormContext extends ExtendedWhenContext {
public TerminalNode IS() {
return getToken(CypherParser.IS, 0);
}
public TerminalNode NORMALIZED() {
return getToken(CypherParser.NORMALIZED, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public NormalFormContext normalForm() {
return getRuleContext(NormalFormContext.class, 0);
}
public WhenFormContext(ExtendedWhenContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class WhenNullContext extends ExtendedWhenContext {
public TerminalNode IS() {
return getToken(CypherParser.IS, 0);
}
public TerminalNode NULL() {
return getToken(CypherParser.NULL, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public WhenNullContext(ExtendedWhenContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class WhenEqualsContext extends ExtendedWhenContext {
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public WhenEqualsContext(ExtendedWhenContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class WhenComparatorContext extends ExtendedWhenContext {
public Expression7Context expression7() {
return getRuleContext(Expression7Context.class, 0);
}
public TerminalNode EQ() {
return getToken(CypherParser.EQ, 0);
}
public TerminalNode NEQ() {
return getToken(CypherParser.NEQ, 0);
}
public TerminalNode INVALID_NEQ() {
return getToken(CypherParser.INVALID_NEQ, 0);
}
public TerminalNode LE() {
return getToken(CypherParser.LE, 0);
}
public TerminalNode GE() {
return getToken(CypherParser.GE, 0);
}
public TerminalNode LT() {
return getToken(CypherParser.LT, 0);
}
public TerminalNode GT() {
return getToken(CypherParser.GT, 0);
}
public WhenComparatorContext(ExtendedWhenContext ctx) {
copyFrom(ctx);
}
}
public final ExtendedWhenContext extendedWhen() throws RecognitionException {
ExtendedWhenContext _localctx = new ExtendedWhenContext(_ctx, getState());
enterRule(_localctx, 198, RULE_extendedWhen);
int _la;
try {
setState(1581);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 154, _ctx)) {
case 1:
_localctx = new WhenStringOrListContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(1553);
_errHandler.sync(this);
switch (_input.LA(1)) {
case REGEQ:
{
setState(1548);
match(REGEQ);
}
break;
case STARTS:
{
setState(1549);
match(STARTS);
setState(1550);
match(WITH);
}
break;
case ENDS:
{
setState(1551);
match(ENDS);
setState(1552);
match(WITH);
}
break;
default:
throw new NoViableAltException(this);
}
setState(1555);
expression6();
}
break;
case 2:
_localctx = new WhenNullContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(1556);
match(IS);
setState(1558);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == NOT) {
{
setState(1557);
match(NOT);
}
}
setState(1560);
match(NULL);
}
break;
case 3:
_localctx = new WhenTypeContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(1567);
_errHandler.sync(this);
switch (_input.LA(1)) {
case IS:
{
setState(1561);
match(IS);
setState(1563);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == NOT) {
{
setState(1562);
match(NOT);
}
}
setState(1565);
match(TYPED);
}
break;
case COLONCOLON:
{
setState(1566);
match(COLONCOLON);
}
break;
default:
throw new NoViableAltException(this);
}
setState(1569);
type();
}
break;
case 4:
_localctx = new WhenFormContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(1570);
match(IS);
setState(1572);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == NOT) {
{
setState(1571);
match(NOT);
}
}
setState(1575);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 159)) & ~0x3f) == 0 && ((1L << (_la - 159)) & 15L) != 0)) {
{
setState(1574);
normalForm();
}
}
setState(1577);
match(NORMALIZED);
}
break;
case 5:
_localctx = new WhenComparatorContext(_localctx);
enterOuterAlt(_localctx, 5);
{
setState(1578);
_la = _input.LA(1);
if (!(((((_la - 95)) & ~0x3f) == 0 && ((1L << (_la - 95)) & 1733903448728010753L) != 0))) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1579);
expression7();
}
break;
case 6:
_localctx = new WhenEqualsContext(_localctx);
enterOuterAlt(_localctx, 6);
{
setState(1580);
expression();
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ListComprehensionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext whereExp;
public ExpressionContext barExp;
public TerminalNode LBRACKET() {
return getToken(CypherParser.LBRACKET, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public TerminalNode IN() {
return getToken(CypherParser.IN, 0);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class, i);
}
public TerminalNode RBRACKET() {
return getToken(CypherParser.RBRACKET, 0);
}
public TerminalNode BAR() {
return getToken(CypherParser.BAR, 0);
}
public TerminalNode WHERE() {
return getToken(CypherParser.WHERE, 0);
}
public ListComprehensionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_listComprehension;
}
}
public final ListComprehensionContext listComprehension() throws RecognitionException {
ListComprehensionContext _localctx = new ListComprehensionContext(_ctx, getState());
enterRule(_localctx, 200, RULE_listComprehension);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1583);
match(LBRACKET);
setState(1584);
variable();
setState(1585);
match(IN);
setState(1586);
expression();
setState(1597);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 157, _ctx)) {
case 1:
{
setState(1589);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE) {
{
setState(1587);
match(WHERE);
setState(1588);
((ListComprehensionContext) _localctx).whereExp = expression();
}
}
setState(1591);
match(BAR);
setState(1592);
((ListComprehensionContext) _localctx).barExp = expression();
}
break;
case 2:
{
setState(1595);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE) {
{
setState(1593);
match(WHERE);
setState(1594);
((ListComprehensionContext) _localctx).whereExp = expression();
}
}
}
break;
}
setState(1599);
match(RBRACKET);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PatternComprehensionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext whereExp;
public ExpressionContext barExp;
public TerminalNode LBRACKET() {
return getToken(CypherParser.LBRACKET, 0);
}
public PathPatternNonEmptyContext pathPatternNonEmpty() {
return getRuleContext(PathPatternNonEmptyContext.class, 0);
}
public TerminalNode BAR() {
return getToken(CypherParser.BAR, 0);
}
public TerminalNode RBRACKET() {
return getToken(CypherParser.RBRACKET, 0);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class, i);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public TerminalNode EQ() {
return getToken(CypherParser.EQ, 0);
}
public TerminalNode WHERE() {
return getToken(CypherParser.WHERE, 0);
}
public PatternComprehensionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_patternComprehension;
}
}
public final PatternComprehensionContext patternComprehension() throws RecognitionException {
PatternComprehensionContext _localctx = new PatternComprehensionContext(_ctx, getState());
enterRule(_localctx, 202, RULE_patternComprehension);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1601);
match(LBRACKET);
setState(1605);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -61572919591936L) != 0)
|| ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -9288676379060225L) != 0)
|| ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -3458764514072989569L) != 0)
|| ((((_la - 193)) & ~0x3f) == 0 && ((1L << (_la - 193)) & -576460823170418305L) != 0)
|| ((((_la - 257)) & ~0x3f) == 0 && ((1L << (_la - 257)) & 4294967295L) != 0)) {
{
setState(1602);
variable();
setState(1603);
match(EQ);
}
}
setState(1607);
pathPatternNonEmpty();
setState(1610);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE) {
{
setState(1608);
match(WHERE);
setState(1609);
((PatternComprehensionContext) _localctx).whereExp = expression();
}
}
setState(1612);
match(BAR);
setState(1613);
((PatternComprehensionContext) _localctx).barExp = expression();
setState(1614);
match(RBRACKET);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ReduceExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode REDUCE() {
return getToken(CypherParser.REDUCE, 0);
}
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public List variable() {
return getRuleContexts(VariableContext.class);
}
public VariableContext variable(int i) {
return getRuleContext(VariableContext.class, i);
}
public TerminalNode EQ() {
return getToken(CypherParser.EQ, 0);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class, i);
}
public TerminalNode COMMA() {
return getToken(CypherParser.COMMA, 0);
}
public TerminalNode IN() {
return getToken(CypherParser.IN, 0);
}
public TerminalNode BAR() {
return getToken(CypherParser.BAR, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public ReduceExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_reduceExpression;
}
}
public final ReduceExpressionContext reduceExpression() throws RecognitionException {
ReduceExpressionContext _localctx = new ReduceExpressionContext(_ctx, getState());
enterRule(_localctx, 204, RULE_reduceExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(1616);
match(REDUCE);
setState(1617);
match(LPAREN);
setState(1618);
variable();
setState(1619);
match(EQ);
setState(1620);
expression();
setState(1621);
match(COMMA);
setState(1622);
variable();
setState(1623);
match(IN);
setState(1624);
expression();
setState(1625);
match(BAR);
setState(1626);
expression();
setState(1627);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ListItemsPredicateContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext inExp;
public ExpressionContext whereExp;
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public TerminalNode IN() {
return getToken(CypherParser.IN, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public TerminalNode ALL() {
return getToken(CypherParser.ALL, 0);
}
public TerminalNode ANY() {
return getToken(CypherParser.ANY, 0);
}
public TerminalNode NONE() {
return getToken(CypherParser.NONE, 0);
}
public TerminalNode SINGLE() {
return getToken(CypherParser.SINGLE, 0);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class, i);
}
public TerminalNode WHERE() {
return getToken(CypherParser.WHERE, 0);
}
public ListItemsPredicateContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_listItemsPredicate;
}
}
public final ListItemsPredicateContext listItemsPredicate() throws RecognitionException {
ListItemsPredicateContext _localctx = new ListItemsPredicateContext(_ctx, getState());
enterRule(_localctx, 206, RULE_listItemsPredicate);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1629);
_la = _input.LA(1);
if (!(_la == ALL || _la == ANY || _la == NONE || _la == SINGLE)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1630);
match(LPAREN);
setState(1631);
variable();
setState(1632);
match(IN);
setState(1633);
((ListItemsPredicateContext) _localctx).inExp = expression();
setState(1636);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE) {
{
setState(1634);
match(WHERE);
setState(1635);
((ListItemsPredicateContext) _localctx).whereExp = expression();
}
}
setState(1638);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NormalizeFunctionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode NORMALIZE() {
return getToken(CypherParser.NORMALIZE, 0);
}
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public TerminalNode COMMA() {
return getToken(CypherParser.COMMA, 0);
}
public NormalFormContext normalForm() {
return getRuleContext(NormalFormContext.class, 0);
}
public NormalizeFunctionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_normalizeFunction;
}
}
public final NormalizeFunctionContext normalizeFunction() throws RecognitionException {
NormalizeFunctionContext _localctx = new NormalizeFunctionContext(_ctx, getState());
enterRule(_localctx, 208, RULE_normalizeFunction);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1640);
match(NORMALIZE);
setState(1641);
match(LPAREN);
setState(1642);
expression();
setState(1645);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == COMMA) {
{
setState(1643);
match(COMMA);
setState(1644);
normalForm();
}
}
setState(1647);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TrimFunctionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext trimCharacterString;
public ExpressionContext trimSource;
public TerminalNode TRIM() {
return getToken(CypherParser.TRIM, 0);
}
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class, i);
}
public TerminalNode FROM() {
return getToken(CypherParser.FROM, 0);
}
public TerminalNode BOTH() {
return getToken(CypherParser.BOTH, 0);
}
public TerminalNode LEADING() {
return getToken(CypherParser.LEADING, 0);
}
public TerminalNode TRAILING() {
return getToken(CypherParser.TRAILING, 0);
}
public TrimFunctionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_trimFunction;
}
}
public final TrimFunctionContext trimFunction() throws RecognitionException {
TrimFunctionContext _localctx = new TrimFunctionContext(_ctx, getState());
enterRule(_localctx, 210, RULE_trimFunction);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1649);
match(TRIM);
setState(1650);
match(LPAREN);
setState(1658);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 164, _ctx)) {
case 1:
{
setState(1652);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 162, _ctx)) {
case 1:
{
setState(1651);
_la = _input.LA(1);
if (!(_la == BOTH || _la == LEADING || _la == TRAILING)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
}
setState(1655);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 163, _ctx)) {
case 1:
{
setState(1654);
((TrimFunctionContext) _localctx).trimCharacterString = expression();
}
break;
}
setState(1657);
match(FROM);
}
break;
}
setState(1660);
((TrimFunctionContext) _localctx).trimSource = expression();
setState(1661);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PatternExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public PathPatternNonEmptyContext pathPatternNonEmpty() {
return getRuleContext(PathPatternNonEmptyContext.class, 0);
}
public PatternExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_patternExpression;
}
}
public final PatternExpressionContext patternExpression() throws RecognitionException {
PatternExpressionContext _localctx = new PatternExpressionContext(_ctx, getState());
enterRule(_localctx, 212, RULE_patternExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(1663);
pathPatternNonEmpty();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShortestPathExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ShortestPathPatternContext shortestPathPattern() {
return getRuleContext(ShortestPathPatternContext.class, 0);
}
public ShortestPathExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_shortestPathExpression;
}
}
public final ShortestPathExpressionContext shortestPathExpression() throws RecognitionException {
ShortestPathExpressionContext _localctx = new ShortestPathExpressionContext(_ctx, getState());
enterRule(_localctx, 214, RULE_shortestPathExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(1665);
shortestPathPattern();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParenthesizedExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public ParenthesizedExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_parenthesizedExpression;
}
}
public final ParenthesizedExpressionContext parenthesizedExpression() throws RecognitionException {
ParenthesizedExpressionContext _localctx = new ParenthesizedExpressionContext(_ctx, getState());
enterRule(_localctx, 216, RULE_parenthesizedExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(1667);
match(LPAREN);
setState(1668);
expression();
setState(1669);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MapProjectionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public TerminalNode LCURLY() {
return getToken(CypherParser.LCURLY, 0);
}
public TerminalNode RCURLY() {
return getToken(CypherParser.RCURLY, 0);
}
public List mapProjectionElement() {
return getRuleContexts(MapProjectionElementContext.class);
}
public MapProjectionElementContext mapProjectionElement(int i) {
return getRuleContext(MapProjectionElementContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public MapProjectionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_mapProjection;
}
}
public final MapProjectionContext mapProjection() throws RecognitionException {
MapProjectionContext _localctx = new MapProjectionContext(_ctx, getState());
enterRule(_localctx, 218, RULE_mapProjection);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1671);
variable();
setState(1672);
match(LCURLY);
setState(1681);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -61572919591936L) != 0)
|| ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -9288676379043841L) != 0)
|| ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -3458764514072989569L) != 0)
|| ((((_la - 193)) & ~0x3f) == 0 && ((1L << (_la - 193)) & -576460823170418305L) != 0)
|| ((((_la - 257)) & ~0x3f) == 0 && ((1L << (_la - 257)) & 4294967295L) != 0)) {
{
setState(1673);
mapProjectionElement();
setState(1678);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(1674);
match(COMMA);
setState(1675);
mapProjectionElement();
}
}
setState(1680);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(1683);
match(RCURLY);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MapProjectionElementContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public PropertyKeyNameContext propertyKeyName() {
return getRuleContext(PropertyKeyNameContext.class, 0);
}
public TerminalNode COLON() {
return getToken(CypherParser.COLON, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public PropertyContext property() {
return getRuleContext(PropertyContext.class, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public TerminalNode DOT() {
return getToken(CypherParser.DOT, 0);
}
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public MapProjectionElementContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_mapProjectionElement;
}
}
public final MapProjectionElementContext mapProjectionElement() throws RecognitionException {
MapProjectionElementContext _localctx = new MapProjectionElementContext(_ctx, getState());
enterRule(_localctx, 220, RULE_mapProjectionElement);
try {
setState(1693);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 167, _ctx)) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(1685);
propertyKeyName();
setState(1686);
match(COLON);
setState(1687);
expression();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(1689);
property();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(1690);
variable();
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(1691);
match(DOT);
setState(1692);
match(TIMES);
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CountStarContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COUNT() {
return getToken(CypherParser.COUNT, 0);
}
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public CountStarContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_countStar;
}
}
public final CountStarContext countStar() throws RecognitionException {
CountStarContext _localctx = new CountStarContext(_ctx, getState());
enterRule(_localctx, 222, RULE_countStar);
try {
enterOuterAlt(_localctx, 1);
{
setState(1695);
match(COUNT);
setState(1696);
match(LPAREN);
setState(1697);
match(TIMES);
setState(1698);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExistsExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public TerminalNode LCURLY() {
return getToken(CypherParser.LCURLY, 0);
}
public TerminalNode RCURLY() {
return getToken(CypherParser.RCURLY, 0);
}
public RegularQueryContext regularQuery() {
return getRuleContext(RegularQueryContext.class, 0);
}
public PatternListContext patternList() {
return getRuleContext(PatternListContext.class, 0);
}
public MatchModeContext matchMode() {
return getRuleContext(MatchModeContext.class, 0);
}
public WhereClauseContext whereClause() {
return getRuleContext(WhereClauseContext.class, 0);
}
public ExistsExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_existsExpression;
}
}
public final ExistsExpressionContext existsExpression() throws RecognitionException {
ExistsExpressionContext _localctx = new ExistsExpressionContext(_ctx, getState());
enterRule(_localctx, 224, RULE_existsExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1700);
match(EXISTS);
setState(1701);
match(LCURLY);
setState(1710);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 170, _ctx)) {
case 1:
{
setState(1702);
regularQuery();
}
break;
case 2:
{
setState(1704);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 168, _ctx)) {
case 1:
{
setState(1703);
matchMode();
}
break;
}
setState(1706);
patternList();
setState(1708);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE) {
{
setState(1707);
whereClause();
}
}
}
break;
}
setState(1712);
match(RCURLY);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CountExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COUNT() {
return getToken(CypherParser.COUNT, 0);
}
public TerminalNode LCURLY() {
return getToken(CypherParser.LCURLY, 0);
}
public TerminalNode RCURLY() {
return getToken(CypherParser.RCURLY, 0);
}
public RegularQueryContext regularQuery() {
return getRuleContext(RegularQueryContext.class, 0);
}
public PatternListContext patternList() {
return getRuleContext(PatternListContext.class, 0);
}
public MatchModeContext matchMode() {
return getRuleContext(MatchModeContext.class, 0);
}
public WhereClauseContext whereClause() {
return getRuleContext(WhereClauseContext.class, 0);
}
public CountExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_countExpression;
}
}
public final CountExpressionContext countExpression() throws RecognitionException {
CountExpressionContext _localctx = new CountExpressionContext(_ctx, getState());
enterRule(_localctx, 226, RULE_countExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1714);
match(COUNT);
setState(1715);
match(LCURLY);
setState(1724);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 173, _ctx)) {
case 1:
{
setState(1716);
regularQuery();
}
break;
case 2:
{
setState(1718);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 171, _ctx)) {
case 1:
{
setState(1717);
matchMode();
}
break;
}
setState(1720);
patternList();
setState(1722);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE) {
{
setState(1721);
whereClause();
}
}
}
break;
}
setState(1726);
match(RCURLY);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CollectExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COLLECT() {
return getToken(CypherParser.COLLECT, 0);
}
public TerminalNode LCURLY() {
return getToken(CypherParser.LCURLY, 0);
}
public RegularQueryContext regularQuery() {
return getRuleContext(RegularQueryContext.class, 0);
}
public TerminalNode RCURLY() {
return getToken(CypherParser.RCURLY, 0);
}
public CollectExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_collectExpression;
}
}
public final CollectExpressionContext collectExpression() throws RecognitionException {
CollectExpressionContext _localctx = new CollectExpressionContext(_ctx, getState());
enterRule(_localctx, 228, RULE_collectExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(1728);
match(COLLECT);
setState(1729);
match(LCURLY);
setState(1730);
regularQuery();
setState(1731);
match(RCURLY);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NumberLiteralContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DECIMAL_DOUBLE() {
return getToken(CypherParser.DECIMAL_DOUBLE, 0);
}
public TerminalNode UNSIGNED_DECIMAL_INTEGER() {
return getToken(CypherParser.UNSIGNED_DECIMAL_INTEGER, 0);
}
public TerminalNode UNSIGNED_HEX_INTEGER() {
return getToken(CypherParser.UNSIGNED_HEX_INTEGER, 0);
}
public TerminalNode UNSIGNED_OCTAL_INTEGER() {
return getToken(CypherParser.UNSIGNED_OCTAL_INTEGER, 0);
}
public TerminalNode MINUS() {
return getToken(CypherParser.MINUS, 0);
}
public NumberLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_numberLiteral;
}
}
public final NumberLiteralContext numberLiteral() throws RecognitionException {
NumberLiteralContext _localctx = new NumberLiteralContext(_ctx, getState());
enterRule(_localctx, 230, RULE_numberLiteral);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1734);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == MINUS) {
{
setState(1733);
match(MINUS);
}
}
setState(1736);
_la = _input.LA(1);
if (!((((_la) & ~0x3f) == 0 && ((1L << _la) & 240L) != 0))) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SignedIntegerLiteralContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode UNSIGNED_DECIMAL_INTEGER() {
return getToken(CypherParser.UNSIGNED_DECIMAL_INTEGER, 0);
}
public TerminalNode MINUS() {
return getToken(CypherParser.MINUS, 0);
}
public SignedIntegerLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_signedIntegerLiteral;
}
}
public final SignedIntegerLiteralContext signedIntegerLiteral() throws RecognitionException {
SignedIntegerLiteralContext _localctx = new SignedIntegerLiteralContext(_ctx, getState());
enterRule(_localctx, 232, RULE_signedIntegerLiteral);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1739);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == MINUS) {
{
setState(1738);
match(MINUS);
}
}
setState(1741);
match(UNSIGNED_DECIMAL_INTEGER);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ListLiteralContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LBRACKET() {
return getToken(CypherParser.LBRACKET, 0);
}
public TerminalNode RBRACKET() {
return getToken(CypherParser.RBRACKET, 0);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public ListLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_listLiteral;
}
}
public final ListLiteralContext listLiteral() throws RecognitionException {
ListLiteralContext _localctx = new ListLiteralContext(_ctx, getState());
enterRule(_localctx, 234, RULE_listLiteral);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1743);
match(LBRACKET);
setState(1752);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -61572919590928L) != 0)
|| ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -9288676379058177L) != 0)
|| ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -2305843009449101697L) != 0)
|| ((((_la - 193)) & ~0x3f) == 0 && ((1L << (_la - 193)) & -576460823170418305L) != 0)
|| ((((_la - 257)) & ~0x3f) == 0 && ((1L << (_la - 257)) & 4294967295L) != 0)) {
{
setState(1744);
expression();
setState(1749);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(1745);
match(COMMA);
setState(1746);
expression();
}
}
setState(1751);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(1754);
match(RBRACKET);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PropertyKeyNameContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public PropertyKeyNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_propertyKeyName;
}
}
public final PropertyKeyNameContext propertyKeyName() throws RecognitionException {
PropertyKeyNameContext _localctx = new PropertyKeyNameContext(_ctx, getState());
enterRule(_localctx, 236, RULE_propertyKeyName);
try {
enterOuterAlt(_localctx, 1);
{
setState(1756);
symbolicNameString();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParameterContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public String paramType;
public TerminalNode DOLLAR() {
return getToken(CypherParser.DOLLAR, 0);
}
public ParameterNameContext parameterName() {
return getRuleContext(ParameterNameContext.class, 0);
}
public ParameterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
public ParameterContext(ParserRuleContext parent, int invokingState, String paramType) {
super(parent, invokingState);
this.paramType = paramType;
}
@Override
public int getRuleIndex() {
return RULE_parameter;
}
}
public final ParameterContext parameter(String paramType) throws RecognitionException {
ParameterContext _localctx = new ParameterContext(_ctx, getState(), paramType);
enterRule(_localctx, 238, RULE_parameter);
try {
enterOuterAlt(_localctx, 1);
{
setState(1758);
match(DOLLAR);
setState(1759);
parameterName(paramType);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParameterNameContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public String paramType;
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public TerminalNode UNSIGNED_DECIMAL_INTEGER() {
return getToken(CypherParser.UNSIGNED_DECIMAL_INTEGER, 0);
}
public ParameterNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
public ParameterNameContext(ParserRuleContext parent, int invokingState, String paramType) {
super(parent, invokingState);
this.paramType = paramType;
}
@Override
public int getRuleIndex() {
return RULE_parameterName;
}
}
public final ParameterNameContext parameterName(String paramType) throws RecognitionException {
ParameterNameContext _localctx = new ParameterNameContext(_ctx, getState(), paramType);
enterRule(_localctx, 240, RULE_parameterName);
try {
enterOuterAlt(_localctx, 1);
{
setState(1763);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
{
setState(1761);
symbolicNameString();
}
break;
case UNSIGNED_DECIMAL_INTEGER:
{
setState(1762);
match(UNSIGNED_DECIMAL_INTEGER);
}
break;
default:
throw new NoViableAltException(this);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FunctionInvocationContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public FunctionNameContext functionName() {
return getRuleContext(FunctionNameContext.class, 0);
}
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public List functionArgument() {
return getRuleContexts(FunctionArgumentContext.class);
}
public FunctionArgumentContext functionArgument(int i) {
return getRuleContext(FunctionArgumentContext.class, i);
}
public TerminalNode DISTINCT() {
return getToken(CypherParser.DISTINCT, 0);
}
public TerminalNode ALL() {
return getToken(CypherParser.ALL, 0);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public FunctionInvocationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_functionInvocation;
}
}
public final FunctionInvocationContext functionInvocation() throws RecognitionException {
FunctionInvocationContext _localctx = new FunctionInvocationContext(_ctx, getState());
enterRule(_localctx, 242, RULE_functionInvocation);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1765);
functionName();
setState(1766);
match(LPAREN);
setState(1768);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 179, _ctx)) {
case 1:
{
setState(1767);
_la = _input.LA(1);
if (!(_la == ALL || _la == DISTINCT)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
}
setState(1778);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -61572919590928L) != 0)
|| ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -9288676379058177L) != 0)
|| ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -2305843009449101697L) != 0)
|| ((((_la - 193)) & ~0x3f) == 0 && ((1L << (_la - 193)) & -576460823170418305L) != 0)
|| ((((_la - 257)) & ~0x3f) == 0 && ((1L << (_la - 257)) & 4294967295L) != 0)) {
{
setState(1770);
functionArgument();
setState(1775);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(1771);
match(COMMA);
setState(1772);
functionArgument();
}
}
setState(1777);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(1780);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FunctionArgumentContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public FunctionArgumentContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_functionArgument;
}
}
public final FunctionArgumentContext functionArgument() throws RecognitionException {
FunctionArgumentContext _localctx = new FunctionArgumentContext(_ctx, getState());
enterRule(_localctx, 244, RULE_functionArgument);
try {
enterOuterAlt(_localctx, 1);
{
setState(1782);
expression();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FunctionNameContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public NamespaceContext namespace() {
return getRuleContext(NamespaceContext.class, 0);
}
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public FunctionNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_functionName;
}
}
public final FunctionNameContext functionName() throws RecognitionException {
FunctionNameContext _localctx = new FunctionNameContext(_ctx, getState());
enterRule(_localctx, 246, RULE_functionName);
try {
enterOuterAlt(_localctx, 1);
{
setState(1784);
namespace();
setState(1785);
symbolicNameString();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NamespaceContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class, i);
}
public List DOT() {
return getTokens(CypherParser.DOT);
}
public TerminalNode DOT(int i) {
return getToken(CypherParser.DOT, i);
}
public NamespaceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_namespace;
}
}
public final NamespaceContext namespace() throws RecognitionException {
NamespaceContext _localctx = new NamespaceContext(_ctx, getState());
enterRule(_localctx, 248, RULE_namespace);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(1792);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 182, _ctx);
while (_alt != 2 && _alt != org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER) {
if (_alt == 1) {
{
{
setState(1787);
symbolicNameString();
setState(1788);
match(DOT);
}
}
}
setState(1794);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 182, _ctx);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class VariableContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public VariableContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_variable;
}
}
public final VariableContext variable() throws RecognitionException {
VariableContext _localctx = new VariableContext(_ctx, getState());
enterRule(_localctx, 250, RULE_variable);
try {
enterOuterAlt(_localctx, 1);
{
setState(1795);
symbolicNameString();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NonEmptyNameListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public NonEmptyNameListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_nonEmptyNameList;
}
}
public final NonEmptyNameListContext nonEmptyNameList() throws RecognitionException {
NonEmptyNameListContext _localctx = new NonEmptyNameListContext(_ctx, getState());
enterRule(_localctx, 252, RULE_nonEmptyNameList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1797);
symbolicNameString();
setState(1802);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(1798);
match(COMMA);
setState(1799);
symbolicNameString();
}
}
setState(1804);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public CreateCommandContext createCommand() {
return getRuleContext(CreateCommandContext.class, 0);
}
public DropCommandContext dropCommand() {
return getRuleContext(DropCommandContext.class, 0);
}
public AlterCommandContext alterCommand() {
return getRuleContext(AlterCommandContext.class, 0);
}
public RenameCommandContext renameCommand() {
return getRuleContext(RenameCommandContext.class, 0);
}
public DenyCommandContext denyCommand() {
return getRuleContext(DenyCommandContext.class, 0);
}
public RevokeCommandContext revokeCommand() {
return getRuleContext(RevokeCommandContext.class, 0);
}
public GrantCommandContext grantCommand() {
return getRuleContext(GrantCommandContext.class, 0);
}
public StartDatabaseContext startDatabase() {
return getRuleContext(StartDatabaseContext.class, 0);
}
public StopDatabaseContext stopDatabase() {
return getRuleContext(StopDatabaseContext.class, 0);
}
public EnableServerCommandContext enableServerCommand() {
return getRuleContext(EnableServerCommandContext.class, 0);
}
public AllocationCommandContext allocationCommand() {
return getRuleContext(AllocationCommandContext.class, 0);
}
public ShowCommandContext showCommand() {
return getRuleContext(ShowCommandContext.class, 0);
}
public TerminateCommandContext terminateCommand() {
return getRuleContext(TerminateCommandContext.class, 0);
}
public UseClauseContext useClause() {
return getRuleContext(UseClauseContext.class, 0);
}
public CommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_command;
}
}
public final CommandContext command() throws RecognitionException {
CommandContext _localctx = new CommandContext(_ctx, getState());
enterRule(_localctx, 254, RULE_command);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1806);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == USE) {
{
setState(1805);
useClause();
}
}
setState(1821);
_errHandler.sync(this);
switch (_input.LA(1)) {
case CREATE:
{
setState(1808);
createCommand();
}
break;
case DROP:
{
setState(1809);
dropCommand();
}
break;
case ALTER:
{
setState(1810);
alterCommand();
}
break;
case RENAME:
{
setState(1811);
renameCommand();
}
break;
case DENY:
{
setState(1812);
denyCommand();
}
break;
case REVOKE:
{
setState(1813);
revokeCommand();
}
break;
case GRANT:
{
setState(1814);
grantCommand();
}
break;
case START:
{
setState(1815);
startDatabase();
}
break;
case STOP:
{
setState(1816);
stopDatabase();
}
break;
case ENABLE:
{
setState(1817);
enableServerCommand();
}
break;
case DEALLOCATE:
case DRYRUN:
case REALLOCATE:
{
setState(1818);
allocationCommand();
}
break;
case SHOW:
{
setState(1819);
showCommand();
}
break;
case TERMINATE:
{
setState(1820);
terminateCommand();
}
break;
default:
throw new NoViableAltException(this);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CREATE() {
return getToken(CypherParser.CREATE, 0);
}
public CreateAliasContext createAlias() {
return getRuleContext(CreateAliasContext.class, 0);
}
public CreateCompositeDatabaseContext createCompositeDatabase() {
return getRuleContext(CreateCompositeDatabaseContext.class, 0);
}
public CreateConstraintContext createConstraint() {
return getRuleContext(CreateConstraintContext.class, 0);
}
public CreateDatabaseContext createDatabase() {
return getRuleContext(CreateDatabaseContext.class, 0);
}
public CreateIndexContext createIndex() {
return getRuleContext(CreateIndexContext.class, 0);
}
public CreateRoleContext createRole() {
return getRuleContext(CreateRoleContext.class, 0);
}
public CreateUserContext createUser() {
return getRuleContext(CreateUserContext.class, 0);
}
public TerminalNode OR() {
return getToken(CypherParser.OR, 0);
}
public TerminalNode REPLACE() {
return getToken(CypherParser.REPLACE, 0);
}
public CreateCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createCommand;
}
}
public final CreateCommandContext createCommand() throws RecognitionException {
CreateCommandContext _localctx = new CreateCommandContext(_ctx, getState());
enterRule(_localctx, 256, RULE_createCommand);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1823);
match(CREATE);
setState(1826);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == OR) {
{
setState(1824);
match(OR);
setState(1825);
match(REPLACE);
}
}
setState(1835);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALIAS:
{
setState(1828);
createAlias();
}
break;
case COMPOSITE:
{
setState(1829);
createCompositeDatabase();
}
break;
case CONSTRAINT:
{
setState(1830);
createConstraint();
}
break;
case DATABASE:
{
setState(1831);
createDatabase();
}
break;
case BTREE:
case FULLTEXT:
case INDEX:
case LOOKUP:
case POINT:
case RANGE:
case TEXT:
case VECTOR:
{
setState(1832);
createIndex();
}
break;
case ROLE:
{
setState(1833);
createRole();
}
break;
case USER:
{
setState(1834);
createUser();
}
break;
default:
throw new NoViableAltException(this);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DROP() {
return getToken(CypherParser.DROP, 0);
}
public DropAliasContext dropAlias() {
return getRuleContext(DropAliasContext.class, 0);
}
public DropConstraintContext dropConstraint() {
return getRuleContext(DropConstraintContext.class, 0);
}
public DropDatabaseContext dropDatabase() {
return getRuleContext(DropDatabaseContext.class, 0);
}
public DropIndexContext dropIndex() {
return getRuleContext(DropIndexContext.class, 0);
}
public DropRoleContext dropRole() {
return getRuleContext(DropRoleContext.class, 0);
}
public DropServerContext dropServer() {
return getRuleContext(DropServerContext.class, 0);
}
public DropUserContext dropUser() {
return getRuleContext(DropUserContext.class, 0);
}
public DropCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_dropCommand;
}
}
public final DropCommandContext dropCommand() throws RecognitionException {
DropCommandContext _localctx = new DropCommandContext(_ctx, getState());
enterRule(_localctx, 258, RULE_dropCommand);
try {
enterOuterAlt(_localctx, 1);
{
setState(1837);
match(DROP);
setState(1845);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALIAS:
{
setState(1838);
dropAlias();
}
break;
case CONSTRAINT:
{
setState(1839);
dropConstraint();
}
break;
case COMPOSITE:
case DATABASE:
{
setState(1840);
dropDatabase();
}
break;
case INDEX:
{
setState(1841);
dropIndex();
}
break;
case ROLE:
{
setState(1842);
dropRole();
}
break;
case SERVER:
{
setState(1843);
dropServer();
}
break;
case USER:
{
setState(1844);
dropUser();
}
break;
default:
throw new NoViableAltException(this);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ALTER() {
return getToken(CypherParser.ALTER, 0);
}
public AlterAliasContext alterAlias() {
return getRuleContext(AlterAliasContext.class, 0);
}
public AlterCurrentUserContext alterCurrentUser() {
return getRuleContext(AlterCurrentUserContext.class, 0);
}
public AlterDatabaseContext alterDatabase() {
return getRuleContext(AlterDatabaseContext.class, 0);
}
public AlterUserContext alterUser() {
return getRuleContext(AlterUserContext.class, 0);
}
public AlterServerContext alterServer() {
return getRuleContext(AlterServerContext.class, 0);
}
public AlterCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_alterCommand;
}
}
public final AlterCommandContext alterCommand() throws RecognitionException {
AlterCommandContext _localctx = new AlterCommandContext(_ctx, getState());
enterRule(_localctx, 260, RULE_alterCommand);
try {
enterOuterAlt(_localctx, 1);
{
setState(1847);
match(ALTER);
setState(1853);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALIAS:
{
setState(1848);
alterAlias();
}
break;
case CURRENT:
{
setState(1849);
alterCurrentUser();
}
break;
case DATABASE:
{
setState(1850);
alterDatabase();
}
break;
case USER:
{
setState(1851);
alterUser();
}
break;
case SERVER:
{
setState(1852);
alterServer();
}
break;
default:
throw new NoViableAltException(this);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RenameCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode RENAME() {
return getToken(CypherParser.RENAME, 0);
}
public RenameRoleContext renameRole() {
return getRuleContext(RenameRoleContext.class, 0);
}
public RenameServerContext renameServer() {
return getRuleContext(RenameServerContext.class, 0);
}
public RenameUserContext renameUser() {
return getRuleContext(RenameUserContext.class, 0);
}
public RenameCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_renameCommand;
}
}
public final RenameCommandContext renameCommand() throws RecognitionException {
RenameCommandContext _localctx = new RenameCommandContext(_ctx, getState());
enterRule(_localctx, 262, RULE_renameCommand);
try {
enterOuterAlt(_localctx, 1);
{
setState(1855);
match(RENAME);
setState(1859);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ROLE:
{
setState(1856);
renameRole();
}
break;
case SERVER:
{
setState(1857);
renameServer();
}
break;
case USER:
{
setState(1858);
renameUser();
}
break;
default:
throw new NoViableAltException(this);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SHOW() {
return getToken(CypherParser.SHOW, 0);
}
public ShowAliasesContext showAliases() {
return getRuleContext(ShowAliasesContext.class, 0);
}
public ShowConstraintCommandContext showConstraintCommand() {
return getRuleContext(ShowConstraintCommandContext.class, 0);
}
public ShowCurrentUserContext showCurrentUser() {
return getRuleContext(ShowCurrentUserContext.class, 0);
}
public ShowDatabaseContext showDatabase() {
return getRuleContext(ShowDatabaseContext.class, 0);
}
public ShowFunctionsContext showFunctions() {
return getRuleContext(ShowFunctionsContext.class, 0);
}
public ShowIndexCommandContext showIndexCommand() {
return getRuleContext(ShowIndexCommandContext.class, 0);
}
public ShowPrivilegesContext showPrivileges() {
return getRuleContext(ShowPrivilegesContext.class, 0);
}
public ShowProceduresContext showProcedures() {
return getRuleContext(ShowProceduresContext.class, 0);
}
public ShowRolePrivilegesContext showRolePrivileges() {
return getRuleContext(ShowRolePrivilegesContext.class, 0);
}
public ShowRolesContext showRoles() {
return getRuleContext(ShowRolesContext.class, 0);
}
public ShowServersContext showServers() {
return getRuleContext(ShowServersContext.class, 0);
}
public ShowSettingsContext showSettings() {
return getRuleContext(ShowSettingsContext.class, 0);
}
public ShowSupportedPrivilegesContext showSupportedPrivileges() {
return getRuleContext(ShowSupportedPrivilegesContext.class, 0);
}
public ShowTransactionsContext showTransactions() {
return getRuleContext(ShowTransactionsContext.class, 0);
}
public ShowUserPrivilegesContext showUserPrivileges() {
return getRuleContext(ShowUserPrivilegesContext.class, 0);
}
public ShowUsersContext showUsers() {
return getRuleContext(ShowUsersContext.class, 0);
}
public ShowCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showCommand;
}
}
public final ShowCommandContext showCommand() throws RecognitionException {
ShowCommandContext _localctx = new ShowCommandContext(_ctx, getState());
enterRule(_localctx, 264, RULE_showCommand);
try {
enterOuterAlt(_localctx, 1);
{
setState(1861);
match(SHOW);
setState(1878);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 191, _ctx)) {
case 1:
{
setState(1862);
showAliases();
}
break;
case 2:
{
setState(1863);
showConstraintCommand();
}
break;
case 3:
{
setState(1864);
showCurrentUser();
}
break;
case 4:
{
setState(1865);
showDatabase();
}
break;
case 5:
{
setState(1866);
showFunctions();
}
break;
case 6:
{
setState(1867);
showIndexCommand();
}
break;
case 7:
{
setState(1868);
showPrivileges();
}
break;
case 8:
{
setState(1869);
showProcedures();
}
break;
case 9:
{
setState(1870);
showRolePrivileges();
}
break;
case 10:
{
setState(1871);
showRoles();
}
break;
case 11:
{
setState(1872);
showServers();
}
break;
case 12:
{
setState(1873);
showSettings();
}
break;
case 13:
{
setState(1874);
showSupportedPrivileges();
}
break;
case 14:
{
setState(1875);
showTransactions();
}
break;
case 15:
{
setState(1876);
showUserPrivileges();
}
break;
case 16:
{
setState(1877);
showUsers();
}
break;
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowCommandYieldContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public YieldClauseContext yieldClause() {
return getRuleContext(YieldClauseContext.class, 0);
}
public ReturnClauseContext returnClause() {
return getRuleContext(ReturnClauseContext.class, 0);
}
public WhereClauseContext whereClause() {
return getRuleContext(WhereClauseContext.class, 0);
}
public ShowCommandYieldContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showCommandYield;
}
}
public final ShowCommandYieldContext showCommandYield() throws RecognitionException {
ShowCommandYieldContext _localctx = new ShowCommandYieldContext(_ctx, getState());
enterRule(_localctx, 266, RULE_showCommandYield);
int _la;
try {
setState(1885);
_errHandler.sync(this);
switch (_input.LA(1)) {
case YIELD:
enterOuterAlt(_localctx, 1);
{
setState(1880);
yieldClause();
setState(1882);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == RETURN) {
{
setState(1881);
returnClause();
}
}
}
break;
case WHERE:
enterOuterAlt(_localctx, 2);
{
setState(1884);
whereClause();
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class YieldItemContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List variable() {
return getRuleContexts(VariableContext.class);
}
public VariableContext variable(int i) {
return getRuleContext(VariableContext.class, i);
}
public TerminalNode AS() {
return getToken(CypherParser.AS, 0);
}
public YieldItemContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_yieldItem;
}
}
public final YieldItemContext yieldItem() throws RecognitionException {
YieldItemContext _localctx = new YieldItemContext(_ctx, getState());
enterRule(_localctx, 268, RULE_yieldItem);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1887);
variable();
setState(1890);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == AS) {
{
setState(1888);
match(AS);
setState(1889);
variable();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class YieldSkipContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SKIPROWS() {
return getToken(CypherParser.SKIPROWS, 0);
}
public SignedIntegerLiteralContext signedIntegerLiteral() {
return getRuleContext(SignedIntegerLiteralContext.class, 0);
}
public YieldSkipContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_yieldSkip;
}
}
public final YieldSkipContext yieldSkip() throws RecognitionException {
YieldSkipContext _localctx = new YieldSkipContext(_ctx, getState());
enterRule(_localctx, 270, RULE_yieldSkip);
try {
enterOuterAlt(_localctx, 1);
{
setState(1892);
match(SKIPROWS);
setState(1893);
signedIntegerLiteral();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class YieldLimitContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LIMITROWS() {
return getToken(CypherParser.LIMITROWS, 0);
}
public SignedIntegerLiteralContext signedIntegerLiteral() {
return getRuleContext(SignedIntegerLiteralContext.class, 0);
}
public YieldLimitContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_yieldLimit;
}
}
public final YieldLimitContext yieldLimit() throws RecognitionException {
YieldLimitContext _localctx = new YieldLimitContext(_ctx, getState());
enterRule(_localctx, 272, RULE_yieldLimit);
try {
enterOuterAlt(_localctx, 1);
{
setState(1895);
match(LIMITROWS);
setState(1896);
signedIntegerLiteral();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class YieldClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode YIELD() {
return getToken(CypherParser.YIELD, 0);
}
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public List yieldItem() {
return getRuleContexts(YieldItemContext.class);
}
public YieldItemContext yieldItem(int i) {
return getRuleContext(YieldItemContext.class, i);
}
public OrderByContext orderBy() {
return getRuleContext(OrderByContext.class, 0);
}
public YieldSkipContext yieldSkip() {
return getRuleContext(YieldSkipContext.class, 0);
}
public YieldLimitContext yieldLimit() {
return getRuleContext(YieldLimitContext.class, 0);
}
public WhereClauseContext whereClause() {
return getRuleContext(WhereClauseContext.class, 0);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public YieldClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_yieldClause;
}
}
public final YieldClauseContext yieldClause() throws RecognitionException {
YieldClauseContext _localctx = new YieldClauseContext(_ctx, getState());
enterRule(_localctx, 274, RULE_yieldClause);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1898);
match(YIELD);
setState(1908);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(1899);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
{
setState(1900);
yieldItem();
setState(1905);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(1901);
match(COMMA);
setState(1902);
yieldItem();
}
}
setState(1907);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(1911);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == ORDER) {
{
setState(1910);
orderBy();
}
}
setState(1914);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == SKIPROWS) {
{
setState(1913);
yieldSkip();
}
}
setState(1917);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == LIMITROWS) {
{
setState(1916);
yieldLimit();
}
}
setState(1920);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE) {
{
setState(1919);
whereClause();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowBriefAndYieldContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode BRIEF() {
return getToken(CypherParser.BRIEF, 0);
}
public TerminalNode VERBOSE() {
return getToken(CypherParser.VERBOSE, 0);
}
public TerminalNode OUTPUT() {
return getToken(CypherParser.OUTPUT, 0);
}
public YieldClauseContext yieldClause() {
return getRuleContext(YieldClauseContext.class, 0);
}
public ReturnClauseContext returnClause() {
return getRuleContext(ReturnClauseContext.class, 0);
}
public WhereClauseContext whereClause() {
return getRuleContext(WhereClauseContext.class, 0);
}
public ShowBriefAndYieldContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showBriefAndYield;
}
}
public final ShowBriefAndYieldContext showBriefAndYield() throws RecognitionException {
ShowBriefAndYieldContext _localctx = new ShowBriefAndYieldContext(_ctx, getState());
enterRule(_localctx, 276, RULE_showBriefAndYield);
int _la;
try {
setState(1931);
_errHandler.sync(this);
switch (_input.LA(1)) {
case BRIEF:
case VERBOSE:
enterOuterAlt(_localctx, 1);
{
setState(1922);
_la = _input.LA(1);
if (!(_la == BRIEF || _la == VERBOSE)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1924);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == OUTPUT) {
{
setState(1923);
match(OUTPUT);
}
}
}
break;
case YIELD:
enterOuterAlt(_localctx, 2);
{
setState(1926);
yieldClause();
setState(1928);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == RETURN) {
{
setState(1927);
returnClause();
}
}
}
break;
case WHERE:
enterOuterAlt(_localctx, 3);
{
setState(1930);
whereClause();
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowIndexCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ShowIndexesNoBriefContext showIndexesNoBrief() {
return getRuleContext(ShowIndexesNoBriefContext.class, 0);
}
public TerminalNode FULLTEXT() {
return getToken(CypherParser.FULLTEXT, 0);
}
public TerminalNode LOOKUP() {
return getToken(CypherParser.LOOKUP, 0);
}
public TerminalNode POINT() {
return getToken(CypherParser.POINT, 0);
}
public TerminalNode RANGE() {
return getToken(CypherParser.RANGE, 0);
}
public TerminalNode TEXT() {
return getToken(CypherParser.TEXT, 0);
}
public TerminalNode VECTOR() {
return getToken(CypherParser.VECTOR, 0);
}
public ShowIndexesAllowBriefContext showIndexesAllowBrief() {
return getRuleContext(ShowIndexesAllowBriefContext.class, 0);
}
public TerminalNode ALL() {
return getToken(CypherParser.ALL, 0);
}
public TerminalNode BTREE() {
return getToken(CypherParser.BTREE, 0);
}
public ShowIndexCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showIndexCommand;
}
}
public final ShowIndexCommandContext showIndexCommand() throws RecognitionException {
ShowIndexCommandContext _localctx = new ShowIndexCommandContext(_ctx, getState());
enterRule(_localctx, 278, RULE_showIndexCommand);
int _la;
try {
setState(1939);
_errHandler.sync(this);
switch (_input.LA(1)) {
case FULLTEXT:
case LOOKUP:
case POINT:
case RANGE:
case TEXT:
case VECTOR:
enterOuterAlt(_localctx, 1);
{
setState(1933);
_la = _input.LA(1);
if (!(_la == FULLTEXT
|| _la == LOOKUP
|| ((((_la - 190)) & ~0x3f) == 0 && ((1L << (_la - 190)) & 576460752303425537L) != 0)
|| _la == VECTOR)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1934);
showIndexesNoBrief();
}
break;
case ALL:
case BTREE:
case INDEX:
case INDEXES:
enterOuterAlt(_localctx, 2);
{
setState(1936);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == ALL || _la == BTREE) {
{
setState(1935);
_la = _input.LA(1);
if (!(_la == ALL || _la == BTREE)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(1938);
showIndexesAllowBrief();
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowIndexesAllowBriefContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public IndexTokenContext indexToken() {
return getRuleContext(IndexTokenContext.class, 0);
}
public ShowBriefAndYieldContext showBriefAndYield() {
return getRuleContext(ShowBriefAndYieldContext.class, 0);
}
public ComposableCommandClausesContext composableCommandClauses() {
return getRuleContext(ComposableCommandClausesContext.class, 0);
}
public ShowIndexesAllowBriefContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showIndexesAllowBrief;
}
}
public final ShowIndexesAllowBriefContext showIndexesAllowBrief() throws RecognitionException {
ShowIndexesAllowBriefContext _localctx = new ShowIndexesAllowBriefContext(_ctx, getState());
enterRule(_localctx, 280, RULE_showIndexesAllowBrief);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1941);
indexToken();
setState(1943);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == BRIEF || ((((_la - 277)) & ~0x3f) == 0 && ((1L << (_la - 277)) & 529L) != 0)) {
{
setState(1942);
showBriefAndYield();
}
}
setState(1946);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == SHOW || _la == TERMINATE) {
{
setState(1945);
composableCommandClauses();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowIndexesNoBriefContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public IndexTokenContext indexToken() {
return getRuleContext(IndexTokenContext.class, 0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class, 0);
}
public ComposableCommandClausesContext composableCommandClauses() {
return getRuleContext(ComposableCommandClausesContext.class, 0);
}
public ShowIndexesNoBriefContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showIndexesNoBrief;
}
}
public final ShowIndexesNoBriefContext showIndexesNoBrief() throws RecognitionException {
ShowIndexesNoBriefContext _localctx = new ShowIndexesNoBriefContext(_ctx, getState());
enterRule(_localctx, 282, RULE_showIndexesNoBrief);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(1948);
indexToken();
setState(1950);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(1949);
showCommandYield();
}
}
setState(1953);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == SHOW || _la == TERMINATE) {
{
setState(1952);
composableCommandClauses();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ShowConstraintCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showConstraintCommand;
}
public ShowConstraintCommandContext() {}
public void copyFrom(ShowConstraintCommandContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintUniqueContext extends ShowConstraintCommandContext {
public TerminalNode UNIQUE() {
return getToken(CypherParser.UNIQUE, 0);
}
public ShowConstraintsAllowYieldContext showConstraintsAllowYield() {
return getRuleContext(ShowConstraintsAllowYieldContext.class, 0);
}
public TerminalNode NODE() {
return getToken(CypherParser.NODE, 0);
}
public TerminalNode RELATIONSHIP() {
return getToken(CypherParser.RELATIONSHIP, 0);
}
public TerminalNode REL() {
return getToken(CypherParser.REL, 0);
}
public ShowConstraintUniqueContext(ShowConstraintCommandContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintMultiContext extends ShowConstraintCommandContext {
public ConstraintAllowYieldTypeContext constraintAllowYieldType() {
return getRuleContext(ConstraintAllowYieldTypeContext.class, 0);
}
public ShowConstraintsAllowYieldContext showConstraintsAllowYield() {
return getRuleContext(ShowConstraintsAllowYieldContext.class, 0);
}
public TerminalNode NODE() {
return getToken(CypherParser.NODE, 0);
}
public TerminalNode RELATIONSHIP() {
return getToken(CypherParser.RELATIONSHIP, 0);
}
public TerminalNode REL() {
return getToken(CypherParser.REL, 0);
}
public ShowConstraintMultiContext(ShowConstraintCommandContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintRelExistContext extends ShowConstraintCommandContext {
public TerminalNode REL() {
return getToken(CypherParser.REL, 0);
}
public TerminalNode EXIST() {
return getToken(CypherParser.EXIST, 0);
}
public ShowConstraintsAllowYieldContext showConstraintsAllowYield() {
return getRuleContext(ShowConstraintsAllowYieldContext.class, 0);
}
public ShowConstraintRelExistContext(ShowConstraintCommandContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintOldExistsContext extends ShowConstraintCommandContext {
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public ShowConstraintsAllowBriefContext showConstraintsAllowBrief() {
return getRuleContext(ShowConstraintsAllowBriefContext.class, 0);
}
public TerminalNode NODE() {
return getToken(CypherParser.NODE, 0);
}
public TerminalNode RELATIONSHIP() {
return getToken(CypherParser.RELATIONSHIP, 0);
}
public ShowConstraintOldExistsContext(ShowConstraintCommandContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintBriefAndYieldContext extends ShowConstraintCommandContext {
public ShowConstraintsAllowBriefAndYieldContext showConstraintsAllowBriefAndYield() {
return getRuleContext(ShowConstraintsAllowBriefAndYieldContext.class, 0);
}
public ConstraintBriefAndYieldTypeContext constraintBriefAndYieldType() {
return getRuleContext(ConstraintBriefAndYieldTypeContext.class, 0);
}
public ShowConstraintBriefAndYieldContext(ShowConstraintCommandContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintKeyContext extends ShowConstraintCommandContext {
public TerminalNode KEY() {
return getToken(CypherParser.KEY, 0);
}
public ShowConstraintsAllowYieldContext showConstraintsAllowYield() {
return getRuleContext(ShowConstraintsAllowYieldContext.class, 0);
}
public TerminalNode RELATIONSHIP() {
return getToken(CypherParser.RELATIONSHIP, 0);
}
public TerminalNode REL() {
return getToken(CypherParser.REL, 0);
}
public ShowConstraintKeyContext(ShowConstraintCommandContext ctx) {
copyFrom(ctx);
}
}
public final ShowConstraintCommandContext showConstraintCommand() throws RecognitionException {
ShowConstraintCommandContext _localctx = new ShowConstraintCommandContext(_ctx, getState());
enterRule(_localctx, 284, RULE_showConstraintCommand);
int _la;
try {
setState(1981);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 214, _ctx)) {
case 1:
_localctx = new ShowConstraintMultiContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(1956);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 164)) & ~0x3f) == 0 && ((1L << (_la - 164)) & 105553116266497L) != 0)) {
{
setState(1955);
_la = _input.LA(1);
if (!(((((_la - 164)) & ~0x3f) == 0
&& ((1L << (_la - 164)) & 105553116266497L) != 0))) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(1958);
constraintAllowYieldType();
setState(1959);
showConstraintsAllowYield();
}
break;
case 2:
_localctx = new ShowConstraintUniqueContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(1961);
_la = _input.LA(1);
if (!(((((_la - 164)) & ~0x3f) == 0 && ((1L << (_la - 164)) & 105553116266497L) != 0))) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(1962);
match(UNIQUE);
setState(1963);
showConstraintsAllowYield();
}
break;
case 3:
_localctx = new ShowConstraintKeyContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(1965);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == REL || _la == RELATIONSHIP) {
{
setState(1964);
_la = _input.LA(1);
if (!(_la == REL || _la == RELATIONSHIP)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(1967);
match(KEY);
setState(1968);
showConstraintsAllowYield();
}
break;
case 4:
_localctx = new ShowConstraintRelExistContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(1969);
match(REL);
setState(1970);
match(EXIST);
setState(1971);
showConstraintsAllowYield();
}
break;
case 5:
_localctx = new ShowConstraintOldExistsContext(_localctx);
enterOuterAlt(_localctx, 5);
{
setState(1973);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == NODE || _la == RELATIONSHIP) {
{
setState(1972);
_la = _input.LA(1);
if (!(_la == NODE || _la == RELATIONSHIP)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(1975);
match(EXISTS);
setState(1976);
showConstraintsAllowBrief();
}
break;
case 6:
_localctx = new ShowConstraintBriefAndYieldContext(_localctx);
enterOuterAlt(_localctx, 6);
{
setState(1978);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == ALL || _la == EXIST || _la == NODE || _la == RELATIONSHIP || _la == UNIQUE) {
{
setState(1977);
constraintBriefAndYieldType();
}
}
setState(1980);
showConstraintsAllowBriefAndYield();
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintAllowYieldTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode UNIQUENESS() {
return getToken(CypherParser.UNIQUENESS, 0);
}
public ConstraintExistTypeContext constraintExistType() {
return getRuleContext(ConstraintExistTypeContext.class, 0);
}
public TerminalNode PROPERTY() {
return getToken(CypherParser.PROPERTY, 0);
}
public TerminalNode TYPE() {
return getToken(CypherParser.TYPE, 0);
}
public ConstraintAllowYieldTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_constraintAllowYieldType;
}
}
public final ConstraintAllowYieldTypeContext constraintAllowYieldType() throws RecognitionException {
ConstraintAllowYieldTypeContext _localctx = new ConstraintAllowYieldTypeContext(_ctx, getState());
enterRule(_localctx, 286, RULE_constraintAllowYieldType);
try {
setState(1987);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 215, _ctx)) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(1983);
match(UNIQUENESS);
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(1984);
constraintExistType();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(1985);
match(PROPERTY);
setState(1986);
match(TYPE);
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintExistTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode EXISTENCE() {
return getToken(CypherParser.EXISTENCE, 0);
}
public TerminalNode PROPERTY() {
return getToken(CypherParser.PROPERTY, 0);
}
public TerminalNode EXIST() {
return getToken(CypherParser.EXIST, 0);
}
public ConstraintExistTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_constraintExistType;
}
}
public final ConstraintExistTypeContext constraintExistType() throws RecognitionException {
ConstraintExistTypeContext _localctx = new ConstraintExistTypeContext(_ctx, getState());
enterRule(_localctx, 288, RULE_constraintExistType);
try {
setState(1994);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 216, _ctx)) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(1989);
match(EXISTENCE);
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(1990);
match(PROPERTY);
setState(1991);
match(EXISTENCE);
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(1992);
match(PROPERTY);
setState(1993);
match(EXIST);
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintBriefAndYieldTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ALL() {
return getToken(CypherParser.ALL, 0);
}
public TerminalNode UNIQUE() {
return getToken(CypherParser.UNIQUE, 0);
}
public TerminalNode EXIST() {
return getToken(CypherParser.EXIST, 0);
}
public TerminalNode NODE() {
return getToken(CypherParser.NODE, 0);
}
public TerminalNode KEY() {
return getToken(CypherParser.KEY, 0);
}
public TerminalNode RELATIONSHIP() {
return getToken(CypherParser.RELATIONSHIP, 0);
}
public ConstraintBriefAndYieldTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_constraintBriefAndYieldType;
}
}
public final ConstraintBriefAndYieldTypeContext constraintBriefAndYieldType() throws RecognitionException {
ConstraintBriefAndYieldTypeContext _localctx = new ConstraintBriefAndYieldTypeContext(_ctx, getState());
enterRule(_localctx, 290, RULE_constraintBriefAndYieldType);
try {
setState(2005);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 217, _ctx)) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(1996);
match(ALL);
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(1997);
match(UNIQUE);
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(1998);
match(EXIST);
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(1999);
match(NODE);
setState(2000);
match(KEY);
}
break;
case 5:
enterOuterAlt(_localctx, 5);
{
setState(2001);
match(NODE);
setState(2002);
match(EXIST);
}
break;
case 6:
enterOuterAlt(_localctx, 6);
{
setState(2003);
match(RELATIONSHIP);
setState(2004);
match(EXIST);
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintsAllowBriefAndYieldContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ConstraintTokenContext constraintToken() {
return getRuleContext(ConstraintTokenContext.class, 0);
}
public ShowBriefAndYieldContext showBriefAndYield() {
return getRuleContext(ShowBriefAndYieldContext.class, 0);
}
public ComposableCommandClausesContext composableCommandClauses() {
return getRuleContext(ComposableCommandClausesContext.class, 0);
}
public ShowConstraintsAllowBriefAndYieldContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showConstraintsAllowBriefAndYield;
}
}
public final ShowConstraintsAllowBriefAndYieldContext showConstraintsAllowBriefAndYield()
throws RecognitionException {
ShowConstraintsAllowBriefAndYieldContext _localctx =
new ShowConstraintsAllowBriefAndYieldContext(_ctx, getState());
enterRule(_localctx, 292, RULE_showConstraintsAllowBriefAndYield);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2007);
constraintToken();
setState(2009);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == BRIEF || ((((_la - 277)) & ~0x3f) == 0 && ((1L << (_la - 277)) & 529L) != 0)) {
{
setState(2008);
showBriefAndYield();
}
}
setState(2012);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == SHOW || _la == TERMINATE) {
{
setState(2011);
composableCommandClauses();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintsAllowBriefContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ConstraintTokenContext constraintToken() {
return getRuleContext(ConstraintTokenContext.class, 0);
}
public ComposableCommandClausesContext composableCommandClauses() {
return getRuleContext(ComposableCommandClausesContext.class, 0);
}
public TerminalNode BRIEF() {
return getToken(CypherParser.BRIEF, 0);
}
public TerminalNode VERBOSE() {
return getToken(CypherParser.VERBOSE, 0);
}
public TerminalNode OUTPUT() {
return getToken(CypherParser.OUTPUT, 0);
}
public ShowConstraintsAllowBriefContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showConstraintsAllowBrief;
}
}
public final ShowConstraintsAllowBriefContext showConstraintsAllowBrief() throws RecognitionException {
ShowConstraintsAllowBriefContext _localctx = new ShowConstraintsAllowBriefContext(_ctx, getState());
enterRule(_localctx, 294, RULE_showConstraintsAllowBrief);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2014);
constraintToken();
setState(2019);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == BRIEF || _la == VERBOSE) {
{
setState(2015);
_la = _input.LA(1);
if (!(_la == BRIEF || _la == VERBOSE)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2017);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == OUTPUT) {
{
setState(2016);
match(OUTPUT);
}
}
}
}
setState(2022);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == SHOW || _la == TERMINATE) {
{
setState(2021);
composableCommandClauses();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowConstraintsAllowYieldContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ConstraintTokenContext constraintToken() {
return getRuleContext(ConstraintTokenContext.class, 0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class, 0);
}
public ComposableCommandClausesContext composableCommandClauses() {
return getRuleContext(ComposableCommandClausesContext.class, 0);
}
public ShowConstraintsAllowYieldContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showConstraintsAllowYield;
}
}
public final ShowConstraintsAllowYieldContext showConstraintsAllowYield() throws RecognitionException {
ShowConstraintsAllowYieldContext _localctx = new ShowConstraintsAllowYieldContext(_ctx, getState());
enterRule(_localctx, 296, RULE_showConstraintsAllowYield);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2024);
constraintToken();
setState(2026);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(2025);
showCommandYield();
}
}
setState(2029);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == SHOW || _la == TERMINATE) {
{
setState(2028);
composableCommandClauses();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowProceduresContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PROCEDURE() {
return getToken(CypherParser.PROCEDURE, 0);
}
public TerminalNode PROCEDURES() {
return getToken(CypherParser.PROCEDURES, 0);
}
public ExecutableByContext executableBy() {
return getRuleContext(ExecutableByContext.class, 0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class, 0);
}
public ComposableCommandClausesContext composableCommandClauses() {
return getRuleContext(ComposableCommandClausesContext.class, 0);
}
public ShowProceduresContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showProcedures;
}
}
public final ShowProceduresContext showProcedures() throws RecognitionException {
ShowProceduresContext _localctx = new ShowProceduresContext(_ctx, getState());
enterRule(_localctx, 298, RULE_showProcedures);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2031);
_la = _input.LA(1);
if (!(_la == PROCEDURE || _la == PROCEDURES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2033);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == EXECUTABLE) {
{
setState(2032);
executableBy();
}
}
setState(2036);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(2035);
showCommandYield();
}
}
setState(2039);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == SHOW || _la == TERMINATE) {
{
setState(2038);
composableCommandClauses();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowFunctionsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode FUNCTIONS() {
return getToken(CypherParser.FUNCTIONS, 0);
}
public ShowFunctionsTypeContext showFunctionsType() {
return getRuleContext(ShowFunctionsTypeContext.class, 0);
}
public ExecutableByContext executableBy() {
return getRuleContext(ExecutableByContext.class, 0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class, 0);
}
public ComposableCommandClausesContext composableCommandClauses() {
return getRuleContext(ComposableCommandClausesContext.class, 0);
}
public ShowFunctionsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showFunctions;
}
}
public final ShowFunctionsContext showFunctions() throws RecognitionException {
ShowFunctionsContext _localctx = new ShowFunctionsContext(_ctx, getState());
enterRule(_localctx, 300, RULE_showFunctions);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2042);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == ALL || _la == BUILT || _la == USER) {
{
setState(2041);
showFunctionsType();
}
}
setState(2044);
match(FUNCTIONS);
setState(2046);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == EXECUTABLE) {
{
setState(2045);
executableBy();
}
}
setState(2049);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(2048);
showCommandYield();
}
}
setState(2052);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == SHOW || _la == TERMINATE) {
{
setState(2051);
composableCommandClauses();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExecutableByContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode EXECUTABLE() {
return getToken(CypherParser.EXECUTABLE, 0);
}
public TerminalNode BY() {
return getToken(CypherParser.BY, 0);
}
public TerminalNode CURRENT() {
return getToken(CypherParser.CURRENT, 0);
}
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public ExecutableByContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_executableBy;
}
}
public final ExecutableByContext executableBy() throws RecognitionException {
ExecutableByContext _localctx = new ExecutableByContext(_ctx, getState());
enterRule(_localctx, 302, RULE_executableBy);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2054);
match(EXECUTABLE);
setState(2061);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == BY) {
{
setState(2055);
match(BY);
setState(2059);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 232, _ctx)) {
case 1:
{
setState(2056);
match(CURRENT);
setState(2057);
match(USER);
}
break;
case 2:
{
setState(2058);
symbolicNameString();
}
break;
}
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowFunctionsTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ALL() {
return getToken(CypherParser.ALL, 0);
}
public TerminalNode BUILT() {
return getToken(CypherParser.BUILT, 0);
}
public TerminalNode IN() {
return getToken(CypherParser.IN, 0);
}
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public TerminalNode DEFINED() {
return getToken(CypherParser.DEFINED, 0);
}
public ShowFunctionsTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showFunctionsType;
}
}
public final ShowFunctionsTypeContext showFunctionsType() throws RecognitionException {
ShowFunctionsTypeContext _localctx = new ShowFunctionsTypeContext(_ctx, getState());
enterRule(_localctx, 304, RULE_showFunctionsType);
try {
setState(2068);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALL:
enterOuterAlt(_localctx, 1);
{
setState(2063);
match(ALL);
}
break;
case BUILT:
enterOuterAlt(_localctx, 2);
{
setState(2064);
match(BUILT);
setState(2065);
match(IN);
}
break;
case USER:
enterOuterAlt(_localctx, 3);
{
setState(2066);
match(USER);
setState(2067);
match(DEFINED);
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowTransactionsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TransactionTokenContext transactionToken() {
return getRuleContext(TransactionTokenContext.class, 0);
}
public NamesAndClausesContext namesAndClauses() {
return getRuleContext(NamesAndClausesContext.class, 0);
}
public ShowTransactionsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showTransactions;
}
}
public final ShowTransactionsContext showTransactions() throws RecognitionException {
ShowTransactionsContext _localctx = new ShowTransactionsContext(_ctx, getState());
enterRule(_localctx, 306, RULE_showTransactions);
try {
enterOuterAlt(_localctx, 1);
{
setState(2070);
transactionToken();
setState(2071);
namesAndClauses();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TerminateCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode TERMINATE() {
return getToken(CypherParser.TERMINATE, 0);
}
public TerminateTransactionsContext terminateTransactions() {
return getRuleContext(TerminateTransactionsContext.class, 0);
}
public TerminateCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_terminateCommand;
}
}
public final TerminateCommandContext terminateCommand() throws RecognitionException {
TerminateCommandContext _localctx = new TerminateCommandContext(_ctx, getState());
enterRule(_localctx, 308, RULE_terminateCommand);
try {
enterOuterAlt(_localctx, 1);
{
setState(2073);
match(TERMINATE);
setState(2074);
terminateTransactions();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TerminateTransactionsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TransactionTokenContext transactionToken() {
return getRuleContext(TransactionTokenContext.class, 0);
}
public NamesAndClausesContext namesAndClauses() {
return getRuleContext(NamesAndClausesContext.class, 0);
}
public TerminateTransactionsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_terminateTransactions;
}
}
public final TerminateTransactionsContext terminateTransactions() throws RecognitionException {
TerminateTransactionsContext _localctx = new TerminateTransactionsContext(_ctx, getState());
enterRule(_localctx, 310, RULE_terminateTransactions);
try {
enterOuterAlt(_localctx, 1);
{
setState(2076);
transactionToken();
setState(2077);
namesAndClauses();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowSettingsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SETTING() {
return getToken(CypherParser.SETTING, 0);
}
public NamesAndClausesContext namesAndClauses() {
return getRuleContext(NamesAndClausesContext.class, 0);
}
public ShowSettingsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showSettings;
}
}
public final ShowSettingsContext showSettings() throws RecognitionException {
ShowSettingsContext _localctx = new ShowSettingsContext(_ctx, getState());
enterRule(_localctx, 312, RULE_showSettings);
try {
enterOuterAlt(_localctx, 1);
{
setState(2079);
match(SETTING);
setState(2080);
namesAndClauses();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NamesAndClausesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public StringsOrExpressionContext stringsOrExpression() {
return getRuleContext(StringsOrExpressionContext.class, 0);
}
public ComposableCommandClausesContext composableCommandClauses() {
return getRuleContext(ComposableCommandClausesContext.class, 0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class, 0);
}
public NamesAndClausesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_namesAndClauses;
}
}
public final NamesAndClausesContext namesAndClauses() throws RecognitionException {
NamesAndClausesContext _localctx = new NamesAndClausesContext(_ctx, getState());
enterRule(_localctx, 314, RULE_namesAndClauses);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2089);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 237, _ctx)) {
case 1:
{
setState(2083);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(2082);
showCommandYield();
}
}
}
break;
case 2:
{
setState(2085);
stringsOrExpression();
setState(2087);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(2086);
showCommandYield();
}
}
}
break;
}
setState(2092);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == SHOW || _la == TERMINATE) {
{
setState(2091);
composableCommandClauses();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ComposableCommandClausesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminateCommandContext terminateCommand() {
return getRuleContext(TerminateCommandContext.class, 0);
}
public ComposableShowCommandClausesContext composableShowCommandClauses() {
return getRuleContext(ComposableShowCommandClausesContext.class, 0);
}
public ComposableCommandClausesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_composableCommandClauses;
}
}
public final ComposableCommandClausesContext composableCommandClauses() throws RecognitionException {
ComposableCommandClausesContext _localctx = new ComposableCommandClausesContext(_ctx, getState());
enterRule(_localctx, 316, RULE_composableCommandClauses);
try {
setState(2096);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TERMINATE:
enterOuterAlt(_localctx, 1);
{
setState(2094);
terminateCommand();
}
break;
case SHOW:
enterOuterAlt(_localctx, 2);
{
setState(2095);
composableShowCommandClauses();
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ComposableShowCommandClausesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SHOW() {
return getToken(CypherParser.SHOW, 0);
}
public ShowIndexCommandContext showIndexCommand() {
return getRuleContext(ShowIndexCommandContext.class, 0);
}
public ShowConstraintCommandContext showConstraintCommand() {
return getRuleContext(ShowConstraintCommandContext.class, 0);
}
public ShowFunctionsContext showFunctions() {
return getRuleContext(ShowFunctionsContext.class, 0);
}
public ShowProceduresContext showProcedures() {
return getRuleContext(ShowProceduresContext.class, 0);
}
public ShowSettingsContext showSettings() {
return getRuleContext(ShowSettingsContext.class, 0);
}
public ShowTransactionsContext showTransactions() {
return getRuleContext(ShowTransactionsContext.class, 0);
}
public ComposableShowCommandClausesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_composableShowCommandClauses;
}
}
public final ComposableShowCommandClausesContext composableShowCommandClauses() throws RecognitionException {
ComposableShowCommandClausesContext _localctx = new ComposableShowCommandClausesContext(_ctx, getState());
enterRule(_localctx, 318, RULE_composableShowCommandClauses);
try {
enterOuterAlt(_localctx, 1);
{
setState(2098);
match(SHOW);
setState(2105);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 240, _ctx)) {
case 1:
{
setState(2099);
showIndexCommand();
}
break;
case 2:
{
setState(2100);
showConstraintCommand();
}
break;
case 3:
{
setState(2101);
showFunctions();
}
break;
case 4:
{
setState(2102);
showProcedures();
}
break;
case 5:
{
setState(2103);
showSettings();
}
break;
case 6:
{
setState(2104);
showTransactions();
}
break;
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StringsOrExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public StringListContext stringList() {
return getRuleContext(StringListContext.class, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public StringsOrExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_stringsOrExpression;
}
}
public final StringsOrExpressionContext stringsOrExpression() throws RecognitionException {
StringsOrExpressionContext _localctx = new StringsOrExpressionContext(_ctx, getState());
enterRule(_localctx, 320, RULE_stringsOrExpression);
try {
setState(2109);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 241, _ctx)) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(2107);
stringList();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(2108);
expression();
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List typePart() {
return getRuleContexts(TypePartContext.class);
}
public TypePartContext typePart(int i) {
return getRuleContext(TypePartContext.class, i);
}
public List BAR() {
return getTokens(CypherParser.BAR);
}
public TerminalNode BAR(int i) {
return getToken(CypherParser.BAR, i);
}
public TypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_type;
}
}
public final TypeContext type() throws RecognitionException {
TypeContext _localctx = new TypeContext(_ctx, getState());
enterRule(_localctx, 322, RULE_type);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(2111);
typePart();
setState(2116);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 242, _ctx);
while (_alt != 2 && _alt != org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER) {
if (_alt == 1) {
{
{
setState(2112);
match(BAR);
setState(2113);
typePart();
}
}
}
setState(2118);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input, 242, _ctx);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypePartContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TypeNameContext typeName() {
return getRuleContext(TypeNameContext.class, 0);
}
public TypeNullabilityContext typeNullability() {
return getRuleContext(TypeNullabilityContext.class, 0);
}
public List typeListSuffix() {
return getRuleContexts(TypeListSuffixContext.class);
}
public TypeListSuffixContext typeListSuffix(int i) {
return getRuleContext(TypeListSuffixContext.class, i);
}
public TypePartContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_typePart;
}
}
public final TypePartContext typePart() throws RecognitionException {
TypePartContext _localctx = new TypePartContext(_ctx, getState());
enterRule(_localctx, 324, RULE_typePart);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2119);
typeName();
setState(2121);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == EXCLAMATION_MARK || _la == NOT) {
{
setState(2120);
typeNullability();
}
}
setState(2126);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == ARRAY || _la == LIST) {
{
{
setState(2123);
typeListSuffix();
}
}
setState(2128);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeNameContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode NOTHING() {
return getToken(CypherParser.NOTHING, 0);
}
public TerminalNode NULL() {
return getToken(CypherParser.NULL, 0);
}
public TerminalNode BOOLEAN() {
return getToken(CypherParser.BOOLEAN, 0);
}
public TerminalNode STRING() {
return getToken(CypherParser.STRING, 0);
}
public TerminalNode INT() {
return getToken(CypherParser.INT, 0);
}
public TerminalNode INTEGER() {
return getToken(CypherParser.INTEGER, 0);
}
public TerminalNode SIGNED() {
return getToken(CypherParser.SIGNED, 0);
}
public TerminalNode FLOAT() {
return getToken(CypherParser.FLOAT, 0);
}
public TerminalNode DATE() {
return getToken(CypherParser.DATE, 0);
}
public TerminalNode LOCAL() {
return getToken(CypherParser.LOCAL, 0);
}
public TerminalNode TIME() {
return getToken(CypherParser.TIME, 0);
}
public TerminalNode DATETIME() {
return getToken(CypherParser.DATETIME, 0);
}
public TerminalNode ZONED() {
return getToken(CypherParser.ZONED, 0);
}
public TerminalNode WITHOUT() {
return getToken(CypherParser.WITHOUT, 0);
}
public TerminalNode TIMEZONE() {
return getToken(CypherParser.TIMEZONE, 0);
}
public TerminalNode WITH() {
return getToken(CypherParser.WITH, 0);
}
public TerminalNode TIMESTAMP() {
return getToken(CypherParser.TIMESTAMP, 0);
}
public TerminalNode DURATION() {
return getToken(CypherParser.DURATION, 0);
}
public TerminalNode POINT() {
return getToken(CypherParser.POINT, 0);
}
public TerminalNode NODE() {
return getToken(CypherParser.NODE, 0);
}
public TerminalNode VERTEX() {
return getToken(CypherParser.VERTEX, 0);
}
public TerminalNode RELATIONSHIP() {
return getToken(CypherParser.RELATIONSHIP, 0);
}
public TerminalNode EDGE() {
return getToken(CypherParser.EDGE, 0);
}
public TerminalNode MAP() {
return getToken(CypherParser.MAP, 0);
}
public TerminalNode LT() {
return getToken(CypherParser.LT, 0);
}
public TypeContext type() {
return getRuleContext(TypeContext.class, 0);
}
public TerminalNode GT() {
return getToken(CypherParser.GT, 0);
}
public TerminalNode LIST() {
return getToken(CypherParser.LIST, 0);
}
public TerminalNode ARRAY() {
return getToken(CypherParser.ARRAY, 0);
}
public TerminalNode PATH() {
return getToken(CypherParser.PATH, 0);
}
public TerminalNode PROPERTY() {
return getToken(CypherParser.PROPERTY, 0);
}
public TerminalNode VALUE() {
return getToken(CypherParser.VALUE, 0);
}
public TerminalNode ANY() {
return getToken(CypherParser.ANY, 0);
}
public TypeNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_typeName;
}
}
public final TypeNameContext typeName() throws RecognitionException {
TypeNameContext _localctx = new TypeNameContext(_ctx, getState());
enterRule(_localctx, 326, RULE_typeName);
int _la;
try {
setState(2191);
_errHandler.sync(this);
switch (_input.LA(1)) {
case NOTHING:
enterOuterAlt(_localctx, 1);
{
setState(2129);
match(NOTHING);
}
break;
case NULL:
enterOuterAlt(_localctx, 2);
{
setState(2130);
match(NULL);
}
break;
case BOOLEAN:
enterOuterAlt(_localctx, 3);
{
setState(2131);
match(BOOLEAN);
}
break;
case STRING:
enterOuterAlt(_localctx, 4);
{
setState(2132);
match(STRING);
}
break;
case INT:
enterOuterAlt(_localctx, 5);
{
setState(2133);
match(INT);
}
break;
case INTEGER:
case SIGNED:
enterOuterAlt(_localctx, 6);
{
setState(2135);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == SIGNED) {
{
setState(2134);
match(SIGNED);
}
}
setState(2137);
match(INTEGER);
}
break;
case FLOAT:
enterOuterAlt(_localctx, 7);
{
setState(2138);
match(FLOAT);
}
break;
case DATE:
enterOuterAlt(_localctx, 8);
{
setState(2139);
match(DATE);
}
break;
case LOCAL:
enterOuterAlt(_localctx, 9);
{
setState(2140);
match(LOCAL);
setState(2141);
_la = _input.LA(1);
if (!(_la == DATETIME || _la == TIME)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
case ZONED:
enterOuterAlt(_localctx, 10);
{
setState(2142);
match(ZONED);
setState(2143);
_la = _input.LA(1);
if (!(_la == DATETIME || _la == TIME)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
case TIME:
enterOuterAlt(_localctx, 11);
{
setState(2144);
match(TIME);
setState(2149);
_errHandler.sync(this);
switch (_input.LA(1)) {
case WITHOUT:
{
setState(2145);
match(WITHOUT);
setState(2146);
match(TIMEZONE);
}
break;
case WITH:
{
setState(2147);
match(WITH);
setState(2148);
match(TIMEZONE);
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
case TIMESTAMP:
enterOuterAlt(_localctx, 12);
{
setState(2151);
match(TIMESTAMP);
setState(2156);
_errHandler.sync(this);
switch (_input.LA(1)) {
case WITHOUT:
{
setState(2152);
match(WITHOUT);
setState(2153);
match(TIMEZONE);
}
break;
case WITH:
{
setState(2154);
match(WITH);
setState(2155);
match(TIMEZONE);
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
case DURATION:
enterOuterAlt(_localctx, 13);
{
setState(2158);
match(DURATION);
}
break;
case POINT:
enterOuterAlt(_localctx, 14);
{
setState(2159);
match(POINT);
}
break;
case NODE:
enterOuterAlt(_localctx, 15);
{
setState(2160);
match(NODE);
}
break;
case VERTEX:
enterOuterAlt(_localctx, 16);
{
setState(2161);
match(VERTEX);
}
break;
case RELATIONSHIP:
enterOuterAlt(_localctx, 17);
{
setState(2162);
match(RELATIONSHIP);
}
break;
case EDGE:
enterOuterAlt(_localctx, 18);
{
setState(2163);
match(EDGE);
}
break;
case MAP:
enterOuterAlt(_localctx, 19);
{
setState(2164);
match(MAP);
}
break;
case ARRAY:
case LIST:
enterOuterAlt(_localctx, 20);
{
setState(2165);
_la = _input.LA(1);
if (!(_la == ARRAY || _la == LIST)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2166);
match(LT);
setState(2167);
type();
setState(2168);
match(GT);
}
break;
case PATH:
enterOuterAlt(_localctx, 21);
{
setState(2170);
match(PATH);
}
break;
case PROPERTY:
enterOuterAlt(_localctx, 22);
{
setState(2171);
match(PROPERTY);
setState(2172);
match(VALUE);
}
break;
case ANY:
enterOuterAlt(_localctx, 23);
{
setState(2173);
match(ANY);
setState(2189);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 249, _ctx)) {
case 1:
{
setState(2174);
match(NODE);
}
break;
case 2:
{
setState(2175);
match(VERTEX);
}
break;
case 3:
{
setState(2176);
match(RELATIONSHIP);
}
break;
case 4:
{
setState(2177);
match(EDGE);
}
break;
case 5:
{
setState(2178);
match(MAP);
}
break;
case 6:
{
setState(2179);
match(PROPERTY);
setState(2180);
match(VALUE);
}
break;
case 7:
{
setState(2182);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == VALUE) {
{
setState(2181);
match(VALUE);
}
}
setState(2184);
match(LT);
setState(2185);
type();
setState(2186);
match(GT);
}
break;
case 8:
{
setState(2188);
match(VALUE);
}
break;
}
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeNullabilityContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public TerminalNode NULL() {
return getToken(CypherParser.NULL, 0);
}
public TerminalNode EXCLAMATION_MARK() {
return getToken(CypherParser.EXCLAMATION_MARK, 0);
}
public TypeNullabilityContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_typeNullability;
}
}
public final TypeNullabilityContext typeNullability() throws RecognitionException {
TypeNullabilityContext _localctx = new TypeNullabilityContext(_ctx, getState());
enterRule(_localctx, 328, RULE_typeNullability);
try {
setState(2196);
_errHandler.sync(this);
switch (_input.LA(1)) {
case NOT:
enterOuterAlt(_localctx, 1);
{
setState(2193);
match(NOT);
setState(2194);
match(NULL);
}
break;
case EXCLAMATION_MARK:
enterOuterAlt(_localctx, 2);
{
setState(2195);
match(EXCLAMATION_MARK);
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeListSuffixContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LIST() {
return getToken(CypherParser.LIST, 0);
}
public TerminalNode ARRAY() {
return getToken(CypherParser.ARRAY, 0);
}
public TypeNullabilityContext typeNullability() {
return getRuleContext(TypeNullabilityContext.class, 0);
}
public TypeListSuffixContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_typeListSuffix;
}
}
public final TypeListSuffixContext typeListSuffix() throws RecognitionException {
TypeListSuffixContext _localctx = new TypeListSuffixContext(_ctx, getState());
enterRule(_localctx, 330, RULE_typeListSuffix);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2198);
_la = _input.LA(1);
if (!(_la == ARRAY || _la == LIST)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2200);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == EXCLAMATION_MARK || _la == NOT) {
{
setState(2199);
typeNullability();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CommandNodePatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public LabelTypeContext labelType() {
return getRuleContext(LabelTypeContext.class, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public CommandNodePatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_commandNodePattern;
}
}
public final CommandNodePatternContext commandNodePattern() throws RecognitionException {
CommandNodePatternContext _localctx = new CommandNodePatternContext(_ctx, getState());
enterRule(_localctx, 332, RULE_commandNodePattern);
try {
enterOuterAlt(_localctx, 1);
{
setState(2202);
match(LPAREN);
setState(2203);
variable();
setState(2204);
labelType();
setState(2205);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CommandRelPatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List LPAREN() {
return getTokens(CypherParser.LPAREN);
}
public TerminalNode LPAREN(int i) {
return getToken(CypherParser.LPAREN, i);
}
public List RPAREN() {
return getTokens(CypherParser.RPAREN);
}
public TerminalNode RPAREN(int i) {
return getToken(CypherParser.RPAREN, i);
}
public List arrowLine() {
return getRuleContexts(ArrowLineContext.class);
}
public ArrowLineContext arrowLine(int i) {
return getRuleContext(ArrowLineContext.class, i);
}
public TerminalNode LBRACKET() {
return getToken(CypherParser.LBRACKET, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public RelTypeContext relType() {
return getRuleContext(RelTypeContext.class, 0);
}
public TerminalNode RBRACKET() {
return getToken(CypherParser.RBRACKET, 0);
}
public LeftArrowContext leftArrow() {
return getRuleContext(LeftArrowContext.class, 0);
}
public RightArrowContext rightArrow() {
return getRuleContext(RightArrowContext.class, 0);
}
public CommandRelPatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_commandRelPattern;
}
}
public final CommandRelPatternContext commandRelPattern() throws RecognitionException {
CommandRelPatternContext _localctx = new CommandRelPatternContext(_ctx, getState());
enterRule(_localctx, 334, RULE_commandRelPattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2207);
match(LPAREN);
setState(2208);
match(RPAREN);
setState(2210);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == LT || _la == ARROW_LEFT_HEAD) {
{
setState(2209);
leftArrow();
}
}
setState(2212);
arrowLine();
setState(2213);
match(LBRACKET);
setState(2214);
variable();
setState(2215);
relType();
setState(2216);
match(RBRACKET);
setState(2217);
arrowLine();
setState(2219);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == GT || _la == ARROW_RIGHT_HEAD) {
{
setState(2218);
rightArrow();
}
}
setState(2221);
match(LPAREN);
setState(2222);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateConstraintContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CONSTRAINT() {
return getToken(CypherParser.CONSTRAINT, 0);
}
public ConstraintTypeContext constraintType() {
return getRuleContext(ConstraintTypeContext.class, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public TerminalNode FOR() {
return getToken(CypherParser.FOR, 0);
}
public CommandNodePatternContext commandNodePattern() {
return getRuleContext(CommandNodePatternContext.class, 0);
}
public CommandRelPatternContext commandRelPattern() {
return getRuleContext(CommandRelPatternContext.class, 0);
}
public SymbolicNameOrStringParameterContext symbolicNameOrStringParameter() {
return getRuleContext(SymbolicNameOrStringParameterContext.class, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public CommandOptionsContext commandOptions() {
return getRuleContext(CommandOptionsContext.class, 0);
}
public CreateConstraintContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createConstraint;
}
}
public final CreateConstraintContext createConstraint() throws RecognitionException {
CreateConstraintContext _localctx = new CreateConstraintContext(_ctx, getState());
enterRule(_localctx, 336, RULE_createConstraint);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2224);
match(CONSTRAINT);
setState(2226);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 255, _ctx)) {
case 1:
{
setState(2225);
symbolicNameOrStringParameter();
}
break;
}
setState(2231);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(2228);
match(IF);
setState(2229);
match(NOT);
setState(2230);
match(EXISTS);
}
}
setState(2233);
_la = _input.LA(1);
if (!(_la == FOR || _la == ON)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2236);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 257, _ctx)) {
case 1:
{
setState(2234);
commandNodePattern();
}
break;
case 2:
{
setState(2235);
commandRelPattern();
}
break;
}
setState(2238);
constraintType();
setState(2240);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == OPTIONS) {
{
setState(2239);
commandOptions();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public ConstraintTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_constraintType;
}
public ConstraintTypeContext() {}
public void copyFrom(ConstraintTypeContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintTypedContext extends ConstraintTypeContext {
public PropertyListContext propertyList() {
return getRuleContext(PropertyListContext.class, 0);
}
public TypeContext type() {
return getRuleContext(TypeContext.class, 0);
}
public TerminalNode REQUIRE() {
return getToken(CypherParser.REQUIRE, 0);
}
public TerminalNode ASSERT() {
return getToken(CypherParser.ASSERT, 0);
}
public TerminalNode COLONCOLON() {
return getToken(CypherParser.COLONCOLON, 0);
}
public TerminalNode IS() {
return getToken(CypherParser.IS, 0);
}
public TerminalNode TYPED() {
return getToken(CypherParser.TYPED, 0);
}
public ConstraintTypedContext(ConstraintTypeContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintExistsContext extends ConstraintTypeContext {
public TerminalNode ASSERT() {
return getToken(CypherParser.ASSERT, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public PropertyListContext propertyList() {
return getRuleContext(PropertyListContext.class, 0);
}
public ConstraintExistsContext(ConstraintTypeContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintKeyContext extends ConstraintTypeContext {
public PropertyListContext propertyList() {
return getRuleContext(PropertyListContext.class, 0);
}
public TerminalNode IS() {
return getToken(CypherParser.IS, 0);
}
public TerminalNode KEY() {
return getToken(CypherParser.KEY, 0);
}
public TerminalNode REQUIRE() {
return getToken(CypherParser.REQUIRE, 0);
}
public TerminalNode ASSERT() {
return getToken(CypherParser.ASSERT, 0);
}
public TerminalNode NODE() {
return getToken(CypherParser.NODE, 0);
}
public TerminalNode RELATIONSHIP() {
return getToken(CypherParser.RELATIONSHIP, 0);
}
public TerminalNode REL() {
return getToken(CypherParser.REL, 0);
}
public ConstraintKeyContext(ConstraintTypeContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintIsNotNullContext extends ConstraintTypeContext {
public PropertyListContext propertyList() {
return getRuleContext(PropertyListContext.class, 0);
}
public TerminalNode IS() {
return getToken(CypherParser.IS, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public TerminalNode NULL() {
return getToken(CypherParser.NULL, 0);
}
public TerminalNode REQUIRE() {
return getToken(CypherParser.REQUIRE, 0);
}
public TerminalNode ASSERT() {
return getToken(CypherParser.ASSERT, 0);
}
public ConstraintIsNotNullContext(ConstraintTypeContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintIsUniqueContext extends ConstraintTypeContext {
public PropertyListContext propertyList() {
return getRuleContext(PropertyListContext.class, 0);
}
public TerminalNode IS() {
return getToken(CypherParser.IS, 0);
}
public TerminalNode UNIQUE() {
return getToken(CypherParser.UNIQUE, 0);
}
public TerminalNode REQUIRE() {
return getToken(CypherParser.REQUIRE, 0);
}
public TerminalNode ASSERT() {
return getToken(CypherParser.ASSERT, 0);
}
public TerminalNode NODE() {
return getToken(CypherParser.NODE, 0);
}
public TerminalNode RELATIONSHIP() {
return getToken(CypherParser.RELATIONSHIP, 0);
}
public TerminalNode REL() {
return getToken(CypherParser.REL, 0);
}
public ConstraintIsUniqueContext(ConstraintTypeContext ctx) {
copyFrom(ctx);
}
}
public final ConstraintTypeContext constraintType() throws RecognitionException {
ConstraintTypeContext _localctx = new ConstraintTypeContext(_ctx, getState());
enterRule(_localctx, 338, RULE_constraintType);
int _la;
try {
setState(2276);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 262, _ctx)) {
case 1:
_localctx = new ConstraintExistsContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(2242);
match(ASSERT);
setState(2243);
match(EXISTS);
setState(2244);
propertyList();
}
break;
case 2:
_localctx = new ConstraintTypedContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(2245);
_la = _input.LA(1);
if (!(_la == ASSERT || _la == REQUIRE)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2246);
propertyList();
setState(2250);
_errHandler.sync(this);
switch (_input.LA(1)) {
case COLONCOLON:
{
setState(2247);
match(COLONCOLON);
}
break;
case IS:
{
setState(2248);
match(IS);
setState(2249);
_la = _input.LA(1);
if (!(_la == COLONCOLON || _la == TYPED)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(2252);
type();
}
break;
case 3:
_localctx = new ConstraintIsUniqueContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(2254);
_la = _input.LA(1);
if (!(_la == ASSERT || _la == REQUIRE)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2255);
propertyList();
setState(2256);
match(IS);
setState(2258);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 164)) & ~0x3f) == 0 && ((1L << (_la - 164)) & 105553116266497L) != 0)) {
{
setState(2257);
_la = _input.LA(1);
if (!(((((_la - 164)) & ~0x3f) == 0
&& ((1L << (_la - 164)) & 105553116266497L) != 0))) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(2260);
match(UNIQUE);
}
break;
case 4:
_localctx = new ConstraintKeyContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(2262);
_la = _input.LA(1);
if (!(_la == ASSERT || _la == REQUIRE)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2263);
propertyList();
setState(2264);
match(IS);
setState(2266);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 164)) & ~0x3f) == 0 && ((1L << (_la - 164)) & 105553116266497L) != 0)) {
{
setState(2265);
_la = _input.LA(1);
if (!(((((_la - 164)) & ~0x3f) == 0
&& ((1L << (_la - 164)) & 105553116266497L) != 0))) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(2268);
match(KEY);
}
break;
case 5:
_localctx = new ConstraintIsNotNullContext(_localctx);
enterOuterAlt(_localctx, 5);
{
setState(2270);
_la = _input.LA(1);
if (!(_la == ASSERT || _la == REQUIRE)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2271);
propertyList();
setState(2272);
match(IS);
setState(2273);
match(NOT);
setState(2274);
match(NULL);
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropConstraintContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CONSTRAINT() {
return getToken(CypherParser.CONSTRAINT, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public TerminalNode ASSERT() {
return getToken(CypherParser.ASSERT, 0);
}
public SymbolicNameOrStringParameterContext symbolicNameOrStringParameter() {
return getRuleContext(SymbolicNameOrStringParameterContext.class, 0);
}
public CommandNodePatternContext commandNodePattern() {
return getRuleContext(CommandNodePatternContext.class, 0);
}
public CommandRelPatternContext commandRelPattern() {
return getRuleContext(CommandRelPatternContext.class, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public PropertyListContext propertyList() {
return getRuleContext(PropertyListContext.class, 0);
}
public TerminalNode IS() {
return getToken(CypherParser.IS, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode UNIQUE() {
return getToken(CypherParser.UNIQUE, 0);
}
public TerminalNode NODE() {
return getToken(CypherParser.NODE, 0);
}
public TerminalNode KEY() {
return getToken(CypherParser.KEY, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public TerminalNode NULL() {
return getToken(CypherParser.NULL, 0);
}
public DropConstraintContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_dropConstraint;
}
}
public final DropConstraintContext dropConstraint() throws RecognitionException {
DropConstraintContext _localctx = new DropConstraintContext(_ctx, getState());
enterRule(_localctx, 340, RULE_dropConstraint);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2278);
match(CONSTRAINT);
setState(2303);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 267, _ctx)) {
case 1:
{
setState(2279);
match(ON);
setState(2282);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 263, _ctx)) {
case 1:
{
setState(2280);
commandNodePattern();
}
break;
case 2:
{
setState(2281);
commandRelPattern();
}
break;
}
setState(2284);
match(ASSERT);
setState(2296);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 265, _ctx)) {
case 1:
{
setState(2285);
match(EXISTS);
setState(2286);
propertyList();
}
break;
case 2:
{
setState(2287);
propertyList();
setState(2288);
match(IS);
setState(2294);
_errHandler.sync(this);
switch (_input.LA(1)) {
case UNIQUE:
{
setState(2289);
match(UNIQUE);
}
break;
case NODE:
{
setState(2290);
match(NODE);
setState(2291);
match(KEY);
}
break;
case NOT:
{
setState(2292);
match(NOT);
setState(2293);
match(NULL);
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
}
}
break;
case 2:
{
setState(2298);
symbolicNameOrStringParameter();
setState(2301);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(2299);
match(IF);
setState(2300);
match(EXISTS);
}
}
}
break;
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateIndexContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode BTREE() {
return getToken(CypherParser.BTREE, 0);
}
public TerminalNode INDEX() {
return getToken(CypherParser.INDEX, 0);
}
public CreateIndex_Context createIndex_() {
return getRuleContext(CreateIndex_Context.class, 0);
}
public TerminalNode RANGE() {
return getToken(CypherParser.RANGE, 0);
}
public TerminalNode TEXT() {
return getToken(CypherParser.TEXT, 0);
}
public TerminalNode POINT() {
return getToken(CypherParser.POINT, 0);
}
public TerminalNode VECTOR() {
return getToken(CypherParser.VECTOR, 0);
}
public TerminalNode LOOKUP() {
return getToken(CypherParser.LOOKUP, 0);
}
public CreateLookupIndexContext createLookupIndex() {
return getRuleContext(CreateLookupIndexContext.class, 0);
}
public TerminalNode FULLTEXT() {
return getToken(CypherParser.FULLTEXT, 0);
}
public CreateFulltextIndexContext createFulltextIndex() {
return getRuleContext(CreateFulltextIndexContext.class, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public OldCreateIndexContext oldCreateIndex() {
return getRuleContext(OldCreateIndexContext.class, 0);
}
public CreateIndexContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createIndex;
}
}
public final CreateIndexContext createIndex() throws RecognitionException {
CreateIndexContext _localctx = new CreateIndexContext(_ctx, getState());
enterRule(_localctx, 342, RULE_createIndex);
try {
setState(2332);
_errHandler.sync(this);
switch (_input.LA(1)) {
case BTREE:
enterOuterAlt(_localctx, 1);
{
setState(2305);
match(BTREE);
setState(2306);
match(INDEX);
setState(2307);
createIndex_();
}
break;
case RANGE:
enterOuterAlt(_localctx, 2);
{
setState(2308);
match(RANGE);
setState(2309);
match(INDEX);
setState(2310);
createIndex_();
}
break;
case TEXT:
enterOuterAlt(_localctx, 3);
{
setState(2311);
match(TEXT);
setState(2312);
match(INDEX);
setState(2313);
createIndex_();
}
break;
case POINT:
enterOuterAlt(_localctx, 4);
{
setState(2314);
match(POINT);
setState(2315);
match(INDEX);
setState(2316);
createIndex_();
}
break;
case VECTOR:
enterOuterAlt(_localctx, 5);
{
setState(2317);
match(VECTOR);
setState(2318);
match(INDEX);
setState(2319);
createIndex_();
}
break;
case LOOKUP:
enterOuterAlt(_localctx, 6);
{
setState(2320);
match(LOOKUP);
setState(2321);
match(INDEX);
setState(2322);
createLookupIndex();
}
break;
case FULLTEXT:
enterOuterAlt(_localctx, 7);
{
setState(2323);
match(FULLTEXT);
setState(2324);
match(INDEX);
setState(2325);
createFulltextIndex();
}
break;
case INDEX:
enterOuterAlt(_localctx, 8);
{
setState(2326);
match(INDEX);
setState(2330);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 268, _ctx)) {
case 1:
{
setState(2327);
match(ON);
setState(2328);
oldCreateIndex();
}
break;
case 2:
{
setState(2329);
createIndex_();
}
break;
}
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class OldCreateIndexContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public LabelTypeContext labelType() {
return getRuleContext(LabelTypeContext.class, 0);
}
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public NonEmptyNameListContext nonEmptyNameList() {
return getRuleContext(NonEmptyNameListContext.class, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public OldCreateIndexContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_oldCreateIndex;
}
}
public final OldCreateIndexContext oldCreateIndex() throws RecognitionException {
OldCreateIndexContext _localctx = new OldCreateIndexContext(_ctx, getState());
enterRule(_localctx, 344, RULE_oldCreateIndex);
try {
enterOuterAlt(_localctx, 1);
{
setState(2334);
labelType();
setState(2335);
match(LPAREN);
setState(2336);
nonEmptyNameList();
setState(2337);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateIndex_Context extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode FOR() {
return getToken(CypherParser.FOR, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public PropertyListContext propertyList() {
return getRuleContext(PropertyListContext.class, 0);
}
public CommandNodePatternContext commandNodePattern() {
return getRuleContext(CommandNodePatternContext.class, 0);
}
public CommandRelPatternContext commandRelPattern() {
return getRuleContext(CommandRelPatternContext.class, 0);
}
public SymbolicNameOrStringParameterContext symbolicNameOrStringParameter() {
return getRuleContext(SymbolicNameOrStringParameterContext.class, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public CommandOptionsContext commandOptions() {
return getRuleContext(CommandOptionsContext.class, 0);
}
public CreateIndex_Context(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createIndex_;
}
}
public final CreateIndex_Context createIndex_() throws RecognitionException {
CreateIndex_Context _localctx = new CreateIndex_Context(_ctx, getState());
enterRule(_localctx, 346, RULE_createIndex_);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2340);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 270, _ctx)) {
case 1:
{
setState(2339);
symbolicNameOrStringParameter();
}
break;
}
setState(2345);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(2342);
match(IF);
setState(2343);
match(NOT);
setState(2344);
match(EXISTS);
}
}
setState(2347);
match(FOR);
setState(2350);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 272, _ctx)) {
case 1:
{
setState(2348);
commandNodePattern();
}
break;
case 2:
{
setState(2349);
commandRelPattern();
}
break;
}
setState(2352);
match(ON);
setState(2353);
propertyList();
setState(2355);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == OPTIONS) {
{
setState(2354);
commandOptions();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateFulltextIndexContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode FOR() {
return getToken(CypherParser.FOR, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public TerminalNode EACH() {
return getToken(CypherParser.EACH, 0);
}
public TerminalNode LBRACKET() {
return getToken(CypherParser.LBRACKET, 0);
}
public List variable() {
return getRuleContexts(VariableContext.class);
}
public VariableContext variable(int i) {
return getRuleContext(VariableContext.class, i);
}
public List property() {
return getRuleContexts(PropertyContext.class);
}
public PropertyContext property(int i) {
return getRuleContext(PropertyContext.class, i);
}
public TerminalNode RBRACKET() {
return getToken(CypherParser.RBRACKET, 0);
}
public FulltextNodePatternContext fulltextNodePattern() {
return getRuleContext(FulltextNodePatternContext.class, 0);
}
public FulltextRelPatternContext fulltextRelPattern() {
return getRuleContext(FulltextRelPatternContext.class, 0);
}
public SymbolicNameOrStringParameterContext symbolicNameOrStringParameter() {
return getRuleContext(SymbolicNameOrStringParameterContext.class, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public CommandOptionsContext commandOptions() {
return getRuleContext(CommandOptionsContext.class, 0);
}
public CreateFulltextIndexContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createFulltextIndex;
}
}
public final CreateFulltextIndexContext createFulltextIndex() throws RecognitionException {
CreateFulltextIndexContext _localctx = new CreateFulltextIndexContext(_ctx, getState());
enterRule(_localctx, 348, RULE_createFulltextIndex);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2358);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 274, _ctx)) {
case 1:
{
setState(2357);
symbolicNameOrStringParameter();
}
break;
}
setState(2363);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(2360);
match(IF);
setState(2361);
match(NOT);
setState(2362);
match(EXISTS);
}
}
setState(2365);
match(FOR);
setState(2368);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 276, _ctx)) {
case 1:
{
setState(2366);
fulltextNodePattern();
}
break;
case 2:
{
setState(2367);
fulltextRelPattern();
}
break;
}
setState(2370);
match(ON);
setState(2371);
match(EACH);
setState(2372);
match(LBRACKET);
setState(2373);
variable();
setState(2374);
property();
setState(2381);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(2375);
match(COMMA);
setState(2376);
variable();
setState(2377);
property();
}
}
setState(2383);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2384);
match(RBRACKET);
setState(2386);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == OPTIONS) {
{
setState(2385);
commandOptions();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FulltextNodePatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public TerminalNode COLON() {
return getToken(CypherParser.COLON, 0);
}
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class, i);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public List BAR() {
return getTokens(CypherParser.BAR);
}
public TerminalNode BAR(int i) {
return getToken(CypherParser.BAR, i);
}
public FulltextNodePatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_fulltextNodePattern;
}
}
public final FulltextNodePatternContext fulltextNodePattern() throws RecognitionException {
FulltextNodePatternContext _localctx = new FulltextNodePatternContext(_ctx, getState());
enterRule(_localctx, 350, RULE_fulltextNodePattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2388);
match(LPAREN);
setState(2389);
variable();
setState(2390);
match(COLON);
setState(2391);
symbolicNameString();
setState(2396);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == BAR) {
{
{
setState(2392);
match(BAR);
setState(2393);
symbolicNameString();
}
}
setState(2398);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2399);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FulltextRelPatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List LPAREN() {
return getTokens(CypherParser.LPAREN);
}
public TerminalNode LPAREN(int i) {
return getToken(CypherParser.LPAREN, i);
}
public List RPAREN() {
return getTokens(CypherParser.RPAREN);
}
public TerminalNode RPAREN(int i) {
return getToken(CypherParser.RPAREN, i);
}
public List arrowLine() {
return getRuleContexts(ArrowLineContext.class);
}
public ArrowLineContext arrowLine(int i) {
return getRuleContext(ArrowLineContext.class, i);
}
public TerminalNode LBRACKET() {
return getToken(CypherParser.LBRACKET, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public TerminalNode COLON() {
return getToken(CypherParser.COLON, 0);
}
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class, i);
}
public TerminalNode RBRACKET() {
return getToken(CypherParser.RBRACKET, 0);
}
public LeftArrowContext leftArrow() {
return getRuleContext(LeftArrowContext.class, 0);
}
public List BAR() {
return getTokens(CypherParser.BAR);
}
public TerminalNode BAR(int i) {
return getToken(CypherParser.BAR, i);
}
public RightArrowContext rightArrow() {
return getRuleContext(RightArrowContext.class, 0);
}
public FulltextRelPatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_fulltextRelPattern;
}
}
public final FulltextRelPatternContext fulltextRelPattern() throws RecognitionException {
FulltextRelPatternContext _localctx = new FulltextRelPatternContext(_ctx, getState());
enterRule(_localctx, 352, RULE_fulltextRelPattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2401);
match(LPAREN);
setState(2402);
match(RPAREN);
setState(2404);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == LT || _la == ARROW_LEFT_HEAD) {
{
setState(2403);
leftArrow();
}
}
setState(2406);
arrowLine();
setState(2407);
match(LBRACKET);
setState(2408);
variable();
setState(2409);
match(COLON);
setState(2410);
symbolicNameString();
setState(2415);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == BAR) {
{
{
setState(2411);
match(BAR);
setState(2412);
symbolicNameString();
}
}
setState(2417);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2418);
match(RBRACKET);
setState(2419);
arrowLine();
setState(2421);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == GT || _la == ARROW_RIGHT_HEAD) {
{
setState(2420);
rightArrow();
}
}
setState(2423);
match(LPAREN);
setState(2424);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateLookupIndexContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode FOR() {
return getToken(CypherParser.FOR, 0);
}
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public LookupIndexNodePatternContext lookupIndexNodePattern() {
return getRuleContext(LookupIndexNodePatternContext.class, 0);
}
public LookupIndexRelPatternContext lookupIndexRelPattern() {
return getRuleContext(LookupIndexRelPatternContext.class, 0);
}
public SymbolicNameOrStringParameterContext symbolicNameOrStringParameter() {
return getRuleContext(SymbolicNameOrStringParameterContext.class, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public CommandOptionsContext commandOptions() {
return getRuleContext(CommandOptionsContext.class, 0);
}
public CreateLookupIndexContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createLookupIndex;
}
}
public final CreateLookupIndexContext createLookupIndex() throws RecognitionException {
CreateLookupIndexContext _localctx = new CreateLookupIndexContext(_ctx, getState());
enterRule(_localctx, 354, RULE_createLookupIndex);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2427);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 283, _ctx)) {
case 1:
{
setState(2426);
symbolicNameOrStringParameter();
}
break;
}
setState(2432);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(2429);
match(IF);
setState(2430);
match(NOT);
setState(2431);
match(EXISTS);
}
}
setState(2434);
match(FOR);
setState(2437);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 285, _ctx)) {
case 1:
{
setState(2435);
lookupIndexNodePattern();
}
break;
case 2:
{
setState(2436);
lookupIndexRelPattern();
}
break;
}
setState(2439);
symbolicNameString();
setState(2440);
match(LPAREN);
setState(2441);
variable();
setState(2442);
match(RPAREN);
setState(2444);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == OPTIONS) {
{
setState(2443);
commandOptions();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LookupIndexNodePatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public TerminalNode EACH() {
return getToken(CypherParser.EACH, 0);
}
public LookupIndexNodePatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_lookupIndexNodePattern;
}
}
public final LookupIndexNodePatternContext lookupIndexNodePattern() throws RecognitionException {
LookupIndexNodePatternContext _localctx = new LookupIndexNodePatternContext(_ctx, getState());
enterRule(_localctx, 356, RULE_lookupIndexNodePattern);
try {
enterOuterAlt(_localctx, 1);
{
setState(2446);
match(LPAREN);
setState(2447);
variable();
setState(2448);
match(RPAREN);
setState(2449);
match(ON);
setState(2450);
match(EACH);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LookupIndexRelPatternContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List LPAREN() {
return getTokens(CypherParser.LPAREN);
}
public TerminalNode LPAREN(int i) {
return getToken(CypherParser.LPAREN, i);
}
public List RPAREN() {
return getTokens(CypherParser.RPAREN);
}
public TerminalNode RPAREN(int i) {
return getToken(CypherParser.RPAREN, i);
}
public List arrowLine() {
return getRuleContexts(ArrowLineContext.class);
}
public ArrowLineContext arrowLine(int i) {
return getRuleContext(ArrowLineContext.class, i);
}
public TerminalNode LBRACKET() {
return getToken(CypherParser.LBRACKET, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public TerminalNode RBRACKET() {
return getToken(CypherParser.RBRACKET, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public LeftArrowContext leftArrow() {
return getRuleContext(LeftArrowContext.class, 0);
}
public RightArrowContext rightArrow() {
return getRuleContext(RightArrowContext.class, 0);
}
public TerminalNode EACH() {
return getToken(CypherParser.EACH, 0);
}
public LookupIndexRelPatternContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_lookupIndexRelPattern;
}
}
public final LookupIndexRelPatternContext lookupIndexRelPattern() throws RecognitionException {
LookupIndexRelPatternContext _localctx = new LookupIndexRelPatternContext(_ctx, getState());
enterRule(_localctx, 358, RULE_lookupIndexRelPattern);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2452);
match(LPAREN);
setState(2453);
match(RPAREN);
setState(2455);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == LT || _la == ARROW_LEFT_HEAD) {
{
setState(2454);
leftArrow();
}
}
setState(2457);
arrowLine();
setState(2458);
match(LBRACKET);
setState(2459);
variable();
setState(2460);
match(RBRACKET);
setState(2461);
arrowLine();
setState(2463);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == GT || _la == ARROW_RIGHT_HEAD) {
{
setState(2462);
rightArrow();
}
}
setState(2465);
match(LPAREN);
setState(2466);
match(RPAREN);
setState(2467);
match(ON);
setState(2469);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 289, _ctx)) {
case 1:
{
setState(2468);
match(EACH);
}
break;
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropIndexContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode INDEX() {
return getToken(CypherParser.INDEX, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public LabelTypeContext labelType() {
return getRuleContext(LabelTypeContext.class, 0);
}
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public NonEmptyNameListContext nonEmptyNameList() {
return getRuleContext(NonEmptyNameListContext.class, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public SymbolicNameOrStringParameterContext symbolicNameOrStringParameter() {
return getRuleContext(SymbolicNameOrStringParameterContext.class, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public DropIndexContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_dropIndex;
}
}
public final DropIndexContext dropIndex() throws RecognitionException {
DropIndexContext _localctx = new DropIndexContext(_ctx, getState());
enterRule(_localctx, 360, RULE_dropIndex);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2471);
match(INDEX);
setState(2483);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 291, _ctx)) {
case 1:
{
setState(2472);
match(ON);
setState(2473);
labelType();
setState(2474);
match(LPAREN);
setState(2475);
nonEmptyNameList();
setState(2476);
match(RPAREN);
}
break;
case 2:
{
setState(2478);
symbolicNameOrStringParameter();
setState(2481);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(2479);
match(IF);
setState(2480);
match(EXISTS);
}
}
}
break;
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PropertyListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List variable() {
return getRuleContexts(VariableContext.class);
}
public VariableContext variable(int i) {
return getRuleContext(VariableContext.class, i);
}
public List property() {
return getRuleContexts(PropertyContext.class);
}
public PropertyContext property(int i) {
return getRuleContext(PropertyContext.class, i);
}
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public PropertyListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_propertyList;
}
}
public final PropertyListContext propertyList() throws RecognitionException {
PropertyListContext _localctx = new PropertyListContext(_ctx, getState());
enterRule(_localctx, 362, RULE_propertyList);
int _la;
try {
setState(2502);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 1);
{
setState(2485);
variable();
setState(2486);
property();
}
break;
case LPAREN:
enterOuterAlt(_localctx, 2);
{
setState(2488);
match(LPAREN);
setState(2489);
variable();
setState(2490);
property();
setState(2497);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(2491);
match(COMMA);
setState(2492);
variable();
setState(2493);
property();
}
}
setState(2499);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(2500);
match(RPAREN);
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class EnableServerCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ENABLE() {
return getToken(CypherParser.ENABLE, 0);
}
public TerminalNode SERVER() {
return getToken(CypherParser.SERVER, 0);
}
public StringOrParameterContext stringOrParameter() {
return getRuleContext(StringOrParameterContext.class, 0);
}
public CommandOptionsContext commandOptions() {
return getRuleContext(CommandOptionsContext.class, 0);
}
public EnableServerCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_enableServerCommand;
}
}
public final EnableServerCommandContext enableServerCommand() throws RecognitionException {
EnableServerCommandContext _localctx = new EnableServerCommandContext(_ctx, getState());
enterRule(_localctx, 364, RULE_enableServerCommand);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2504);
match(ENABLE);
setState(2505);
match(SERVER);
setState(2506);
stringOrParameter();
setState(2508);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == OPTIONS) {
{
setState(2507);
commandOptions();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterServerContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SERVER() {
return getToken(CypherParser.SERVER, 0);
}
public StringOrParameterContext stringOrParameter() {
return getRuleContext(StringOrParameterContext.class, 0);
}
public TerminalNode SET() {
return getToken(CypherParser.SET, 0);
}
public CommandOptionsContext commandOptions() {
return getRuleContext(CommandOptionsContext.class, 0);
}
public AlterServerContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_alterServer;
}
}
public final AlterServerContext alterServer() throws RecognitionException {
AlterServerContext _localctx = new AlterServerContext(_ctx, getState());
enterRule(_localctx, 366, RULE_alterServer);
try {
enterOuterAlt(_localctx, 1);
{
setState(2510);
match(SERVER);
setState(2511);
stringOrParameter();
setState(2512);
match(SET);
setState(2513);
commandOptions();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RenameServerContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SERVER() {
return getToken(CypherParser.SERVER, 0);
}
public List stringOrParameter() {
return getRuleContexts(StringOrParameterContext.class);
}
public StringOrParameterContext stringOrParameter(int i) {
return getRuleContext(StringOrParameterContext.class, i);
}
public TerminalNode TO() {
return getToken(CypherParser.TO, 0);
}
public RenameServerContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_renameServer;
}
}
public final RenameServerContext renameServer() throws RecognitionException {
RenameServerContext _localctx = new RenameServerContext(_ctx, getState());
enterRule(_localctx, 368, RULE_renameServer);
try {
enterOuterAlt(_localctx, 1);
{
setState(2515);
match(SERVER);
setState(2516);
stringOrParameter();
setState(2517);
match(TO);
setState(2518);
stringOrParameter();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropServerContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SERVER() {
return getToken(CypherParser.SERVER, 0);
}
public StringOrParameterContext stringOrParameter() {
return getRuleContext(StringOrParameterContext.class, 0);
}
public DropServerContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_dropServer;
}
}
public final DropServerContext dropServer() throws RecognitionException {
DropServerContext _localctx = new DropServerContext(_ctx, getState());
enterRule(_localctx, 370, RULE_dropServer);
try {
enterOuterAlt(_localctx, 1);
{
setState(2520);
match(SERVER);
setState(2521);
stringOrParameter();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowServersContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SERVER() {
return getToken(CypherParser.SERVER, 0);
}
public TerminalNode SERVERS() {
return getToken(CypherParser.SERVERS, 0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class, 0);
}
public ShowServersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showServers;
}
}
public final ShowServersContext showServers() throws RecognitionException {
ShowServersContext _localctx = new ShowServersContext(_ctx, getState());
enterRule(_localctx, 372, RULE_showServers);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2523);
_la = _input.LA(1);
if (!(_la == SERVER || _la == SERVERS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2525);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(2524);
showCommandYield();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AllocationCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public DeallocateDatabaseFromServersContext deallocateDatabaseFromServers() {
return getRuleContext(DeallocateDatabaseFromServersContext.class, 0);
}
public ReallocateDatabasesContext reallocateDatabases() {
return getRuleContext(ReallocateDatabasesContext.class, 0);
}
public TerminalNode DRYRUN() {
return getToken(CypherParser.DRYRUN, 0);
}
public AllocationCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_allocationCommand;
}
}
public final AllocationCommandContext allocationCommand() throws RecognitionException {
AllocationCommandContext _localctx = new AllocationCommandContext(_ctx, getState());
enterRule(_localctx, 374, RULE_allocationCommand);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2528);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == DRYRUN) {
{
setState(2527);
match(DRYRUN);
}
}
setState(2532);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DEALLOCATE:
{
setState(2530);
deallocateDatabaseFromServers();
}
break;
case REALLOCATE:
{
setState(2531);
reallocateDatabases();
}
break;
default:
throw new NoViableAltException(this);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DeallocateDatabaseFromServersContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DEALLOCATE() {
return getToken(CypherParser.DEALLOCATE, 0);
}
public TerminalNode FROM() {
return getToken(CypherParser.FROM, 0);
}
public List stringOrParameter() {
return getRuleContexts(StringOrParameterContext.class);
}
public StringOrParameterContext stringOrParameter(int i) {
return getRuleContext(StringOrParameterContext.class, i);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public TerminalNode DATABASES() {
return getToken(CypherParser.DATABASES, 0);
}
public TerminalNode SERVER() {
return getToken(CypherParser.SERVER, 0);
}
public TerminalNode SERVERS() {
return getToken(CypherParser.SERVERS, 0);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public DeallocateDatabaseFromServersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_deallocateDatabaseFromServers;
}
}
public final DeallocateDatabaseFromServersContext deallocateDatabaseFromServers() throws RecognitionException {
DeallocateDatabaseFromServersContext _localctx = new DeallocateDatabaseFromServersContext(_ctx, getState());
enterRule(_localctx, 376, RULE_deallocateDatabaseFromServers);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2534);
match(DEALLOCATE);
setState(2535);
_la = _input.LA(1);
if (!(_la == DATABASE || _la == DATABASES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2536);
match(FROM);
setState(2537);
_la = _input.LA(1);
if (!(_la == SERVER || _la == SERVERS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2538);
stringOrParameter();
setState(2543);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(2539);
match(COMMA);
setState(2540);
stringOrParameter();
}
}
setState(2545);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ReallocateDatabasesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode REALLOCATE() {
return getToken(CypherParser.REALLOCATE, 0);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public TerminalNode DATABASES() {
return getToken(CypherParser.DATABASES, 0);
}
public ReallocateDatabasesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_reallocateDatabases;
}
}
public final ReallocateDatabasesContext reallocateDatabases() throws RecognitionException {
ReallocateDatabasesContext _localctx = new ReallocateDatabasesContext(_ctx, getState());
enterRule(_localctx, 378, RULE_reallocateDatabases);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2546);
match(REALLOCATE);
setState(2547);
_la = _input.LA(1);
if (!(_la == DATABASE || _la == DATABASES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateRoleContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ROLE() {
return getToken(CypherParser.ROLE, 0);
}
public List commandNameExpression() {
return getRuleContexts(CommandNameExpressionContext.class);
}
public CommandNameExpressionContext commandNameExpression(int i) {
return getRuleContext(CommandNameExpressionContext.class, i);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public TerminalNode AS() {
return getToken(CypherParser.AS, 0);
}
public TerminalNode COPY() {
return getToken(CypherParser.COPY, 0);
}
public TerminalNode OF() {
return getToken(CypherParser.OF, 0);
}
public CreateRoleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createRole;
}
}
public final CreateRoleContext createRole() throws RecognitionException {
CreateRoleContext _localctx = new CreateRoleContext(_ctx, getState());
enterRule(_localctx, 380, RULE_createRole);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2549);
match(ROLE);
setState(2550);
commandNameExpression();
setState(2554);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(2551);
match(IF);
setState(2552);
match(NOT);
setState(2553);
match(EXISTS);
}
}
setState(2560);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == AS) {
{
setState(2556);
match(AS);
setState(2557);
match(COPY);
setState(2558);
match(OF);
setState(2559);
commandNameExpression();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropRoleContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ROLE() {
return getToken(CypherParser.ROLE, 0);
}
public CommandNameExpressionContext commandNameExpression() {
return getRuleContext(CommandNameExpressionContext.class, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public DropRoleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_dropRole;
}
}
public final DropRoleContext dropRole() throws RecognitionException {
DropRoleContext _localctx = new DropRoleContext(_ctx, getState());
enterRule(_localctx, 382, RULE_dropRole);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2562);
match(ROLE);
setState(2563);
commandNameExpression();
setState(2566);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(2564);
match(IF);
setState(2565);
match(EXISTS);
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RenameRoleContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ROLE() {
return getToken(CypherParser.ROLE, 0);
}
public List commandNameExpression() {
return getRuleContexts(CommandNameExpressionContext.class);
}
public CommandNameExpressionContext commandNameExpression(int i) {
return getRuleContext(CommandNameExpressionContext.class, i);
}
public TerminalNode TO() {
return getToken(CypherParser.TO, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public RenameRoleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_renameRole;
}
}
public final RenameRoleContext renameRole() throws RecognitionException {
RenameRoleContext _localctx = new RenameRoleContext(_ctx, getState());
enterRule(_localctx, 384, RULE_renameRole);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2568);
match(ROLE);
setState(2569);
commandNameExpression();
setState(2572);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(2570);
match(IF);
setState(2571);
match(EXISTS);
}
}
setState(2574);
match(TO);
setState(2575);
commandNameExpression();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowRolesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public RoleTokenContext roleToken() {
return getRuleContext(RoleTokenContext.class, 0);
}
public TerminalNode WITH() {
return getToken(CypherParser.WITH, 0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class, 0);
}
public TerminalNode ALL() {
return getToken(CypherParser.ALL, 0);
}
public TerminalNode POPULATED() {
return getToken(CypherParser.POPULATED, 0);
}
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public TerminalNode USERS() {
return getToken(CypherParser.USERS, 0);
}
public ShowRolesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showRoles;
}
}
public final ShowRolesContext showRoles() throws RecognitionException {
ShowRolesContext _localctx = new ShowRolesContext(_ctx, getState());
enterRule(_localctx, 386, RULE_showRoles);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2578);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == ALL || _la == POPULATED) {
{
setState(2577);
_la = _input.LA(1);
if (!(_la == ALL || _la == POPULATED)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(2580);
roleToken();
setState(2583);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WITH) {
{
setState(2581);
match(WITH);
setState(2582);
_la = _input.LA(1);
if (!(_la == USER || _la == USERS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(2586);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(2585);
showCommandYield();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RoleTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ROLES() {
return getToken(CypherParser.ROLES, 0);
}
public TerminalNode ROLE() {
return getToken(CypherParser.ROLE, 0);
}
public RoleTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_roleToken;
}
}
public final RoleTokenContext roleToken() throws RecognitionException {
RoleTokenContext _localctx = new RoleTokenContext(_ctx, getState());
enterRule(_localctx, 388, RULE_roleToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2588);
_la = _input.LA(1);
if (!(_la == ROLE || _la == ROLES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateUserContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public CommandNameExpressionContext commandNameExpression() {
return getRuleContext(CommandNameExpressionContext.class, 0);
}
public List SET() {
return getTokens(CypherParser.SET);
}
public TerminalNode SET(int i) {
return getToken(CypherParser.SET, i);
}
public PasswordContext password() {
return getRuleContext(PasswordContext.class, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public List PASSWORD() {
return getTokens(CypherParser.PASSWORD);
}
public TerminalNode PASSWORD(int i) {
return getToken(CypherParser.PASSWORD, i);
}
public List passwordChangeRequired() {
return getRuleContexts(PasswordChangeRequiredContext.class);
}
public PasswordChangeRequiredContext passwordChangeRequired(int i) {
return getRuleContext(PasswordChangeRequiredContext.class, i);
}
public List userStatus() {
return getRuleContexts(UserStatusContext.class);
}
public UserStatusContext userStatus(int i) {
return getRuleContext(UserStatusContext.class, i);
}
public List homeDatabase() {
return getRuleContexts(HomeDatabaseContext.class);
}
public HomeDatabaseContext homeDatabase(int i) {
return getRuleContext(HomeDatabaseContext.class, i);
}
public CreateUserContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createUser;
}
}
public final CreateUserContext createUser() throws RecognitionException {
CreateUserContext _localctx = new CreateUserContext(_ctx, getState());
enterRule(_localctx, 390, RULE_createUser);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2590);
match(USER);
setState(2591);
commandNameExpression();
setState(2595);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(2592);
match(IF);
setState(2593);
match(NOT);
setState(2594);
match(EXISTS);
}
}
setState(2597);
match(SET);
setState(2598);
password();
setState(2608);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == SET) {
{
{
setState(2599);
match(SET);
setState(2604);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PASSWORD:
{
setState(2600);
match(PASSWORD);
setState(2601);
passwordChangeRequired();
}
break;
case STATUS:
{
setState(2602);
userStatus();
}
break;
case HOME:
{
setState(2603);
homeDatabase();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
setState(2610);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropUserContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public CommandNameExpressionContext commandNameExpression() {
return getRuleContext(CommandNameExpressionContext.class, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public DropUserContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_dropUser;
}
}
public final DropUserContext dropUser() throws RecognitionException {
DropUserContext _localctx = new DropUserContext(_ctx, getState());
enterRule(_localctx, 392, RULE_dropUser);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2611);
match(USER);
setState(2612);
commandNameExpression();
setState(2615);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(2613);
match(IF);
setState(2614);
match(EXISTS);
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RenameUserContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public List commandNameExpression() {
return getRuleContexts(CommandNameExpressionContext.class);
}
public CommandNameExpressionContext commandNameExpression(int i) {
return getRuleContext(CommandNameExpressionContext.class, i);
}
public TerminalNode TO() {
return getToken(CypherParser.TO, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public RenameUserContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_renameUser;
}
}
public final RenameUserContext renameUser() throws RecognitionException {
RenameUserContext _localctx = new RenameUserContext(_ctx, getState());
enterRule(_localctx, 394, RULE_renameUser);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2617);
match(USER);
setState(2618);
commandNameExpression();
setState(2621);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(2619);
match(IF);
setState(2620);
match(EXISTS);
}
}
setState(2623);
match(TO);
setState(2624);
commandNameExpression();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterCurrentUserContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CURRENT() {
return getToken(CypherParser.CURRENT, 0);
}
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public TerminalNode SET() {
return getToken(CypherParser.SET, 0);
}
public TerminalNode PASSWORD() {
return getToken(CypherParser.PASSWORD, 0);
}
public TerminalNode FROM() {
return getToken(CypherParser.FROM, 0);
}
public List passwordExpression() {
return getRuleContexts(PasswordExpressionContext.class);
}
public PasswordExpressionContext passwordExpression(int i) {
return getRuleContext(PasswordExpressionContext.class, i);
}
public TerminalNode TO() {
return getToken(CypherParser.TO, 0);
}
public AlterCurrentUserContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_alterCurrentUser;
}
}
public final AlterCurrentUserContext alterCurrentUser() throws RecognitionException {
AlterCurrentUserContext _localctx = new AlterCurrentUserContext(_ctx, getState());
enterRule(_localctx, 396, RULE_alterCurrentUser);
try {
enterOuterAlt(_localctx, 1);
{
setState(2626);
match(CURRENT);
setState(2627);
match(USER);
setState(2628);
match(SET);
setState(2629);
match(PASSWORD);
setState(2630);
match(FROM);
setState(2631);
passwordExpression();
setState(2632);
match(TO);
setState(2633);
passwordExpression();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterUserContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public CommandNameExpressionContext commandNameExpression() {
return getRuleContext(CommandNameExpressionContext.class, 0);
}
public TerminalNode REMOVE() {
return getToken(CypherParser.REMOVE, 0);
}
public TerminalNode HOME() {
return getToken(CypherParser.HOME, 0);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public List SET() {
return getTokens(CypherParser.SET);
}
public TerminalNode SET(int i) {
return getToken(CypherParser.SET, i);
}
public List password() {
return getRuleContexts(PasswordContext.class);
}
public PasswordContext password(int i) {
return getRuleContext(PasswordContext.class, i);
}
public List PASSWORD() {
return getTokens(CypherParser.PASSWORD);
}
public TerminalNode PASSWORD(int i) {
return getToken(CypherParser.PASSWORD, i);
}
public List passwordChangeRequired() {
return getRuleContexts(PasswordChangeRequiredContext.class);
}
public PasswordChangeRequiredContext passwordChangeRequired(int i) {
return getRuleContext(PasswordChangeRequiredContext.class, i);
}
public List userStatus() {
return getRuleContexts(UserStatusContext.class);
}
public UserStatusContext userStatus(int i) {
return getRuleContext(UserStatusContext.class, i);
}
public List homeDatabase() {
return getRuleContexts(HomeDatabaseContext.class);
}
public HomeDatabaseContext homeDatabase(int i) {
return getRuleContext(HomeDatabaseContext.class, i);
}
public AlterUserContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_alterUser;
}
}
public final AlterUserContext alterUser() throws RecognitionException {
AlterUserContext _localctx = new AlterUserContext(_ctx, getState());
enterRule(_localctx, 398, RULE_alterUser);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2635);
match(USER);
setState(2636);
commandNameExpression();
setState(2639);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(2637);
match(IF);
setState(2638);
match(EXISTS);
}
}
setState(2656);
_errHandler.sync(this);
switch (_input.LA(1)) {
case SET:
{
setState(2649);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(2641);
match(SET);
setState(2647);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 312, _ctx)) {
case 1:
{
setState(2642);
password();
}
break;
case 2:
{
setState(2643);
match(PASSWORD);
setState(2644);
passwordChangeRequired();
}
break;
case 3:
{
setState(2645);
userStatus();
}
break;
case 4:
{
setState(2646);
homeDatabase();
}
break;
}
}
}
setState(2651);
_errHandler.sync(this);
_la = _input.LA(1);
} while (_la == SET);
}
break;
case REMOVE:
{
setState(2653);
match(REMOVE);
setState(2654);
match(HOME);
setState(2655);
match(DATABASE);
}
break;
default:
throw new NoViableAltException(this);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PasswordContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PASSWORD() {
return getToken(CypherParser.PASSWORD, 0);
}
public PasswordExpressionContext passwordExpression() {
return getRuleContext(PasswordExpressionContext.class, 0);
}
public PasswordChangeRequiredContext passwordChangeRequired() {
return getRuleContext(PasswordChangeRequiredContext.class, 0);
}
public TerminalNode PLAINTEXT() {
return getToken(CypherParser.PLAINTEXT, 0);
}
public TerminalNode ENCRYPTED() {
return getToken(CypherParser.ENCRYPTED, 0);
}
public PasswordContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_password;
}
}
public final PasswordContext password() throws RecognitionException {
PasswordContext _localctx = new PasswordContext(_ctx, getState());
enterRule(_localctx, 400, RULE_password);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2659);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == ENCRYPTED || _la == PLAINTEXT) {
{
setState(2658);
_la = _input.LA(1);
if (!(_la == ENCRYPTED || _la == PLAINTEXT)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(2661);
match(PASSWORD);
setState(2662);
passwordExpression();
setState(2664);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == CHANGE) {
{
setState(2663);
passwordChangeRequired();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PasswordExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class, 0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class, 0);
}
public PasswordExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_passwordExpression;
}
}
public final PasswordExpressionContext passwordExpression() throws RecognitionException {
PasswordExpressionContext _localctx = new PasswordExpressionContext(_ctx, getState());
enterRule(_localctx, 402, RULE_passwordExpression);
try {
setState(2668);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STRING_LITERAL1:
case STRING_LITERAL2:
enterOuterAlt(_localctx, 1);
{
setState(2666);
stringLiteral();
}
break;
case DOLLAR:
enterOuterAlt(_localctx, 2);
{
setState(2667);
parameter("STRING");
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PasswordChangeRequiredContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CHANGE() {
return getToken(CypherParser.CHANGE, 0);
}
public TerminalNode REQUIRED() {
return getToken(CypherParser.REQUIRED, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public PasswordChangeRequiredContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_passwordChangeRequired;
}
}
public final PasswordChangeRequiredContext passwordChangeRequired() throws RecognitionException {
PasswordChangeRequiredContext _localctx = new PasswordChangeRequiredContext(_ctx, getState());
enterRule(_localctx, 404, RULE_passwordChangeRequired);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2670);
match(CHANGE);
setState(2672);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == NOT) {
{
setState(2671);
match(NOT);
}
}
setState(2674);
match(REQUIRED);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UserStatusContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode STATUS() {
return getToken(CypherParser.STATUS, 0);
}
public TerminalNode SUSPENDED() {
return getToken(CypherParser.SUSPENDED, 0);
}
public TerminalNode ACTIVE() {
return getToken(CypherParser.ACTIVE, 0);
}
public UserStatusContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_userStatus;
}
}
public final UserStatusContext userStatus() throws RecognitionException {
UserStatusContext _localctx = new UserStatusContext(_ctx, getState());
enterRule(_localctx, 406, RULE_userStatus);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2676);
match(STATUS);
setState(2677);
_la = _input.LA(1);
if (!(_la == ACTIVE || _la == SUSPENDED)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class HomeDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode HOME() {
return getToken(CypherParser.HOME, 0);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class, 0);
}
public HomeDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_homeDatabase;
}
}
public final HomeDatabaseContext homeDatabase() throws RecognitionException {
HomeDatabaseContext _localctx = new HomeDatabaseContext(_ctx, getState());
enterRule(_localctx, 408, RULE_homeDatabase);
try {
enterOuterAlt(_localctx, 1);
{
setState(2679);
match(HOME);
setState(2680);
match(DATABASE);
setState(2681);
symbolicAliasNameOrParameter();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowUsersContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public TerminalNode USERS() {
return getToken(CypherParser.USERS, 0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class, 0);
}
public ShowUsersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showUsers;
}
}
public final ShowUsersContext showUsers() throws RecognitionException {
ShowUsersContext _localctx = new ShowUsersContext(_ctx, getState());
enterRule(_localctx, 410, RULE_showUsers);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2683);
_la = _input.LA(1);
if (!(_la == USER || _la == USERS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2685);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(2684);
showCommandYield();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowCurrentUserContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CURRENT() {
return getToken(CypherParser.CURRENT, 0);
}
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class, 0);
}
public ShowCurrentUserContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showCurrentUser;
}
}
public final ShowCurrentUserContext showCurrentUser() throws RecognitionException {
ShowCurrentUserContext _localctx = new ShowCurrentUserContext(_ctx, getState());
enterRule(_localctx, 412, RULE_showCurrentUser);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2687);
match(CURRENT);
setState(2688);
match(USER);
setState(2690);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(2689);
showCommandYield();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowPrivilegesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public PrivilegeTokenContext privilegeToken() {
return getRuleContext(PrivilegeTokenContext.class, 0);
}
public TerminalNode ALL() {
return getToken(CypherParser.ALL, 0);
}
public PrivilegeAsCommandContext privilegeAsCommand() {
return getRuleContext(PrivilegeAsCommandContext.class, 0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class, 0);
}
public ShowPrivilegesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showPrivileges;
}
}
public final ShowPrivilegesContext showPrivileges() throws RecognitionException {
ShowPrivilegesContext _localctx = new ShowPrivilegesContext(_ctx, getState());
enterRule(_localctx, 414, RULE_showPrivileges);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2693);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == ALL) {
{
setState(2692);
match(ALL);
}
}
setState(2695);
privilegeToken();
setState(2697);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == AS) {
{
setState(2696);
privilegeAsCommand();
}
}
setState(2700);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(2699);
showCommandYield();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowSupportedPrivilegesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SUPPORTED() {
return getToken(CypherParser.SUPPORTED, 0);
}
public PrivilegeTokenContext privilegeToken() {
return getRuleContext(PrivilegeTokenContext.class, 0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class, 0);
}
public ShowSupportedPrivilegesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showSupportedPrivileges;
}
}
public final ShowSupportedPrivilegesContext showSupportedPrivileges() throws RecognitionException {
ShowSupportedPrivilegesContext _localctx = new ShowSupportedPrivilegesContext(_ctx, getState());
enterRule(_localctx, 416, RULE_showSupportedPrivileges);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2702);
match(SUPPORTED);
setState(2703);
privilegeToken();
setState(2705);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(2704);
showCommandYield();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowRolePrivilegesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicNameOrStringParameterListContext symbolicNameOrStringParameterList() {
return getRuleContext(SymbolicNameOrStringParameterListContext.class, 0);
}
public PrivilegeTokenContext privilegeToken() {
return getRuleContext(PrivilegeTokenContext.class, 0);
}
public TerminalNode ROLE() {
return getToken(CypherParser.ROLE, 0);
}
public TerminalNode ROLES() {
return getToken(CypherParser.ROLES, 0);
}
public PrivilegeAsCommandContext privilegeAsCommand() {
return getRuleContext(PrivilegeAsCommandContext.class, 0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class, 0);
}
public ShowRolePrivilegesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showRolePrivileges;
}
}
public final ShowRolePrivilegesContext showRolePrivileges() throws RecognitionException {
ShowRolePrivilegesContext _localctx = new ShowRolePrivilegesContext(_ctx, getState());
enterRule(_localctx, 418, RULE_showRolePrivileges);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2707);
_la = _input.LA(1);
if (!(_la == ROLE || _la == ROLES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2708);
symbolicNameOrStringParameterList();
setState(2709);
privilegeToken();
setState(2711);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == AS) {
{
setState(2710);
privilegeAsCommand();
}
}
setState(2714);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(2713);
showCommandYield();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowUserPrivilegesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public PrivilegeTokenContext privilegeToken() {
return getRuleContext(PrivilegeTokenContext.class, 0);
}
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public TerminalNode USERS() {
return getToken(CypherParser.USERS, 0);
}
public SymbolicNameOrStringParameterListContext symbolicNameOrStringParameterList() {
return getRuleContext(SymbolicNameOrStringParameterListContext.class, 0);
}
public PrivilegeAsCommandContext privilegeAsCommand() {
return getRuleContext(PrivilegeAsCommandContext.class, 0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class, 0);
}
public ShowUserPrivilegesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showUserPrivileges;
}
}
public final ShowUserPrivilegesContext showUserPrivileges() throws RecognitionException {
ShowUserPrivilegesContext _localctx = new ShowUserPrivilegesContext(_ctx, getState());
enterRule(_localctx, 420, RULE_showUserPrivileges);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2716);
_la = _input.LA(1);
if (!(_la == USER || _la == USERS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2718);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 327, _ctx)) {
case 1:
{
setState(2717);
symbolicNameOrStringParameterList();
}
break;
}
setState(2720);
privilegeToken();
setState(2722);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == AS) {
{
setState(2721);
privilegeAsCommand();
}
}
setState(2725);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(2724);
showCommandYield();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PrivilegeAsCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode AS() {
return getToken(CypherParser.AS, 0);
}
public TerminalNode COMMAND() {
return getToken(CypherParser.COMMAND, 0);
}
public TerminalNode COMMANDS() {
return getToken(CypherParser.COMMANDS, 0);
}
public TerminalNode REVOKE() {
return getToken(CypherParser.REVOKE, 0);
}
public PrivilegeAsCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_privilegeAsCommand;
}
}
public final PrivilegeAsCommandContext privilegeAsCommand() throws RecognitionException {
PrivilegeAsCommandContext _localctx = new PrivilegeAsCommandContext(_ctx, getState());
enterRule(_localctx, 422, RULE_privilegeAsCommand);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2727);
match(AS);
setState(2729);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == REVOKE) {
{
setState(2728);
match(REVOKE);
}
}
setState(2731);
_la = _input.LA(1);
if (!(_la == COMMAND || _la == COMMANDS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PrivilegeTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PRIVILEGE() {
return getToken(CypherParser.PRIVILEGE, 0);
}
public TerminalNode PRIVILEGES() {
return getToken(CypherParser.PRIVILEGES, 0);
}
public PrivilegeTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_privilegeToken;
}
}
public final PrivilegeTokenContext privilegeToken() throws RecognitionException {
PrivilegeTokenContext _localctx = new PrivilegeTokenContext(_ctx, getState());
enterRule(_localctx, 424, RULE_privilegeToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2733);
_la = _input.LA(1);
if (!(_la == PRIVILEGE || _la == PRIVILEGES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GrantCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode GRANT() {
return getToken(CypherParser.GRANT, 0);
}
public PrivilegeContext privilege() {
return getRuleContext(PrivilegeContext.class, 0);
}
public TerminalNode TO() {
return getToken(CypherParser.TO, 0);
}
public SymbolicNameOrStringParameterListContext symbolicNameOrStringParameterList() {
return getRuleContext(SymbolicNameOrStringParameterListContext.class, 0);
}
public RoleTokenContext roleToken() {
return getRuleContext(RoleTokenContext.class, 0);
}
public GrantRoleContext grantRole() {
return getRuleContext(GrantRoleContext.class, 0);
}
public TerminalNode IMMUTABLE() {
return getToken(CypherParser.IMMUTABLE, 0);
}
public GrantCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_grantCommand;
}
}
public final GrantCommandContext grantCommand() throws RecognitionException {
GrantCommandContext _localctx = new GrantCommandContext(_ctx, getState());
enterRule(_localctx, 426, RULE_grantCommand);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2735);
match(GRANT);
setState(2746);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 332, _ctx)) {
case 1:
{
setState(2737);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IMMUTABLE) {
{
setState(2736);
match(IMMUTABLE);
}
}
setState(2739);
privilege();
setState(2740);
match(TO);
setState(2741);
symbolicNameOrStringParameterList();
}
break;
case 2:
{
setState(2743);
roleToken();
setState(2744);
grantRole();
}
break;
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GrantRoleContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List symbolicNameOrStringParameterList() {
return getRuleContexts(SymbolicNameOrStringParameterListContext.class);
}
public SymbolicNameOrStringParameterListContext symbolicNameOrStringParameterList(int i) {
return getRuleContext(SymbolicNameOrStringParameterListContext.class, i);
}
public TerminalNode TO() {
return getToken(CypherParser.TO, 0);
}
public GrantRoleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_grantRole;
}
}
public final GrantRoleContext grantRole() throws RecognitionException {
GrantRoleContext _localctx = new GrantRoleContext(_ctx, getState());
enterRule(_localctx, 428, RULE_grantRole);
try {
enterOuterAlt(_localctx, 1);
{
setState(2748);
symbolicNameOrStringParameterList();
setState(2749);
match(TO);
setState(2750);
symbolicNameOrStringParameterList();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DenyCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DENY() {
return getToken(CypherParser.DENY, 0);
}
public PrivilegeContext privilege() {
return getRuleContext(PrivilegeContext.class, 0);
}
public TerminalNode TO() {
return getToken(CypherParser.TO, 0);
}
public SymbolicNameOrStringParameterListContext symbolicNameOrStringParameterList() {
return getRuleContext(SymbolicNameOrStringParameterListContext.class, 0);
}
public TerminalNode IMMUTABLE() {
return getToken(CypherParser.IMMUTABLE, 0);
}
public DenyCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_denyCommand;
}
}
public final DenyCommandContext denyCommand() throws RecognitionException {
DenyCommandContext _localctx = new DenyCommandContext(_ctx, getState());
enterRule(_localctx, 430, RULE_denyCommand);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2752);
match(DENY);
setState(2754);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IMMUTABLE) {
{
setState(2753);
match(IMMUTABLE);
}
}
setState(2756);
privilege();
setState(2757);
match(TO);
setState(2758);
symbolicNameOrStringParameterList();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RevokeCommandContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode REVOKE() {
return getToken(CypherParser.REVOKE, 0);
}
public PrivilegeContext privilege() {
return getRuleContext(PrivilegeContext.class, 0);
}
public TerminalNode FROM() {
return getToken(CypherParser.FROM, 0);
}
public SymbolicNameOrStringParameterListContext symbolicNameOrStringParameterList() {
return getRuleContext(SymbolicNameOrStringParameterListContext.class, 0);
}
public RoleTokenContext roleToken() {
return getRuleContext(RoleTokenContext.class, 0);
}
public RevokeRoleContext revokeRole() {
return getRuleContext(RevokeRoleContext.class, 0);
}
public TerminalNode IMMUTABLE() {
return getToken(CypherParser.IMMUTABLE, 0);
}
public TerminalNode DENY() {
return getToken(CypherParser.DENY, 0);
}
public TerminalNode GRANT() {
return getToken(CypherParser.GRANT, 0);
}
public RevokeCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_revokeCommand;
}
}
public final RevokeCommandContext revokeCommand() throws RecognitionException {
RevokeCommandContext _localctx = new RevokeCommandContext(_ctx, getState());
enterRule(_localctx, 432, RULE_revokeCommand);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2760);
match(REVOKE);
setState(2774);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 336, _ctx)) {
case 1:
{
setState(2762);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == DENY || _la == GRANT) {
{
setState(2761);
_la = _input.LA(1);
if (!(_la == DENY || _la == GRANT)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(2765);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IMMUTABLE) {
{
setState(2764);
match(IMMUTABLE);
}
}
setState(2767);
privilege();
setState(2768);
match(FROM);
setState(2769);
symbolicNameOrStringParameterList();
}
break;
case 2:
{
setState(2771);
roleToken();
setState(2772);
revokeRole();
}
break;
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RevokeRoleContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List symbolicNameOrStringParameterList() {
return getRuleContexts(SymbolicNameOrStringParameterListContext.class);
}
public SymbolicNameOrStringParameterListContext symbolicNameOrStringParameterList(int i) {
return getRuleContext(SymbolicNameOrStringParameterListContext.class, i);
}
public TerminalNode FROM() {
return getToken(CypherParser.FROM, 0);
}
public RevokeRoleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_revokeRole;
}
}
public final RevokeRoleContext revokeRole() throws RecognitionException {
RevokeRoleContext _localctx = new RevokeRoleContext(_ctx, getState());
enterRule(_localctx, 434, RULE_revokeRole);
try {
enterOuterAlt(_localctx, 1);
{
setState(2776);
symbolicNameOrStringParameterList();
setState(2777);
match(FROM);
setState(2778);
symbolicNameOrStringParameterList();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public AllPrivilegeContext allPrivilege() {
return getRuleContext(AllPrivilegeContext.class, 0);
}
public CreatePrivilegeContext createPrivilege() {
return getRuleContext(CreatePrivilegeContext.class, 0);
}
public DatabasePrivilegeContext databasePrivilege() {
return getRuleContext(DatabasePrivilegeContext.class, 0);
}
public DbmsPrivilegeContext dbmsPrivilege() {
return getRuleContext(DbmsPrivilegeContext.class, 0);
}
public DropPrivilegeContext dropPrivilege() {
return getRuleContext(DropPrivilegeContext.class, 0);
}
public LoadPrivilegeContext loadPrivilege() {
return getRuleContext(LoadPrivilegeContext.class, 0);
}
public QualifiedGraphPrivilegesContext qualifiedGraphPrivileges() {
return getRuleContext(QualifiedGraphPrivilegesContext.class, 0);
}
public QualifiedGraphPrivilegesWithPropertyContext qualifiedGraphPrivilegesWithProperty() {
return getRuleContext(QualifiedGraphPrivilegesWithPropertyContext.class, 0);
}
public RemovePrivilegeContext removePrivilege() {
return getRuleContext(RemovePrivilegeContext.class, 0);
}
public SetPrivilegeContext setPrivilege() {
return getRuleContext(SetPrivilegeContext.class, 0);
}
public ShowPrivilegeContext showPrivilege() {
return getRuleContext(ShowPrivilegeContext.class, 0);
}
public WritePrivilegeContext writePrivilege() {
return getRuleContext(WritePrivilegeContext.class, 0);
}
public PrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_privilege;
}
}
public final PrivilegeContext privilege() throws RecognitionException {
PrivilegeContext _localctx = new PrivilegeContext(_ctx, getState());
enterRule(_localctx, 436, RULE_privilege);
try {
setState(2792);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALL:
enterOuterAlt(_localctx, 1);
{
setState(2780);
allPrivilege();
}
break;
case CREATE:
enterOuterAlt(_localctx, 2);
{
setState(2781);
createPrivilege();
}
break;
case ACCESS:
case CONSTRAINT:
case CONSTRAINTS:
case INDEX:
case INDEXES:
case NAME:
case START:
case STOP:
case TERMINATE:
case TRANSACTION:
enterOuterAlt(_localctx, 3);
{
setState(2782);
databasePrivilege();
}
break;
case ALIAS:
case ALTER:
case ASSIGN:
case COMPOSITE:
case DATABASE:
case EXECUTE:
case IMPERSONATE:
case PRIVILEGE:
case RENAME:
case ROLE:
case SERVER:
case USER:
enterOuterAlt(_localctx, 4);
{
setState(2783);
dbmsPrivilege();
}
break;
case DROP:
enterOuterAlt(_localctx, 5);
{
setState(2784);
dropPrivilege();
}
break;
case LOAD:
enterOuterAlt(_localctx, 6);
{
setState(2785);
loadPrivilege();
}
break;
case DELETE:
case MERGE:
enterOuterAlt(_localctx, 7);
{
setState(2786);
qualifiedGraphPrivileges();
}
break;
case MATCH:
case READ:
case TRAVERSE:
enterOuterAlt(_localctx, 8);
{
setState(2787);
qualifiedGraphPrivilegesWithProperty();
}
break;
case REMOVE:
enterOuterAlt(_localctx, 9);
{
setState(2788);
removePrivilege();
}
break;
case SET:
enterOuterAlt(_localctx, 10);
{
setState(2789);
setPrivilege();
}
break;
case SHOW:
enterOuterAlt(_localctx, 11);
{
setState(2790);
showPrivilege();
}
break;
case WRITE:
enterOuterAlt(_localctx, 12);
{
setState(2791);
writePrivilege();
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AllPrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ALL() {
return getToken(CypherParser.ALL, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public AllPrivilegeTargetContext allPrivilegeTarget() {
return getRuleContext(AllPrivilegeTargetContext.class, 0);
}
public AllPrivilegeTypeContext allPrivilegeType() {
return getRuleContext(AllPrivilegeTypeContext.class, 0);
}
public AllPrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_allPrivilege;
}
}
public final AllPrivilegeContext allPrivilege() throws RecognitionException {
AllPrivilegeContext _localctx = new AllPrivilegeContext(_ctx, getState());
enterRule(_localctx, 438, RULE_allPrivilege);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2794);
match(ALL);
setState(2796);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 61)) & ~0x3f) == 0 && ((1L << (_la - 61)) & 9007199254741009L) != 0)
|| _la == PRIVILEGES) {
{
setState(2795);
allPrivilegeType();
}
}
setState(2798);
match(ON);
setState(2799);
allPrivilegeTarget();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AllPrivilegeTypeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PRIVILEGES() {
return getToken(CypherParser.PRIVILEGES, 0);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public TerminalNode GRAPH() {
return getToken(CypherParser.GRAPH, 0);
}
public TerminalNode DBMS() {
return getToken(CypherParser.DBMS, 0);
}
public AllPrivilegeTypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_allPrivilegeType;
}
}
public final AllPrivilegeTypeContext allPrivilegeType() throws RecognitionException {
AllPrivilegeTypeContext _localctx = new AllPrivilegeTypeContext(_ctx, getState());
enterRule(_localctx, 440, RULE_allPrivilegeType);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2802);
_errHandler.sync(this);
_la = _input.LA(1);
if (((((_la - 61)) & ~0x3f) == 0 && ((1L << (_la - 61)) & 9007199254741009L) != 0)) {
{
setState(2801);
_la = _input.LA(1);
if (!(((((_la - 61)) & ~0x3f) == 0 && ((1L << (_la - 61)) & 9007199254741009L) != 0))) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
setState(2804);
match(PRIVILEGES);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AllPrivilegeTargetContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public AllPrivilegeTargetContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_allPrivilegeTarget;
}
public AllPrivilegeTargetContext() {}
public void copyFrom(AllPrivilegeTargetContext ctx) {
super.copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class DefaultTargetContext extends AllPrivilegeTargetContext {
public TerminalNode DEFAULT() {
return getToken(CypherParser.DEFAULT, 0);
}
public TerminalNode HOME() {
return getToken(CypherParser.HOME, 0);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public TerminalNode GRAPH() {
return getToken(CypherParser.GRAPH, 0);
}
public DefaultTargetContext(AllPrivilegeTargetContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class DatabaseVariableTargetContext extends AllPrivilegeTargetContext {
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public TerminalNode DATABASES() {
return getToken(CypherParser.DATABASES, 0);
}
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public SymbolicAliasNameListContext symbolicAliasNameList() {
return getRuleContext(SymbolicAliasNameListContext.class, 0);
}
public DatabaseVariableTargetContext(AllPrivilegeTargetContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class GraphVariableTargetContext extends AllPrivilegeTargetContext {
public TerminalNode GRAPH() {
return getToken(CypherParser.GRAPH, 0);
}
public TerminalNode GRAPHS() {
return getToken(CypherParser.GRAPHS, 0);
}
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public SymbolicAliasNameListContext symbolicAliasNameList() {
return getRuleContext(SymbolicAliasNameListContext.class, 0);
}
public GraphVariableTargetContext(AllPrivilegeTargetContext ctx) {
copyFrom(ctx);
}
}
@SuppressWarnings("CheckReturnValue")
public static class DBMSTargetContext extends AllPrivilegeTargetContext {
public TerminalNode DBMS() {
return getToken(CypherParser.DBMS, 0);
}
public DBMSTargetContext(AllPrivilegeTargetContext ctx) {
copyFrom(ctx);
}
}
public final AllPrivilegeTargetContext allPrivilegeTarget() throws RecognitionException {
AllPrivilegeTargetContext _localctx = new AllPrivilegeTargetContext(_ctx, getState());
enterRule(_localctx, 442, RULE_allPrivilegeTarget);
int _la;
try {
setState(2819);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DEFAULT:
case HOME:
_localctx = new DefaultTargetContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(2806);
_la = _input.LA(1);
if (!(_la == DEFAULT || _la == HOME)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2807);
_la = _input.LA(1);
if (!(_la == DATABASE || _la == GRAPH)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
case DATABASE:
case DATABASES:
_localctx = new DatabaseVariableTargetContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(2808);
_la = _input.LA(1);
if (!(_la == DATABASE || _la == DATABASES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2811);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(2809);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
{
setState(2810);
symbolicAliasNameList();
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
case GRAPH:
case GRAPHS:
_localctx = new GraphVariableTargetContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(2813);
_la = _input.LA(1);
if (!(_la == GRAPH || _la == GRAPHS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2816);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(2814);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
{
setState(2815);
symbolicAliasNameList();
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
case DBMS:
_localctx = new DBMSTargetContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(2818);
match(DBMS);
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreatePrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CREATE() {
return getToken(CypherParser.CREATE, 0);
}
public CreatePrivilegeForDatabaseContext createPrivilegeForDatabase() {
return getRuleContext(CreatePrivilegeForDatabaseContext.class, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public DatabaseScopeContext databaseScope() {
return getRuleContext(DatabaseScopeContext.class, 0);
}
public ActionForDBMSContext actionForDBMS() {
return getRuleContext(ActionForDBMSContext.class, 0);
}
public TerminalNode DBMS() {
return getToken(CypherParser.DBMS, 0);
}
public GraphScopeContext graphScope() {
return getRuleContext(GraphScopeContext.class, 0);
}
public GraphQualifierContext graphQualifier() {
return getRuleContext(GraphQualifierContext.class, 0);
}
public CreatePrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createPrivilege;
}
}
public final CreatePrivilegeContext createPrivilege() throws RecognitionException {
CreatePrivilegeContext _localctx = new CreatePrivilegeContext(_ctx, getState());
enterRule(_localctx, 444, RULE_createPrivilege);
try {
enterOuterAlt(_localctx, 1);
{
setState(2821);
match(CREATE);
setState(2834);
_errHandler.sync(this);
switch (_input.LA(1)) {
case CONSTRAINT:
case CONSTRAINTS:
case INDEX:
case INDEXES:
case NEW:
{
setState(2822);
createPrivilegeForDatabase();
setState(2823);
match(ON);
setState(2824);
databaseScope();
}
break;
case ALIAS:
case COMPOSITE:
case DATABASE:
case ROLE:
case USER:
{
setState(2826);
actionForDBMS();
setState(2827);
match(ON);
setState(2828);
match(DBMS);
}
break;
case ON:
{
setState(2830);
match(ON);
setState(2831);
graphScope();
setState(2832);
graphQualifier();
}
break;
default:
throw new NoViableAltException(this);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreatePrivilegeForDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public IndexTokenContext indexToken() {
return getRuleContext(IndexTokenContext.class, 0);
}
public ConstraintTokenContext constraintToken() {
return getRuleContext(ConstraintTokenContext.class, 0);
}
public CreateNodePrivilegeTokenContext createNodePrivilegeToken() {
return getRuleContext(CreateNodePrivilegeTokenContext.class, 0);
}
public CreateRelPrivilegeTokenContext createRelPrivilegeToken() {
return getRuleContext(CreateRelPrivilegeTokenContext.class, 0);
}
public CreatePropertyPrivilegeTokenContext createPropertyPrivilegeToken() {
return getRuleContext(CreatePropertyPrivilegeTokenContext.class, 0);
}
public CreatePrivilegeForDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createPrivilegeForDatabase;
}
}
public final CreatePrivilegeForDatabaseContext createPrivilegeForDatabase() throws RecognitionException {
CreatePrivilegeForDatabaseContext _localctx = new CreatePrivilegeForDatabaseContext(_ctx, getState());
enterRule(_localctx, 446, RULE_createPrivilegeForDatabase);
try {
setState(2841);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 344, _ctx)) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(2836);
indexToken();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(2837);
constraintToken();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(2838);
createNodePrivilegeToken();
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(2839);
createRelPrivilegeToken();
}
break;
case 5:
enterOuterAlt(_localctx, 5);
{
setState(2840);
createPropertyPrivilegeToken();
}
break;
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateNodePrivilegeTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode NEW() {
return getToken(CypherParser.NEW, 0);
}
public TerminalNode LABEL() {
return getToken(CypherParser.LABEL, 0);
}
public TerminalNode LABELS() {
return getToken(CypherParser.LABELS, 0);
}
public TerminalNode NODE() {
return getToken(CypherParser.NODE, 0);
}
public CreateNodePrivilegeTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createNodePrivilegeToken;
}
}
public final CreateNodePrivilegeTokenContext createNodePrivilegeToken() throws RecognitionException {
CreateNodePrivilegeTokenContext _localctx = new CreateNodePrivilegeTokenContext(_ctx, getState());
enterRule(_localctx, 448, RULE_createNodePrivilegeToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2843);
match(NEW);
setState(2845);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == NODE) {
{
setState(2844);
match(NODE);
}
}
setState(2847);
_la = _input.LA(1);
if (!(_la == LABEL || _la == LABELS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateRelPrivilegeTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode NEW() {
return getToken(CypherParser.NEW, 0);
}
public TerminalNode TYPE() {
return getToken(CypherParser.TYPE, 0);
}
public TerminalNode TYPES() {
return getToken(CypherParser.TYPES, 0);
}
public TerminalNode RELATIONSHIP() {
return getToken(CypherParser.RELATIONSHIP, 0);
}
public CreateRelPrivilegeTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createRelPrivilegeToken;
}
}
public final CreateRelPrivilegeTokenContext createRelPrivilegeToken() throws RecognitionException {
CreateRelPrivilegeTokenContext _localctx = new CreateRelPrivilegeTokenContext(_ctx, getState());
enterRule(_localctx, 450, RULE_createRelPrivilegeToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2849);
match(NEW);
setState(2851);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == RELATIONSHIP) {
{
setState(2850);
match(RELATIONSHIP);
}
}
setState(2853);
_la = _input.LA(1);
if (!(_la == TYPE || _la == TYPES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreatePropertyPrivilegeTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode NEW() {
return getToken(CypherParser.NEW, 0);
}
public TerminalNode NAME() {
return getToken(CypherParser.NAME, 0);
}
public TerminalNode NAMES() {
return getToken(CypherParser.NAMES, 0);
}
public TerminalNode PROPERTY() {
return getToken(CypherParser.PROPERTY, 0);
}
public CreatePropertyPrivilegeTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createPropertyPrivilegeToken;
}
}
public final CreatePropertyPrivilegeTokenContext createPropertyPrivilegeToken() throws RecognitionException {
CreatePropertyPrivilegeTokenContext _localctx = new CreatePropertyPrivilegeTokenContext(_ctx, getState());
enterRule(_localctx, 452, RULE_createPropertyPrivilegeToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2855);
match(NEW);
setState(2857);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == PROPERTY) {
{
setState(2856);
match(PROPERTY);
}
}
setState(2859);
_la = _input.LA(1);
if (!(_la == NAME || _la == NAMES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ActionForDBMSContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ALIAS() {
return getToken(CypherParser.ALIAS, 0);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public TerminalNode COMPOSITE() {
return getToken(CypherParser.COMPOSITE, 0);
}
public TerminalNode ROLE() {
return getToken(CypherParser.ROLE, 0);
}
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public ActionForDBMSContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_actionForDBMS;
}
}
public final ActionForDBMSContext actionForDBMS() throws RecognitionException {
ActionForDBMSContext _localctx = new ActionForDBMSContext(_ctx, getState());
enterRule(_localctx, 454, RULE_actionForDBMS);
int _la;
try {
setState(2868);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALIAS:
enterOuterAlt(_localctx, 1);
{
setState(2861);
match(ALIAS);
}
break;
case COMPOSITE:
case DATABASE:
enterOuterAlt(_localctx, 2);
{
setState(2863);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == COMPOSITE) {
{
setState(2862);
match(COMPOSITE);
}
}
setState(2865);
match(DATABASE);
}
break;
case ROLE:
enterOuterAlt(_localctx, 3);
{
setState(2866);
match(ROLE);
}
break;
case USER:
enterOuterAlt(_localctx, 4);
{
setState(2867);
match(USER);
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropPrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DROP() {
return getToken(CypherParser.DROP, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public DatabaseScopeContext databaseScope() {
return getRuleContext(DatabaseScopeContext.class, 0);
}
public ActionForDBMSContext actionForDBMS() {
return getRuleContext(ActionForDBMSContext.class, 0);
}
public TerminalNode DBMS() {
return getToken(CypherParser.DBMS, 0);
}
public IndexTokenContext indexToken() {
return getRuleContext(IndexTokenContext.class, 0);
}
public ConstraintTokenContext constraintToken() {
return getRuleContext(ConstraintTokenContext.class, 0);
}
public DropPrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_dropPrivilege;
}
}
public final DropPrivilegeContext dropPrivilege() throws RecognitionException {
DropPrivilegeContext _localctx = new DropPrivilegeContext(_ctx, getState());
enterRule(_localctx, 456, RULE_dropPrivilege);
try {
enterOuterAlt(_localctx, 1);
{
setState(2870);
match(DROP);
setState(2882);
_errHandler.sync(this);
switch (_input.LA(1)) {
case CONSTRAINT:
case CONSTRAINTS:
case INDEX:
case INDEXES:
{
setState(2873);
_errHandler.sync(this);
switch (_input.LA(1)) {
case INDEX:
case INDEXES:
{
setState(2871);
indexToken();
}
break;
case CONSTRAINT:
case CONSTRAINTS:
{
setState(2872);
constraintToken();
}
break;
default:
throw new NoViableAltException(this);
}
setState(2875);
match(ON);
setState(2876);
databaseScope();
}
break;
case ALIAS:
case COMPOSITE:
case DATABASE:
case ROLE:
case USER:
{
setState(2878);
actionForDBMS();
setState(2879);
match(ON);
setState(2880);
match(DBMS);
}
break;
default:
throw new NoViableAltException(this);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LoadPrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LOAD() {
return getToken(CypherParser.LOAD, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public StringOrParameterContext stringOrParameter() {
return getRuleContext(StringOrParameterContext.class, 0);
}
public TerminalNode ALL() {
return getToken(CypherParser.ALL, 0);
}
public TerminalNode DATA() {
return getToken(CypherParser.DATA, 0);
}
public TerminalNode URL() {
return getToken(CypherParser.URL, 0);
}
public TerminalNode CIDR() {
return getToken(CypherParser.CIDR, 0);
}
public LoadPrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_loadPrivilege;
}
}
public final LoadPrivilegeContext loadPrivilege() throws RecognitionException {
LoadPrivilegeContext _localctx = new LoadPrivilegeContext(_ctx, getState());
enterRule(_localctx, 458, RULE_loadPrivilege);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2884);
match(LOAD);
setState(2885);
match(ON);
setState(2890);
_errHandler.sync(this);
switch (_input.LA(1)) {
case CIDR:
case URL:
{
setState(2886);
_la = _input.LA(1);
if (!(_la == CIDR || _la == URL)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2887);
stringOrParameter();
}
break;
case ALL:
{
setState(2888);
match(ALL);
setState(2889);
match(DATA);
}
break;
default:
throw new NoViableAltException(this);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowPrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SHOW() {
return getToken(CypherParser.SHOW, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public DatabaseScopeContext databaseScope() {
return getRuleContext(DatabaseScopeContext.class, 0);
}
public TerminalNode DBMS() {
return getToken(CypherParser.DBMS, 0);
}
public IndexTokenContext indexToken() {
return getRuleContext(IndexTokenContext.class, 0);
}
public ConstraintTokenContext constraintToken() {
return getRuleContext(ConstraintTokenContext.class, 0);
}
public TransactionTokenContext transactionToken() {
return getRuleContext(TransactionTokenContext.class, 0);
}
public TerminalNode ALIAS() {
return getToken(CypherParser.ALIAS, 0);
}
public TerminalNode PRIVILEGE() {
return getToken(CypherParser.PRIVILEGE, 0);
}
public TerminalNode ROLE() {
return getToken(CypherParser.ROLE, 0);
}
public TerminalNode SERVER() {
return getToken(CypherParser.SERVER, 0);
}
public TerminalNode SERVERS() {
return getToken(CypherParser.SERVERS, 0);
}
public TerminalNode SETTING() {
return getToken(CypherParser.SETTING, 0);
}
public SettingQualifierContext settingQualifier() {
return getRuleContext(SettingQualifierContext.class, 0);
}
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public UserQualifierContext userQualifier() {
return getRuleContext(UserQualifierContext.class, 0);
}
public ShowPrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showPrivilege;
}
}
public final ShowPrivilegeContext showPrivilege() throws RecognitionException {
ShowPrivilegeContext _localctx = new ShowPrivilegeContext(_ctx, getState());
enterRule(_localctx, 460, RULE_showPrivilege);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2892);
match(SHOW);
setState(2916);
_errHandler.sync(this);
switch (_input.LA(1)) {
case CONSTRAINT:
case CONSTRAINTS:
case INDEX:
case INDEXES:
case TRANSACTION:
case TRANSACTIONS:
{
setState(2899);
_errHandler.sync(this);
switch (_input.LA(1)) {
case INDEX:
case INDEXES:
{
setState(2893);
indexToken();
}
break;
case CONSTRAINT:
case CONSTRAINTS:
{
setState(2894);
constraintToken();
}
break;
case TRANSACTION:
case TRANSACTIONS:
{
setState(2895);
transactionToken();
setState(2897);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == LPAREN) {
{
setState(2896);
userQualifier();
}
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(2901);
match(ON);
setState(2902);
databaseScope();
}
break;
case ALIAS:
case PRIVILEGE:
case ROLE:
case SERVER:
case SERVERS:
case SETTING:
case USER:
{
setState(2912);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALIAS:
{
setState(2904);
match(ALIAS);
}
break;
case PRIVILEGE:
{
setState(2905);
match(PRIVILEGE);
}
break;
case ROLE:
{
setState(2906);
match(ROLE);
}
break;
case SERVER:
{
setState(2907);
match(SERVER);
}
break;
case SERVERS:
{
setState(2908);
match(SERVERS);
}
break;
case SETTING:
{
setState(2909);
match(SETTING);
setState(2910);
settingQualifier();
}
break;
case USER:
{
setState(2911);
match(USER);
}
break;
default:
throw new NoViableAltException(this);
}
setState(2914);
match(ON);
setState(2915);
match(DBMS);
}
break;
default:
throw new NoViableAltException(this);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SetPrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode SET() {
return getToken(CypherParser.SET, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public TerminalNode DBMS() {
return getToken(CypherParser.DBMS, 0);
}
public TerminalNode LABEL() {
return getToken(CypherParser.LABEL, 0);
}
public LabelsResourceContext labelsResource() {
return getRuleContext(LabelsResourceContext.class, 0);
}
public GraphScopeContext graphScope() {
return getRuleContext(GraphScopeContext.class, 0);
}
public TerminalNode PROPERTY() {
return getToken(CypherParser.PROPERTY, 0);
}
public PropertiesResourceContext propertiesResource() {
return getRuleContext(PropertiesResourceContext.class, 0);
}
public GraphQualifierContext graphQualifier() {
return getRuleContext(GraphQualifierContext.class, 0);
}
public PasswordTokenContext passwordToken() {
return getRuleContext(PasswordTokenContext.class, 0);
}
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public TerminalNode ACCESS() {
return getToken(CypherParser.ACCESS, 0);
}
public TerminalNode STATUS() {
return getToken(CypherParser.STATUS, 0);
}
public TerminalNode HOME() {
return getToken(CypherParser.HOME, 0);
}
public SetPrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_setPrivilege;
}
}
public final SetPrivilegeContext setPrivilege() throws RecognitionException {
SetPrivilegeContext _localctx = new SetPrivilegeContext(_ctx, getState());
enterRule(_localctx, 462, RULE_setPrivilege);
try {
enterOuterAlt(_localctx, 1);
{
setState(2918);
match(SET);
setState(2943);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DATABASE:
case PASSWORD:
case PASSWORDS:
case USER:
{
setState(2928);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PASSWORD:
case PASSWORDS:
{
setState(2919);
passwordToken();
}
break;
case USER:
{
setState(2920);
match(USER);
setState(2924);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STATUS:
{
setState(2921);
match(STATUS);
}
break;
case HOME:
{
setState(2922);
match(HOME);
setState(2923);
match(DATABASE);
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
case DATABASE:
{
setState(2926);
match(DATABASE);
setState(2927);
match(ACCESS);
}
break;
default:
throw new NoViableAltException(this);
}
setState(2930);
match(ON);
setState(2931);
match(DBMS);
}
break;
case LABEL:
{
setState(2932);
match(LABEL);
setState(2933);
labelsResource();
setState(2934);
match(ON);
setState(2935);
graphScope();
}
break;
case PROPERTY:
{
setState(2937);
match(PROPERTY);
setState(2938);
propertiesResource();
setState(2939);
match(ON);
setState(2940);
graphScope();
setState(2941);
graphQualifier();
}
break;
default:
throw new NoViableAltException(this);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PasswordTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PASSWORD() {
return getToken(CypherParser.PASSWORD, 0);
}
public TerminalNode PASSWORDS() {
return getToken(CypherParser.PASSWORDS, 0);
}
public PasswordTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_passwordToken;
}
}
public final PasswordTokenContext passwordToken() throws RecognitionException {
PasswordTokenContext _localctx = new PasswordTokenContext(_ctx, getState());
enterRule(_localctx, 464, RULE_passwordToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2945);
_la = _input.LA(1);
if (!(_la == PASSWORD || _la == PASSWORDS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RemovePrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode REMOVE() {
return getToken(CypherParser.REMOVE, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public TerminalNode DBMS() {
return getToken(CypherParser.DBMS, 0);
}
public TerminalNode LABEL() {
return getToken(CypherParser.LABEL, 0);
}
public LabelsResourceContext labelsResource() {
return getRuleContext(LabelsResourceContext.class, 0);
}
public GraphScopeContext graphScope() {
return getRuleContext(GraphScopeContext.class, 0);
}
public TerminalNode PRIVILEGE() {
return getToken(CypherParser.PRIVILEGE, 0);
}
public TerminalNode ROLE() {
return getToken(CypherParser.ROLE, 0);
}
public RemovePrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_removePrivilege;
}
}
public final RemovePrivilegeContext removePrivilege() throws RecognitionException {
RemovePrivilegeContext _localctx = new RemovePrivilegeContext(_ctx, getState());
enterRule(_localctx, 466, RULE_removePrivilege);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2947);
match(REMOVE);
setState(2956);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PRIVILEGE:
case ROLE:
{
setState(2948);
_la = _input.LA(1);
if (!(_la == PRIVILEGE || _la == ROLE)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(2949);
match(ON);
setState(2950);
match(DBMS);
}
break;
case LABEL:
{
setState(2951);
match(LABEL);
setState(2952);
labelsResource();
setState(2953);
match(ON);
setState(2954);
graphScope();
}
break;
default:
throw new NoViableAltException(this);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class WritePrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode WRITE() {
return getToken(CypherParser.WRITE, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public GraphScopeContext graphScope() {
return getRuleContext(GraphScopeContext.class, 0);
}
public WritePrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_writePrivilege;
}
}
public final WritePrivilegeContext writePrivilege() throws RecognitionException {
WritePrivilegeContext _localctx = new WritePrivilegeContext(_ctx, getState());
enterRule(_localctx, 468, RULE_writePrivilege);
try {
enterOuterAlt(_localctx, 1);
{
setState(2958);
match(WRITE);
setState(2959);
match(ON);
setState(2960);
graphScope();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DatabasePrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public DatabaseScopeContext databaseScope() {
return getRuleContext(DatabaseScopeContext.class, 0);
}
public TerminalNode ACCESS() {
return getToken(CypherParser.ACCESS, 0);
}
public TerminalNode START() {
return getToken(CypherParser.START, 0);
}
public TerminalNode STOP() {
return getToken(CypherParser.STOP, 0);
}
public IndexTokenContext indexToken() {
return getRuleContext(IndexTokenContext.class, 0);
}
public ConstraintTokenContext constraintToken() {
return getRuleContext(ConstraintTokenContext.class, 0);
}
public TerminalNode NAME() {
return getToken(CypherParser.NAME, 0);
}
public TerminalNode TRANSACTION() {
return getToken(CypherParser.TRANSACTION, 0);
}
public TerminalNode TERMINATE() {
return getToken(CypherParser.TERMINATE, 0);
}
public TransactionTokenContext transactionToken() {
return getRuleContext(TransactionTokenContext.class, 0);
}
public TerminalNode MANAGEMENT() {
return getToken(CypherParser.MANAGEMENT, 0);
}
public UserQualifierContext userQualifier() {
return getRuleContext(UserQualifierContext.class, 0);
}
public DatabasePrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_databasePrivilege;
}
}
public final DatabasePrivilegeContext databasePrivilege() throws RecognitionException {
DatabasePrivilegeContext _localctx = new DatabasePrivilegeContext(_ctx, getState());
enterRule(_localctx, 470, RULE_databasePrivilege);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(2984);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ACCESS:
{
setState(2962);
match(ACCESS);
}
break;
case START:
{
setState(2963);
match(START);
}
break;
case STOP:
{
setState(2964);
match(STOP);
}
break;
case CONSTRAINT:
case CONSTRAINTS:
case INDEX:
case INDEXES:
case NAME:
{
setState(2968);
_errHandler.sync(this);
switch (_input.LA(1)) {
case INDEX:
case INDEXES:
{
setState(2965);
indexToken();
}
break;
case CONSTRAINT:
case CONSTRAINTS:
{
setState(2966);
constraintToken();
}
break;
case NAME:
{
setState(2967);
match(NAME);
}
break;
default:
throw new NoViableAltException(this);
}
setState(2971);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == MANAGEMENT) {
{
setState(2970);
match(MANAGEMENT);
}
}
}
break;
case TERMINATE:
case TRANSACTION:
{
setState(2979);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TRANSACTION:
{
setState(2973);
match(TRANSACTION);
setState(2975);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == MANAGEMENT) {
{
setState(2974);
match(MANAGEMENT);
}
}
}
break;
case TERMINATE:
{
setState(2977);
match(TERMINATE);
setState(2978);
transactionToken();
}
break;
default:
throw new NoViableAltException(this);
}
setState(2982);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == LPAREN) {
{
setState(2981);
userQualifier();
}
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(2986);
match(ON);
setState(2987);
databaseScope();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DbmsPrivilegeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public TerminalNode DBMS() {
return getToken(CypherParser.DBMS, 0);
}
public TerminalNode ALTER() {
return getToken(CypherParser.ALTER, 0);
}
public TerminalNode ASSIGN() {
return getToken(CypherParser.ASSIGN, 0);
}
public TerminalNode MANAGEMENT() {
return getToken(CypherParser.MANAGEMENT, 0);
}
public DbmsPrivilegeExecuteContext dbmsPrivilegeExecute() {
return getRuleContext(DbmsPrivilegeExecuteContext.class, 0);
}
public TerminalNode RENAME() {
return getToken(CypherParser.RENAME, 0);
}
public TerminalNode IMPERSONATE() {
return getToken(CypherParser.IMPERSONATE, 0);
}
public TerminalNode ALIAS() {
return getToken(CypherParser.ALIAS, 0);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public TerminalNode PRIVILEGE() {
return getToken(CypherParser.PRIVILEGE, 0);
}
public TerminalNode ROLE() {
return getToken(CypherParser.ROLE, 0);
}
public TerminalNode SERVER() {
return getToken(CypherParser.SERVER, 0);
}
public UserQualifierContext userQualifier() {
return getRuleContext(UserQualifierContext.class, 0);
}
public TerminalNode COMPOSITE() {
return getToken(CypherParser.COMPOSITE, 0);
}
public DbmsPrivilegeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_dbmsPrivilege;
}
}
public final DbmsPrivilegeContext dbmsPrivilege() throws RecognitionException {
DbmsPrivilegeContext _localctx = new DbmsPrivilegeContext(_ctx, getState());
enterRule(_localctx, 472, RULE_dbmsPrivilege);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3012);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALTER:
{
setState(2989);
match(ALTER);
setState(2990);
_la = _input.LA(1);
if (!(_la == ALIAS || _la == DATABASE || _la == USER)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
case ASSIGN:
{
setState(2991);
match(ASSIGN);
setState(2992);
_la = _input.LA(1);
if (!(_la == PRIVILEGE || _la == ROLE)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
case ALIAS:
case COMPOSITE:
case DATABASE:
case PRIVILEGE:
case ROLE:
case SERVER:
case USER:
{
setState(3002);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ALIAS:
{
setState(2993);
match(ALIAS);
}
break;
case COMPOSITE:
case DATABASE:
{
setState(2995);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == COMPOSITE) {
{
setState(2994);
match(COMPOSITE);
}
}
setState(2997);
match(DATABASE);
}
break;
case PRIVILEGE:
{
setState(2998);
match(PRIVILEGE);
}
break;
case ROLE:
{
setState(2999);
match(ROLE);
}
break;
case SERVER:
{
setState(3000);
match(SERVER);
}
break;
case USER:
{
setState(3001);
match(USER);
}
break;
default:
throw new NoViableAltException(this);
}
setState(3004);
match(MANAGEMENT);
}
break;
case EXECUTE:
{
setState(3005);
dbmsPrivilegeExecute();
}
break;
case RENAME:
{
setState(3006);
match(RENAME);
setState(3007);
_la = _input.LA(1);
if (!(_la == ROLE || _la == USER)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
break;
case IMPERSONATE:
{
setState(3008);
match(IMPERSONATE);
setState(3010);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == LPAREN) {
{
setState(3009);
userQualifier();
}
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(3014);
match(ON);
setState(3015);
match(DBMS);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DbmsPrivilegeExecuteContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode EXECUTE() {
return getToken(CypherParser.EXECUTE, 0);
}
public AdminTokenContext adminToken() {
return getRuleContext(AdminTokenContext.class, 0);
}
public TerminalNode PROCEDURES() {
return getToken(CypherParser.PROCEDURES, 0);
}
public ProcedureTokenContext procedureToken() {
return getRuleContext(ProcedureTokenContext.class, 0);
}
public ExecuteProcedureQualifierContext executeProcedureQualifier() {
return getRuleContext(ExecuteProcedureQualifierContext.class, 0);
}
public TerminalNode FUNCTIONS() {
return getToken(CypherParser.FUNCTIONS, 0);
}
public ExecuteFunctionQualifierContext executeFunctionQualifier() {
return getRuleContext(ExecuteFunctionQualifierContext.class, 0);
}
public TerminalNode BOOSTED() {
return getToken(CypherParser.BOOSTED, 0);
}
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public TerminalNode DEFINED() {
return getToken(CypherParser.DEFINED, 0);
}
public DbmsPrivilegeExecuteContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_dbmsPrivilegeExecute;
}
}
public final DbmsPrivilegeExecuteContext dbmsPrivilegeExecute() throws RecognitionException {
DbmsPrivilegeExecuteContext _localctx = new DbmsPrivilegeExecuteContext(_ctx, getState());
enterRule(_localctx, 474, RULE_dbmsPrivilegeExecute);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3017);
match(EXECUTE);
setState(3037);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ADMIN:
case ADMINISTRATOR:
{
setState(3018);
adminToken();
setState(3019);
match(PROCEDURES);
}
break;
case BOOSTED:
case FUNCTIONS:
case PROCEDURE:
case PROCEDURES:
case USER:
{
setState(3022);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == BOOSTED) {
{
setState(3021);
match(BOOSTED);
}
}
setState(3035);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PROCEDURE:
case PROCEDURES:
{
setState(3024);
procedureToken();
setState(3025);
executeProcedureQualifier();
}
break;
case FUNCTIONS:
case USER:
{
setState(3031);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == USER) {
{
setState(3027);
match(USER);
setState(3029);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == DEFINED) {
{
setState(3028);
match(DEFINED);
}
}
}
}
setState(3033);
match(FUNCTIONS);
setState(3034);
executeFunctionQualifier();
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
default:
throw new NoViableAltException(this);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AdminTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ADMIN() {
return getToken(CypherParser.ADMIN, 0);
}
public TerminalNode ADMINISTRATOR() {
return getToken(CypherParser.ADMINISTRATOR, 0);
}
public AdminTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_adminToken;
}
}
public final AdminTokenContext adminToken() throws RecognitionException {
AdminTokenContext _localctx = new AdminTokenContext(_ctx, getState());
enterRule(_localctx, 476, RULE_adminToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3039);
_la = _input.LA(1);
if (!(_la == ADMIN || _la == ADMINISTRATOR)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ProcedureTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PROCEDURE() {
return getToken(CypherParser.PROCEDURE, 0);
}
public TerminalNode PROCEDURES() {
return getToken(CypherParser.PROCEDURES, 0);
}
public ProcedureTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_procedureToken;
}
}
public final ProcedureTokenContext procedureToken() throws RecognitionException {
ProcedureTokenContext _localctx = new ProcedureTokenContext(_ctx, getState());
enterRule(_localctx, 478, RULE_procedureToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3041);
_la = _input.LA(1);
if (!(_la == PROCEDURE || _la == PROCEDURES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class IndexTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode INDEX() {
return getToken(CypherParser.INDEX, 0);
}
public TerminalNode INDEXES() {
return getToken(CypherParser.INDEXES, 0);
}
public IndexTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_indexToken;
}
}
public final IndexTokenContext indexToken() throws RecognitionException {
IndexTokenContext _localctx = new IndexTokenContext(_ctx, getState());
enterRule(_localctx, 480, RULE_indexToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3043);
_la = _input.LA(1);
if (!(_la == INDEX || _la == INDEXES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ConstraintTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode CONSTRAINT() {
return getToken(CypherParser.CONSTRAINT, 0);
}
public TerminalNode CONSTRAINTS() {
return getToken(CypherParser.CONSTRAINTS, 0);
}
public ConstraintTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_constraintToken;
}
}
public final ConstraintTokenContext constraintToken() throws RecognitionException {
ConstraintTokenContext _localctx = new ConstraintTokenContext(_ctx, getState());
enterRule(_localctx, 482, RULE_constraintToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3045);
_la = _input.LA(1);
if (!(_la == CONSTRAINT || _la == CONSTRAINTS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TransactionTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode TRANSACTION() {
return getToken(CypherParser.TRANSACTION, 0);
}
public TerminalNode TRANSACTIONS() {
return getToken(CypherParser.TRANSACTIONS, 0);
}
public TransactionTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_transactionToken;
}
}
public final TransactionTokenContext transactionToken() throws RecognitionException {
TransactionTokenContext _localctx = new TransactionTokenContext(_ctx, getState());
enterRule(_localctx, 484, RULE_transactionToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3047);
_la = _input.LA(1);
if (!(_la == TRANSACTION || _la == TRANSACTIONS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UserQualifierContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public SymbolicNameOrStringParameterListContext symbolicNameOrStringParameterList() {
return getRuleContext(SymbolicNameOrStringParameterListContext.class, 0);
}
public UserQualifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_userQualifier;
}
}
public final UserQualifierContext userQualifier() throws RecognitionException {
UserQualifierContext _localctx = new UserQualifierContext(_ctx, getState());
enterRule(_localctx, 486, RULE_userQualifier);
try {
enterOuterAlt(_localctx, 1);
{
setState(3049);
match(LPAREN);
setState(3052);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(3050);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
{
setState(3051);
symbolicNameOrStringParameterList();
}
break;
default:
throw new NoViableAltException(this);
}
setState(3054);
match(RPAREN);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExecuteFunctionQualifierContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public GlobsContext globs() {
return getRuleContext(GlobsContext.class, 0);
}
public ExecuteFunctionQualifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_executeFunctionQualifier;
}
}
public final ExecuteFunctionQualifierContext executeFunctionQualifier() throws RecognitionException {
ExecuteFunctionQualifierContext _localctx = new ExecuteFunctionQualifierContext(_ctx, getState());
enterRule(_localctx, 488, RULE_executeFunctionQualifier);
try {
enterOuterAlt(_localctx, 1);
{
setState(3056);
globs();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExecuteProcedureQualifierContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public GlobsContext globs() {
return getRuleContext(GlobsContext.class, 0);
}
public ExecuteProcedureQualifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_executeProcedureQualifier;
}
}
public final ExecuteProcedureQualifierContext executeProcedureQualifier() throws RecognitionException {
ExecuteProcedureQualifierContext _localctx = new ExecuteProcedureQualifierContext(_ctx, getState());
enterRule(_localctx, 490, RULE_executeProcedureQualifier);
try {
enterOuterAlt(_localctx, 1);
{
setState(3058);
globs();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SettingQualifierContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public GlobsContext globs() {
return getRuleContext(GlobsContext.class, 0);
}
public SettingQualifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_settingQualifier;
}
}
public final SettingQualifierContext settingQualifier() throws RecognitionException {
SettingQualifierContext _localctx = new SettingQualifierContext(_ctx, getState());
enterRule(_localctx, 492, RULE_settingQualifier);
try {
enterOuterAlt(_localctx, 1);
{
setState(3060);
globs();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GlobsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List glob() {
return getRuleContexts(GlobContext.class);
}
public GlobContext glob(int i) {
return getRuleContext(GlobContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public GlobsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_globs;
}
}
public final GlobsContext globs() throws RecognitionException {
GlobsContext _localctx = new GlobsContext(_ctx, getState());
enterRule(_localctx, 494, RULE_globs);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3062);
glob();
setState(3067);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(3063);
match(COMMA);
setState(3064);
glob();
}
}
setState(3069);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class QualifiedGraphPrivilegesWithPropertyContext
extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public GraphScopeContext graphScope() {
return getRuleContext(GraphScopeContext.class, 0);
}
public GraphQualifierContext graphQualifier() {
return getRuleContext(GraphQualifierContext.class, 0);
}
public TerminalNode TRAVERSE() {
return getToken(CypherParser.TRAVERSE, 0);
}
public PropertiesResourceContext propertiesResource() {
return getRuleContext(PropertiesResourceContext.class, 0);
}
public TerminalNode READ() {
return getToken(CypherParser.READ, 0);
}
public TerminalNode MATCH() {
return getToken(CypherParser.MATCH, 0);
}
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public QualifiedGraphPrivilegesWithPropertyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_qualifiedGraphPrivilegesWithProperty;
}
}
public final QualifiedGraphPrivilegesWithPropertyContext qualifiedGraphPrivilegesWithProperty()
throws RecognitionException {
QualifiedGraphPrivilegesWithPropertyContext _localctx =
new QualifiedGraphPrivilegesWithPropertyContext(_ctx, getState());
enterRule(_localctx, 496, RULE_qualifiedGraphPrivilegesWithProperty);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3073);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TRAVERSE:
{
setState(3070);
match(TRAVERSE);
}
break;
case MATCH:
case READ:
{
setState(3071);
_la = _input.LA(1);
if (!(_la == MATCH || _la == READ)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3072);
propertiesResource();
}
break;
default:
throw new NoViableAltException(this);
}
setState(3075);
match(ON);
setState(3076);
graphScope();
setState(3077);
graphQualifier();
setState(3081);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == LPAREN) {
{
setState(3078);
match(LPAREN);
setState(3079);
match(TIMES);
setState(3080);
match(RPAREN);
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class QualifiedGraphPrivilegesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public GraphScopeContext graphScope() {
return getRuleContext(GraphScopeContext.class, 0);
}
public GraphQualifierContext graphQualifier() {
return getRuleContext(GraphQualifierContext.class, 0);
}
public TerminalNode DELETE() {
return getToken(CypherParser.DELETE, 0);
}
public TerminalNode MERGE() {
return getToken(CypherParser.MERGE, 0);
}
public PropertiesResourceContext propertiesResource() {
return getRuleContext(PropertiesResourceContext.class, 0);
}
public QualifiedGraphPrivilegesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_qualifiedGraphPrivileges;
}
}
public final QualifiedGraphPrivilegesContext qualifiedGraphPrivileges() throws RecognitionException {
QualifiedGraphPrivilegesContext _localctx = new QualifiedGraphPrivilegesContext(_ctx, getState());
enterRule(_localctx, 498, RULE_qualifiedGraphPrivileges);
try {
enterOuterAlt(_localctx, 1);
{
setState(3086);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DELETE:
{
setState(3083);
match(DELETE);
}
break;
case MERGE:
{
setState(3084);
match(MERGE);
setState(3085);
propertiesResource();
}
break;
default:
throw new NoViableAltException(this);
}
setState(3088);
match(ON);
setState(3089);
graphScope();
setState(3090);
graphQualifier();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LabelsResourceContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public NonEmptyStringListContext nonEmptyStringList() {
return getRuleContext(NonEmptyStringListContext.class, 0);
}
public LabelsResourceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_labelsResource;
}
}
public final LabelsResourceContext labelsResource() throws RecognitionException {
LabelsResourceContext _localctx = new LabelsResourceContext(_ctx, getState());
enterRule(_localctx, 500, RULE_labelsResource);
try {
setState(3094);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
enterOuterAlt(_localctx, 1);
{
setState(3092);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 2);
{
setState(3093);
nonEmptyStringList();
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PropertiesResourceContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LCURLY() {
return getToken(CypherParser.LCURLY, 0);
}
public TerminalNode RCURLY() {
return getToken(CypherParser.RCURLY, 0);
}
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public NonEmptyStringListContext nonEmptyStringList() {
return getRuleContext(NonEmptyStringListContext.class, 0);
}
public PropertiesResourceContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_propertiesResource;
}
}
public final PropertiesResourceContext propertiesResource() throws RecognitionException {
PropertiesResourceContext _localctx = new PropertiesResourceContext(_ctx, getState());
enterRule(_localctx, 502, RULE_propertiesResource);
try {
enterOuterAlt(_localctx, 1);
{
setState(3096);
match(LCURLY);
setState(3099);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(3097);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
{
setState(3098);
nonEmptyStringList();
}
break;
default:
throw new NoViableAltException(this);
}
setState(3101);
match(RCURLY);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NonEmptyStringListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public NonEmptyStringListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_nonEmptyStringList;
}
}
public final NonEmptyStringListContext nonEmptyStringList() throws RecognitionException {
NonEmptyStringListContext _localctx = new NonEmptyStringListContext(_ctx, getState());
enterRule(_localctx, 504, RULE_nonEmptyStringList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3103);
symbolicNameString();
setState(3108);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(3104);
match(COMMA);
setState(3105);
symbolicNameString();
}
}
setState(3110);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GraphQualifierContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public GraphQualifierTokenContext graphQualifierToken() {
return getRuleContext(GraphQualifierTokenContext.class, 0);
}
public TerminalNode FOR() {
return getToken(CypherParser.FOR, 0);
}
public TerminalNode LPAREN() {
return getToken(CypherParser.LPAREN, 0);
}
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public NonEmptyStringListContext nonEmptyStringList() {
return getRuleContext(NonEmptyStringListContext.class, 0);
}
public TerminalNode RPAREN() {
return getToken(CypherParser.RPAREN, 0);
}
public TerminalNode WHERE() {
return getToken(CypherParser.WHERE, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public VariableContext variable() {
return getRuleContext(VariableContext.class, 0);
}
public TerminalNode COLON() {
return getToken(CypherParser.COLON, 0);
}
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class, i);
}
public MapContext map() {
return getRuleContext(MapContext.class, 0);
}
public List BAR() {
return getTokens(CypherParser.BAR);
}
public TerminalNode BAR(int i) {
return getToken(CypherParser.BAR, i);
}
public GraphQualifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_graphQualifier;
}
}
public final GraphQualifierContext graphQualifier() throws RecognitionException {
GraphQualifierContext _localctx = new GraphQualifierContext(_ctx, getState());
enterRule(_localctx, 506, RULE_graphQualifier);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3144);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ELEMENT:
case ELEMENTS:
case NODE:
case NODES:
case RELATIONSHIP:
case RELATIONSHIPS:
{
setState(3111);
graphQualifierToken();
setState(3114);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(3112);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
{
setState(3113);
nonEmptyStringList();
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
case FOR:
{
setState(3116);
match(FOR);
setState(3117);
match(LPAREN);
setState(3119);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 385, _ctx)) {
case 1:
{
setState(3118);
variable();
}
break;
}
setState(3130);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == COLON) {
{
setState(3121);
match(COLON);
setState(3122);
symbolicNameString();
setState(3127);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == BAR) {
{
{
setState(3123);
match(BAR);
setState(3124);
symbolicNameString();
}
}
setState(3129);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(3142);
_errHandler.sync(this);
switch (_input.LA(1)) {
case RPAREN:
{
setState(3132);
match(RPAREN);
setState(3133);
match(WHERE);
setState(3134);
expression();
}
break;
case LCURLY:
case WHERE:
{
setState(3138);
_errHandler.sync(this);
switch (_input.LA(1)) {
case WHERE:
{
setState(3135);
match(WHERE);
setState(3136);
expression();
}
break;
case LCURLY:
{
setState(3137);
map();
}
break;
default:
throw new NoViableAltException(this);
}
setState(3140);
match(RPAREN);
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
case FROM:
case LPAREN:
case TO:
break;
default:
break;
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GraphQualifierTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public RelTokenContext relToken() {
return getRuleContext(RelTokenContext.class, 0);
}
public NodeTokenContext nodeToken() {
return getRuleContext(NodeTokenContext.class, 0);
}
public ElementTokenContext elementToken() {
return getRuleContext(ElementTokenContext.class, 0);
}
public GraphQualifierTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_graphQualifierToken;
}
}
public final GraphQualifierTokenContext graphQualifierToken() throws RecognitionException {
GraphQualifierTokenContext _localctx = new GraphQualifierTokenContext(_ctx, getState());
enterRule(_localctx, 508, RULE_graphQualifierToken);
try {
setState(3149);
_errHandler.sync(this);
switch (_input.LA(1)) {
case RELATIONSHIP:
case RELATIONSHIPS:
enterOuterAlt(_localctx, 1);
{
setState(3146);
relToken();
}
break;
case NODE:
case NODES:
enterOuterAlt(_localctx, 2);
{
setState(3147);
nodeToken();
}
break;
case ELEMENT:
case ELEMENTS:
enterOuterAlt(_localctx, 3);
{
setState(3148);
elementToken();
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class RelTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode RELATIONSHIP() {
return getToken(CypherParser.RELATIONSHIP, 0);
}
public TerminalNode RELATIONSHIPS() {
return getToken(CypherParser.RELATIONSHIPS, 0);
}
public RelTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_relToken;
}
}
public final RelTokenContext relToken() throws RecognitionException {
RelTokenContext _localctx = new RelTokenContext(_ctx, getState());
enterRule(_localctx, 510, RULE_relToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3151);
_la = _input.LA(1);
if (!(_la == RELATIONSHIP || _la == RELATIONSHIPS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ElementTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ELEMENT() {
return getToken(CypherParser.ELEMENT, 0);
}
public TerminalNode ELEMENTS() {
return getToken(CypherParser.ELEMENTS, 0);
}
public ElementTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_elementToken;
}
}
public final ElementTokenContext elementToken() throws RecognitionException {
ElementTokenContext _localctx = new ElementTokenContext(_ctx, getState());
enterRule(_localctx, 512, RULE_elementToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3153);
_la = _input.LA(1);
if (!(_la == ELEMENT || _la == ELEMENTS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NodeTokenContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode NODE() {
return getToken(CypherParser.NODE, 0);
}
public TerminalNode NODES() {
return getToken(CypherParser.NODES, 0);
}
public NodeTokenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_nodeToken;
}
}
public final NodeTokenContext nodeToken() throws RecognitionException {
NodeTokenContext _localctx = new NodeTokenContext(_ctx, getState());
enterRule(_localctx, 514, RULE_nodeToken);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3155);
_la = _input.LA(1);
if (!(_la == NODE || _la == NODES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateCompositeDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode COMPOSITE() {
return getToken(CypherParser.COMPOSITE, 0);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public CommandOptionsContext commandOptions() {
return getRuleContext(CommandOptionsContext.class, 0);
}
public WaitClauseContext waitClause() {
return getRuleContext(WaitClauseContext.class, 0);
}
public CreateCompositeDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createCompositeDatabase;
}
}
public final CreateCompositeDatabaseContext createCompositeDatabase() throws RecognitionException {
CreateCompositeDatabaseContext _localctx = new CreateCompositeDatabaseContext(_ctx, getState());
enterRule(_localctx, 516, RULE_createCompositeDatabase);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3157);
match(COMPOSITE);
setState(3158);
match(DATABASE);
setState(3159);
symbolicAliasNameOrParameter();
setState(3163);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(3160);
match(IF);
setState(3161);
match(NOT);
setState(3162);
match(EXISTS);
}
}
setState(3166);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == OPTIONS) {
{
setState(3165);
commandOptions();
}
}
setState(3169);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == NOWAIT || _la == WAIT) {
{
setState(3168);
waitClause();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public TerminalNode TOPOLOGY() {
return getToken(CypherParser.TOPOLOGY, 0);
}
public CommandOptionsContext commandOptions() {
return getRuleContext(CommandOptionsContext.class, 0);
}
public WaitClauseContext waitClause() {
return getRuleContext(WaitClauseContext.class, 0);
}
public List primaryTopology() {
return getRuleContexts(PrimaryTopologyContext.class);
}
public PrimaryTopologyContext primaryTopology(int i) {
return getRuleContext(PrimaryTopologyContext.class, i);
}
public List secondaryTopology() {
return getRuleContexts(SecondaryTopologyContext.class);
}
public SecondaryTopologyContext secondaryTopology(int i) {
return getRuleContext(SecondaryTopologyContext.class, i);
}
public CreateDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createDatabase;
}
}
public final CreateDatabaseContext createDatabase() throws RecognitionException {
CreateDatabaseContext _localctx = new CreateDatabaseContext(_ctx, getState());
enterRule(_localctx, 518, RULE_createDatabase);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3171);
match(DATABASE);
setState(3172);
symbolicAliasNameOrParameter();
setState(3176);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(3173);
match(IF);
setState(3174);
match(NOT);
setState(3175);
match(EXISTS);
}
}
setState(3185);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == TOPOLOGY) {
{
setState(3178);
match(TOPOLOGY);
setState(3181);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
setState(3181);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 396, _ctx)) {
case 1:
{
setState(3179);
primaryTopology();
}
break;
case 2:
{
setState(3180);
secondaryTopology();
}
break;
}
}
setState(3183);
_errHandler.sync(this);
_la = _input.LA(1);
} while (_la == UNSIGNED_DECIMAL_INTEGER);
}
}
setState(3188);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == OPTIONS) {
{
setState(3187);
commandOptions();
}
}
setState(3191);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == NOWAIT || _la == WAIT) {
{
setState(3190);
waitClause();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PrimaryTopologyContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode UNSIGNED_DECIMAL_INTEGER() {
return getToken(CypherParser.UNSIGNED_DECIMAL_INTEGER, 0);
}
public TerminalNode PRIMARY() {
return getToken(CypherParser.PRIMARY, 0);
}
public PrimaryTopologyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_primaryTopology;
}
}
public final PrimaryTopologyContext primaryTopology() throws RecognitionException {
PrimaryTopologyContext _localctx = new PrimaryTopologyContext(_ctx, getState());
enterRule(_localctx, 520, RULE_primaryTopology);
try {
enterOuterAlt(_localctx, 1);
{
setState(3193);
match(UNSIGNED_DECIMAL_INTEGER);
setState(3194);
match(PRIMARY);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SecondaryTopologyContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode UNSIGNED_DECIMAL_INTEGER() {
return getToken(CypherParser.UNSIGNED_DECIMAL_INTEGER, 0);
}
public TerminalNode SECONDARY() {
return getToken(CypherParser.SECONDARY, 0);
}
public SecondaryTopologyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_secondaryTopology;
}
}
public final SecondaryTopologyContext secondaryTopology() throws RecognitionException {
SecondaryTopologyContext _localctx = new SecondaryTopologyContext(_ctx, getState());
enterRule(_localctx, 522, RULE_secondaryTopology);
try {
enterOuterAlt(_localctx, 1);
{
setState(3196);
match(UNSIGNED_DECIMAL_INTEGER);
setState(3197);
match(SECONDARY);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class, 0);
}
public TerminalNode COMPOSITE() {
return getToken(CypherParser.COMPOSITE, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public TerminalNode DATA() {
return getToken(CypherParser.DATA, 0);
}
public WaitClauseContext waitClause() {
return getRuleContext(WaitClauseContext.class, 0);
}
public TerminalNode DUMP() {
return getToken(CypherParser.DUMP, 0);
}
public TerminalNode DESTROY() {
return getToken(CypherParser.DESTROY, 0);
}
public DropDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_dropDatabase;
}
}
public final DropDatabaseContext dropDatabase() throws RecognitionException {
DropDatabaseContext _localctx = new DropDatabaseContext(_ctx, getState());
enterRule(_localctx, 524, RULE_dropDatabase);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3200);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == COMPOSITE) {
{
setState(3199);
match(COMPOSITE);
}
}
setState(3202);
match(DATABASE);
setState(3203);
symbolicAliasNameOrParameter();
setState(3206);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(3204);
match(IF);
setState(3205);
match(EXISTS);
}
}
setState(3210);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == DESTROY || _la == DUMP) {
{
setState(3208);
_la = _input.LA(1);
if (!(_la == DESTROY || _la == DUMP)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3209);
match(DATA);
}
}
setState(3213);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == NOWAIT || _la == WAIT) {
{
setState(3212);
waitClause();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public WaitClauseContext waitClause() {
return getRuleContext(WaitClauseContext.class, 0);
}
public List SET() {
return getTokens(CypherParser.SET);
}
public TerminalNode SET(int i) {
return getToken(CypherParser.SET, i);
}
public List REMOVE() {
return getTokens(CypherParser.REMOVE);
}
public TerminalNode REMOVE(int i) {
return getToken(CypherParser.REMOVE, i);
}
public List OPTION() {
return getTokens(CypherParser.OPTION);
}
public TerminalNode OPTION(int i) {
return getToken(CypherParser.OPTION, i);
}
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class, i);
}
public List alterDatabaseAccess() {
return getRuleContexts(AlterDatabaseAccessContext.class);
}
public AlterDatabaseAccessContext alterDatabaseAccess(int i) {
return getRuleContext(AlterDatabaseAccessContext.class, i);
}
public List alterDatabaseTopology() {
return getRuleContexts(AlterDatabaseTopologyContext.class);
}
public AlterDatabaseTopologyContext alterDatabaseTopology(int i) {
return getRuleContext(AlterDatabaseTopologyContext.class, i);
}
public List alterDatabaseOption() {
return getRuleContexts(AlterDatabaseOptionContext.class);
}
public AlterDatabaseOptionContext alterDatabaseOption(int i) {
return getRuleContext(AlterDatabaseOptionContext.class, i);
}
public AlterDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_alterDatabase;
}
}
public final AlterDatabaseContext alterDatabase() throws RecognitionException {
AlterDatabaseContext _localctx = new AlterDatabaseContext(_ctx, getState());
enterRule(_localctx, 526, RULE_alterDatabase);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3215);
match(DATABASE);
setState(3216);
symbolicAliasNameOrParameter();
setState(3219);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(3217);
match(IF);
setState(3218);
match(EXISTS);
}
}
setState(3238);
_errHandler.sync(this);
switch (_input.LA(1)) {
case SET:
{
setState(3227);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(3221);
match(SET);
setState(3225);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ACCESS:
{
setState(3222);
alterDatabaseAccess();
}
break;
case TOPOLOGY:
{
setState(3223);
alterDatabaseTopology();
}
break;
case OPTION:
{
setState(3224);
alterDatabaseOption();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
setState(3229);
_errHandler.sync(this);
_la = _input.LA(1);
} while (_la == SET);
}
break;
case REMOVE:
{
setState(3234);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(3231);
match(REMOVE);
setState(3232);
match(OPTION);
setState(3233);
symbolicNameString();
}
}
setState(3236);
_errHandler.sync(this);
_la = _input.LA(1);
} while (_la == REMOVE);
}
break;
default:
throw new NoViableAltException(this);
}
setState(3241);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == NOWAIT || _la == WAIT) {
{
setState(3240);
waitClause();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterDatabaseAccessContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ACCESS() {
return getToken(CypherParser.ACCESS, 0);
}
public TerminalNode READ() {
return getToken(CypherParser.READ, 0);
}
public TerminalNode ONLY() {
return getToken(CypherParser.ONLY, 0);
}
public TerminalNode WRITE() {
return getToken(CypherParser.WRITE, 0);
}
public AlterDatabaseAccessContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_alterDatabaseAccess;
}
}
public final AlterDatabaseAccessContext alterDatabaseAccess() throws RecognitionException {
AlterDatabaseAccessContext _localctx = new AlterDatabaseAccessContext(_ctx, getState());
enterRule(_localctx, 528, RULE_alterDatabaseAccess);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3243);
match(ACCESS);
setState(3244);
match(READ);
setState(3245);
_la = _input.LA(1);
if (!(_la == ONLY || _la == WRITE)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterDatabaseTopologyContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode TOPOLOGY() {
return getToken(CypherParser.TOPOLOGY, 0);
}
public List primaryTopology() {
return getRuleContexts(PrimaryTopologyContext.class);
}
public PrimaryTopologyContext primaryTopology(int i) {
return getRuleContext(PrimaryTopologyContext.class, i);
}
public List secondaryTopology() {
return getRuleContexts(SecondaryTopologyContext.class);
}
public SecondaryTopologyContext secondaryTopology(int i) {
return getRuleContext(SecondaryTopologyContext.class, i);
}
public AlterDatabaseTopologyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_alterDatabaseTopology;
}
}
public final AlterDatabaseTopologyContext alterDatabaseTopology() throws RecognitionException {
AlterDatabaseTopologyContext _localctx = new AlterDatabaseTopologyContext(_ctx, getState());
enterRule(_localctx, 530, RULE_alterDatabaseTopology);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3247);
match(TOPOLOGY);
setState(3250);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
setState(3250);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 411, _ctx)) {
case 1:
{
setState(3248);
primaryTopology();
}
break;
case 2:
{
setState(3249);
secondaryTopology();
}
break;
}
}
setState(3252);
_errHandler.sync(this);
_la = _input.LA(1);
} while (_la == UNSIGNED_DECIMAL_INTEGER);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterDatabaseOptionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode OPTION() {
return getToken(CypherParser.OPTION, 0);
}
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class, 0);
}
public AlterDatabaseOptionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_alterDatabaseOption;
}
}
public final AlterDatabaseOptionContext alterDatabaseOption() throws RecognitionException {
AlterDatabaseOptionContext _localctx = new AlterDatabaseOptionContext(_ctx, getState());
enterRule(_localctx, 532, RULE_alterDatabaseOption);
try {
enterOuterAlt(_localctx, 1);
{
setState(3254);
match(OPTION);
setState(3255);
symbolicNameString();
setState(3256);
expression();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StartDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode START() {
return getToken(CypherParser.START, 0);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class, 0);
}
public WaitClauseContext waitClause() {
return getRuleContext(WaitClauseContext.class, 0);
}
public StartDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_startDatabase;
}
}
public final StartDatabaseContext startDatabase() throws RecognitionException {
StartDatabaseContext _localctx = new StartDatabaseContext(_ctx, getState());
enterRule(_localctx, 534, RULE_startDatabase);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3258);
match(START);
setState(3259);
match(DATABASE);
setState(3260);
symbolicAliasNameOrParameter();
setState(3262);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == NOWAIT || _la == WAIT) {
{
setState(3261);
waitClause();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StopDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode STOP() {
return getToken(CypherParser.STOP, 0);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class, 0);
}
public WaitClauseContext waitClause() {
return getRuleContext(WaitClauseContext.class, 0);
}
public StopDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_stopDatabase;
}
}
public final StopDatabaseContext stopDatabase() throws RecognitionException {
StopDatabaseContext _localctx = new StopDatabaseContext(_ctx, getState());
enterRule(_localctx, 536, RULE_stopDatabase);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3264);
match(STOP);
setState(3265);
match(DATABASE);
setState(3266);
symbolicAliasNameOrParameter();
setState(3268);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == NOWAIT || _la == WAIT) {
{
setState(3267);
waitClause();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class WaitClauseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode WAIT() {
return getToken(CypherParser.WAIT, 0);
}
public TerminalNode UNSIGNED_DECIMAL_INTEGER() {
return getToken(CypherParser.UNSIGNED_DECIMAL_INTEGER, 0);
}
public TerminalNode SECONDS() {
return getToken(CypherParser.SECONDS, 0);
}
public TerminalNode NOWAIT() {
return getToken(CypherParser.NOWAIT, 0);
}
public WaitClauseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_waitClause;
}
}
public final WaitClauseContext waitClause() throws RecognitionException {
WaitClauseContext _localctx = new WaitClauseContext(_ctx, getState());
enterRule(_localctx, 538, RULE_waitClause);
int _la;
try {
setState(3278);
_errHandler.sync(this);
switch (_input.LA(1)) {
case WAIT:
enterOuterAlt(_localctx, 1);
{
setState(3270);
match(WAIT);
setState(3275);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == UNSIGNED_DECIMAL_INTEGER) {
{
setState(3271);
match(UNSIGNED_DECIMAL_INTEGER);
setState(3273);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == SECONDS) {
{
setState(3272);
match(SECONDS);
}
}
}
}
}
break;
case NOWAIT:
enterOuterAlt(_localctx, 2);
{
setState(3277);
match(NOWAIT);
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowDatabaseContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public TerminalNode DEFAULT() {
return getToken(CypherParser.DEFAULT, 0);
}
public TerminalNode HOME() {
return getToken(CypherParser.HOME, 0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class, 0);
}
public TerminalNode DATABASES() {
return getToken(CypherParser.DATABASES, 0);
}
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class, 0);
}
public ShowDatabaseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showDatabase;
}
}
public final ShowDatabaseContext showDatabase() throws RecognitionException {
ShowDatabaseContext _localctx = new ShowDatabaseContext(_ctx, getState());
enterRule(_localctx, 540, RULE_showDatabase);
int _la;
try {
setState(3292);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DEFAULT:
case HOME:
enterOuterAlt(_localctx, 1);
{
setState(3280);
_la = _input.LA(1);
if (!(_la == DEFAULT || _la == HOME)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3281);
match(DATABASE);
setState(3283);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(3282);
showCommandYield();
}
}
}
break;
case DATABASE:
case DATABASES:
enterOuterAlt(_localctx, 2);
{
setState(3285);
_la = _input.LA(1);
if (!(_la == DATABASE || _la == DATABASES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3287);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 419, _ctx)) {
case 1:
{
setState(3286);
symbolicAliasNameOrParameter();
}
break;
}
setState(3290);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(3289);
showCommandYield();
}
}
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DatabaseScopeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public TerminalNode DEFAULT() {
return getToken(CypherParser.DEFAULT, 0);
}
public TerminalNode HOME() {
return getToken(CypherParser.HOME, 0);
}
public TerminalNode DATABASES() {
return getToken(CypherParser.DATABASES, 0);
}
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public SymbolicAliasNameListContext symbolicAliasNameList() {
return getRuleContext(SymbolicAliasNameListContext.class, 0);
}
public DatabaseScopeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_databaseScope;
}
}
public final DatabaseScopeContext databaseScope() throws RecognitionException {
DatabaseScopeContext _localctx = new DatabaseScopeContext(_ctx, getState());
enterRule(_localctx, 542, RULE_databaseScope);
int _la;
try {
setState(3301);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DEFAULT:
case HOME:
enterOuterAlt(_localctx, 1);
{
setState(3294);
_la = _input.LA(1);
if (!(_la == DEFAULT || _la == HOME)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3295);
match(DATABASE);
}
break;
case DATABASE:
case DATABASES:
enterOuterAlt(_localctx, 2);
{
setState(3296);
_la = _input.LA(1);
if (!(_la == DATABASE || _la == DATABASES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3299);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(3297);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
{
setState(3298);
symbolicAliasNameList();
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GraphScopeContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode GRAPH() {
return getToken(CypherParser.GRAPH, 0);
}
public TerminalNode DEFAULT() {
return getToken(CypherParser.DEFAULT, 0);
}
public TerminalNode HOME() {
return getToken(CypherParser.HOME, 0);
}
public TerminalNode GRAPHS() {
return getToken(CypherParser.GRAPHS, 0);
}
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public SymbolicAliasNameListContext symbolicAliasNameList() {
return getRuleContext(SymbolicAliasNameListContext.class, 0);
}
public GraphScopeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_graphScope;
}
}
public final GraphScopeContext graphScope() throws RecognitionException {
GraphScopeContext _localctx = new GraphScopeContext(_ctx, getState());
enterRule(_localctx, 544, RULE_graphScope);
int _la;
try {
setState(3310);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DEFAULT:
case HOME:
enterOuterAlt(_localctx, 1);
{
setState(3303);
_la = _input.LA(1);
if (!(_la == DEFAULT || _la == HOME)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3304);
match(GRAPH);
}
break;
case GRAPH:
case GRAPHS:
enterOuterAlt(_localctx, 2);
{
setState(3305);
_la = _input.LA(1);
if (!(_la == GRAPH || _la == GRAPHS)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3308);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TIMES:
{
setState(3306);
match(TIMES);
}
break;
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
{
setState(3307);
symbolicAliasNameList();
}
break;
default:
throw new NoViableAltException(this);
}
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CommandOptionsContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode OPTIONS() {
return getToken(CypherParser.OPTIONS, 0);
}
public MapOrParameterContext mapOrParameter() {
return getRuleContext(MapOrParameterContext.class, 0);
}
public CommandOptionsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_commandOptions;
}
}
public final CommandOptionsContext commandOptions() throws RecognitionException {
CommandOptionsContext _localctx = new CommandOptionsContext(_ctx, getState());
enterRule(_localctx, 546, RULE_commandOptions);
try {
enterOuterAlt(_localctx, 1);
{
setState(3312);
match(OPTIONS);
setState(3313);
mapOrParameter();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CommandNameExpressionContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class, 0);
}
public CommandNameExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_commandNameExpression;
}
}
public final CommandNameExpressionContext commandNameExpression() throws RecognitionException {
CommandNameExpressionContext _localctx = new CommandNameExpressionContext(_ctx, getState());
enterRule(_localctx, 548, RULE_commandNameExpression);
try {
setState(3317);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 1);
{
setState(3315);
symbolicNameString();
}
break;
case DOLLAR:
enterOuterAlt(_localctx, 2);
{
setState(3316);
parameter("STRING");
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SymbolicNameOrStringParameterContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicNameStringContext symbolicNameString() {
return getRuleContext(SymbolicNameStringContext.class, 0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class, 0);
}
public SymbolicNameOrStringParameterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_symbolicNameOrStringParameter;
}
}
public final SymbolicNameOrStringParameterContext symbolicNameOrStringParameter() throws RecognitionException {
SymbolicNameOrStringParameterContext _localctx = new SymbolicNameOrStringParameterContext(_ctx, getState());
enterRule(_localctx, 550, RULE_symbolicNameOrStringParameter);
try {
setState(3321);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 1);
{
setState(3319);
symbolicNameString();
}
break;
case DOLLAR:
enterOuterAlt(_localctx, 2);
{
setState(3320);
parameter("STRING");
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class CreateAliasContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ALIAS() {
return getToken(CypherParser.ALIAS, 0);
}
public List symbolicAliasNameOrParameter() {
return getRuleContexts(SymbolicAliasNameOrParameterContext.class);
}
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter(int i) {
return getRuleContext(SymbolicAliasNameOrParameterContext.class, i);
}
public TerminalNode FOR() {
return getToken(CypherParser.FOR, 0);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public TerminalNode AT() {
return getToken(CypherParser.AT, 0);
}
public StringOrParameterContext stringOrParameter() {
return getRuleContext(StringOrParameterContext.class, 0);
}
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public CommandNameExpressionContext commandNameExpression() {
return getRuleContext(CommandNameExpressionContext.class, 0);
}
public TerminalNode PASSWORD() {
return getToken(CypherParser.PASSWORD, 0);
}
public PasswordExpressionContext passwordExpression() {
return getRuleContext(PasswordExpressionContext.class, 0);
}
public TerminalNode PROPERTIES() {
return getToken(CypherParser.PROPERTIES, 0);
}
public List mapOrParameter() {
return getRuleContexts(MapOrParameterContext.class);
}
public MapOrParameterContext mapOrParameter(int i) {
return getRuleContext(MapOrParameterContext.class, i);
}
public TerminalNode DRIVER() {
return getToken(CypherParser.DRIVER, 0);
}
public CreateAliasContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_createAlias;
}
}
public final CreateAliasContext createAlias() throws RecognitionException {
CreateAliasContext _localctx = new CreateAliasContext(_ctx, getState());
enterRule(_localctx, 552, RULE_createAlias);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3323);
match(ALIAS);
setState(3324);
symbolicAliasNameOrParameter();
setState(3328);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(3325);
match(IF);
setState(3326);
match(NOT);
setState(3327);
match(EXISTS);
}
}
setState(3330);
match(FOR);
setState(3331);
match(DATABASE);
setState(3332);
symbolicAliasNameOrParameter();
setState(3343);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == AT) {
{
setState(3333);
match(AT);
setState(3334);
stringOrParameter();
setState(3335);
match(USER);
setState(3336);
commandNameExpression();
setState(3337);
match(PASSWORD);
setState(3338);
passwordExpression();
setState(3341);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == DRIVER) {
{
setState(3339);
match(DRIVER);
setState(3340);
mapOrParameter();
}
}
}
}
setState(3347);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == PROPERTIES) {
{
setState(3345);
match(PROPERTIES);
setState(3346);
mapOrParameter();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DropAliasContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ALIAS() {
return getToken(CypherParser.ALIAS, 0);
}
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class, 0);
}
public TerminalNode FOR() {
return getToken(CypherParser.FOR, 0);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public DropAliasContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_dropAlias;
}
}
public final DropAliasContext dropAlias() throws RecognitionException {
DropAliasContext _localctx = new DropAliasContext(_ctx, getState());
enterRule(_localctx, 554, RULE_dropAlias);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3349);
match(ALIAS);
setState(3350);
symbolicAliasNameOrParameter();
setState(3353);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(3351);
match(IF);
setState(3352);
match(EXISTS);
}
}
setState(3355);
match(FOR);
setState(3356);
match(DATABASE);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterAliasContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ALIAS() {
return getToken(CypherParser.ALIAS, 0);
}
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class, 0);
}
public TerminalNode SET() {
return getToken(CypherParser.SET, 0);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public List alterAliasTarget() {
return getRuleContexts(AlterAliasTargetContext.class);
}
public AlterAliasTargetContext alterAliasTarget(int i) {
return getRuleContext(AlterAliasTargetContext.class, i);
}
public List alterAliasUser() {
return getRuleContexts(AlterAliasUserContext.class);
}
public AlterAliasUserContext alterAliasUser(int i) {
return getRuleContext(AlterAliasUserContext.class, i);
}
public List alterAliasPassword() {
return getRuleContexts(AlterAliasPasswordContext.class);
}
public AlterAliasPasswordContext alterAliasPassword(int i) {
return getRuleContext(AlterAliasPasswordContext.class, i);
}
public List alterAliasDriver() {
return getRuleContexts(AlterAliasDriverContext.class);
}
public AlterAliasDriverContext alterAliasDriver(int i) {
return getRuleContext(AlterAliasDriverContext.class, i);
}
public List alterAliasProperties() {
return getRuleContexts(AlterAliasPropertiesContext.class);
}
public AlterAliasPropertiesContext alterAliasProperties(int i) {
return getRuleContext(AlterAliasPropertiesContext.class, i);
}
public AlterAliasContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_alterAlias;
}
}
public final AlterAliasContext alterAlias() throws RecognitionException {
AlterAliasContext _localctx = new AlterAliasContext(_ctx, getState());
enterRule(_localctx, 556, RULE_alterAlias);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3358);
match(ALIAS);
setState(3359);
symbolicAliasNameOrParameter();
setState(3362);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == IF) {
{
setState(3360);
match(IF);
setState(3361);
match(EXISTS);
}
}
setState(3364);
match(SET);
setState(3365);
match(DATABASE);
setState(3371);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
setState(3371);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TARGET:
{
setState(3366);
alterAliasTarget();
}
break;
case USER:
{
setState(3367);
alterAliasUser();
}
break;
case PASSWORD:
{
setState(3368);
alterAliasPassword();
}
break;
case DRIVER:
{
setState(3369);
alterAliasDriver();
}
break;
case PROPERTIES:
{
setState(3370);
alterAliasProperties();
}
break;
default:
throw new NoViableAltException(this);
}
}
setState(3373);
_errHandler.sync(this);
_la = _input.LA(1);
} while (_la == DRIVER || _la == PASSWORD || _la == PROPERTIES || _la == TARGET || _la == USER);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterAliasTargetContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode TARGET() {
return getToken(CypherParser.TARGET, 0);
}
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class, 0);
}
public TerminalNode AT() {
return getToken(CypherParser.AT, 0);
}
public StringOrParameterContext stringOrParameter() {
return getRuleContext(StringOrParameterContext.class, 0);
}
public AlterAliasTargetContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_alterAliasTarget;
}
}
public final AlterAliasTargetContext alterAliasTarget() throws RecognitionException {
AlterAliasTargetContext _localctx = new AlterAliasTargetContext(_ctx, getState());
enterRule(_localctx, 558, RULE_alterAliasTarget);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3375);
match(TARGET);
setState(3376);
symbolicAliasNameOrParameter();
setState(3379);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == AT) {
{
setState(3377);
match(AT);
setState(3378);
stringOrParameter();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterAliasUserContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public CommandNameExpressionContext commandNameExpression() {
return getRuleContext(CommandNameExpressionContext.class, 0);
}
public AlterAliasUserContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_alterAliasUser;
}
}
public final AlterAliasUserContext alterAliasUser() throws RecognitionException {
AlterAliasUserContext _localctx = new AlterAliasUserContext(_ctx, getState());
enterRule(_localctx, 560, RULE_alterAliasUser);
try {
enterOuterAlt(_localctx, 1);
{
setState(3381);
match(USER);
setState(3382);
commandNameExpression();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterAliasPasswordContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PASSWORD() {
return getToken(CypherParser.PASSWORD, 0);
}
public PasswordExpressionContext passwordExpression() {
return getRuleContext(PasswordExpressionContext.class, 0);
}
public AlterAliasPasswordContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_alterAliasPassword;
}
}
public final AlterAliasPasswordContext alterAliasPassword() throws RecognitionException {
AlterAliasPasswordContext _localctx = new AlterAliasPasswordContext(_ctx, getState());
enterRule(_localctx, 562, RULE_alterAliasPassword);
try {
enterOuterAlt(_localctx, 1);
{
setState(3384);
match(PASSWORD);
setState(3385);
passwordExpression();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterAliasDriverContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DRIVER() {
return getToken(CypherParser.DRIVER, 0);
}
public MapOrParameterContext mapOrParameter() {
return getRuleContext(MapOrParameterContext.class, 0);
}
public AlterAliasDriverContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_alterAliasDriver;
}
}
public final AlterAliasDriverContext alterAliasDriver() throws RecognitionException {
AlterAliasDriverContext _localctx = new AlterAliasDriverContext(_ctx, getState());
enterRule(_localctx, 564, RULE_alterAliasDriver);
try {
enterOuterAlt(_localctx, 1);
{
setState(3387);
match(DRIVER);
setState(3388);
mapOrParameter();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AlterAliasPropertiesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode PROPERTIES() {
return getToken(CypherParser.PROPERTIES, 0);
}
public MapOrParameterContext mapOrParameter() {
return getRuleContext(MapOrParameterContext.class, 0);
}
public AlterAliasPropertiesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_alterAliasProperties;
}
}
public final AlterAliasPropertiesContext alterAliasProperties() throws RecognitionException {
AlterAliasPropertiesContext _localctx = new AlterAliasPropertiesContext(_ctx, getState());
enterRule(_localctx, 566, RULE_alterAliasProperties);
try {
enterOuterAlt(_localctx, 1);
{
setState(3390);
match(PROPERTIES);
setState(3391);
mapOrParameter();
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ShowAliasesContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode FOR() {
return getToken(CypherParser.FOR, 0);
}
public TerminalNode ALIAS() {
return getToken(CypherParser.ALIAS, 0);
}
public TerminalNode ALIASES() {
return getToken(CypherParser.ALIASES, 0);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public TerminalNode DATABASES() {
return getToken(CypherParser.DATABASES, 0);
}
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() {
return getRuleContext(SymbolicAliasNameOrParameterContext.class, 0);
}
public ShowCommandYieldContext showCommandYield() {
return getRuleContext(ShowCommandYieldContext.class, 0);
}
public ShowAliasesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_showAliases;
}
}
public final ShowAliasesContext showAliases() throws RecognitionException {
ShowAliasesContext _localctx = new ShowAliasesContext(_ctx, getState());
enterRule(_localctx, 568, RULE_showAliases);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3393);
_la = _input.LA(1);
if (!(_la == ALIAS || _la == ALIASES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3395);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 437, _ctx)) {
case 1:
{
setState(3394);
symbolicAliasNameOrParameter();
}
break;
}
setState(3397);
match(FOR);
setState(3398);
_la = _input.LA(1);
if (!(_la == DATABASE || _la == DATABASES)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(3400);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == WHERE || _la == YIELD) {
{
setState(3399);
showCommandYield();
}
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SymbolicAliasNameListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List symbolicAliasNameOrParameter() {
return getRuleContexts(SymbolicAliasNameOrParameterContext.class);
}
public SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter(int i) {
return getRuleContext(SymbolicAliasNameOrParameterContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public SymbolicAliasNameListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_symbolicAliasNameList;
}
}
public final SymbolicAliasNameListContext symbolicAliasNameList() throws RecognitionException {
SymbolicAliasNameListContext _localctx = new SymbolicAliasNameListContext(_ctx, getState());
enterRule(_localctx, 570, RULE_symbolicAliasNameList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3402);
symbolicAliasNameOrParameter();
setState(3407);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(3403);
match(COMMA);
setState(3404);
symbolicAliasNameOrParameter();
}
}
setState(3409);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SymbolicAliasNameOrParameterContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public SymbolicAliasNameContext symbolicAliasName() {
return getRuleContext(SymbolicAliasNameContext.class, 0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class, 0);
}
public SymbolicAliasNameOrParameterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_symbolicAliasNameOrParameter;
}
}
public final SymbolicAliasNameOrParameterContext symbolicAliasNameOrParameter() throws RecognitionException {
SymbolicAliasNameOrParameterContext _localctx = new SymbolicAliasNameOrParameterContext(_ctx, getState());
enterRule(_localctx, 572, RULE_symbolicAliasNameOrParameter);
try {
setState(3412);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 1);
{
setState(3410);
symbolicAliasName();
}
break;
case DOLLAR:
enterOuterAlt(_localctx, 2);
{
setState(3411);
parameter("STRING");
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SymbolicAliasNameContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List symbolicNameString() {
return getRuleContexts(SymbolicNameStringContext.class);
}
public SymbolicNameStringContext symbolicNameString(int i) {
return getRuleContext(SymbolicNameStringContext.class, i);
}
public List DOT() {
return getTokens(CypherParser.DOT);
}
public TerminalNode DOT(int i) {
return getToken(CypherParser.DOT, i);
}
public SymbolicAliasNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_symbolicAliasName;
}
}
public final SymbolicAliasNameContext symbolicAliasName() throws RecognitionException {
SymbolicAliasNameContext _localctx = new SymbolicAliasNameContext(_ctx, getState());
enterRule(_localctx, 574, RULE_symbolicAliasName);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3414);
symbolicNameString();
setState(3419);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == DOT) {
{
{
setState(3415);
match(DOT);
setState(3416);
symbolicNameString();
}
}
setState(3421);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SymbolicNameOrStringParameterListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List commandNameExpression() {
return getRuleContexts(CommandNameExpressionContext.class);
}
public CommandNameExpressionContext commandNameExpression(int i) {
return getRuleContext(CommandNameExpressionContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public SymbolicNameOrStringParameterListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_symbolicNameOrStringParameterList;
}
}
public final SymbolicNameOrStringParameterListContext symbolicNameOrStringParameterList()
throws RecognitionException {
SymbolicNameOrStringParameterListContext _localctx =
new SymbolicNameOrStringParameterListContext(_ctx, getState());
enterRule(_localctx, 576, RULE_symbolicNameOrStringParameterList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3422);
commandNameExpression();
setState(3427);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(3423);
match(COMMA);
setState(3424);
commandNameExpression();
}
}
setState(3429);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GlobContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public EscapedSymbolicNameStringContext escapedSymbolicNameString() {
return getRuleContext(EscapedSymbolicNameStringContext.class, 0);
}
public GlobRecursiveContext globRecursive() {
return getRuleContext(GlobRecursiveContext.class, 0);
}
public GlobContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_glob;
}
}
public final GlobContext glob() throws RecognitionException {
GlobContext _localctx = new GlobContext(_ctx, getState());
enterRule(_localctx, 578, RULE_glob);
try {
setState(3435);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
enterOuterAlt(_localctx, 1);
{
setState(3430);
escapedSymbolicNameString();
setState(3432);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 443, _ctx)) {
case 1:
{
setState(3431);
globRecursive();
}
break;
}
}
break;
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DOT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case QUESTION:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMES:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 2);
{
setState(3434);
globRecursive();
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GlobRecursiveContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public GlobPartContext globPart() {
return getRuleContext(GlobPartContext.class, 0);
}
public GlobRecursiveContext globRecursive() {
return getRuleContext(GlobRecursiveContext.class, 0);
}
public GlobRecursiveContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_globRecursive;
}
}
public final GlobRecursiveContext globRecursive() throws RecognitionException {
GlobRecursiveContext _localctx = new GlobRecursiveContext(_ctx, getState());
enterRule(_localctx, 580, RULE_globRecursive);
try {
enterOuterAlt(_localctx, 1);
{
setState(3437);
globPart();
setState(3439);
_errHandler.sync(this);
switch (getInterpreter().adaptivePredict(_input, 445, _ctx)) {
case 1:
{
setState(3438);
globRecursive();
}
break;
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GlobPartContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode DOT() {
return getToken(CypherParser.DOT, 0);
}
public EscapedSymbolicNameStringContext escapedSymbolicNameString() {
return getRuleContext(EscapedSymbolicNameStringContext.class, 0);
}
public TerminalNode QUESTION() {
return getToken(CypherParser.QUESTION, 0);
}
public TerminalNode TIMES() {
return getToken(CypherParser.TIMES, 0);
}
public UnescapedSymbolicNameStringContext unescapedSymbolicNameString() {
return getRuleContext(UnescapedSymbolicNameStringContext.class, 0);
}
public GlobPartContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_globPart;
}
}
public final GlobPartContext globPart() throws RecognitionException {
GlobPartContext _localctx = new GlobPartContext(_ctx, getState());
enterRule(_localctx, 582, RULE_globPart);
int _la;
try {
setState(3448);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DOT:
enterOuterAlt(_localctx, 1);
{
setState(3441);
match(DOT);
setState(3443);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la == ESCAPED_SYMBOLIC_NAME) {
{
setState(3442);
escapedSymbolicNameString();
}
}
}
break;
case QUESTION:
enterOuterAlt(_localctx, 2);
{
setState(3445);
match(QUESTION);
}
break;
case TIMES:
enterOuterAlt(_localctx, 3);
{
setState(3446);
match(TIMES);
}
break;
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 4);
{
setState(3447);
unescapedSymbolicNameString();
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StringListContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public List stringLiteral() {
return getRuleContexts(StringLiteralContext.class);
}
public StringLiteralContext stringLiteral(int i) {
return getRuleContext(StringLiteralContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public StringListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_stringList;
}
}
public final StringListContext stringList() throws RecognitionException {
StringListContext _localctx = new StringListContext(_ctx, getState());
enterRule(_localctx, 584, RULE_stringList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3450);
stringLiteral();
setState(3453);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(3451);
match(COMMA);
setState(3452);
stringLiteral();
}
}
setState(3455);
_errHandler.sync(this);
_la = _input.LA(1);
} while (_la == COMMA);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StringLiteralContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode STRING_LITERAL1() {
return getToken(CypherParser.STRING_LITERAL1, 0);
}
public TerminalNode STRING_LITERAL2() {
return getToken(CypherParser.STRING_LITERAL2, 0);
}
public StringLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_stringLiteral;
}
}
public final StringLiteralContext stringLiteral() throws RecognitionException {
StringLiteralContext _localctx = new StringLiteralContext(_ctx, getState());
enterRule(_localctx, 586, RULE_stringLiteral);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3457);
_la = _input.LA(1);
if (!(_la == STRING_LITERAL1 || _la == STRING_LITERAL2)) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StringOrParameterContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class, 0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class, 0);
}
public StringOrParameterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_stringOrParameter;
}
}
public final StringOrParameterContext stringOrParameter() throws RecognitionException {
StringOrParameterContext _localctx = new StringOrParameterContext(_ctx, getState());
enterRule(_localctx, 588, RULE_stringOrParameter);
try {
setState(3461);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STRING_LITERAL1:
case STRING_LITERAL2:
enterOuterAlt(_localctx, 1);
{
setState(3459);
stringLiteral();
}
break;
case DOLLAR:
enterOuterAlt(_localctx, 2);
{
setState(3460);
parameter("STRING");
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MapOrParameterContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public MapContext map() {
return getRuleContext(MapContext.class, 0);
}
public ParameterContext parameter() {
return getRuleContext(ParameterContext.class, 0);
}
public MapOrParameterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_mapOrParameter;
}
}
public final MapOrParameterContext mapOrParameter() throws RecognitionException {
MapOrParameterContext _localctx = new MapOrParameterContext(_ctx, getState());
enterRule(_localctx, 590, RULE_mapOrParameter);
try {
setState(3465);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LCURLY:
enterOuterAlt(_localctx, 1);
{
setState(3463);
map();
}
break;
case DOLLAR:
enterOuterAlt(_localctx, 2);
{
setState(3464);
parameter("MAP");
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MapContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode LCURLY() {
return getToken(CypherParser.LCURLY, 0);
}
public TerminalNode RCURLY() {
return getToken(CypherParser.RCURLY, 0);
}
public List propertyKeyName() {
return getRuleContexts(PropertyKeyNameContext.class);
}
public PropertyKeyNameContext propertyKeyName(int i) {
return getRuleContext(PropertyKeyNameContext.class, i);
}
public List COLON() {
return getTokens(CypherParser.COLON);
}
public TerminalNode COLON(int i) {
return getToken(CypherParser.COLON, i);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class, i);
}
public List COMMA() {
return getTokens(CypherParser.COMMA);
}
public TerminalNode COMMA(int i) {
return getToken(CypherParser.COMMA, i);
}
public MapContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_map;
}
}
public final MapContext map() throws RecognitionException {
MapContext _localctx = new MapContext(_ctx, getState());
enterRule(_localctx, 592, RULE_map);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3467);
match(LCURLY);
setState(3481);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & -61572919591936L) != 0)
|| ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -9288676379060225L) != 0)
|| ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -3458764514072989569L) != 0)
|| ((((_la - 193)) & ~0x3f) == 0 && ((1L << (_la - 193)) & -576460823170418305L) != 0)
|| ((((_la - 257)) & ~0x3f) == 0 && ((1L << (_la - 257)) & 4294967295L) != 0)) {
{
setState(3468);
propertyKeyName();
setState(3469);
match(COLON);
setState(3470);
expression();
setState(3478);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la == COMMA) {
{
{
setState(3471);
match(COMMA);
setState(3472);
propertyKeyName();
setState(3473);
match(COLON);
setState(3474);
expression();
}
}
setState(3480);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(3483);
match(RCURLY);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SymbolicNameStringContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public EscapedSymbolicNameStringContext escapedSymbolicNameString() {
return getRuleContext(EscapedSymbolicNameStringContext.class, 0);
}
public UnescapedSymbolicNameStringContext unescapedSymbolicNameString() {
return getRuleContext(UnescapedSymbolicNameStringContext.class, 0);
}
public SymbolicNameStringContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_symbolicNameString;
}
}
public final SymbolicNameStringContext symbolicNameString() throws RecognitionException {
SymbolicNameStringContext _localctx = new SymbolicNameStringContext(_ctx, getState());
enterRule(_localctx, 594, RULE_symbolicNameString);
try {
setState(3487);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
enterOuterAlt(_localctx, 1);
{
setState(3485);
escapedSymbolicNameString();
}
break;
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NFC:
case NFD:
case NFKC:
case NFKD:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NORMALIZED:
case NOT:
case NOTHING:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPED:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 2);
{
setState(3486);
unescapedSymbolicNameString();
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class EscapedSymbolicNameStringContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode ESCAPED_SYMBOLIC_NAME() {
return getToken(CypherParser.ESCAPED_SYMBOLIC_NAME, 0);
}
public EscapedSymbolicNameStringContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_escapedSymbolicNameString;
}
}
public final EscapedSymbolicNameStringContext escapedSymbolicNameString() throws RecognitionException {
EscapedSymbolicNameStringContext _localctx = new EscapedSymbolicNameStringContext(_ctx, getState());
enterRule(_localctx, 596, RULE_escapedSymbolicNameString);
try {
enterOuterAlt(_localctx, 1);
{
setState(3489);
match(ESCAPED_SYMBOLIC_NAME);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UnescapedSymbolicNameStringContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public UnescapedLabelSymbolicNameStringContext unescapedLabelSymbolicNameString() {
return getRuleContext(UnescapedLabelSymbolicNameStringContext.class, 0);
}
public TerminalNode NOT() {
return getToken(CypherParser.NOT, 0);
}
public TerminalNode NULL() {
return getToken(CypherParser.NULL, 0);
}
public TerminalNode TYPED() {
return getToken(CypherParser.TYPED, 0);
}
public TerminalNode NORMALIZED() {
return getToken(CypherParser.NORMALIZED, 0);
}
public TerminalNode NFC() {
return getToken(CypherParser.NFC, 0);
}
public TerminalNode NFD() {
return getToken(CypherParser.NFD, 0);
}
public TerminalNode NFKC() {
return getToken(CypherParser.NFKC, 0);
}
public TerminalNode NFKD() {
return getToken(CypherParser.NFKD, 0);
}
public UnescapedSymbolicNameStringContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_unescapedSymbolicNameString;
}
}
public final UnescapedSymbolicNameStringContext unescapedSymbolicNameString() throws RecognitionException {
UnescapedSymbolicNameStringContext _localctx = new UnescapedSymbolicNameStringContext(_ctx, getState());
enterRule(_localctx, 598, RULE_unescapedSymbolicNameString);
try {
setState(3500);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NOTHING:
case NOWAIT:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 1);
{
setState(3491);
unescapedLabelSymbolicNameString();
}
break;
case NOT:
enterOuterAlt(_localctx, 2);
{
setState(3492);
match(NOT);
}
break;
case NULL:
enterOuterAlt(_localctx, 3);
{
setState(3493);
match(NULL);
}
break;
case TYPED:
enterOuterAlt(_localctx, 4);
{
setState(3494);
match(TYPED);
}
break;
case NORMALIZED:
enterOuterAlt(_localctx, 5);
{
setState(3495);
match(NORMALIZED);
}
break;
case NFC:
enterOuterAlt(_localctx, 6);
{
setState(3496);
match(NFC);
}
break;
case NFD:
enterOuterAlt(_localctx, 7);
{
setState(3497);
match(NFD);
}
break;
case NFKC:
enterOuterAlt(_localctx, 8);
{
setState(3498);
match(NFKC);
}
break;
case NFKD:
enterOuterAlt(_localctx, 9);
{
setState(3499);
match(NFKD);
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SymbolicLabelNameStringContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public EscapedSymbolicNameStringContext escapedSymbolicNameString() {
return getRuleContext(EscapedSymbolicNameStringContext.class, 0);
}
public UnescapedLabelSymbolicNameStringContext unescapedLabelSymbolicNameString() {
return getRuleContext(UnescapedLabelSymbolicNameStringContext.class, 0);
}
public SymbolicLabelNameStringContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_symbolicLabelNameString;
}
}
public final SymbolicLabelNameStringContext symbolicLabelNameString() throws RecognitionException {
SymbolicLabelNameStringContext _localctx = new SymbolicLabelNameStringContext(_ctx, getState());
enterRule(_localctx, 600, RULE_symbolicLabelNameString);
try {
setState(3504);
_errHandler.sync(this);
switch (_input.LA(1)) {
case ESCAPED_SYMBOLIC_NAME:
enterOuterAlt(_localctx, 1);
{
setState(3502);
escapedSymbolicNameString();
}
break;
case ACCESS:
case ACTIVE:
case ADMIN:
case ADMINISTRATOR:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATHS:
case ALL:
case ALTER:
case AND:
case ANY:
case ARRAY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BINDINGS:
case BOOLEAN:
case BOOSTED:
case BOTH:
case BREAK:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CHANGE:
case CIDR:
case COLLECT:
case COMMAND:
case COMMANDS:
case COMMIT:
case COMPOSITE:
case CONCURRENT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case CONTINUE:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DATE:
case DATETIME:
case DBMS:
case DEALLOCATE:
case DEFAULT:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DIFFERENT:
case DISTINCT:
case DRIVER:
case DROP:
case DRYRUN:
case DUMP:
case DURATION:
case EACH:
case EDGE:
case ENABLE:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXECUTE:
case EXIST:
case EXISTENCE:
case EXISTS:
case ERROR:
case FAIL:
case FALSE:
case FIELDTERMINATOR:
case FINISH:
case FLOAT:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case GROUP:
case HEADERS:
case HOME:
case IF:
case IMPERSONATE:
case IMMUTABLE:
case IN:
case INDEX:
case INDEXES:
case INFINITY:
case INSERT:
case INT:
case INTEGER:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LEADING:
case LIMITROWS:
case LIST:
case LOAD:
case LOCAL:
case LOOKUP:
case MANAGEMENT:
case MAP:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NAN:
case NEW:
case NODE:
case NODETACH:
case NODES:
case NONE:
case NORMALIZE:
case NOTHING:
case NOWAIT:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OPTION:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PATH:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIMARY:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTIES:
case PROPERTY:
case RANGE:
case READ:
case REALLOCATE:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPEATABLE:
case REPLACE:
case REPORT:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SECONDARY:
case SECONDS:
case SEEK:
case SERVER:
case SERVERS:
case SET:
case SETTING:
case SHORTEST_PATH:
case SHORTEST:
case SHOW:
case SIGNED:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case STRING:
case SUPPORTED:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TIME:
case TIMESTAMP:
case TIMEZONE:
case TO:
case TOPOLOGY:
case TRAILING:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRIM:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNIQUENESS:
case UNWIND:
case URL:
case USE:
case USER:
case USERS:
case USING:
case VALUE:
case VECTOR:
case VERBOSE:
case VERTEX:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WITHOUT:
case WRITE:
case XOR:
case YIELD:
case ZONED:
case IDENTIFIER:
enterOuterAlt(_localctx, 2);
{
setState(3503);
unescapedLabelSymbolicNameString();
}
break;
default:
throw new NoViableAltException(this);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UnescapedLabelSymbolicNameStringContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode IDENTIFIER() {
return getToken(CypherParser.IDENTIFIER, 0);
}
public TerminalNode ACCESS() {
return getToken(CypherParser.ACCESS, 0);
}
public TerminalNode ACTIVE() {
return getToken(CypherParser.ACTIVE, 0);
}
public TerminalNode ADMIN() {
return getToken(CypherParser.ADMIN, 0);
}
public TerminalNode ADMINISTRATOR() {
return getToken(CypherParser.ADMINISTRATOR, 0);
}
public TerminalNode ALIAS() {
return getToken(CypherParser.ALIAS, 0);
}
public TerminalNode ALIASES() {
return getToken(CypherParser.ALIASES, 0);
}
public TerminalNode ALL_SHORTEST_PATHS() {
return getToken(CypherParser.ALL_SHORTEST_PATHS, 0);
}
public TerminalNode ALL() {
return getToken(CypherParser.ALL, 0);
}
public TerminalNode ALTER() {
return getToken(CypherParser.ALTER, 0);
}
public TerminalNode AND() {
return getToken(CypherParser.AND, 0);
}
public TerminalNode ANY() {
return getToken(CypherParser.ANY, 0);
}
public TerminalNode ARRAY() {
return getToken(CypherParser.ARRAY, 0);
}
public TerminalNode AS() {
return getToken(CypherParser.AS, 0);
}
public TerminalNode ASC() {
return getToken(CypherParser.ASC, 0);
}
public TerminalNode ASSERT() {
return getToken(CypherParser.ASSERT, 0);
}
public TerminalNode ASSIGN() {
return getToken(CypherParser.ASSIGN, 0);
}
public TerminalNode AT() {
return getToken(CypherParser.AT, 0);
}
public TerminalNode BINDINGS() {
return getToken(CypherParser.BINDINGS, 0);
}
public TerminalNode BOOLEAN() {
return getToken(CypherParser.BOOLEAN, 0);
}
public TerminalNode BOOSTED() {
return getToken(CypherParser.BOOSTED, 0);
}
public TerminalNode BOTH() {
return getToken(CypherParser.BOTH, 0);
}
public TerminalNode BREAK() {
return getToken(CypherParser.BREAK, 0);
}
public TerminalNode BRIEF() {
return getToken(CypherParser.BRIEF, 0);
}
public TerminalNode BTREE() {
return getToken(CypherParser.BTREE, 0);
}
public TerminalNode BUILT() {
return getToken(CypherParser.BUILT, 0);
}
public TerminalNode BY() {
return getToken(CypherParser.BY, 0);
}
public TerminalNode CALL() {
return getToken(CypherParser.CALL, 0);
}
public TerminalNode CASE() {
return getToken(CypherParser.CASE, 0);
}
public TerminalNode CHANGE() {
return getToken(CypherParser.CHANGE, 0);
}
public TerminalNode CIDR() {
return getToken(CypherParser.CIDR, 0);
}
public TerminalNode COLLECT() {
return getToken(CypherParser.COLLECT, 0);
}
public TerminalNode COMMAND() {
return getToken(CypherParser.COMMAND, 0);
}
public TerminalNode COMMANDS() {
return getToken(CypherParser.COMMANDS, 0);
}
public TerminalNode COMMIT() {
return getToken(CypherParser.COMMIT, 0);
}
public TerminalNode COMPOSITE() {
return getToken(CypherParser.COMPOSITE, 0);
}
public TerminalNode CONCURRENT() {
return getToken(CypherParser.CONCURRENT, 0);
}
public TerminalNode CONSTRAINT() {
return getToken(CypherParser.CONSTRAINT, 0);
}
public TerminalNode CONSTRAINTS() {
return getToken(CypherParser.CONSTRAINTS, 0);
}
public TerminalNode CONTAINS() {
return getToken(CypherParser.CONTAINS, 0);
}
public TerminalNode CONTINUE() {
return getToken(CypherParser.CONTINUE, 0);
}
public TerminalNode COPY() {
return getToken(CypherParser.COPY, 0);
}
public TerminalNode COUNT() {
return getToken(CypherParser.COUNT, 0);
}
public TerminalNode CREATE() {
return getToken(CypherParser.CREATE, 0);
}
public TerminalNode CSV() {
return getToken(CypherParser.CSV, 0);
}
public TerminalNode CURRENT() {
return getToken(CypherParser.CURRENT, 0);
}
public TerminalNode DATA() {
return getToken(CypherParser.DATA, 0);
}
public TerminalNode DATABASE() {
return getToken(CypherParser.DATABASE, 0);
}
public TerminalNode DATABASES() {
return getToken(CypherParser.DATABASES, 0);
}
public TerminalNode DATE() {
return getToken(CypherParser.DATE, 0);
}
public TerminalNode DATETIME() {
return getToken(CypherParser.DATETIME, 0);
}
public TerminalNode DBMS() {
return getToken(CypherParser.DBMS, 0);
}
public TerminalNode DEALLOCATE() {
return getToken(CypherParser.DEALLOCATE, 0);
}
public TerminalNode DEFAULT() {
return getToken(CypherParser.DEFAULT, 0);
}
public TerminalNode DEFINED() {
return getToken(CypherParser.DEFINED, 0);
}
public TerminalNode DELETE() {
return getToken(CypherParser.DELETE, 0);
}
public TerminalNode DENY() {
return getToken(CypherParser.DENY, 0);
}
public TerminalNode DESC() {
return getToken(CypherParser.DESC, 0);
}
public TerminalNode DESTROY() {
return getToken(CypherParser.DESTROY, 0);
}
public TerminalNode DETACH() {
return getToken(CypherParser.DETACH, 0);
}
public TerminalNode DIFFERENT() {
return getToken(CypherParser.DIFFERENT, 0);
}
public TerminalNode DISTINCT() {
return getToken(CypherParser.DISTINCT, 0);
}
public TerminalNode DRIVER() {
return getToken(CypherParser.DRIVER, 0);
}
public TerminalNode DROP() {
return getToken(CypherParser.DROP, 0);
}
public TerminalNode DRYRUN() {
return getToken(CypherParser.DRYRUN, 0);
}
public TerminalNode DUMP() {
return getToken(CypherParser.DUMP, 0);
}
public TerminalNode DURATION() {
return getToken(CypherParser.DURATION, 0);
}
public TerminalNode EACH() {
return getToken(CypherParser.EACH, 0);
}
public TerminalNode EDGE() {
return getToken(CypherParser.EDGE, 0);
}
public TerminalNode ELEMENT() {
return getToken(CypherParser.ELEMENT, 0);
}
public TerminalNode ELEMENTS() {
return getToken(CypherParser.ELEMENTS, 0);
}
public TerminalNode ELSE() {
return getToken(CypherParser.ELSE, 0);
}
public TerminalNode ENABLE() {
return getToken(CypherParser.ENABLE, 0);
}
public TerminalNode ENCRYPTED() {
return getToken(CypherParser.ENCRYPTED, 0);
}
public TerminalNode END() {
return getToken(CypherParser.END, 0);
}
public TerminalNode ENDS() {
return getToken(CypherParser.ENDS, 0);
}
public TerminalNode ERROR() {
return getToken(CypherParser.ERROR, 0);
}
public TerminalNode EXECUTABLE() {
return getToken(CypherParser.EXECUTABLE, 0);
}
public TerminalNode EXECUTE() {
return getToken(CypherParser.EXECUTE, 0);
}
public TerminalNode EXIST() {
return getToken(CypherParser.EXIST, 0);
}
public TerminalNode EXISTENCE() {
return getToken(CypherParser.EXISTENCE, 0);
}
public TerminalNode EXISTS() {
return getToken(CypherParser.EXISTS, 0);
}
public TerminalNode FAIL() {
return getToken(CypherParser.FAIL, 0);
}
public TerminalNode FALSE() {
return getToken(CypherParser.FALSE, 0);
}
public TerminalNode FIELDTERMINATOR() {
return getToken(CypherParser.FIELDTERMINATOR, 0);
}
public TerminalNode FINISH() {
return getToken(CypherParser.FINISH, 0);
}
public TerminalNode FLOAT() {
return getToken(CypherParser.FLOAT, 0);
}
public TerminalNode FOREACH() {
return getToken(CypherParser.FOREACH, 0);
}
public TerminalNode FOR() {
return getToken(CypherParser.FOR, 0);
}
public TerminalNode FROM() {
return getToken(CypherParser.FROM, 0);
}
public TerminalNode FULLTEXT() {
return getToken(CypherParser.FULLTEXT, 0);
}
public TerminalNode FUNCTIONS() {
return getToken(CypherParser.FUNCTIONS, 0);
}
public TerminalNode GRANT() {
return getToken(CypherParser.GRANT, 0);
}
public TerminalNode GRAPH() {
return getToken(CypherParser.GRAPH, 0);
}
public TerminalNode GRAPHS() {
return getToken(CypherParser.GRAPHS, 0);
}
public TerminalNode GROUP() {
return getToken(CypherParser.GROUP, 0);
}
public TerminalNode HEADERS() {
return getToken(CypherParser.HEADERS, 0);
}
public TerminalNode HOME() {
return getToken(CypherParser.HOME, 0);
}
public TerminalNode IF() {
return getToken(CypherParser.IF, 0);
}
public TerminalNode IMMUTABLE() {
return getToken(CypherParser.IMMUTABLE, 0);
}
public TerminalNode IMPERSONATE() {
return getToken(CypherParser.IMPERSONATE, 0);
}
public TerminalNode IN() {
return getToken(CypherParser.IN, 0);
}
public TerminalNode INDEX() {
return getToken(CypherParser.INDEX, 0);
}
public TerminalNode INDEXES() {
return getToken(CypherParser.INDEXES, 0);
}
public TerminalNode INFINITY() {
return getToken(CypherParser.INFINITY, 0);
}
public TerminalNode INSERT() {
return getToken(CypherParser.INSERT, 0);
}
public TerminalNode INT() {
return getToken(CypherParser.INT, 0);
}
public TerminalNode INTEGER() {
return getToken(CypherParser.INTEGER, 0);
}
public TerminalNode IS() {
return getToken(CypherParser.IS, 0);
}
public TerminalNode JOIN() {
return getToken(CypherParser.JOIN, 0);
}
public TerminalNode KEY() {
return getToken(CypherParser.KEY, 0);
}
public TerminalNode LABEL() {
return getToken(CypherParser.LABEL, 0);
}
public TerminalNode LABELS() {
return getToken(CypherParser.LABELS, 0);
}
public TerminalNode LEADING() {
return getToken(CypherParser.LEADING, 0);
}
public TerminalNode LIMITROWS() {
return getToken(CypherParser.LIMITROWS, 0);
}
public TerminalNode LIST() {
return getToken(CypherParser.LIST, 0);
}
public TerminalNode LOAD() {
return getToken(CypherParser.LOAD, 0);
}
public TerminalNode LOCAL() {
return getToken(CypherParser.LOCAL, 0);
}
public TerminalNode LOOKUP() {
return getToken(CypherParser.LOOKUP, 0);
}
public TerminalNode MATCH() {
return getToken(CypherParser.MATCH, 0);
}
public TerminalNode MANAGEMENT() {
return getToken(CypherParser.MANAGEMENT, 0);
}
public TerminalNode MAP() {
return getToken(CypherParser.MAP, 0);
}
public TerminalNode MERGE() {
return getToken(CypherParser.MERGE, 0);
}
public TerminalNode NAME() {
return getToken(CypherParser.NAME, 0);
}
public TerminalNode NAMES() {
return getToken(CypherParser.NAMES, 0);
}
public TerminalNode NAN() {
return getToken(CypherParser.NAN, 0);
}
public TerminalNode NEW() {
return getToken(CypherParser.NEW, 0);
}
public TerminalNode NODE() {
return getToken(CypherParser.NODE, 0);
}
public TerminalNode NODETACH() {
return getToken(CypherParser.NODETACH, 0);
}
public TerminalNode NODES() {
return getToken(CypherParser.NODES, 0);
}
public TerminalNode NONE() {
return getToken(CypherParser.NONE, 0);
}
public TerminalNode NORMALIZE() {
return getToken(CypherParser.NORMALIZE, 0);
}
public TerminalNode NOTHING() {
return getToken(CypherParser.NOTHING, 0);
}
public TerminalNode NOWAIT() {
return getToken(CypherParser.NOWAIT, 0);
}
public TerminalNode OF() {
return getToken(CypherParser.OF, 0);
}
public TerminalNode ON() {
return getToken(CypherParser.ON, 0);
}
public TerminalNode ONLY() {
return getToken(CypherParser.ONLY, 0);
}
public TerminalNode OPTIONAL() {
return getToken(CypherParser.OPTIONAL, 0);
}
public TerminalNode OPTIONS() {
return getToken(CypherParser.OPTIONS, 0);
}
public TerminalNode OPTION() {
return getToken(CypherParser.OPTION, 0);
}
public TerminalNode OR() {
return getToken(CypherParser.OR, 0);
}
public TerminalNode ORDER() {
return getToken(CypherParser.ORDER, 0);
}
public TerminalNode OUTPUT() {
return getToken(CypherParser.OUTPUT, 0);
}
public TerminalNode PASSWORD() {
return getToken(CypherParser.PASSWORD, 0);
}
public TerminalNode PASSWORDS() {
return getToken(CypherParser.PASSWORDS, 0);
}
public TerminalNode PATH() {
return getToken(CypherParser.PATH, 0);
}
public TerminalNode PERIODIC() {
return getToken(CypherParser.PERIODIC, 0);
}
public TerminalNode PLAINTEXT() {
return getToken(CypherParser.PLAINTEXT, 0);
}
public TerminalNode POINT() {
return getToken(CypherParser.POINT, 0);
}
public TerminalNode POPULATED() {
return getToken(CypherParser.POPULATED, 0);
}
public TerminalNode PRIMARY() {
return getToken(CypherParser.PRIMARY, 0);
}
public TerminalNode PRIVILEGE() {
return getToken(CypherParser.PRIVILEGE, 0);
}
public TerminalNode PRIVILEGES() {
return getToken(CypherParser.PRIVILEGES, 0);
}
public TerminalNode PROCEDURE() {
return getToken(CypherParser.PROCEDURE, 0);
}
public TerminalNode PROCEDURES() {
return getToken(CypherParser.PROCEDURES, 0);
}
public TerminalNode PROPERTIES() {
return getToken(CypherParser.PROPERTIES, 0);
}
public TerminalNode PROPERTY() {
return getToken(CypherParser.PROPERTY, 0);
}
public TerminalNode RANGE() {
return getToken(CypherParser.RANGE, 0);
}
public TerminalNode READ() {
return getToken(CypherParser.READ, 0);
}
public TerminalNode REALLOCATE() {
return getToken(CypherParser.REALLOCATE, 0);
}
public TerminalNode REDUCE() {
return getToken(CypherParser.REDUCE, 0);
}
public TerminalNode REL() {
return getToken(CypherParser.REL, 0);
}
public TerminalNode RELATIONSHIP() {
return getToken(CypherParser.RELATIONSHIP, 0);
}
public TerminalNode RELATIONSHIPS() {
return getToken(CypherParser.RELATIONSHIPS, 0);
}
public TerminalNode REMOVE() {
return getToken(CypherParser.REMOVE, 0);
}
public TerminalNode RENAME() {
return getToken(CypherParser.RENAME, 0);
}
public TerminalNode REPEATABLE() {
return getToken(CypherParser.REPEATABLE, 0);
}
public TerminalNode REPLACE() {
return getToken(CypherParser.REPLACE, 0);
}
public TerminalNode REPORT() {
return getToken(CypherParser.REPORT, 0);
}
public TerminalNode REQUIRE() {
return getToken(CypherParser.REQUIRE, 0);
}
public TerminalNode REQUIRED() {
return getToken(CypherParser.REQUIRED, 0);
}
public TerminalNode RETURN() {
return getToken(CypherParser.RETURN, 0);
}
public TerminalNode REVOKE() {
return getToken(CypherParser.REVOKE, 0);
}
public TerminalNode ROLE() {
return getToken(CypherParser.ROLE, 0);
}
public TerminalNode ROLES() {
return getToken(CypherParser.ROLES, 0);
}
public TerminalNode ROW() {
return getToken(CypherParser.ROW, 0);
}
public TerminalNode ROWS() {
return getToken(CypherParser.ROWS, 0);
}
public TerminalNode SCAN() {
return getToken(CypherParser.SCAN, 0);
}
public TerminalNode SECONDARY() {
return getToken(CypherParser.SECONDARY, 0);
}
public TerminalNode SECONDS() {
return getToken(CypherParser.SECONDS, 0);
}
public TerminalNode SEEK() {
return getToken(CypherParser.SEEK, 0);
}
public TerminalNode SERVER() {
return getToken(CypherParser.SERVER, 0);
}
public TerminalNode SERVERS() {
return getToken(CypherParser.SERVERS, 0);
}
public TerminalNode SET() {
return getToken(CypherParser.SET, 0);
}
public TerminalNode SETTING() {
return getToken(CypherParser.SETTING, 0);
}
public TerminalNode SHORTEST() {
return getToken(CypherParser.SHORTEST, 0);
}
public TerminalNode SHORTEST_PATH() {
return getToken(CypherParser.SHORTEST_PATH, 0);
}
public TerminalNode SHOW() {
return getToken(CypherParser.SHOW, 0);
}
public TerminalNode SIGNED() {
return getToken(CypherParser.SIGNED, 0);
}
public TerminalNode SINGLE() {
return getToken(CypherParser.SINGLE, 0);
}
public TerminalNode SKIPROWS() {
return getToken(CypherParser.SKIPROWS, 0);
}
public TerminalNode START() {
return getToken(CypherParser.START, 0);
}
public TerminalNode STARTS() {
return getToken(CypherParser.STARTS, 0);
}
public TerminalNode STATUS() {
return getToken(CypherParser.STATUS, 0);
}
public TerminalNode STOP() {
return getToken(CypherParser.STOP, 0);
}
public TerminalNode STRING() {
return getToken(CypherParser.STRING, 0);
}
public TerminalNode SUPPORTED() {
return getToken(CypherParser.SUPPORTED, 0);
}
public TerminalNode SUSPENDED() {
return getToken(CypherParser.SUSPENDED, 0);
}
public TerminalNode TARGET() {
return getToken(CypherParser.TARGET, 0);
}
public TerminalNode TERMINATE() {
return getToken(CypherParser.TERMINATE, 0);
}
public TerminalNode TEXT() {
return getToken(CypherParser.TEXT, 0);
}
public TerminalNode THEN() {
return getToken(CypherParser.THEN, 0);
}
public TerminalNode TIME() {
return getToken(CypherParser.TIME, 0);
}
public TerminalNode TIMESTAMP() {
return getToken(CypherParser.TIMESTAMP, 0);
}
public TerminalNode TIMEZONE() {
return getToken(CypherParser.TIMEZONE, 0);
}
public TerminalNode TO() {
return getToken(CypherParser.TO, 0);
}
public TerminalNode TOPOLOGY() {
return getToken(CypherParser.TOPOLOGY, 0);
}
public TerminalNode TRAILING() {
return getToken(CypherParser.TRAILING, 0);
}
public TerminalNode TRANSACTION() {
return getToken(CypherParser.TRANSACTION, 0);
}
public TerminalNode TRANSACTIONS() {
return getToken(CypherParser.TRANSACTIONS, 0);
}
public TerminalNode TRAVERSE() {
return getToken(CypherParser.TRAVERSE, 0);
}
public TerminalNode TRIM() {
return getToken(CypherParser.TRIM, 0);
}
public TerminalNode TRUE() {
return getToken(CypherParser.TRUE, 0);
}
public TerminalNode TYPE() {
return getToken(CypherParser.TYPE, 0);
}
public TerminalNode TYPES() {
return getToken(CypherParser.TYPES, 0);
}
public TerminalNode UNION() {
return getToken(CypherParser.UNION, 0);
}
public TerminalNode UNIQUE() {
return getToken(CypherParser.UNIQUE, 0);
}
public TerminalNode UNIQUENESS() {
return getToken(CypherParser.UNIQUENESS, 0);
}
public TerminalNode UNWIND() {
return getToken(CypherParser.UNWIND, 0);
}
public TerminalNode URL() {
return getToken(CypherParser.URL, 0);
}
public TerminalNode USE() {
return getToken(CypherParser.USE, 0);
}
public TerminalNode USER() {
return getToken(CypherParser.USER, 0);
}
public TerminalNode USERS() {
return getToken(CypherParser.USERS, 0);
}
public TerminalNode USING() {
return getToken(CypherParser.USING, 0);
}
public TerminalNode VALUE() {
return getToken(CypherParser.VALUE, 0);
}
public TerminalNode VECTOR() {
return getToken(CypherParser.VECTOR, 0);
}
public TerminalNode VERBOSE() {
return getToken(CypherParser.VERBOSE, 0);
}
public TerminalNode VERTEX() {
return getToken(CypherParser.VERTEX, 0);
}
public TerminalNode WAIT() {
return getToken(CypherParser.WAIT, 0);
}
public TerminalNode WHEN() {
return getToken(CypherParser.WHEN, 0);
}
public TerminalNode WHERE() {
return getToken(CypherParser.WHERE, 0);
}
public TerminalNode WITH() {
return getToken(CypherParser.WITH, 0);
}
public TerminalNode WITHOUT() {
return getToken(CypherParser.WITHOUT, 0);
}
public TerminalNode WRITE() {
return getToken(CypherParser.WRITE, 0);
}
public TerminalNode XOR() {
return getToken(CypherParser.XOR, 0);
}
public TerminalNode YIELD() {
return getToken(CypherParser.YIELD, 0);
}
public TerminalNode ZONED() {
return getToken(CypherParser.ZONED, 0);
}
public UnescapedLabelSymbolicNameStringContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_unescapedLabelSymbolicNameString;
}
}
public final UnescapedLabelSymbolicNameStringContext unescapedLabelSymbolicNameString()
throws RecognitionException {
UnescapedLabelSymbolicNameStringContext _localctx =
new UnescapedLabelSymbolicNameStringContext(_ctx, getState());
enterRule(_localctx, 602, RULE_unescapedLabelSymbolicNameString);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(3506);
_la = _input.LA(1);
if (!((((_la) & ~0x3f) == 0 && ((1L << _la) & -61572919592960L) != 0)
|| ((((_la - 64)) & ~0x3f) == 0 && ((1L << (_la - 64)) & -9288676379060225L) != 0)
|| ((((_la - 128)) & ~0x3f) == 0 && ((1L << (_la - 128)) & -3458806327727099777L) != 0)
|| ((((_la - 193)) & ~0x3f) == 0 && ((1L << (_la - 193)) & -576460823170418305L) != 0)
|| ((((_la - 257)) & ~0x3f) == 0 && ((1L << (_la - 257)) & 4294967167L) != 0))) {
_errHandler.recoverInline(this);
} else {
if (_input.LA(1) == Token.EOF) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class EndOfFileContext extends org.neo4j.cypher.internal.parser.AstRuleCtx {
public TerminalNode EOF() {
return getToken(CypherParser.EOF, 0);
}
public EndOfFileContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override
public int getRuleIndex() {
return RULE_endOfFile;
}
}
public final EndOfFileContext endOfFile() throws RecognitionException {
EndOfFileContext _localctx = new EndOfFileContext(_ctx, getState());
enterRule(_localctx, 604, RULE_endOfFile);
try {
enterOuterAlt(_localctx, 1);
{
setState(3508);
match(EOF);
}
} catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
} finally {
exitRule();
}
return _localctx;
}
private static final String _serializedATNSegment0 =
"\u0004\u0001\u0124\u0db7\u0002\u0000\u0007\u0000\u0002\u0001\u0007\u0001"
+ "\u0002\u0002\u0007\u0002\u0002\u0003\u0007\u0003\u0002\u0004\u0007\u0004"
+ "\u0002\u0005\u0007\u0005\u0002\u0006\u0007\u0006\u0002\u0007\u0007\u0007"
+ "\u0002\b\u0007\b\u0002\t\u0007\t\u0002\n\u0007\n\u0002\u000b\u0007\u000b"
+ "\u0002\f\u0007\f\u0002\r\u0007\r\u0002\u000e\u0007\u000e\u0002\u000f\u0007"
+ "\u000f\u0002\u0010\u0007\u0010\u0002\u0011\u0007\u0011\u0002\u0012\u0007"
+ "\u0012\u0002\u0013\u0007\u0013\u0002\u0014\u0007\u0014\u0002\u0015\u0007"
+ "\u0015\u0002\u0016\u0007\u0016\u0002\u0017\u0007\u0017\u0002\u0018\u0007"
+ "\u0018\u0002\u0019\u0007\u0019\u0002\u001a\u0007\u001a\u0002\u001b\u0007"
+ "\u001b\u0002\u001c\u0007\u001c\u0002\u001d\u0007\u001d\u0002\u001e\u0007"
+ "\u001e\u0002\u001f\u0007\u001f\u0002 \u0007 \u0002!\u0007!\u0002\"\u0007"
+ "\"\u0002#\u0007#\u0002$\u0007$\u0002%\u0007%\u0002&\u0007&\u0002\'\u0007"
+ "\'\u0002(\u0007(\u0002)\u0007)\u0002*\u0007*\u0002+\u0007+\u0002,\u0007"
+ ",\u0002-\u0007-\u0002.\u0007.\u0002/\u0007/\u00020\u00070\u00021\u0007"
+ "1\u00022\u00072\u00023\u00073\u00024\u00074\u00025\u00075\u00026\u0007"
+ "6\u00027\u00077\u00028\u00078\u00029\u00079\u0002:\u0007:\u0002;\u0007"
+ ";\u0002<\u0007<\u0002=\u0007=\u0002>\u0007>\u0002?\u0007?\u0002@\u0007"
+ "@\u0002A\u0007A\u0002B\u0007B\u0002C\u0007C\u0002D\u0007D\u0002E\u0007"
+ "E\u0002F\u0007F\u0002G\u0007G\u0002H\u0007H\u0002I\u0007I\u0002J\u0007"
+ "J\u0002K\u0007K\u0002L\u0007L\u0002M\u0007M\u0002N\u0007N\u0002O\u0007"
+ "O\u0002P\u0007P\u0002Q\u0007Q\u0002R\u0007R\u0002S\u0007S\u0002T\u0007"
+ "T\u0002U\u0007U\u0002V\u0007V\u0002W\u0007W\u0002X\u0007X\u0002Y\u0007"
+ "Y\u0002Z\u0007Z\u0002[\u0007[\u0002\\\u0007\\\u0002]\u0007]\u0002^\u0007"
+ "^\u0002_\u0007_\u0002`\u0007`\u0002a\u0007a\u0002b\u0007b\u0002c\u0007"
+ "c\u0002d\u0007d\u0002e\u0007e\u0002f\u0007f\u0002g\u0007g\u0002h\u0007"
+ "h\u0002i\u0007i\u0002j\u0007j\u0002k\u0007k\u0002l\u0007l\u0002m\u0007"
+ "m\u0002n\u0007n\u0002o\u0007o\u0002p\u0007p\u0002q\u0007q\u0002r\u0007"
+ "r\u0002s\u0007s\u0002t\u0007t\u0002u\u0007u\u0002v\u0007v\u0002w\u0007"
+ "w\u0002x\u0007x\u0002y\u0007y\u0002z\u0007z\u0002{\u0007{\u0002|\u0007"
+ "|\u0002}\u0007}\u0002~\u0007~\u0002\u007f\u0007\u007f\u0002\u0080\u0007"
+ "\u0080\u0002\u0081\u0007\u0081\u0002\u0082\u0007\u0082\u0002\u0083\u0007"
+ "\u0083\u0002\u0084\u0007\u0084\u0002\u0085\u0007\u0085\u0002\u0086\u0007"
+ "\u0086\u0002\u0087\u0007\u0087\u0002\u0088\u0007\u0088\u0002\u0089\u0007"
+ "\u0089\u0002\u008a\u0007\u008a\u0002\u008b\u0007\u008b\u0002\u008c\u0007"
+ "\u008c\u0002\u008d\u0007\u008d\u0002\u008e\u0007\u008e\u0002\u008f\u0007"
+ "\u008f\u0002\u0090\u0007\u0090\u0002\u0091\u0007\u0091\u0002\u0092\u0007"
+ "\u0092\u0002\u0093\u0007\u0093\u0002\u0094\u0007\u0094\u0002\u0095\u0007"
+ "\u0095\u0002\u0096\u0007\u0096\u0002\u0097\u0007\u0097\u0002\u0098\u0007"
+ "\u0098\u0002\u0099\u0007\u0099\u0002\u009a\u0007\u009a\u0002\u009b\u0007"
+ "\u009b\u0002\u009c\u0007\u009c\u0002\u009d\u0007\u009d\u0002\u009e\u0007"
+ "\u009e\u0002\u009f\u0007\u009f\u0002\u00a0\u0007\u00a0\u0002\u00a1\u0007"
+ "\u00a1\u0002\u00a2\u0007\u00a2\u0002\u00a3\u0007\u00a3\u0002\u00a4\u0007"
+ "\u00a4\u0002\u00a5\u0007\u00a5\u0002\u00a6\u0007\u00a6\u0002\u00a7\u0007"
+ "\u00a7\u0002\u00a8\u0007\u00a8\u0002\u00a9\u0007\u00a9\u0002\u00aa\u0007"
+ "\u00aa\u0002\u00ab\u0007\u00ab\u0002\u00ac\u0007\u00ac\u0002\u00ad\u0007"
+ "\u00ad\u0002\u00ae\u0007\u00ae\u0002\u00af\u0007\u00af\u0002\u00b0\u0007"
+ "\u00b0\u0002\u00b1\u0007\u00b1\u0002\u00b2\u0007\u00b2\u0002\u00b3\u0007"
+ "\u00b3\u0002\u00b4\u0007\u00b4\u0002\u00b5\u0007\u00b5\u0002\u00b6\u0007"
+ "\u00b6\u0002\u00b7\u0007\u00b7\u0002\u00b8\u0007\u00b8\u0002\u00b9\u0007"
+ "\u00b9\u0002\u00ba\u0007\u00ba\u0002\u00bb\u0007\u00bb\u0002\u00bc\u0007"
+ "\u00bc\u0002\u00bd\u0007\u00bd\u0002\u00be\u0007\u00be\u0002\u00bf\u0007"
+ "\u00bf\u0002\u00c0\u0007\u00c0\u0002\u00c1\u0007\u00c1\u0002\u00c2\u0007"
+ "\u00c2\u0002\u00c3\u0007\u00c3\u0002\u00c4\u0007\u00c4\u0002\u00c5\u0007"
+ "\u00c5\u0002\u00c6\u0007\u00c6\u0002\u00c7\u0007\u00c7\u0002\u00c8\u0007"
+ "\u00c8\u0002\u00c9\u0007\u00c9\u0002\u00ca\u0007\u00ca\u0002\u00cb\u0007"
+ "\u00cb\u0002\u00cc\u0007\u00cc\u0002\u00cd\u0007\u00cd\u0002\u00ce\u0007"
+ "\u00ce\u0002\u00cf\u0007\u00cf\u0002\u00d0\u0007\u00d0\u0002\u00d1\u0007"
+ "\u00d1\u0002\u00d2\u0007\u00d2\u0002\u00d3\u0007\u00d3\u0002\u00d4\u0007"
+ "\u00d4\u0002\u00d5\u0007\u00d5\u0002\u00d6\u0007\u00d6\u0002\u00d7\u0007"
+ "\u00d7\u0002\u00d8\u0007\u00d8\u0002\u00d9\u0007\u00d9\u0002\u00da\u0007"
+ "\u00da\u0002\u00db\u0007\u00db\u0002\u00dc\u0007\u00dc\u0002\u00dd\u0007"
+ "\u00dd\u0002\u00de\u0007\u00de\u0002\u00df\u0007\u00df\u0002\u00e0\u0007"
+ "\u00e0\u0002\u00e1\u0007\u00e1\u0002\u00e2\u0007\u00e2\u0002\u00e3\u0007"
+ "\u00e3\u0002\u00e4\u0007\u00e4\u0002\u00e5\u0007\u00e5\u0002\u00e6\u0007"
+ "\u00e6\u0002\u00e7\u0007\u00e7\u0002\u00e8\u0007\u00e8\u0002\u00e9\u0007"
+ "\u00e9\u0002\u00ea\u0007\u00ea\u0002\u00eb\u0007\u00eb\u0002\u00ec\u0007"
+ "\u00ec\u0002\u00ed\u0007\u00ed\u0002\u00ee\u0007\u00ee\u0002\u00ef\u0007"
+ "\u00ef\u0002\u00f0\u0007\u00f0\u0002\u00f1\u0007\u00f1\u0002\u00f2\u0007"
+ "\u00f2\u0002\u00f3\u0007\u00f3\u0002\u00f4\u0007\u00f4\u0002\u00f5\u0007"
+ "\u00f5\u0002\u00f6\u0007\u00f6\u0002\u00f7\u0007\u00f7\u0002\u00f8\u0007"
+ "\u00f8\u0002\u00f9\u0007\u00f9\u0002\u00fa\u0007\u00fa\u0002\u00fb\u0007"
+ "\u00fb\u0002\u00fc\u0007\u00fc\u0002\u00fd\u0007\u00fd\u0002\u00fe\u0007"
+ "\u00fe\u0002\u00ff\u0007\u00ff\u0002\u0100\u0007\u0100\u0002\u0101\u0007"
+ "\u0101\u0002\u0102\u0007\u0102\u0002\u0103\u0007\u0103\u0002\u0104\u0007"
+ "\u0104\u0002\u0105\u0007\u0105\u0002\u0106\u0007\u0106\u0002\u0107\u0007"
+ "\u0107\u0002\u0108\u0007\u0108\u0002\u0109\u0007\u0109\u0002\u010a\u0007"
+ "\u010a\u0002\u010b\u0007\u010b\u0002\u010c\u0007\u010c\u0002\u010d\u0007"
+ "\u010d\u0002\u010e\u0007\u010e\u0002\u010f\u0007\u010f\u0002\u0110\u0007"
+ "\u0110\u0002\u0111\u0007\u0111\u0002\u0112\u0007\u0112\u0002\u0113\u0007"
+ "\u0113\u0002\u0114\u0007\u0114\u0002\u0115\u0007\u0115\u0002\u0116\u0007"
+ "\u0116\u0002\u0117\u0007\u0117\u0002\u0118\u0007\u0118\u0002\u0119\u0007"
+ "\u0119\u0002\u011a\u0007\u011a\u0002\u011b\u0007\u011b\u0002\u011c\u0007"
+ "\u011c\u0002\u011d\u0007\u011d\u0002\u011e\u0007\u011e\u0002\u011f\u0007"
+ "\u011f\u0002\u0120\u0007\u0120\u0002\u0121\u0007\u0121\u0002\u0122\u0007"
+ "\u0122\u0002\u0123\u0007\u0123\u0002\u0124\u0007\u0124\u0002\u0125\u0007"
+ "\u0125\u0002\u0126\u0007\u0126\u0002\u0127\u0007\u0127\u0002\u0128\u0007"
+ "\u0128\u0002\u0129\u0007\u0129\u0002\u012a\u0007\u012a\u0002\u012b\u0007"
+ "\u012b\u0002\u012c\u0007\u012c\u0002\u012d\u0007\u012d\u0002\u012e\u0007"
+ "\u012e\u0001\u0000\u0001\u0000\u0001\u0000\u0005\u0000\u0262\b\u0000\n"
+ "\u0000\f\u0000\u0265\t\u0000\u0001\u0000\u0003\u0000\u0268\b\u0000\u0001"
+ "\u0000\u0001\u0000\u0001\u0001\u0003\u0001\u026d\b\u0001\u0001\u0001\u0001"
+ "\u0001\u0003\u0001\u0271\b\u0001\u0001\u0002\u0001\u0002\u0001\u0002\u0001"
+ "\u0002\u0003\u0002\u0277\b\u0002\u0001\u0003\u0001\u0003\u0001\u0003\u0003"
+ "\u0003\u027c\b\u0003\u0001\u0003\u0005\u0003\u027f\b\u0003\n\u0003\f\u0003"
+ "\u0282\t\u0003\u0001\u0004\u0004\u0004\u0285\b\u0004\u000b\u0004\f\u0004"
+ "\u0286\u0001\u0005\u0001\u0005\u0001\u0005\u0001\u0005\u0001\u0005\u0001"
+ "\u0005\u0001\u0005\u0001\u0005\u0001\u0005\u0001\u0005\u0001\u0005\u0001"
+ "\u0005\u0001\u0005\u0001\u0005\u0001\u0005\u0001\u0005\u0003\u0005\u0299"
+ "\b\u0005\u0001\u0006\u0001\u0006\u0003\u0006\u029d\b\u0006\u0001\u0006"
+ "\u0001\u0006\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"
+ "\u0001\u0007\u0003\u0007\u02a7\b\u0007\u0001\b\u0001\b\u0001\t\u0001\t"
+ "\u0001\t\u0001\n\u0003\n\u02af\b\n\u0001\n\u0001\n\u0003\n\u02b3\b\n\u0001"
+ "\n\u0003\n\u02b6\b\n\u0001\n\u0003\n\u02b9\b\n\u0001\u000b\u0001\u000b"
+ "\u0001\u000b\u0003\u000b\u02be\b\u000b\u0001\f\u0001\f\u0003\f\u02c2\b"
+ "\f\u0001\f\u0001\f\u0005\f\u02c6\b\f\n\f\f\f\u02c9\t\f\u0001\r\u0001\r"
+ "\u0003\r\u02cd\b\r\u0001\u000e\u0001\u000e\u0001\u000e\u0001\u000e\u0001"
+ "\u000e\u0005\u000e\u02d4\b\u000e\n\u000e\f\u000e\u02d7\t\u000e\u0001\u000f"
+ "\u0001\u000f\u0001\u000f\u0001\u0010\u0001\u0010\u0001\u0010\u0001\u0011"
+ "\u0001\u0011\u0001\u0011\u0001\u0012\u0001\u0012\u0001\u0012\u0003\u0012"
+ "\u02e5\b\u0012\u0001\u0013\u0001\u0013\u0001\u0013\u0001\u0014\u0001\u0014"
+ "\u0001\u0014\u0001\u0015\u0001\u0015\u0001\u0015\u0001\u0015\u0005\u0015"
+ "\u02f1\b\u0015\n\u0015\f\u0015\u02f4\t\u0015\u0001\u0016\u0001\u0016\u0001"
+ "\u0016\u0001\u0016\u0001\u0016\u0001\u0016\u0001\u0016\u0001\u0016\u0001"
+ "\u0016\u0001\u0016\u0001\u0016\u0001\u0016\u0001\u0016\u0001\u0016\u0001"
+ "\u0016\u0001\u0016\u0001\u0016\u0001\u0016\u0003\u0016\u0308\b\u0016\u0001"
+ "\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0005\u0017\u030e\b\u0017\n"
+ "\u0017\f\u0017\u0311\t\u0017\u0001\u0018\u0001\u0018\u0001\u0018\u0001"
+ "\u0018\u0001\u0018\u0001\u0018\u0001\u0018\u0003\u0018\u031a\b\u0018\u0001"
+ "\u0019\u0003\u0019\u031d\b\u0019\u0001\u0019\u0001\u0019\u0001\u0019\u0001"
+ "\u0019\u0005\u0019\u0323\b\u0019\n\u0019\f\u0019\u0326\t\u0019\u0001\u001a"
+ "\u0003\u001a\u0329\b\u001a\u0001\u001a\u0001\u001a\u0003\u001a\u032d\b"
+ "\u001a\u0001\u001a\u0001\u001a\u0005\u001a\u0331\b\u001a\n\u001a\f\u001a"
+ "\u0334\t\u001a\u0001\u001a\u0003\u001a\u0337\b\u001a\u0001\u001b\u0001"
+ "\u001b\u0001\u001b\u0003\u001b\u033c\b\u001b\u0001\u001b\u0003\u001b\u033f"
+ "\b\u001b\u0001\u001b\u0001\u001b\u0001\u001b\u0003\u001b\u0344\b\u001b"
+ "\u0001\u001b\u0003\u001b\u0347\b\u001b\u0003\u001b\u0349\b\u001b\u0001"
+ "\u001c\u0001\u001c\u0001\u001c\u0001\u001c\u0001\u001c\u0001\u001c\u0001"
+ "\u001c\u0001\u001c\u0001\u001c\u0001\u001c\u0003\u001c\u0355\b\u001c\u0001"
+ "\u001c\u0003\u001c\u0358\b\u001c\u0001\u001c\u0001\u001c\u0001\u001c\u0001"
+ "\u001c\u0001\u001c\u0001\u001c\u0001\u001c\u0001\u001c\u0001\u001c\u0001"
+ "\u001c\u0001\u001c\u0001\u001c\u0001\u001c\u0003\u001c\u0367\b\u001c\u0001"
+ "\u001d\u0001\u001d\u0001\u001d\u0005\u001d\u036c\b\u001d\n\u001d\f\u001d"
+ "\u036f\t\u001d\u0001\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0001\u001f"
+ "\u0001\u001f\u0001\u001f\u0001\u001f\u0001\u001f\u0001 \u0001 \u0001 "
+ "\u0001 \u0001 \u0001 \u0001 \u0005 \u0381\b \n \f \u0384\t \u0003 \u0386"
+ "\b \u0001 \u0003 \u0389\b \u0001 \u0001 \u0001 \u0001 \u0001 \u0005 \u0390"
+ "\b \n \f \u0393\t \u0001 \u0003 \u0396\b \u0003 \u0398\b \u0003 \u039a"
+ "\b \u0001!\u0001!\u0001!\u0003!\u039f\b!\u0001\"\u0001\"\u0001\"\u0001"
+ "\"\u0003\"\u03a5\b\"\u0001\"\u0001\"\u0001\"\u0001\"\u0001\"\u0001\"\u0003"
+ "\"\u03ad\b\"\u0001#\u0001#\u0001#\u0001#\u0001#\u0001#\u0001#\u0004#\u03b6"
+ "\b#\u000b#\f#\u03b7\u0001#\u0001#\u0001$\u0001$\u0001$\u0001$\u0001$\u0003"
+ "$\u03c1\b$\u0001%\u0001%\u0003%\u03c5\b%\u0001%\u0003%\u03c8\b%\u0001"
+ "%\u0001%\u0001%\u0001%\u0005%\u03ce\b%\n%\f%\u03d1\t%\u0001&\u0001&\u0001"
+ "&\u0001&\u0001\'\u0001\'\u0001\'\u0001\'\u0001(\u0001(\u0001(\u0001(\u0001"
+ "(\u0001)\u0001)\u0001)\u0005)\u03e3\b)\n)\f)\u03e6\t)\u0001*\u0001*\u0001"
+ "*\u0005*\u03eb\b*\n*\f*\u03ee\t*\u0001+\u0001+\u0001+\u0003+\u03f3\b+"
+ "\u0001+\u0003+\u03f6\b+\u0001+\u0001+\u0001,\u0001,\u0001,\u0003,\u03fd"
+ "\b,\u0001,\u0001,\u0001,\u0001,\u0005,\u0403\b,\n,\f,\u0406\t,\u0001-"
+ "\u0001-\u0001-\u0001-\u0001-\u0003-\u040d\b-\u0001-\u0001-\u0003-\u0411"
+ "\b-\u0001-\u0001-\u0001-\u0003-\u0416\b-\u0001.\u0001.\u0003.\u041a\b"
+ ".\u0001/\u0001/\u0001/\u0001/\u0001/\u00010\u00010\u00010\u00030\u0424"
+ "\b0\u00010\u00010\u00050\u0428\b0\n0\f0\u042b\t0\u00010\u00040\u042e\b"
+ "0\u000b0\f0\u042f\u00011\u00011\u00011\u00031\u0435\b1\u00011\u00011\u0001"
+ "1\u00031\u043a\b1\u00011\u00011\u00031\u043e\b1\u00011\u00031\u0441\b"
+ "1\u00011\u00011\u00031\u0445\b1\u00011\u00011\u00031\u0449\b1\u00011\u0003"
+ "1\u044c\b1\u00011\u00011\u00011\u00011\u00031\u0452\b1\u00031\u0454\b"
+ "1\u00012\u00012\u00012\u00012\u00042\u045a\b2\u000b2\f2\u045b\u00013\u0001"
+ "3\u00033\u0460\b3\u00013\u00033\u0463\b3\u00013\u00033\u0466\b3\u0001"
+ "3\u00013\u00033\u046a\b3\u00013\u00013\u00014\u00014\u00034\u0470\b4\u0001"
+ "4\u00034\u0473\b4\u00014\u00034\u0476\b4\u00014\u00014\u00015\u00015\u0001"
+ "5\u00015\u00035\u047e\b5\u00015\u00015\u00035\u0482\b5\u00016\u00046\u0485"
+ "\b6\u000b6\f6\u0486\u00017\u00017\u00017\u00057\u048c\b7\n7\f7\u048f\t"
+ "7\u00018\u00018\u00018\u00019\u00019\u00019\u0001:\u0001:\u0001:\u0001"
+ ";\u0001;\u0003;\u049c\b;\u0001<\u0003<\u049f\b<\u0001<\u0001<\u0001<\u0003"
+ "<\u04a4\b<\u0001<\u0003<\u04a7\b<\u0001<\u0003<\u04aa\b<\u0001<\u0003"
+ "<\u04ad\b<\u0001<\u0001<\u0003<\u04b1\b<\u0001<\u0003<\u04b4\b<\u0001"
+ "<\u0001<\u0003<\u04b8\b<\u0001=\u0003=\u04bb\b=\u0001=\u0001=\u0001=\u0003"
+ "=\u04c0\b=\u0001=\u0001=\u0003=\u04c4\b=\u0001=\u0001=\u0001=\u0003=\u04c9"
+ "\b=\u0001>\u0001>\u0001?\u0001?\u0001@\u0001@\u0001A\u0001A\u0003A\u04d3"
+ "\bA\u0001A\u0001A\u0003A\u04d7\bA\u0001A\u0003A\u04da\bA\u0001B\u0001"
+ "B\u0001B\u0001B\u0003B\u04e0\bB\u0001C\u0001C\u0001C\u0003C\u04e5\bC\u0001"
+ "C\u0005C\u04e8\bC\nC\fC\u04eb\tC\u0001D\u0001D\u0001D\u0003D\u04f0\bD"
+ "\u0001D\u0005D\u04f3\bD\nD\fD\u04f6\tD\u0001E\u0001E\u0001E\u0005E\u04fb"
+ "\bE\nE\fE\u04fe\tE\u0001F\u0001F\u0001F\u0005F\u0503\bF\nF\fF\u0506\t"
+ "F\u0001G\u0005G\u0509\bG\nG\fG\u050c\tG\u0001G\u0001G\u0001H\u0005H\u0511"
+ "\bH\nH\fH\u0514\tH\u0001H\u0001H\u0001I\u0001I\u0001I\u0001I\u0001I\u0001"
+ "I\u0003I\u051e\bI\u0001J\u0001J\u0001J\u0001J\u0001J\u0001J\u0003J\u0526"
+ "\bJ\u0001K\u0001K\u0001K\u0001K\u0005K\u052c\bK\nK\fK\u052f\tK\u0001L"
+ "\u0001L\u0001L\u0001M\u0001M\u0001M\u0005M\u0537\bM\nM\fM\u053a\tM\u0001"
+ "N\u0001N\u0001N\u0005N\u053f\bN\nN\fN\u0542\tN\u0001O\u0001O\u0001O\u0005"
+ "O\u0547\bO\nO\fO\u054a\tO\u0001P\u0005P\u054d\bP\nP\fP\u0550\tP\u0001"
+ "P\u0001P\u0001Q\u0001Q\u0001Q\u0005Q\u0557\bQ\nQ\fQ\u055a\tQ\u0001R\u0001"
+ "R\u0003R\u055e\bR\u0001S\u0001S\u0001S\u0001S\u0001S\u0001S\u0001S\u0003"
+ "S\u0567\bS\u0001S\u0001S\u0001S\u0003S\u056c\bS\u0001S\u0001S\u0001S\u0003"
+ "S\u0571\bS\u0001S\u0001S\u0003S\u0575\bS\u0001S\u0001S\u0001S\u0003S\u057a"
+ "\bS\u0001S\u0003S\u057d\bS\u0001S\u0003S\u0580\bS\u0001T\u0001T\u0001"
+ "U\u0001U\u0001U\u0005U\u0587\bU\nU\fU\u058a\tU\u0001V\u0001V\u0001V\u0005"
+ "V\u058f\bV\nV\fV\u0592\tV\u0001W\u0001W\u0001W\u0005W\u0597\bW\nW\fW\u059a"
+ "\tW\u0001X\u0001X\u0001X\u0003X\u059f\bX\u0001Y\u0001Y\u0005Y\u05a3\b"
+ "Y\nY\fY\u05a6\tY\u0001Z\u0001Z\u0001Z\u0001Z\u0001Z\u0001Z\u0001Z\u0001"
+ "Z\u0003Z\u05b0\bZ\u0001Z\u0001Z\u0003Z\u05b4\bZ\u0001Z\u0003Z\u05b7\b"
+ "Z\u0001[\u0001[\u0001[\u0001\\\u0001\\\u0004\\\u05be\b\\\u000b\\\f\\\u05bf"
+ "\u0001]\u0001]\u0001]\u0001]\u0001]\u0001]\u0001]\u0001]\u0001]\u0001"
+ "]\u0001]\u0001]\u0001]\u0001]\u0001]\u0001]\u0001]\u0001]\u0001]\u0001"
+ "]\u0001]\u0003]\u05d7\b]\u0001^\u0001^\u0001^\u0001^\u0001^\u0001^\u0001"
+ "^\u0001^\u0003^\u05e1\b^\u0001_\u0001_\u0004_\u05e5\b_\u000b_\f_\u05e6"
+ "\u0001_\u0001_\u0003_\u05eb\b_\u0001_\u0001_\u0001`\u0001`\u0001`\u0001"
+ "`\u0001`\u0001a\u0001a\u0001a\u0004a\u05f7\ba\u000ba\fa\u05f8\u0001a\u0001"
+ "a\u0003a\u05fd\ba\u0001a\u0001a\u0001b\u0001b\u0001b\u0001b\u0005b\u0605"
+ "\bb\nb\fb\u0608\tb\u0001b\u0001b\u0001b\u0001c\u0001c\u0001c\u0001c\u0001"
+ "c\u0003c\u0612\bc\u0001c\u0001c\u0001c\u0003c\u0617\bc\u0001c\u0001c\u0001"
+ "c\u0003c\u061c\bc\u0001c\u0001c\u0003c\u0620\bc\u0001c\u0001c\u0001c\u0003"
+ "c\u0625\bc\u0001c\u0003c\u0628\bc\u0001c\u0001c\u0001c\u0001c\u0003c\u062e"
+ "\bc\u0001d\u0001d\u0001d\u0001d\u0001d\u0001d\u0003d\u0636\bd\u0001d\u0001"
+ "d\u0001d\u0001d\u0003d\u063c\bd\u0003d\u063e\bd\u0001d\u0001d\u0001e\u0001"
+ "e\u0001e\u0001e\u0003e\u0646\be\u0001e\u0001e\u0001e\u0003e\u064b\be\u0001"
+ "e\u0001e\u0001e\u0001e\u0001f\u0001f\u0001f\u0001f\u0001f\u0001f\u0001"
+ "f\u0001f\u0001f\u0001f\u0001f\u0001f\u0001f\u0001g\u0001g\u0001g\u0001"
+ "g\u0001g\u0001g\u0001g\u0003g\u0665\bg\u0001g\u0001g\u0001h\u0001h\u0001"
+ "h\u0001h\u0001h\u0003h\u066e\bh\u0001h\u0001h\u0001i\u0001i\u0001i\u0003"
+ "i\u0675\bi\u0001i\u0003i\u0678\bi\u0001i\u0003i\u067b\bi\u0001i\u0001"
+ "i\u0001i\u0001j\u0001j\u0001k\u0001k\u0001l\u0001l\u0001l\u0001l\u0001"
+ "m\u0001m\u0001m\u0001m\u0001m\u0005m\u068d\bm\nm\fm\u0690\tm\u0003m\u0692"
+ "\bm\u0001m\u0001m\u0001n\u0001n\u0001n\u0001n\u0001n\u0001n\u0001n\u0001"
+ "n\u0003n\u069e\bn\u0001o\u0001o\u0001o\u0001o\u0001o\u0001p\u0001p\u0001"
+ "p\u0001p\u0003p\u06a9\bp\u0001p\u0001p\u0003p\u06ad\bp\u0003p\u06af\b"
+ "p\u0001p\u0001p\u0001q\u0001q\u0001q\u0001q\u0003q\u06b7\bq\u0001q\u0001"
+ "q\u0003q\u06bb\bq\u0003q\u06bd\bq\u0001q\u0001q\u0001r\u0001r\u0001r\u0001"
+ "r\u0001r\u0001s\u0003s\u06c7\bs\u0001s\u0001s\u0001t\u0003t\u06cc\bt\u0001"
+ "t\u0001t\u0001u\u0001u\u0001u\u0001u\u0005u\u06d4\bu\nu\fu\u06d7\tu\u0003"
+ "u\u06d9\bu\u0001u\u0001u\u0001v\u0001v\u0001w\u0001w\u0001w\u0001x\u0001"
+ "x\u0003x\u06e4\bx\u0001y\u0001y\u0001y\u0003y\u06e9\by\u0001y\u0001y\u0001"
+ "y\u0005y\u06ee\by\ny\fy\u06f1\ty\u0003y\u06f3\by\u0001y\u0001y\u0001z"
+ "\u0001z\u0001{\u0001{\u0001{\u0001|\u0001|\u0001|\u0005|\u06ff\b|\n|\f"
+ "|\u0702\t|\u0001}\u0001}\u0001~\u0001~\u0001~\u0005~\u0709\b~\n~\f~\u070c"
+ "\t~\u0001\u007f\u0003\u007f\u070f\b\u007f\u0001\u007f\u0001\u007f\u0001"
+ "\u007f\u0001\u007f\u0001\u007f\u0001\u007f\u0001\u007f\u0001\u007f\u0001"
+ "\u007f\u0001\u007f\u0001\u007f\u0001\u007f\u0001\u007f\u0003\u007f\u071e"
+ "\b\u007f\u0001\u0080\u0001\u0080\u0001\u0080\u0003\u0080\u0723\b\u0080"
+ "\u0001\u0080\u0001\u0080\u0001\u0080\u0001\u0080\u0001\u0080\u0001\u0080"
+ "\u0001\u0080\u0003\u0080\u072c\b\u0080\u0001\u0081\u0001\u0081\u0001\u0081"
+ "\u0001\u0081\u0001\u0081\u0001\u0081\u0001\u0081\u0001\u0081\u0003\u0081"
+ "\u0736\b\u0081\u0001\u0082\u0001\u0082\u0001\u0082\u0001\u0082\u0001\u0082"
+ "\u0001\u0082\u0003\u0082\u073e\b\u0082\u0001\u0083\u0001\u0083\u0001\u0083"
+ "\u0001\u0083\u0003\u0083\u0744\b\u0083\u0001\u0084\u0001\u0084\u0001\u0084"
+ "\u0001\u0084\u0001\u0084\u0001\u0084\u0001\u0084\u0001\u0084\u0001\u0084"
+ "\u0001\u0084\u0001\u0084\u0001\u0084\u0001\u0084\u0001\u0084\u0001\u0084"
+ "\u0001\u0084\u0001\u0084\u0003\u0084\u0757\b\u0084\u0001\u0085\u0001\u0085"
+ "\u0003\u0085\u075b\b\u0085\u0001\u0085\u0003\u0085\u075e\b\u0085\u0001"
+ "\u0086\u0001\u0086\u0001\u0086\u0003\u0086\u0763\b\u0086\u0001\u0087\u0001"
+ "\u0087\u0001\u0087\u0001\u0088\u0001\u0088\u0001\u0088\u0001\u0089\u0001"
+ "\u0089\u0001\u0089\u0001\u0089\u0001\u0089\u0005\u0089\u0770\b\u0089\n"
+ "\u0089\f\u0089\u0773\t\u0089\u0003\u0089\u0775\b\u0089\u0001\u0089\u0003"
+ "\u0089\u0778\b\u0089\u0001\u0089\u0003\u0089\u077b\b\u0089\u0001\u0089"
+ "\u0003\u0089\u077e\b\u0089\u0001\u0089\u0003\u0089\u0781\b\u0089\u0001"
+ "\u008a\u0001\u008a\u0003\u008a\u0785\b\u008a\u0001\u008a\u0001\u008a\u0003"
+ "\u008a\u0789\b\u008a\u0001\u008a\u0003\u008a\u078c\b\u008a\u0001\u008b"
+ "\u0001\u008b\u0001\u008b\u0003\u008b\u0791\b\u008b\u0001\u008b\u0003\u008b"
+ "\u0794\b\u008b\u0001\u008c\u0001\u008c\u0003\u008c\u0798\b\u008c\u0001"
+ "\u008c\u0003\u008c\u079b\b\u008c\u0001\u008d\u0001\u008d\u0003\u008d\u079f"
+ "\b\u008d\u0001\u008d\u0003\u008d\u07a2\b\u008d\u0001\u008e\u0003\u008e"
+ "\u07a5\b\u008e\u0001\u008e\u0001\u008e\u0001\u008e\u0001\u008e\u0001\u008e"
+ "\u0001\u008e\u0001\u008e\u0003\u008e\u07ae\b\u008e\u0001\u008e\u0001\u008e"
+ "\u0001\u008e\u0001\u008e\u0001\u008e\u0001\u008e\u0003\u008e\u07b6\b\u008e"
+ "\u0001\u008e\u0001\u008e\u0001\u008e\u0003\u008e\u07bb\b\u008e\u0001\u008e"
+ "\u0003\u008e\u07be\b\u008e\u0001\u008f\u0001\u008f\u0001\u008f\u0001\u008f"
+ "\u0003\u008f\u07c4\b\u008f\u0001\u0090\u0001\u0090\u0001\u0090\u0001\u0090"
+ "\u0001\u0090\u0003\u0090\u07cb\b\u0090\u0001\u0091\u0001\u0091\u0001\u0091"
+ "\u0001\u0091\u0001\u0091\u0001\u0091\u0001\u0091\u0001\u0091\u0001\u0091"
+ "\u0003\u0091\u07d6\b\u0091\u0001\u0092\u0001\u0092\u0003\u0092\u07da\b"
+ "\u0092\u0001\u0092\u0003\u0092\u07dd\b\u0092\u0001\u0093\u0001\u0093\u0001"
+ "\u0093\u0003\u0093\u07e2\b\u0093\u0003\u0093\u07e4\b\u0093\u0001\u0093"
+ "\u0003\u0093\u07e7\b\u0093\u0001\u0094\u0001\u0094\u0003\u0094\u07eb\b"
+ "\u0094\u0001\u0094\u0003\u0094\u07ee\b\u0094\u0001\u0095\u0001\u0095\u0003"
+ "\u0095\u07f2\b\u0095\u0001\u0095\u0003\u0095\u07f5\b\u0095\u0001\u0095"
+ "\u0003\u0095\u07f8\b\u0095\u0001\u0096\u0003\u0096\u07fb\b\u0096\u0001"
+ "\u0096\u0001\u0096\u0003\u0096\u07ff\b\u0096\u0001\u0096\u0003\u0096\u0802"
+ "\b\u0096\u0001\u0096\u0003\u0096\u0805\b\u0096\u0001\u0097\u0001\u0097"
+ "\u0001\u0097\u0001\u0097\u0001\u0097\u0003\u0097\u080c\b\u0097\u0003\u0097"
+ "\u080e\b\u0097\u0001\u0098\u0001\u0098\u0001\u0098\u0001\u0098\u0001\u0098"
+ "\u0003\u0098\u0815\b\u0098\u0001\u0099\u0001\u0099\u0001\u0099\u0001\u009a"
+ "\u0001\u009a\u0001\u009a\u0001\u009b\u0001\u009b\u0001\u009b\u0001\u009c"
+ "\u0001\u009c\u0001\u009c\u0001\u009d\u0003\u009d\u0824\b\u009d\u0001\u009d"
+ "\u0001\u009d\u0003\u009d\u0828\b\u009d\u0003\u009d\u082a\b\u009d\u0001"
+ "\u009d\u0003\u009d\u082d\b\u009d\u0001\u009e\u0001\u009e\u0003\u009e\u0831"
+ "\b\u009e\u0001\u009f\u0001\u009f\u0001\u009f\u0001\u009f\u0001\u009f\u0001"
+ "\u009f\u0001\u009f\u0003\u009f\u083a\b\u009f\u0001\u00a0\u0001\u00a0\u0003"
+ "\u00a0\u083e\b\u00a0\u0001\u00a1\u0001\u00a1\u0001\u00a1\u0005\u00a1\u0843"
+ "\b\u00a1\n\u00a1\f\u00a1\u0846\t\u00a1\u0001\u00a2\u0001\u00a2\u0003\u00a2"
+ "\u084a\b\u00a2\u0001\u00a2\u0005\u00a2\u084d\b\u00a2\n\u00a2\f\u00a2\u0850"
+ "\t\u00a2\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001"
+ "\u00a3\u0003\u00a3\u0858\b\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001"
+ "\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001"
+ "\u00a3\u0001\u00a3\u0001\u00a3\u0003\u00a3\u0866\b\u00a3\u0001\u00a3\u0001"
+ "\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0003\u00a3\u086d\b\u00a3\u0001"
+ "\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001"
+ "\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001"
+ "\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001"
+ "\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0003"
+ "\u00a3\u0887\b\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001\u00a3\u0001"
+ "\u00a3\u0003\u00a3\u088e\b\u00a3\u0003\u00a3\u0890\b\u00a3\u0001\u00a4"
+ "\u0001\u00a4\u0001\u00a4\u0003\u00a4\u0895\b\u00a4\u0001\u00a5\u0001\u00a5"
+ "\u0003\u00a5\u0899\b\u00a5\u0001\u00a6\u0001\u00a6\u0001\u00a6\u0001\u00a6"
+ "\u0001\u00a6\u0001\u00a7\u0001\u00a7\u0001\u00a7\u0003\u00a7\u08a3\b\u00a7"
+ "\u0001\u00a7\u0001\u00a7\u0001\u00a7\u0001\u00a7\u0001\u00a7\u0001\u00a7"
+ "\u0001\u00a7\u0003\u00a7\u08ac\b\u00a7\u0001\u00a7\u0001\u00a7\u0001\u00a7"
+ "\u0001\u00a8\u0001\u00a8\u0003\u00a8\u08b3\b\u00a8\u0001\u00a8\u0001\u00a8"
+ "\u0001\u00a8\u0003\u00a8\u08b8\b\u00a8\u0001\u00a8\u0001\u00a8\u0001\u00a8"
+ "\u0003\u00a8\u08bd\b\u00a8\u0001\u00a8\u0001\u00a8\u0003\u00a8\u08c1\b"
+ "\u00a8\u0001\u00a9\u0001\u00a9\u0001\u00a9\u0001\u00a9\u0001\u00a9\u0001"
+ "\u00a9\u0001\u00a9\u0001\u00a9\u0003\u00a9\u08cb\b\u00a9\u0001\u00a9\u0001"
+ "\u00a9\u0001\u00a9\u0001\u00a9\u0001\u00a9\u0001\u00a9\u0003\u00a9\u08d3"
+ "\b\u00a9\u0001\u00a9\u0001\u00a9\u0001\u00a9\u0001\u00a9\u0001\u00a9\u0001"
+ "\u00a9\u0003\u00a9\u08db\b\u00a9\u0001\u00a9\u0001\u00a9\u0001\u00a9\u0001"
+ "\u00a9\u0001\u00a9\u0001\u00a9\u0001\u00a9\u0001\u00a9\u0003\u00a9\u08e5"
+ "\b\u00a9\u0001\u00aa\u0001\u00aa\u0001\u00aa\u0001\u00aa\u0003\u00aa\u08eb"
+ "\b\u00aa\u0001\u00aa\u0001\u00aa\u0001\u00aa\u0001\u00aa\u0001\u00aa\u0001"
+ "\u00aa\u0001\u00aa\u0001\u00aa\u0001\u00aa\u0001\u00aa\u0003\u00aa\u08f7"
+ "\b\u00aa\u0003\u00aa\u08f9\b\u00aa\u0001\u00aa\u0001\u00aa\u0001\u00aa"
+ "\u0003\u00aa\u08fe\b\u00aa\u0003\u00aa\u0900\b\u00aa\u0001\u00ab\u0001"
+ "\u00ab\u0001\u00ab\u0001\u00ab\u0001\u00ab\u0001\u00ab\u0001\u00ab\u0001"
+ "\u00ab\u0001\u00ab\u0001\u00ab\u0001\u00ab\u0001\u00ab\u0001\u00ab\u0001"
+ "\u00ab\u0001\u00ab\u0001\u00ab\u0001\u00ab\u0001\u00ab\u0001\u00ab\u0001"
+ "\u00ab\u0001\u00ab\u0001\u00ab\u0001\u00ab\u0001\u00ab\u0001\u00ab\u0003"
+ "\u00ab\u091b\b\u00ab\u0003\u00ab\u091d\b\u00ab\u0001\u00ac\u0001\u00ac"
+ "\u0001\u00ac\u0001\u00ac\u0001\u00ac\u0001\u00ad\u0003\u00ad\u0925\b\u00ad"
+ "\u0001\u00ad\u0001\u00ad\u0001\u00ad\u0003\u00ad\u092a\b\u00ad\u0001\u00ad"
+ "\u0001\u00ad\u0001\u00ad\u0003\u00ad\u092f\b\u00ad\u0001\u00ad\u0001\u00ad"
+ "\u0001\u00ad\u0003\u00ad\u0934\b\u00ad\u0001\u00ae\u0003\u00ae\u0937\b"
+ "\u00ae\u0001\u00ae\u0001\u00ae\u0001\u00ae\u0003\u00ae\u093c\b\u00ae\u0001"
+ "\u00ae\u0001\u00ae\u0001\u00ae\u0003\u00ae\u0941\b\u00ae\u0001\u00ae\u0001"
+ "\u00ae\u0001\u00ae\u0001\u00ae\u0001\u00ae\u0001\u00ae\u0001\u00ae\u0001"
+ "\u00ae\u0001\u00ae\u0005\u00ae\u094c\b\u00ae\n\u00ae\f\u00ae\u094f\t\u00ae"
+ "\u0001\u00ae\u0001\u00ae\u0003\u00ae\u0953\b\u00ae\u0001\u00af\u0001\u00af"
+ "\u0001\u00af\u0001\u00af\u0001\u00af\u0001\u00af\u0005\u00af\u095b\b\u00af"
+ "\n\u00af\f\u00af\u095e\t\u00af\u0001\u00af\u0001\u00af\u0001\u00b0\u0001"
+ "\u00b0\u0001\u00b0\u0003\u00b0\u0965\b\u00b0\u0001\u00b0\u0001\u00b0\u0001"
+ "\u00b0\u0001\u00b0\u0001\u00b0\u0001\u00b0\u0001\u00b0\u0005\u00b0\u096e"
+ "\b\u00b0\n\u00b0\f\u00b0\u0971\t\u00b0\u0001\u00b0\u0001\u00b0\u0001\u00b0"
+ "\u0003\u00b0\u0976\b\u00b0\u0001\u00b0\u0001\u00b0\u0001\u00b0\u0001\u00b1"
+ "\u0003\u00b1\u097c\b\u00b1\u0001\u00b1\u0001\u00b1\u0001\u00b1\u0003\u00b1"
+ "\u0981\b\u00b1\u0001\u00b1\u0001\u00b1\u0001\u00b1\u0003\u00b1\u0986\b"
+ "\u00b1\u0001\u00b1\u0001\u00b1\u0001\u00b1\u0001\u00b1\u0001\u00b1\u0003"
+ "\u00b1\u098d\b\u00b1\u0001\u00b2\u0001\u00b2\u0001\u00b2\u0001\u00b2\u0001"
+ "\u00b2\u0001\u00b2\u0001\u00b3\u0001\u00b3\u0001\u00b3\u0003\u00b3\u0998"
+ "\b\u00b3\u0001\u00b3\u0001\u00b3\u0001\u00b3\u0001\u00b3\u0001\u00b3\u0001"
+ "\u00b3\u0003\u00b3\u09a0\b\u00b3\u0001\u00b3\u0001\u00b3\u0001\u00b3\u0001"
+ "\u00b3\u0003\u00b3\u09a6\b\u00b3\u0001\u00b4\u0001\u00b4\u0001\u00b4\u0001"
+ "\u00b4\u0001\u00b4\u0001\u00b4\u0001\u00b4\u0001\u00b4\u0001\u00b4\u0001"
+ "\u00b4\u0003\u00b4\u09b2\b\u00b4\u0003\u00b4\u09b4\b\u00b4\u0001\u00b5"
+ "\u0001\u00b5\u0001\u00b5\u0001\u00b5\u0001\u00b5\u0001\u00b5\u0001\u00b5"
+ "\u0001\u00b5\u0001\u00b5\u0001\u00b5\u0005\u00b5\u09c0\b\u00b5\n\u00b5"
+ "\f\u00b5\u09c3\t\u00b5\u0001\u00b5\u0001\u00b5\u0003\u00b5\u09c7\b\u00b5"
+ "\u0001\u00b6\u0001\u00b6\u0001\u00b6\u0001\u00b6\u0003\u00b6\u09cd\b\u00b6"
+ "\u0001\u00b7\u0001\u00b7\u0001\u00b7\u0001\u00b7\u0001\u00b7\u0001\u00b8"
+ "\u0001\u00b8\u0001\u00b8\u0001\u00b8\u0001\u00b8\u0001\u00b9\u0001\u00b9"
+ "\u0001\u00b9\u0001\u00ba\u0001\u00ba\u0003\u00ba\u09de\b\u00ba\u0001\u00bb"
+ "\u0003\u00bb\u09e1\b\u00bb\u0001\u00bb\u0001\u00bb\u0003\u00bb\u09e5\b"
+ "\u00bb\u0001\u00bc\u0001\u00bc\u0001\u00bc\u0001\u00bc\u0001\u00bc\u0001"
+ "\u00bc\u0001\u00bc\u0005\u00bc\u09ee\b\u00bc\n\u00bc\f\u00bc\u09f1\t\u00bc"
+ "\u0001\u00bd\u0001\u00bd\u0001\u00bd\u0001\u00be\u0001\u00be\u0001\u00be"
+ "\u0001\u00be\u0001\u00be\u0003\u00be\u09fb\b\u00be\u0001\u00be\u0001\u00be"
+ "\u0001\u00be\u0001\u00be\u0003\u00be\u0a01\b\u00be\u0001\u00bf\u0001\u00bf"
+ "\u0001\u00bf\u0001\u00bf\u0003\u00bf\u0a07\b\u00bf\u0001\u00c0\u0001\u00c0"
+ "\u0001\u00c0\u0001\u00c0\u0003\u00c0\u0a0d\b\u00c0\u0001\u00c0\u0001\u00c0"
+ "\u0001\u00c0\u0001\u00c1\u0003\u00c1\u0a13\b\u00c1\u0001\u00c1\u0001\u00c1"
+ "\u0001\u00c1\u0003\u00c1\u0a18\b\u00c1\u0001\u00c1\u0003\u00c1\u0a1b\b"
+ "\u00c1\u0001\u00c2\u0001\u00c2\u0001\u00c3\u0001\u00c3\u0001\u00c3\u0001"
+ "\u00c3\u0001\u00c3\u0003\u00c3\u0a24\b\u00c3\u0001\u00c3\u0001\u00c3\u0001"
+ "\u00c3\u0001\u00c3\u0001\u00c3\u0001\u00c3\u0001\u00c3\u0003\u00c3\u0a2d"
+ "\b\u00c3\u0005\u00c3\u0a2f\b\u00c3\n\u00c3\f\u00c3\u0a32\t\u00c3\u0001"
+ "\u00c4\u0001\u00c4\u0001\u00c4\u0001\u00c4\u0003\u00c4\u0a38\b\u00c4\u0001"
+ "\u00c5\u0001\u00c5\u0001\u00c5\u0001\u00c5\u0003\u00c5\u0a3e\b\u00c5\u0001"
+ "\u00c5\u0001\u00c5\u0001\u00c5\u0001\u00c6\u0001\u00c6\u0001\u00c6\u0001"
+ "\u00c6\u0001\u00c6\u0001\u00c6\u0001\u00c6\u0001\u00c6\u0001\u00c6\u0001"
+ "\u00c7\u0001\u00c7\u0001\u00c7\u0001\u00c7\u0003\u00c7\u0a50\b\u00c7\u0001"
+ "\u00c7\u0001\u00c7\u0001\u00c7\u0001\u00c7\u0001\u00c7\u0001\u00c7\u0003"
+ "\u00c7\u0a58\b\u00c7\u0004\u00c7\u0a5a\b\u00c7\u000b\u00c7\f\u00c7\u0a5b"
+ "\u0001\u00c7\u0001\u00c7\u0001\u00c7\u0003\u00c7\u0a61\b\u00c7\u0001\u00c8"
+ "\u0003\u00c8\u0a64\b\u00c8\u0001\u00c8\u0001\u00c8\u0001\u00c8\u0003\u00c8"
+ "\u0a69\b\u00c8\u0001\u00c9\u0001\u00c9\u0003\u00c9\u0a6d\b\u00c9\u0001"
+ "\u00ca\u0001\u00ca\u0003\u00ca\u0a71\b\u00ca\u0001\u00ca\u0001\u00ca\u0001"
+ "\u00cb\u0001\u00cb\u0001\u00cb\u0001\u00cc\u0001\u00cc\u0001\u00cc\u0001"
+ "\u00cc\u0001\u00cd\u0001\u00cd\u0003\u00cd\u0a7e\b\u00cd\u0001\u00ce\u0001"
+ "\u00ce\u0001\u00ce\u0003\u00ce\u0a83\b\u00ce\u0001\u00cf\u0003\u00cf\u0a86"
+ "\b\u00cf\u0001\u00cf\u0001\u00cf\u0003\u00cf\u0a8a\b\u00cf\u0001\u00cf"
+ "\u0003\u00cf\u0a8d\b\u00cf\u0001\u00d0\u0001\u00d0\u0001\u00d0\u0003\u00d0"
+ "\u0a92\b\u00d0\u0001\u00d1\u0001\u00d1\u0001\u00d1\u0001\u00d1\u0003\u00d1"
+ "\u0a98\b\u00d1\u0001\u00d1\u0003\u00d1\u0a9b\b\u00d1\u0001\u00d2\u0001"
+ "\u00d2\u0003\u00d2\u0a9f\b\u00d2\u0001\u00d2\u0001\u00d2\u0003\u00d2\u0aa3"
+ "\b\u00d2\u0001\u00d2\u0003\u00d2\u0aa6\b\u00d2\u0001\u00d3\u0001\u00d3"
+ "\u0003\u00d3\u0aaa\b\u00d3\u0001\u00d3\u0001\u00d3\u0001\u00d4\u0001\u00d4"
+ "\u0001\u00d5\u0001\u00d5\u0003\u00d5\u0ab2\b\u00d5\u0001\u00d5\u0001\u00d5"
+ "\u0001\u00d5\u0001\u00d5\u0001\u00d5\u0001\u00d5\u0001\u00d5\u0003\u00d5"
+ "\u0abb\b\u00d5\u0001\u00d6\u0001\u00d6\u0001\u00d6\u0001\u00d6\u0001\u00d7"
+ "\u0001\u00d7\u0003\u00d7\u0ac3\b\u00d7\u0001\u00d7\u0001\u00d7\u0001\u00d7"
+ "\u0001\u00d7\u0001\u00d8\u0001\u00d8\u0003\u00d8\u0acb\b\u00d8\u0001\u00d8"
+ "\u0003\u00d8\u0ace\b\u00d8\u0001\u00d8\u0001\u00d8\u0001\u00d8\u0001\u00d8"
+ "\u0001\u00d8\u0001\u00d8\u0001\u00d8\u0003\u00d8\u0ad7\b\u00d8\u0001\u00d9"
+ "\u0001\u00d9\u0001\u00d9\u0001\u00d9\u0001\u00da\u0001\u00da\u0001\u00da"
+ "\u0001\u00da\u0001\u00da\u0001\u00da\u0001\u00da\u0001\u00da\u0001\u00da"
+ "\u0001\u00da\u0001\u00da\u0001\u00da\u0003\u00da\u0ae9\b\u00da\u0001\u00db"
+ "\u0001\u00db\u0003\u00db\u0aed\b\u00db\u0001\u00db\u0001\u00db\u0001\u00db"
+ "\u0001\u00dc\u0003\u00dc\u0af3\b\u00dc\u0001\u00dc\u0001\u00dc\u0001\u00dd"
+ "\u0001\u00dd\u0001\u00dd\u0001\u00dd\u0001\u00dd\u0003\u00dd\u0afc\b\u00dd"
+ "\u0001\u00dd\u0001\u00dd\u0001\u00dd\u0003\u00dd\u0b01\b\u00dd\u0001\u00dd"
+ "\u0003\u00dd\u0b04\b\u00dd\u0001\u00de\u0001\u00de\u0001\u00de\u0001\u00de"
+ "\u0001\u00de\u0001\u00de\u0001\u00de\u0001\u00de\u0001\u00de\u0001\u00de"
+ "\u0001\u00de\u0001\u00de\u0001\u00de\u0003\u00de\u0b13\b\u00de\u0001\u00df"
+ "\u0001\u00df\u0001\u00df\u0001\u00df\u0001\u00df\u0003\u00df\u0b1a\b\u00df"
+ "\u0001\u00e0\u0001\u00e0\u0003\u00e0\u0b1e\b\u00e0\u0001\u00e0\u0001\u00e0"
+ "\u0001\u00e1\u0001\u00e1\u0003\u00e1\u0b24\b\u00e1\u0001\u00e1\u0001\u00e1"
+ "\u0001\u00e2\u0001\u00e2\u0003\u00e2\u0b2a\b\u00e2\u0001\u00e2\u0001\u00e2"
+ "\u0001\u00e3\u0001\u00e3\u0003\u00e3\u0b30\b\u00e3\u0001\u00e3\u0001\u00e3"
+ "\u0001\u00e3\u0003\u00e3\u0b35\b\u00e3\u0001\u00e4\u0001\u00e4\u0001\u00e4"
+ "\u0003\u00e4\u0b3a\b\u00e4\u0001\u00e4\u0001\u00e4\u0001\u00e4\u0001\u00e4"
+ "\u0001\u00e4\u0001\u00e4\u0001\u00e4\u0003\u00e4\u0b43\b\u00e4\u0001\u00e5"
+ "\u0001\u00e5\u0001\u00e5\u0001\u00e5\u0001\u00e5\u0001\u00e5\u0003\u00e5"
+ "\u0b4b\b\u00e5\u0001\u00e6\u0001\u00e6\u0001\u00e6\u0001\u00e6\u0001\u00e6"
+ "\u0003\u00e6\u0b52\b\u00e6\u0003\u00e6\u0b54\b\u00e6\u0001\u00e6\u0001"
+ "\u00e6\u0001\u00e6\u0001\u00e6\u0001\u00e6\u0001\u00e6\u0001\u00e6\u0001"
+ "\u00e6\u0001\u00e6\u0001\u00e6\u0001\u00e6\u0003\u00e6\u0b61\b\u00e6\u0001"
+ "\u00e6\u0001\u00e6\u0003\u00e6\u0b65\b\u00e6\u0001\u00e7\u0001\u00e7\u0001"
+ "\u00e7\u0001\u00e7\u0001\u00e7\u0001\u00e7\u0003\u00e7\u0b6d\b\u00e7\u0001"
+ "\u00e7\u0001\u00e7\u0003\u00e7\u0b71\b\u00e7\u0001\u00e7\u0001\u00e7\u0001"
+ "\u00e7\u0001\u00e7\u0001\u00e7\u0001\u00e7\u0001\u00e7\u0001\u00e7\u0001"
+ "\u00e7\u0001\u00e7\u0001\u00e7\u0001\u00e7\u0001\u00e7\u0003\u00e7\u0b80"
+ "\b\u00e7\u0001\u00e8\u0001\u00e8\u0001\u00e9\u0001\u00e9\u0001\u00e9\u0001"
+ "\u00e9\u0001\u00e9\u0001\u00e9\u0001\u00e9\u0001\u00e9\u0001\u00e9\u0003"
+ "\u00e9\u0b8d\b\u00e9\u0001\u00ea\u0001\u00ea\u0001\u00ea\u0001\u00ea\u0001"
+ "\u00eb\u0001\u00eb\u0001\u00eb\u0001\u00eb\u0001\u00eb\u0001\u00eb\u0003"
+ "\u00eb\u0b99\b\u00eb\u0001\u00eb\u0003\u00eb\u0b9c\b\u00eb\u0001\u00eb"
+ "\u0001\u00eb\u0003\u00eb\u0ba0\b\u00eb\u0001\u00eb\u0001\u00eb\u0003\u00eb"
+ "\u0ba4\b\u00eb\u0001\u00eb\u0003\u00eb\u0ba7\b\u00eb\u0003\u00eb\u0ba9"
+ "\b\u00eb\u0001\u00eb\u0001\u00eb\u0001\u00eb\u0001\u00ec\u0001\u00ec\u0001"
+ "\u00ec\u0001\u00ec\u0001\u00ec\u0001\u00ec\u0003\u00ec\u0bb4\b\u00ec\u0001"
+ "\u00ec\u0001\u00ec\u0001\u00ec\u0001\u00ec\u0001\u00ec\u0003\u00ec\u0bbb"
+ "\b\u00ec\u0001\u00ec\u0001\u00ec\u0001\u00ec\u0001\u00ec\u0001\u00ec\u0001"
+ "\u00ec\u0003\u00ec\u0bc3\b\u00ec\u0003\u00ec\u0bc5\b\u00ec\u0001\u00ec"
+ "\u0001\u00ec\u0001\u00ec\u0001\u00ed\u0001\u00ed\u0001\u00ed\u0001\u00ed"
+ "\u0001\u00ed\u0003\u00ed\u0bcf\b\u00ed\u0001\u00ed\u0001\u00ed\u0001\u00ed"
+ "\u0001\u00ed\u0001\u00ed\u0003\u00ed\u0bd6\b\u00ed\u0003\u00ed\u0bd8\b"
+ "\u00ed\u0001\u00ed\u0001\u00ed\u0003\u00ed\u0bdc\b\u00ed\u0003\u00ed\u0bde"
+ "\b\u00ed\u0001\u00ee\u0001\u00ee\u0001\u00ef\u0001\u00ef\u0001\u00f0\u0001"
+ "\u00f0\u0001\u00f1\u0001\u00f1\u0001\u00f2\u0001\u00f2\u0001\u00f3\u0001"
+ "\u00f3\u0001\u00f3\u0003\u00f3\u0bed\b\u00f3\u0001\u00f3\u0001\u00f3\u0001"
+ "\u00f4\u0001\u00f4\u0001\u00f5\u0001\u00f5\u0001\u00f6\u0001\u00f6\u0001"
+ "\u00f7\u0001\u00f7\u0001\u00f7\u0005\u00f7\u0bfa\b\u00f7\n\u00f7\f\u00f7"
+ "\u0bfd\t\u00f7\u0001\u00f8\u0001\u00f8\u0001\u00f8\u0003\u00f8\u0c02\b"
+ "\u00f8\u0001\u00f8\u0001\u00f8\u0001\u00f8\u0001\u00f8\u0001\u00f8\u0001"
+ "\u00f8\u0003\u00f8\u0c0a\b\u00f8\u0001\u00f9\u0001\u00f9\u0001\u00f9\u0003"
+ "\u00f9\u0c0f\b\u00f9\u0001\u00f9\u0001\u00f9\u0001\u00f9\u0001\u00f9\u0001"
+ "\u00fa\u0001\u00fa\u0003\u00fa\u0c17\b\u00fa\u0001\u00fb\u0001\u00fb\u0001"
+ "\u00fb\u0003\u00fb\u0c1c\b\u00fb\u0001\u00fb\u0001\u00fb\u0001\u00fc\u0001"
+ "\u00fc\u0001\u00fc\u0005\u00fc\u0c23\b\u00fc\n\u00fc\f\u00fc\u0c26\t\u00fc"
+ "\u0001\u00fd\u0001\u00fd\u0001\u00fd\u0003\u00fd\u0c2b\b\u00fd\u0001\u00fd"
+ "\u0001\u00fd\u0001\u00fd\u0003\u00fd\u0c30\b\u00fd\u0001\u00fd\u0001\u00fd"
+ "\u0001\u00fd\u0001\u00fd\u0005\u00fd\u0c36\b\u00fd\n\u00fd\f\u00fd\u0c39"
+ "\t\u00fd\u0003\u00fd\u0c3b\b\u00fd\u0001\u00fd\u0001\u00fd\u0001\u00fd"
+ "\u0001\u00fd\u0001\u00fd\u0001\u00fd\u0003\u00fd\u0c43\b\u00fd\u0001\u00fd"
+ "\u0001\u00fd\u0003\u00fd\u0c47\b\u00fd\u0003\u00fd\u0c49\b\u00fd\u0001"
+ "\u00fe\u0001\u00fe\u0001\u00fe\u0003\u00fe\u0c4e\b\u00fe\u0001\u00ff\u0001"
+ "\u00ff\u0001\u0100\u0001\u0100\u0001\u0101\u0001\u0101\u0001\u0102\u0001"
+ "\u0102\u0001\u0102\u0001\u0102\u0001\u0102\u0001\u0102\u0003\u0102\u0c5c"
+ "\b\u0102\u0001\u0102\u0003\u0102\u0c5f\b\u0102\u0001\u0102\u0003\u0102"
+ "\u0c62\b\u0102\u0001\u0103\u0001\u0103\u0001\u0103\u0001\u0103\u0001\u0103"
+ "\u0003\u0103\u0c69\b\u0103\u0001\u0103\u0001\u0103\u0001\u0103\u0004\u0103"
+ "\u0c6e\b\u0103\u000b\u0103\f\u0103\u0c6f\u0003\u0103\u0c72\b\u0103\u0001"
+ "\u0103\u0003\u0103\u0c75\b\u0103\u0001\u0103\u0003\u0103\u0c78\b\u0103"
+ "\u0001\u0104\u0001\u0104\u0001\u0104\u0001\u0105\u0001\u0105\u0001\u0105"
+ "\u0001\u0106\u0003\u0106\u0c81\b\u0106\u0001\u0106\u0001\u0106\u0001\u0106"
+ "\u0001\u0106\u0003\u0106\u0c87\b\u0106\u0001\u0106\u0001\u0106\u0003\u0106"
+ "\u0c8b\b\u0106\u0001\u0106\u0003\u0106\u0c8e\b\u0106\u0001\u0107\u0001"
+ "\u0107\u0001\u0107\u0001\u0107\u0003\u0107\u0c94\b\u0107\u0001\u0107\u0001"
+ "\u0107\u0001\u0107\u0001\u0107\u0003\u0107\u0c9a\b\u0107\u0004\u0107\u0c9c"
+ "\b\u0107\u000b\u0107\f\u0107\u0c9d\u0001\u0107\u0001\u0107\u0001\u0107"
+ "\u0004\u0107\u0ca3\b\u0107\u000b\u0107\f\u0107\u0ca4\u0003\u0107\u0ca7"
+ "\b\u0107\u0001\u0107\u0003\u0107\u0caa\b\u0107\u0001\u0108\u0001\u0108"
+ "\u0001\u0108\u0001\u0108\u0001\u0109\u0001\u0109\u0001\u0109\u0004\u0109"
+ "\u0cb3\b\u0109\u000b\u0109\f\u0109\u0cb4\u0001\u010a\u0001\u010a\u0001"
+ "\u010a\u0001\u010a\u0001\u010b\u0001\u010b\u0001\u010b\u0001\u010b\u0003"
+ "\u010b\u0cbf\b\u010b\u0001\u010c\u0001\u010c\u0001\u010c\u0001\u010c\u0003"
+ "\u010c\u0cc5\b\u010c\u0001\u010d\u0001\u010d\u0001\u010d\u0003\u010d\u0cca"
+ "\b\u010d\u0003\u010d\u0ccc\b\u010d\u0001\u010d\u0003\u010d\u0ccf\b\u010d"
+ "\u0001\u010e\u0001\u010e\u0001\u010e\u0003\u010e\u0cd4\b\u010e\u0001\u010e"
+ "\u0001\u010e\u0003\u010e\u0cd8\b\u010e\u0001\u010e\u0003\u010e\u0cdb\b"
+ "\u010e\u0003\u010e\u0cdd\b\u010e\u0001\u010f\u0001\u010f\u0001\u010f\u0001"
+ "\u010f\u0001\u010f\u0003\u010f\u0ce4\b\u010f\u0003\u010f\u0ce6\b\u010f"
+ "\u0001\u0110\u0001\u0110\u0001\u0110\u0001\u0110\u0001\u0110\u0003\u0110"
+ "\u0ced\b\u0110\u0003\u0110\u0cef\b\u0110\u0001\u0111\u0001\u0111\u0001"
+ "\u0111\u0001\u0112\u0001\u0112\u0003\u0112\u0cf6\b\u0112\u0001\u0113\u0001"
+ "\u0113\u0003\u0113\u0cfa\b\u0113\u0001\u0114\u0001\u0114\u0001\u0114\u0001"
+ "\u0114\u0001\u0114\u0003\u0114\u0d01\b\u0114\u0001\u0114\u0001\u0114\u0001"
+ "\u0114\u0001\u0114\u0001\u0114\u0001\u0114\u0001\u0114\u0001\u0114\u0001"
+ "\u0114\u0001\u0114\u0001\u0114\u0003\u0114\u0d0e\b\u0114\u0003\u0114\u0d10"
+ "\b\u0114\u0001\u0114\u0001\u0114\u0003\u0114\u0d14\b\u0114\u0001\u0115"
+ "\u0001\u0115\u0001\u0115\u0001\u0115\u0003\u0115\u0d1a\b\u0115\u0001\u0115"
+ "\u0001\u0115\u0001\u0115\u0001\u0116\u0001\u0116\u0001\u0116\u0001\u0116"
+ "\u0003\u0116\u0d23\b\u0116\u0001\u0116\u0001\u0116\u0001\u0116\u0001\u0116"
+ "\u0001\u0116\u0001\u0116\u0001\u0116\u0004\u0116\u0d2c\b\u0116\u000b\u0116"
+ "\f\u0116\u0d2d\u0001\u0117\u0001\u0117\u0001\u0117\u0001\u0117\u0003\u0117"
+ "\u0d34\b\u0117\u0001\u0118\u0001\u0118\u0001\u0118\u0001\u0119\u0001\u0119"
+ "\u0001\u0119\u0001\u011a\u0001\u011a\u0001\u011a\u0001\u011b\u0001\u011b"
+ "\u0001\u011b\u0001\u011c\u0001\u011c\u0003\u011c\u0d44\b\u011c\u0001\u011c"
+ "\u0001\u011c\u0001\u011c\u0003\u011c\u0d49\b\u011c\u0001\u011d\u0001\u011d"
+ "\u0001\u011d\u0005\u011d\u0d4e\b\u011d\n\u011d\f\u011d\u0d51\t\u011d\u0001"
+ "\u011e\u0001\u011e\u0003\u011e\u0d55\b\u011e\u0001\u011f\u0001\u011f\u0001"
+ "\u011f\u0005\u011f\u0d5a\b\u011f\n\u011f\f\u011f\u0d5d\t\u011f\u0001\u0120"
+ "\u0001\u0120\u0001\u0120\u0005\u0120\u0d62\b\u0120\n\u0120\f\u0120\u0d65"
+ "\t\u0120\u0001\u0121\u0001\u0121\u0003\u0121\u0d69\b\u0121\u0001\u0121"
+ "\u0003\u0121\u0d6c\b\u0121\u0001\u0122\u0001\u0122\u0003\u0122\u0d70\b"
+ "\u0122\u0001\u0123\u0001\u0123\u0003\u0123\u0d74\b\u0123\u0001\u0123\u0001"
+ "\u0123\u0001\u0123\u0003\u0123\u0d79\b\u0123\u0001\u0124\u0001\u0124\u0001"
+ "\u0124\u0004\u0124\u0d7e\b\u0124\u000b\u0124\f\u0124\u0d7f\u0001\u0125"
+ "\u0001\u0125\u0001\u0126\u0001\u0126\u0003\u0126\u0d86\b\u0126\u0001\u0127"
+ "\u0001\u0127\u0003\u0127\u0d8a\b\u0127\u0001\u0128\u0001\u0128\u0001\u0128"
+ "\u0001\u0128\u0001\u0128\u0001\u0128\u0001\u0128\u0001\u0128\u0001\u0128"
+ "\u0005\u0128\u0d95\b\u0128\n\u0128\f\u0128\u0d98\t\u0128\u0003\u0128\u0d9a"
+ "\b\u0128\u0001\u0128\u0001\u0128\u0001\u0129\u0001\u0129\u0003\u0129\u0da0"
+ "\b\u0129\u0001\u012a\u0001\u012a\u0001\u012b\u0001\u012b\u0001\u012b\u0001"
+ "\u012b\u0001\u012b\u0001\u012b\u0001\u012b\u0001\u012b\u0001\u012b\u0003"
+ "\u012b\u0dad\b\u012b\u0001\u012c\u0001\u012c\u0003\u012c\u0db1\b\u012c"
+ "\u0001\u012d\u0001\u012d\u0001\u012e\u0001\u012e\u0001\u012e\u0000\u0000"
+ "\u012f\u0000\u0002\u0004\u0006\b\n\f\u000e\u0010\u0012\u0014\u0016\u0018"
+ "\u001a\u001c\u001e \"$&(*,.02468:<>@BDFHJLNPRTVXZ\\^`bdfhjlnprtvxz|~\u0080"
+ "\u0082\u0084\u0086\u0088\u008a\u008c\u008e\u0090\u0092\u0094\u0096\u0098"
+ "\u009a\u009c\u009e\u00a0\u00a2\u00a4\u00a6\u00a8\u00aa\u00ac\u00ae\u00b0"
+ "\u00b2\u00b4\u00b6\u00b8\u00ba\u00bc\u00be\u00c0\u00c2\u00c4\u00c6\u00c8"
+ "\u00ca\u00cc\u00ce\u00d0\u00d2\u00d4\u00d6\u00d8\u00da\u00dc\u00de\u00e0"
+ "\u00e2\u00e4\u00e6\u00e8\u00ea\u00ec\u00ee\u00f0\u00f2\u00f4\u00f6\u00f8"
+ "\u00fa\u00fc\u00fe\u0100\u0102\u0104\u0106\u0108\u010a\u010c\u010e\u0110"
+ "\u0112\u0114\u0116\u0118\u011a\u011c\u011e\u0120\u0122\u0124\u0126\u0128"
+ "\u012a\u012c\u012e\u0130\u0132\u0134\u0136\u0138\u013a\u013c\u013e\u0140"
+ "\u0142\u0144\u0146\u0148\u014a\u014c\u014e\u0150\u0152\u0154\u0156\u0158"
+ "\u015a\u015c\u015e\u0160\u0162\u0164\u0166\u0168\u016a\u016c\u016e\u0170"
+ "\u0172\u0174\u0176\u0178\u017a\u017c\u017e\u0180\u0182\u0184\u0186\u0188"
+ "\u018a\u018c\u018e\u0190\u0192\u0194\u0196\u0198\u019a\u019c\u019e\u01a0"
+ "\u01a2\u01a4\u01a6\u01a8\u01aa\u01ac\u01ae\u01b0\u01b2\u01b4\u01b6\u01b8"
+ "\u01ba\u01bc\u01be\u01c0\u01c2\u01c4\u01c6\u01c8\u01ca\u01cc\u01ce\u01d0"
+ "\u01d2\u01d4\u01d6\u01d8\u01da\u01dc\u01de\u01e0\u01e2\u01e4\u01e6\u01e8"
+ "\u01ea\u01ec\u01ee\u01f0\u01f2\u01f4\u01f6\u01f8\u01fa\u01fc\u01fe\u0200"
+ "\u0202\u0204\u0206\u0208\u020a\u020c\u020e\u0210\u0212\u0214\u0216\u0218"
+ "\u021a\u021c\u021e\u0220\u0222\u0224\u0226\u0228\u022a\u022c\u022e\u0230"
+ "\u0232\u0234\u0236\u0238\u023a\u023c\u023e\u0240\u0242\u0244\u0246\u0248"
+ "\u024a\u024c\u024e\u0250\u0252\u0254\u0256\u0258\u025a\u025c\u0000C\u0002"
+ "\u0000\u0012\u0012LL\u0002\u0000\u0018\u0018GG\u0002\u0000II\u00a5\u00a5"
+ "\u0002\u000099\u0096\u0096\u0001\u0000\u00de\u00df\u0003\u0000!!77ff\u0002"
+ "\u0000\u0011\u0011\u00ea\u00ea\u0002\u0000\u0093\u0093\u0122\u0122\u0002"
+ "\u0000\u0098\u0098\u0121\u0121\u0002\u0000uu\u0123\u0123\u0002\u0000+"
+ "+\u0087\u0087\u0002\u0000++\u0082\u0082\u0006\u0000__ppuu\u008b\u008b"
+ "\u0093\u0093\u009a\u009b\u0002\u0000,,\u0108\u0108\u0001\u0000\u009f\u00a2"
+ "\u0003\u0000PP\u0098\u0098\u00bc\u00bc\u0003\u0000MM\u0099\u0099\u00fc"
+ "\u00fc\u0002\u0000\u0098\u0098\u00bc\u00bc\u0004\u0000\u0012\u0012\u0015"
+ "\u0015\u00a7\u00a7\u00ee\u00ee\u0003\u0000 \u008c\u008c\u0101\u0101\u0001"
+ "\u0000\u0004\u0007\u0002\u0000\"\"\u0115\u0115\u0006\u0000nn\u0091\u0091"
+ "\u00be\u00be\u00c9\u00c9\u00f9\u00f9\u0114\u0114\u0002\u0000\u0012\u0012"
+ "##\u0002\u0000\u00a4\u00a4\u00d1\u00d2\u0001\u0000\u00d1\u00d2\u0002\u0000"
+ "\u00a4\u00a4\u00d2\u00d2\u0001\u0000\u00c4\u00c5\u0002\u0000@@\u00fb\u00fb"
+ "\u0002\u0000\u0016\u0016\u008e\u008e\u0002\u0000kk\u00af\u00af\u0002\u0000"
+ "\u0019\u0019\u00d8\u00d8\u0001\u0000\u00e6\u00e7\u0001\u0000=>\u0002\u0000"
+ "\u0012\u0012\u00bf\u00bf\u0001\u0000\u0110\u0111\u0001\u0000\u00dc\u00dd"
+ "\u0002\u0000\\\\\u00bb\u00bb\u0002\u0000\f\f\u00f6\u00f6\u0001\u0000."
+ "/\u0001\u0000\u00c2\u00c3\u0002\u0000FFqq\u0003\u0000==AArr\u0002\u0000"
+ "CCww\u0002\u0000==rr\u0001\u0000rs\u0001\u0000\u0085\u0086\u0002\u0000"
+ "\u0107\u0107\u0109\u0109\u0001\u0000\u009c\u009d\u0002\u0000))\u010e\u010e"
+ "\u0001\u0000\u00b7\u00b8\u0002\u0000\u00c2\u00c2\u00dc\u00dc\u0003\u0000"
+ "\u000f\u000f==\u0110\u0110\u0002\u0000\u00dc\u00dc\u0110\u0110\u0001\u0000"
+ "\r\u000e\u0001\u0000|}\u0001\u000034\u0001\u0000\u0102\u0103\u0002\u0000"
+ "\u0096\u0096\u00cc\u00cc\u0001\u0000\u00d2\u00d3\u0001\u0000YZ\u0002\u0000"
+ "\u00a4\u00a4\u00a6\u00a6\u0002\u0000HHTT\u0002\u0000\u00b0\u00b0\u011c"
+ "\u011c\u0001\u0000\u000f\u0010\u0001\u0000\b\t\u0017\u0000\u000b\u001b"
+ "\u001d*.JLLQ^`oqtv\u0086\u008c\u0091\u0094\u0097\u009c\u009e\u00a3\u00a8"
+ "\u00ab\u00ac\u00ae\u00bb\u00be\u00bf\u00c1\u00c7\u00c9\u00c9\u00cc\u00cf"
+ "\u00d1\u00df\u00e1\u00e4\u00e6\u00fb\u00fd\u0107\u0109\u0120\u0f2c\u0000"
+ "\u025e\u0001\u0000\u0000\u0000\u0002\u026c\u0001\u0000\u0000\u0000\u0004"
+ "\u0272\u0001\u0000\u0000\u0000\u0006\u0278\u0001\u0000\u0000\u0000\b\u0284"
+ "\u0001\u0000\u0000\u0000\n\u0298\u0001\u0000\u0000\u0000\f\u029a\u0001"
+ "\u0000\u0000\u0000\u000e\u02a6\u0001\u0000\u0000\u0000\u0010\u02a8\u0001"
+ "\u0000\u0000\u0000\u0012\u02aa\u0001\u0000\u0000\u0000\u0014\u02ae\u0001"
+ "\u0000\u0000\u0000\u0016\u02ba\u0001\u0000\u0000\u0000\u0018\u02c1\u0001"
+ "\u0000\u0000\u0000\u001a\u02ca\u0001\u0000\u0000\u0000\u001c\u02ce\u0001"
+ "\u0000\u0000\u0000\u001e\u02d8\u0001\u0000\u0000\u0000 \u02db\u0001\u0000"
+ "\u0000\u0000\"\u02de\u0001\u0000\u0000\u0000$\u02e1\u0001\u0000\u0000"
+ "\u0000&\u02e6\u0001\u0000\u0000\u0000(\u02e9\u0001\u0000\u0000\u0000*"
+ "\u02ec\u0001\u0000\u0000\u0000,\u0307\u0001\u0000\u0000\u0000.\u0309\u0001"
+ "\u0000\u0000\u00000\u0319\u0001\u0000\u0000\u00002\u031c\u0001\u0000\u0000"
+ "\u00004\u0328\u0001\u0000\u0000\u00006\u0348\u0001\u0000\u0000\u00008"
+ "\u034a\u0001\u0000\u0000\u0000:\u0368\u0001\u0000\u0000\u0000<\u0370\u0001"
+ "\u0000\u0000\u0000>\u0374\u0001\u0000\u0000\u0000@\u0379\u0001\u0000\u0000"
+ "\u0000B\u039b\u0001\u0000\u0000\u0000D\u03a0\u0001\u0000\u0000\u0000F"
+ "\u03ae\u0001\u0000\u0000\u0000H\u03bb\u0001\u0000\u0000\u0000J\u03c2\u0001"
+ "\u0000\u0000\u0000L\u03d2\u0001\u0000\u0000\u0000N\u03d6\u0001\u0000\u0000"
+ "\u0000P\u03da\u0001\u0000\u0000\u0000R\u03df\u0001\u0000\u0000\u0000T"
+ "\u03e7\u0001\u0000\u0000\u0000V\u03f2\u0001\u0000\u0000\u0000X\u03fc\u0001"
+ "\u0000\u0000\u0000Z\u0415\u0001\u0000\u0000\u0000\\\u0419\u0001\u0000"
+ "\u0000\u0000^\u041b\u0001\u0000\u0000\u0000`\u042d\u0001\u0000\u0000\u0000"
+ "b\u0453\u0001\u0000\u0000\u0000d\u0455\u0001\u0000\u0000\u0000f\u045d"
+ "\u0001\u0000\u0000\u0000h\u046d\u0001\u0000\u0000\u0000j\u0479\u0001\u0000"
+ "\u0000\u0000l\u0484\u0001\u0000\u0000\u0000n\u0488\u0001\u0000\u0000\u0000"
+ "p\u0490\u0001\u0000\u0000\u0000r\u0493\u0001\u0000\u0000\u0000t\u0496"
+ "\u0001\u0000\u0000\u0000v\u049b\u0001\u0000\u0000\u0000x\u049e\u0001\u0000"
+ "\u0000\u0000z\u04ba\u0001\u0000\u0000\u0000|\u04ca\u0001\u0000\u0000\u0000"
+ "~\u04cc\u0001\u0000\u0000\u0000\u0080\u04ce\u0001\u0000\u0000\u0000\u0082"
+ "\u04d0\u0001\u0000\u0000\u0000\u0084\u04df\u0001\u0000\u0000\u0000\u0086"
+ "\u04e1\u0001\u0000\u0000\u0000\u0088\u04ec\u0001\u0000\u0000\u0000\u008a"
+ "\u04f7\u0001\u0000\u0000\u0000\u008c\u04ff\u0001\u0000\u0000\u0000\u008e"
+ "\u050a\u0001\u0000\u0000\u0000\u0090\u0512\u0001\u0000\u0000\u0000\u0092"
+ "\u051d\u0001\u0000\u0000\u0000\u0094\u0525\u0001\u0000\u0000\u0000\u0096"
+ "\u0527\u0001\u0000\u0000\u0000\u0098\u0530\u0001\u0000\u0000\u0000\u009a"
+ "\u0533\u0001\u0000\u0000\u0000\u009c\u053b\u0001\u0000\u0000\u0000\u009e"
+ "\u0543\u0001\u0000\u0000\u0000\u00a0\u054e\u0001\u0000\u0000\u0000\u00a2"
+ "\u0553\u0001\u0000\u0000\u0000\u00a4\u055b\u0001\u0000\u0000\u0000\u00a6"
+ "\u057f\u0001\u0000\u0000\u0000\u00a8\u0581\u0001\u0000\u0000\u0000\u00aa"
+ "\u0583\u0001\u0000\u0000\u0000\u00ac\u058b\u0001\u0000\u0000\u0000\u00ae"
+ "\u0593\u0001\u0000\u0000\u0000\u00b0\u059e\u0001\u0000\u0000\u0000\u00b2"
+ "\u05a0\u0001\u0000\u0000\u0000\u00b4\u05b6\u0001\u0000\u0000\u0000\u00b6"
+ "\u05b8\u0001\u0000\u0000\u0000\u00b8\u05bb\u0001\u0000\u0000\u0000\u00ba"
+ "\u05d6\u0001\u0000\u0000\u0000\u00bc\u05e0\u0001\u0000\u0000\u0000\u00be"
+ "\u05e2\u0001\u0000\u0000\u0000\u00c0\u05ee\u0001\u0000\u0000\u0000\u00c2"
+ "\u05f3\u0001\u0000\u0000\u0000\u00c4\u0600\u0001\u0000\u0000\u0000\u00c6"
+ "\u062d\u0001\u0000\u0000\u0000\u00c8\u062f\u0001\u0000\u0000\u0000\u00ca"
+ "\u0641\u0001\u0000\u0000\u0000\u00cc\u0650\u0001\u0000\u0000\u0000\u00ce"
+ "\u065d\u0001\u0000\u0000\u0000\u00d0\u0668\u0001\u0000\u0000\u0000\u00d2"
+ "\u0671\u0001\u0000\u0000\u0000\u00d4\u067f\u0001\u0000\u0000\u0000\u00d6"
+ "\u0681\u0001\u0000\u0000\u0000\u00d8\u0683\u0001\u0000\u0000\u0000\u00da"
+ "\u0687\u0001\u0000\u0000\u0000\u00dc\u069d\u0001\u0000\u0000\u0000\u00de"
+ "\u069f\u0001\u0000\u0000\u0000\u00e0\u06a4\u0001\u0000\u0000\u0000\u00e2"
+ "\u06b2\u0001\u0000\u0000\u0000\u00e4\u06c0\u0001\u0000\u0000\u0000\u00e6"
+ "\u06c6\u0001\u0000\u0000\u0000\u00e8\u06cb\u0001\u0000\u0000\u0000\u00ea"
+ "\u06cf\u0001\u0000\u0000\u0000\u00ec\u06dc\u0001\u0000\u0000\u0000\u00ee"
+ "\u06de\u0001\u0000\u0000\u0000\u00f0\u06e3\u0001\u0000\u0000\u0000\u00f2"
+ "\u06e5\u0001\u0000\u0000\u0000\u00f4\u06f6\u0001\u0000\u0000\u0000\u00f6"
+ "\u06f8\u0001\u0000\u0000\u0000\u00f8\u0700\u0001\u0000\u0000\u0000\u00fa"
+ "\u0703\u0001\u0000\u0000\u0000\u00fc\u0705\u0001\u0000\u0000\u0000\u00fe"
+ "\u070e\u0001\u0000\u0000\u0000\u0100\u071f\u0001\u0000\u0000\u0000\u0102"
+ "\u072d\u0001\u0000\u0000\u0000\u0104\u0737\u0001\u0000\u0000\u0000\u0106"
+ "\u073f\u0001\u0000\u0000\u0000\u0108\u0745\u0001\u0000\u0000\u0000\u010a"
+ "\u075d\u0001\u0000\u0000\u0000\u010c\u075f\u0001\u0000\u0000\u0000\u010e"
+ "\u0764\u0001\u0000\u0000\u0000\u0110\u0767\u0001\u0000\u0000\u0000\u0112"
+ "\u076a\u0001\u0000\u0000\u0000\u0114\u078b\u0001\u0000\u0000\u0000\u0116"
+ "\u0793\u0001\u0000\u0000\u0000\u0118\u0795\u0001\u0000\u0000\u0000\u011a"
+ "\u079c\u0001\u0000\u0000\u0000\u011c\u07bd\u0001\u0000\u0000\u0000\u011e"
+ "\u07c3\u0001\u0000\u0000\u0000\u0120\u07ca\u0001\u0000\u0000\u0000\u0122"
+ "\u07d5\u0001\u0000\u0000\u0000\u0124\u07d7\u0001\u0000\u0000\u0000\u0126"
+ "\u07de\u0001\u0000\u0000\u0000\u0128\u07e8\u0001\u0000\u0000\u0000\u012a"
+ "\u07ef\u0001\u0000\u0000\u0000\u012c\u07fa\u0001\u0000\u0000\u0000\u012e"
+ "\u0806\u0001\u0000\u0000\u0000\u0130\u0814\u0001\u0000\u0000\u0000\u0132"
+ "\u0816\u0001\u0000\u0000\u0000\u0134\u0819\u0001\u0000\u0000\u0000\u0136"
+ "\u081c\u0001\u0000\u0000\u0000\u0138\u081f\u0001\u0000\u0000\u0000\u013a"
+ "\u0829\u0001\u0000\u0000\u0000\u013c\u0830\u0001\u0000\u0000\u0000\u013e"
+ "\u0832\u0001\u0000\u0000\u0000\u0140\u083d\u0001\u0000\u0000\u0000\u0142"
+ "\u083f\u0001\u0000\u0000\u0000\u0144\u0847\u0001\u0000\u0000\u0000\u0146"
+ "\u088f\u0001\u0000\u0000\u0000\u0148\u0894\u0001\u0000\u0000\u0000\u014a"
+ "\u0896\u0001\u0000\u0000\u0000\u014c\u089a\u0001\u0000\u0000\u0000\u014e"
+ "\u089f\u0001\u0000\u0000\u0000\u0150\u08b0\u0001\u0000\u0000\u0000\u0152"
+ "\u08e4\u0001\u0000\u0000\u0000\u0154\u08e6\u0001\u0000\u0000\u0000\u0156"
+ "\u091c\u0001\u0000\u0000\u0000\u0158\u091e\u0001\u0000\u0000\u0000\u015a"
+ "\u0924\u0001\u0000\u0000\u0000\u015c\u0936\u0001\u0000\u0000\u0000\u015e"
+ "\u0954\u0001\u0000\u0000\u0000\u0160\u0961\u0001\u0000\u0000\u0000\u0162"
+ "\u097b\u0001\u0000\u0000\u0000\u0164\u098e\u0001\u0000\u0000\u0000\u0166"
+ "\u0994\u0001\u0000\u0000\u0000\u0168\u09a7\u0001\u0000\u0000\u0000\u016a"
+ "\u09c6\u0001\u0000\u0000\u0000\u016c\u09c8\u0001\u0000\u0000\u0000\u016e"
+ "\u09ce\u0001\u0000\u0000\u0000\u0170\u09d3\u0001\u0000\u0000\u0000\u0172"
+ "\u09d8\u0001\u0000\u0000\u0000\u0174\u09db\u0001\u0000\u0000\u0000\u0176"
+ "\u09e0\u0001\u0000\u0000\u0000\u0178\u09e6\u0001\u0000\u0000\u0000\u017a"
+ "\u09f2\u0001\u0000\u0000\u0000\u017c\u09f5\u0001\u0000\u0000\u0000\u017e"
+ "\u0a02\u0001\u0000\u0000\u0000\u0180\u0a08\u0001\u0000\u0000\u0000\u0182"
+ "\u0a12\u0001\u0000\u0000\u0000\u0184\u0a1c\u0001\u0000\u0000\u0000\u0186"
+ "\u0a1e\u0001\u0000\u0000\u0000\u0188\u0a33\u0001\u0000\u0000\u0000\u018a"
+ "\u0a39\u0001\u0000\u0000\u0000\u018c\u0a42\u0001\u0000\u0000\u0000\u018e"
+ "\u0a4b\u0001\u0000\u0000\u0000\u0190\u0a63\u0001\u0000\u0000\u0000\u0192"
+ "\u0a6c\u0001\u0000\u0000\u0000\u0194\u0a6e\u0001\u0000\u0000\u0000\u0196"
+ "\u0a74\u0001\u0000\u0000\u0000\u0198\u0a77\u0001\u0000\u0000\u0000\u019a"
+ "\u0a7b\u0001\u0000\u0000\u0000\u019c\u0a7f\u0001\u0000\u0000\u0000\u019e"
+ "\u0a85\u0001\u0000\u0000\u0000\u01a0\u0a8e\u0001\u0000\u0000\u0000\u01a2"
+ "\u0a93\u0001\u0000\u0000\u0000\u01a4\u0a9c\u0001\u0000\u0000\u0000\u01a6"
+ "\u0aa7\u0001\u0000\u0000\u0000\u01a8\u0aad\u0001\u0000\u0000\u0000\u01aa"
+ "\u0aaf\u0001\u0000\u0000\u0000\u01ac\u0abc\u0001\u0000\u0000\u0000\u01ae"
+ "\u0ac0\u0001\u0000\u0000\u0000\u01b0\u0ac8\u0001\u0000\u0000\u0000\u01b2"
+ "\u0ad8\u0001\u0000\u0000\u0000\u01b4\u0ae8\u0001\u0000\u0000\u0000\u01b6"
+ "\u0aea\u0001\u0000\u0000\u0000\u01b8\u0af2\u0001\u0000\u0000\u0000\u01ba"
+ "\u0b03\u0001\u0000\u0000\u0000\u01bc\u0b05\u0001\u0000\u0000\u0000\u01be"
+ "\u0b19\u0001\u0000\u0000\u0000\u01c0\u0b1b\u0001\u0000\u0000\u0000\u01c2"
+ "\u0b21\u0001\u0000\u0000\u0000\u01c4\u0b27\u0001\u0000\u0000\u0000\u01c6"
+ "\u0b34\u0001\u0000\u0000\u0000\u01c8\u0b36\u0001\u0000\u0000\u0000\u01ca"
+ "\u0b44\u0001\u0000\u0000\u0000\u01cc\u0b4c\u0001\u0000\u0000\u0000\u01ce"
+ "\u0b66\u0001\u0000\u0000\u0000\u01d0\u0b81\u0001\u0000\u0000\u0000\u01d2"
+ "\u0b83\u0001\u0000\u0000\u0000\u01d4\u0b8e\u0001\u0000\u0000\u0000\u01d6"
+ "\u0ba8\u0001\u0000\u0000\u0000\u01d8\u0bc4\u0001\u0000\u0000\u0000\u01da"
+ "\u0bc9\u0001\u0000\u0000\u0000\u01dc\u0bdf\u0001\u0000\u0000\u0000\u01de"
+ "\u0be1\u0001\u0000\u0000\u0000\u01e0\u0be3\u0001\u0000\u0000\u0000\u01e2"
+ "\u0be5\u0001\u0000\u0000\u0000\u01e4\u0be7\u0001\u0000\u0000\u0000\u01e6"
+ "\u0be9\u0001\u0000\u0000\u0000\u01e8\u0bf0\u0001\u0000\u0000\u0000\u01ea"
+ "\u0bf2\u0001\u0000\u0000\u0000\u01ec\u0bf4\u0001\u0000\u0000\u0000\u01ee"
+ "\u0bf6\u0001\u0000\u0000\u0000\u01f0\u0c01\u0001\u0000\u0000\u0000\u01f2"
+ "\u0c0e\u0001\u0000\u0000\u0000\u01f4\u0c16\u0001\u0000\u0000\u0000\u01f6"
+ "\u0c18\u0001\u0000\u0000\u0000\u01f8\u0c1f\u0001\u0000\u0000\u0000\u01fa"
+ "\u0c48\u0001\u0000\u0000\u0000\u01fc\u0c4d\u0001\u0000\u0000\u0000\u01fe"
+ "\u0c4f\u0001\u0000\u0000\u0000\u0200\u0c51\u0001\u0000\u0000\u0000\u0202"
+ "\u0c53\u0001\u0000\u0000\u0000\u0204\u0c55\u0001\u0000\u0000\u0000\u0206"
+ "\u0c63\u0001\u0000\u0000\u0000\u0208\u0c79\u0001\u0000\u0000\u0000\u020a"
+ "\u0c7c\u0001\u0000\u0000\u0000\u020c\u0c80\u0001\u0000\u0000\u0000\u020e"
+ "\u0c8f\u0001\u0000\u0000\u0000\u0210\u0cab\u0001\u0000\u0000\u0000\u0212"
+ "\u0caf\u0001\u0000\u0000\u0000\u0214\u0cb6\u0001\u0000\u0000\u0000\u0216"
+ "\u0cba\u0001\u0000\u0000\u0000\u0218\u0cc0\u0001\u0000\u0000\u0000\u021a"
+ "\u0cce\u0001\u0000\u0000\u0000\u021c\u0cdc\u0001\u0000\u0000\u0000\u021e"
+ "\u0ce5\u0001\u0000\u0000\u0000\u0220\u0cee\u0001\u0000\u0000\u0000\u0222"
+ "\u0cf0\u0001\u0000\u0000\u0000\u0224\u0cf5\u0001\u0000\u0000\u0000\u0226"
+ "\u0cf9\u0001\u0000\u0000\u0000\u0228\u0cfb\u0001\u0000\u0000\u0000\u022a"
+ "\u0d15\u0001\u0000\u0000\u0000\u022c\u0d1e\u0001\u0000\u0000\u0000\u022e"
+ "\u0d2f\u0001\u0000\u0000\u0000\u0230\u0d35\u0001\u0000\u0000\u0000\u0232"
+ "\u0d38\u0001\u0000\u0000\u0000\u0234\u0d3b\u0001\u0000\u0000\u0000\u0236"
+ "\u0d3e\u0001\u0000\u0000\u0000\u0238\u0d41\u0001\u0000\u0000\u0000\u023a"
+ "\u0d4a\u0001\u0000\u0000\u0000\u023c\u0d54\u0001\u0000\u0000\u0000\u023e"
+ "\u0d56\u0001\u0000\u0000\u0000\u0240\u0d5e\u0001\u0000\u0000\u0000\u0242"
+ "\u0d6b\u0001\u0000\u0000\u0000\u0244\u0d6d\u0001\u0000\u0000\u0000\u0246"
+ "\u0d78\u0001\u0000\u0000\u0000\u0248\u0d7a\u0001\u0000\u0000\u0000\u024a"
+ "\u0d81\u0001\u0000\u0000\u0000\u024c\u0d85\u0001\u0000\u0000\u0000\u024e"
+ "\u0d89\u0001\u0000\u0000\u0000\u0250\u0d8b\u0001\u0000\u0000\u0000\u0252"
+ "\u0d9f\u0001\u0000\u0000\u0000\u0254\u0da1\u0001\u0000\u0000\u0000\u0256"
+ "\u0dac\u0001\u0000\u0000\u0000\u0258\u0db0\u0001\u0000\u0000\u0000\u025a"
+ "\u0db2\u0001\u0000\u0000\u0000\u025c\u0db4\u0001\u0000\u0000\u0000\u025e"
+ "\u0263\u0003\u0002\u0001\u0000\u025f\u0260\u0005\u00e5\u0000\u0000\u0260"
+ "\u0262\u0003\u0002\u0001\u0000\u0261\u025f\u0001\u0000\u0000\u0000\u0262"
+ "\u0265\u0001\u0000\u0000\u0000\u0263\u0261\u0001\u0000\u0000\u0000\u0263"
+ "\u0264\u0001\u0000\u0000\u0000\u0264\u0267\u0001\u0000\u0000\u0000\u0265"
+ "\u0263\u0001\u0000\u0000\u0000\u0266\u0268\u0005\u00e5\u0000\u0000\u0267"
+ "\u0266\u0001\u0000\u0000\u0000\u0267\u0268\u0001\u0000\u0000\u0000\u0268"
+ "\u0269\u0001\u0000\u0000\u0000\u0269\u026a\u0005\u0000\u0000\u0001\u026a"
+ "\u0001\u0001\u0000\u0000\u0000\u026b\u026d\u0003\u0004\u0002\u0000\u026c"
+ "\u026b\u0001\u0000\u0000\u0000\u026c\u026d\u0001\u0000\u0000\u0000\u026d"
+ "\u0270\u0001\u0000\u0000\u0000\u026e\u0271\u0003\u00fe\u007f\u0000\u026f"
+ "\u0271\u0003\u0006\u0003\u0000\u0270\u026e\u0001\u0000\u0000\u0000\u0270"
+ "\u026f\u0001\u0000\u0000\u0000\u0271\u0003\u0001\u0000\u0000\u0000\u0272"
+ "\u0273\u0005\u0112\u0000\u0000\u0273\u0274\u0005\u00ba\u0000\u0000\u0274"
+ "\u0276\u00050\u0000\u0000\u0275\u0277\u0005\u0005\u0000\u0000\u0276\u0275"
+ "\u0001\u0000\u0000\u0000\u0276\u0277\u0001\u0000\u0000\u0000\u0277\u0005"
+ "\u0001\u0000\u0000\u0000\u0278\u0280\u0003\b\u0004\u0000\u0279\u027b\u0005"
+ "\u010a\u0000\u0000\u027a\u027c\u0007\u0000\u0000\u0000\u027b\u027a\u0001"
+ "\u0000\u0000\u0000\u027b\u027c\u0001\u0000\u0000\u0000\u027c\u027d\u0001"
+ "\u0000\u0000\u0000\u027d\u027f\u0003\b\u0004\u0000\u027e\u0279\u0001\u0000"
+ "\u0000\u0000\u027f\u0282\u0001\u0000\u0000\u0000\u0280\u027e\u0001\u0000"
+ "\u0000\u0000\u0280\u0281\u0001\u0000\u0000\u0000\u0281\u0007\u0001\u0000"
+ "\u0000\u0000\u0282\u0280\u0001\u0000\u0000\u0000\u0283\u0285\u0003\n\u0005"
+ "\u0000\u0284\u0283\u0001\u0000\u0000\u0000\u0285\u0286\u0001\u0000\u0000"
+ "\u0000\u0286\u0284\u0001\u0000\u0000\u0000\u0286\u0287\u0001\u0000\u0000"
+ "\u0000\u0287\t\u0001\u0000\u0000\u0000\u0288\u0299\u0003\f\u0006\u0000"
+ "\u0289\u0299\u0003\u0010\b\u0000\u028a\u0299\u0003\u0012\t\u0000\u028b"
+ "\u0299\u0003&\u0013\u0000\u028c\u0299\u0003(\u0014\u0000\u028d\u0299\u0003"
+ "2\u0019\u0000\u028e\u0299\u0003*\u0015\u0000\u028f\u0299\u0003.\u0017"
+ "\u0000\u0290\u0299\u00034\u001a\u0000\u0291\u0299\u0003:\u001d\u0000\u0292"
+ "\u0299\u0003$\u0012\u0000\u0293\u0299\u0003>\u001f\u0000\u0294\u0299\u0003"
+ "@ \u0000\u0295\u0299\u0003H$\u0000\u0296\u0299\u0003D\"\u0000\u0297\u0299"
+ "\u0003F#\u0000\u0298\u0288\u0001\u0000\u0000\u0000\u0298\u0289\u0001\u0000"
+ "\u0000\u0000\u0298\u028a\u0001\u0000\u0000\u0000\u0298\u028b\u0001\u0000"
+ "\u0000\u0000\u0298\u028c\u0001\u0000\u0000\u0000\u0298\u028d\u0001\u0000"
+ "\u0000\u0000\u0298\u028e\u0001\u0000\u0000\u0000\u0298\u028f\u0001\u0000"
+ "\u0000\u0000\u0298\u0290\u0001\u0000\u0000\u0000\u0298\u0291\u0001\u0000"
+ "\u0000\u0000\u0298\u0292\u0001\u0000\u0000\u0000\u0298\u0293\u0001\u0000"
+ "\u0000\u0000\u0298\u0294\u0001\u0000\u0000\u0000\u0298\u0295\u0001\u0000"
+ "\u0000\u0000\u0298\u0296\u0001\u0000\u0000\u0000\u0298\u0297\u0001\u0000"
+ "\u0000\u0000\u0299\u000b\u0001\u0000\u0000\u0000\u029a\u029c\u0005\u010f"
+ "\u0000\u0000\u029b\u029d\u0005r\u0000\u0000\u029c\u029b\u0001\u0000\u0000"
+ "\u0000\u029c\u029d\u0001\u0000\u0000\u0000\u029d\u029e\u0001\u0000\u0000"
+ "\u0000\u029e\u029f\u0003\u000e\u0007\u0000\u029f\r\u0001\u0000\u0000\u0000"
+ "\u02a0\u02a1\u0005\u0092\u0000\u0000\u02a1\u02a2\u0003\u000e\u0007\u0000"
+ "\u02a2\u02a3\u0005\u00e0\u0000\u0000\u02a3\u02a7\u0001\u0000\u0000\u0000"
+ "\u02a4\u02a7\u0003\u00f2y\u0000\u02a5\u02a7\u0003\u023e\u011f\u0000\u02a6"
+ "\u02a0\u0001\u0000\u0000\u0000\u02a6\u02a4\u0001\u0000\u0000\u0000\u02a6"
+ "\u02a5\u0001\u0000\u0000\u0000\u02a7\u000f\u0001\u0000\u0000\u0000\u02a8"
+ "\u02a9\u0005i\u0000\u0000\u02a9\u0011\u0001\u0000\u0000\u0000\u02aa\u02ab"
+ "\u0005\u00da\u0000\u0000\u02ab\u02ac\u0003\u0014\n\u0000\u02ac\u0013\u0001"
+ "\u0000\u0000\u0000\u02ad\u02af\u0005L\u0000\u0000\u02ae\u02ad\u0001\u0000"
+ "\u0000\u0000\u02ae\u02af\u0001\u0000\u0000\u0000\u02af\u02b0\u0001\u0000"
+ "\u0000\u0000\u02b0\u02b2\u0003\u0018\f\u0000\u02b1\u02b3\u0003\u001c\u000e"
+ "\u0000\u02b2\u02b1\u0001\u0000\u0000\u0000\u02b2\u02b3\u0001\u0000\u0000"
+ "\u0000\u02b3\u02b5\u0001\u0000\u0000\u0000\u02b4\u02b6\u0003\u001e\u000f"
+ "\u0000\u02b5\u02b4\u0001\u0000\u0000\u0000\u02b5\u02b6\u0001\u0000\u0000"
+ "\u0000\u02b6\u02b8\u0001\u0000\u0000\u0000\u02b7\u02b9\u0003 \u0010\u0000"
+ "\u02b8\u02b7\u0001\u0000\u0000\u0000\u02b8\u02b9\u0001\u0000\u0000\u0000"
+ "\u02b9\u0015\u0001\u0000\u0000\u0000\u02ba\u02bd\u0003\u009aM\u0000\u02bb"
+ "\u02bc\u0005\u0017\u0000\u0000\u02bc\u02be\u0003\u00fa}\u0000\u02bd\u02bb"
+ "\u0001\u0000\u0000\u0000\u02bd\u02be\u0001\u0000\u0000\u0000\u02be\u0017"
+ "\u0001\u0000\u0000\u0000\u02bf\u02c2\u0005\u00fc\u0000\u0000\u02c0\u02c2"
+ "\u0003\u0016\u000b\u0000\u02c1\u02bf\u0001\u0000\u0000\u0000\u02c1\u02c0"
+ "\u0001\u0000\u0000\u0000\u02c2\u02c7\u0001\u0000\u0000\u0000\u02c3\u02c4"
+ "\u0005-\u0000\u0000\u02c4\u02c6\u0003\u0016\u000b\u0000\u02c5\u02c3\u0001"
+ "\u0000\u0000\u0000\u02c6\u02c9\u0001\u0000\u0000\u0000\u02c7\u02c5\u0001"
+ "\u0000\u0000\u0000\u02c7\u02c8\u0001\u0000\u0000\u0000\u02c8\u0019\u0001"
+ "\u0000\u0000\u0000\u02c9\u02c7\u0001\u0000\u0000\u0000\u02ca\u02cc\u0003"
+ "\u009aM\u0000\u02cb\u02cd\u0007\u0001\u0000\u0000\u02cc\u02cb\u0001\u0000"
+ "\u0000\u0000\u02cc\u02cd\u0001\u0000\u0000\u0000\u02cd\u001b\u0001\u0000"
+ "\u0000\u0000\u02ce\u02cf\u0005\u00b5\u0000\u0000\u02cf\u02d0\u0005%\u0000"
+ "\u0000\u02d0\u02d5\u0003\u001a\r\u0000\u02d1\u02d2\u0005-\u0000\u0000"
+ "\u02d2\u02d4\u0003\u001a\r\u0000\u02d3\u02d1\u0001\u0000\u0000\u0000\u02d4"
+ "\u02d7\u0001\u0000\u0000\u0000\u02d5\u02d3\u0001\u0000\u0000\u0000\u02d5"
+ "\u02d6\u0001\u0000\u0000\u0000\u02d6\u001d\u0001\u0000\u0000\u0000\u02d7"
+ "\u02d5\u0001\u0000\u0000\u0000\u02d8\u02d9\u0005\u00ef\u0000\u0000\u02d9"
+ "\u02da\u0003\u009aM\u0000\u02da\u001f\u0001\u0000\u0000\u0000\u02db\u02dc"
+ "\u0005\u008d\u0000\u0000\u02dc\u02dd\u0003\u009aM\u0000\u02dd!\u0001\u0000"
+ "\u0000\u0000\u02de\u02df\u0005\u0119\u0000\u0000\u02df\u02e0\u0003\u009a"
+ "M\u0000\u02e0#\u0001\u0000\u0000\u0000\u02e1\u02e2\u0005\u011a\u0000\u0000"
+ "\u02e2\u02e4\u0003\u0014\n\u0000\u02e3\u02e5\u0003\"\u0011\u0000\u02e4"
+ "\u02e3\u0001\u0000\u0000\u0000\u02e4\u02e5\u0001\u0000\u0000\u0000\u02e5"
+ "%\u0001\u0000\u0000\u0000\u02e6\u02e7\u00059\u0000\u0000\u02e7\u02e8\u0003"
+ "R)\u0000\u02e8\'\u0001\u0000\u0000\u0000\u02e9\u02ea\u0005\u007f\u0000"
+ "\u0000\u02ea\u02eb\u0003T*\u0000\u02eb)\u0001\u0000\u0000\u0000\u02ec"
+ "\u02ed\u0005\u00e8\u0000\u0000\u02ed\u02f2\u0003,\u0016\u0000\u02ee\u02ef"
+ "\u0005-\u0000\u0000\u02ef\u02f1\u0003,\u0016\u0000\u02f0\u02ee\u0001\u0000"
+ "\u0000\u0000\u02f1\u02f4\u0001\u0000\u0000\u0000\u02f2\u02f0\u0001\u0000"
+ "\u0000\u0000\u02f2\u02f3\u0001\u0000\u0000\u0000\u02f3+\u0001\u0000\u0000"
+ "\u0000\u02f4\u02f2\u0001\u0000\u0000\u0000\u02f5\u02f6\u0003\u00b8\\\u0000"
+ "\u02f6\u02f7\u0005_\u0000\u0000\u02f7\u02f8\u0003\u009aM\u0000\u02f8\u0308"
+ "\u0001\u0000\u0000\u0000\u02f9\u02fa\u0003\u00fa}\u0000\u02fa\u02fb\u0005"
+ "_\u0000\u0000\u02fb\u02fc\u0003\u009aM\u0000\u02fc\u0308\u0001\u0000\u0000"
+ "\u0000\u02fd\u02fe\u0003\u00fa}\u0000\u02fe\u02ff\u0005\u00bd\u0000\u0000"
+ "\u02ff\u0300\u0003\u009aM\u0000\u0300\u0308\u0001\u0000\u0000\u0000\u0301"
+ "\u0302\u0003\u00fa}\u0000\u0302\u0303\u0003l6\u0000\u0303\u0308\u0001"
+ "\u0000\u0000\u0000\u0304\u0305\u0003\u00fa}\u0000\u0305\u0306\u0003n7"
+ "\u0000\u0306\u0308\u0001\u0000\u0000\u0000\u0307\u02f5\u0001\u0000\u0000"
+ "\u0000\u0307\u02f9\u0001\u0000\u0000\u0000\u0307\u02fd\u0001\u0000\u0000"
+ "\u0000\u0307\u0301\u0001\u0000\u0000\u0000\u0307\u0304\u0001\u0000\u0000"
+ "\u0000\u0308-\u0001\u0000\u0000\u0000\u0309\u030a\u0005\u00d4\u0000\u0000"
+ "\u030a\u030f\u00030\u0018\u0000\u030b\u030c\u0005-\u0000\u0000\u030c\u030e"
+ "\u00030\u0018\u0000\u030d\u030b\u0001\u0000\u0000\u0000\u030e\u0311\u0001"
+ "\u0000\u0000\u0000\u030f\u030d\u0001\u0000\u0000\u0000\u030f\u0310\u0001"
+ "\u0000\u0000\u0000\u0310/\u0001\u0000\u0000\u0000\u0311\u030f\u0001\u0000"
+ "\u0000\u0000\u0312\u031a\u0003\u00b8\\\u0000\u0313\u0314\u0003\u00fa}"
+ "\u0000\u0314\u0315\u0003l6\u0000\u0315\u031a\u0001\u0000\u0000\u0000\u0316"
+ "\u0317\u0003\u00fa}\u0000\u0317\u0318\u0003n7\u0000\u0318\u031a\u0001"
+ "\u0000\u0000\u0000\u0319\u0312\u0001\u0000\u0000\u0000\u0319\u0313\u0001"
+ "\u0000\u0000\u0000\u0319\u0316\u0001\u0000\u0000\u0000\u031a1\u0001\u0000"
+ "\u0000\u0000\u031b\u031d\u0007\u0002\u0000\u0000\u031c\u031b\u0001\u0000"
+ "\u0000\u0000\u031c\u031d\u0001\u0000\u0000\u0000\u031d\u031e\u0001\u0000"
+ "\u0000\u0000\u031e\u031f\u0005E\u0000\u0000\u031f\u0324\u0003\u009aM\u0000"
+ "\u0320\u0321\u0005-\u0000\u0000\u0321\u0323\u0003\u009aM\u0000\u0322\u0320"
+ "\u0001\u0000\u0000\u0000\u0323\u0326\u0001\u0000\u0000\u0000\u0324\u0322"
+ "\u0001\u0000\u0000\u0000\u0324\u0325\u0001\u0000\u0000\u0000\u03253\u0001"
+ "\u0000\u0000\u0000\u0326\u0324\u0001\u0000\u0000\u0000\u0327\u0329\u0005"
+ "\u00b1\u0000\u0000\u0328\u0327\u0001\u0000\u0000\u0000\u0328\u0329\u0001"
+ "\u0000\u0000\u0000\u0329\u032a\u0001\u0000\u0000\u0000\u032a\u032c\u0005"
+ "\u0096\u0000\u0000\u032b\u032d\u00036\u001b\u0000\u032c\u032b\u0001\u0000"
+ "\u0000\u0000\u032c\u032d\u0001\u0000\u0000\u0000\u032d\u032e\u0001\u0000"
+ "\u0000\u0000\u032e\u0332\u0003R)\u0000\u032f\u0331\u00038\u001c\u0000"
+ "\u0330\u032f\u0001\u0000\u0000\u0000\u0331\u0334\u0001\u0000\u0000\u0000"
+ "\u0332\u0330\u0001\u0000\u0000\u0000\u0332\u0333\u0001\u0000\u0000\u0000"
+ "\u0333\u0336\u0001\u0000\u0000\u0000\u0334\u0332\u0001\u0000\u0000\u0000"
+ "\u0335\u0337\u0003\"\u0011\u0000\u0336\u0335\u0001\u0000\u0000\u0000\u0336"
+ "\u0337\u0001\u0000\u0000\u0000\u03375\u0001\u0000\u0000\u0000\u0338\u033e"
+ "\u0005\u00d5\u0000\u0000\u0339\u033b\u0005Y\u0000\u0000\u033a\u033c\u0005"
+ "\u001d\u0000\u0000\u033b\u033a\u0001\u0000\u0000\u0000\u033b\u033c\u0001"
+ "\u0000\u0000\u0000\u033c\u033f\u0001\u0000\u0000\u0000\u033d\u033f\u0005"
+ "Z\u0000\u0000\u033e\u0339\u0001\u0000\u0000\u0000\u033e\u033d\u0001\u0000"
+ "\u0000\u0000\u033f\u0349\u0001\u0000\u0000\u0000\u0340\u0346\u0005J\u0000"
+ "\u0000\u0341\u0343\u0005\u00d2\u0000\u0000\u0342\u0344\u0005\u001d\u0000"
+ "\u0000\u0343\u0342\u0001\u0000\u0000\u0000\u0343\u0344\u0001\u0000\u0000"
+ "\u0000\u0344\u0347\u0001\u0000\u0000\u0000\u0345\u0347\u0005\u00d3\u0000"
+ "\u0000\u0346\u0341\u0001\u0000\u0000\u0000\u0346\u0345\u0001\u0000\u0000"
+ "\u0000\u0347\u0349\u0001\u0000\u0000\u0000\u0348\u0338\u0001\u0000\u0000"
+ "\u0000\u0348\u0340\u0001\u0000\u0000\u0000\u03497\u0001\u0000\u0000\u0000"
+ "\u034a\u0366\u0005\u0112\u0000\u0000\u034b\u0355\u0005|\u0000\u0000\u034c"
+ "\u034d\u0005#\u0000\u0000\u034d\u0355\u0005|\u0000\u0000\u034e\u034f\u0005"
+ "\u00f9\u0000\u0000\u034f\u0355\u0005|\u0000\u0000\u0350\u0351\u0005\u00c9"
+ "\u0000\u0000\u0351\u0355\u0005|\u0000\u0000\u0352\u0353\u0005\u00be\u0000"
+ "\u0000\u0353\u0355\u0005|\u0000\u0000\u0354\u034b\u0001\u0000\u0000\u0000"
+ "\u0354\u034c\u0001\u0000\u0000\u0000\u0354\u034e\u0001\u0000\u0000\u0000"
+ "\u0354\u0350\u0001\u0000\u0000\u0000\u0354\u0352\u0001\u0000\u0000\u0000"
+ "\u0355\u0357\u0001\u0000\u0000\u0000\u0356\u0358\u0005\u00e4\u0000\u0000"
+ "\u0357\u0356\u0001\u0000\u0000\u0000\u0357\u0358\u0001\u0000\u0000\u0000"
+ "\u0358\u0359\u0001\u0000\u0000\u0000\u0359\u035a\u0003\u00fa}\u0000\u035a"
+ "\u035b\u0003t:\u0000\u035b\u035c\u0005\u0092\u0000\u0000\u035c\u035d\u0003"
+ "\u00fc~\u0000\u035d\u035e\u0005\u00e0\u0000\u0000\u035e\u0367\u0001\u0000"
+ "\u0000\u0000\u035f\u0360\u0005\u0083\u0000\u0000\u0360\u0361\u0005\u00af"
+ "\u0000\u0000\u0361\u0367\u0003\u00fc~\u0000\u0362\u0363\u0005\u00e1\u0000"
+ "\u0000\u0363\u0364\u0003\u00fa}\u0000\u0364\u0365\u0003t:\u0000\u0365"
+ "\u0367\u0001\u0000\u0000\u0000\u0366\u0354\u0001\u0000\u0000\u0000\u0366"
+ "\u035f\u0001\u0000\u0000\u0000\u0366\u0362\u0001\u0000\u0000\u0000\u0367"
+ "9\u0001\u0000\u0000\u0000\u0368\u0369\u0005\u0097\u0000\u0000\u0369\u036d"
+ "\u0003V+\u0000\u036a\u036c\u0003<\u001e\u0000\u036b\u036a\u0001\u0000"
+ "\u0000\u0000\u036c\u036f\u0001\u0000\u0000\u0000\u036d\u036b\u0001\u0000"
+ "\u0000\u0000\u036d\u036e\u0001\u0000\u0000\u0000\u036e;\u0001\u0000\u0000"
+ "\u0000\u036f\u036d\u0001\u0000\u0000\u0000\u0370\u0371\u0005\u00af\u0000"
+ "\u0000\u0371\u0372\u0007\u0003\u0000\u0000\u0372\u0373\u0003*\u0015\u0000"
+ "\u0373=\u0001\u0000\u0000\u0000\u0374\u0375\u0005\u010d\u0000\u0000\u0375"
+ "\u0376\u0003\u009aM\u0000\u0376\u0377\u0005\u0017\u0000\u0000\u0377\u0378"
+ "\u0003\u00fa}\u0000\u0378?\u0001\u0000\u0000\u0000\u0379\u037a\u0005&"
+ "\u0000\u0000\u037a\u037b\u0003\u00f8|\u0000\u037b\u0388\u0003\u0252\u0129"
+ "\u0000\u037c\u0385\u0005\u0092\u0000\u0000\u037d\u0382\u0003\u009aM\u0000"
+ "\u037e\u037f\u0005-\u0000\u0000\u037f\u0381\u0003\u009aM\u0000\u0380\u037e"
+ "\u0001\u0000\u0000\u0000\u0381\u0384\u0001\u0000\u0000\u0000\u0382\u0380"
+ "\u0001\u0000\u0000\u0000\u0382\u0383\u0001\u0000\u0000\u0000\u0383\u0386"
+ "\u0001\u0000\u0000\u0000\u0384\u0382\u0001\u0000\u0000\u0000\u0385\u037d"
+ "\u0001\u0000\u0000\u0000\u0385\u0386\u0001\u0000\u0000\u0000\u0386\u0387"
+ "\u0001\u0000\u0000\u0000\u0387\u0389\u0005\u00e0\u0000\u0000\u0388\u037c"
+ "\u0001\u0000\u0000\u0000\u0388\u0389\u0001\u0000\u0000\u0000\u0389\u0399"
+ "\u0001\u0000\u0000\u0000\u038a\u0397\u0005\u011e\u0000\u0000\u038b\u0398"
+ "\u0005\u00fc\u0000\u0000\u038c\u0391\u0003B!\u0000\u038d\u038e\u0005-"
+ "\u0000\u0000\u038e\u0390\u0003B!\u0000\u038f\u038d\u0001\u0000\u0000\u0000"
+ "\u0390\u0393\u0001\u0000\u0000\u0000\u0391\u038f\u0001\u0000\u0000\u0000"
+ "\u0391\u0392\u0001\u0000\u0000\u0000\u0392\u0395\u0001\u0000\u0000\u0000"
+ "\u0393\u0391\u0001\u0000\u0000\u0000\u0394\u0396\u0003\"\u0011\u0000\u0395"
+ "\u0394\u0001\u0000\u0000\u0000\u0395\u0396\u0001\u0000\u0000\u0000\u0396"
+ "\u0398\u0001\u0000\u0000\u0000\u0397\u038b\u0001\u0000\u0000\u0000\u0397"
+ "\u038c\u0001\u0000\u0000\u0000\u0398\u039a\u0001\u0000\u0000\u0000\u0399"
+ "\u038a\u0001\u0000\u0000\u0000\u0399\u039a\u0001\u0000\u0000\u0000\u039a"
+ "A\u0001\u0000\u0000\u0000\u039b\u039e\u0003\u0252\u0129\u0000\u039c\u039d"
+ "\u0005\u0017\u0000\u0000\u039d\u039f\u0003\u00fa}\u0000\u039e\u039c\u0001"
+ "\u0000\u0000\u0000\u039e\u039f\u0001\u0000\u0000\u0000\u039fC\u0001\u0000"
+ "\u0000\u0000\u03a0\u03a1\u0005\u008f\u0000\u0000\u03a1\u03a4\u0005:\u0000"
+ "\u0000\u03a2\u03a3\u0005\u011a\u0000\u0000\u03a3\u03a5\u0005v\u0000\u0000"
+ "\u03a4\u03a2\u0001\u0000\u0000\u0000\u03a4\u03a5\u0001\u0000\u0000\u0000"
+ "\u03a5\u03a6\u0001\u0000\u0000\u0000\u03a6\u03a7\u0005m\u0000\u0000\u03a7"
+ "\u03a8\u0003\u009aM\u0000\u03a8\u03a9\u0005\u0017\u0000\u0000\u03a9\u03ac"
+ "\u0003\u00fa}\u0000\u03aa\u03ab\u0005h\u0000\u0000\u03ab\u03ad\u0003\u024a"
+ "\u0125\u0000\u03ac\u03aa\u0001\u0000\u0000\u0000\u03ac\u03ad\u0001\u0000"
+ "\u0000\u0000\u03adE\u0001\u0000\u0000\u0000\u03ae\u03af\u0005l\u0000\u0000"
+ "\u03af\u03b0\u0005\u0092\u0000\u0000\u03b0\u03b1\u0003\u00fa}\u0000\u03b1"
+ "\u03b2\u0005{\u0000\u0000\u03b2\u03b3\u0003\u009aM\u0000\u03b3\u03b5\u0005"
+ "\u001c\u0000\u0000\u03b4\u03b6\u0003\n\u0005\u0000\u03b5\u03b4\u0001\u0000"
+ "\u0000\u0000\u03b6\u03b7\u0001\u0000\u0000\u0000\u03b7\u03b5\u0001\u0000"
+ "\u0000\u0000\u03b7\u03b8\u0001\u0000\u0000\u0000\u03b8\u03b9\u0001\u0000"
+ "\u0000\u0000\u03b9\u03ba\u0005\u00e0\u0000\u0000\u03baG\u0001\u0000\u0000"
+ "\u0000\u03bb\u03bc\u0005&\u0000\u0000\u03bc\u03bd\u0005\u008a\u0000\u0000"
+ "\u03bd\u03be\u0003\u0006\u0003\u0000\u03be\u03c0\u0005\u00cb\u0000\u0000"
+ "\u03bf\u03c1\u0003J%\u0000\u03c0\u03bf\u0001\u0000\u0000\u0000\u03c0\u03c1"
+ "\u0001\u0000\u0000\u0000\u03c1I\u0001\u0000\u0000\u0000\u03c2\u03c7\u0005"
+ "{\u0000\u0000\u03c3\u03c5\u0003\u009aM\u0000\u03c4\u03c3\u0001\u0000\u0000"
+ "\u0000\u03c4\u03c5\u0001\u0000\u0000\u0000\u03c5\u03c6\u0001\u0000\u0000"
+ "\u0000\u03c6\u03c8\u00052\u0000\u0000\u03c7\u03c4\u0001\u0000\u0000\u0000"
+ "\u03c7\u03c8\u0001\u0000\u0000\u0000\u03c8\u03c9\u0001\u0000\u0000\u0000"
+ "\u03c9\u03cf\u0005\u0103\u0000\u0000\u03ca\u03ce\u0003L&\u0000\u03cb\u03ce"
+ "\u0003N\'\u0000\u03cc\u03ce\u0003P(\u0000\u03cd\u03ca\u0001\u0000\u0000"
+ "\u0000\u03cd\u03cb\u0001\u0000\u0000\u0000\u03cd\u03cc\u0001\u0000\u0000"
+ "\u0000\u03ce\u03d1\u0001\u0000\u0000\u0000\u03cf\u03cd\u0001\u0000\u0000"
+ "\u0000\u03cf\u03d0\u0001\u0000\u0000\u0000\u03d0K\u0001\u0000\u0000\u0000"
+ "\u03d1\u03cf\u0001\u0000\u0000\u0000\u03d2\u03d3\u0005\u00ae\u0000\u0000"
+ "\u03d3\u03d4\u0003\u009aM\u0000\u03d4\u03d5\u0007\u0004\u0000\u0000\u03d5"
+ "M\u0001\u0000\u0000\u0000\u03d6\u03d7\u0005\u00af\u0000\u0000\u03d7\u03d8"
+ "\u0005e\u0000\u0000\u03d8\u03d9\u0007\u0005\u0000\u0000\u03d9O\u0001\u0000"
+ "\u0000\u0000\u03da\u03db\u0005\u00d7\u0000\u0000\u03db\u03dc\u0005\u00f2"
+ "\u0000\u0000\u03dc\u03dd\u0005\u0017\u0000\u0000\u03dd\u03de\u0003\u00fa"
+ "}\u0000\u03deQ\u0001\u0000\u0000\u0000\u03df\u03e4\u0003V+\u0000\u03e0"
+ "\u03e1\u0005-\u0000\u0000\u03e1\u03e3\u0003V+\u0000\u03e2\u03e0\u0001"
+ "\u0000\u0000\u0000\u03e3\u03e6\u0001\u0000\u0000\u0000\u03e4\u03e2\u0001"
+ "\u0000\u0000\u0000\u03e4\u03e5\u0001\u0000\u0000\u0000\u03e5S\u0001\u0000"
+ "\u0000\u0000\u03e6\u03e4\u0001\u0000\u0000\u0000\u03e7\u03ec\u0003X,\u0000"
+ "\u03e8\u03e9\u0005-\u0000\u0000\u03e9\u03eb\u0003X,\u0000\u03ea\u03e8"
+ "\u0001\u0000\u0000\u0000\u03eb\u03ee\u0001\u0000\u0000\u0000\u03ec\u03ea"
+ "\u0001\u0000\u0000\u0000\u03ec\u03ed\u0001\u0000\u0000\u0000\u03edU\u0001"
+ "\u0000\u0000\u0000\u03ee\u03ec\u0001\u0000\u0000\u0000\u03ef\u03f0\u0003"
+ "\u00fa}\u0000\u03f0\u03f1\u0005_\u0000\u0000\u03f1\u03f3\u0001\u0000\u0000"
+ "\u0000\u03f2\u03ef\u0001\u0000\u0000\u0000\u03f2\u03f3\u0001\u0000\u0000"
+ "\u0000\u03f3\u03f5\u0001\u0000\u0000\u0000\u03f4\u03f6\u0003b1\u0000\u03f5"
+ "\u03f4\u0001\u0000\u0000\u0000\u03f5\u03f6\u0001\u0000\u0000\u0000\u03f6"
+ "\u03f7\u0001\u0000\u0000\u0000\u03f7\u03f8\u0003\\.\u0000\u03f8W\u0001"
+ "\u0000\u0000\u0000\u03f9\u03fa\u0003\u0252\u0129\u0000\u03fa\u03fb\u0005"
+ "_\u0000\u0000\u03fb\u03fd\u0001\u0000\u0000\u0000\u03fc\u03f9\u0001\u0000"
+ "\u0000\u0000\u03fc\u03fd\u0001\u0000\u0000\u0000\u03fd\u03fe\u0001\u0000"
+ "\u0000\u0000\u03fe\u0404\u0003h4\u0000\u03ff\u0400\u0003z=\u0000\u0400"
+ "\u0401\u0003h4\u0000\u0401\u0403\u0001\u0000\u0000\u0000\u0402\u03ff\u0001"
+ "\u0000\u0000\u0000\u0403\u0406\u0001\u0000\u0000\u0000\u0404\u0402\u0001"
+ "\u0000\u0000\u0000\u0404\u0405\u0001\u0000\u0000\u0000\u0405Y\u0001\u0000"
+ "\u0000\u0000\u0406\u0404\u0001\u0000\u0000\u0000\u0407\u0408\u0005\u008a"
+ "\u0000\u0000\u0408\u0409\u0005\u0005\u0000\u0000\u0409\u0416\u0005\u00cb"
+ "\u0000\u0000\u040a\u040c\u0005\u008a\u0000\u0000\u040b\u040d\u0005\u0005"
+ "\u0000\u0000\u040c\u040b\u0001\u0000\u0000\u0000\u040c\u040d\u0001\u0000"
+ "\u0000\u0000\u040d\u040e\u0001\u0000\u0000\u0000\u040e\u0410\u0005-\u0000"
+ "\u0000\u040f\u0411\u0005\u0005\u0000\u0000\u0410\u040f\u0001\u0000\u0000"
+ "\u0000\u0410\u0411\u0001\u0000\u0000\u0000\u0411\u0412\u0001\u0000\u0000"
+ "\u0000\u0412\u0416\u0005\u00cb\u0000\u0000\u0413\u0416\u0005\u00bc\u0000"
+ "\u0000\u0414\u0416\u0005\u00fc\u0000\u0000\u0415\u0407\u0001\u0000\u0000"
+ "\u0000\u0415\u040a\u0001\u0000\u0000\u0000\u0415\u0413\u0001\u0000\u0000"
+ "\u0000\u0415\u0414\u0001\u0000\u0000\u0000\u0416[\u0001\u0000\u0000\u0000"
+ "\u0417\u041a\u0003^/\u0000\u0418\u041a\u0003`0\u0000\u0419\u0417\u0001"
+ "\u0000\u0000\u0000\u0419\u0418\u0001\u0000\u0000\u0000\u041a]\u0001\u0000"
+ "\u0000\u0000\u041b\u041c\u0007\u0006\u0000\u0000\u041c\u041d\u0005\u0092"
+ "\u0000\u0000\u041d\u041e\u0003`0\u0000\u041e\u041f\u0005\u00e0\u0000\u0000"
+ "\u041f_\u0001\u0000\u0000\u0000\u0420\u0429\u0003f3\u0000\u0421\u0423"
+ "\u0003x<\u0000\u0422\u0424\u0003Z-\u0000\u0423\u0422\u0001\u0000\u0000"
+ "\u0000\u0423\u0424\u0001\u0000\u0000\u0000\u0424\u0425\u0001\u0000\u0000"
+ "\u0000\u0425\u0426\u0003f3\u0000\u0426\u0428\u0001\u0000\u0000\u0000\u0427"
+ "\u0421\u0001\u0000\u0000\u0000\u0428\u042b\u0001\u0000\u0000\u0000\u0429"
+ "\u0427\u0001\u0000\u0000\u0000\u0429\u042a\u0001\u0000\u0000\u0000\u042a"
+ "\u042e\u0001\u0000\u0000\u0000\u042b\u0429\u0001\u0000\u0000\u0000\u042c"
+ "\u042e\u0003j5\u0000\u042d\u0420\u0001\u0000\u0000\u0000\u042d\u042c\u0001"
+ "\u0000\u0000\u0000\u042e\u042f\u0001\u0000\u0000\u0000\u042f\u042d\u0001"
+ "\u0000\u0000\u0000\u042f\u0430\u0001\u0000\u0000\u0000\u0430a\u0001\u0000"
+ "\u0000\u0000\u0431\u0432\u0005\u0015\u0000\u0000\u0432\u0434\u0005\u00eb"
+ "\u0000\u0000\u0433\u0435\u0005\u00b9\u0000\u0000\u0434\u0433\u0001\u0000"
+ "\u0000\u0000\u0434\u0435\u0001\u0000\u0000\u0000\u0435\u0454\u0001\u0000"
+ "\u0000\u0000\u0436\u0437\u0005\u0012\u0000\u0000\u0437\u0439\u0005\u00eb"
+ "\u0000\u0000\u0438\u043a\u0005\u00b9\u0000\u0000\u0439\u0438\u0001\u0000"
+ "\u0000\u0000\u0439\u043a\u0001\u0000\u0000\u0000\u043a\u0454\u0001\u0000"
+ "\u0000\u0000\u043b\u043d\u0005\u0015\u0000\u0000\u043c\u043e\u0005\u0005"
+ "\u0000\u0000\u043d\u043c\u0001\u0000\u0000\u0000\u043d\u043e\u0001\u0000"
+ "\u0000\u0000\u043e\u0440\u0001\u0000\u0000\u0000\u043f\u0441\u0005\u00b9"
+ "\u0000\u0000\u0440\u043f\u0001\u0000\u0000\u0000\u0440\u0441\u0001\u0000"
+ "\u0000\u0000\u0441\u0454\u0001\u0000\u0000\u0000\u0442\u0444\u0005\u0012"
+ "\u0000\u0000\u0443\u0445\u0005\u00b9\u0000\u0000\u0444\u0443\u0001\u0000"
+ "\u0000\u0000\u0444\u0445\u0001\u0000\u0000\u0000\u0445\u0454\u0001\u0000"
+ "\u0000\u0000\u0446\u0448\u0005\u00eb\u0000\u0000\u0447\u0449\u0005\u0005"
+ "\u0000\u0000\u0448\u0447\u0001\u0000\u0000\u0000\u0448\u0449\u0001\u0000"
+ "\u0000\u0000\u0449\u044b\u0001\u0000\u0000\u0000\u044a\u044c\u0005\u00b9"
+ "\u0000\u0000\u044b\u044a\u0001\u0000\u0000\u0000\u044b\u044c\u0001\u0000"
+ "\u0000\u0000\u044c\u044d\u0001\u0000\u0000\u0000\u044d\u0454\u0005t\u0000"
+ "\u0000\u044e\u044f\u0005\u00eb\u0000\u0000\u044f\u0451\u0005\u0005\u0000"
+ "\u0000\u0450\u0452\u0005\u00b9\u0000\u0000\u0451\u0450\u0001\u0000\u0000"
+ "\u0000\u0451\u0452\u0001\u0000\u0000\u0000\u0452\u0454\u0001\u0000\u0000"
+ "\u0000\u0453\u0431\u0001\u0000\u0000\u0000\u0453\u0436\u0001\u0000\u0000"
+ "\u0000\u0453\u043b\u0001\u0000\u0000\u0000\u0453\u0442\u0001\u0000\u0000"
+ "\u0000\u0453\u0446\u0001\u0000\u0000\u0000\u0453\u044e\u0001\u0000\u0000"
+ "\u0000\u0454c\u0001\u0000\u0000\u0000\u0455\u0459\u0003f3\u0000\u0456"
+ "\u0457\u0003x<\u0000\u0457\u0458\u0003f3\u0000\u0458\u045a\u0001\u0000"
+ "\u0000\u0000\u0459\u0456\u0001\u0000\u0000\u0000\u045a\u045b\u0001\u0000"
+ "\u0000\u0000\u045b\u0459\u0001\u0000\u0000\u0000\u045b\u045c\u0001\u0000"
+ "\u0000\u0000\u045ce\u0001\u0000\u0000\u0000\u045d\u045f\u0005\u0092\u0000"
+ "\u0000\u045e\u0460\u0003\u00fa}\u0000\u045f\u045e\u0001\u0000\u0000\u0000"
+ "\u045f\u0460\u0001\u0000\u0000\u0000\u0460\u0462\u0001\u0000\u0000\u0000"
+ "\u0461\u0463\u0003\u0084B\u0000\u0462\u0461\u0001\u0000\u0000\u0000\u0462"
+ "\u0463\u0001\u0000\u0000\u0000\u0463\u0465\u0001\u0000\u0000\u0000\u0464"
+ "\u0466\u0003v;\u0000\u0465\u0464\u0001\u0000\u0000\u0000\u0465\u0466\u0001"
+ "\u0000\u0000\u0000\u0466\u0469\u0001\u0000\u0000\u0000\u0467\u0468\u0005"
+ "\u0119\u0000\u0000\u0468\u046a\u0003\u009aM\u0000\u0469\u0467\u0001\u0000"
+ "\u0000\u0000\u0469\u046a\u0001\u0000\u0000\u0000\u046a\u046b\u0001\u0000"
+ "\u0000\u0000\u046b\u046c\u0005\u00e0\u0000\u0000\u046cg\u0001\u0000\u0000"
+ "\u0000\u046d\u046f\u0005\u0092\u0000\u0000\u046e\u0470\u0003\u00fa}\u0000"
+ "\u046f\u046e\u0001\u0000\u0000\u0000\u046f\u0470\u0001\u0000\u0000\u0000"
+ "\u0470\u0472\u0001\u0000\u0000\u0000\u0471\u0473\u0003\u0096K\u0000\u0472"
+ "\u0471\u0001\u0000\u0000\u0000\u0472\u0473\u0001\u0000\u0000\u0000\u0473"
+ "\u0475\u0001\u0000\u0000\u0000\u0474\u0476\u0003v;\u0000\u0475\u0474\u0001"
+ "\u0000\u0000\u0000\u0475\u0476\u0001\u0000\u0000\u0000\u0476\u0477\u0001"
+ "\u0000\u0000\u0000\u0477\u0478\u0005\u00e0\u0000\u0000\u0478i\u0001\u0000"
+ "\u0000\u0000\u0479\u047a\u0005\u0092\u0000\u0000\u047a\u047d\u0003V+\u0000"
+ "\u047b\u047c\u0005\u0119\u0000\u0000\u047c\u047e\u0003\u009aM\u0000\u047d"
+ "\u047b\u0001\u0000\u0000\u0000\u047d\u047e\u0001\u0000\u0000\u0000\u047e"
+ "\u047f\u0001\u0000\u0000\u0000\u047f\u0481\u0005\u00e0\u0000\u0000\u0480"
+ "\u0482\u0003Z-\u0000\u0481\u0480\u0001\u0000\u0000\u0000\u0481\u0482\u0001"
+ "\u0000\u0000\u0000\u0482k\u0001\u0000\u0000\u0000\u0483\u0485\u0003p8"
+ "\u0000\u0484\u0483\u0001\u0000\u0000\u0000\u0485\u0486\u0001\u0000\u0000"
+ "\u0000\u0486\u0484\u0001\u0000\u0000\u0000\u0486\u0487\u0001\u0000\u0000"
+ "\u0000\u0487m\u0001\u0000\u0000\u0000\u0488\u0489\u0005\u0082\u0000\u0000"
+ "\u0489\u048d\u0003\u0252\u0129\u0000\u048a\u048c\u0003p8\u0000\u048b\u048a"
+ "\u0001\u0000\u0000\u0000\u048c\u048f\u0001\u0000\u0000\u0000\u048d\u048b"
+ "\u0001\u0000\u0000\u0000\u048d\u048e\u0001\u0000\u0000\u0000\u048eo\u0001"
+ "\u0000\u0000\u0000\u048f\u048d\u0001\u0000\u0000\u0000\u0490\u0491\u0005"
+ "+\u0000\u0000\u0491\u0492\u0003\u0252\u0129\u0000\u0492q\u0001\u0000\u0000"
+ "\u0000\u0493\u0494\u0005+\u0000\u0000\u0494\u0495\u0003\u0252\u0129\u0000"
+ "\u0495s\u0001\u0000\u0000\u0000\u0496\u0497\u0005+\u0000\u0000\u0497\u0498"
+ "\u0003\u0252\u0129\u0000\u0498u\u0001\u0000\u0000\u0000\u0499\u049c\u0003"
+ "\u0250\u0128\u0000\u049a\u049c\u0003\u00eew\u0000\u049b\u0499\u0001\u0000"
+ "\u0000\u0000\u049b\u049a\u0001\u0000\u0000\u0000\u049cw\u0001\u0000\u0000"
+ "\u0000\u049d\u049f\u0003|>\u0000\u049e\u049d\u0001\u0000\u0000\u0000\u049e"
+ "\u049f\u0001\u0000\u0000\u0000\u049f\u04a0\u0001\u0000\u0000\u0000\u04a0"
+ "\u04b3\u0003~?\u0000\u04a1\u04a3\u0005\u0089\u0000\u0000\u04a2\u04a4\u0003"
+ "\u00fa}\u0000\u04a3\u04a2\u0001\u0000\u0000\u0000\u04a3\u04a4\u0001\u0000"
+ "\u0000\u0000\u04a4\u04a6\u0001\u0000\u0000\u0000\u04a5\u04a7\u0003\u0084"
+ "B\u0000\u04a6\u04a5\u0001\u0000\u0000\u0000\u04a6\u04a7\u0001\u0000\u0000"
+ "\u0000\u04a7\u04a9\u0001\u0000\u0000\u0000\u04a8\u04aa\u0003\u0082A\u0000"
+ "\u04a9\u04a8\u0001\u0000\u0000\u0000\u04a9\u04aa\u0001\u0000\u0000\u0000"
+ "\u04aa\u04ac\u0001\u0000\u0000\u0000\u04ab\u04ad\u0003v;\u0000\u04ac\u04ab"
+ "\u0001\u0000\u0000\u0000\u04ac\u04ad\u0001\u0000\u0000\u0000\u04ad\u04b0"
+ "\u0001\u0000\u0000\u0000\u04ae\u04af\u0005\u0119\u0000\u0000\u04af\u04b1"
+ "\u0003\u009aM\u0000\u04b0\u04ae\u0001\u0000\u0000\u0000\u04b0\u04b1\u0001"
+ "\u0000\u0000\u0000\u04b1\u04b2\u0001\u0000\u0000\u0000\u04b2\u04b4\u0005"
+ "\u00ca\u0000\u0000\u04b3\u04a1\u0001\u0000\u0000\u0000\u04b3\u04b4\u0001"
+ "\u0000\u0000\u0000\u04b4\u04b5\u0001\u0000\u0000\u0000\u04b5\u04b7\u0003"
+ "~?\u0000\u04b6\u04b8\u0003\u0080@\u0000\u04b7\u04b6\u0001\u0000\u0000"
+ "\u0000\u04b7\u04b8\u0001\u0000\u0000\u0000\u04b8y\u0001\u0000\u0000\u0000"
+ "\u04b9\u04bb\u0003|>\u0000\u04ba\u04b9\u0001\u0000\u0000\u0000\u04ba\u04bb"
+ "\u0001\u0000\u0000\u0000\u04bb\u04bc\u0001\u0000\u0000\u0000\u04bc\u04bd"
+ "\u0003~?\u0000\u04bd\u04bf\u0005\u0089\u0000\u0000\u04be\u04c0\u0003\u00fa"
+ "}\u0000\u04bf\u04be\u0001\u0000\u0000\u0000\u04bf\u04c0\u0001\u0000\u0000"
+ "\u0000\u04c0\u04c1\u0001\u0000\u0000\u0000\u04c1\u04c3\u0003\u0098L\u0000"
+ "\u04c2\u04c4\u0003v;\u0000\u04c3\u04c2\u0001\u0000\u0000\u0000\u04c3\u04c4"
+ "\u0001\u0000\u0000\u0000\u04c4\u04c5\u0001\u0000\u0000\u0000\u04c5\u04c6"
+ "\u0005\u00ca\u0000\u0000\u04c6\u04c8\u0003~?\u0000\u04c7\u04c9\u0003\u0080"
+ "@\u0000\u04c8\u04c7\u0001\u0000\u0000\u0000\u04c8\u04c9\u0001\u0000\u0000"
+ "\u0000\u04c9{\u0001\u0000\u0000\u0000\u04ca\u04cb\u0007\u0007\u0000\u0000"
+ "\u04cb}\u0001\u0000\u0000\u0000\u04cc\u04cd\u0007\b\u0000\u0000\u04cd"
+ "\u007f\u0001\u0000\u0000\u0000\u04ce\u04cf\u0007\t\u0000\u0000\u04cf\u0081"
+ "\u0001\u0000\u0000\u0000\u04d0\u04d9\u0005\u00fc\u0000\u0000\u04d1\u04d3"
+ "\u0005\u0005\u0000\u0000\u04d2\u04d1\u0001\u0000\u0000\u0000\u04d2\u04d3"
+ "\u0001\u0000\u0000\u0000\u04d3\u04d4\u0001\u0000\u0000\u0000\u04d4\u04d6"
+ "\u0005O\u0000\u0000\u04d5\u04d7\u0005\u0005\u0000\u0000\u04d6\u04d5\u0001"
+ "\u0000\u0000\u0000\u04d6\u04d7\u0001\u0000\u0000\u0000\u04d7\u04da\u0001"
+ "\u0000\u0000\u0000\u04d8\u04da\u0005\u0005\u0000\u0000\u04d9\u04d2\u0001"
+ "\u0000\u0000\u0000\u04d9\u04d8\u0001\u0000\u0000\u0000\u04d9\u04da\u0001"
+ "\u0000\u0000\u0000\u04da\u0083\u0001\u0000\u0000\u0000\u04db\u04dc\u0005"
+ "+\u0000\u0000\u04dc\u04e0\u0003\u0086C\u0000\u04dd\u04de\u0005\u0082\u0000"
+ "\u0000\u04de\u04e0\u0003\u0088D\u0000\u04df\u04db\u0001\u0000\u0000\u0000"
+ "\u04df\u04dd\u0001\u0000\u0000\u0000\u04e0\u0085\u0001\u0000\u0000\u0000"
+ "\u04e1\u04e9\u0003\u008aE\u0000\u04e2\u04e4\u0005\u001c\u0000\u0000\u04e3"
+ "\u04e5\u0005+\u0000\u0000\u04e4\u04e3\u0001\u0000\u0000\u0000\u04e4\u04e5"
+ "\u0001\u0000\u0000\u0000\u04e5\u04e6\u0001\u0000\u0000\u0000\u04e6\u04e8"
+ "\u0003\u008aE\u0000\u04e7\u04e2\u0001\u0000\u0000\u0000\u04e8\u04eb\u0001"
+ "\u0000\u0000\u0000\u04e9\u04e7\u0001\u0000\u0000\u0000\u04e9\u04ea\u0001"
+ "\u0000\u0000\u0000\u04ea\u0087\u0001\u0000\u0000\u0000\u04eb\u04e9\u0001"
+ "\u0000\u0000\u0000\u04ec\u04f4\u0003\u008cF\u0000\u04ed\u04ef\u0005\u001c"
+ "\u0000\u0000\u04ee\u04f0\u0005+\u0000\u0000\u04ef\u04ee\u0001\u0000\u0000"
+ "\u0000\u04ef\u04f0\u0001\u0000\u0000\u0000\u04f0\u04f1\u0001\u0000\u0000"
+ "\u0000\u04f1\u04f3\u0003\u008cF\u0000\u04f2\u04ed\u0001\u0000\u0000\u0000"
+ "\u04f3\u04f6\u0001\u0000\u0000\u0000\u04f4\u04f2\u0001\u0000\u0000\u0000"
+ "\u04f4\u04f5\u0001\u0000\u0000\u0000\u04f5\u0089\u0001\u0000\u0000\u0000"
+ "\u04f6\u04f4\u0001\u0000\u0000\u0000\u04f7\u04fc\u0003\u008eG\u0000\u04f8"
+ "\u04f9\u0007\n\u0000\u0000\u04f9\u04fb\u0003\u008eG\u0000\u04fa\u04f8"
+ "\u0001\u0000\u0000\u0000\u04fb\u04fe\u0001\u0000\u0000\u0000\u04fc\u04fa"
+ "\u0001\u0000\u0000\u0000\u04fc\u04fd\u0001\u0000\u0000\u0000\u04fd\u008b"
+ "\u0001\u0000\u0000\u0000\u04fe\u04fc\u0001\u0000\u0000\u0000\u04ff\u0504"
+ "\u0003\u0090H\u0000\u0500\u0501\u0007\n\u0000\u0000\u0501\u0503\u0003"
+ "\u0090H\u0000\u0502\u0500\u0001\u0000\u0000\u0000\u0503\u0506\u0001\u0000"
+ "\u0000\u0000\u0504\u0502\u0001\u0000\u0000\u0000\u0504\u0505\u0001\u0000"
+ "\u0000\u0000\u0505\u008d\u0001\u0000\u0000\u0000\u0506\u0504\u0001\u0000"
+ "\u0000\u0000\u0507\u0509\u0005\u0088\u0000\u0000\u0508\u0507\u0001\u0000"
+ "\u0000\u0000\u0509\u050c\u0001\u0000\u0000\u0000\u050a\u0508\u0001\u0000"
+ "\u0000\u0000\u050a\u050b\u0001\u0000\u0000\u0000\u050b\u050d\u0001\u0000"
+ "\u0000\u0000\u050c\u050a\u0001\u0000\u0000\u0000\u050d\u050e\u0003\u0092"
+ "I\u0000\u050e\u008f\u0001\u0000\u0000\u0000\u050f\u0511\u0005\u0088\u0000"
+ "\u0000\u0510\u050f\u0001\u0000\u0000\u0000\u0511\u0514\u0001\u0000\u0000"
+ "\u0000\u0512\u0510\u0001\u0000\u0000\u0000\u0512\u0513\u0001\u0000\u0000"
+ "\u0000\u0513\u0515\u0001\u0000\u0000\u0000\u0514\u0512\u0001\u0000\u0000"
+ "\u0000\u0515\u0516\u0003\u0094J\u0000\u0516\u0091\u0001\u0000\u0000\u0000"
+ "\u0517\u0518\u0005\u0092\u0000\u0000\u0518\u0519\u0003\u0086C\u0000\u0519"
+ "\u051a\u0005\u00e0\u0000\u0000\u051a\u051e\u0001\u0000\u0000\u0000\u051b"
+ "\u051e\u0005\u0099\u0000\u0000\u051c\u051e\u0003\u0252\u0129\u0000\u051d"
+ "\u0517\u0001\u0000\u0000\u0000\u051d\u051b\u0001\u0000\u0000\u0000\u051d"
+ "\u051c\u0001\u0000\u0000\u0000\u051e\u0093\u0001\u0000\u0000\u0000\u051f"
+ "\u0520\u0005\u0092\u0000\u0000\u0520\u0521\u0003\u0088D\u0000\u0521\u0522"
+ "\u0005\u00e0\u0000\u0000\u0522\u0526\u0001\u0000\u0000\u0000\u0523\u0526"
+ "\u0005\u0099\u0000\u0000\u0524\u0526\u0003\u0258\u012c\u0000\u0525\u051f"
+ "\u0001\u0000\u0000\u0000\u0525\u0523\u0001\u0000\u0000\u0000\u0525\u0524"
+ "\u0001\u0000\u0000\u0000\u0526\u0095\u0001\u0000\u0000\u0000\u0527\u0528"
+ "\u0007\u000b\u0000\u0000\u0528\u052d\u0003\u0252\u0129\u0000\u0529\u052a"
+ "\u0007\n\u0000\u0000\u052a\u052c\u0003\u0252\u0129\u0000\u052b\u0529\u0001"
+ "\u0000\u0000\u0000\u052c\u052f\u0001\u0000\u0000\u0000\u052d\u052b\u0001"
+ "\u0000\u0000\u0000\u052d\u052e\u0001\u0000\u0000\u0000\u052e\u0097\u0001"
+ "\u0000\u0000\u0000\u052f\u052d\u0001\u0000\u0000\u0000\u0530\u0531\u0007"
+ "\u000b\u0000\u0000\u0531\u0532\u0003\u0252\u0129\u0000\u0532\u0099\u0001"
+ "\u0000\u0000\u0000\u0533\u0538\u0003\u009cN\u0000\u0534\u0535\u0005\u00b4"
+ "\u0000\u0000\u0535\u0537\u0003\u009cN\u0000\u0536\u0534\u0001\u0000\u0000"
+ "\u0000\u0537\u053a\u0001\u0000\u0000\u0000\u0538\u0536\u0001\u0000\u0000"
+ "\u0000\u0538\u0539\u0001\u0000\u0000\u0000\u0539\u009b\u0001\u0000\u0000"
+ "\u0000\u053a\u0538\u0001\u0000\u0000\u0000\u053b\u0540\u0003\u009eO\u0000"
+ "\u053c\u053d\u0005\u011d\u0000\u0000\u053d\u053f\u0003\u009eO\u0000\u053e"
+ "\u053c\u0001\u0000\u0000\u0000\u053f\u0542\u0001\u0000\u0000\u0000\u0540"
+ "\u053e\u0001\u0000\u0000\u0000\u0540\u0541\u0001\u0000\u0000\u0000\u0541"
+ "\u009d\u0001\u0000\u0000\u0000\u0542\u0540\u0001\u0000\u0000\u0000\u0543"
+ "\u0548\u0003\u00a0P\u0000\u0544\u0545\u0005\u0014\u0000\u0000\u0545\u0547"
+ "\u0003\u00a0P\u0000\u0546\u0544\u0001\u0000\u0000\u0000\u0547\u054a\u0001"
+ "\u0000\u0000\u0000\u0548\u0546\u0001\u0000\u0000\u0000\u0548\u0549\u0001"
+ "\u0000\u0000\u0000\u0549\u009f\u0001\u0000\u0000\u0000\u054a\u0548\u0001"
+ "\u0000\u0000\u0000\u054b\u054d\u0005\u00aa\u0000\u0000\u054c\u054b\u0001"
+ "\u0000\u0000\u0000\u054d\u0550\u0001\u0000\u0000\u0000\u054e\u054c\u0001"
+ "\u0000\u0000\u0000\u054e\u054f\u0001\u0000\u0000\u0000\u054f\u0551\u0001"
+ "\u0000\u0000\u0000\u0550\u054e\u0001\u0000\u0000\u0000\u0551\u0552\u0003"
+ "\u00a2Q\u0000\u0552\u00a1\u0001\u0000\u0000\u0000\u0553\u0558\u0003\u00a4"
+ "R\u0000\u0554\u0555\u0007\f\u0000\u0000\u0555\u0557\u0003\u00a4R\u0000"
+ "\u0556\u0554\u0001\u0000\u0000\u0000\u0557\u055a\u0001\u0000\u0000\u0000"
+ "\u0558\u0556\u0001\u0000\u0000\u0000\u0558\u0559\u0001\u0000\u0000\u0000"
+ "\u0559\u00a3\u0001\u0000\u0000\u0000\u055a\u0558\u0001\u0000\u0000\u0000"
+ "\u055b\u055d\u0003\u00aaU\u0000\u055c\u055e\u0003\u00a6S\u0000\u055d\u055c"
+ "\u0001\u0000\u0000\u0000\u055d\u055e\u0001\u0000\u0000\u0000\u055e\u00a5"
+ "\u0001\u0000\u0000\u0000\u055f\u0567\u0005\u00d0\u0000\u0000\u0560\u0561"
+ "\u0005\u00f1\u0000\u0000\u0561\u0567\u0005\u011a\u0000\u0000\u0562\u0563"
+ "\u0005^\u0000\u0000\u0563\u0567\u0005\u011a\u0000\u0000\u0564\u0567\u0005"
+ "5\u0000\u0000\u0565\u0567\u0005{\u0000\u0000\u0566\u055f\u0001\u0000\u0000"
+ "\u0000\u0566\u0560\u0001\u0000\u0000\u0000\u0566\u0562\u0001\u0000\u0000"
+ "\u0000\u0566\u0564\u0001\u0000\u0000\u0000\u0566\u0565\u0001\u0000\u0000"
+ "\u0000\u0567\u0568\u0001\u0000\u0000\u0000\u0568\u0580\u0003\u00aaU\u0000"
+ "\u0569\u056b\u0005\u0082\u0000\u0000\u056a\u056c\u0005\u00aa\u0000\u0000"
+ "\u056b\u056a\u0001\u0000\u0000\u0000\u056b\u056c\u0001\u0000\u0000\u0000"
+ "\u056c\u056d\u0001\u0000\u0000\u0000\u056d\u0580\u0005\u00ad\u0000\u0000"
+ "\u056e\u0570\u0005\u0082\u0000\u0000\u056f\u0571\u0005\u00aa\u0000\u0000"
+ "\u0570\u056f\u0001\u0000\u0000\u0000\u0570\u0571\u0001\u0000\u0000\u0000"
+ "\u0571\u0572\u0001\u0000\u0000\u0000\u0572\u0575\u0007\r\u0000\u0000\u0573"
+ "\u0575\u0005,\u0000\u0000\u0574\u056e\u0001\u0000\u0000\u0000\u0574\u0573"
+ "\u0001\u0000\u0000\u0000\u0575\u0576\u0001\u0000\u0000\u0000\u0576\u0580"
+ "\u0003\u0142\u00a1\u0000\u0577\u0579\u0005\u0082\u0000\u0000\u0578\u057a"
+ "\u0005\u00aa\u0000\u0000\u0579\u0578\u0001\u0000\u0000\u0000\u0579\u057a"
+ "\u0001\u0000\u0000\u0000\u057a\u057c\u0001\u0000\u0000\u0000\u057b\u057d"
+ "\u0003\u00a8T\u0000\u057c\u057b\u0001\u0000\u0000\u0000\u057c\u057d\u0001"
+ "\u0000\u0000\u0000\u057d\u057e\u0001\u0000\u0000\u0000\u057e\u0580\u0005"
+ "\u00a9\u0000\u0000\u057f\u0566\u0001\u0000\u0000\u0000\u057f\u0569\u0001"
+ "\u0000\u0000\u0000\u057f\u0574\u0001\u0000\u0000\u0000\u057f\u0577\u0001"
+ "\u0000\u0000\u0000\u0580\u00a7\u0001\u0000\u0000\u0000\u0581\u0582\u0007"
+ "\u000e\u0000\u0000\u0582\u00a9\u0001\u0000\u0000\u0000\u0583\u0588\u0003"
+ "\u00acV\u0000\u0584\u0585\u0007\u000f\u0000\u0000\u0585\u0587\u0003\u00ac"
+ "V\u0000\u0586\u0584\u0001\u0000\u0000\u0000\u0587\u058a\u0001\u0000\u0000"
+ "\u0000\u0588\u0586\u0001\u0000\u0000\u0000\u0588\u0589\u0001\u0000\u0000"
+ "\u0000\u0589\u00ab\u0001\u0000\u0000\u0000\u058a\u0588\u0001\u0000\u0000"
+ "\u0000\u058b\u0590\u0003\u00aeW\u0000\u058c\u058d\u0007\u0010\u0000\u0000"
+ "\u058d\u058f\u0003\u00aeW\u0000\u058e\u058c\u0001\u0000\u0000\u0000\u058f"
+ "\u0592\u0001\u0000\u0000\u0000\u0590\u058e\u0001\u0000\u0000\u0000\u0590"
+ "\u0591\u0001\u0000\u0000\u0000\u0591\u00ad\u0001\u0000\u0000\u0000\u0592"
+ "\u0590\u0001\u0000\u0000\u0000\u0593\u0598\u0003\u00b0X\u0000\u0594\u0595"
+ "\u0005\u00c0\u0000\u0000\u0595\u0597\u0003\u00b0X\u0000\u0596\u0594\u0001"
+ "\u0000\u0000\u0000\u0597\u059a\u0001\u0000\u0000\u0000\u0598\u0596\u0001"
+ "\u0000\u0000\u0000\u0598\u0599\u0001\u0000\u0000\u0000\u0599\u00af\u0001"
+ "\u0000\u0000\u0000\u059a\u0598\u0001\u0000\u0000\u0000\u059b\u059f\u0003"
+ "\u00b2Y\u0000\u059c\u059d\u0007\u0011\u0000\u0000\u059d\u059f\u0003\u00b2"
+ "Y\u0000\u059e\u059b\u0001\u0000\u0000\u0000\u059e\u059c\u0001\u0000\u0000"
+ "\u0000\u059f\u00b1\u0001\u0000\u0000\u0000\u05a0\u05a4\u0003\u00ba]\u0000"
+ "\u05a1\u05a3\u0003\u00b4Z\u0000\u05a2\u05a1\u0001\u0000\u0000\u0000\u05a3"
+ "\u05a6\u0001\u0000\u0000\u0000\u05a4\u05a2\u0001\u0000\u0000\u0000\u05a4"
+ "\u05a5\u0001\u0000\u0000\u0000\u05a5\u00b3\u0001\u0000\u0000\u0000\u05a6"
+ "\u05a4\u0001\u0000\u0000\u0000\u05a7\u05b7\u0003\u00b6[\u0000\u05a8\u05b7"
+ "\u0003\u0084B\u0000\u05a9\u05aa\u0005\u0089\u0000\u0000\u05aa\u05ab\u0003"
+ "\u009aM\u0000\u05ab\u05ac\u0005\u00ca\u0000\u0000\u05ac\u05b7\u0001\u0000"
+ "\u0000\u0000\u05ad\u05af\u0005\u0089\u0000\u0000\u05ae\u05b0\u0003\u009a"
+ "M\u0000\u05af\u05ae\u0001\u0000\u0000\u0000\u05af\u05b0\u0001\u0000\u0000"
+ "\u0000\u05b0\u05b1\u0001\u0000\u0000\u0000\u05b1\u05b3\u0005O\u0000\u0000"
+ "\u05b2\u05b4\u0003\u009aM\u0000\u05b3\u05b2\u0001\u0000\u0000\u0000\u05b3"
+ "\u05b4\u0001\u0000\u0000\u0000\u05b4\u05b5\u0001\u0000\u0000\u0000\u05b5"
+ "\u05b7\u0005\u00ca\u0000\u0000\u05b6\u05a7\u0001\u0000\u0000\u0000\u05b6"
+ "\u05a8\u0001\u0000\u0000\u0000\u05b6\u05a9\u0001\u0000\u0000\u0000\u05b6"
+ "\u05ad\u0001\u0000\u0000\u0000\u05b7\u00b5\u0001\u0000\u0000\u0000\u05b8"
+ "\u05b9\u0005N\u0000\u0000\u05b9\u05ba\u0003\u00ecv\u0000\u05ba\u00b7\u0001"
+ "\u0000\u0000\u0000\u05bb\u05bd\u0003\u00ba]\u0000\u05bc\u05be\u0003\u00b6"
+ "[\u0000\u05bd\u05bc\u0001\u0000\u0000\u0000\u05be\u05bf\u0001\u0000\u0000"
+ "\u0000\u05bf\u05bd\u0001\u0000\u0000\u0000\u05bf\u05c0\u0001\u0000\u0000"
+ "\u0000\u05c0\u00b9\u0001\u0000\u0000\u0000\u05c1\u05d7\u0003\u00bc^\u0000"
+ "\u05c2\u05d7\u0003\u00eew\u0000\u05c3\u05d7\u0003\u00be_\u0000\u05c4\u05d7"
+ "\u0003\u00c2a\u0000\u05c5\u05d7\u0003\u00deo\u0000\u05c6\u05d7\u0003\u00e0"
+ "p\u0000\u05c7\u05d7\u0003\u00e2q\u0000\u05c8\u05d7\u0003\u00e4r\u0000"
+ "\u05c9\u05d7\u0003\u00dam\u0000\u05ca\u05d7\u0003\u00c8d\u0000\u05cb\u05d7"
+ "\u0003\u00eau\u0000\u05cc\u05d7\u0003\u00cae\u0000\u05cd\u05d7\u0003\u00cc"
+ "f\u0000\u05ce\u05d7\u0003\u00ceg\u0000\u05cf\u05d7\u0003\u00d0h\u0000"
+ "\u05d0\u05d7\u0003\u00d2i\u0000\u05d1\u05d7\u0003\u00d4j\u0000\u05d2\u05d7"
+ "\u0003\u00d6k\u0000\u05d3\u05d7\u0003\u00d8l\u0000\u05d4\u05d7\u0003\u00f2"
+ "y\u0000\u05d5\u05d7\u0003\u00fa}\u0000\u05d6\u05c1\u0001\u0000\u0000\u0000"
+ "\u05d6\u05c2\u0001\u0000\u0000\u0000\u05d6\u05c3\u0001\u0000\u0000\u0000"
+ "\u05d6\u05c4\u0001\u0000\u0000\u0000\u05d6\u05c5\u0001\u0000\u0000\u0000"
+ "\u05d6\u05c6\u0001\u0000\u0000\u0000\u05d6\u05c7\u0001\u0000\u0000\u0000"
+ "\u05d6\u05c8\u0001\u0000\u0000\u0000\u05d6\u05c9\u0001\u0000\u0000\u0000"
+ "\u05d6\u05ca\u0001\u0000\u0000\u0000\u05d6\u05cb\u0001\u0000\u0000\u0000"
+ "\u05d6\u05cc\u0001\u0000\u0000\u0000\u05d6\u05cd\u0001\u0000\u0000\u0000"
+ "\u05d6\u05ce\u0001\u0000\u0000\u0000\u05d6\u05cf\u0001\u0000\u0000\u0000"
+ "\u05d6\u05d0\u0001\u0000\u0000\u0000\u05d6\u05d1\u0001\u0000\u0000\u0000"
+ "\u05d6\u05d2\u0001\u0000\u0000\u0000\u05d6\u05d3\u0001\u0000\u0000\u0000"
+ "\u05d6\u05d4\u0001\u0000\u0000\u0000\u05d6\u05d5\u0001\u0000\u0000\u0000"
+ "\u05d7\u00bb\u0001\u0000\u0000\u0000\u05d8\u05e1\u0003\u00e6s\u0000\u05d9"
+ "\u05e1\u0003\u024a\u0125\u0000\u05da\u05e1\u0003\u0250\u0128\u0000\u05db"
+ "\u05e1\u0005\u0106\u0000\u0000\u05dc\u05e1\u0005g\u0000\u0000\u05dd\u05e1"
+ "\u0005~\u0000\u0000\u05de\u05e1\u0005\u009e\u0000\u0000\u05df\u05e1\u0005"
+ "\u00ad\u0000\u0000\u05e0\u05d8\u0001\u0000\u0000\u0000\u05e0\u05d9\u0001"
+ "\u0000\u0000\u0000\u05e0\u05da\u0001\u0000\u0000\u0000\u05e0\u05db\u0001"
+ "\u0000\u0000\u0000\u05e0\u05dc\u0001\u0000\u0000\u0000\u05e0\u05dd\u0001"
+ "\u0000\u0000\u0000\u05e0\u05de\u0001\u0000\u0000\u0000\u05e0\u05df\u0001"
+ "\u0000\u0000\u0000\u05e1\u00bd\u0001\u0000\u0000\u0000\u05e2\u05e4\u0005"
+ "\'\u0000\u0000\u05e3\u05e5\u0003\u00c0`\u0000\u05e4\u05e3\u0001\u0000"
+ "\u0000\u0000\u05e5\u05e6\u0001\u0000\u0000\u0000\u05e6\u05e4\u0001\u0000"
+ "\u0000\u0000\u05e6\u05e7\u0001\u0000\u0000\u0000\u05e7\u05ea\u0001\u0000"
+ "\u0000\u0000\u05e8\u05e9\u0005[\u0000\u0000\u05e9\u05eb\u0003\u009aM\u0000"
+ "\u05ea\u05e8\u0001\u0000\u0000\u0000\u05ea\u05eb\u0001\u0000\u0000\u0000"
+ "\u05eb\u05ec\u0001\u0000\u0000\u0000\u05ec\u05ed\u0005]\u0000\u0000\u05ed"
+ "\u00bf\u0001\u0000\u0000\u0000\u05ee\u05ef\u0005\u0118\u0000\u0000\u05ef"
+ "\u05f0\u0003\u009aM\u0000\u05f0\u05f1\u0005\u00fa\u0000\u0000\u05f1\u05f2"
+ "\u0003\u009aM\u0000\u05f2\u00c1\u0001\u0000\u0000\u0000\u05f3\u05f4\u0005"
+ "\'\u0000\u0000\u05f4\u05f6\u0003\u009aM\u0000\u05f5\u05f7\u0003\u00c4"
+ "b\u0000\u05f6\u05f5\u0001\u0000\u0000\u0000\u05f7\u05f8\u0001\u0000\u0000"
+ "\u0000\u05f8\u05f6\u0001\u0000\u0000\u0000\u05f8\u05f9\u0001\u0000\u0000"
+ "\u0000\u05f9\u05fc\u0001\u0000\u0000\u0000\u05fa\u05fb\u0005[\u0000\u0000"
+ "\u05fb\u05fd\u0003\u009aM\u0000\u05fc\u05fa\u0001\u0000\u0000\u0000\u05fc"
+ "\u05fd\u0001\u0000\u0000\u0000\u05fd\u05fe\u0001\u0000\u0000\u0000\u05fe"
+ "\u05ff\u0005]\u0000\u0000\u05ff\u00c3\u0001\u0000\u0000\u0000\u0600\u0601"
+ "\u0005\u0118\u0000\u0000\u0601\u0606\u0003\u00c6c\u0000\u0602\u0603\u0005"
+ "-\u0000\u0000\u0603\u0605\u0003\u00c6c\u0000\u0604\u0602\u0001\u0000\u0000"
+ "\u0000\u0605\u0608\u0001\u0000\u0000\u0000\u0606\u0604\u0001\u0000\u0000"
+ "\u0000\u0606\u0607\u0001\u0000\u0000\u0000\u0607\u0609\u0001\u0000\u0000"
+ "\u0000\u0608\u0606\u0001\u0000\u0000\u0000\u0609\u060a\u0005\u00fa\u0000"
+ "\u0000\u060a\u060b\u0003\u009aM\u0000\u060b\u00c5\u0001\u0000\u0000\u0000"
+ "\u060c\u0612\u0005\u00d0\u0000\u0000\u060d\u060e\u0005\u00f1\u0000\u0000"
+ "\u060e\u0612\u0005\u011a\u0000\u0000\u060f\u0610\u0005^\u0000\u0000\u0610"
+ "\u0612\u0005\u011a\u0000\u0000\u0611\u060c\u0001\u0000\u0000\u0000\u0611"
+ "\u060d\u0001\u0000\u0000\u0000\u0611\u060f\u0001\u0000\u0000\u0000\u0612"
+ "\u0613\u0001\u0000\u0000\u0000\u0613\u062e\u0003\u00aaU\u0000\u0614\u0616"
+ "\u0005\u0082\u0000\u0000\u0615\u0617\u0005\u00aa\u0000\u0000\u0616\u0615"
+ "\u0001\u0000\u0000\u0000\u0616\u0617\u0001\u0000\u0000\u0000\u0617\u0618"
+ "\u0001\u0000\u0000\u0000\u0618\u062e\u0005\u00ad\u0000\u0000\u0619\u061b"
+ "\u0005\u0082\u0000\u0000\u061a\u061c\u0005\u00aa\u0000\u0000\u061b\u061a"
+ "\u0001\u0000\u0000\u0000\u061b\u061c\u0001\u0000\u0000\u0000\u061c\u061d"
+ "\u0001\u0000\u0000\u0000\u061d\u0620\u0005\u0108\u0000\u0000\u061e\u0620"
+ "\u0005,\u0000\u0000\u061f\u0619\u0001\u0000\u0000\u0000\u061f\u061e\u0001"
+ "\u0000\u0000\u0000\u0620\u0621\u0001\u0000\u0000\u0000\u0621\u062e\u0003"
+ "\u0142\u00a1\u0000\u0622\u0624\u0005\u0082\u0000\u0000\u0623\u0625\u0005"
+ "\u00aa\u0000\u0000\u0624\u0623\u0001\u0000\u0000\u0000\u0624\u0625\u0001"
+ "\u0000\u0000\u0000\u0625\u0627\u0001\u0000\u0000\u0000\u0626\u0628\u0003"
+ "\u00a8T\u0000\u0627\u0626\u0001\u0000\u0000\u0000\u0627\u0628\u0001\u0000"
+ "\u0000\u0000\u0628\u0629\u0001\u0000\u0000\u0000\u0629\u062e\u0005\u00a9"
+ "\u0000\u0000\u062a\u062b\u0007\f\u0000\u0000\u062b\u062e\u0003\u00a4R"
+ "\u0000\u062c\u062e\u0003\u009aM\u0000\u062d\u0611\u0001\u0000\u0000\u0000"
+ "\u062d\u0614\u0001\u0000\u0000\u0000\u062d\u061f\u0001\u0000\u0000\u0000"
+ "\u062d\u0622\u0001\u0000\u0000\u0000\u062d\u062a\u0001\u0000\u0000\u0000"
+ "\u062d\u062c\u0001\u0000\u0000\u0000\u062e\u00c7\u0001\u0000\u0000\u0000"
+ "\u062f\u0630\u0005\u0089\u0000\u0000\u0630\u0631\u0003\u00fa}\u0000\u0631"
+ "\u0632\u0005{\u0000\u0000\u0632\u063d\u0003\u009aM\u0000\u0633\u0634\u0005"
+ "\u0119\u0000\u0000\u0634\u0636\u0003\u009aM\u0000\u0635\u0633\u0001\u0000"
+ "\u0000\u0000\u0635\u0636\u0001\u0000\u0000\u0000\u0636\u0637\u0001\u0000"
+ "\u0000\u0000\u0637\u0638\u0005\u001c\u0000\u0000\u0638\u063e\u0003\u009a"
+ "M\u0000\u0639\u063a\u0005\u0119\u0000\u0000\u063a\u063c\u0003\u009aM\u0000"
+ "\u063b\u0639\u0001\u0000\u0000\u0000\u063b\u063c\u0001\u0000\u0000\u0000"
+ "\u063c\u063e\u0001\u0000\u0000\u0000\u063d\u0635\u0001\u0000\u0000\u0000"
+ "\u063d\u063b\u0001\u0000\u0000\u0000\u063e\u063f\u0001\u0000\u0000\u0000"
+ "\u063f\u0640\u0005\u00ca\u0000\u0000\u0640\u00c9\u0001\u0000\u0000\u0000"
+ "\u0641\u0645\u0005\u0089\u0000\u0000\u0642\u0643\u0003\u00fa}\u0000\u0643"
+ "\u0644\u0005_\u0000\u0000\u0644\u0646\u0001\u0000\u0000\u0000\u0645\u0642"
+ "\u0001\u0000\u0000\u0000\u0645\u0646\u0001\u0000\u0000\u0000\u0646\u0647"
+ "\u0001\u0000\u0000\u0000\u0647\u064a\u0003d2\u0000\u0648\u0649\u0005\u0119"
+ "\u0000\u0000\u0649\u064b\u0003\u009aM\u0000\u064a\u0648\u0001\u0000\u0000"
+ "\u0000\u064a\u064b\u0001\u0000\u0000\u0000\u064b\u064c\u0001\u0000\u0000"
+ "\u0000\u064c\u064d\u0005\u001c\u0000\u0000\u064d\u064e\u0003\u009aM\u0000"
+ "\u064e\u064f\u0005\u00ca\u0000\u0000\u064f\u00cb\u0001\u0000\u0000\u0000"
+ "\u0650\u0651\u0005\u00ce\u0000\u0000\u0651\u0652\u0005\u0092\u0000\u0000"
+ "\u0652\u0653\u0003\u00fa}\u0000\u0653\u0654\u0005_\u0000\u0000\u0654\u0655"
+ "\u0003\u009aM\u0000\u0655\u0656\u0005-\u0000\u0000\u0656\u0657\u0003\u00fa"
+ "}\u0000\u0657\u0658\u0005{\u0000\u0000\u0658\u0659\u0003\u009aM\u0000"
+ "\u0659\u065a\u0005\u001c\u0000\u0000\u065a\u065b\u0003\u009aM\u0000\u065b"
+ "\u065c\u0005\u00e0\u0000\u0000\u065c\u00cd\u0001\u0000\u0000\u0000\u065d"
+ "\u065e\u0007\u0012\u0000\u0000\u065e\u065f\u0005\u0092\u0000\u0000\u065f"
+ "\u0660\u0003\u00fa}\u0000\u0660\u0661\u0005{\u0000\u0000\u0661\u0664\u0003"
+ "\u009aM\u0000\u0662\u0663\u0005\u0119\u0000\u0000\u0663\u0665\u0003\u009a"
+ "M\u0000\u0664\u0662\u0001\u0000\u0000\u0000\u0664\u0665\u0001\u0000\u0000"
+ "\u0000\u0665\u0666\u0001\u0000\u0000\u0000\u0666\u0667\u0005\u00e0\u0000"
+ "\u0000\u0667\u00cf\u0001\u0000\u0000\u0000\u0668\u0669\u0005\u00a8\u0000"
+ "\u0000\u0669\u066a\u0005\u0092\u0000\u0000\u066a\u066d\u0003\u009aM\u0000"
+ "\u066b\u066c\u0005-\u0000\u0000\u066c\u066e\u0003\u00a8T\u0000\u066d\u066b"
+ "\u0001\u0000\u0000\u0000\u066d\u066e\u0001\u0000\u0000\u0000\u066e\u066f"
+ "\u0001\u0000\u0000\u0000\u066f\u0670\u0005\u00e0\u0000\u0000\u0670\u00d1"
+ "\u0001\u0000\u0000\u0000\u0671\u0672\u0005\u0105\u0000\u0000\u0672\u067a"
+ "\u0005\u0092\u0000\u0000\u0673\u0675\u0007\u0013\u0000\u0000\u0674\u0673"
+ "\u0001\u0000\u0000\u0000\u0674\u0675\u0001\u0000\u0000\u0000\u0675\u0677"
+ "\u0001\u0000\u0000\u0000\u0676\u0678\u0003\u009aM\u0000\u0677\u0676\u0001"
+ "\u0000\u0000\u0000\u0677\u0678\u0001\u0000\u0000\u0000\u0678\u0679\u0001"
+ "\u0000\u0000\u0000\u0679\u067b\u0005m\u0000\u0000\u067a\u0674\u0001\u0000"
+ "\u0000\u0000\u067a\u067b\u0001\u0000\u0000\u0000\u067b\u067c\u0001\u0000"
+ "\u0000\u0000\u067c\u067d\u0003\u009aM\u0000\u067d\u067e\u0005\u00e0\u0000"
+ "\u0000\u067e\u00d3\u0001\u0000\u0000\u0000\u067f\u0680\u0003d2\u0000\u0680"
+ "\u00d5\u0001\u0000\u0000\u0000\u0681\u0682\u0003^/\u0000\u0682\u00d7\u0001"
+ "\u0000\u0000\u0000\u0683\u0684\u0005\u0092\u0000\u0000\u0684\u0685\u0003"
+ "\u009aM\u0000\u0685\u0686\u0005\u00e0\u0000\u0000\u0686\u00d9\u0001\u0000"
+ "\u0000\u0000\u0687\u0688\u0003\u00fa}\u0000\u0688\u0691\u0005\u008a\u0000"
+ "\u0000\u0689\u068e\u0003\u00dcn\u0000\u068a\u068b\u0005-\u0000\u0000\u068b"
+ "\u068d\u0003\u00dcn\u0000\u068c\u068a\u0001\u0000\u0000\u0000\u068d\u0690"
+ "\u0001\u0000\u0000\u0000\u068e\u068c\u0001\u0000\u0000\u0000\u068e\u068f"
+ "\u0001\u0000\u0000\u0000\u068f\u0692\u0001\u0000\u0000\u0000\u0690\u068e"
+ "\u0001\u0000\u0000\u0000\u0691\u0689\u0001\u0000\u0000\u0000\u0691\u0692"
+ "\u0001\u0000\u0000\u0000\u0692\u0693\u0001\u0000\u0000\u0000\u0693\u0694"
+ "\u0005\u00cb\u0000\u0000\u0694\u00db\u0001\u0000\u0000\u0000\u0695\u0696"
+ "\u0003\u00ecv\u0000\u0696\u0697\u0005+\u0000\u0000\u0697\u0698\u0003\u009a"
+ "M\u0000\u0698\u069e\u0001\u0000\u0000\u0000\u0699\u069e\u0003\u00b6[\u0000"
+ "\u069a\u069e\u0003\u00fa}\u0000\u069b\u069c\u0005N\u0000\u0000\u069c\u069e"
+ "\u0005\u00fc\u0000\u0000\u069d\u0695\u0001\u0000\u0000\u0000\u069d\u0699"
+ "\u0001\u0000\u0000\u0000\u069d\u069a\u0001\u0000\u0000\u0000\u069d\u069b"
+ "\u0001\u0000\u0000\u0000\u069e\u00dd\u0001\u0000\u0000\u0000\u069f\u06a0"
+ "\u00058\u0000\u0000\u06a0\u06a1\u0005\u0092\u0000\u0000\u06a1\u06a2\u0005"
+ "\u00fc\u0000\u0000\u06a2\u06a3\u0005\u00e0\u0000\u0000\u06a3\u00df\u0001"
+ "\u0000\u0000\u0000\u06a4\u06a5\u0005d\u0000\u0000\u06a5\u06ae\u0005\u008a"
+ "\u0000\u0000\u06a6\u06af\u0003\u0006\u0003\u0000\u06a7\u06a9\u00036\u001b"
+ "\u0000\u06a8\u06a7\u0001\u0000\u0000\u0000\u06a8\u06a9\u0001\u0000\u0000"
+ "\u0000\u06a9\u06aa\u0001\u0000\u0000\u0000\u06aa\u06ac\u0003R)\u0000\u06ab"
+ "\u06ad\u0003\"\u0011\u0000\u06ac\u06ab\u0001\u0000\u0000\u0000\u06ac\u06ad"
+ "\u0001\u0000\u0000\u0000\u06ad\u06af\u0001\u0000\u0000\u0000\u06ae\u06a6"
+ "\u0001\u0000\u0000\u0000\u06ae\u06a8\u0001\u0000\u0000\u0000\u06af\u06b0"
+ "\u0001\u0000\u0000\u0000\u06b0\u06b1\u0005\u00cb\u0000\u0000\u06b1\u00e1"
+ "\u0001\u0000\u0000\u0000\u06b2\u06b3\u00058\u0000\u0000\u06b3\u06bc\u0005"
+ "\u008a\u0000\u0000\u06b4\u06bd\u0003\u0006\u0003\u0000\u06b5\u06b7\u0003"
+ "6\u001b\u0000\u06b6\u06b5\u0001\u0000\u0000\u0000\u06b6\u06b7\u0001\u0000"
+ "\u0000\u0000\u06b7\u06b8\u0001\u0000\u0000\u0000\u06b8\u06ba\u0003R)\u0000"
+ "\u06b9\u06bb\u0003\"\u0011\u0000\u06ba\u06b9\u0001\u0000\u0000\u0000\u06ba"
+ "\u06bb\u0001\u0000\u0000\u0000\u06bb\u06bd\u0001\u0000\u0000\u0000\u06bc"
+ "\u06b4\u0001\u0000\u0000\u0000\u06bc\u06b6\u0001\u0000\u0000\u0000\u06bd"
+ "\u06be\u0001\u0000\u0000\u0000\u06be\u06bf\u0005\u00cb\u0000\u0000\u06bf"
+ "\u00e3\u0001\u0000\u0000\u0000\u06c0\u06c1\u0005*\u0000\u0000\u06c1\u06c2"
+ "\u0005\u008a\u0000\u0000\u06c2\u06c3\u0003\u0006\u0003\u0000\u06c3\u06c4"
+ "\u0005\u00cb\u0000\u0000\u06c4\u00e5\u0001\u0000\u0000\u0000\u06c5\u06c7"
+ "\u0005\u0098\u0000\u0000\u06c6\u06c5\u0001\u0000\u0000\u0000\u06c6\u06c7"
+ "\u0001\u0000\u0000\u0000\u06c7\u06c8\u0001\u0000\u0000\u0000\u06c8\u06c9"
+ "\u0007\u0014\u0000\u0000\u06c9\u00e7\u0001\u0000\u0000\u0000\u06ca\u06cc"
+ "\u0005\u0098\u0000\u0000\u06cb\u06ca\u0001\u0000\u0000\u0000\u06cb\u06cc"
+ "\u0001\u0000\u0000\u0000\u06cc\u06cd\u0001\u0000\u0000\u0000\u06cd\u06ce"
+ "\u0005\u0005\u0000\u0000\u06ce\u00e9\u0001\u0000\u0000\u0000\u06cf\u06d8"
+ "\u0005\u0089\u0000\u0000\u06d0\u06d5\u0003\u009aM\u0000\u06d1\u06d2\u0005"
+ "-\u0000\u0000\u06d2\u06d4\u0003\u009aM\u0000\u06d3\u06d1\u0001\u0000\u0000"
+ "\u0000\u06d4\u06d7\u0001\u0000\u0000\u0000\u06d5\u06d3\u0001\u0000\u0000"
+ "\u0000\u06d5\u06d6\u0001\u0000\u0000\u0000\u06d6\u06d9\u0001\u0000\u0000"
+ "\u0000\u06d7\u06d5\u0001\u0000\u0000\u0000\u06d8\u06d0\u0001\u0000\u0000"
+ "\u0000\u06d8\u06d9\u0001\u0000\u0000\u0000\u06d9\u06da\u0001\u0000\u0000"
+ "\u0000\u06da\u06db\u0005\u00ca\u0000\u0000\u06db\u00eb\u0001\u0000\u0000"
+ "\u0000\u06dc\u06dd\u0003\u0252\u0129\u0000\u06dd\u00ed\u0001\u0000\u0000"
+ "\u0000\u06de\u06df\u0005K\u0000\u0000\u06df\u06e0\u0003\u00f0x\u0000\u06e0"
+ "\u00ef\u0001\u0000\u0000\u0000\u06e1\u06e4\u0003\u0252\u0129\u0000\u06e2"
+ "\u06e4\u0005\u0005\u0000\u0000\u06e3\u06e1\u0001\u0000\u0000\u0000\u06e3"
+ "\u06e2\u0001\u0000\u0000\u0000\u06e4\u00f1\u0001\u0000\u0000\u0000\u06e5"
+ "\u06e6\u0003\u00f6{\u0000\u06e6\u06e8\u0005\u0092\u0000\u0000\u06e7\u06e9"
+ "\u0007\u0000\u0000\u0000\u06e8\u06e7\u0001\u0000\u0000\u0000\u06e8\u06e9"
+ "\u0001\u0000\u0000\u0000\u06e9\u06f2\u0001\u0000\u0000\u0000\u06ea\u06ef"
+ "\u0003\u00f4z\u0000\u06eb\u06ec\u0005-\u0000\u0000\u06ec\u06ee\u0003\u00f4"
+ "z\u0000\u06ed\u06eb\u0001\u0000\u0000\u0000\u06ee\u06f1\u0001\u0000\u0000"
+ "\u0000\u06ef\u06ed\u0001\u0000\u0000\u0000\u06ef\u06f0\u0001\u0000\u0000"
+ "\u0000\u06f0\u06f3\u0001\u0000\u0000\u0000\u06f1\u06ef\u0001\u0000\u0000"
+ "\u0000\u06f2\u06ea\u0001\u0000\u0000\u0000\u06f2\u06f3\u0001\u0000\u0000"
+ "\u0000\u06f3\u06f4\u0001\u0000\u0000\u0000\u06f4\u06f5\u0005\u00e0\u0000"
+ "\u0000\u06f5\u00f3\u0001\u0000\u0000\u0000\u06f6\u06f7\u0003\u009aM\u0000"
+ "\u06f7\u00f5\u0001\u0000\u0000\u0000\u06f8\u06f9\u0003\u00f8|\u0000\u06f9"
+ "\u06fa\u0003\u0252\u0129\u0000\u06fa\u00f7\u0001\u0000\u0000\u0000\u06fb"
+ "\u06fc\u0003\u0252\u0129\u0000\u06fc\u06fd\u0005N\u0000\u0000\u06fd\u06ff"
+ "\u0001\u0000\u0000\u0000\u06fe\u06fb\u0001\u0000\u0000\u0000\u06ff\u0702"
+ "\u0001\u0000\u0000\u0000\u0700\u06fe\u0001\u0000\u0000\u0000\u0700\u0701"
+ "\u0001\u0000\u0000\u0000\u0701\u00f9\u0001\u0000\u0000\u0000\u0702\u0700"
+ "\u0001\u0000\u0000\u0000\u0703\u0704\u0003\u0252\u0129\u0000\u0704\u00fb"
+ "\u0001\u0000\u0000\u0000\u0705\u070a\u0003\u0252\u0129\u0000\u0706\u0707"
+ "\u0005-\u0000\u0000\u0707\u0709\u0003\u0252\u0129\u0000\u0708\u0706\u0001"
+ "\u0000\u0000\u0000\u0709\u070c\u0001\u0000\u0000\u0000\u070a\u0708\u0001"
+ "\u0000\u0000\u0000\u070a\u070b\u0001\u0000\u0000\u0000\u070b\u00fd\u0001"
+ "\u0000\u0000\u0000\u070c\u070a\u0001\u0000\u0000\u0000\u070d\u070f\u0003"
+ "\f\u0006\u0000\u070e\u070d\u0001\u0000\u0000\u0000\u070e\u070f\u0001\u0000"
+ "\u0000\u0000\u070f\u071d\u0001\u0000\u0000\u0000\u0710\u071e\u0003\u0100"
+ "\u0080\u0000\u0711\u071e\u0003\u0102\u0081\u0000\u0712\u071e\u0003\u0104"
+ "\u0082\u0000\u0713\u071e\u0003\u0106\u0083\u0000\u0714\u071e\u0003\u01ae"
+ "\u00d7\u0000\u0715\u071e\u0003\u01b0\u00d8\u0000\u0716\u071e\u0003\u01aa"
+ "\u00d5\u0000\u0717\u071e\u0003\u0216\u010b\u0000\u0718\u071e\u0003\u0218"
+ "\u010c\u0000\u0719\u071e\u0003\u016c\u00b6\u0000\u071a\u071e\u0003\u0176"
+ "\u00bb\u0000\u071b\u071e\u0003\u0108\u0084\u0000\u071c\u071e\u0003\u0134"
+ "\u009a\u0000\u071d\u0710\u0001\u0000\u0000\u0000\u071d\u0711\u0001\u0000"
+ "\u0000\u0000\u071d\u0712\u0001\u0000\u0000\u0000\u071d\u0713\u0001\u0000"
+ "\u0000\u0000\u071d\u0714\u0001\u0000\u0000\u0000\u071d\u0715\u0001\u0000"
+ "\u0000\u0000\u071d\u0716\u0001\u0000\u0000\u0000\u071d\u0717\u0001\u0000"
+ "\u0000\u0000\u071d\u0718\u0001\u0000\u0000\u0000\u071d\u0719\u0001\u0000"
+ "\u0000\u0000\u071d\u071a\u0001\u0000\u0000\u0000\u071d\u071b\u0001\u0000"
+ "\u0000\u0000\u071d\u071c\u0001\u0000\u0000\u0000\u071e\u00ff\u0001\u0000"
+ "\u0000\u0000\u071f\u0722\u00059\u0000\u0000\u0720\u0721\u0005\u00b4\u0000"
+ "\u0000\u0721\u0723\u0005\u00d6\u0000\u0000\u0722\u0720\u0001\u0000\u0000"
+ "\u0000\u0722\u0723\u0001\u0000\u0000\u0000\u0723\u072b\u0001\u0000\u0000"
+ "\u0000\u0724\u072c\u0003\u0228\u0114\u0000\u0725\u072c\u0003\u0204\u0102"
+ "\u0000\u0726\u072c\u0003\u0150\u00a8\u0000\u0727\u072c\u0003\u0206\u0103"
+ "\u0000\u0728\u072c\u0003\u0156\u00ab\u0000\u0729\u072c\u0003\u017c\u00be"
+ "\u0000\u072a\u072c\u0003\u0186\u00c3\u0000\u072b\u0724\u0001\u0000\u0000"
+ "\u0000\u072b\u0725\u0001\u0000\u0000\u0000\u072b\u0726\u0001\u0000\u0000"
+ "\u0000\u072b\u0727\u0001\u0000\u0000\u0000\u072b\u0728\u0001\u0000\u0000"
+ "\u0000\u072b\u0729\u0001\u0000\u0000\u0000\u072b\u072a\u0001\u0000\u0000"
+ "\u0000\u072c\u0101\u0001\u0000\u0000\u0000\u072d\u0735\u0005R\u0000\u0000"
+ "\u072e\u0736\u0003\u022a\u0115\u0000\u072f\u0736\u0003\u0154\u00aa\u0000"
+ "\u0730\u0736\u0003\u020c\u0106\u0000\u0731\u0736\u0003\u0168\u00b4\u0000"
+ "\u0732\u0736\u0003\u017e\u00bf\u0000\u0733\u0736\u0003\u0172\u00b9\u0000"
+ "\u0734\u0736\u0003\u0188\u00c4\u0000\u0735\u072e\u0001\u0000\u0000\u0000"
+ "\u0735\u072f\u0001\u0000\u0000\u0000\u0735\u0730\u0001\u0000\u0000\u0000"
+ "\u0735\u0731\u0001\u0000\u0000\u0000\u0735\u0732\u0001\u0000\u0000\u0000"
+ "\u0735\u0733\u0001\u0000\u0000\u0000\u0735\u0734\u0001\u0000\u0000\u0000"
+ "\u0736\u0103\u0001\u0000\u0000\u0000\u0737\u073d\u0005\u0013\u0000\u0000"
+ "\u0738\u073e\u0003\u022c\u0116\u0000\u0739\u073e\u0003\u018c\u00c6\u0000"
+ "\u073a\u073e\u0003\u020e\u0107\u0000\u073b\u073e\u0003\u018e\u00c7\u0000"
+ "\u073c\u073e\u0003\u016e\u00b7\u0000\u073d\u0738\u0001\u0000\u0000\u0000"
+ "\u073d\u0739\u0001\u0000\u0000\u0000\u073d\u073a\u0001\u0000\u0000\u0000"
+ "\u073d\u073b\u0001\u0000\u0000\u0000\u073d\u073c\u0001\u0000\u0000\u0000"
+ "\u073e\u0105\u0001\u0000\u0000\u0000\u073f\u0743\u0005\u00cf\u0000\u0000"
+ "\u0740\u0744\u0003\u0180\u00c0\u0000\u0741\u0744\u0003\u0170\u00b8\u0000"
+ "\u0742\u0744\u0003\u018a\u00c5\u0000\u0743\u0740\u0001\u0000\u0000\u0000"
+ "\u0743\u0741\u0001\u0000\u0000\u0000\u0743\u0742\u0001\u0000\u0000\u0000"
+ "\u0744\u0107\u0001\u0000\u0000\u0000\u0745\u0756\u0005\u00ec\u0000\u0000"
+ "\u0746\u0757\u0003\u0238\u011c\u0000\u0747\u0757\u0003\u011c\u008e\u0000"
+ "\u0748\u0757\u0003\u019c\u00ce\u0000\u0749\u0757\u0003\u021c\u010e\u0000"
+ "\u074a\u0757\u0003\u012c\u0096\u0000\u074b\u0757\u0003\u0116\u008b\u0000"
+ "\u074c\u0757\u0003\u019e\u00cf\u0000\u074d\u0757\u0003\u012a\u0095\u0000"
+ "\u074e\u0757\u0003\u01a2\u00d1\u0000\u074f\u0757\u0003\u0182\u00c1\u0000"
+ "\u0750\u0757\u0003\u0174\u00ba\u0000\u0751\u0757\u0003\u0138\u009c\u0000"
+ "\u0752\u0757\u0003\u01a0\u00d0\u0000\u0753\u0757\u0003\u0132\u0099\u0000"
+ "\u0754\u0757\u0003\u01a4\u00d2\u0000\u0755\u0757\u0003\u019a\u00cd\u0000"
+ "\u0756\u0746\u0001\u0000\u0000\u0000\u0756\u0747\u0001\u0000\u0000\u0000"
+ "\u0756\u0748\u0001\u0000\u0000\u0000\u0756\u0749\u0001\u0000\u0000\u0000"
+ "\u0756\u074a\u0001\u0000\u0000\u0000\u0756\u074b\u0001\u0000\u0000\u0000"
+ "\u0756\u074c\u0001\u0000\u0000\u0000\u0756\u074d\u0001\u0000\u0000\u0000"
+ "\u0756\u074e\u0001\u0000\u0000\u0000\u0756\u074f\u0001\u0000\u0000\u0000"
+ "\u0756\u0750\u0001\u0000\u0000\u0000\u0756\u0751\u0001\u0000\u0000\u0000"
+ "\u0756\u0752\u0001\u0000\u0000\u0000\u0756\u0753\u0001\u0000\u0000\u0000"
+ "\u0756\u0754\u0001\u0000\u0000\u0000\u0756\u0755\u0001\u0000\u0000\u0000"
+ "\u0757\u0109\u0001\u0000\u0000\u0000\u0758\u075a\u0003\u0112\u0089\u0000"
+ "\u0759\u075b\u0003\u0012\t\u0000\u075a\u0759\u0001\u0000\u0000\u0000\u075a"
+ "\u075b\u0001\u0000\u0000\u0000\u075b\u075e\u0001\u0000\u0000\u0000\u075c"
+ "\u075e\u0003\"\u0011\u0000\u075d\u0758\u0001\u0000\u0000\u0000\u075d\u075c"
+ "\u0001\u0000\u0000\u0000\u075e\u010b\u0001\u0000\u0000\u0000\u075f\u0762"
+ "\u0003\u00fa}\u0000\u0760\u0761\u0005\u0017\u0000\u0000\u0761\u0763\u0003"
+ "\u00fa}\u0000\u0762\u0760\u0001\u0000\u0000\u0000\u0762\u0763\u0001\u0000"
+ "\u0000\u0000\u0763\u010d\u0001\u0000\u0000\u0000\u0764\u0765\u0005\u00ef"
+ "\u0000\u0000\u0765\u0766\u0003\u00e8t\u0000\u0766\u010f\u0001\u0000\u0000"
+ "\u0000\u0767\u0768\u0005\u008d\u0000\u0000\u0768\u0769\u0003\u00e8t\u0000"
+ "\u0769\u0111\u0001\u0000\u0000\u0000\u076a\u0774\u0005\u011e\u0000\u0000"
+ "\u076b\u0775\u0005\u00fc\u0000\u0000\u076c\u0771\u0003\u010c\u0086\u0000"
+ "\u076d\u076e\u0005-\u0000\u0000\u076e\u0770\u0003\u010c\u0086\u0000\u076f"
+ "\u076d\u0001\u0000\u0000\u0000\u0770\u0773\u0001\u0000\u0000\u0000\u0771"
+ "\u076f\u0001\u0000\u0000\u0000\u0771\u0772\u0001\u0000\u0000\u0000\u0772"
+ "\u0775\u0001\u0000\u0000\u0000\u0773\u0771\u0001\u0000\u0000\u0000\u0774"
+ "\u076b\u0001\u0000\u0000\u0000\u0774\u076c\u0001\u0000\u0000\u0000\u0775"
+ "\u0777\u0001\u0000\u0000\u0000\u0776\u0778\u0003\u001c\u000e\u0000\u0777"
+ "\u0776\u0001\u0000\u0000\u0000\u0777\u0778\u0001\u0000\u0000\u0000\u0778"
+ "\u077a\u0001\u0000\u0000\u0000\u0779\u077b\u0003\u010e\u0087\u0000\u077a"
+ "\u0779\u0001\u0000\u0000\u0000\u077a\u077b\u0001\u0000\u0000\u0000\u077b"
+ "\u077d\u0001\u0000\u0000\u0000\u077c\u077e\u0003\u0110\u0088\u0000\u077d"
+ "\u077c\u0001\u0000\u0000\u0000\u077d\u077e\u0001\u0000\u0000\u0000\u077e"
+ "\u0780\u0001\u0000\u0000\u0000\u077f\u0781\u0003\"\u0011\u0000\u0780\u077f"
+ "\u0001\u0000\u0000\u0000\u0780\u0781\u0001\u0000\u0000\u0000\u0781\u0113"
+ "\u0001\u0000\u0000\u0000\u0782\u0784\u0007\u0015\u0000\u0000\u0783\u0785"
+ "\u0005\u00b6\u0000\u0000\u0784\u0783\u0001\u0000\u0000\u0000\u0784\u0785"
+ "\u0001\u0000\u0000\u0000\u0785\u078c\u0001\u0000\u0000\u0000\u0786\u0788"
+ "\u0003\u0112\u0089\u0000\u0787\u0789\u0003\u0012\t\u0000\u0788\u0787\u0001"
+ "\u0000\u0000\u0000\u0788\u0789\u0001\u0000\u0000\u0000\u0789\u078c\u0001"
+ "\u0000\u0000\u0000\u078a\u078c\u0003\"\u0011\u0000\u078b\u0782\u0001\u0000"
+ "\u0000\u0000\u078b\u0786\u0001\u0000\u0000\u0000\u078b\u078a\u0001\u0000"
+ "\u0000\u0000\u078c\u0115\u0001\u0000\u0000\u0000\u078d\u078e\u0007\u0016"
+ "\u0000\u0000\u078e\u0794\u0003\u011a\u008d\u0000\u078f\u0791\u0007\u0017"
+ "\u0000\u0000\u0790\u078f\u0001\u0000\u0000\u0000\u0790\u0791\u0001\u0000"
+ "\u0000\u0000\u0791\u0792\u0001\u0000\u0000\u0000\u0792\u0794\u0003\u0118"
+ "\u008c\u0000\u0793\u078d\u0001\u0000\u0000\u0000\u0793\u0790\u0001\u0000"
+ "\u0000\u0000\u0794\u0117\u0001\u0000\u0000\u0000\u0795\u0797\u0003\u01e0"
+ "\u00f0\u0000\u0796\u0798\u0003\u0114\u008a\u0000\u0797\u0796\u0001\u0000"
+ "\u0000\u0000\u0797\u0798\u0001\u0000\u0000\u0000\u0798\u079a\u0001\u0000"
+ "\u0000\u0000\u0799\u079b\u0003\u013c\u009e\u0000\u079a\u0799\u0001\u0000"
+ "\u0000\u0000\u079a\u079b\u0001\u0000\u0000\u0000\u079b\u0119\u0001\u0000"
+ "\u0000\u0000\u079c\u079e\u0003\u01e0\u00f0\u0000\u079d\u079f\u0003\u010a"
+ "\u0085\u0000\u079e\u079d\u0001\u0000\u0000\u0000\u079e\u079f\u0001\u0000"
+ "\u0000\u0000\u079f\u07a1\u0001\u0000\u0000\u0000\u07a0\u07a2\u0003\u013c"
+ "\u009e\u0000\u07a1\u07a0\u0001\u0000\u0000\u0000\u07a1\u07a2\u0001\u0000"
+ "\u0000\u0000\u07a2\u011b\u0001\u0000\u0000\u0000\u07a3\u07a5\u0007\u0018"
+ "\u0000\u0000\u07a4\u07a3\u0001\u0000\u0000\u0000\u07a4\u07a5\u0001\u0000"
+ "\u0000\u0000\u07a5\u07a6\u0001\u0000\u0000\u0000\u07a6\u07a7\u0003\u011e"
+ "\u008f\u0000\u07a7\u07a8\u0003\u0128\u0094\u0000\u07a8\u07be\u0001\u0000"
+ "\u0000\u0000\u07a9\u07aa\u0007\u0018\u0000\u0000\u07aa\u07ab\u0005\u010b"
+ "\u0000\u0000\u07ab\u07be\u0003\u0128\u0094\u0000\u07ac\u07ae\u0007\u0019"
+ "\u0000\u0000\u07ad\u07ac\u0001\u0000\u0000\u0000\u07ad\u07ae\u0001\u0000"
+ "\u0000\u0000\u07ae\u07af\u0001\u0000\u0000\u0000\u07af\u07b0\u0005\u0084"
+ "\u0000\u0000\u07b0\u07be\u0003\u0128\u0094\u0000\u07b1\u07b2\u0005\u00d1"
+ "\u0000\u0000\u07b2\u07b3\u0005b\u0000\u0000\u07b3\u07be\u0003\u0128\u0094"
+ "\u0000\u07b4\u07b6\u0007\u001a\u0000\u0000\u07b5\u07b4\u0001\u0000\u0000"
+ "\u0000\u07b5\u07b6\u0001\u0000\u0000\u0000\u07b6\u07b7\u0001\u0000\u0000"
+ "\u0000\u07b7\u07b8\u0005d\u0000\u0000\u07b8\u07be\u0003\u0126\u0093\u0000"
+ "\u07b9\u07bb\u0003\u0122\u0091\u0000\u07ba\u07b9\u0001\u0000\u0000\u0000"
+ "\u07ba\u07bb\u0001\u0000\u0000\u0000\u07bb\u07bc\u0001\u0000\u0000\u0000"
+ "\u07bc\u07be\u0003\u0124\u0092\u0000\u07bd\u07a4\u0001\u0000\u0000\u0000"
+ "\u07bd\u07a9\u0001\u0000\u0000\u0000\u07bd\u07ad\u0001\u0000\u0000\u0000"
+ "\u07bd\u07b1\u0001\u0000\u0000\u0000\u07bd\u07b5\u0001\u0000\u0000\u0000"
+ "\u07bd\u07ba\u0001\u0000\u0000\u0000\u07be\u011d\u0001\u0000\u0000\u0000"
+ "\u07bf\u07c4\u0005\u010c\u0000\u0000\u07c0\u07c4\u0003\u0120\u0090\u0000"
+ "\u07c1\u07c2\u0005\u00c7\u0000\u0000\u07c2\u07c4\u0005\u0107\u0000\u0000"
+ "\u07c3\u07bf\u0001\u0000\u0000\u0000\u07c3\u07c0\u0001\u0000\u0000\u0000"
+ "\u07c3\u07c1\u0001\u0000\u0000\u0000\u07c4\u011f\u0001\u0000\u0000\u0000"
+ "\u07c5\u07cb\u0005c\u0000\u0000\u07c6\u07c7\u0005\u00c7\u0000\u0000\u07c7"
+ "\u07cb\u0005c\u0000\u0000\u07c8\u07c9\u0005\u00c7\u0000\u0000\u07c9\u07cb"
+ "\u0005b\u0000\u0000\u07ca\u07c5\u0001\u0000\u0000\u0000\u07ca\u07c6\u0001"
+ "\u0000\u0000\u0000\u07ca\u07c8\u0001\u0000\u0000\u0000\u07cb\u0121\u0001"
+ "\u0000\u0000\u0000\u07cc\u07d6\u0005\u0012\u0000\u0000\u07cd\u07d6\u0005"
+ "\u010b\u0000\u0000\u07ce\u07d6\u0005b\u0000\u0000\u07cf\u07d0\u0005\u00a4"
+ "\u0000\u0000\u07d0\u07d6\u0005\u0084\u0000\u0000\u07d1\u07d2\u0005\u00a4"
+ "\u0000\u0000\u07d2\u07d6\u0005b\u0000\u0000\u07d3\u07d4\u0005\u00d2\u0000"
+ "\u0000\u07d4\u07d6\u0005b\u0000\u0000\u07d5\u07cc\u0001\u0000\u0000\u0000"
+ "\u07d5\u07cd\u0001\u0000\u0000\u0000\u07d5\u07ce\u0001\u0000\u0000\u0000"
+ "\u07d5\u07cf\u0001\u0000\u0000\u0000\u07d5\u07d1\u0001\u0000\u0000\u0000"
+ "\u07d5\u07d3\u0001\u0000\u0000\u0000\u07d6\u0123\u0001\u0000\u0000\u0000"
+ "\u07d7\u07d9\u0003\u01e2\u00f1\u0000\u07d8\u07da\u0003\u0114\u008a\u0000"
+ "\u07d9\u07d8\u0001\u0000\u0000\u0000\u07d9\u07da\u0001\u0000\u0000\u0000"
+ "\u07da\u07dc\u0001\u0000\u0000\u0000\u07db\u07dd\u0003\u013c\u009e\u0000"
+ "\u07dc\u07db\u0001\u0000\u0000\u0000\u07dc\u07dd\u0001\u0000\u0000\u0000"
+ "\u07dd\u0125\u0001\u0000\u0000\u0000\u07de\u07e3\u0003\u01e2\u00f1\u0000"
+ "\u07df\u07e1\u0007\u0015\u0000\u0000\u07e0\u07e2\u0005\u00b6\u0000\u0000"
+ "\u07e1\u07e0\u0001\u0000\u0000\u0000\u07e1\u07e2\u0001\u0000\u0000\u0000"
+ "\u07e2\u07e4\u0001\u0000\u0000\u0000\u07e3\u07df\u0001\u0000\u0000\u0000"
+ "\u07e3\u07e4\u0001\u0000\u0000\u0000\u07e4\u07e6\u0001\u0000\u0000\u0000"
+ "\u07e5\u07e7\u0003\u013c\u009e\u0000\u07e6\u07e5\u0001\u0000\u0000\u0000"
+ "\u07e6\u07e7\u0001\u0000\u0000\u0000\u07e7\u0127\u0001\u0000\u0000\u0000"
+ "\u07e8\u07ea\u0003\u01e2\u00f1\u0000\u07e9\u07eb\u0003\u010a\u0085\u0000"
+ "\u07ea\u07e9\u0001\u0000\u0000\u0000\u07ea\u07eb\u0001\u0000\u0000\u0000"
+ "\u07eb\u07ed\u0001\u0000\u0000\u0000\u07ec\u07ee\u0003\u013c\u009e\u0000"
+ "\u07ed\u07ec\u0001\u0000\u0000\u0000\u07ed\u07ee\u0001\u0000\u0000\u0000"
+ "\u07ee\u0129\u0001\u0000\u0000\u0000\u07ef\u07f1\u0007\u001b\u0000\u0000"
+ "\u07f0\u07f2\u0003\u012e\u0097\u0000\u07f1\u07f0\u0001\u0000\u0000\u0000"
+ "\u07f1\u07f2\u0001\u0000\u0000\u0000\u07f2\u07f4\u0001\u0000\u0000\u0000"
+ "\u07f3\u07f5\u0003\u010a\u0085\u0000\u07f4\u07f3\u0001\u0000\u0000\u0000"
+ "\u07f4\u07f5\u0001\u0000\u0000\u0000\u07f5\u07f7\u0001\u0000\u0000\u0000"
+ "\u07f6\u07f8\u0003\u013c\u009e\u0000\u07f7\u07f6\u0001\u0000\u0000\u0000"
+ "\u07f7\u07f8\u0001\u0000\u0000\u0000\u07f8\u012b\u0001\u0000\u0000\u0000"
+ "\u07f9\u07fb\u0003\u0130\u0098\u0000\u07fa\u07f9\u0001\u0000\u0000\u0000"
+ "\u07fa\u07fb\u0001\u0000\u0000\u0000\u07fb\u07fc\u0001\u0000\u0000\u0000"
+ "\u07fc\u07fe\u0005o\u0000\u0000\u07fd\u07ff\u0003\u012e\u0097\u0000\u07fe"
+ "\u07fd\u0001\u0000\u0000\u0000\u07fe\u07ff\u0001\u0000\u0000\u0000\u07ff"
+ "\u0801\u0001\u0000\u0000\u0000\u0800\u0802\u0003\u010a\u0085\u0000\u0801"
+ "\u0800\u0001\u0000\u0000\u0000\u0801\u0802\u0001\u0000\u0000\u0000\u0802"
+ "\u0804\u0001\u0000\u0000\u0000\u0803\u0805\u0003\u013c\u009e\u0000\u0804"
+ "\u0803\u0001\u0000\u0000\u0000\u0804\u0805\u0001\u0000\u0000\u0000\u0805"
+ "\u012d\u0001\u0000\u0000\u0000\u0806\u080d\u0005`\u0000\u0000\u0807\u080b"
+ "\u0005%\u0000\u0000\u0808\u0809\u0005;\u0000\u0000\u0809\u080c\u0005\u0110"
+ "\u0000\u0000\u080a\u080c\u0003\u0252\u0129\u0000\u080b\u0808\u0001\u0000"
+ "\u0000\u0000\u080b\u080a\u0001\u0000\u0000\u0000\u080c\u080e\u0001\u0000"
+ "\u0000\u0000\u080d\u0807\u0001\u0000\u0000\u0000\u080d\u080e\u0001\u0000"
+ "\u0000\u0000\u080e\u012f\u0001\u0000\u0000\u0000\u080f\u0815\u0005\u0012"
+ "\u0000\u0000\u0810\u0811\u0005$\u0000\u0000\u0811\u0815\u0005{\u0000\u0000"
+ "\u0812\u0813\u0005\u0110\u0000\u0000\u0813\u0815\u0005D\u0000\u0000\u0814"
+ "\u080f\u0001\u0000\u0000\u0000\u0814\u0810\u0001\u0000\u0000\u0000\u0814"
+ "\u0812\u0001\u0000\u0000\u0000\u0815\u0131\u0001\u0000\u0000\u0000\u0816"
+ "\u0817\u0003\u01e4\u00f2\u0000\u0817\u0818\u0003\u013a\u009d\u0000\u0818"
+ "\u0133\u0001\u0000\u0000\u0000\u0819\u081a\u0005\u00f8\u0000\u0000\u081a"
+ "\u081b\u0003\u0136\u009b\u0000\u081b\u0135\u0001\u0000\u0000\u0000\u081c"
+ "\u081d\u0003\u01e4\u00f2\u0000\u081d\u081e\u0003\u013a\u009d\u0000\u081e"
+ "\u0137\u0001\u0000\u0000\u0000\u081f\u0820\u0005\u00e9\u0000\u0000\u0820"
+ "\u0821\u0003\u013a\u009d\u0000\u0821\u0139\u0001\u0000\u0000\u0000\u0822"
+ "\u0824\u0003\u010a\u0085\u0000\u0823\u0822\u0001\u0000\u0000\u0000\u0823"
+ "\u0824\u0001\u0000\u0000\u0000\u0824\u082a\u0001\u0000\u0000\u0000\u0825"
+ "\u0827\u0003\u0140\u00a0\u0000\u0826\u0828\u0003\u010a\u0085\u0000\u0827"
+ "\u0826\u0001\u0000\u0000\u0000\u0827\u0828\u0001\u0000\u0000\u0000\u0828"
+ "\u082a\u0001\u0000\u0000\u0000\u0829\u0823\u0001\u0000\u0000\u0000\u0829"
+ "\u0825\u0001\u0000\u0000\u0000\u082a\u082c\u0001\u0000\u0000\u0000\u082b"
+ "\u082d\u0003\u013c\u009e\u0000\u082c\u082b\u0001\u0000\u0000\u0000\u082c"
+ "\u082d\u0001\u0000\u0000\u0000\u082d\u013b\u0001\u0000\u0000\u0000\u082e"
+ "\u0831\u0003\u0134\u009a\u0000\u082f\u0831\u0003\u013e\u009f\u0000\u0830"
+ "\u082e\u0001\u0000\u0000\u0000\u0830\u082f\u0001\u0000\u0000\u0000\u0831"
+ "\u013d\u0001\u0000\u0000\u0000\u0832\u0839\u0005\u00ec\u0000\u0000\u0833"
+ "\u083a\u0003\u0116\u008b\u0000\u0834\u083a\u0003\u011c\u008e\u0000\u0835"
+ "\u083a\u0003\u012c\u0096\u0000\u0836\u083a\u0003\u012a\u0095\u0000\u0837"
+ "\u083a\u0003\u0138\u009c\u0000\u0838\u083a\u0003\u0132\u0099\u0000\u0839"
+ "\u0833\u0001\u0000\u0000\u0000\u0839\u0834\u0001\u0000\u0000\u0000\u0839"
+ "\u0835\u0001\u0000\u0000\u0000\u0839\u0836\u0001\u0000\u0000\u0000\u0839"
+ "\u0837\u0001\u0000\u0000\u0000\u0839\u0838\u0001\u0000\u0000\u0000\u083a"
+ "\u013f\u0001\u0000\u0000\u0000\u083b\u083e\u0003\u0248\u0124\u0000\u083c"
+ "\u083e\u0003\u009aM\u0000\u083d\u083b\u0001\u0000\u0000\u0000\u083d\u083c"
+ "\u0001\u0000\u0000\u0000\u083e\u0141\u0001\u0000\u0000\u0000\u083f\u0844"
+ "\u0003\u0144\u00a2\u0000\u0840\u0841\u0005\u001c\u0000\u0000\u0841\u0843"
+ "\u0003\u0144\u00a2\u0000\u0842\u0840\u0001\u0000\u0000\u0000\u0843\u0846"
+ "\u0001\u0000\u0000\u0000\u0844\u0842\u0001\u0000\u0000\u0000\u0844\u0845"
+ "\u0001\u0000\u0000\u0000\u0845\u0143\u0001\u0000\u0000\u0000\u0846\u0844"
+ "\u0001\u0000\u0000\u0000\u0847\u0849\u0003\u0146\u00a3\u0000\u0848\u084a"
+ "\u0003\u0148\u00a4\u0000\u0849\u0848\u0001\u0000\u0000\u0000\u0849\u084a"
+ "\u0001\u0000\u0000\u0000\u084a\u084e\u0001\u0000\u0000\u0000\u084b\u084d"
+ "\u0003\u014a\u00a5\u0000\u084c\u084b\u0001\u0000\u0000\u0000\u084d\u0850"
+ "\u0001\u0000\u0000\u0000\u084e\u084c\u0001\u0000\u0000\u0000\u084e\u084f"
+ "\u0001\u0000\u0000\u0000\u084f\u0145\u0001\u0000\u0000\u0000\u0850\u084e"
+ "\u0001\u0000\u0000\u0000\u0851\u0890\u0005\u00ab\u0000\u0000\u0852\u0890"
+ "\u0005\u00ad\u0000\u0000\u0853\u0890\u0005\u001e\u0000\u0000\u0854\u0890"
+ "\u0005\u00f4\u0000\u0000\u0855\u0890\u0005\u0080\u0000\u0000\u0856\u0858"
+ "\u0005\u00ed\u0000\u0000\u0857\u0856\u0001\u0000\u0000\u0000\u0857\u0858"
+ "\u0001\u0000\u0000\u0000\u0858\u0859\u0001\u0000\u0000\u0000\u0859\u0890"
+ "\u0005\u0081\u0000\u0000\u085a\u0890\u0005j\u0000\u0000\u085b\u0890\u0005"
+ "?\u0000\u0000\u085c\u085d\u0005\u0090\u0000\u0000\u085d\u0890\u0007\u001c"
+ "\u0000\u0000\u085e\u085f\u0005\u011f\u0000\u0000\u085f\u0890\u0007\u001c"
+ "\u0000\u0000\u0860\u0865\u0005\u00fb\u0000\u0000\u0861\u0862\u0005\u011b"
+ "\u0000\u0000\u0862\u0866\u0005\u00fe\u0000\u0000\u0863\u0864\u0005\u011a"
+ "\u0000\u0000\u0864\u0866\u0005\u00fe\u0000\u0000\u0865\u0861\u0001\u0000"
+ "\u0000\u0000\u0865\u0863\u0001\u0000\u0000\u0000\u0866\u0890\u0001\u0000"
+ "\u0000\u0000\u0867\u086c\u0005\u00fd\u0000\u0000\u0868\u0869\u0005\u011b"
+ "\u0000\u0000\u0869\u086d\u0005\u00fe\u0000\u0000\u086a\u086b\u0005\u011a"
+ "\u0000\u0000\u086b\u086d\u0005\u00fe\u0000\u0000\u086c\u0868\u0001\u0000"
+ "\u0000\u0000\u086c\u086a\u0001\u0000\u0000\u0000\u086d\u0890\u0001\u0000"
+ "\u0000\u0000\u086e\u0890\u0005U\u0000\u0000\u086f\u0890\u0005\u00be\u0000"
+ "\u0000\u0870\u0890\u0005\u00a4\u0000\u0000\u0871\u0890\u0005\u0116\u0000"
+ "\u0000\u0872\u0890\u0005\u00d2\u0000\u0000\u0873\u0890\u0005W\u0000\u0000"
+ "\u0874\u0890\u0005\u0095\u0000\u0000\u0875\u0876\u0007\u001d\u0000\u0000"
+ "\u0876\u0877\u0005\u0093\u0000\u0000\u0877\u0878\u0003\u0142\u00a1\u0000"
+ "\u0878\u0879\u0005u\u0000\u0000\u0879\u0890\u0001\u0000\u0000\u0000\u087a"
+ "\u0890\u0005\u00b9\u0000\u0000\u087b\u087c\u0005\u00c7\u0000\u0000\u087c"
+ "\u0890\u0005\u0113\u0000\u0000\u087d\u088d\u0005\u0015\u0000\u0000\u087e"
+ "\u088e\u0005\u00a4\u0000\u0000\u087f\u088e\u0005\u0116\u0000\u0000\u0880"
+ "\u088e\u0005\u00d2\u0000\u0000\u0881\u088e\u0005W\u0000\u0000\u0882\u088e"
+ "\u0005\u0095\u0000\u0000\u0883\u0884\u0005\u00c7\u0000\u0000\u0884\u088e"
+ "\u0005\u0113\u0000\u0000\u0885\u0887\u0005\u0113\u0000\u0000\u0886\u0885"
+ "\u0001\u0000\u0000\u0000\u0886\u0887\u0001\u0000\u0000\u0000\u0887\u0888"
+ "\u0001\u0000\u0000\u0000\u0888\u0889\u0005\u0093\u0000\u0000\u0889\u088a"
+ "\u0003\u0142\u00a1\u0000\u088a\u088b\u0005u\u0000";
private static final String _serializedATNSegment1 =
"\u0000\u088b\u088e\u0001\u0000\u0000\u0000\u088c\u088e\u0005\u0113\u0000"
+ "\u0000\u088d\u087e\u0001\u0000\u0000\u0000\u088d\u087f\u0001\u0000\u0000"
+ "\u0000\u088d\u0880\u0001\u0000\u0000\u0000\u088d\u0881\u0001\u0000\u0000"
+ "\u0000\u088d\u0882\u0001\u0000\u0000\u0000\u088d\u0883\u0001\u0000\u0000"
+ "\u0000\u088d\u0886\u0001\u0000\u0000\u0000\u088d\u088c\u0001\u0000\u0000"
+ "\u0000\u088d\u088e\u0001\u0000\u0000\u0000\u088e\u0890\u0001\u0000\u0000"
+ "\u0000\u088f\u0851\u0001\u0000\u0000\u0000\u088f\u0852\u0001\u0000\u0000"
+ "\u0000\u088f\u0853\u0001\u0000\u0000\u0000\u088f\u0854\u0001\u0000\u0000"
+ "\u0000\u088f\u0855\u0001\u0000\u0000\u0000\u088f\u0857\u0001\u0000\u0000"
+ "\u0000\u088f\u085a\u0001\u0000\u0000\u0000\u088f\u085b\u0001\u0000\u0000"
+ "\u0000\u088f\u085c\u0001\u0000\u0000\u0000\u088f\u085e\u0001\u0000\u0000"
+ "\u0000\u088f\u0860\u0001\u0000\u0000\u0000\u088f\u0867\u0001\u0000\u0000"
+ "\u0000\u088f\u086e\u0001\u0000\u0000\u0000\u088f\u086f\u0001\u0000\u0000"
+ "\u0000\u088f\u0870\u0001\u0000\u0000\u0000\u088f\u0871\u0001\u0000\u0000"
+ "\u0000\u088f\u0872\u0001\u0000\u0000\u0000\u088f\u0873\u0001\u0000\u0000"
+ "\u0000\u088f\u0874\u0001\u0000\u0000\u0000\u088f\u0875\u0001\u0000\u0000"
+ "\u0000\u088f\u087a\u0001\u0000\u0000\u0000\u088f\u087b\u0001\u0000\u0000"
+ "\u0000\u088f\u087d\u0001\u0000\u0000\u0000\u0890\u0147\u0001\u0000\u0000"
+ "\u0000\u0891\u0892\u0005\u00aa\u0000\u0000\u0892\u0895\u0005\u00ad\u0000"
+ "\u0000\u0893\u0895\u0005\u0088\u0000\u0000\u0894\u0891\u0001\u0000\u0000"
+ "\u0000\u0894\u0893\u0001\u0000\u0000\u0000\u0895\u0149\u0001\u0000\u0000"
+ "\u0000\u0896\u0898\u0007\u001d\u0000\u0000\u0897\u0899\u0003\u0148\u00a4"
+ "\u0000\u0898\u0897\u0001\u0000\u0000\u0000\u0898\u0899\u0001\u0000\u0000"
+ "\u0000\u0899\u014b\u0001\u0000\u0000\u0000\u089a\u089b\u0005\u0092\u0000"
+ "\u0000\u089b\u089c\u0003\u00fa}\u0000\u089c\u089d\u0003p8\u0000\u089d"
+ "\u089e\u0005\u00e0\u0000\u0000\u089e\u014d\u0001\u0000\u0000\u0000\u089f"
+ "\u08a0\u0005\u0092\u0000\u0000\u08a0\u08a2\u0005\u00e0\u0000\u0000\u08a1"
+ "\u08a3\u0003|>\u0000\u08a2\u08a1\u0001\u0000\u0000\u0000\u08a2\u08a3\u0001"
+ "\u0000\u0000\u0000\u08a3\u08a4\u0001\u0000\u0000\u0000\u08a4\u08a5\u0003"
+ "~?\u0000\u08a5\u08a6\u0005\u0089\u0000\u0000\u08a6\u08a7\u0003\u00fa}"
+ "\u0000\u08a7\u08a8\u0003r9\u0000\u08a8\u08a9\u0005\u00ca\u0000\u0000\u08a9"
+ "\u08ab\u0003~?\u0000\u08aa\u08ac\u0003\u0080@\u0000\u08ab\u08aa\u0001"
+ "\u0000\u0000\u0000\u08ab\u08ac\u0001\u0000\u0000\u0000\u08ac\u08ad\u0001"
+ "\u0000\u0000\u0000\u08ad\u08ae\u0005\u0092\u0000\u0000\u08ae\u08af\u0005"
+ "\u00e0\u0000\u0000\u08af\u014f\u0001\u0000\u0000\u0000\u08b0\u08b2\u0005"
+ "3\u0000\u0000\u08b1\u08b3\u0003\u0226\u0113\u0000\u08b2\u08b1\u0001\u0000"
+ "\u0000\u0000\u08b2\u08b3\u0001\u0000\u0000\u0000\u08b3\u08b7\u0001\u0000"
+ "\u0000\u0000\u08b4\u08b5\u0005x\u0000\u0000\u08b5\u08b6\u0005\u00aa\u0000"
+ "\u0000\u08b6\u08b8\u0005d\u0000\u0000\u08b7\u08b4\u0001\u0000\u0000\u0000"
+ "\u08b7\u08b8\u0001\u0000\u0000\u0000\u08b8\u08b9\u0001\u0000\u0000\u0000"
+ "\u08b9\u08bc\u0007\u001e\u0000\u0000\u08ba\u08bd\u0003\u014c\u00a6\u0000"
+ "\u08bb\u08bd\u0003\u014e\u00a7\u0000\u08bc\u08ba\u0001\u0000\u0000\u0000"
+ "\u08bc\u08bb\u0001\u0000\u0000\u0000\u08bd\u08be\u0001\u0000\u0000\u0000"
+ "\u08be\u08c0\u0003\u0152\u00a9\u0000\u08bf\u08c1\u0003\u0222\u0111\u0000"
+ "\u08c0\u08bf\u0001\u0000\u0000\u0000\u08c0\u08c1\u0001\u0000\u0000\u0000"
+ "\u08c1\u0151\u0001\u0000\u0000\u0000\u08c2\u08c3\u0005\u0019\u0000\u0000"
+ "\u08c3\u08c4\u0005d\u0000\u0000\u08c4\u08e5\u0003\u016a\u00b5\u0000\u08c5"
+ "\u08c6\u0007\u001f\u0000\u0000\u08c6\u08ca\u0003\u016a\u00b5\u0000\u08c7"
+ "\u08cb\u0005,\u0000\u0000\u08c8\u08c9\u0005\u0082\u0000\u0000\u08c9\u08cb"
+ "\u0007\r\u0000\u0000\u08ca\u08c7\u0001\u0000\u0000\u0000\u08ca\u08c8\u0001"
+ "\u0000\u0000\u0000\u08cb\u08cc\u0001\u0000\u0000\u0000\u08cc\u08cd\u0003"
+ "\u0142\u00a1\u0000\u08cd\u08e5\u0001\u0000\u0000\u0000\u08ce\u08cf\u0007"
+ "\u001f\u0000\u0000\u08cf\u08d0\u0003\u016a\u00b5\u0000\u08d0\u08d2\u0005"
+ "\u0082\u0000\u0000\u08d1\u08d3\u0007\u0018\u0000\u0000\u08d2\u08d1\u0001"
+ "\u0000\u0000\u0000\u08d2\u08d3\u0001\u0000\u0000\u0000\u08d3\u08d4\u0001"
+ "\u0000\u0000\u0000\u08d4\u08d5\u0005\u010b\u0000\u0000\u08d5\u08e5\u0001"
+ "\u0000\u0000\u0000\u08d6\u08d7\u0007\u001f\u0000\u0000\u08d7\u08d8\u0003"
+ "\u016a\u00b5\u0000\u08d8\u08da\u0005\u0082\u0000\u0000\u08d9\u08db\u0007"
+ "\u0018\u0000\u0000\u08da\u08d9\u0001\u0000\u0000\u0000\u08da\u08db\u0001"
+ "\u0000\u0000\u0000\u08db\u08dc\u0001\u0000\u0000\u0000\u08dc\u08dd\u0005"
+ "\u0084\u0000\u0000\u08dd\u08e5\u0001\u0000\u0000\u0000\u08de\u08df\u0007"
+ "\u001f\u0000\u0000\u08df\u08e0\u0003\u016a\u00b5\u0000\u08e0\u08e1\u0005"
+ "\u0082\u0000\u0000\u08e1\u08e2\u0005\u00aa\u0000\u0000\u08e2\u08e3\u0005"
+ "\u00ad\u0000\u0000\u08e3\u08e5\u0001\u0000\u0000\u0000\u08e4\u08c2\u0001"
+ "\u0000\u0000\u0000\u08e4\u08c5\u0001\u0000\u0000\u0000\u08e4\u08ce\u0001"
+ "\u0000\u0000\u0000\u08e4\u08d6\u0001\u0000\u0000\u0000\u08e4\u08de\u0001"
+ "\u0000\u0000\u0000\u08e5\u0153\u0001\u0000\u0000\u0000\u08e6\u08ff\u0005"
+ "3\u0000\u0000\u08e7\u08ea\u0005\u00af\u0000\u0000\u08e8\u08eb\u0003\u014c"
+ "\u00a6\u0000\u08e9\u08eb\u0003\u014e\u00a7\u0000\u08ea\u08e8\u0001\u0000"
+ "\u0000\u0000\u08ea\u08e9\u0001\u0000\u0000\u0000\u08eb\u08ec\u0001\u0000"
+ "\u0000\u0000\u08ec\u08f8\u0005\u0019\u0000\u0000\u08ed\u08ee\u0005d\u0000"
+ "\u0000\u08ee\u08f9\u0003\u016a\u00b5\u0000\u08ef\u08f0\u0003\u016a\u00b5"
+ "\u0000\u08f0\u08f6\u0005\u0082\u0000\u0000\u08f1\u08f7\u0005\u010b\u0000"
+ "\u0000\u08f2\u08f3\u0005\u00a4\u0000\u0000\u08f3\u08f7\u0005\u0084\u0000"
+ "\u0000\u08f4\u08f5\u0005\u00aa\u0000\u0000\u08f5\u08f7\u0005\u00ad\u0000"
+ "\u0000\u08f6\u08f1\u0001\u0000\u0000\u0000\u08f6\u08f2\u0001\u0000\u0000"
+ "\u0000\u08f6\u08f4\u0001\u0000\u0000\u0000\u08f7\u08f9\u0001\u0000\u0000"
+ "\u0000\u08f8\u08ed\u0001\u0000\u0000\u0000\u08f8\u08ef\u0001\u0000\u0000"
+ "\u0000\u08f9\u0900\u0001\u0000\u0000\u0000\u08fa\u08fd\u0003\u0226\u0113"
+ "\u0000\u08fb\u08fc\u0005x\u0000\u0000\u08fc\u08fe\u0005d\u0000\u0000\u08fd"
+ "\u08fb\u0001\u0000\u0000\u0000\u08fd\u08fe\u0001\u0000\u0000\u0000\u08fe"
+ "\u0900\u0001\u0000\u0000\u0000\u08ff\u08e7\u0001\u0000\u0000\u0000\u08ff"
+ "\u08fa\u0001\u0000\u0000\u0000\u0900\u0155\u0001\u0000\u0000\u0000\u0901"
+ "\u0902\u0005#\u0000\u0000\u0902\u0903\u0005|\u0000\u0000\u0903\u091d\u0003"
+ "\u015a\u00ad\u0000\u0904\u0905\u0005\u00c9\u0000\u0000\u0905\u0906\u0005"
+ "|\u0000\u0000\u0906\u091d\u0003\u015a\u00ad\u0000\u0907\u0908\u0005\u00f9"
+ "\u0000\u0000\u0908\u0909\u0005|\u0000\u0000\u0909\u091d\u0003\u015a\u00ad"
+ "\u0000\u090a\u090b\u0005\u00be\u0000\u0000\u090b\u090c\u0005|\u0000\u0000"
+ "\u090c\u091d\u0003\u015a\u00ad\u0000\u090d\u090e\u0005\u0114\u0000\u0000"
+ "\u090e\u090f\u0005|\u0000\u0000\u090f\u091d\u0003\u015a\u00ad\u0000\u0910"
+ "\u0911\u0005\u0091\u0000\u0000\u0911\u0912\u0005|\u0000\u0000\u0912\u091d"
+ "\u0003\u0162\u00b1\u0000\u0913\u0914\u0005n\u0000\u0000\u0914\u0915\u0005"
+ "|\u0000\u0000\u0915\u091d\u0003\u015c\u00ae\u0000\u0916\u091a\u0005|\u0000"
+ "\u0000\u0917\u0918\u0005\u00af\u0000\u0000\u0918\u091b\u0003\u0158\u00ac"
+ "\u0000\u0919\u091b\u0003\u015a\u00ad\u0000\u091a\u0917\u0001\u0000\u0000"
+ "\u0000\u091a\u0919\u0001\u0000\u0000\u0000\u091b\u091d\u0001\u0000\u0000"
+ "\u0000\u091c\u0901\u0001\u0000\u0000\u0000\u091c\u0904\u0001\u0000\u0000"
+ "\u0000\u091c\u0907\u0001\u0000\u0000\u0000\u091c\u090a\u0001\u0000\u0000"
+ "\u0000\u091c\u090d\u0001\u0000\u0000\u0000\u091c\u0910\u0001\u0000\u0000"
+ "\u0000\u091c\u0913\u0001\u0000\u0000\u0000\u091c\u0916\u0001\u0000\u0000"
+ "\u0000\u091d\u0157\u0001\u0000\u0000\u0000\u091e\u091f\u0003p8\u0000\u091f"
+ "\u0920\u0005\u0092\u0000\u0000\u0920\u0921\u0003\u00fc~\u0000\u0921\u0922"
+ "\u0005\u00e0\u0000\u0000\u0922\u0159\u0001\u0000\u0000\u0000\u0923\u0925"
+ "\u0003\u0226\u0113\u0000\u0924\u0923\u0001\u0000\u0000\u0000\u0924\u0925"
+ "\u0001\u0000\u0000\u0000\u0925\u0929\u0001\u0000\u0000\u0000\u0926\u0927"
+ "\u0005x\u0000\u0000\u0927\u0928\u0005\u00aa\u0000\u0000\u0928\u092a\u0005"
+ "d\u0000\u0000\u0929\u0926\u0001\u0000\u0000\u0000\u0929\u092a\u0001\u0000"
+ "\u0000\u0000\u092a\u092b\u0001\u0000\u0000\u0000\u092b\u092e\u0005k\u0000"
+ "\u0000\u092c\u092f\u0003\u014c\u00a6\u0000\u092d\u092f\u0003\u014e\u00a7"
+ "\u0000\u092e\u092c\u0001\u0000\u0000\u0000\u092e\u092d\u0001\u0000\u0000"
+ "\u0000\u092f\u0930\u0001\u0000\u0000\u0000\u0930\u0931\u0005\u00af\u0000"
+ "\u0000\u0931\u0933\u0003\u016a\u00b5\u0000\u0932\u0934\u0003\u0222\u0111"
+ "\u0000\u0933\u0932\u0001\u0000\u0000\u0000\u0933\u0934\u0001\u0000\u0000"
+ "\u0000\u0934\u015b\u0001\u0000\u0000\u0000\u0935\u0937\u0003\u0226\u0113"
+ "\u0000\u0936\u0935\u0001\u0000\u0000\u0000\u0936\u0937\u0001\u0000\u0000"
+ "\u0000\u0937\u093b\u0001\u0000\u0000\u0000\u0938\u0939\u0005x\u0000\u0000"
+ "\u0939\u093a\u0005\u00aa\u0000\u0000\u093a\u093c\u0005d\u0000\u0000\u093b"
+ "\u0938\u0001\u0000\u0000\u0000\u093b\u093c\u0001\u0000\u0000\u0000\u093c"
+ "\u093d\u0001\u0000\u0000\u0000\u093d\u0940\u0005k\u0000\u0000\u093e\u0941"
+ "\u0003\u015e\u00af\u0000\u093f\u0941\u0003\u0160\u00b0\u0000\u0940\u093e"
+ "\u0001\u0000\u0000\u0000\u0940\u093f\u0001\u0000\u0000\u0000\u0941\u0942"
+ "\u0001\u0000\u0000\u0000\u0942\u0943\u0005\u00af\u0000\u0000\u0943\u0944"
+ "\u0005V\u0000\u0000\u0944\u0945\u0005\u0089\u0000\u0000\u0945\u0946\u0003"
+ "\u00fa}\u0000\u0946\u094d\u0003\u00b6[\u0000\u0947\u0948\u0005-\u0000"
+ "\u0000\u0948\u0949\u0003\u00fa}\u0000\u0949\u094a\u0003\u00b6[\u0000\u094a"
+ "\u094c\u0001\u0000\u0000\u0000\u094b\u0947\u0001\u0000\u0000\u0000\u094c"
+ "\u094f\u0001\u0000\u0000\u0000\u094d\u094b\u0001\u0000\u0000\u0000\u094d"
+ "\u094e\u0001\u0000\u0000\u0000\u094e\u0950\u0001\u0000\u0000\u0000\u094f"
+ "\u094d\u0001\u0000\u0000\u0000\u0950\u0952\u0005\u00ca\u0000\u0000\u0951"
+ "\u0953\u0003\u0222\u0111\u0000\u0952\u0951\u0001\u0000\u0000\u0000\u0952"
+ "\u0953\u0001\u0000\u0000\u0000\u0953\u015d\u0001\u0000\u0000\u0000\u0954"
+ "\u0955\u0005\u0092\u0000\u0000\u0955\u0956\u0003\u00fa}\u0000\u0956\u0957"
+ "\u0005+\u0000\u0000\u0957\u095c\u0003\u0252\u0129\u0000\u0958\u0959\u0005"
+ "\u001c\u0000\u0000\u0959\u095b\u0003\u0252\u0129\u0000\u095a\u0958\u0001"
+ "\u0000\u0000\u0000\u095b\u095e\u0001\u0000\u0000\u0000\u095c\u095a\u0001"
+ "\u0000\u0000\u0000\u095c\u095d\u0001\u0000\u0000\u0000\u095d\u095f\u0001"
+ "\u0000\u0000\u0000\u095e\u095c\u0001\u0000\u0000\u0000\u095f\u0960\u0005"
+ "\u00e0\u0000\u0000\u0960\u015f\u0001\u0000\u0000\u0000\u0961\u0962\u0005"
+ "\u0092\u0000\u0000\u0962\u0964\u0005\u00e0\u0000\u0000\u0963\u0965\u0003"
+ "|>\u0000\u0964\u0963\u0001\u0000\u0000\u0000\u0964\u0965\u0001\u0000\u0000"
+ "\u0000\u0965\u0966\u0001\u0000\u0000\u0000\u0966\u0967\u0003~?\u0000\u0967"
+ "\u0968\u0005\u0089\u0000\u0000\u0968\u0969\u0003\u00fa}\u0000\u0969\u096a"
+ "\u0005+\u0000\u0000\u096a\u096f\u0003\u0252\u0129\u0000\u096b\u096c\u0005"
+ "\u001c\u0000\u0000\u096c\u096e\u0003\u0252\u0129\u0000\u096d\u096b\u0001"
+ "\u0000\u0000\u0000\u096e\u0971\u0001\u0000\u0000\u0000\u096f\u096d\u0001"
+ "\u0000\u0000\u0000\u096f\u0970\u0001\u0000\u0000\u0000\u0970\u0972\u0001"
+ "\u0000\u0000\u0000\u0971\u096f\u0001\u0000\u0000\u0000\u0972\u0973\u0005"
+ "\u00ca\u0000\u0000\u0973\u0975\u0003~?\u0000\u0974\u0976\u0003\u0080@"
+ "\u0000\u0975\u0974\u0001\u0000\u0000\u0000\u0975\u0976\u0001\u0000\u0000"
+ "\u0000\u0976\u0977\u0001\u0000\u0000\u0000\u0977\u0978\u0005\u0092\u0000"
+ "\u0000\u0978\u0979\u0005\u00e0\u0000\u0000\u0979\u0161\u0001\u0000\u0000"
+ "\u0000\u097a\u097c\u0003\u0226\u0113\u0000\u097b\u097a\u0001\u0000\u0000"
+ "\u0000\u097b\u097c\u0001\u0000\u0000\u0000\u097c\u0980\u0001\u0000\u0000"
+ "\u0000\u097d\u097e\u0005x\u0000\u0000\u097e\u097f\u0005\u00aa\u0000\u0000"
+ "\u097f\u0981\u0005d\u0000\u0000\u0980\u097d\u0001\u0000\u0000\u0000\u0980"
+ "\u0981\u0001\u0000\u0000\u0000\u0981\u0982\u0001\u0000\u0000\u0000\u0982"
+ "\u0985\u0005k\u0000\u0000\u0983\u0986\u0003\u0164\u00b2\u0000\u0984\u0986"
+ "\u0003\u0166\u00b3\u0000\u0985\u0983\u0001\u0000\u0000\u0000\u0985\u0984"
+ "\u0001\u0000\u0000\u0000\u0986\u0987\u0001\u0000\u0000\u0000\u0987\u0988"
+ "\u0003\u0252\u0129\u0000\u0988\u0989\u0005\u0092\u0000\u0000\u0989\u098a"
+ "\u0003\u00fa}\u0000\u098a\u098c\u0005\u00e0\u0000\u0000\u098b\u098d\u0003"
+ "\u0222\u0111\u0000\u098c\u098b\u0001\u0000\u0000\u0000\u098c\u098d\u0001"
+ "\u0000\u0000\u0000\u098d\u0163\u0001\u0000\u0000\u0000\u098e\u098f\u0005"
+ "\u0092\u0000\u0000\u098f\u0990\u0003\u00fa}\u0000\u0990\u0991\u0005\u00e0"
+ "\u0000\u0000\u0991\u0992\u0005\u00af\u0000\u0000\u0992\u0993\u0005V\u0000"
+ "\u0000\u0993\u0165\u0001\u0000\u0000\u0000\u0994\u0995\u0005\u0092\u0000"
+ "\u0000\u0995\u0997\u0005\u00e0\u0000\u0000\u0996\u0998\u0003|>\u0000\u0997"
+ "\u0996\u0001\u0000\u0000\u0000\u0997\u0998\u0001\u0000\u0000\u0000\u0998"
+ "\u0999\u0001\u0000\u0000\u0000\u0999\u099a\u0003~?\u0000\u099a\u099b\u0005"
+ "\u0089\u0000\u0000\u099b\u099c\u0003\u00fa}\u0000\u099c\u099d\u0005\u00ca"
+ "\u0000\u0000\u099d\u099f\u0003~?\u0000\u099e\u09a0\u0003\u0080@\u0000"
+ "\u099f\u099e\u0001\u0000\u0000\u0000\u099f\u09a0\u0001\u0000\u0000\u0000"
+ "\u09a0\u09a1\u0001\u0000\u0000\u0000\u09a1\u09a2\u0005\u0092\u0000\u0000"
+ "\u09a2\u09a3\u0005\u00e0\u0000\u0000\u09a3\u09a5\u0005\u00af\u0000\u0000"
+ "\u09a4\u09a6\u0005V\u0000\u0000\u09a5\u09a4\u0001\u0000\u0000\u0000\u09a5"
+ "\u09a6\u0001\u0000\u0000\u0000\u09a6\u0167\u0001\u0000\u0000\u0000\u09a7"
+ "\u09b3\u0005|\u0000\u0000\u09a8\u09a9\u0005\u00af\u0000\u0000\u09a9\u09aa"
+ "\u0003p8\u0000\u09aa\u09ab\u0005\u0092\u0000\u0000\u09ab\u09ac\u0003\u00fc"
+ "~\u0000\u09ac\u09ad\u0005\u00e0\u0000\u0000\u09ad\u09b4\u0001\u0000\u0000"
+ "\u0000\u09ae\u09b1\u0003\u0226\u0113\u0000\u09af\u09b0\u0005x\u0000\u0000"
+ "\u09b0\u09b2\u0005d\u0000\u0000\u09b1\u09af\u0001\u0000\u0000\u0000\u09b1"
+ "\u09b2\u0001\u0000\u0000\u0000\u09b2\u09b4\u0001\u0000\u0000\u0000\u09b3"
+ "\u09a8\u0001\u0000\u0000\u0000\u09b3\u09ae\u0001\u0000\u0000\u0000\u09b4"
+ "\u0169\u0001\u0000\u0000\u0000\u09b5\u09b6\u0003\u00fa}\u0000\u09b6\u09b7"
+ "\u0003\u00b6[\u0000\u09b7\u09c7\u0001\u0000\u0000\u0000\u09b8\u09b9\u0005"
+ "\u0092\u0000\u0000\u09b9\u09ba\u0003\u00fa}\u0000\u09ba\u09c1\u0003\u00b6"
+ "[\u0000\u09bb\u09bc\u0005-\u0000\u0000\u09bc\u09bd\u0003\u00fa}\u0000"
+ "\u09bd\u09be\u0003\u00b6[\u0000\u09be\u09c0\u0001\u0000\u0000\u0000\u09bf"
+ "\u09bb\u0001\u0000\u0000\u0000\u09c0\u09c3\u0001\u0000\u0000\u0000\u09c1"
+ "\u09bf\u0001\u0000\u0000\u0000\u09c1\u09c2\u0001\u0000\u0000\u0000\u09c2"
+ "\u09c4\u0001\u0000\u0000\u0000\u09c3\u09c1\u0001\u0000\u0000\u0000\u09c4"
+ "\u09c5\u0005\u00e0\u0000\u0000\u09c5\u09c7\u0001\u0000\u0000\u0000\u09c6"
+ "\u09b5\u0001\u0000\u0000\u0000\u09c6\u09b8\u0001\u0000\u0000\u0000\u09c7"
+ "\u016b\u0001\u0000\u0000\u0000\u09c8\u09c9\u0005X\u0000\u0000\u09c9\u09ca"
+ "\u0005\u00e6\u0000\u0000\u09ca\u09cc\u0003\u024c\u0126\u0000\u09cb\u09cd"
+ "\u0003\u0222\u0111\u0000\u09cc\u09cb\u0001\u0000\u0000\u0000\u09cc\u09cd"
+ "\u0001\u0000\u0000\u0000\u09cd\u016d\u0001\u0000\u0000\u0000\u09ce\u09cf"
+ "\u0005\u00e6\u0000\u0000\u09cf\u09d0\u0003\u024c\u0126\u0000\u09d0\u09d1"
+ "\u0005\u00e8\u0000\u0000\u09d1\u09d2\u0003\u0222\u0111\u0000\u09d2\u016f"
+ "\u0001\u0000\u0000\u0000\u09d3\u09d4\u0005\u00e6\u0000\u0000\u09d4\u09d5"
+ "\u0003\u024c\u0126\u0000\u09d5\u09d6\u0005\u00ff\u0000\u0000\u09d6\u09d7"
+ "\u0003\u024c\u0126\u0000\u09d7\u0171\u0001\u0000\u0000\u0000\u09d8\u09d9"
+ "\u0005\u00e6\u0000\u0000\u09d9\u09da\u0003\u024c\u0126\u0000\u09da\u0173"
+ "\u0001\u0000\u0000\u0000\u09db\u09dd\u0007 \u0000\u0000\u09dc\u09de\u0003"
+ "\u010a\u0085\u0000\u09dd\u09dc\u0001\u0000\u0000\u0000\u09dd\u09de\u0001"
+ "\u0000\u0000\u0000\u09de\u0175\u0001\u0000\u0000\u0000\u09df\u09e1\u0005"
+ "S\u0000\u0000\u09e0\u09df\u0001\u0000\u0000\u0000\u09e0\u09e1\u0001\u0000"
+ "\u0000\u0000\u09e1\u09e4\u0001\u0000\u0000\u0000\u09e2\u09e5\u0003\u0178"
+ "\u00bc\u0000\u09e3\u09e5\u0003\u017a\u00bd\u0000\u09e4\u09e2\u0001\u0000"
+ "\u0000\u0000\u09e4\u09e3\u0001\u0000\u0000\u0000\u09e5\u0177\u0001\u0000"
+ "\u0000\u0000\u09e6\u09e7\u0005B\u0000\u0000\u09e7\u09e8\u0007!\u0000\u0000"
+ "\u09e8\u09e9\u0005m\u0000\u0000\u09e9\u09ea\u0007 \u0000\u0000\u09ea\u09ef"
+ "\u0003\u024c\u0126\u0000\u09eb\u09ec\u0005-\u0000\u0000\u09ec\u09ee\u0003"
+ "\u024c\u0126\u0000\u09ed\u09eb\u0001\u0000\u0000\u0000\u09ee\u09f1\u0001"
+ "\u0000\u0000\u0000\u09ef\u09ed\u0001\u0000\u0000\u0000\u09ef\u09f0\u0001"
+ "\u0000\u0000\u0000\u09f0\u0179\u0001\u0000\u0000\u0000\u09f1\u09ef\u0001"
+ "\u0000\u0000\u0000\u09f2\u09f3\u0005\u00cd\u0000\u0000\u09f3\u09f4\u0007"
+ "!\u0000\u0000\u09f4\u017b\u0001\u0000\u0000\u0000\u09f5\u09f6\u0005\u00dc"
+ "\u0000\u0000\u09f6\u09fa\u0003\u0224\u0112\u0000\u09f7\u09f8\u0005x\u0000"
+ "\u0000\u09f8\u09f9\u0005\u00aa\u0000\u0000\u09f9\u09fb\u0005d\u0000\u0000"
+ "\u09fa\u09f7\u0001\u0000\u0000\u0000\u09fa\u09fb\u0001\u0000\u0000\u0000"
+ "\u09fb\u0a00\u0001\u0000\u0000\u0000\u09fc\u09fd\u0005\u0017\u0000\u0000"
+ "\u09fd\u09fe\u00056\u0000\u0000\u09fe\u09ff\u0005\u00ae\u0000\u0000\u09ff"
+ "\u0a01\u0003\u0224\u0112\u0000\u0a00\u09fc\u0001\u0000\u0000\u0000\u0a00"
+ "\u0a01\u0001\u0000\u0000\u0000\u0a01\u017d\u0001\u0000\u0000\u0000\u0a02"
+ "\u0a03\u0005\u00dc\u0000\u0000\u0a03\u0a06\u0003\u0224\u0112\u0000\u0a04"
+ "\u0a05\u0005x\u0000\u0000\u0a05\u0a07\u0005d\u0000\u0000\u0a06\u0a04\u0001"
+ "\u0000\u0000\u0000\u0a06\u0a07\u0001\u0000\u0000\u0000\u0a07\u017f\u0001"
+ "\u0000\u0000\u0000\u0a08\u0a09\u0005\u00dc\u0000\u0000\u0a09\u0a0c\u0003"
+ "\u0224\u0112\u0000\u0a0a\u0a0b\u0005x\u0000\u0000\u0a0b\u0a0d\u0005d\u0000"
+ "\u0000\u0a0c\u0a0a\u0001\u0000\u0000\u0000\u0a0c\u0a0d\u0001\u0000\u0000"
+ "\u0000\u0a0d\u0a0e\u0001\u0000\u0000\u0000\u0a0e\u0a0f\u0005\u00ff\u0000"
+ "\u0000\u0a0f\u0a10\u0003\u0224\u0112\u0000\u0a10\u0181\u0001\u0000\u0000"
+ "\u0000\u0a11\u0a13\u0007\"\u0000\u0000\u0a12\u0a11\u0001\u0000\u0000\u0000"
+ "\u0a12\u0a13\u0001\u0000\u0000\u0000\u0a13\u0a14\u0001\u0000\u0000\u0000"
+ "\u0a14\u0a17\u0003\u0184\u00c2\u0000\u0a15\u0a16\u0005\u011a\u0000\u0000"
+ "\u0a16\u0a18\u0007#\u0000\u0000\u0a17\u0a15\u0001\u0000\u0000\u0000\u0a17"
+ "\u0a18\u0001\u0000\u0000\u0000\u0a18\u0a1a\u0001\u0000\u0000\u0000\u0a19"
+ "\u0a1b\u0003\u010a\u0085\u0000\u0a1a\u0a19\u0001\u0000\u0000\u0000\u0a1a"
+ "\u0a1b\u0001\u0000\u0000\u0000\u0a1b\u0183\u0001\u0000\u0000\u0000\u0a1c"
+ "\u0a1d\u0007$\u0000\u0000\u0a1d\u0185\u0001\u0000\u0000\u0000\u0a1e\u0a1f"
+ "\u0005\u0110\u0000\u0000\u0a1f\u0a23\u0003\u0224\u0112\u0000\u0a20\u0a21"
+ "\u0005x\u0000\u0000\u0a21\u0a22\u0005\u00aa\u0000\u0000\u0a22\u0a24\u0005"
+ "d\u0000\u0000\u0a23\u0a20\u0001\u0000\u0000\u0000\u0a23\u0a24\u0001\u0000"
+ "\u0000\u0000\u0a24\u0a25\u0001\u0000\u0000\u0000\u0a25\u0a26\u0005\u00e8"
+ "\u0000\u0000\u0a26\u0a30\u0003\u0190\u00c8\u0000\u0a27\u0a2c\u0005\u00e8"
+ "\u0000\u0000\u0a28\u0a29\u0005\u00b7\u0000\u0000\u0a29\u0a2d\u0003\u0194"
+ "\u00ca\u0000\u0a2a\u0a2d\u0003\u0196\u00cb\u0000\u0a2b\u0a2d\u0003\u0198"
+ "\u00cc\u0000\u0a2c\u0a28\u0001\u0000\u0000\u0000\u0a2c\u0a2a\u0001\u0000"
+ "\u0000\u0000\u0a2c\u0a2b\u0001\u0000\u0000\u0000\u0a2d\u0a2f\u0001\u0000"
+ "\u0000\u0000\u0a2e\u0a27\u0001\u0000\u0000\u0000\u0a2f\u0a32\u0001\u0000"
+ "\u0000\u0000\u0a30\u0a2e\u0001\u0000\u0000\u0000\u0a30\u0a31\u0001\u0000"
+ "\u0000\u0000\u0a31\u0187\u0001\u0000\u0000\u0000\u0a32\u0a30\u0001\u0000"
+ "\u0000\u0000\u0a33\u0a34\u0005\u0110\u0000\u0000\u0a34\u0a37\u0003\u0224"
+ "\u0112\u0000\u0a35\u0a36\u0005x\u0000\u0000\u0a36\u0a38\u0005d\u0000\u0000"
+ "\u0a37\u0a35\u0001\u0000\u0000\u0000\u0a37\u0a38\u0001\u0000\u0000\u0000"
+ "\u0a38\u0189\u0001\u0000\u0000\u0000\u0a39\u0a3a\u0005\u0110\u0000\u0000"
+ "\u0a3a\u0a3d\u0003\u0224\u0112\u0000\u0a3b\u0a3c\u0005x\u0000\u0000\u0a3c"
+ "\u0a3e\u0005d\u0000\u0000\u0a3d\u0a3b\u0001\u0000\u0000\u0000\u0a3d\u0a3e"
+ "\u0001\u0000\u0000\u0000\u0a3e\u0a3f\u0001\u0000\u0000\u0000\u0a3f\u0a40"
+ "\u0005\u00ff\u0000\u0000\u0a40\u0a41\u0003\u0224\u0112\u0000\u0a41\u018b"
+ "\u0001\u0000\u0000\u0000\u0a42\u0a43\u0005;\u0000\u0000\u0a43\u0a44\u0005"
+ "\u0110\u0000\u0000\u0a44\u0a45\u0005\u00e8\u0000\u0000\u0a45\u0a46\u0005"
+ "\u00b7\u0000\u0000\u0a46\u0a47\u0005m\u0000\u0000\u0a47\u0a48\u0003\u0192"
+ "\u00c9\u0000\u0a48\u0a49\u0005\u00ff\u0000\u0000\u0a49\u0a4a\u0003\u0192"
+ "\u00c9\u0000\u0a4a\u018d\u0001\u0000\u0000\u0000\u0a4b\u0a4c\u0005\u0110"
+ "\u0000\u0000\u0a4c\u0a4f\u0003\u0224\u0112\u0000\u0a4d\u0a4e\u0005x\u0000"
+ "\u0000\u0a4e\u0a50\u0005d\u0000\u0000\u0a4f\u0a4d\u0001\u0000\u0000\u0000"
+ "\u0a4f\u0a50\u0001\u0000\u0000\u0000\u0a50\u0a60\u0001\u0000\u0000\u0000"
+ "\u0a51\u0a57\u0005\u00e8\u0000\u0000\u0a52\u0a58\u0003\u0190\u00c8\u0000"
+ "\u0a53\u0a54\u0005\u00b7\u0000\u0000\u0a54\u0a58\u0003\u0194\u00ca\u0000"
+ "\u0a55\u0a58\u0003\u0196\u00cb\u0000\u0a56\u0a58\u0003\u0198\u00cc\u0000"
+ "\u0a57\u0a52\u0001\u0000\u0000\u0000\u0a57\u0a53\u0001\u0000\u0000\u0000"
+ "\u0a57\u0a55\u0001\u0000\u0000\u0000\u0a57\u0a56\u0001\u0000\u0000\u0000"
+ "\u0a58\u0a5a\u0001\u0000\u0000\u0000\u0a59\u0a51\u0001\u0000\u0000\u0000"
+ "\u0a5a\u0a5b\u0001\u0000\u0000\u0000\u0a5b\u0a59\u0001\u0000\u0000\u0000"
+ "\u0a5b\u0a5c\u0001\u0000\u0000\u0000\u0a5c\u0a61\u0001\u0000\u0000\u0000"
+ "\u0a5d\u0a5e\u0005\u00d4\u0000\u0000\u0a5e\u0a5f\u0005w\u0000\u0000\u0a5f"
+ "\u0a61\u0005=\u0000\u0000\u0a60\u0a59\u0001\u0000\u0000\u0000\u0a60\u0a5d"
+ "\u0001\u0000\u0000\u0000\u0a61\u018f\u0001\u0000\u0000\u0000\u0a62\u0a64"
+ "\u0007%\u0000\u0000\u0a63\u0a62\u0001\u0000\u0000\u0000\u0a63\u0a64\u0001"
+ "\u0000\u0000\u0000\u0a64\u0a65\u0001\u0000\u0000\u0000\u0a65\u0a66\u0005"
+ "\u00b7\u0000\u0000\u0a66\u0a68\u0003\u0192\u00c9\u0000\u0a67\u0a69\u0003"
+ "\u0194\u00ca\u0000\u0a68\u0a67\u0001\u0000\u0000\u0000\u0a68\u0a69\u0001"
+ "\u0000\u0000\u0000\u0a69\u0191\u0001\u0000\u0000\u0000\u0a6a\u0a6d\u0003"
+ "\u024a\u0125\u0000\u0a6b\u0a6d\u0003\u00eew\u0000\u0a6c\u0a6a\u0001\u0000"
+ "\u0000\u0000\u0a6c\u0a6b\u0001\u0000\u0000\u0000\u0a6d\u0193\u0001\u0000"
+ "\u0000\u0000\u0a6e\u0a70\u0005(\u0000\u0000\u0a6f\u0a71\u0005\u00aa\u0000"
+ "\u0000\u0a70\u0a6f\u0001\u0000\u0000\u0000\u0a70\u0a71\u0001\u0000\u0000"
+ "\u0000\u0a71\u0a72\u0001\u0000\u0000\u0000\u0a72\u0a73\u0005\u00d9\u0000"
+ "\u0000\u0a73\u0195\u0001\u0000\u0000\u0000\u0a74\u0a75\u0005\u00f2\u0000"
+ "\u0000\u0a75\u0a76\u0007&\u0000\u0000\u0a76\u0197\u0001\u0000\u0000\u0000"
+ "\u0a77\u0a78\u0005w\u0000\u0000\u0a78\u0a79\u0005=\u0000\u0000\u0a79\u0a7a"
+ "\u0003\u023c\u011e\u0000\u0a7a\u0199\u0001\u0000\u0000\u0000\u0a7b\u0a7d"
+ "\u0007#\u0000\u0000\u0a7c\u0a7e\u0003\u010a\u0085\u0000\u0a7d\u0a7c\u0001"
+ "\u0000\u0000\u0000\u0a7d\u0a7e\u0001\u0000\u0000\u0000\u0a7e\u019b\u0001"
+ "\u0000\u0000\u0000\u0a7f\u0a80\u0005;\u0000\u0000\u0a80\u0a82\u0005\u0110"
+ "\u0000\u0000\u0a81\u0a83\u0003\u010a\u0085\u0000\u0a82\u0a81\u0001\u0000"
+ "\u0000\u0000\u0a82\u0a83\u0001\u0000\u0000\u0000\u0a83\u019d\u0001\u0000"
+ "\u0000\u0000\u0a84\u0a86\u0005\u0012\u0000\u0000\u0a85\u0a84\u0001\u0000"
+ "\u0000\u0000\u0a85\u0a86\u0001\u0000\u0000\u0000\u0a86\u0a87\u0001\u0000"
+ "\u0000\u0000\u0a87\u0a89\u0003\u01a8\u00d4\u0000\u0a88\u0a8a\u0003\u01a6"
+ "\u00d3\u0000\u0a89\u0a88\u0001\u0000\u0000\u0000\u0a89\u0a8a\u0001\u0000"
+ "\u0000\u0000\u0a8a\u0a8c\u0001\u0000\u0000\u0000\u0a8b\u0a8d\u0003\u010a"
+ "\u0085\u0000\u0a8c\u0a8b\u0001\u0000\u0000\u0000\u0a8c\u0a8d\u0001\u0000"
+ "\u0000\u0000\u0a8d\u019f\u0001\u0000\u0000\u0000\u0a8e\u0a8f\u0005\u00f5"
+ "\u0000\u0000\u0a8f\u0a91\u0003\u01a8\u00d4\u0000\u0a90\u0a92\u0003\u010a"
+ "\u0085\u0000\u0a91\u0a90\u0001\u0000\u0000\u0000\u0a91\u0a92\u0001\u0000"
+ "\u0000\u0000\u0a92\u01a1\u0001\u0000\u0000\u0000\u0a93\u0a94\u0007$\u0000"
+ "\u0000\u0a94\u0a95\u0003\u0240\u0120\u0000\u0a95\u0a97\u0003\u01a8\u00d4"
+ "\u0000\u0a96\u0a98\u0003\u01a6\u00d3\u0000\u0a97\u0a96\u0001\u0000\u0000"
+ "\u0000\u0a97\u0a98\u0001\u0000\u0000\u0000\u0a98\u0a9a\u0001\u0000\u0000"
+ "\u0000\u0a99\u0a9b\u0003\u010a\u0085\u0000\u0a9a\u0a99\u0001\u0000\u0000"
+ "\u0000\u0a9a\u0a9b\u0001\u0000\u0000\u0000\u0a9b\u01a3\u0001\u0000\u0000"
+ "\u0000\u0a9c\u0a9e\u0007#\u0000\u0000\u0a9d\u0a9f\u0003\u0240\u0120\u0000"
+ "\u0a9e\u0a9d\u0001\u0000\u0000\u0000\u0a9e\u0a9f\u0001\u0000\u0000\u0000"
+ "\u0a9f\u0aa0\u0001\u0000\u0000\u0000\u0aa0\u0aa2\u0003\u01a8\u00d4\u0000"
+ "\u0aa1\u0aa3\u0003\u01a6\u00d3\u0000\u0aa2\u0aa1\u0001\u0000\u0000\u0000"
+ "\u0aa2\u0aa3\u0001\u0000\u0000\u0000\u0aa3\u0aa5\u0001\u0000\u0000\u0000"
+ "\u0aa4\u0aa6\u0003\u010a\u0085\u0000\u0aa5\u0aa4\u0001\u0000\u0000\u0000"
+ "\u0aa5\u0aa6\u0001\u0000\u0000\u0000\u0aa6\u01a5\u0001\u0000\u0000\u0000"
+ "\u0aa7\u0aa9\u0005\u0017\u0000\u0000\u0aa8\u0aaa\u0005\u00db\u0000\u0000"
+ "\u0aa9\u0aa8\u0001\u0000\u0000\u0000\u0aa9\u0aaa\u0001\u0000\u0000\u0000"
+ "\u0aaa\u0aab\u0001\u0000\u0000\u0000\u0aab\u0aac\u0007\'\u0000\u0000\u0aac"
+ "\u01a7\u0001\u0000\u0000\u0000\u0aad\u0aae\u0007(\u0000\u0000\u0aae\u01a9"
+ "\u0001\u0000\u0000\u0000\u0aaf\u0aba\u0005q\u0000\u0000\u0ab0\u0ab2\u0005"
+ "z\u0000\u0000\u0ab1\u0ab0\u0001\u0000\u0000\u0000\u0ab1\u0ab2\u0001\u0000"
+ "\u0000\u0000\u0ab2\u0ab3\u0001\u0000\u0000\u0000\u0ab3\u0ab4\u0003\u01b4"
+ "\u00da\u0000\u0ab4\u0ab5\u0005\u00ff\u0000\u0000\u0ab5\u0ab6\u0003\u0240"
+ "\u0120\u0000\u0ab6\u0abb\u0001\u0000\u0000\u0000\u0ab7\u0ab8\u0003\u0184"
+ "\u00c2\u0000\u0ab8\u0ab9\u0003\u01ac\u00d6\u0000\u0ab9\u0abb\u0001\u0000"
+ "\u0000\u0000\u0aba\u0ab1\u0001\u0000\u0000\u0000\u0aba\u0ab7\u0001\u0000"
+ "\u0000\u0000\u0abb\u01ab\u0001\u0000\u0000\u0000\u0abc\u0abd\u0003\u0240"
+ "\u0120\u0000\u0abd\u0abe\u0005\u00ff\u0000\u0000\u0abe\u0abf\u0003\u0240"
+ "\u0120\u0000\u0abf\u01ad\u0001\u0000\u0000\u0000\u0ac0\u0ac2\u0005F\u0000"
+ "\u0000\u0ac1\u0ac3\u0005z\u0000\u0000\u0ac2\u0ac1\u0001\u0000\u0000\u0000"
+ "\u0ac2\u0ac3\u0001\u0000\u0000\u0000\u0ac3\u0ac4\u0001\u0000\u0000\u0000"
+ "\u0ac4\u0ac5\u0003\u01b4\u00da\u0000\u0ac5\u0ac6\u0005\u00ff\u0000\u0000"
+ "\u0ac6\u0ac7\u0003\u0240\u0120\u0000\u0ac7\u01af\u0001\u0000\u0000\u0000"
+ "\u0ac8\u0ad6\u0005\u00db\u0000\u0000\u0ac9\u0acb\u0007)\u0000\u0000\u0aca"
+ "\u0ac9\u0001\u0000\u0000\u0000\u0aca\u0acb\u0001\u0000\u0000\u0000\u0acb"
+ "\u0acd\u0001\u0000\u0000\u0000\u0acc\u0ace\u0005z\u0000\u0000\u0acd\u0acc"
+ "\u0001\u0000\u0000\u0000\u0acd\u0ace\u0001\u0000\u0000\u0000\u0ace\u0acf"
+ "\u0001\u0000\u0000\u0000\u0acf\u0ad0\u0003\u01b4\u00da\u0000\u0ad0\u0ad1"
+ "\u0005m\u0000\u0000\u0ad1\u0ad2\u0003\u0240\u0120\u0000\u0ad2\u0ad7\u0001"
+ "\u0000\u0000\u0000\u0ad3\u0ad4\u0003\u0184\u00c2\u0000\u0ad4\u0ad5\u0003"
+ "\u01b2\u00d9\u0000\u0ad5\u0ad7\u0001\u0000\u0000\u0000\u0ad6\u0aca\u0001"
+ "\u0000\u0000\u0000\u0ad6\u0ad3\u0001\u0000\u0000\u0000\u0ad7\u01b1\u0001"
+ "\u0000\u0000\u0000\u0ad8\u0ad9\u0003\u0240\u0120\u0000\u0ad9\u0ada\u0005"
+ "m\u0000\u0000\u0ada\u0adb\u0003\u0240\u0120\u0000\u0adb\u01b3\u0001\u0000"
+ "\u0000\u0000\u0adc\u0ae9\u0003\u01b6\u00db\u0000\u0add\u0ae9\u0003\u01bc"
+ "\u00de\u0000\u0ade\u0ae9\u0003\u01d6\u00eb\u0000\u0adf\u0ae9\u0003\u01d8"
+ "\u00ec\u0000\u0ae0\u0ae9\u0003\u01c8\u00e4\u0000\u0ae1\u0ae9\u0003\u01ca"
+ "\u00e5\u0000\u0ae2\u0ae9\u0003\u01f2\u00f9\u0000\u0ae3\u0ae9\u0003\u01f0"
+ "\u00f8\u0000\u0ae4\u0ae9\u0003\u01d2\u00e9\u0000\u0ae5\u0ae9\u0003\u01ce"
+ "\u00e7\u0000\u0ae6\u0ae9\u0003\u01cc\u00e6\u0000\u0ae7\u0ae9\u0003\u01d4"
+ "\u00ea\u0000\u0ae8\u0adc\u0001\u0000\u0000\u0000\u0ae8\u0add\u0001\u0000"
+ "\u0000\u0000\u0ae8\u0ade\u0001\u0000\u0000\u0000\u0ae8\u0adf\u0001\u0000"
+ "\u0000\u0000\u0ae8\u0ae0\u0001\u0000\u0000\u0000\u0ae8\u0ae1\u0001\u0000"
+ "\u0000\u0000\u0ae8\u0ae2\u0001\u0000\u0000\u0000\u0ae8\u0ae3\u0001\u0000"
+ "\u0000\u0000\u0ae8\u0ae4\u0001\u0000\u0000\u0000\u0ae8\u0ae5\u0001\u0000"
+ "\u0000\u0000\u0ae8\u0ae6\u0001\u0000\u0000\u0000\u0ae8\u0ae7\u0001\u0000"
+ "\u0000\u0000\u0ae9\u01b5\u0001\u0000\u0000\u0000\u0aea\u0aec\u0005\u0012"
+ "\u0000\u0000\u0aeb\u0aed\u0003\u01b8\u00dc\u0000\u0aec\u0aeb\u0001\u0000"
+ "\u0000\u0000\u0aec\u0aed\u0001\u0000\u0000\u0000\u0aed\u0aee\u0001\u0000"
+ "\u0000\u0000\u0aee\u0aef\u0005\u00af\u0000\u0000\u0aef\u0af0\u0003\u01ba"
+ "\u00dd\u0000\u0af0\u01b7\u0001\u0000\u0000\u0000\u0af1\u0af3\u0007*\u0000"
+ "\u0000\u0af2\u0af1\u0001\u0000\u0000\u0000\u0af2\u0af3\u0001\u0000\u0000"
+ "\u0000\u0af3\u0af4\u0001\u0000\u0000\u0000\u0af4\u0af5\u0005\u00c3\u0000"
+ "\u0000\u0af5\u01b9\u0001\u0000\u0000\u0000\u0af6\u0af7\u0007+\u0000\u0000"
+ "\u0af7\u0b04\u0007,\u0000\u0000\u0af8\u0afb\u0007!\u0000\u0000\u0af9\u0afc"
+ "\u0005\u00fc\u0000\u0000\u0afa\u0afc\u0003\u023a\u011d\u0000\u0afb\u0af9"
+ "\u0001\u0000\u0000\u0000\u0afb\u0afa\u0001\u0000\u0000\u0000\u0afc\u0b04"
+ "\u0001\u0000\u0000\u0000\u0afd\u0b00\u0007-\u0000\u0000\u0afe\u0b01\u0005"
+ "\u00fc\u0000\u0000\u0aff\u0b01\u0003\u023a\u011d\u0000\u0b00\u0afe\u0001"
+ "\u0000\u0000\u0000\u0b00\u0aff\u0001\u0000\u0000\u0000\u0b01\u0b04\u0001"
+ "\u0000\u0000\u0000\u0b02\u0b04\u0005A\u0000\u0000\u0b03\u0af6\u0001\u0000"
+ "\u0000\u0000\u0b03\u0af8\u0001\u0000\u0000\u0000\u0b03\u0afd\u0001\u0000"
+ "\u0000\u0000\u0b03\u0b02\u0001\u0000\u0000\u0000\u0b04\u01bb\u0001\u0000"
+ "\u0000\u0000\u0b05\u0b12\u00059\u0000\u0000\u0b06\u0b07\u0003\u01be\u00df"
+ "\u0000\u0b07\u0b08\u0005\u00af\u0000\u0000\u0b08\u0b09\u0003\u021e\u010f"
+ "\u0000\u0b09\u0b13\u0001\u0000\u0000\u0000\u0b0a\u0b0b\u0003\u01c6\u00e3"
+ "\u0000\u0b0b\u0b0c\u0005\u00af\u0000\u0000\u0b0c\u0b0d\u0005A\u0000\u0000"
+ "\u0b0d\u0b13\u0001\u0000\u0000\u0000\u0b0e\u0b0f\u0005\u00af\u0000\u0000"
+ "\u0b0f\u0b10\u0003\u0220\u0110\u0000\u0b10\u0b11\u0003\u01fa\u00fd\u0000"
+ "\u0b11\u0b13\u0001\u0000\u0000\u0000\u0b12\u0b06\u0001\u0000\u0000\u0000"
+ "\u0b12\u0b0a\u0001\u0000\u0000\u0000\u0b12\u0b0e\u0001\u0000\u0000\u0000"
+ "\u0b13\u01bd\u0001\u0000\u0000\u0000\u0b14\u0b1a\u0003\u01e0\u00f0\u0000"
+ "\u0b15\u0b1a\u0003\u01e2\u00f1\u0000\u0b16\u0b1a\u0003\u01c0\u00e0\u0000"
+ "\u0b17\u0b1a\u0003\u01c2\u00e1\u0000\u0b18\u0b1a\u0003\u01c4\u00e2\u0000"
+ "\u0b19\u0b14\u0001\u0000\u0000\u0000\u0b19\u0b15\u0001\u0000\u0000\u0000"
+ "\u0b19\u0b16\u0001\u0000\u0000\u0000\u0b19\u0b17\u0001\u0000\u0000\u0000"
+ "\u0b19\u0b18\u0001\u0000\u0000\u0000\u0b1a\u01bf\u0001\u0000\u0000\u0000"
+ "\u0b1b\u0b1d\u0005\u00a3\u0000\u0000\u0b1c\u0b1e\u0005\u00a4\u0000\u0000"
+ "\u0b1d\u0b1c\u0001\u0000\u0000\u0000\u0b1d\u0b1e\u0001\u0000\u0000\u0000"
+ "\u0b1e\u0b1f\u0001\u0000\u0000\u0000\u0b1f\u0b20\u0007.\u0000\u0000\u0b20"
+ "\u01c1\u0001\u0000\u0000\u0000\u0b21\u0b23\u0005\u00a3\u0000\u0000\u0b22"
+ "\u0b24\u0005\u00d2\u0000\u0000\u0b23\u0b22\u0001\u0000\u0000\u0000\u0b23"
+ "\u0b24\u0001\u0000\u0000\u0000\u0b24\u0b25\u0001\u0000\u0000\u0000\u0b25"
+ "\u0b26\u0007/\u0000\u0000\u0b26\u01c3\u0001\u0000\u0000\u0000\u0b27\u0b29"
+ "\u0005\u00a3\u0000\u0000\u0b28\u0b2a\u0005\u00c7\u0000\u0000\u0b29\u0b28"
+ "\u0001\u0000\u0000\u0000\u0b29\u0b2a\u0001\u0000\u0000\u0000\u0b2a\u0b2b"
+ "\u0001\u0000\u0000\u0000\u0b2b\u0b2c\u00070\u0000\u0000\u0b2c\u01c5\u0001"
+ "\u0000\u0000\u0000\u0b2d\u0b35\u0005\u000f\u0000\u0000\u0b2e\u0b30\u0005"
+ "1\u0000\u0000\u0b2f\u0b2e\u0001\u0000\u0000\u0000\u0b2f\u0b30\u0001\u0000"
+ "\u0000\u0000\u0b30\u0b31\u0001\u0000\u0000\u0000\u0b31\u0b35\u0005=\u0000"
+ "\u0000\u0b32\u0b35\u0005\u00dc\u0000\u0000\u0b33\u0b35\u0005\u0110\u0000"
+ "\u0000\u0b34\u0b2d\u0001\u0000\u0000\u0000\u0b34\u0b2f\u0001\u0000\u0000"
+ "\u0000\u0b34\u0b32\u0001\u0000\u0000\u0000\u0b34\u0b33\u0001\u0000\u0000"
+ "\u0000\u0b35\u01c7\u0001\u0000\u0000\u0000\u0b36\u0b42\u0005R\u0000\u0000"
+ "\u0b37\u0b3a\u0003\u01e0\u00f0\u0000\u0b38\u0b3a\u0003\u01e2\u00f1\u0000"
+ "\u0b39\u0b37\u0001\u0000\u0000\u0000\u0b39\u0b38\u0001\u0000\u0000\u0000"
+ "\u0b3a\u0b3b\u0001\u0000\u0000\u0000\u0b3b\u0b3c\u0005\u00af\u0000\u0000"
+ "\u0b3c\u0b3d\u0003\u021e\u010f\u0000\u0b3d\u0b43\u0001\u0000\u0000\u0000"
+ "\u0b3e\u0b3f\u0003\u01c6\u00e3\u0000\u0b3f\u0b40\u0005\u00af\u0000\u0000"
+ "\u0b40\u0b41\u0005A\u0000\u0000\u0b41\u0b43\u0001\u0000\u0000\u0000\u0b42"
+ "\u0b39\u0001\u0000\u0000\u0000\u0b42\u0b3e\u0001\u0000\u0000\u0000\u0b43"
+ "\u01c9\u0001\u0000\u0000\u0000\u0b44\u0b45\u0005\u008f\u0000\u0000\u0b45"
+ "\u0b4a\u0005\u00af\u0000\u0000\u0b46\u0b47\u00071\u0000\u0000\u0b47\u0b4b"
+ "\u0003\u024c\u0126\u0000\u0b48\u0b49\u0005\u0012\u0000\u0000\u0b49\u0b4b"
+ "\u0005<\u0000\u0000\u0b4a\u0b46\u0001\u0000\u0000\u0000\u0b4a\u0b48\u0001"
+ "\u0000\u0000\u0000\u0b4b\u01cb\u0001\u0000\u0000\u0000\u0b4c\u0b64\u0005"
+ "\u00ec\u0000\u0000\u0b4d\u0b54\u0003\u01e0\u00f0\u0000\u0b4e\u0b54\u0003"
+ "\u01e2\u00f1\u0000\u0b4f\u0b51\u0003\u01e4\u00f2\u0000\u0b50\u0b52\u0003"
+ "\u01e6\u00f3\u0000\u0b51\u0b50\u0001\u0000\u0000\u0000\u0b51\u0b52\u0001"
+ "\u0000\u0000\u0000\u0b52\u0b54\u0001\u0000\u0000\u0000\u0b53\u0b4d\u0001"
+ "\u0000\u0000\u0000\u0b53\u0b4e\u0001\u0000\u0000\u0000\u0b53\u0b4f\u0001"
+ "\u0000\u0000\u0000\u0b54\u0b55\u0001\u0000\u0000\u0000\u0b55\u0b56\u0005"
+ "\u00af\u0000\u0000\u0b56\u0b57\u0003\u021e\u010f\u0000\u0b57\u0b65\u0001"
+ "\u0000\u0000\u0000\u0b58\u0b61\u0005\u000f\u0000\u0000\u0b59\u0b61\u0005"
+ "\u00c2\u0000\u0000\u0b5a\u0b61\u0005\u00dc\u0000\u0000\u0b5b\u0b61\u0005"
+ "\u00e6\u0000\u0000\u0b5c\u0b61\u0005\u00e7\u0000\u0000\u0b5d\u0b5e\u0005"
+ "\u00e9\u0000\u0000\u0b5e\u0b61\u0003\u01ec\u00f6\u0000\u0b5f\u0b61\u0005"
+ "\u0110\u0000\u0000\u0b60\u0b58\u0001\u0000\u0000\u0000\u0b60\u0b59\u0001"
+ "\u0000\u0000\u0000\u0b60\u0b5a\u0001\u0000\u0000\u0000\u0b60\u0b5b\u0001"
+ "\u0000\u0000\u0000\u0b60\u0b5c\u0001\u0000\u0000\u0000\u0b60\u0b5d\u0001"
+ "\u0000\u0000\u0000\u0b60\u0b5f\u0001\u0000\u0000\u0000\u0b61\u0b62\u0001"
+ "\u0000\u0000\u0000\u0b62\u0b63\u0005\u00af\u0000\u0000\u0b63\u0b65\u0005"
+ "A\u0000\u0000\u0b64\u0b53\u0001\u0000\u0000\u0000\u0b64\u0b60\u0001\u0000"
+ "\u0000\u0000\u0b65\u01cd\u0001\u0000\u0000\u0000\u0b66\u0b7f\u0005\u00e8"
+ "\u0000\u0000\u0b67\u0b71\u0003\u01d0\u00e8\u0000\u0b68\u0b6c\u0005\u0110"
+ "\u0000\u0000\u0b69\u0b6d\u0005\u00f2\u0000\u0000\u0b6a\u0b6b\u0005w\u0000"
+ "\u0000\u0b6b\u0b6d\u0005=\u0000\u0000\u0b6c\u0b69\u0001\u0000\u0000\u0000"
+ "\u0b6c\u0b6a\u0001\u0000\u0000\u0000\u0b6d\u0b71\u0001\u0000\u0000\u0000"
+ "\u0b6e\u0b6f\u0005=\u0000\u0000\u0b6f\u0b71\u0005\u000b\u0000\u0000\u0b70"
+ "\u0b67\u0001\u0000\u0000\u0000\u0b70\u0b68\u0001\u0000\u0000\u0000\u0b70"
+ "\u0b6e\u0001\u0000\u0000\u0000\u0b71\u0b72\u0001\u0000\u0000\u0000\u0b72"
+ "\u0b73\u0005\u00af\u0000\u0000\u0b73\u0b80\u0005A\u0000\u0000\u0b74\u0b75"
+ "\u0005\u0085\u0000\u0000\u0b75\u0b76\u0003\u01f4\u00fa\u0000\u0b76\u0b77"
+ "\u0005\u00af\u0000\u0000\u0b77\u0b78\u0003\u0220\u0110\u0000\u0b78\u0b80"
+ "\u0001\u0000\u0000\u0000\u0b79\u0b7a\u0005\u00c7\u0000\u0000\u0b7a\u0b7b"
+ "\u0003\u01f6\u00fb\u0000\u0b7b\u0b7c\u0005\u00af\u0000\u0000\u0b7c\u0b7d"
+ "\u0003\u0220\u0110\u0000\u0b7d\u0b7e\u0003\u01fa\u00fd\u0000\u0b7e\u0b80"
+ "\u0001\u0000\u0000\u0000\u0b7f\u0b70\u0001\u0000\u0000\u0000\u0b7f\u0b74"
+ "\u0001\u0000\u0000\u0000\u0b7f\u0b79\u0001\u0000\u0000\u0000\u0b80\u01cf"
+ "\u0001\u0000\u0000\u0000\u0b81\u0b82\u00072\u0000\u0000\u0b82\u01d1\u0001"
+ "\u0000\u0000\u0000\u0b83\u0b8c\u0005\u00d4\u0000\u0000\u0b84\u0b85\u0007"
+ "3\u0000\u0000\u0b85\u0b86\u0005\u00af\u0000\u0000\u0b86\u0b8d\u0005A\u0000"
+ "\u0000\u0b87\u0b88\u0005\u0085\u0000\u0000\u0b88\u0b89\u0003\u01f4\u00fa"
+ "\u0000\u0b89\u0b8a\u0005\u00af\u0000\u0000\u0b8a\u0b8b\u0003\u0220\u0110"
+ "\u0000\u0b8b\u0b8d\u0001\u0000\u0000\u0000\u0b8c\u0b84\u0001\u0000\u0000"
+ "\u0000\u0b8c\u0b87\u0001\u0000\u0000\u0000\u0b8d\u01d3\u0001\u0000\u0000"
+ "\u0000\u0b8e\u0b8f\u0005\u011c\u0000\u0000\u0b8f\u0b90\u0005\u00af\u0000"
+ "\u0000\u0b90\u0b91\u0003\u0220\u0110\u0000\u0b91\u01d5\u0001\u0000\u0000"
+ "\u0000\u0b92\u0ba9\u0005\u000b\u0000\u0000\u0b93\u0ba9\u0005\u00f0\u0000"
+ "\u0000\u0b94\u0ba9\u0005\u00f3\u0000\u0000\u0b95\u0b99\u0003\u01e0\u00f0"
+ "\u0000\u0b96\u0b99\u0003\u01e2\u00f1\u0000\u0b97\u0b99\u0005\u009c\u0000"
+ "\u0000\u0b98\u0b95\u0001\u0000\u0000\u0000\u0b98\u0b96\u0001\u0000\u0000"
+ "\u0000\u0b98\u0b97\u0001\u0000\u0000\u0000\u0b99\u0b9b\u0001\u0000\u0000"
+ "\u0000\u0b9a\u0b9c\u0005\u0094\u0000\u0000\u0b9b\u0b9a\u0001\u0000\u0000"
+ "\u0000\u0b9b\u0b9c\u0001\u0000\u0000\u0000\u0b9c\u0ba9\u0001\u0000\u0000"
+ "\u0000\u0b9d\u0b9f\u0005\u0102\u0000\u0000\u0b9e\u0ba0\u0005\u0094\u0000"
+ "\u0000\u0b9f\u0b9e\u0001\u0000\u0000\u0000\u0b9f\u0ba0\u0001\u0000\u0000"
+ "\u0000\u0ba0\u0ba4\u0001\u0000\u0000\u0000\u0ba1\u0ba2\u0005\u00f8\u0000"
+ "\u0000\u0ba2\u0ba4\u0003\u01e4\u00f2\u0000\u0ba3\u0b9d\u0001\u0000\u0000"
+ "\u0000\u0ba3\u0ba1\u0001\u0000\u0000\u0000\u0ba4\u0ba6\u0001\u0000\u0000"
+ "\u0000\u0ba5\u0ba7\u0003\u01e6\u00f3\u0000\u0ba6\u0ba5\u0001\u0000\u0000"
+ "\u0000\u0ba6\u0ba7\u0001\u0000\u0000\u0000\u0ba7\u0ba9\u0001\u0000\u0000"
+ "\u0000\u0ba8\u0b92\u0001\u0000\u0000\u0000\u0ba8\u0b93\u0001\u0000\u0000"
+ "\u0000\u0ba8\u0b94\u0001\u0000\u0000\u0000\u0ba8\u0b98\u0001\u0000\u0000"
+ "\u0000\u0ba8\u0ba3\u0001\u0000\u0000\u0000\u0ba9\u0baa\u0001\u0000\u0000"
+ "\u0000\u0baa\u0bab\u0005\u00af\u0000\u0000\u0bab\u0bac\u0003\u021e\u010f"
+ "\u0000\u0bac\u01d7\u0001\u0000\u0000\u0000\u0bad\u0bae\u0005\u0013\u0000"
+ "\u0000\u0bae\u0bc5\u00074\u0000\u0000\u0baf\u0bb0\u0005\u001a\u0000\u0000"
+ "\u0bb0\u0bc5\u00073\u0000\u0000\u0bb1\u0bbb\u0005\u000f\u0000\u0000\u0bb2"
+ "\u0bb4\u00051\u0000\u0000\u0bb3\u0bb2\u0001\u0000\u0000\u0000\u0bb3\u0bb4"
+ "\u0001\u0000\u0000\u0000\u0bb4\u0bb5\u0001\u0000\u0000\u0000\u0bb5\u0bbb"
+ "\u0005=\u0000\u0000\u0bb6\u0bbb\u0005\u00c2\u0000\u0000\u0bb7\u0bbb\u0005"
+ "\u00dc\u0000\u0000\u0bb8\u0bbb\u0005\u00e6\u0000\u0000\u0bb9\u0bbb\u0005"
+ "\u0110\u0000\u0000\u0bba\u0bb1\u0001\u0000\u0000\u0000\u0bba\u0bb3\u0001"
+ "\u0000\u0000\u0000\u0bba\u0bb6\u0001\u0000\u0000\u0000\u0bba\u0bb7\u0001"
+ "\u0000\u0000\u0000\u0bba\u0bb8\u0001\u0000\u0000\u0000\u0bba\u0bb9\u0001"
+ "\u0000\u0000\u0000\u0bbb\u0bbc\u0001\u0000\u0000\u0000\u0bbc\u0bc5\u0005"
+ "\u0094\u0000\u0000\u0bbd\u0bc5\u0003\u01da\u00ed\u0000\u0bbe\u0bbf\u0005"
+ "\u00cf\u0000\u0000\u0bbf\u0bc5\u00075\u0000\u0000\u0bc0\u0bc2\u0005y\u0000"
+ "\u0000\u0bc1\u0bc3\u0003\u01e6\u00f3\u0000\u0bc2\u0bc1\u0001\u0000\u0000"
+ "\u0000\u0bc2\u0bc3\u0001\u0000\u0000\u0000\u0bc3\u0bc5\u0001\u0000\u0000"
+ "\u0000\u0bc4\u0bad\u0001\u0000\u0000\u0000\u0bc4\u0baf\u0001\u0000\u0000"
+ "\u0000\u0bc4\u0bba\u0001\u0000\u0000\u0000\u0bc4\u0bbd\u0001\u0000\u0000"
+ "\u0000\u0bc4\u0bbe\u0001\u0000\u0000\u0000\u0bc4\u0bc0\u0001\u0000\u0000"
+ "\u0000\u0bc5\u0bc6\u0001\u0000\u0000\u0000\u0bc6\u0bc7\u0005\u00af\u0000"
+ "\u0000\u0bc7\u0bc8\u0005A\u0000\u0000\u0bc8\u01d9\u0001\u0000\u0000\u0000"
+ "\u0bc9\u0bdd\u0005a\u0000\u0000\u0bca\u0bcb\u0003\u01dc\u00ee\u0000\u0bcb"
+ "\u0bcc\u0005\u00c5\u0000\u0000\u0bcc\u0bde\u0001\u0000\u0000\u0000\u0bcd"
+ "\u0bcf\u0005\u001f\u0000\u0000\u0bce\u0bcd\u0001\u0000\u0000\u0000\u0bce"
+ "\u0bcf\u0001\u0000\u0000\u0000\u0bcf\u0bdb\u0001\u0000\u0000\u0000\u0bd0"
+ "\u0bd1\u0003\u01de\u00ef\u0000\u0bd1\u0bd2\u0003\u01ea\u00f5\u0000\u0bd2"
+ "\u0bdc\u0001\u0000\u0000\u0000\u0bd3\u0bd5\u0005\u0110\u0000\u0000\u0bd4"
+ "\u0bd6\u0005D\u0000\u0000\u0bd5\u0bd4\u0001\u0000\u0000\u0000\u0bd5\u0bd6"
+ "\u0001\u0000\u0000\u0000\u0bd6\u0bd8\u0001\u0000\u0000\u0000\u0bd7\u0bd3"
+ "\u0001\u0000\u0000\u0000\u0bd7\u0bd8\u0001\u0000\u0000\u0000\u0bd8\u0bd9"
+ "\u0001\u0000\u0000\u0000\u0bd9\u0bda\u0005o\u0000\u0000\u0bda\u0bdc\u0003"
+ "\u01e8\u00f4\u0000\u0bdb\u0bd0\u0001\u0000\u0000\u0000\u0bdb\u0bd7\u0001"
+ "\u0000\u0000\u0000\u0bdc\u0bde\u0001\u0000\u0000\u0000\u0bdd\u0bca\u0001"
+ "\u0000\u0000\u0000\u0bdd\u0bce\u0001\u0000\u0000\u0000\u0bde\u01db\u0001"
+ "\u0000\u0000\u0000\u0bdf\u0be0\u00076\u0000\u0000\u0be0\u01dd\u0001\u0000"
+ "\u0000\u0000\u0be1\u0be2\u0007\u001b\u0000\u0000\u0be2\u01df\u0001\u0000"
+ "\u0000\u0000\u0be3\u0be4\u00077\u0000\u0000\u0be4\u01e1\u0001\u0000\u0000"
+ "\u0000\u0be5\u0be6\u00078\u0000\u0000\u0be6\u01e3\u0001\u0000\u0000\u0000"
+ "\u0be7\u0be8\u00079\u0000\u0000\u0be8\u01e5\u0001\u0000\u0000\u0000\u0be9"
+ "\u0bec\u0005\u0092\u0000\u0000\u0bea\u0bed\u0005\u00fc\u0000\u0000\u0beb"
+ "\u0bed\u0003\u0240\u0120\u0000\u0bec\u0bea\u0001\u0000\u0000\u0000\u0bec"
+ "\u0beb\u0001\u0000\u0000\u0000\u0bed\u0bee\u0001\u0000\u0000\u0000\u0bee"
+ "\u0bef\u0005\u00e0\u0000\u0000\u0bef\u01e7\u0001\u0000\u0000\u0000\u0bf0"
+ "\u0bf1\u0003\u01ee\u00f7\u0000\u0bf1\u01e9\u0001\u0000\u0000\u0000\u0bf2"
+ "\u0bf3\u0003\u01ee\u00f7\u0000\u0bf3\u01eb\u0001\u0000\u0000\u0000\u0bf4"
+ "\u0bf5\u0003\u01ee\u00f7\u0000\u0bf5\u01ed\u0001\u0000\u0000\u0000\u0bf6"
+ "\u0bfb\u0003\u0242\u0121\u0000\u0bf7\u0bf8\u0005-\u0000\u0000\u0bf8\u0bfa"
+ "\u0003\u0242\u0121\u0000\u0bf9\u0bf7\u0001\u0000\u0000\u0000\u0bfa\u0bfd"
+ "\u0001\u0000\u0000\u0000\u0bfb\u0bf9\u0001\u0000\u0000\u0000\u0bfb\u0bfc"
+ "\u0001\u0000\u0000\u0000\u0bfc\u01ef\u0001\u0000\u0000\u0000\u0bfd\u0bfb"
+ "\u0001\u0000\u0000\u0000\u0bfe\u0c02\u0005\u0104\u0000\u0000\u0bff\u0c00"
+ "\u0007:\u0000\u0000\u0c00\u0c02\u0003\u01f6\u00fb\u0000\u0c01\u0bfe\u0001"
+ "\u0000\u0000\u0000\u0c01\u0bff\u0001\u0000\u0000\u0000\u0c02\u0c03\u0001"
+ "\u0000\u0000\u0000\u0c03\u0c04\u0005\u00af\u0000\u0000\u0c04\u0c05\u0003"
+ "\u0220\u0110\u0000\u0c05\u0c09\u0003\u01fa\u00fd\u0000\u0c06\u0c07\u0005"
+ "\u0092\u0000\u0000\u0c07\u0c08\u0005\u00fc\u0000\u0000\u0c08\u0c0a\u0005"
+ "\u00e0\u0000\u0000\u0c09\u0c06\u0001\u0000\u0000\u0000\u0c09\u0c0a\u0001"
+ "\u0000\u0000\u0000\u0c0a\u01f1\u0001\u0000\u0000\u0000\u0c0b\u0c0f\u0005"
+ "E\u0000\u0000\u0c0c\u0c0d\u0005\u0097\u0000\u0000\u0c0d\u0c0f\u0003\u01f6"
+ "\u00fb\u0000\u0c0e\u0c0b\u0001\u0000\u0000\u0000\u0c0e\u0c0c\u0001\u0000"
+ "\u0000\u0000\u0c0f\u0c10\u0001\u0000\u0000\u0000\u0c10\u0c11\u0005\u00af"
+ "\u0000\u0000\u0c11\u0c12\u0003\u0220\u0110\u0000\u0c12\u0c13\u0003\u01fa"
+ "\u00fd\u0000\u0c13\u01f3\u0001\u0000\u0000\u0000\u0c14\u0c17\u0005\u00fc"
+ "\u0000\u0000\u0c15\u0c17\u0003\u01f8\u00fc\u0000\u0c16\u0c14\u0001\u0000"
+ "\u0000\u0000\u0c16\u0c15\u0001\u0000\u0000\u0000\u0c17\u01f5\u0001\u0000"
+ "\u0000\u0000\u0c18\u0c1b\u0005\u008a\u0000\u0000\u0c19\u0c1c\u0005\u00fc"
+ "\u0000\u0000\u0c1a\u0c1c\u0003\u01f8\u00fc\u0000\u0c1b\u0c19\u0001\u0000"
+ "\u0000\u0000\u0c1b\u0c1a\u0001\u0000\u0000\u0000\u0c1c\u0c1d\u0001\u0000"
+ "\u0000\u0000\u0c1d\u0c1e\u0005\u00cb\u0000\u0000\u0c1e\u01f7\u0001\u0000"
+ "\u0000\u0000\u0c1f\u0c24\u0003\u0252\u0129\u0000\u0c20\u0c21\u0005-\u0000"
+ "\u0000\u0c21\u0c23\u0003\u0252\u0129\u0000\u0c22\u0c20\u0001\u0000\u0000"
+ "\u0000\u0c23\u0c26\u0001\u0000\u0000\u0000\u0c24\u0c22\u0001\u0000\u0000"
+ "\u0000\u0c24\u0c25\u0001\u0000\u0000\u0000\u0c25\u01f9\u0001\u0000\u0000"
+ "\u0000\u0c26\u0c24\u0001\u0000\u0000\u0000\u0c27\u0c2a\u0003\u01fc\u00fe"
+ "\u0000\u0c28\u0c2b\u0005\u00fc\u0000\u0000\u0c29\u0c2b\u0003\u01f8\u00fc"
+ "\u0000\u0c2a\u0c28\u0001\u0000\u0000\u0000\u0c2a\u0c29\u0001\u0000\u0000"
+ "\u0000\u0c2b\u0c49\u0001\u0000\u0000\u0000\u0c2c\u0c2d\u0005k\u0000\u0000"
+ "\u0c2d\u0c2f\u0005\u0092\u0000\u0000\u0c2e\u0c30\u0003\u00fa}\u0000\u0c2f"
+ "\u0c2e\u0001\u0000\u0000\u0000\u0c2f\u0c30\u0001\u0000\u0000\u0000\u0c30"
+ "\u0c3a\u0001\u0000\u0000\u0000\u0c31\u0c32\u0005+\u0000\u0000\u0c32\u0c37"
+ "\u0003\u0252\u0129\u0000\u0c33\u0c34\u0005\u001c\u0000\u0000\u0c34\u0c36"
+ "\u0003\u0252\u0129\u0000\u0c35\u0c33\u0001\u0000\u0000\u0000\u0c36\u0c39"
+ "\u0001\u0000\u0000\u0000\u0c37\u0c35\u0001\u0000\u0000\u0000\u0c37\u0c38"
+ "\u0001\u0000\u0000\u0000\u0c38\u0c3b\u0001\u0000\u0000\u0000\u0c39\u0c37"
+ "\u0001\u0000\u0000\u0000\u0c3a\u0c31\u0001\u0000\u0000\u0000\u0c3a\u0c3b"
+ "\u0001\u0000\u0000\u0000\u0c3b\u0c46\u0001\u0000\u0000\u0000\u0c3c\u0c3d"
+ "\u0005\u00e0\u0000\u0000\u0c3d\u0c3e\u0005\u0119\u0000\u0000\u0c3e\u0c47"
+ "\u0003\u009aM\u0000\u0c3f\u0c40\u0005\u0119\u0000\u0000\u0c40\u0c43\u0003"
+ "\u009aM\u0000\u0c41\u0c43\u0003\u0250\u0128\u0000\u0c42\u0c3f\u0001\u0000"
+ "\u0000\u0000\u0c42\u0c41\u0001\u0000\u0000\u0000\u0c43\u0c44\u0001\u0000"
+ "\u0000\u0000\u0c44\u0c45\u0005\u00e0\u0000\u0000\u0c45\u0c47\u0001\u0000"
+ "\u0000\u0000\u0c46\u0c3c\u0001\u0000\u0000\u0000\u0c46\u0c42\u0001\u0000"
+ "\u0000\u0000\u0c47\u0c49\u0001\u0000\u0000\u0000\u0c48\u0c27\u0001\u0000"
+ "\u0000\u0000\u0c48\u0c2c\u0001\u0000\u0000\u0000\u0c48\u0c49\u0001\u0000"
+ "\u0000\u0000\u0c49\u01fb\u0001\u0000\u0000\u0000\u0c4a\u0c4e\u0003\u01fe"
+ "\u00ff\u0000\u0c4b\u0c4e\u0003\u0202\u0101\u0000\u0c4c\u0c4e\u0003\u0200"
+ "\u0100\u0000\u0c4d\u0c4a\u0001\u0000\u0000\u0000\u0c4d\u0c4b\u0001\u0000"
+ "\u0000\u0000\u0c4d\u0c4c\u0001\u0000\u0000\u0000\u0c4e\u01fd\u0001\u0000"
+ "\u0000\u0000\u0c4f\u0c50\u0007;\u0000\u0000\u0c50\u01ff\u0001\u0000\u0000"
+ "\u0000\u0c51\u0c52\u0007<\u0000\u0000\u0c52\u0201\u0001\u0000\u0000\u0000"
+ "\u0c53\u0c54\u0007=\u0000\u0000\u0c54\u0203\u0001\u0000\u0000\u0000\u0c55"
+ "\u0c56\u00051\u0000\u0000\u0c56\u0c57\u0005=\u0000\u0000\u0c57\u0c5b\u0003"
+ "\u023c\u011e\u0000\u0c58\u0c59\u0005x\u0000\u0000\u0c59\u0c5a\u0005\u00aa"
+ "\u0000\u0000\u0c5a\u0c5c\u0005d\u0000\u0000\u0c5b\u0c58\u0001\u0000\u0000"
+ "\u0000\u0c5b\u0c5c\u0001\u0000\u0000\u0000\u0c5c\u0c5e\u0001\u0000\u0000"
+ "\u0000\u0c5d\u0c5f\u0003\u0222\u0111\u0000\u0c5e\u0c5d\u0001\u0000\u0000"
+ "\u0000\u0c5e\u0c5f\u0001\u0000\u0000\u0000\u0c5f\u0c61\u0001\u0000\u0000"
+ "\u0000\u0c60\u0c62\u0003\u021a\u010d\u0000\u0c61\u0c60\u0001\u0000\u0000"
+ "\u0000\u0c61\u0c62\u0001\u0000\u0000\u0000\u0c62\u0205\u0001\u0000\u0000"
+ "\u0000\u0c63\u0c64\u0005=\u0000\u0000\u0c64\u0c68\u0003\u023c\u011e\u0000"
+ "\u0c65\u0c66\u0005x\u0000\u0000\u0c66\u0c67\u0005\u00aa\u0000\u0000\u0c67"
+ "\u0c69\u0005d\u0000\u0000\u0c68\u0c65\u0001\u0000\u0000\u0000\u0c68\u0c69"
+ "\u0001\u0000\u0000\u0000\u0c69\u0c71\u0001\u0000\u0000\u0000\u0c6a\u0c6d"
+ "\u0005\u0100\u0000\u0000\u0c6b\u0c6e\u0003\u0208\u0104\u0000\u0c6c\u0c6e"
+ "\u0003\u020a\u0105\u0000\u0c6d\u0c6b\u0001\u0000\u0000\u0000\u0c6d\u0c6c"
+ "\u0001\u0000\u0000\u0000\u0c6e\u0c6f\u0001\u0000\u0000\u0000\u0c6f\u0c6d"
+ "\u0001\u0000\u0000\u0000\u0c6f\u0c70\u0001\u0000\u0000\u0000\u0c70\u0c72"
+ "\u0001\u0000\u0000\u0000\u0c71\u0c6a\u0001\u0000\u0000\u0000\u0c71\u0c72"
+ "\u0001\u0000\u0000\u0000\u0c72\u0c74\u0001\u0000\u0000\u0000\u0c73\u0c75"
+ "\u0003\u0222\u0111\u0000\u0c74\u0c73\u0001\u0000\u0000\u0000\u0c74\u0c75"
+ "\u0001\u0000\u0000\u0000\u0c75\u0c77\u0001\u0000\u0000\u0000\u0c76\u0c78"
+ "\u0003\u021a\u010d\u0000\u0c77\u0c76\u0001\u0000\u0000\u0000\u0c77\u0c78"
+ "\u0001\u0000\u0000\u0000\u0c78\u0207\u0001\u0000\u0000\u0000\u0c79\u0c7a"
+ "\u0005\u0005\u0000\u0000\u0c7a\u0c7b\u0005\u00c1\u0000\u0000\u0c7b\u0209"
+ "\u0001\u0000\u0000\u0000\u0c7c\u0c7d\u0005\u0005\u0000\u0000\u0c7d\u0c7e"
+ "\u0005\u00e2\u0000\u0000\u0c7e\u020b\u0001\u0000\u0000\u0000\u0c7f\u0c81"
+ "\u00051\u0000\u0000\u0c80\u0c7f\u0001\u0000\u0000\u0000\u0c80\u0c81\u0001"
+ "\u0000\u0000\u0000\u0c81\u0c82\u0001\u0000\u0000\u0000\u0c82\u0c83\u0005"
+ "=\u0000\u0000\u0c83\u0c86\u0003\u023c\u011e\u0000\u0c84\u0c85\u0005x\u0000"
+ "\u0000\u0c85\u0c87\u0005d\u0000\u0000\u0c86\u0c84\u0001\u0000\u0000\u0000"
+ "\u0c86\u0c87\u0001\u0000\u0000\u0000\u0c87\u0c8a\u0001\u0000\u0000\u0000"
+ "\u0c88\u0c89\u0007>\u0000\u0000\u0c89\u0c8b\u0005<\u0000\u0000\u0c8a\u0c88"
+ "\u0001\u0000\u0000\u0000\u0c8a\u0c8b\u0001\u0000\u0000\u0000\u0c8b\u0c8d"
+ "\u0001\u0000\u0000\u0000\u0c8c\u0c8e\u0003\u021a\u010d\u0000\u0c8d\u0c8c"
+ "\u0001\u0000\u0000\u0000\u0c8d\u0c8e\u0001\u0000\u0000\u0000\u0c8e\u020d"
+ "\u0001\u0000\u0000\u0000\u0c8f\u0c90\u0005=\u0000\u0000\u0c90\u0c93\u0003"
+ "\u023c\u011e\u0000\u0c91\u0c92\u0005x\u0000\u0000\u0c92\u0c94\u0005d\u0000"
+ "\u0000\u0c93\u0c91\u0001\u0000\u0000\u0000\u0c93\u0c94\u0001\u0000\u0000"
+ "\u0000\u0c94\u0ca6\u0001\u0000\u0000\u0000\u0c95\u0c99\u0005\u00e8\u0000"
+ "\u0000\u0c96\u0c9a\u0003\u0210\u0108\u0000\u0c97\u0c9a\u0003\u0212\u0109"
+ "\u0000\u0c98\u0c9a\u0003\u0214\u010a\u0000\u0c99\u0c96\u0001\u0000\u0000"
+ "\u0000\u0c99\u0c97\u0001\u0000\u0000\u0000\u0c99\u0c98\u0001\u0000\u0000"
+ "\u0000\u0c9a\u0c9c\u0001\u0000\u0000\u0000\u0c9b\u0c95\u0001\u0000\u0000"
+ "\u0000\u0c9c\u0c9d\u0001\u0000\u0000\u0000\u0c9d\u0c9b\u0001\u0000\u0000"
+ "\u0000\u0c9d\u0c9e\u0001\u0000\u0000\u0000\u0c9e\u0ca7\u0001\u0000\u0000"
+ "\u0000\u0c9f\u0ca0\u0005\u00d4\u0000\u0000\u0ca0\u0ca1\u0005\u00b3\u0000"
+ "\u0000\u0ca1\u0ca3\u0003\u0252\u0129\u0000\u0ca2\u0c9f\u0001\u0000\u0000"
+ "\u0000\u0ca3\u0ca4\u0001\u0000\u0000\u0000\u0ca4\u0ca2\u0001\u0000\u0000"
+ "\u0000\u0ca4\u0ca5\u0001\u0000\u0000\u0000\u0ca5\u0ca7\u0001\u0000\u0000"
+ "\u0000\u0ca6\u0c9b\u0001\u0000\u0000\u0000\u0ca6\u0ca2\u0001\u0000\u0000"
+ "\u0000\u0ca7\u0ca9\u0001\u0000\u0000\u0000\u0ca8\u0caa\u0003\u021a\u010d"
+ "\u0000\u0ca9\u0ca8\u0001\u0000\u0000\u0000\u0ca9\u0caa\u0001\u0000\u0000"
+ "\u0000\u0caa\u020f\u0001\u0000\u0000\u0000\u0cab\u0cac\u0005\u000b\u0000"
+ "\u0000\u0cac\u0cad\u0005\u00cc\u0000\u0000\u0cad\u0cae\u0007?\u0000\u0000"
+ "\u0cae\u0211\u0001\u0000\u0000\u0000\u0caf\u0cb2\u0005\u0100\u0000\u0000"
+ "\u0cb0\u0cb3\u0003\u0208\u0104\u0000\u0cb1\u0cb3\u0003\u020a\u0105\u0000"
+ "\u0cb2\u0cb0\u0001\u0000\u0000\u0000\u0cb2\u0cb1\u0001\u0000\u0000\u0000"
+ "\u0cb3\u0cb4\u0001\u0000\u0000\u0000\u0cb4\u0cb2\u0001\u0000\u0000\u0000"
+ "\u0cb4\u0cb5\u0001\u0000\u0000\u0000\u0cb5\u0213\u0001\u0000\u0000\u0000"
+ "\u0cb6\u0cb7\u0005\u00b3\u0000\u0000\u0cb7\u0cb8\u0003\u0252\u0129\u0000"
+ "\u0cb8\u0cb9\u0003\u009aM\u0000\u0cb9\u0215\u0001\u0000\u0000\u0000\u0cba"
+ "\u0cbb\u0005\u00f0\u0000\u0000\u0cbb\u0cbc\u0005=\u0000\u0000\u0cbc\u0cbe"
+ "\u0003\u023c\u011e\u0000\u0cbd\u0cbf\u0003\u021a\u010d\u0000\u0cbe\u0cbd"
+ "\u0001\u0000\u0000\u0000\u0cbe\u0cbf\u0001\u0000\u0000\u0000\u0cbf\u0217"
+ "\u0001\u0000\u0000\u0000\u0cc0\u0cc1\u0005\u00f3\u0000\u0000\u0cc1\u0cc2"
+ "\u0005=\u0000\u0000\u0cc2\u0cc4\u0003\u023c\u011e\u0000\u0cc3\u0cc5\u0003"
+ "\u021a\u010d\u0000\u0cc4\u0cc3\u0001\u0000\u0000\u0000\u0cc4\u0cc5\u0001"
+ "\u0000\u0000\u0000\u0cc5\u0219\u0001\u0000\u0000\u0000\u0cc6\u0ccb\u0005"
+ "\u0117\u0000\u0000\u0cc7\u0cc9\u0005\u0005\u0000\u0000\u0cc8\u0cca\u0005"
+ "\u00e3\u0000\u0000\u0cc9\u0cc8\u0001\u0000\u0000\u0000\u0cc9\u0cca\u0001"
+ "\u0000\u0000\u0000\u0cca\u0ccc\u0001\u0000\u0000\u0000\u0ccb\u0cc7\u0001"
+ "\u0000\u0000\u0000\u0ccb\u0ccc\u0001\u0000\u0000\u0000\u0ccc\u0ccf\u0001"
+ "\u0000\u0000\u0000\u0ccd\u0ccf\u0005\u00ac\u0000\u0000\u0cce\u0cc6\u0001"
+ "\u0000\u0000\u0000\u0cce\u0ccd\u0001\u0000\u0000\u0000\u0ccf\u021b\u0001"
+ "\u0000\u0000\u0000\u0cd0\u0cd1\u0007+\u0000\u0000\u0cd1\u0cd3\u0005=\u0000"
+ "\u0000\u0cd2\u0cd4\u0003\u010a\u0085\u0000\u0cd3\u0cd2\u0001\u0000\u0000"
+ "\u0000\u0cd3\u0cd4\u0001\u0000\u0000\u0000\u0cd4\u0cdd\u0001\u0000\u0000"
+ "\u0000\u0cd5\u0cd7\u0007!\u0000\u0000\u0cd6\u0cd8\u0003\u023c\u011e\u0000"
+ "\u0cd7\u0cd6\u0001\u0000\u0000\u0000\u0cd7\u0cd8\u0001\u0000\u0000\u0000"
+ "\u0cd8\u0cda\u0001\u0000\u0000\u0000\u0cd9\u0cdb\u0003\u010a\u0085\u0000"
+ "\u0cda\u0cd9\u0001\u0000\u0000\u0000\u0cda\u0cdb\u0001\u0000\u0000\u0000"
+ "\u0cdb\u0cdd\u0001\u0000\u0000\u0000\u0cdc\u0cd0\u0001\u0000\u0000\u0000"
+ "\u0cdc\u0cd5\u0001\u0000\u0000\u0000\u0cdd\u021d\u0001\u0000\u0000\u0000"
+ "\u0cde\u0cdf\u0007+\u0000\u0000\u0cdf\u0ce6\u0005=\u0000\u0000\u0ce0\u0ce3"
+ "\u0007!\u0000\u0000\u0ce1\u0ce4\u0005\u00fc\u0000\u0000\u0ce2\u0ce4\u0003"
+ "\u023a\u011d\u0000\u0ce3\u0ce1\u0001\u0000\u0000\u0000\u0ce3\u0ce2\u0001"
+ "\u0000\u0000\u0000\u0ce4\u0ce6\u0001\u0000\u0000\u0000\u0ce5\u0cde\u0001"
+ "\u0000\u0000\u0000\u0ce5\u0ce0\u0001\u0000\u0000\u0000\u0ce6\u021f\u0001"
+ "\u0000\u0000\u0000\u0ce7\u0ce8\u0007+\u0000\u0000\u0ce8\u0cef\u0005r\u0000"
+ "\u0000\u0ce9\u0cec\u0007-\u0000\u0000\u0cea\u0ced\u0005\u00fc\u0000\u0000"
+ "\u0ceb\u0ced\u0003\u023a\u011d\u0000\u0cec\u0cea\u0001\u0000\u0000\u0000"
+ "\u0cec\u0ceb\u0001\u0000\u0000\u0000\u0ced\u0cef\u0001\u0000\u0000\u0000"
+ "\u0cee\u0ce7\u0001\u0000\u0000\u0000\u0cee\u0ce9\u0001\u0000\u0000\u0000"
+ "\u0cef\u0221\u0001\u0000\u0000\u0000\u0cf0\u0cf1\u0005\u00b2\u0000\u0000"
+ "\u0cf1\u0cf2\u0003\u024e\u0127\u0000\u0cf2\u0223\u0001\u0000\u0000\u0000"
+ "\u0cf3\u0cf6\u0003\u0252\u0129\u0000\u0cf4\u0cf6\u0003\u00eew\u0000\u0cf5"
+ "\u0cf3\u0001\u0000\u0000\u0000\u0cf5\u0cf4\u0001\u0000\u0000\u0000\u0cf6"
+ "\u0225\u0001\u0000\u0000\u0000\u0cf7\u0cfa\u0003\u0252\u0129\u0000\u0cf8"
+ "\u0cfa\u0003\u00eew\u0000\u0cf9\u0cf7\u0001\u0000\u0000\u0000\u0cf9\u0cf8"
+ "\u0001\u0000\u0000\u0000\u0cfa\u0227\u0001\u0000\u0000\u0000\u0cfb\u0cfc"
+ "\u0005\u000f\u0000\u0000\u0cfc\u0d00\u0003\u023c\u011e\u0000\u0cfd\u0cfe"
+ "\u0005x\u0000\u0000\u0cfe\u0cff\u0005\u00aa\u0000\u0000\u0cff\u0d01\u0005"
+ "d\u0000\u0000\u0d00\u0cfd\u0001\u0000\u0000\u0000\u0d00\u0d01\u0001\u0000"
+ "\u0000\u0000\u0d01\u0d02\u0001\u0000\u0000\u0000\u0d02\u0d03\u0005k\u0000"
+ "\u0000\u0d03\u0d04\u0005=\u0000\u0000\u0d04\u0d0f\u0003\u023c\u011e\u0000"
+ "\u0d05\u0d06\u0005\u001b\u0000\u0000\u0d06\u0d07\u0003\u024c\u0126\u0000"
+ "\u0d07\u0d08\u0005\u0110\u0000\u0000\u0d08\u0d09\u0003\u0224\u0112\u0000"
+ "\u0d09\u0d0a\u0005\u00b7\u0000\u0000\u0d0a\u0d0d\u0003\u0192\u00c9\u0000"
+ "\u0d0b\u0d0c\u0005Q\u0000\u0000\u0d0c\u0d0e\u0003\u024e\u0127\u0000\u0d0d"
+ "\u0d0b\u0001\u0000\u0000\u0000\u0d0d\u0d0e\u0001\u0000\u0000\u0000\u0d0e"
+ "\u0d10\u0001\u0000\u0000\u0000\u0d0f\u0d05\u0001\u0000\u0000\u0000\u0d0f"
+ "\u0d10\u0001\u0000\u0000\u0000\u0d10\u0d13\u0001\u0000\u0000\u0000\u0d11"
+ "\u0d12\u0005\u00c6\u0000\u0000\u0d12\u0d14\u0003\u024e\u0127\u0000\u0d13"
+ "\u0d11\u0001\u0000\u0000\u0000\u0d13\u0d14\u0001\u0000\u0000\u0000\u0d14"
+ "\u0229\u0001\u0000\u0000\u0000\u0d15\u0d16\u0005\u000f\u0000\u0000\u0d16"
+ "\u0d19\u0003\u023c\u011e\u0000\u0d17\u0d18\u0005x\u0000\u0000\u0d18\u0d1a"
+ "\u0005d\u0000\u0000\u0d19\u0d17\u0001\u0000\u0000\u0000\u0d19\u0d1a\u0001"
+ "\u0000\u0000\u0000\u0d1a\u0d1b\u0001\u0000\u0000\u0000\u0d1b\u0d1c\u0005"
+ "k\u0000\u0000\u0d1c\u0d1d\u0005=\u0000\u0000\u0d1d\u022b\u0001\u0000\u0000"
+ "\u0000\u0d1e\u0d1f\u0005\u000f\u0000\u0000\u0d1f\u0d22\u0003\u023c\u011e"
+ "\u0000\u0d20\u0d21\u0005x\u0000\u0000\u0d21\u0d23\u0005d\u0000\u0000\u0d22"
+ "\u0d20\u0001\u0000\u0000\u0000\u0d22\u0d23\u0001\u0000\u0000\u0000\u0d23"
+ "\u0d24\u0001\u0000\u0000\u0000\u0d24\u0d25\u0005\u00e8\u0000\u0000\u0d25"
+ "\u0d2b\u0005=\u0000\u0000\u0d26\u0d2c\u0003\u022e\u0117\u0000\u0d27\u0d2c"
+ "\u0003\u0230\u0118\u0000\u0d28\u0d2c\u0003\u0232\u0119\u0000\u0d29\u0d2c"
+ "\u0003\u0234\u011a\u0000\u0d2a\u0d2c\u0003\u0236\u011b\u0000\u0d2b\u0d26"
+ "\u0001\u0000\u0000\u0000\u0d2b\u0d27\u0001\u0000\u0000\u0000\u0d2b\u0d28"
+ "\u0001\u0000\u0000\u0000\u0d2b\u0d29\u0001\u0000\u0000\u0000\u0d2b\u0d2a"
+ "\u0001\u0000\u0000\u0000\u0d2c\u0d2d\u0001\u0000\u0000\u0000\u0d2d\u0d2b"
+ "\u0001\u0000\u0000\u0000\u0d2d\u0d2e\u0001\u0000\u0000\u0000\u0d2e\u022d"
+ "\u0001\u0000\u0000\u0000\u0d2f\u0d30\u0005\u00f7\u0000\u0000\u0d30\u0d33"
+ "\u0003\u023c\u011e\u0000\u0d31\u0d32\u0005\u001b\u0000\u0000\u0d32\u0d34"
+ "\u0003\u024c\u0126\u0000\u0d33\u0d31\u0001\u0000\u0000\u0000\u0d33\u0d34"
+ "\u0001\u0000\u0000\u0000\u0d34\u022f\u0001\u0000\u0000\u0000\u0d35\u0d36"
+ "\u0005\u0110\u0000\u0000\u0d36\u0d37\u0003\u0224\u0112\u0000\u0d37\u0231"
+ "\u0001\u0000\u0000\u0000\u0d38\u0d39\u0005\u00b7\u0000\u0000\u0d39\u0d3a"
+ "\u0003\u0192\u00c9\u0000\u0d3a\u0233\u0001\u0000\u0000\u0000\u0d3b\u0d3c"
+ "\u0005Q\u0000\u0000\u0d3c\u0d3d\u0003\u024e\u0127\u0000\u0d3d\u0235\u0001"
+ "\u0000\u0000\u0000\u0d3e\u0d3f\u0005\u00c6\u0000\u0000\u0d3f\u0d40\u0003"
+ "\u024e\u0127\u0000\u0d40\u0237\u0001\u0000\u0000\u0000\u0d41\u0d43\u0007"
+ "@\u0000\u0000\u0d42\u0d44\u0003\u023c\u011e\u0000\u0d43\u0d42\u0001\u0000"
+ "\u0000\u0000\u0d43\u0d44\u0001\u0000\u0000\u0000\u0d44\u0d45\u0001\u0000"
+ "\u0000\u0000\u0d45\u0d46\u0005k\u0000\u0000\u0d46\u0d48\u0007!\u0000\u0000"
+ "\u0d47\u0d49\u0003\u010a\u0085\u0000\u0d48\u0d47\u0001\u0000\u0000\u0000"
+ "\u0d48\u0d49\u0001\u0000\u0000\u0000\u0d49\u0239\u0001\u0000\u0000\u0000"
+ "\u0d4a\u0d4f\u0003\u023c\u011e\u0000\u0d4b\u0d4c\u0005-\u0000\u0000\u0d4c"
+ "\u0d4e\u0003\u023c\u011e\u0000\u0d4d\u0d4b\u0001\u0000\u0000\u0000\u0d4e"
+ "\u0d51\u0001\u0000\u0000\u0000\u0d4f\u0d4d\u0001\u0000\u0000\u0000\u0d4f"
+ "\u0d50\u0001\u0000\u0000\u0000\u0d50\u023b\u0001\u0000\u0000\u0000\u0d51"
+ "\u0d4f\u0001\u0000\u0000\u0000\u0d52\u0d55\u0003\u023e\u011f\u0000\u0d53"
+ "\u0d55\u0003\u00eew\u0000\u0d54\u0d52\u0001\u0000\u0000\u0000\u0d54\u0d53"
+ "\u0001\u0000\u0000\u0000\u0d55\u023d\u0001\u0000\u0000\u0000\u0d56\u0d5b"
+ "\u0003\u0252\u0129\u0000\u0d57\u0d58\u0005N\u0000\u0000\u0d58\u0d5a\u0003"
+ "\u0252\u0129\u0000\u0d59\u0d57\u0001\u0000\u0000\u0000\u0d5a\u0d5d\u0001"
+ "\u0000\u0000\u0000\u0d5b\u0d59\u0001\u0000\u0000\u0000\u0d5b\u0d5c\u0001"
+ "\u0000\u0000\u0000\u0d5c\u023f\u0001\u0000\u0000\u0000\u0d5d\u0d5b\u0001"
+ "\u0000\u0000\u0000\u0d5e\u0d63\u0003\u0224\u0112\u0000\u0d5f\u0d60\u0005"
+ "-\u0000\u0000\u0d60\u0d62\u0003\u0224\u0112\u0000\u0d61\u0d5f\u0001\u0000"
+ "\u0000\u0000\u0d62\u0d65\u0001\u0000\u0000\u0000\u0d63\u0d61\u0001\u0000"
+ "\u0000\u0000\u0d63\u0d64\u0001\u0000\u0000\u0000\u0d64\u0241\u0001\u0000"
+ "\u0000\u0000\u0d65\u0d63\u0001\u0000\u0000\u0000\u0d66\u0d68\u0003\u0254"
+ "\u012a\u0000\u0d67\u0d69\u0003\u0244\u0122\u0000\u0d68\u0d67\u0001\u0000"
+ "\u0000\u0000\u0d68\u0d69\u0001\u0000\u0000\u0000\u0d69\u0d6c\u0001\u0000"
+ "\u0000\u0000\u0d6a\u0d6c\u0003\u0244\u0122\u0000\u0d6b\u0d66\u0001\u0000"
+ "\u0000\u0000\u0d6b\u0d6a\u0001\u0000\u0000\u0000\u0d6c\u0243\u0001\u0000"
+ "\u0000\u0000\u0d6d\u0d6f\u0003\u0246\u0123\u0000\u0d6e\u0d70\u0003\u0244"
+ "\u0122\u0000\u0d6f\u0d6e\u0001\u0000\u0000\u0000\u0d6f\u0d70\u0001\u0000"
+ "\u0000\u0000\u0d70\u0245\u0001\u0000\u0000\u0000\u0d71\u0d73\u0005N\u0000"
+ "\u0000\u0d72\u0d74\u0003\u0254\u012a\u0000\u0d73\u0d72\u0001\u0000\u0000"
+ "\u0000\u0d73\u0d74\u0001\u0000\u0000\u0000\u0d74\u0d79\u0001\u0000\u0000"
+ "\u0000\u0d75\u0d79\u0005\u00c8\u0000\u0000\u0d76\u0d79\u0005\u00fc\u0000"
+ "\u0000\u0d77\u0d79\u0003\u0256\u012b\u0000\u0d78\u0d71\u0001\u0000\u0000"
+ "\u0000\u0d78\u0d75\u0001\u0000\u0000\u0000\u0d78\u0d76\u0001\u0000\u0000"
+ "\u0000\u0d78\u0d77\u0001\u0000\u0000\u0000\u0d79\u0247\u0001\u0000\u0000"
+ "\u0000\u0d7a\u0d7d\u0003\u024a\u0125\u0000\u0d7b\u0d7c\u0005-\u0000\u0000"
+ "\u0d7c\u0d7e\u0003\u024a\u0125\u0000\u0d7d\u0d7b\u0001\u0000\u0000\u0000"
+ "\u0d7e\u0d7f\u0001\u0000\u0000\u0000\u0d7f\u0d7d\u0001\u0000\u0000\u0000"
+ "\u0d7f\u0d80\u0001\u0000\u0000\u0000\u0d80\u0249\u0001\u0000\u0000\u0000"
+ "\u0d81\u0d82\u0007A\u0000\u0000\u0d82\u024b\u0001\u0000\u0000\u0000\u0d83"
+ "\u0d86\u0003\u024a\u0125\u0000\u0d84\u0d86\u0003\u00eew\u0000\u0d85\u0d83"
+ "\u0001\u0000\u0000\u0000\u0d85\u0d84\u0001\u0000\u0000\u0000\u0d86\u024d"
+ "\u0001\u0000\u0000\u0000\u0d87\u0d8a\u0003\u0250\u0128\u0000\u0d88\u0d8a"
+ "\u0003\u00eew\u0000\u0d89\u0d87\u0001\u0000\u0000\u0000\u0d89\u0d88\u0001"
+ "\u0000\u0000\u0000\u0d8a\u024f\u0001\u0000\u0000\u0000\u0d8b\u0d99\u0005"
+ "\u008a\u0000\u0000\u0d8c\u0d8d\u0003\u00ecv\u0000\u0d8d\u0d8e\u0005+\u0000"
+ "\u0000\u0d8e\u0d96\u0003\u009aM\u0000\u0d8f\u0d90\u0005-\u0000\u0000\u0d90"
+ "\u0d91\u0003\u00ecv\u0000\u0d91\u0d92\u0005+\u0000\u0000\u0d92\u0d93\u0003"
+ "\u009aM\u0000\u0d93\u0d95\u0001\u0000\u0000\u0000\u0d94\u0d8f\u0001\u0000"
+ "\u0000\u0000\u0d95\u0d98\u0001\u0000\u0000\u0000\u0d96\u0d94\u0001\u0000"
+ "\u0000\u0000\u0d96\u0d97\u0001\u0000\u0000\u0000\u0d97\u0d9a\u0001\u0000"
+ "\u0000\u0000\u0d98\u0d96\u0001\u0000\u0000\u0000\u0d99\u0d8c\u0001\u0000"
+ "\u0000\u0000\u0d99\u0d9a\u0001\u0000\u0000\u0000\u0d9a\u0d9b\u0001\u0000"
+ "\u0000\u0000\u0d9b\u0d9c\u0005\u00cb\u0000\u0000\u0d9c\u0251\u0001\u0000"
+ "\u0000\u0000\u0d9d\u0da0\u0003\u0254\u012a\u0000\u0d9e\u0da0\u0003\u0256"
+ "\u012b\u0000\u0d9f\u0d9d\u0001\u0000\u0000\u0000\u0d9f\u0d9e\u0001\u0000"
+ "\u0000\u0000\u0da0\u0253\u0001\u0000\u0000\u0000\u0da1\u0da2\u0005\n\u0000"
+ "\u0000\u0da2\u0255\u0001\u0000\u0000\u0000\u0da3\u0dad\u0003\u025a\u012d"
+ "\u0000\u0da4\u0dad\u0005\u00aa\u0000\u0000\u0da5\u0dad\u0005\u00ad\u0000"
+ "\u0000\u0da6\u0dad\u0005\u0108\u0000\u0000\u0da7\u0dad\u0005\u00a9\u0000"
+ "\u0000\u0da8\u0dad\u0005\u009f\u0000\u0000\u0da9\u0dad\u0005\u00a0\u0000"
+ "\u0000\u0daa\u0dad\u0005\u00a1\u0000\u0000\u0dab\u0dad\u0005\u00a2\u0000"
+ "\u0000\u0dac\u0da3\u0001\u0000\u0000\u0000\u0dac\u0da4\u0001\u0000\u0000"
+ "\u0000\u0dac\u0da5\u0001\u0000\u0000\u0000\u0dac\u0da6\u0001\u0000\u0000"
+ "\u0000\u0dac\u0da7\u0001\u0000\u0000\u0000\u0dac\u0da8\u0001\u0000\u0000"
+ "\u0000\u0dac\u0da9\u0001\u0000\u0000\u0000\u0dac\u0daa\u0001\u0000\u0000"
+ "\u0000\u0dac\u0dab\u0001\u0000\u0000\u0000\u0dad\u0257\u0001\u0000\u0000"
+ "\u0000\u0dae\u0db1\u0003\u0254\u012a\u0000\u0daf\u0db1\u0003\u025a\u012d"
+ "\u0000\u0db0\u0dae\u0001\u0000\u0000\u0000\u0db0\u0daf\u0001\u0000\u0000"
+ "\u0000\u0db1\u0259\u0001\u0000\u0000\u0000\u0db2\u0db3\u0007B\u0000\u0000"
+ "\u0db3\u025b\u0001\u0000\u0000\u0000\u0db4\u0db5\u0005\u0000\u0000\u0001"
+ "\u0db5\u025d\u0001\u0000\u0000\u0000\u01c8\u0263\u0267\u026c\u0270\u0276"
+ "\u027b\u0280\u0286\u0298\u029c\u02a6\u02ae\u02b2\u02b5\u02b8\u02bd\u02c1"
+ "\u02c7\u02cc\u02d5\u02e4\u02f2\u0307\u030f\u0319\u031c\u0324\u0328\u032c"
+ "\u0332\u0336\u033b\u033e\u0343\u0346\u0348\u0354\u0357\u0366\u036d\u0382"
+ "\u0385\u0388\u0391\u0395\u0397\u0399\u039e\u03a4\u03ac\u03b7\u03c0\u03c4"
+ "\u03c7\u03cd\u03cf\u03e4\u03ec\u03f2\u03f5\u03fc\u0404\u040c\u0410\u0415"
+ "\u0419\u0423\u0429\u042d\u042f\u0434\u0439\u043d\u0440\u0444\u0448\u044b"
+ "\u0451\u0453\u045b\u045f\u0462\u0465\u0469\u046f\u0472\u0475\u047d\u0481"
+ "\u0486\u048d\u049b\u049e\u04a3\u04a6\u04a9\u04ac\u04b0\u04b3\u04b7\u04ba"
+ "\u04bf\u04c3\u04c8\u04d2\u04d6\u04d9\u04df\u04e4\u04e9\u04ef\u04f4\u04fc"
+ "\u0504\u050a\u0512\u051d\u0525\u052d\u0538\u0540\u0548\u054e\u0558\u055d"
+ "\u0566\u056b\u0570\u0574\u0579\u057c\u057f\u0588\u0590\u0598\u059e\u05a4"
+ "\u05af\u05b3\u05b6\u05bf\u05d6\u05e0\u05e6\u05ea\u05f8\u05fc\u0606\u0611"
+ "\u0616\u061b\u061f\u0624\u0627\u062d\u0635\u063b\u063d\u0645\u064a\u0664"
+ "\u066d\u0674\u0677\u067a\u068e\u0691\u069d\u06a8\u06ac\u06ae\u06b6\u06ba"
+ "\u06bc\u06c6\u06cb\u06d5\u06d8\u06e3\u06e8\u06ef\u06f2\u0700\u070a\u070e"
+ "\u071d\u0722\u072b\u0735\u073d\u0743\u0756\u075a\u075d\u0762\u0771\u0774"
+ "\u0777\u077a\u077d\u0780\u0784\u0788\u078b\u0790\u0793\u0797\u079a\u079e"
+ "\u07a1\u07a4\u07ad\u07b5\u07ba\u07bd\u07c3\u07ca\u07d5\u07d9\u07dc\u07e1"
+ "\u07e3\u07e6\u07ea\u07ed\u07f1\u07f4\u07f7\u07fa\u07fe\u0801\u0804\u080b"
+ "\u080d\u0814\u0823\u0827\u0829\u082c\u0830\u0839\u083d\u0844\u0849\u084e"
+ "\u0857\u0865\u086c\u0886\u088d\u088f\u0894\u0898\u08a2\u08ab\u08b2\u08b7"
+ "\u08bc\u08c0\u08ca\u08d2\u08da\u08e4\u08ea\u08f6\u08f8\u08fd\u08ff\u091a"
+ "\u091c\u0924\u0929\u092e\u0933\u0936\u093b\u0940\u094d\u0952\u095c\u0964"
+ "\u096f\u0975\u097b\u0980\u0985\u098c\u0997\u099f\u09a5\u09b1\u09b3\u09c1"
+ "\u09c6\u09cc\u09dd\u09e0\u09e4\u09ef\u09fa\u0a00\u0a06\u0a0c\u0a12\u0a17"
+ "\u0a1a\u0a23\u0a2c\u0a30\u0a37\u0a3d\u0a4f\u0a57\u0a5b\u0a60\u0a63\u0a68"
+ "\u0a6c\u0a70\u0a7d\u0a82\u0a85\u0a89\u0a8c\u0a91\u0a97\u0a9a\u0a9e\u0aa2"
+ "\u0aa5\u0aa9\u0ab1\u0aba\u0ac2\u0aca\u0acd\u0ad6\u0ae8\u0aec\u0af2\u0afb"
+ "\u0b00\u0b03\u0b12\u0b19\u0b1d\u0b23\u0b29\u0b2f\u0b34\u0b39\u0b42\u0b4a"
+ "\u0b51\u0b53\u0b60\u0b64\u0b6c\u0b70\u0b7f\u0b8c\u0b98\u0b9b\u0b9f\u0ba3"
+ "\u0ba6\u0ba8\u0bb3\u0bba\u0bc2\u0bc4\u0bce\u0bd5\u0bd7\u0bdb\u0bdd\u0bec"
+ "\u0bfb\u0c01\u0c09\u0c0e\u0c16\u0c1b\u0c24\u0c2a\u0c2f\u0c37\u0c3a\u0c42"
+ "\u0c46\u0c48\u0c4d\u0c5b\u0c5e\u0c61\u0c68\u0c6d\u0c6f\u0c71\u0c74\u0c77"
+ "\u0c80\u0c86\u0c8a\u0c8d\u0c93\u0c99\u0c9d\u0ca4\u0ca6\u0ca9\u0cb2\u0cb4"
+ "\u0cbe\u0cc4\u0cc9\u0ccb\u0cce\u0cd3\u0cd7\u0cda\u0cdc\u0ce3\u0ce5\u0cec"
+ "\u0cee\u0cf5\u0cf9\u0d00\u0d0d\u0d0f\u0d13\u0d19\u0d22\u0d2b\u0d2d\u0d33"
+ "\u0d43\u0d48\u0d4f\u0d54\u0d5b\u0d63\u0d68\u0d6b\u0d6f\u0d73\u0d78\u0d7f"
+ "\u0d85\u0d89\u0d96\u0d99\u0d9f\u0dac\u0db0";
public static final String _serializedATN =
Utils.join(new String[] {_serializedATNSegment0, _serializedATNSegment1}, "");
public static final ATN _ATN = new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy