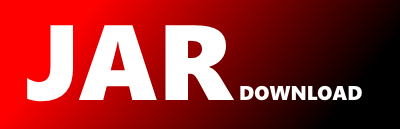
org.neo4j.cypher.internal.parser.javacc.Cypher Maven / Gradle / Ivy
/* Cypher.java */
/* Generated by: ParserGeneratorCC: Do not edit this line. Cypher.java */
/*
* Copyright (c) 2002-2019 "Neo4j,"
* Neo4j Sweden AB [http://neo4j.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.cypher.internal.parser.javacc;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import org.neo4j.cypher.internal.ast.factory.AccessType;
import org.neo4j.cypher.internal.ast.factory.ASTExceptionFactory;
import org.neo4j.cypher.internal.ast.factory.ASTFactory;
import org.neo4j.cypher.internal.ast.factory.ASTFactory.StringPos;
import org.neo4j.cypher.internal.ast.factory.ActionType;
import org.neo4j.cypher.internal.ast.factory.ConstraintType;
import org.neo4j.cypher.internal.ast.factory.ConstraintVersion;
import org.neo4j.cypher.internal.ast.factory.CreateIndexTypes;
import org.neo4j.cypher.internal.ast.factory.HintIndexType;
import org.neo4j.cypher.internal.ast.factory.ParameterType;
import org.neo4j.cypher.internal.ast.factory.ScopeType;
import org.neo4j.cypher.internal.ast.factory.ShowCommandFilterTypes;
import org.neo4j.cypher.internal.ast.factory.SimpleEither;
/** Simple brace matcher. */
public class Cypher implements CypherConstants {
ASTExceptionFactory exceptionFactory;
ASTFactory astFactory;
public Cypher(ASTFactory astFactory,
ASTExceptionFactory exceptionFactory,
CharStream stream) {
this(stream);
this.astFactory = astFactory;
this.exceptionFactory = exceptionFactory;
}
private POS pos( Token t )
{
return t != null ? astFactory.inputPosition( t.beginOffset, t.beginLine, t.beginColumn ) : null;
}
private void assertValidType( Token t, String expected, String actual ) throws Exception
{
if ( expected != null && !expected.equals(actual) )
{
throw exceptionFactory.syntaxException( new ParseException(
String.format("Invalid input '%s': expected \"%s\"", t.image, expected ) ), t.beginOffset, t.beginLine, t.beginColumn );
}
}
private void assertNotAlreadySet( Object object, Token token, String errorMessage ) throws Exception
{
if ( object != null )
{
throw exceptionFactory.syntaxException( new ParseException( errorMessage ), token.beginOffset, token.beginLine, token.beginColumn );
}
}
/** Root production. */
final public List Statements() throws ParseException, Exception {STATEMENT x;
List stmts = new ArrayList<>();
try {
x = Statement();
stmts.add( x );
label_1:
while (true) {
if (jj_2_1(2)) {
} else {
break label_1;
}
jj_consume_token(249);
x = Statement();
stmts.add( x );
}
if (jj_2_2(2)) {
jj_consume_token(249);
} else {
;
}
jj_consume_token(0);
{if ("" != null) return stmts;}
} catch (ParseException e) {
Token t = e.currentToken.next;
if ( e.getMessage().contains( "Encountered \"\"" ) )
{
throw exceptionFactory.syntaxException( t.image, ParseExceptions.expected( e.expectedTokenSequences, e.tokenImage ), e,
t.endOffset + 1, t.endLine, t.endColumn + 1 );
}
else
{
throw exceptionFactory.syntaxException( t.image, ParseExceptions.expected( e.expectedTokenSequences, e.tokenImage ), e,
t.beginOffset, t.beginLine, t.beginColumn );
}
} catch (InvalidUnicodeLiteral e) {
throw exceptionFactory.syntaxException( e, e.offset, e.line, e.column );
}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT Statement() throws ParseException, Exception {STATEMENT statement;
USE_CLAUSE useClause = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case USING:{
statement = PeriodicCommitQuery();
break;
}
case USE:{
useClause = UseClause();
statement = SingleQueryOrCommandWithUseClause(useClause);
break;
}
case ALTER:
case CALL:
case CATALOG:
case CREATE:
case DELETE:
case DENY:
case DETACH:
case DROP:
case FOREACH:
case GRANT:
case LOAD:
case MATCH:
case MERGE:
case OPTIONAL:
case RENAME:
case REMOVE:
case RETURN:
case REVOKE:
case SET:
case SHOW:
case START:
case STOP:
case TERMINATE:
case UNWIND:
case WITH:{
statement = SingleQueryOrCommand();
break;
}
default:
jj_la1[0] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
{if ("" != null) return statement;}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT SingleQueryOrCommand() throws ParseException, Exception {STATEMENT statement = null;
QUERY query = null;
boolean hasCatalog = false;
if (jj_2_3(2)) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case CATALOG:{
jj_consume_token(CATALOG);
hasCatalog = true;
break;
}
default:
jj_la1[1] = jj_gen;
;
}
statement = CreateCommand(null);
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ALTER:
case CATALOG:
case DENY:
case DROP:
case GRANT:
case RENAME:
case REVOKE:
case SHOW:
case START:
case STOP:
case TERMINATE:{
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case CATALOG:{
jj_consume_token(CATALOG);
hasCatalog = true;
break;
}
default:
jj_la1[2] = jj_gen;
;
}
statement = Command(null);
break;
}
case CALL:
case CREATE:
case DELETE:
case DETACH:
case FOREACH:
case LOAD:
case MATCH:
case MERGE:
case OPTIONAL:
case REMOVE:
case RETURN:
case SET:
case UNWIND:
case USE:
case WITH:{
query = SingleQuery();
label_2:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case UNION:{
break;
}
default:
jj_la1[3] = jj_gen;
break label_2;
}
query = Union(query);
}
break;
}
default:
jj_la1[4] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
if ( query != null )
{
{if ("" != null) return query;}
}
{if ("" != null) return hasCatalog ? astFactory.hasCatalog( statement ) : statement;}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT SingleQueryOrCommandWithUseClause(USE_CLAUSE useClause) throws ParseException, Exception {STATEMENT statement = null;
QUERY query = null;
boolean hasCatalog = false;
if (jj_2_4(2)) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case CATALOG:{
jj_consume_token(CATALOG);
hasCatalog = true;
break;
}
default:
jj_la1[5] = jj_gen;
;
}
statement = CreateCommand(useClause);
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ALTER:
case CATALOG:
case DENY:
case DROP:
case GRANT:
case RENAME:
case REVOKE:
case SHOW:
case START:
case STOP:
case TERMINATE:{
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case CATALOG:{
jj_consume_token(CATALOG);
hasCatalog = true;
break;
}
default:
jj_la1[6] = jj_gen;
;
}
statement = Command(useClause);
break;
}
default:
jj_la1[8] = jj_gen;
query = SingleQueryWithUseClause(useClause);
label_3:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case UNION:{
break;
}
default:
jj_la1[7] = jj_gen;
break label_3;
}
query = Union(query);
}
}
}
if ( query != null )
{
{if ("" != null) return query;}
}
{if ("" != null) return hasCatalog ? astFactory.hasCatalog( statement ) : statement;}
throw new IllegalStateException ("Missing return statement in function");
}
final public QUERY PeriodicCommitQuery() throws ParseException {Token t;
Token tPeriodicCommit;
Token batchSize = null;
CLAUSE loadCsv;
List queryBody;
t = jj_consume_token(USING);
tPeriodicCommit = jj_consume_token(PERIODIC);
jj_consume_token(COMMIT);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case UNSIGNED_DECIMAL_INTEGER:{
batchSize = jj_consume_token(UNSIGNED_DECIMAL_INTEGER);
break;
}
default:
jj_la1[9] = jj_gen;
;
}
loadCsv = LoadCSVClause();
queryBody = PeriodicCommitQueryBody();
{if ("" != null) return astFactory.periodicCommitQuery( pos( t ), pos( tPeriodicCommit ), batchSize == null ? null : batchSize.image, loadCsv, queryBody );}
throw new IllegalStateException ("Missing return statement in function");
}
final public List PeriodicCommitQueryBody() throws ParseException {CLAUSE x;
List clauses = new ArrayList<>();
label_4:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case CALL:
case CREATE:
case DELETE:
case DETACH:
case FOREACH:
case LOAD:
case MATCH:
case MERGE:
case OPTIONAL:
case REMOVE:
case RETURN:
case SET:
case UNWIND:
case USE:
case WITH:{
break;
}
default:
jj_la1[10] = jj_gen;
break label_4;
}
x = Clause();
clauses.add( x );
}
{if ("" != null) return clauses;}
throw new IllegalStateException ("Missing return statement in function");
}
final public QUERY RegularQuery() throws ParseException {QUERY x;
x = SingleQuery();
label_5:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case UNION:{
break;
}
default:
jj_la1[11] = jj_gen;
break label_5;
}
x = Union(x);
}
{if ("" != null) return x;}
throw new IllegalStateException ("Missing return statement in function");
}
final public QUERY Union(QUERY lhs) throws ParseException {Token t;
QUERY rhs;
boolean all = false;
t = jj_consume_token(UNION);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ALL:{
jj_consume_token(ALL);
all = true;
break;
}
default:
jj_la1[12] = jj_gen;
;
}
rhs = SingleQuery();
{if ("" != null) return astFactory.newUnion( pos( t ), lhs, rhs, all );}
throw new IllegalStateException ("Missing return statement in function");
}
final public QUERY SingleQuery() throws ParseException {CLAUSE x;
List clauses = new ArrayList<>();
label_6:
while (true) {
x = Clause();
clauses.add( x );
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case CALL:
case CREATE:
case DELETE:
case DETACH:
case FOREACH:
case LOAD:
case MATCH:
case MERGE:
case OPTIONAL:
case REMOVE:
case RETURN:
case SET:
case UNWIND:
case USE:
case WITH:{
break;
}
default:
jj_la1[13] = jj_gen;
break label_6;
}
}
{if ("" != null) return astFactory.newSingleQuery( clauses );}
throw new IllegalStateException ("Missing return statement in function");
}
final public QUERY SingleQueryWithUseClause(CLAUSE useClause) throws ParseException {CLAUSE x;
List clauses = new ArrayList<>();
if ( useClause != null )
{
clauses.add( useClause );
}
label_7:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case CALL:
case CREATE:
case DELETE:
case DETACH:
case FOREACH:
case LOAD:
case MATCH:
case MERGE:
case OPTIONAL:
case REMOVE:
case RETURN:
case SET:
case UNWIND:
case USE:
case WITH:{
break;
}
default:
jj_la1[14] = jj_gen;
break label_7;
}
x = Clause();
clauses.add( x );
}
{if ("" != null) return astFactory.newSingleQuery( clauses );}
throw new IllegalStateException ("Missing return statement in function");
}
final public CLAUSE Clause() throws ParseException {CLAUSE x = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case USE:{
x = UseClause();
break;
}
case RETURN:{
x = ReturnClause();
break;
}
case CREATE:{
x = CreateClause();
break;
}
case DELETE:
case DETACH:{
x = DeleteClause();
break;
}
case SET:{
x = SetClause();
break;
}
case REMOVE:{
x = RemoveClause();
break;
}
case MATCH:
case OPTIONAL:{
x = MatchClause();
break;
}
case MERGE:{
x = MergeClause();
break;
}
case WITH:{
x = WithClause();
break;
}
case UNWIND:{
x = UnwindClause();
break;
}
default:
jj_la1[15] = jj_gen;
if (jj_2_5(2)) {
x = CallClause();
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case CALL:{
x = SubqueryClause();
break;
}
case LOAD:{
x = LoadCSVClause();
break;
}
case FOREACH:{
x = ForeachClause();
break;
}
default:
jj_la1[16] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
}
{if ("" != null) return x;}
throw new IllegalStateException ("Missing return statement in function");
}
// USE
final public
USE_CLAUSE UseClause() throws ParseException {Token t;
EXPRESSION e;
t = jj_consume_token(USE);
if (jj_2_6(2)) {
jj_consume_token(GRAPH);
} else {
;
}
e = Expression();
{if ("" != null) return astFactory.useClause( pos( t ), e );}
throw new IllegalStateException ("Missing return statement in function");
}
// RETURN
final public
RETURN_CLAUSE ReturnClause() throws ParseException {Token t;
RETURN_CLAUSE clause = null;
t = jj_consume_token(RETURN);
clause = ReturnBody(t);
{if ("" != null) return clause;}
throw new IllegalStateException ("Missing return statement in function");
}
final public RETURN_CLAUSE ReturnBody(Token t) throws ParseException {Token skipPosition = null;
Token limitPosition = null;
boolean distinct = false;
List order = new ArrayList<>();
Token orderPos = null;
EXPRESSION skip = null;
EXPRESSION limit = null;
ORDER_ITEM o = null;
RETURN_ITEM x;
RETURN_ITEMS returnItems;
if (jj_2_7(2)) {
jj_consume_token(DISTINCT);
distinct = true;
} else {
;
}
returnItems = ReturnItems();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ORDER:{
orderPos = jj_consume_token(ORDER);
jj_consume_token(BY);
o = OrderItem();
order.add( o );
label_8:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[17] = jj_gen;
break label_8;
}
jj_consume_token(250);
o = OrderItem();
order.add( o );
}
break;
}
default:
jj_la1[18] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case SKIPROWS:{
skipPosition=token.next;
skip = Skip();
break;
}
default:
jj_la1[19] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case LIMITROWS:{
limitPosition=token.next;
limit = Limit();
break;
}
default:
jj_la1[20] = jj_gen;
;
}
{if ("" != null) return astFactory.newReturnClause( pos( t ), distinct, returnItems, order, pos( orderPos ), skip, pos( skipPosition ), limit, pos( limitPosition ) );}
throw new IllegalStateException ("Missing return statement in function");
}
final public RETURN_ITEM ReturnItem() throws ParseException {EXPRESSION e;
VARIABLE v = null;
Token eStart;
Token eEnd;
eStart = token;
e = Expression();
eEnd = token;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case AS:{
jj_consume_token(AS);
v = Variable();
break;
}
default:
jj_la1[21] = jj_gen;
;
}
if ( v != null )
{
{if ("" != null) return astFactory.newReturnItem( pos( eStart.next ), e, v );}
}
else
{
{if ("" != null) return astFactory.newReturnItem( pos( eStart.next ), e, eStart.next.beginOffset, eEnd.endOffset );}
}
throw new IllegalStateException ("Missing return statement in function");
}
final public RETURN_ITEMS ReturnItems() throws ParseException {Token returnItemsPosition;
RETURN_ITEM x;
List returnItems = new ArrayList<>();
boolean returnAll = false;
returnItemsPosition = token;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case TIMES:{
jj_consume_token(TIMES);
returnAll = true;
label_9:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[22] = jj_gen;
break label_9;
}
jj_consume_token(250);
x = ReturnItem();
returnItems.add( x );
}
break;
}
case DECIMAL_DOUBLE:
case UNSIGNED_DECIMAL_INTEGER:
case UNSIGNED_HEX_INTEGER:
case UNSIGNED_OCTAL_INTEGER:
case STRING_LITERAL1:
case STRING_LITERAL2:
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LBRACKET:
case LCURLY:
case LIMITROWS:
case LOAD:
case LOOKUP:
case LPAREN:
case MANAGEMENT:
case MATCH:
case MERGE:
case MINUS:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case PLUS:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
x = ReturnItem();
returnItems.add( x );
label_10:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[23] = jj_gen;
break label_10;
}
jj_consume_token(250);
x = ReturnItem();
returnItems.add( x );
}
break;
}
default:
jj_la1[24] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
{if ("" != null) return astFactory.newReturnItems( pos( returnItemsPosition.next ), returnAll, returnItems );}
throw new IllegalStateException ("Missing return statement in function");
}
final public ORDER_ITEM OrderItem() throws ParseException {Token t;
EXPRESSION e;
t = token;
e = Expression();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DESC:{
jj_consume_token(DESC);
{if ("" != null) return astFactory.orderDesc( pos( t.next ), e );}
break;
}
default:
jj_la1[26] = jj_gen;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ASC:{
jj_consume_token(ASC);
break;
}
default:
jj_la1[25] = jj_gen;
;
}
{if ("" != null) return astFactory.orderAsc( pos( t.next ), e );}
}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION Skip() throws ParseException {EXPRESSION e;
jj_consume_token(SKIPROWS);
e = Expression();
{if ("" != null) return e;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION Limit() throws ParseException {EXPRESSION e;
jj_consume_token(LIMITROWS);
e = Expression();
{if ("" != null) return e;}
throw new IllegalStateException ("Missing return statement in function");
}
// WHERE
final public
WHERE WhereClause() throws ParseException {Token t;
EXPRESSION e;
t = jj_consume_token(WHERE);
e = Expression();
{if ("" != null) return astFactory.whereClause( pos( t ), e );}
throw new IllegalStateException ("Missing return statement in function");
}
// WITH
final public
CLAUSE WithClause() throws ParseException {Token t;
RETURN_CLAUSE returnClause;
WHERE where = null;
t = jj_consume_token(WITH);
returnClause = ReturnBody(t);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:{
where = WhereClause();
break;
}
default:
jj_la1[27] = jj_gen;
;
}
{if ("" != null) return astFactory.withClause( pos( t ), returnClause, where );}
throw new IllegalStateException ("Missing return statement in function");
}
// CREATE
final public
CLAUSE CreateClause() throws ParseException {Token t;
List patterns;
t = jj_consume_token(CREATE);
patterns = PatternList();
{if ("" != null) return astFactory.createClause( pos( t ), patterns );}
throw new IllegalStateException ("Missing return statement in function");
}
// SET
final public
SET_CLAUSE SetClause() throws ParseException {Token t;
SET_ITEM item;
List items = new ArrayList<>();
t = jj_consume_token(SET);
item = SetItem();
items.add( item );
label_11:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[28] = jj_gen;
break label_11;
}
jj_consume_token(250);
item = SetItem();
items.add( item );
}
{if ("" != null) return astFactory.setClause( pos( t ), items );}
throw new IllegalStateException ("Missing return statement in function");
}
final public SET_ITEM SetItem() throws ParseException {EXPRESSION e;
PROPERTY p;
VARIABLE v;
List> labels;
if (jj_2_8(2)) {
p = PropertyExpression();
jj_consume_token(EQ);
e = Expression();
{if ("" != null) return astFactory.setProperty( p, e );}
} else if (jj_2_9(2)) {
v = Variable();
jj_consume_token(EQ);
e = Expression();
{if ("" != null) return astFactory.setVariable( v, e );}
} else if (jj_2_10(2)) {
v = Variable();
jj_consume_token(251);
e = Expression();
{if ("" != null) return astFactory.addAndSetVariable( v, e );}
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
v = Variable();
labels = NodeLabels();
{if ("" != null) return astFactory.setLabels( v, labels );}
break;
}
default:
jj_la1[29] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
throw new IllegalStateException ("Missing return statement in function");
}
// REMOVE
final public
CLAUSE RemoveClause() throws ParseException {Token t;
REMOVE_ITEM item;
List items = new ArrayList<>();
t = jj_consume_token(REMOVE);
item = RemoveItem();
items.add( item );
label_12:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[30] = jj_gen;
break label_12;
}
jj_consume_token(250);
item = RemoveItem();
items.add( item );
}
{if ("" != null) return astFactory.removeClause( pos( t ), items );}
throw new IllegalStateException ("Missing return statement in function");
}
final public REMOVE_ITEM RemoveItem() throws ParseException {EXPRESSION e;
PROPERTY p;
VARIABLE v;
List> labels;
if (jj_2_11(2)) {
p = PropertyExpression();
{if ("" != null) return astFactory.removeProperty( p );}
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
v = Variable();
labels = NodeLabels();
{if ("" != null) return astFactory.removeLabels( v, labels );}
break;
}
default:
jj_la1[31] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
throw new IllegalStateException ("Missing return statement in function");
}
// DELETE
final public
CLAUSE DeleteClause() throws ParseException {Token detachT = null;
Token t;
boolean detach = false;
EXPRESSION e;
List list = new ArrayList<>();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DETACH:{
detachT = jj_consume_token(DETACH);
detach = true;
break;
}
default:
jj_la1[32] = jj_gen;
;
}
t = jj_consume_token(DELETE);
e = Expression();
list.add( e );
label_13:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[33] = jj_gen;
break label_13;
}
jj_consume_token(250);
e = Expression();
list.add( e );
}
{if ("" != null) return astFactory.deleteClause( pos( detachT != null ? detachT : t ), detach, list );}
throw new IllegalStateException ("Missing return statement in function");
}
// MATCH
final public
CLAUSE MatchClause() throws ParseException {Token optionalT = null;
Token t;
Token whereToken = null;
boolean optional = false;
List patterns;
List hints;
WHERE where = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case OPTIONAL:{
optionalT = jj_consume_token(OPTIONAL);
optional = true;
break;
}
default:
jj_la1[34] = jj_gen;
;
}
t = jj_consume_token(MATCH);
patterns = PatternList();
hints = Hints();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:{
where = WhereClause();
break;
}
default:
jj_la1[35] = jj_gen;
;
}
{if ("" != null) return astFactory.matchClause( pos( optionalT != null ? optionalT : t ), optional, patterns, pos( t.next ), hints, where );}
throw new IllegalStateException ("Missing return statement in function");
}
final public List Hints() throws ParseException {Token t;
boolean seek;
VARIABLE v;
Token labelOrRelType;
List joinVariables;
HINT hint;
List hints = null;
label_14:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case USING:{
break;
}
default:
jj_la1[36] = jj_gen;
break label_14;
}
t = jj_consume_token(USING);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case INDEX:{
jj_consume_token(INDEX);
hint = IndexHintBody(HintIndexType.ANY, pos( t ));
break;
}
case BTREE:{
jj_consume_token(BTREE);
jj_consume_token(INDEX);
hint = IndexHintBody(HintIndexType.BTREE, pos( t ));
break;
}
case TEXT:{
jj_consume_token(TEXT);
jj_consume_token(INDEX);
hint = IndexHintBody(HintIndexType.TEXT, pos( t ));
break;
}
case JOIN:{
jj_consume_token(JOIN);
jj_consume_token(ON);
joinVariables = VariableList1();
hint = astFactory.usingJoin( pos( t ), joinVariables );
break;
}
case SCAN:{
jj_consume_token(SCAN);
v = Variable();
labelOrRelType = LabelOrRelType();
hint = astFactory.usingScan( pos( t ), v, labelOrRelType.image );
break;
}
default:
jj_la1[37] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
if ( hints == null )
{
hints = new ArrayList<>();
}
hints.add( hint );
}
{if ("" != null) return hints;}
throw new IllegalStateException ("Missing return statement in function");
}
final public HINT IndexHintBody(HintIndexType indexType, POS p) throws ParseException {boolean seek = false;
VARIABLE v;
Token labelOrRelType;
List propNames;
if (jj_2_12(2)) {
jj_consume_token(SEEK);
seek = true;
} else {
;
}
v = Variable();
labelOrRelType = LabelOrRelType();
jj_consume_token(LPAREN);
propNames = SymbolicNameList1();
jj_consume_token(RPAREN);
{if ("" != null) return astFactory.usingIndexHint( p, v, labelOrRelType.image, propNames, seek, indexType);}
throw new IllegalStateException ("Missing return statement in function");
}
// MERGE
final public
CLAUSE MergeClause() throws ParseException {Token t;
Token onToken;
PATTERN p;
SET_CLAUSE c;
ArrayList clauses = new ArrayList<>();
ArrayList positions = new ArrayList<>();
ArrayList actionTypes = new ArrayList<>();
t = jj_consume_token(MERGE);
p = Pattern();
label_15:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ON:{
break;
}
default:
jj_la1[38] = jj_gen;
break label_15;
}
onToken = jj_consume_token(ON);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case MATCH:{
jj_consume_token(MATCH);
c = SetClause();
clauses.add( c ); actionTypes.add( ASTFactory.MergeActionType.OnMatch ); positions.add( pos( onToken ) );
break;
}
case CREATE:{
jj_consume_token(CREATE);
c = SetClause();
clauses.add( c ); actionTypes.add( ASTFactory.MergeActionType.OnCreate ); positions.add( pos( onToken ) );
break;
}
default:
jj_la1[39] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
{if ("" != null) return astFactory.mergeClause( pos( t ), p, clauses, actionTypes, positions );}
throw new IllegalStateException ("Missing return statement in function");
}
// UNWIND
final public
CLAUSE UnwindClause() throws ParseException {Token t;
EXPRESSION e;
VARIABLE v;
t = jj_consume_token(UNWIND);
e = Expression();
jj_consume_token(AS);
v = Variable();
{if ("" != null) return astFactory.unwindClause( pos( t ), e, v );}
throw new IllegalStateException ("Missing return statement in function");
}
// CALL
final public
CLAUSE CallClause() throws ParseException {Token t;
Token procedureNamePosition;
Token procedureResultPosition = null;
List namespace;
String name;
EXPRESSION e;
List arguments = null;
boolean yieldAll = false;
CALL_RESULT_ITEM x;
List items = null;
WHERE where = null;
t = jj_consume_token(CALL);
namespace = Namespace();
procedureNamePosition = token;
name = ProcedureName();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case LPAREN:{
jj_consume_token(LPAREN);
arguments = new ArrayList<>();
if (jj_2_13(2)) {
e = Expression();
arguments.add( e );
} else {
;
}
label_16:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[40] = jj_gen;
break label_16;
}
jj_consume_token(250);
e = Expression();
arguments.add( e );
}
jj_consume_token(RPAREN);
break;
}
default:
jj_la1[41] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case YIELD:{
procedureResultPosition = jj_consume_token(YIELD);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case TIMES:{
jj_consume_token(TIMES);
yieldAll = true;
break;
}
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
items = new ArrayList<>();
x = ProcedureResultItem();
items.add( x );
label_17:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[42] = jj_gen;
break label_17;
}
jj_consume_token(250);
x = ProcedureResultItem();
items.add( x );
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:{
where = WhereClause();
break;
}
default:
jj_la1[43] = jj_gen;
;
}
break;
}
default:
jj_la1[44] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
break;
}
default:
jj_la1[45] = jj_gen;
;
}
{if ("" != null) return astFactory.callClause( pos( t ),
pos( t.next ),
pos( procedureNamePosition.next ),
pos( procedureResultPosition ),
namespace,
name,
arguments,
yieldAll,
items,
where );}
throw new IllegalStateException ("Missing return statement in function");
}
final public String ProcedureName() throws ParseException {Token t;
t = SymbolicNameString();
{if ("" != null) return t.image;}
throw new IllegalStateException ("Missing return statement in function");
}
final public CALL_RESULT_ITEM ProcedureResultItem() throws ParseException {Token t;
VARIABLE v = null;
t = SymbolicNameString();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case AS:{
jj_consume_token(AS);
v = Variable();
break;
}
default:
jj_la1[46] = jj_gen;
;
}
{if ("" != null) return astFactory.callResultItem( pos( t ), t.image, v );}
throw new IllegalStateException ("Missing return statement in function");
}
// LOAD CSV
final public
CLAUSE LoadCSVClause() throws ParseException {Token t;
boolean headers = false;
EXPRESSION source;
VARIABLE v;
Token sep = null;
t = jj_consume_token(LOAD);
jj_consume_token(CSV);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WITH:{
jj_consume_token(WITH);
jj_consume_token(HEADERS);
headers = true;
break;
}
default:
jj_la1[47] = jj_gen;
;
}
jj_consume_token(FROM);
source = Expression();
jj_consume_token(AS);
v = Variable();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case FIELDTERMINATOR:{
jj_consume_token(FIELDTERMINATOR);
sep = StringToken();
break;
}
default:
jj_la1[48] = jj_gen;
;
}
{if ("" != null) return astFactory.loadCsvClause( pos( t ), headers, source, v, sep == null ? null : sep.image );}
throw new IllegalStateException ("Missing return statement in function");
}
// FOREACH
final public
CLAUSE ForeachClause() throws ParseException {Token t;
VARIABLE v;
EXPRESSION list;
CLAUSE c;
List clauses = new ArrayList<>();
t = jj_consume_token(FOREACH);
jj_consume_token(LPAREN);
v = Variable();
jj_consume_token(IN);
list = Expression();
jj_consume_token(BAR);
label_18:
while (true) {
c = Clause();
clauses.add( c );
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case CALL:
case CREATE:
case DELETE:
case DETACH:
case FOREACH:
case LOAD:
case MATCH:
case MERGE:
case OPTIONAL:
case REMOVE:
case RETURN:
case SET:
case UNWIND:
case USE:
case WITH:{
break;
}
default:
jj_la1[49] = jj_gen;
break label_18;
}
}
jj_consume_token(RPAREN);
{if ("" != null) return astFactory.foreachClause( pos( t ), v, list, clauses );}
throw new IllegalStateException ("Missing return statement in function");
}
final public CLAUSE SubqueryClause() throws ParseException {Token t;
QUERY q;
SUBQUERY_IN_TRANSACTIONS_PARAMETERS inTransactionsParams = null;
t = jj_consume_token(CALL);
jj_consume_token(LCURLY);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case USING:{
q = PeriodicCommitQuery();
break;
}
case CALL:
case CREATE:
case DELETE:
case DETACH:
case FOREACH:
case LOAD:
case MATCH:
case MERGE:
case OPTIONAL:
case REMOVE:
case RETURN:
case SET:
case UNWIND:
case USE:
case WITH:{
q = RegularQuery();
break;
}
default:
jj_la1[50] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
jj_consume_token(RCURLY);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case IN:{
inTransactionsParams = SubqueryInTransactionsParameters();
break;
}
default:
jj_la1[51] = jj_gen;
;
}
{if ("" != null) return astFactory.subqueryClause( pos( t ), q, inTransactionsParams );}
throw new IllegalStateException ("Missing return statement in function");
}
final public SUBQUERY_IN_TRANSACTIONS_PARAMETERS SubqueryInTransactionsParameters() throws ParseException {Token t;
EXPRESSION batchSize = null;
t = jj_consume_token(IN);
jj_consume_token(TRANSACTIONS);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case OF:{
jj_consume_token(OF);
batchSize = Expression();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ROW:{
jj_consume_token(ROW);
break;
}
case ROWS:{
jj_consume_token(ROWS);
break;
}
default:
jj_la1[52] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
break;
}
default:
jj_la1[53] = jj_gen;
;
}
{if ("" != null) return astFactory.subqueryInTransactionsParams( pos( t ), batchSize );}
throw new IllegalStateException ("Missing return statement in function");
}
// PATTERN
final public
List PatternList() throws ParseException {PATTERN p;
List patterns = new ArrayList<>();
p = Pattern();
patterns.add( p );
label_19:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[54] = jj_gen;
break label_19;
}
jj_consume_token(250);
p = Pattern();
patterns.add( p );
}
{if ("" != null) return patterns;}
throw new IllegalStateException ("Missing return statement in function");
}
final public PATTERN Pattern() throws ParseException {VARIABLE v;
PATTERN p;
if (jj_2_14(2)) {
v = Variable();
jj_consume_token(EQ);
p = AnonymousPattern();
{if ("" != null) return astFactory.namedPattern( v, p );}
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ALL_SHORTEST_PATH:
case LPAREN:
case SHORTEST_PATH:{
p = AnonymousPattern();
{if ("" != null) return p;}
break;
}
default:
jj_la1[55] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
throw new IllegalStateException ("Missing return statement in function");
}
final public PATTERN AnonymousPattern() throws ParseException {PATTERN p;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ALL_SHORTEST_PATH:
case SHORTEST_PATH:{
p = ShortestPathPattern();
break;
}
default:
jj_la1[56] = jj_gen;
if (jj_2_15(3)) {
p = EveryPathPattern();
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case LPAREN:{
jj_consume_token(LPAREN);
p = AnonymousPattern();
jj_consume_token(RPAREN);
break;
}
default:
jj_la1[57] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
}
{if ("" != null) return p;}
throw new IllegalStateException ("Missing return statement in function");
}
final public PATTERN ShortestPathPattern() throws ParseException {Token t;
PATTERN p;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case SHORTEST_PATH:{
t = jj_consume_token(SHORTEST_PATH);
jj_consume_token(LPAREN);
p = EveryPathPattern();
jj_consume_token(RPAREN);
{if ("" != null) return astFactory.shortestPathPattern( pos( t ), p );}
break;
}
case ALL_SHORTEST_PATH:{
t = jj_consume_token(ALL_SHORTEST_PATH);
jj_consume_token(LPAREN);
p = EveryPathPattern();
jj_consume_token(RPAREN);
{if ("" != null) return astFactory.allShortestPathsPattern( pos( t ), p );}
break;
}
default:
jj_la1[58] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
throw new IllegalStateException ("Missing return statement in function");
}
final public PATTERN EveryPathPattern() throws ParseException {NODE_PATTERN n;
REL_PATTERN r;
List relationships = new ArrayList<>();
List nodes = new ArrayList<>();
n = NodePattern();
nodes.add( n );
label_20:
while (true) {
if (jj_2_16(2)) {
} else {
break label_20;
}
r = RelationshipPattern();
relationships.add( r );
n = NodePattern();
nodes.add( n );
}
{if ("" != null) return astFactory.everyPathPattern( nodes, relationships );}
throw new IllegalStateException ("Missing return statement in function");
}
final public PATTERN EveryPathPatternNonEmpty() throws ParseException {NODE_PATTERN n;
REL_PATTERN r;
List relationships = new ArrayList<>();
List nodes = new ArrayList<>();
n = NodePattern();
nodes.add( n );
label_21:
while (true) {
r = RelationshipPattern();
relationships.add( r );
n = NodePattern();
nodes.add( n );
if (jj_2_17(2)) {
} else {
break label_21;
}
}
{if ("" != null) return astFactory.everyPathPattern( nodes, relationships );}
throw new IllegalStateException ("Missing return statement in function");
}
final public NODE_PATTERN NodePattern() throws ParseException {Token t;
VARIABLE v = null;
List> labels = new ArrayList<>();
EXPRESSION properties = null;
EXPRESSION predicate = null;
t = jj_consume_token(LPAREN);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
v = Variable();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 252:{
labels = NodeLabels();
break;
}
default:
jj_la1[59] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DOLLAR:
case LCURLY:{
properties = Properties();
break;
}
default:
jj_la1[60] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:{
jj_consume_token(WHERE);
predicate = Expression();
break;
}
default:
jj_la1[61] = jj_gen;
;
}
break;
}
default:
jj_la1[64] = jj_gen;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 252:{
labels = NodeLabels();
break;
}
default:
jj_la1[62] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DOLLAR:
case LCURLY:{
properties = Properties();
break;
}
default:
jj_la1[63] = jj_gen;
;
}
}
jj_consume_token(RPAREN);
{if ("" != null) return astFactory.nodePattern( pos( t ), v, labels, properties, predicate );}
throw new IllegalStateException ("Missing return statement in function");
}
final public List> NodeLabels() throws ParseException {Token label;
List> labels = new ArrayList<>();
label_22:
while (true) {
label = LabelOrRelType();
labels.add( new StringPos<>( label.image, pos( label ) ) );
if (jj_2_18(2)) {
} else {
break label_22;
}
}
{if ("" != null) return labels;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION HasLabels(EXPRESSION subject) throws ParseException {List> labels;
labels = NodeLabels();
{if ("" != null) return astFactory.hasLabelsOrTypes( subject, labels );}
throw new IllegalStateException ("Missing return statement in function");
}
final public Token LabelOrRelType() throws ParseException {Token t;
jj_consume_token(252);
t = SymbolicNameString();
{if ("" != null) return t;}
throw new IllegalStateException ("Missing return statement in function");
}
final public List> LabelOrRelTypes() throws ParseException {List> labels = new ArrayList<>();
StringPos label;
jj_consume_token(252);
SymbolicNameString();
labels.add( new StringPos( token.image, pos(token) ) );
label_23:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case BAR:{
break;
}
default:
jj_la1[65] = jj_gen;
break label_23;
}
jj_consume_token(BAR);
SymbolicNameString();
labels.add( new StringPos( token.image, pos(token) ) );
}
{if ("" != null) return labels;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION Properties() throws ParseException {EXPRESSION e;
if (jj_2_19(3)) {
e = MapLiteral();
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DOLLAR:{
e = Parameter(ParameterType.ANY);
break;
}
case LCURLY:{
e = OldParameter();
break;
}
default:
jj_la1[66] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
{if ("" != null) return e;}
throw new IllegalStateException ("Missing return statement in function");
}
final public REL_PATTERN RelationshipPattern() throws ParseException {Token firstToken = token.next;
Token t;
boolean left = false;
boolean right = false;
VARIABLE v = null;
List> relTypes = new ArrayList<>();
boolean legacyTypeSeparator = false;
PATH_LENGTH pathLength = null;
EXPRESSION properties = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case LT:
case ARROW_LEFT_HEAD:{
LeftArrow();
left = true;
break;
}
default:
jj_la1[67] = jj_gen;
;
}
ArrowLine();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case LBRACKET:{
jj_consume_token(LBRACKET);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
v = Variable();
break;
}
default:
jj_la1[68] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 252:{
jj_consume_token(252);
t = SymbolicNameString();
relTypes = new ArrayList<>();
relTypes.add(new StringPos<>( t.image, pos( t ) ) );
label_24:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case BAR:{
break;
}
default:
jj_la1[69] = jj_gen;
break label_24;
}
jj_consume_token(BAR);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 252:{
jj_consume_token(252);
legacyTypeSeparator = true;
break;
}
default:
jj_la1[70] = jj_gen;
;
}
t = SymbolicNameString();
relTypes.add( new StringPos<>( t.image, pos( t ) ) );
}
break;
}
default:
jj_la1[71] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case TIMES:{
pathLength = PathLength();
break;
}
default:
jj_la1[72] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DOLLAR:
case LCURLY:{
properties = Properties();
break;
}
default:
jj_la1[73] = jj_gen;
;
}
jj_consume_token(RBRACKET);
break;
}
default:
jj_la1[74] = jj_gen;
;
}
ArrowLine();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case GT:
case ARROW_RIGHT_HEAD:{
RightArrow();
right = true;
break;
}
default:
jj_la1[75] = jj_gen;
;
}
{if ("" != null) return astFactory.relationshipPattern( pos( firstToken ), left, right, v, relTypes, pathLength, properties, legacyTypeSeparator );}
throw new IllegalStateException ("Missing return statement in function");
}
final public void LeftArrow() throws ParseException {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case LT:{
jj_consume_token(LT);
break;
}
case ARROW_LEFT_HEAD:{
jj_consume_token(ARROW_LEFT_HEAD);
break;
}
default:
jj_la1[76] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
final public void ArrowLine() throws ParseException {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ARROW_LINE:{
jj_consume_token(ARROW_LINE);
break;
}
case MINUS:{
jj_consume_token(MINUS);
break;
}
default:
jj_la1[77] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
final public void RightArrow() throws ParseException {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case GT:{
jj_consume_token(GT);
break;
}
case ARROW_RIGHT_HEAD:{
jj_consume_token(ARROW_RIGHT_HEAD);
break;
}
default:
jj_la1[78] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
final public PATH_LENGTH PathLength() throws ParseException {Token t;
PATH_LENGTH p = null;
t = jj_consume_token(TIMES);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case UNSIGNED_DECIMAL_INTEGER:
case DOTDOT:{
p = PathLengthLiteral(t);
break;
}
default:
jj_la1[79] = jj_gen;
;
}
{if ("" != null) return p == null ? astFactory.pathLength( pos( t ), null, null, null, null ) : p;}
throw new IllegalStateException ("Missing return statement in function");
}
final public PATH_LENGTH PathLengthLiteral(Token t) throws ParseException {Token v1 = null;
Token v2 = null;
if (jj_2_20(2)) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case UNSIGNED_DECIMAL_INTEGER:{
v1 = jj_consume_token(UNSIGNED_DECIMAL_INTEGER);
break;
}
default:
jj_la1[80] = jj_gen;
;
}
t = jj_consume_token(DOTDOT);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case UNSIGNED_DECIMAL_INTEGER:{
v2 = jj_consume_token(UNSIGNED_DECIMAL_INTEGER);
break;
}
default:
jj_la1[81] = jj_gen;
;
}
{if ("" != null) return astFactory.pathLength( pos( t ), v1 == null ? null : pos ( v1 ), v2 == null ? null : pos ( v2 ), v1 == null ? "" : v1.image, v2 == null ? "" : v2.image );}
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case UNSIGNED_DECIMAL_INTEGER:{
v1 = jj_consume_token(UNSIGNED_DECIMAL_INTEGER);
String v = v1 == null ? "" : v1.image;
{if ("" != null) return astFactory.pathLength( pos( t ), v1 == null ? null : pos ( v1 ), v1 == null ? null : pos ( v1 ), v, v);}
break;
}
default:
jj_la1[82] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
throw new IllegalStateException ("Missing return statement in function");
}
// EXPRESSIONS
final public
EXPRESSION Expression() throws ParseException {EXPRESSION e;
e = Expression12();
{if ("" != null) return e;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION Expression12() throws ParseException {Token t;
EXPRESSION e;
EXPRESSION temp;
e = Expression11();
label_25:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case OR:{
break;
}
default:
jj_la1[83] = jj_gen;
break label_25;
}
t = jj_consume_token(OR);
temp = Expression11();
e = astFactory.or( pos( t ), e, temp );
}
{if ("" != null) return e;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION Expression11() throws ParseException {Token t;
EXPRESSION e;
EXPRESSION temp;
e = Expression10();
label_26:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case XOR:{
break;
}
default:
jj_la1[84] = jj_gen;
break label_26;
}
t = jj_consume_token(XOR);
temp = Expression10();
e = astFactory.xor( pos( t ), e, temp );
}
{if ("" != null) return e;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION Expression10() throws ParseException {Token t;
EXPRESSION e;
EXPRESSION temp;
e = Expression9();
label_27:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case AND:{
break;
}
default:
jj_la1[85] = jj_gen;
break label_27;
}
t = jj_consume_token(AND);
temp = Expression9();
e = astFactory.and( pos( t ), e, temp );
}
{if ("" != null) return e;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION Expression9() throws ParseException {Token t;
EXPRESSION e;
if (jj_2_21(3)) {
t = jj_consume_token(NOT);
e = Expression9();
e = astFactory.not( pos( t ), e );
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DECIMAL_DOUBLE:
case UNSIGNED_DECIMAL_INTEGER:
case UNSIGNED_HEX_INTEGER:
case UNSIGNED_OCTAL_INTEGER:
case STRING_LITERAL1:
case STRING_LITERAL2:
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LBRACKET:
case LCURLY:
case LIMITROWS:
case LOAD:
case LOOKUP:
case LPAREN:
case MANAGEMENT:
case MATCH:
case MERGE:
case MINUS:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case PLUS:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
e = Expression8();
break;
}
default:
jj_la1[86] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
{if ("" != null) return e;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION Expression8() throws ParseException {Token t;
EXPRESSION e;
EXPRESSION lhs;
EXPRESSION rhs;
List expressions = new ArrayList();
e = Expression7();
lhs = e;
label_28:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case EQ:
case GE:
case GT:
case LE:
case LT:
case NEQ:
case NEQ2:{
break;
}
default:
jj_la1[87] = jj_gen;
break label_28;
}
if (jj_2_22(2)) {
t = jj_consume_token(EQ);
rhs = Expression7();
expressions.add( astFactory.eq( pos( t ), lhs, rhs)); lhs = rhs;
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case NEQ:{
t = jj_consume_token(NEQ);
rhs = Expression7();
expressions.add( astFactory.neq( pos( t ), lhs, rhs ) ); lhs = rhs;
break;
}
case NEQ2:{
t = jj_consume_token(NEQ2);
rhs = Expression7();
expressions.add( astFactory.neq2( pos( t ), lhs, rhs ) ); lhs = rhs;
break;
}
case LE:{
t = jj_consume_token(LE);
rhs = Expression7();
expressions.add( astFactory.lte( pos( t ), lhs, rhs ) ); lhs = rhs;
break;
}
case GE:{
t = jj_consume_token(GE);
rhs = Expression7();
expressions.add( astFactory.gte( pos( t ), lhs, rhs ) ); lhs = rhs;
break;
}
case LT:{
t = jj_consume_token(LT);
rhs = Expression7();
expressions.add( astFactory.lt( pos( t ), lhs, rhs ) ); lhs = rhs;
break;
}
case GT:{
t = jj_consume_token(GT);
rhs = Expression7();
expressions.add( astFactory.gt( pos( t ), lhs, rhs ) ); lhs = rhs;
break;
}
default:
jj_la1[88] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
}
if ( expressions.isEmpty() )
{
{if ("" != null) return e;}
}
else if ( expressions.size() == 1 )
{
{if ("" != null) return expressions.get( 0 );}
}
else
{
{if ("" != null) return astFactory.ands( expressions );}
}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION Expression7() throws ParseException {EXPRESSION e;
e = Expression6();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case CONTAINS:
case ENDS:
case IN:
case IS:
case REGEQ:
case STARTS:{
e = ComparisonExpression6(e);
break;
}
default:
jj_la1[89] = jj_gen;
;
}
{if ("" != null) return e;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION ComparisonExpression6(EXPRESSION lhs) throws ParseException {Token t;
EXPRESSION rhs;
if (jj_2_23(2)) {
t = jj_consume_token(REGEQ);
rhs = Expression6();
{if ("" != null) return astFactory.regeq( pos( t ), lhs, rhs );}
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case STARTS:{
t = jj_consume_token(STARTS);
jj_consume_token(WITH);
rhs = Expression6();
{if ("" != null) return astFactory.startsWith( pos( t ), lhs, rhs );}
break;
}
case ENDS:{
t = jj_consume_token(ENDS);
jj_consume_token(WITH);
rhs = Expression6();
{if ("" != null) return astFactory.endsWith( pos( t ), lhs, rhs );}
break;
}
case CONTAINS:{
t = jj_consume_token(CONTAINS);
rhs = Expression6();
{if ("" != null) return astFactory.contains( pos( t ), lhs, rhs );}
break;
}
case IN:{
t = jj_consume_token(IN);
rhs = Expression6();
{if ("" != null) return astFactory.in( pos( t ), lhs, rhs );}
break;
}
case IS:{
t = jj_consume_token(IS);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case NULL:{
jj_consume_token(NULL);
{if ("" != null) return astFactory.isNull( pos( t ), lhs );}
break;
}
case NOT:{
jj_consume_token(NOT);
jj_consume_token(NULL);
{if ("" != null) return astFactory.isNotNull( pos( t ), lhs );}
break;
}
default:
jj_la1[90] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
break;
}
default:
jj_la1[91] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION Expression6() throws ParseException {Token t;
EXPRESSION lhs;
EXPRESSION rhs;
lhs = Expression5();
label_29:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case MINUS:
case PLUS:{
break;
}
default:
jj_la1[92] = jj_gen;
break label_29;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case PLUS:{
t = jj_consume_token(PLUS);
rhs = Expression5();
lhs = astFactory.plus( pos( t ), lhs, rhs);
break;
}
case MINUS:{
t = jj_consume_token(MINUS);
rhs = Expression5();
lhs = astFactory.minus( pos( t ), lhs, rhs);
break;
}
default:
jj_la1[93] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
{if ("" != null) return lhs;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION Expression5() throws ParseException {Token t;
EXPRESSION lhs;
EXPRESSION rhs;
lhs = Expression4();
label_30:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DIVIDE:
case MODULO:
case TIMES:{
break;
}
default:
jj_la1[94] = jj_gen;
break label_30;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case TIMES:{
t = jj_consume_token(TIMES);
rhs = Expression4();
lhs = astFactory.multiply( pos( t ), lhs, rhs );
break;
}
case DIVIDE:{
t = jj_consume_token(DIVIDE);
rhs = Expression4();
lhs = astFactory.divide( pos( t ), lhs, rhs );
break;
}
case MODULO:{
t = jj_consume_token(MODULO);
rhs = Expression4();
lhs = astFactory.modulo( pos( t ), lhs, rhs );
break;
}
default:
jj_la1[95] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
{if ("" != null) return lhs;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION Expression4() throws ParseException {Token t;
EXPRESSION lhs;
EXPRESSION rhs;
lhs = Expression3();
label_31:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case POW:{
break;
}
default:
jj_la1[96] = jj_gen;
break label_31;
}
t = jj_consume_token(POW);
rhs = Expression3();
lhs = astFactory.pow( pos( t ), lhs, rhs );
}
{if ("" != null) return lhs;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION Expression3() throws ParseException {Token t;
EXPRESSION e;
if (jj_2_24(3)) {
e = Expression2();
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case PLUS:{
t = jj_consume_token(PLUS);
e = Expression2();
e = astFactory.unaryPlus( pos( t ), e );
break;
}
case MINUS:{
t = jj_consume_token(MINUS);
e = Expression2();
e = astFactory.unaryMinus( pos( t ), e );
break;
}
default:
jj_la1[97] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
{if ("" != null) return e;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION Expression2() throws ParseException {EXPRESSION e;
e = Expression1();
label_32:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DOT:
case LBRACKET:
case 252:{
break;
}
default:
jj_la1[98] = jj_gen;
break label_32;
}
e = PostFix1(e);
}
{if ("" != null) return e;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION PostFix1(EXPRESSION subject) throws ParseException {Token t;
EXPRESSION e1 = null;
EXPRESSION e2 = null;
EXPRESSION ret;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DOT:{
ret = Property(subject);
break;
}
case 252:{
ret = HasLabels(subject);
break;
}
default:
jj_la1[101] = jj_gen;
if (jj_2_25(2147483647)) {
jj_consume_token(LBRACKET);
e1 = Expression();
jj_consume_token(RBRACKET);
ret=astFactory.listLookup( subject, e1 );
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case LBRACKET:{
t = jj_consume_token(LBRACKET);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DECIMAL_DOUBLE:
case UNSIGNED_DECIMAL_INTEGER:
case UNSIGNED_HEX_INTEGER:
case UNSIGNED_OCTAL_INTEGER:
case STRING_LITERAL1:
case STRING_LITERAL2:
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LBRACKET:
case LCURLY:
case LIMITROWS:
case LOAD:
case LOOKUP:
case LPAREN:
case MANAGEMENT:
case MATCH:
case MERGE:
case MINUS:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case PLUS:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
e1 = Expression();
break;
}
default:
jj_la1[99] = jj_gen;
;
}
jj_consume_token(DOTDOT);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DECIMAL_DOUBLE:
case UNSIGNED_DECIMAL_INTEGER:
case UNSIGNED_HEX_INTEGER:
case UNSIGNED_OCTAL_INTEGER:
case STRING_LITERAL1:
case STRING_LITERAL2:
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LBRACKET:
case LCURLY:
case LIMITROWS:
case LOAD:
case LOOKUP:
case LPAREN:
case MANAGEMENT:
case MATCH:
case MERGE:
case MINUS:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case PLUS:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
e2 = Expression();
break;
}
default:
jj_la1[100] = jj_gen;
;
}
jj_consume_token(RBRACKET);
ret=astFactory.listSlice( pos( t ), subject, e1, e2 );
break;
}
default:
jj_la1[102] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
}
{if ("" != null) return ret;}
throw new IllegalStateException ("Missing return statement in function");
}
final public PROPERTY Property(EXPRESSION subject) throws ParseException {EXPRESSION e;
StringPos propKeyName;
jj_consume_token(DOT);
propKeyName = PropertyKeyName();
{if ("" != null) return astFactory.property( subject, propKeyName );}
throw new IllegalStateException ("Missing return statement in function");
}
final public PROPERTY PropertyExpression() throws ParseException {EXPRESSION subject;
PROPERTY p;
subject = Expression1();
label_33:
while (true) {
p = Property(subject);
subject = p;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DOT:{
break;
}
default:
jj_la1[103] = jj_gen;
break label_33;
}
}
{if ("" != null) return p;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION Expression1() throws ParseException {EXPRESSION e = null;
Token t;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DECIMAL_DOUBLE:
case UNSIGNED_DECIMAL_INTEGER:
case UNSIGNED_HEX_INTEGER:
case UNSIGNED_OCTAL_INTEGER:
case MINUS:{
e = NumberLiteral();
break;
}
case STRING_LITERAL1:
case STRING_LITERAL2:{
e = StringLiteral();
break;
}
case DOLLAR:{
e = Parameter(ParameterType.ANY);
break;
}
case TRUE:{
t = jj_consume_token(TRUE);
e = astFactory.newTrueLiteral( pos( t ) );
break;
}
case FALSE:{
t = jj_consume_token(FALSE);
e = astFactory.newFalseLiteral( pos( t ) );
break;
}
default:
jj_la1[104] = jj_gen;
if (jj_2_26(2)) {
t = jj_consume_token(NULL);
e = astFactory.newNullLiteral( pos( t ) );
} else if (jj_2_27(3)) {
e = CaseExpression();
} else if (jj_2_28(3)) {
t = jj_consume_token(COUNT);
jj_consume_token(LPAREN);
jj_consume_token(TIMES);
jj_consume_token(RPAREN);
e = astFactory.newCountStar( pos( t ) );
} else if (jj_2_29(3)) {
e = MapLiteral();
} else if (jj_2_30(3)) {
e = ExistsSubQuery();
} else if (jj_2_31(2)) {
e = MapProjection();
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case LCURLY:{
e = OldParameter();
break;
}
default:
jj_la1[105] = jj_gen;
if (jj_2_32(2147483647)) {
e = ListComprehension();
} else if (jj_2_33(2147483647)) {
e = PatternComprehension();
} else if (jj_2_34(3)) {
e = ListLiteral();
} else if (jj_2_35(3)) {
e = FilterExpression();
} else if (jj_2_36(3)) {
e = ExtractExpression();
} else if (jj_2_37(3)) {
e = ReduceExpression();
} else if (jj_2_38(3)) {
e = AllExpression();
} else if (jj_2_39(3)) {
e = AnyExpression();
} else if (jj_2_40(3)) {
e = NoneExpression();
} else if (jj_2_41(3)) {
e = SingleExpression();
} else if (jj_2_42(2147483647)) {
e = PatternExpression();
} else if (jj_2_43(2)) {
e = ShortestPathExpression();
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case LPAREN:{
jj_consume_token(LPAREN);
e = Expression();
jj_consume_token(RPAREN);
break;
}
default:
jj_la1[106] = jj_gen;
if (jj_2_44(2147483647)) {
e = FunctionInvocation();
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
e = Variable();
break;
}
default:
jj_la1[107] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
}
}
}
}
}
{if ("" != null) return e;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION CaseExpression() throws ParseException {Token t;
EXPRESSION caseExpr = null;
EXPRESSION e;
List when = new ArrayList<>();
List then = new ArrayList<>();
EXPRESSION elseCase = null;
t = jj_consume_token(CASE);
if (jj_2_45(2147483647)) {
caseExpr = Expression();
jj_consume_token(WHEN);
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHEN:{
jj_consume_token(WHEN);
break;
}
default:
jj_la1[108] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
e = Expression();
when.add( e );
jj_consume_token(THEN);
e = Expression();
then.add( e );
label_34:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHEN:{
break;
}
default:
jj_la1[109] = jj_gen;
break label_34;
}
jj_consume_token(WHEN);
e = Expression();
when.add( e );
jj_consume_token(THEN);
e = Expression();
then.add( e );
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ELSE:{
jj_consume_token(ELSE);
elseCase = Expression();
break;
}
default:
jj_la1[110] = jj_gen;
;
}
jj_consume_token(END);
{if ("" != null) return astFactory.caseExpression( pos( t ), caseExpr, when, then, elseCase);}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION ListComprehension() throws ParseException {Token t;
VARIABLE v;
EXPRESSION e;
EXPRESSION where = null;
EXPRESSION projection = null;
t = jj_consume_token(LBRACKET);
v = Variable();
jj_consume_token(IN);
e = Expression();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:{
jj_consume_token(WHERE);
where = Expression();
break;
}
default:
jj_la1[111] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case BAR:{
jj_consume_token(BAR);
projection = Expression();
break;
}
default:
jj_la1[112] = jj_gen;
;
}
jj_consume_token(RBRACKET);
{if ("" != null) return astFactory.listComprehension( pos( t ), v, e, where, projection );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION PatternComprehension() throws ParseException {Token t;
Token relationshipPatternPosition;
VARIABLE v = null;
PATTERN p;
EXPRESSION where = null;
EXPRESSION projection = null;
t = jj_consume_token(LBRACKET);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
v = Variable();
jj_consume_token(EQ);
break;
}
default:
jj_la1[113] = jj_gen;
;
}
relationshipPatternPosition = token;
p = EveryPathPatternNonEmpty();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:{
jj_consume_token(WHERE);
where = Expression();
break;
}
default:
jj_la1[114] = jj_gen;
;
}
jj_consume_token(BAR);
projection = Expression();
jj_consume_token(RBRACKET);
{if ("" != null) return astFactory.patternComprehension( pos( t ), pos( relationshipPatternPosition.next ), v, p, where, projection );}
throw new IllegalStateException ("Missing return statement in function");
}
final public void PatternComprehensionPrefix() throws ParseException {
jj_consume_token(LBRACKET);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
Variable();
jj_consume_token(EQ);
break;
}
default:
jj_la1[115] = jj_gen;
;
}
EveryPathPattern();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:{
jj_consume_token(WHERE);
break;
}
case BAR:{
jj_consume_token(BAR);
break;
}
default:
jj_la1[116] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
final public EXPRESSION FilterExpression() throws ParseException {Token t;
VARIABLE v;
EXPRESSION e;
EXPRESSION where = null;
t = jj_consume_token(FILTER);
jj_consume_token(LPAREN);
v = Variable();
jj_consume_token(IN);
e = Expression();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:{
jj_consume_token(WHERE);
where = Expression();
break;
}
default:
jj_la1[117] = jj_gen;
;
}
jj_consume_token(RPAREN);
{if ("" != null) return astFactory.filterExpression( pos( t ), v, e, where );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION ExtractExpression() throws ParseException {Token t;
VARIABLE v;
EXPRESSION e;
EXPRESSION where = null;
EXPRESSION projection = null;
t = jj_consume_token(EXTRACT);
jj_consume_token(LPAREN);
v = Variable();
jj_consume_token(IN);
e = Expression();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:{
jj_consume_token(WHERE);
where = Expression();
break;
}
default:
jj_la1[118] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case BAR:{
jj_consume_token(BAR);
projection = Expression();
break;
}
default:
jj_la1[119] = jj_gen;
;
}
jj_consume_token(RPAREN);
{if ("" != null) return astFactory.extractExpression( pos( t ), v, e, where, projection );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION ReduceExpression() throws ParseException {Token t;
VARIABLE acc;
EXPRESSION accExpr;
VARIABLE v;
EXPRESSION vExpr;
EXPRESSION innerExpr;
t = jj_consume_token(REDUCE);
jj_consume_token(LPAREN);
acc = Variable();
jj_consume_token(EQ);
accExpr = Expression();
jj_consume_token(250);
v = Variable();
jj_consume_token(IN);
vExpr = Expression();
jj_consume_token(BAR);
innerExpr = Expression();
jj_consume_token(RPAREN);
{if ("" != null) return astFactory.reduceExpression( pos( t ), acc, accExpr, v, vExpr, innerExpr );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION AllExpression() throws ParseException {Token t;
VARIABLE v;
EXPRESSION e;
EXPRESSION where = null;
t = jj_consume_token(ALL);
jj_consume_token(LPAREN);
v = Variable();
jj_consume_token(IN);
e = Expression();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:{
jj_consume_token(WHERE);
where = Expression();
break;
}
default:
jj_la1[120] = jj_gen;
;
}
jj_consume_token(RPAREN);
{if ("" != null) return astFactory.allExpression( pos( t ), v, e, where );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION AnyExpression() throws ParseException {Token t;
VARIABLE v;
EXPRESSION e;
EXPRESSION where = null;
t = jj_consume_token(ANY);
jj_consume_token(LPAREN);
v = Variable();
jj_consume_token(IN);
e = Expression();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:{
jj_consume_token(WHERE);
where = Expression();
break;
}
default:
jj_la1[121] = jj_gen;
;
}
jj_consume_token(RPAREN);
{if ("" != null) return astFactory.anyExpression( pos( t ), v, e, where );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION NoneExpression() throws ParseException {Token t;
VARIABLE v;
EXPRESSION e;
EXPRESSION where = null;
t = jj_consume_token(NONE);
jj_consume_token(LPAREN);
v = Variable();
jj_consume_token(IN);
e = Expression();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:{
jj_consume_token(WHERE);
where = Expression();
break;
}
default:
jj_la1[122] = jj_gen;
;
}
jj_consume_token(RPAREN);
{if ("" != null) return astFactory.noneExpression( pos( t ), v, e, where );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION SingleExpression() throws ParseException {Token t;
VARIABLE v;
EXPRESSION e;
EXPRESSION where = null;
t = jj_consume_token(SINGLE);
jj_consume_token(LPAREN);
v = Variable();
jj_consume_token(IN);
e = Expression();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:{
jj_consume_token(WHERE);
where = Expression();
break;
}
default:
jj_la1[123] = jj_gen;
;
}
jj_consume_token(RPAREN);
{if ("" != null) return astFactory.singleExpression( pos( t ), v, e, where );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION PatternExpression() throws ParseException {PATTERN p;
Token t;
t = token;
p = EveryPathPatternNonEmpty();
{if ("" != null) return astFactory.patternExpression( pos( t.next ), p );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION ShortestPathExpression() throws ParseException {PATTERN p;
Token t;
t = token;
p = ShortestPathPattern();
{if ("" != null) return astFactory.patternExpression( pos( t.next ), p );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION MapProjection() throws ParseException {Token t;
VARIABLE v;
MAP_PROJECTION_ITEM x;
List items = new ArrayList<>();
v = Variable();
t = jj_consume_token(LCURLY);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DOT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
x = MapProjectionItem();
items.add( x );
break;
}
default:
jj_la1[124] = jj_gen;
;
}
label_35:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[125] = jj_gen;
break label_35;
}
jj_consume_token(250);
x = MapProjectionItem();
items.add( x );
}
jj_consume_token(RCURLY);
{if ("" != null) return astFactory.mapProjection( pos( t ), v, items );}
throw new IllegalStateException ("Missing return statement in function");
}
final public MAP_PROJECTION_ITEM MapProjectionItem() throws ParseException {Token t;
StringPos p;
EXPRESSION e;
VARIABLE v;
if (jj_2_46(2)) {
p = PropertyKeyName();
jj_consume_token(252);
e = Expression();
{if ("" != null) return astFactory.mapProjectionLiteralEntry( p, e );}
} else if (jj_2_47(2)) {
jj_consume_token(DOT);
p = PropertyKeyName();
{if ("" != null) return astFactory.mapProjectionProperty( p );}
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
v = Variable();
{if ("" != null) return astFactory.mapProjectionVariable( v );}
break;
}
case DOT:{
jj_consume_token(DOT);
t = jj_consume_token(TIMES);
{if ("" != null) return astFactory.mapProjectionAll( pos( t ) );}
break;
}
default:
jj_la1[126] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION ExistsSubQuery() throws ParseException {Token t;
List patterns;
EXPRESSION where = null;
t = jj_consume_token(EXISTS);
jj_consume_token(LCURLY);
if (jj_2_48(2)) {
jj_consume_token(MATCH);
} else {
;
}
patterns = PatternList();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:{
jj_consume_token(WHERE);
where = Expression();
break;
}
default:
jj_la1[127] = jj_gen;
;
}
jj_consume_token(RCURLY);
{if ("" != null) return astFactory.existsSubQuery( pos( t ), patterns, where );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION Literal() throws ParseException {Token t;
EXPRESSION e;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DECIMAL_DOUBLE:
case UNSIGNED_DECIMAL_INTEGER:
case UNSIGNED_HEX_INTEGER:
case UNSIGNED_OCTAL_INTEGER:
case MINUS:{
e = NumberLiteral();
break;
}
case STRING_LITERAL1:
case STRING_LITERAL2:{
e = StringLiteral();
break;
}
case DOLLAR:{
e = Parameter(ParameterType.ANY);
break;
}
case TRUE:{
t = jj_consume_token(TRUE);
e = astFactory.newTrueLiteral( pos( t ) );
break;
}
case FALSE:{
t = jj_consume_token(FALSE);
e = astFactory.newFalseLiteral( pos( t ) );
break;
}
default:
jj_la1[128] = jj_gen;
if (jj_2_49(2)) {
t = jj_consume_token(NULL);
e = astFactory.newNullLiteral( pos( t ) );
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case LBRACKET:{
e = ListLiteralOfLiterals();
break;
}
case LCURLY:{
e = MapLiteralOfLiterals();
break;
}
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
e = FunctionInvocation();
break;
}
default:
jj_la1[129] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
}
{if ("" != null) return e;}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION ListLiteralOfLiterals() throws ParseException {Token t;
EXPRESSION e;
List list = new ArrayList<>();
t = jj_consume_token(LBRACKET);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DECIMAL_DOUBLE:
case UNSIGNED_DECIMAL_INTEGER:
case UNSIGNED_HEX_INTEGER:
case UNSIGNED_OCTAL_INTEGER:
case STRING_LITERAL1:
case STRING_LITERAL2:
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LBRACKET:
case LCURLY:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case MINUS:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
e = Literal();
list.add(e);
break;
}
default:
jj_la1[130] = jj_gen;
;
}
label_36:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[131] = jj_gen;
break label_36;
}
jj_consume_token(250);
e = Literal();
list.add( e );
}
jj_consume_token(RBRACKET);
{if ("" != null) return astFactory.listLiteral( pos( t ), list );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION MapLiteralOfLiterals() throws ParseException {Token t;
StringPos key;
EXPRESSION value;
List> keys = new ArrayList<>();
List values = new ArrayList<>();
t = jj_consume_token(LCURLY);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
key = PropertyKeyName();
jj_consume_token(252);
value = Literal();
keys.add( key ); values.add( value );
break;
}
default:
jj_la1[132] = jj_gen;
;
}
label_37:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[133] = jj_gen;
break label_37;
}
jj_consume_token(250);
key = PropertyKeyName();
jj_consume_token(252);
value = Literal();
keys.add( key ); values.add( value );
}
jj_consume_token(RCURLY);
{if ("" != null) return astFactory.mapLiteral( pos( t ), keys, values );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION StringLiteral() throws ParseException {Token t;
t = StringToken();
{if ("" != null) return astFactory.newString( pos( t ), token.image );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION NumberLiteral() throws ParseException {Token sign = null;
Token t;
boolean negated = false;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case MINUS:{
sign = jj_consume_token(MINUS);
negated = true;
break;
}
default:
jj_la1[134] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DECIMAL_DOUBLE:{
t = jj_consume_token(DECIMAL_DOUBLE);
{if ("" != null) return astFactory.newDouble( pos( sign != null ? sign : t ) , sign != null ? sign.image + token.image : token.image );}
break;
}
case UNSIGNED_DECIMAL_INTEGER:{
t = jj_consume_token(UNSIGNED_DECIMAL_INTEGER);
{if ("" != null) return astFactory.newDecimalInteger( pos( sign != null ? sign : t ), token.image, negated );}
break;
}
case UNSIGNED_HEX_INTEGER:{
t = jj_consume_token(UNSIGNED_HEX_INTEGER);
{if ("" != null) return astFactory.newHexInteger( pos( sign != null ? sign : t ), token.image, negated );}
break;
}
case UNSIGNED_OCTAL_INTEGER:{
t = jj_consume_token(UNSIGNED_OCTAL_INTEGER);
{if ("" != null) return astFactory.newOctalInteger( pos( sign != null ? sign : t ), token.image, negated );}
break;
}
default:
jj_la1[135] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION SignedIntegerLiteral() throws ParseException {Token sign = null;
Token number;
boolean negated = false;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case MINUS:{
sign = jj_consume_token(MINUS);
negated = true;
break;
}
default:
jj_la1[136] = jj_gen;
;
}
number = jj_consume_token(UNSIGNED_DECIMAL_INTEGER);
{if ("" != null) return astFactory.newDecimalInteger( pos( sign != null ? sign : number ), token.image, negated );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION ListLiteral() throws ParseException {Token t;
EXPRESSION e;
List list = new ArrayList<>();
t = jj_consume_token(LBRACKET);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DECIMAL_DOUBLE:
case UNSIGNED_DECIMAL_INTEGER:
case UNSIGNED_HEX_INTEGER:
case UNSIGNED_OCTAL_INTEGER:
case STRING_LITERAL1:
case STRING_LITERAL2:
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LBRACKET:
case LCURLY:
case LIMITROWS:
case LOAD:
case LOOKUP:
case LPAREN:
case MANAGEMENT:
case MATCH:
case MERGE:
case MINUS:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case PLUS:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
e = Expression();
list.add( e );
break;
}
default:
jj_la1[137] = jj_gen;
;
}
label_38:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[138] = jj_gen;
break label_38;
}
jj_consume_token(250);
e = Expression();
list.add( e );
}
jj_consume_token(RBRACKET);
{if ("" != null) return astFactory.listLiteral( pos( t ), list );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION MapLiteral() throws ParseException {Token t;
StringPos key;
EXPRESSION value;
List> keys = new ArrayList<>();
List values = new ArrayList<>();
t = jj_consume_token(LCURLY);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
key = PropertyKeyName();
jj_consume_token(252);
value = Expression();
keys.add( key ); values.add( value );
break;
}
default:
jj_la1[139] = jj_gen;
;
}
label_39:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[140] = jj_gen;
break label_39;
}
jj_consume_token(250);
key = PropertyKeyName();
jj_consume_token(252);
value = Expression();
keys.add( key ); values.add( value );
}
jj_consume_token(RCURLY);
{if ("" != null) return astFactory.mapLiteral( pos( t ), keys, values);}
throw new IllegalStateException ("Missing return statement in function");
}
final public StringPos PropertyKeyName() throws ParseException {Token t;
t = SymbolicNameString();
{if ("" != null) return new StringPos( t.image, pos( t ) );}
throw new IllegalStateException ("Missing return statement in function");
}
final public PARAMETER Parameter(ParameterType type) throws ParseException {Token t;
VARIABLE v;
t = jj_consume_token(DOLLAR);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
v = Variable();
{if ("" != null) return astFactory.newParameter( pos( t ), v, type );}
break;
}
case UNSIGNED_DECIMAL_INTEGER:{
jj_consume_token(UNSIGNED_DECIMAL_INTEGER);
{if ("" != null) return astFactory.newParameter( pos( t ), token.image, type );}
break;
}
default:
jj_la1[141] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION OldParameter() throws ParseException {Token t;
VARIABLE v;
t = jj_consume_token(LCURLY);
v = Variable();
jj_consume_token(RCURLY);
{if ("" != null) return astFactory.oldParameter( pos( t ), v );}
throw new IllegalStateException ("Missing return statement in function");
}
final public EXPRESSION FunctionInvocation() throws ParseException {Token before = token;
Token nameSpaceToken;
Token nameToken;
List namespace;
boolean distinct = false;
EXPRESSION e;
List arguments = new ArrayList<>();
namespace = Namespace();
nameToken = SymbolicNameString();
jj_consume_token(LPAREN);
if (jj_2_50(2)) {
jj_consume_token(DISTINCT);
distinct=true;
} else {
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DECIMAL_DOUBLE:
case UNSIGNED_DECIMAL_INTEGER:
case UNSIGNED_HEX_INTEGER:
case UNSIGNED_OCTAL_INTEGER:
case STRING_LITERAL1:
case STRING_LITERAL2:
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DOLLAR:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LBRACKET:
case LCURLY:
case LIMITROWS:
case LOAD:
case LOOKUP:
case LPAREN:
case MANAGEMENT:
case MATCH:
case MERGE:
case MINUS:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case PLUS:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
e = Expression();
arguments.add( e );
break;
}
default:
jj_la1[142] = jj_gen;
;
}
label_40:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[143] = jj_gen;
break label_40;
}
jj_consume_token(250);
e = Expression();
arguments.add( e );
}
jj_consume_token(RPAREN);
{if ("" != null) return astFactory.functionInvocation( pos( before.next ), pos( nameToken ), namespace, nameToken.image, distinct, arguments );}
throw new IllegalStateException ("Missing return statement in function");
}
final public List Namespace() throws ParseException {Token t;
List parts = new ArrayList<>();
label_41:
while (true) {
if (jj_2_51(2)) {
} else {
break label_41;
}
t = SymbolicNameString();
parts.add( t.image );
jj_consume_token(DOT);
}
{if ("" != null) return parts;}
throw new IllegalStateException ("Missing return statement in function");
}
final public String FunctionName() throws ParseException {Token t;
t = SymbolicNameString();
{if ("" != null) return t.image;}
throw new IllegalStateException ("Missing return statement in function");
}
final public List VariableList1() throws ParseException {Token t;
List list = new ArrayList<>();
t = SymbolicNameString();
list.add( astFactory.newVariable( pos( t ), t.image ) );
label_42:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[144] = jj_gen;
break label_42;
}
jj_consume_token(250);
t = SymbolicNameString();
list.add( astFactory.newVariable( pos( t ), t.image) );
}
{if ("" != null) return list;}
throw new IllegalStateException ("Missing return statement in function");
}
final public VARIABLE Variable() throws ParseException {Token t;
t = SymbolicNameString();
{if ("" != null) return astFactory.newVariable( pos( t ), t.image );}
throw new IllegalStateException ("Missing return statement in function");
}
final public List SymbolicNameList1() throws ParseException {Token n;
List list = new ArrayList<>();
n = SymbolicNameString();
list.add(n.image);
label_43:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[145] = jj_gen;
break label_43;
}
jj_consume_token(250);
n = SymbolicNameString();
list.add( n.image );
}
{if ("" != null) return list;}
throw new IllegalStateException ("Missing return statement in function");
}
// Command Section
final public
STATEMENT_WITH_GRAPH CreateCommand(USE_CLAUSE useClause) throws ParseException, Exception {Token start;
boolean replace = false;
STATEMENT_WITH_GRAPH statement;
start = jj_consume_token(CREATE);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case OR:{
jj_consume_token(OR);
jj_consume_token(REPLACE);
replace = true;
break;
}
default:
jj_la1[146] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ROLE:{
statement = CreateRole(start, replace);
break;
}
case USER:{
statement = CreateUser(start, replace);
break;
}
case DATABASE:{
statement = CreateDatabase(start, replace);
break;
}
case CONSTRAINT:{
statement = CreateConstraint(start, replace);
break;
}
case BTREE:
case FULLTEXT:
case INDEX:
case LOOKUP:
case POINT:
case RANGE:
case TEXT:{
statement = CreateIndex(start, replace);
break;
}
case ALIAS:{
statement = CreateAlias(start, replace);
break;
}
default:
jj_la1[147] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
{if ("" != null) return astFactory.useGraph( statement, useClause );}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT Command(USE_CLAUSE useClause) throws ParseException, Exception {STATEMENT statement = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ALTER:
case DENY:
case DROP:
case GRANT:
case RENAME:
case REVOKE:
case START:
case STOP:{
statement = CommandWithUseGraph(useClause);
break;
}
case SHOW:{
statement = ShowCommand(useClause);
break;
}
case TERMINATE:{
statement = TerminateCommand(useClause);
break;
}
default:
jj_la1[148] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
{if ("" != null) return statement;}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT_WITH_GRAPH CommandWithUseGraph(USE_CLAUSE useClause) throws ParseException, Exception {STATEMENT_WITH_GRAPH s;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DROP:{
s = DropCommand();
break;
}
case ALTER:{
s = AlterCommand();
break;
}
case RENAME:{
s = RenameCommand();
break;
}
case DENY:{
s = DenyPrivilege();
break;
}
case REVOKE:{
s = RevokeCommand();
break;
}
case GRANT:{
s = GrantCommand();
break;
}
case START:{
s = StartDatabase();
break;
}
case STOP:{
s = StopDatabase();
break;
}
default:
jj_la1[149] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
{if ("" != null) return astFactory.useGraph( s, useClause );}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT_WITH_GRAPH DropCommand() throws ParseException, Exception {Token start;
STATEMENT_WITH_GRAPH s;
start = jj_consume_token(DROP);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ROLE:{
s = DropRole(start);
break;
}
case USER:{
s = DropUser(start);
break;
}
case DATABASE:{
s = DropDatabase(start);
break;
}
case CONSTRAINT:{
s = DropConstraint(start);
break;
}
case INDEX:{
s = DropIndex(start);
break;
}
case ALIAS:{
s = DropAlias(start);
break;
}
default:
jj_la1[150] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
{if ("" != null) return s;}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT_WITH_GRAPH AlterCommand() throws ParseException, Exception {Token start = null;
STATEMENT_WITH_GRAPH s;
start = jj_consume_token(ALTER);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case DATABASE:{
s = AlterDatabase(start);
break;
}
case ALIAS:{
s = AlterAlias(start);
break;
}
case CURRENT:{
s = AlterCurrentUser(start);
break;
}
case USER:{
s = AlterUser(start);
break;
}
default:
jj_la1[151] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
{if ("" != null) return s;}
throw new IllegalStateException ("Missing return statement in function");
}
// SHOW commands
final public
STATEMENT ShowCommand(USE_CLAUSE useClause) throws ParseException {Token start = null;
Token showCommandType = null;
STATEMENT statement = null;
start = jj_consume_token(SHOW);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ALL:{
showCommandType = jj_consume_token(ALL);
statement = ShowAllCommand(start, useClause);
break;
}
case POPULATED:{
jj_consume_token(POPULATED);
statement = ShowRoles(start, useClause, false);
break;
}
case BTREE:{
showCommandType = jj_consume_token(BTREE);
statement = ShowIndexesAllowBrief(start, useClause, ShowCommandFilterTypes.BTREE);
break;
}
case RANGE:{
showCommandType = jj_consume_token(RANGE);
statement = ShowIndexesNoBrief(start, useClause, ShowCommandFilterTypes.RANGE);
break;
}
case FULLTEXT:{
showCommandType = jj_consume_token(FULLTEXT);
statement = ShowIndexesNoBrief(start, useClause, ShowCommandFilterTypes.FULLTEXT);
break;
}
case TEXT:{
showCommandType = jj_consume_token(TEXT);
statement = ShowIndexesNoBrief(start, useClause, ShowCommandFilterTypes.TEXT);
break;
}
case POINT:{
showCommandType = jj_consume_token(POINT);
statement = ShowIndexesNoBrief(start, useClause, ShowCommandFilterTypes.POINT);
break;
}
case LOOKUP:{
showCommandType = jj_consume_token(LOOKUP);
statement = ShowIndexesNoBrief(start, useClause, ShowCommandFilterTypes.LOOKUP);
break;
}
case UNIQUE:{
showCommandType = jj_consume_token(UNIQUE);
statement = ShowConstraintsAllowBriefAndYield(start, useClause, ShowCommandFilterTypes.UNIQUE);
break;
}
case NODE:{
showCommandType = jj_consume_token(NODE);
statement = ShowNodeCommand(start, useClause);
break;
}
case PROPERTY:{
showCommandType = jj_consume_token(PROPERTY);
statement = ShowPropertyCommand(start, useClause, ShowCommandFilterTypes.EXIST);
break;
}
case EXISTENCE:{
showCommandType = jj_consume_token(EXISTENCE);
statement = ShowConstraintsAllowYield(start, useClause, ShowCommandFilterTypes.EXIST);
break;
}
case EXISTS:{
showCommandType = jj_consume_token(EXISTS);
statement = ShowConstraintsAllowBrief(start, useClause, ShowCommandFilterTypes.OLD_EXISTS);
break;
}
case EXIST:{
showCommandType = jj_consume_token(EXIST);
statement = ShowConstraintsAllowBriefAndYield(start, useClause, ShowCommandFilterTypes.OLD_EXIST);
break;
}
case RELATIONSHIP:{
showCommandType = jj_consume_token(RELATIONSHIP);
statement = ShowRelationshipCommand(start, useClause);
break;
}
case REL:{
showCommandType = jj_consume_token(REL);
statement = ShowRelCommand(start, useClause);
break;
}
case BUILT:{
showCommandType = jj_consume_token(BUILT);
jj_consume_token(IN);
statement = ShowFunctions(start, useClause, ShowCommandFilterTypes.BUILT_IN);
break;
}
case USER:{
showCommandType = jj_consume_token(USER);
jj_consume_token(DEFINED);
statement = ShowFunctions(start, useClause, ShowCommandFilterTypes.USER_DEFINED);
break;
}
case ROLES:{
statement = ShowRoles(start, useClause, true);
break;
}
case INDEX:
case INDEXES:{
statement = ShowIndexesAllowBrief(start, useClause, ShowCommandFilterTypes.ALL);
break;
}
case DATABASE:
case DATABASES:
case DEFAULT_TOKEN:
case HOME:{
statement = ShowDatabase(start, useClause);
break;
}
case USERS:{
statement = ShowUsers(start, useClause);
break;
}
case CURRENT:{
statement = ShowCurrentUser(start, useClause);
break;
}
case CONSTRAINT:
case CONSTRAINTS:{
statement = ShowConstraintsAllowBriefAndYield(start, useClause, ShowCommandFilterTypes.ALL);
break;
}
case PROCEDURE:
case PROCEDURES:{
statement = ShowProcedures(start, useClause);
break;
}
case FUNCTION:
case FUNCTIONS:{
statement = ShowFunctions(start, useClause, ShowCommandFilterTypes.ALL);
break;
}
case TRANSACTION:
case TRANSACTIONS:{
statement = ShowTransactions(start, useClause);
break;
}
case ALIAS:
case ALIASES:{
statement = ShowAliases(start, useClause);
break;
}
default:
jj_la1[152] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
{if ("" != null) return statement;}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT TerminateCommand(USE_CLAUSE useClause) throws ParseException {Token start = null;
STATEMENT statement = null;
start = jj_consume_token(TERMINATE);
statement = TerminateTransactions(start, useClause);
{if ("" != null) return statement;}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT ShowAllCommand(Token start, USE_CLAUSE useClause) throws ParseException {STATEMENT statement = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ROLES:{
statement = ShowRoles(start, useClause, true);
break;
}
case INDEX:
case INDEXES:{
statement = ShowIndexesAllowBrief(start, useClause, ShowCommandFilterTypes.ALL);
break;
}
case CONSTRAINT:
case CONSTRAINTS:{
statement = ShowConstraintsAllowBriefAndYield(start, useClause, ShowCommandFilterTypes.ALL);
break;
}
case FUNCTION:
case FUNCTIONS:{
statement = ShowFunctions(start, useClause, ShowCommandFilterTypes.ALL);
break;
}
default:
jj_la1[153] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
{if ("" != null) return statement;}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT ShowNodeCommand(Token start, USE_CLAUSE useClause) throws ParseException {Token constraintType = null;
STATEMENT statement = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case KEY:{
constraintType = jj_consume_token(KEY);
statement = ShowConstraintsAllowBriefAndYield(start, useClause, ShowCommandFilterTypes.NODE_KEY);
break;
}
case PROPERTY:{
constraintType = jj_consume_token(PROPERTY);
statement = ShowPropertyCommand(start, useClause, ShowCommandFilterTypes.NODE_EXIST);
break;
}
case EXISTENCE:{
constraintType = jj_consume_token(EXISTENCE);
statement = ShowConstraintsAllowYield(start, useClause, ShowCommandFilterTypes.NODE_EXIST);
break;
}
case EXISTS:{
constraintType = jj_consume_token(EXISTS);
statement = ShowConstraintsAllowBrief(start, useClause, ShowCommandFilterTypes.NODE_OLD_EXISTS);
break;
}
case EXIST:{
constraintType = jj_consume_token(EXIST);
statement = ShowConstraintsAllowBriefAndYield(start, useClause, ShowCommandFilterTypes.NODE_OLD_EXIST);
break;
}
default:
jj_la1[154] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
{if ("" != null) return statement;}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT ShowRelationshipCommand(Token start, USE_CLAUSE useClause) throws ParseException {Token constraintType = null;
STATEMENT statement = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case PROPERTY:{
constraintType = jj_consume_token(PROPERTY);
statement = ShowPropertyCommand(start, useClause, ShowCommandFilterTypes.RELATIONSHIP_EXIST);
break;
}
case EXISTENCE:{
constraintType = jj_consume_token(EXISTENCE);
statement = ShowConstraintsAllowYield(start, useClause, ShowCommandFilterTypes.RELATIONSHIP_EXIST);
break;
}
case EXISTS:{
constraintType = jj_consume_token(EXISTS);
statement = ShowConstraintsAllowBrief(start, useClause, ShowCommandFilterTypes.RELATIONSHIP_OLD_EXISTS);
break;
}
case EXIST:{
constraintType = jj_consume_token(EXIST);
statement = ShowConstraintsAllowBriefAndYield(start, useClause, ShowCommandFilterTypes.RELATIONSHIP_OLD_EXIST);
break;
}
default:
jj_la1[155] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
{if ("" != null) return statement;}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT ShowRelCommand(Token start, USE_CLAUSE useClause) throws ParseException {STATEMENT statement = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case PROPERTY:{
jj_consume_token(PROPERTY);
statement = ShowPropertyCommand(start, useClause, ShowCommandFilterTypes.RELATIONSHIP_EXIST);
break;
}
case EXISTENCE:{
jj_consume_token(EXISTENCE);
statement = ShowConstraintsAllowYield(start, useClause, ShowCommandFilterTypes.RELATIONSHIP_EXIST);
break;
}
case EXIST:{
jj_consume_token(EXIST);
statement = ShowConstraintsAllowYield(start, useClause, ShowCommandFilterTypes.RELATIONSHIP_EXIST);
break;
}
default:
jj_la1[156] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
{if ("" != null) return statement;}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT ShowPropertyCommand(Token start, USE_CLAUSE useClause, ShowCommandFilterTypes constraintType) throws ParseException {STATEMENT statement = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case EXISTENCE:{
jj_consume_token(EXISTENCE);
statement = ShowConstraintsAllowYield(start, useClause, constraintType);
break;
}
case EXIST:{
jj_consume_token(EXIST);
statement = ShowConstraintsAllowYield(start, useClause, constraintType);
break;
}
default:
jj_la1[157] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
{if ("" != null) return statement;}
throw new IllegalStateException ("Missing return statement in function");
}
final public RETURN_ITEM YieldItem() throws ParseException {VARIABLE e;
VARIABLE v = null;
Token eStart;
Token eEnd;
eStart = token;
e = Variable();
eEnd = token;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case AS:{
jj_consume_token(AS);
v = Variable();
break;
}
default:
jj_la1[158] = jj_gen;
;
}
if ( v != null )
{
{if ("" != null) return astFactory.newReturnItem( pos( eStart.next ), e, v );}
}
else
{
{if ("" != null) return astFactory.newReturnItem( pos( eStart.next ), e, eStart.next.beginOffset, eEnd.endOffset );}
}
throw new IllegalStateException ("Missing return statement in function");
}
final public YIELD YieldClause() throws ParseException {Token start;
Token skipPosition = null;
Token limitPosition = null;
Token orderToken = null;
boolean returnAll = false;
RETURN_ITEM item;
ORDER_ITEM o;
List returnItems = new ArrayList<>();
List orders = new ArrayList<>();
EXPRESSION skip = null;
EXPRESSION limit = null;
WHERE where = null;
start = jj_consume_token(YIELD);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case TIMES:{
jj_consume_token(TIMES);
returnAll = true;
break;
}
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
item = YieldItem();
returnItems.add( item );
label_44:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[159] = jj_gen;
break label_44;
}
jj_consume_token(250);
item = YieldItem();
returnItems.add( item );
}
break;
}
default:
jj_la1[160] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ORDER:{
orderToken = jj_consume_token(ORDER);
jj_consume_token(BY);
o = OrderItem();
orders.add( o );
label_45:
while (true) {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case 250:{
break;
}
default:
jj_la1[161] = jj_gen;
break label_45;
}
jj_consume_token(250);
o = OrderItem();
orders.add( o );
}
break;
}
default:
jj_la1[162] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case SKIPROWS:{
skipPosition = jj_consume_token(SKIPROWS);
skip = SignedIntegerLiteral();
break;
}
default:
jj_la1[163] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case LIMITROWS:{
limitPosition = jj_consume_token(LIMITROWS);
limit = SignedIntegerLiteral();
break;
}
default:
jj_la1[164] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:{
where = WhereClause();
break;
}
default:
jj_la1[165] = jj_gen;
;
}
{if ("" != null) return astFactory.yieldClause( pos( start ), returnAll, returnItems, pos( start.next ), orders, pos( orderToken ), skip, pos( skipPosition ), limit, pos( limitPosition ), where );}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT ShowIndexesAllowBrief(Token start, USE_CLAUSE useClause, ShowCommandFilterTypes indexType) throws ParseException {// all and btree indexes
List clauses = new ArrayList<>();
if ( useClause != null )
{
clauses.add( useClause );
}
boolean brief = false;
boolean verbose = false;
WHERE where = null;
YIELD yieldClause = null;
RETURN_CLAUSE returnClause = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case INDEX:{
jj_consume_token(INDEX);
break;
}
case INDEXES:{
jj_consume_token(INDEXES);
break;
}
default:
jj_la1[166] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case BRIEF:
case VERBOSE:
case WHERE:
case YIELD:{
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case BRIEF:
case VERBOSE:{
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case BRIEF:{
jj_consume_token(BRIEF);
brief = true;
break;
}
case VERBOSE:{
jj_consume_token(VERBOSE);
verbose = true;
break;
}
default:
jj_la1[167] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case OUTPUT:{
jj_consume_token(OUTPUT);
break;
}
default:
jj_la1[168] = jj_gen;
;
}
break;
}
case YIELD:{
yieldClause = YieldClause();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case RETURN:{
returnClause = ReturnClause();
break;
}
default:
jj_la1[169] = jj_gen;
;
}
break;
}
case WHERE:{
where = WhereClause();
break;
}
default:
jj_la1[170] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
break;
}
default:
jj_la1[171] = jj_gen;
;
}
if ( yieldClause != null )
{
clauses.add( astFactory.showIndexClause( pos( start ), indexType, brief, verbose, where, true ) );
clauses.add( yieldClause );
if ( returnClause != null )
{
clauses.add( returnClause );
}
}
else
{
clauses.add( astFactory.showIndexClause( pos( start ), indexType, brief, verbose, where, false ) );
}
{if ("" != null) return astFactory.newSingleQuery( pos( start ), clauses );}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT ShowIndexesNoBrief(Token start, USE_CLAUSE useClause, ShowCommandFilterTypes indexType) throws ParseException {// fulltext, text and lookup indexes
List clauses = new ArrayList<>();
if (useClause != null) {
clauses.add( useClause );
}
WHERE where = null;
YIELD yieldClause = null;
RETURN_CLAUSE returnClause = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case INDEX:{
jj_consume_token(INDEX);
break;
}
case INDEXES:{
jj_consume_token(INDEXES);
break;
}
default:
jj_la1[172] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:
case YIELD:{
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case YIELD:{
yieldClause = YieldClause();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case RETURN:{
returnClause = ReturnClause();
break;
}
default:
jj_la1[173] = jj_gen;
;
}
break;
}
case WHERE:{
where = WhereClause();
break;
}
default:
jj_la1[174] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
break;
}
default:
jj_la1[175] = jj_gen;
;
}
if ( yieldClause != null )
{
clauses.add( astFactory.showIndexClause( pos( start ), indexType, false, false, where, true ) );
clauses.add( yieldClause );
if ( returnClause != null )
{
clauses.add( returnClause );
}
}
else
{
clauses.add( astFactory.showIndexClause( pos( start ), indexType, false, false, where, false ) );
}
{if ("" != null) return astFactory.newSingleQuery( pos( start ), clauses );}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT ShowConstraintsAllowBriefAndYield(Token start, USE_CLAUSE useClause, ShowCommandFilterTypes constraintType) throws ParseException {// all, node key, uniqueness and old valid existence constraints
List clauses = new ArrayList<>();
if ( useClause != null )
{
clauses.add( useClause );
}
boolean brief = false;
boolean verbose = false;
WHERE where = null;
YIELD yieldClause = null;
RETURN_CLAUSE returnClause = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case CONSTRAINT:{
jj_consume_token(CONSTRAINT);
break;
}
case CONSTRAINTS:{
jj_consume_token(CONSTRAINTS);
break;
}
default:
jj_la1[176] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case BRIEF:
case VERBOSE:
case WHERE:
case YIELD:{
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case BRIEF:
case VERBOSE:{
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case BRIEF:{
jj_consume_token(BRIEF);
brief = true;
break;
}
case VERBOSE:{
jj_consume_token(VERBOSE);
verbose = true;
break;
}
default:
jj_la1[177] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case OUTPUT:{
jj_consume_token(OUTPUT);
break;
}
default:
jj_la1[178] = jj_gen;
;
}
break;
}
case YIELD:{
yieldClause = YieldClause();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case RETURN:{
returnClause = ReturnClause();
break;
}
default:
jj_la1[179] = jj_gen;
;
}
break;
}
case WHERE:{
where = WhereClause();
break;
}
default:
jj_la1[180] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
break;
}
default:
jj_la1[181] = jj_gen;
;
}
if ( yieldClause != null )
{
clauses.add( astFactory.showConstraintClause( pos( start ), constraintType, brief, verbose, where, true ) );
clauses.add( yieldClause );
if ( returnClause != null )
{
clauses.add( returnClause );
}
}
else
{
clauses.add( astFactory.showConstraintClause( pos( start ), constraintType, brief, verbose, where, false ) );
}
{if ("" != null) return astFactory.newSingleQuery( pos( start ), clauses );}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT ShowConstraintsAllowBrief(Token start, USE_CLAUSE useClause, ShowCommandFilterTypes constraintType) throws ParseException {// old deprecated existence constraints
List clauses = new ArrayList<>();
if ( useClause != null )
{
clauses.add( useClause );
}
boolean brief = false;
boolean verbose = false;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case CONSTRAINT:{
jj_consume_token(CONSTRAINT);
break;
}
case CONSTRAINTS:{
jj_consume_token(CONSTRAINTS);
break;
}
default:
jj_la1[182] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case BRIEF:
case VERBOSE:{
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case BRIEF:{
jj_consume_token(BRIEF);
brief = true;
break;
}
case VERBOSE:{
jj_consume_token(VERBOSE);
verbose = true;
break;
}
default:
jj_la1[183] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case OUTPUT:{
jj_consume_token(OUTPUT);
break;
}
default:
jj_la1[184] = jj_gen;
;
}
break;
}
default:
jj_la1[185] = jj_gen;
;
}
clauses.add( astFactory.showConstraintClause( pos( start ), constraintType, brief, verbose, null, false ) );
{if ("" != null) return astFactory.newSingleQuery( pos( start ), clauses );}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT ShowConstraintsAllowYield(Token start, USE_CLAUSE useClause, ShowCommandFilterTypes constraintType) throws ParseException {// new existence constraints
List clauses = new ArrayList<>();
if (useClause != null)
{
clauses.add( useClause );
}
WHERE where = null;
YIELD yieldClause = null;
RETURN_CLAUSE returnClause = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case CONSTRAINT:{
jj_consume_token(CONSTRAINT);
break;
}
case CONSTRAINTS:{
jj_consume_token(CONSTRAINTS);
break;
}
default:
jj_la1[186] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:
case YIELD:{
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case YIELD:{
yieldClause = YieldClause();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case RETURN:{
returnClause = ReturnClause();
break;
}
default:
jj_la1[187] = jj_gen;
;
}
break;
}
case WHERE:{
where = WhereClause();
break;
}
default:
jj_la1[188] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
break;
}
default:
jj_la1[189] = jj_gen;
;
}
if ( yieldClause != null )
{
clauses.add( astFactory.showConstraintClause( pos( start ), constraintType, false, false, where, true ) );
clauses.add( yieldClause );
if ( returnClause != null )
{
clauses.add( returnClause );
}
}
else
{
clauses.add( astFactory.showConstraintClause( pos( start ), constraintType, false, false, where, false ) );
}
{if ("" != null) return astFactory.newSingleQuery( pos( start ), clauses );}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT ShowProcedures(Token start, USE_CLAUSE useClause) throws ParseException {List clauses = new ArrayList<>();
if (useClause != null)
{
clauses.add( useClause );
}
boolean currentUser = false;
Token userToken = null;
String user = null;
WHERE where = null;
YIELD yieldClause = null;
RETURN_CLAUSE returnClause = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case PROCEDURE:{
jj_consume_token(PROCEDURE);
break;
}
case PROCEDURES:{
jj_consume_token(PROCEDURES);
break;
}
default:
jj_la1[190] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case EXECUTABLE:{
jj_consume_token(EXECUTABLE);
currentUser = true;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case BY:{
jj_consume_token(BY);
if (jj_2_52(2147483647)) {
jj_consume_token(CURRENT);
jj_consume_token(USER);
currentUser = true;
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
userToken = SymbolicNameString();
user = userToken.image; currentUser = false;
break;
}
default:
jj_la1[191] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
break;
}
default:
jj_la1[192] = jj_gen;
;
}
break;
}
default:
jj_la1[193] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:
case YIELD:{
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case YIELD:{
yieldClause = YieldClause();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case RETURN:{
returnClause = ReturnClause();
break;
}
default:
jj_la1[194] = jj_gen;
;
}
break;
}
case WHERE:{
where = WhereClause();
break;
}
default:
jj_la1[195] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
break;
}
default:
jj_la1[196] = jj_gen;
;
}
if ( yieldClause != null )
{
clauses.add( astFactory.showProcedureClause( pos( start ), currentUser, user, where, true ) );
clauses.add( yieldClause );
if ( returnClause != null )
{
clauses.add( returnClause );
}
}
else
{
clauses.add( astFactory.showProcedureClause( pos( start ), currentUser, user, where, false ) );
}
{if ("" != null) return astFactory.newSingleQuery( pos( start ), clauses );}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT ShowFunctions(Token start, USE_CLAUSE useClause, ShowCommandFilterTypes functionType) throws ParseException {List clauses = new ArrayList<>();
if (useClause != null)
{
clauses.add( useClause );
}
boolean currentUser = false;
Token userToken = null;
String user = null;
WHERE where = null;
YIELD yieldClause = null;
RETURN_CLAUSE returnClause = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case FUNCTION:{
jj_consume_token(FUNCTION);
break;
}
case FUNCTIONS:{
jj_consume_token(FUNCTIONS);
break;
}
default:
jj_la1[197] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case EXECUTABLE:{
jj_consume_token(EXECUTABLE);
currentUser = true;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case BY:{
jj_consume_token(BY);
if (jj_2_53(2147483647)) {
jj_consume_token(CURRENT);
jj_consume_token(USER);
currentUser = true;
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case ESCAPED_SYMBOLIC_NAME:
case ACCESS:
case ACTIVE:
case ALIAS:
case ALIASES:
case ALL_SHORTEST_PATH:
case ALL:
case ALTER:
case AND:
case ANY:
case AS:
case ASC:
case ASSERT:
case ASSIGN:
case AT:
case BRIEF:
case BTREE:
case BUILT:
case BY:
case CALL:
case CASE:
case CATALOG:
case CHANGE:
case COMMIT:
case CONSTRAINT:
case CONSTRAINTS:
case CONTAINS:
case COPY:
case COUNT:
case CREATE:
case CSV:
case CURRENT:
case DATA:
case DATABASE:
case DATABASES:
case DBMS:
case DEFAULT_TOKEN:
case DEFINED:
case DELETE:
case DENY:
case DESC:
case DESTROY:
case DETACH:
case DISTINCT:
case DRIVER:
case DROP:
case DUMP:
case EACH:
case ELEMENT:
case ELEMENTS:
case ELSE:
case ENCRYPTED:
case END:
case ENDS:
case EXECUTABLE:
case EXIST:
case EXISTENCE:
case EXISTS:
case EXTRACT:
case FALSE:
case FIELDTERMINATOR:
case FILTER:
case FOR:
case FOREACH:
case FROM:
case FULLTEXT:
case FUNCTION:
case FUNCTIONS:
case GRANT:
case GRAPH:
case GRAPHS:
case HEADERS:
case HOME:
case IF:
case IN:
case INDEX:
case INDEXES:
case IS:
case JOIN:
case KEY:
case LABEL:
case LABELS:
case LIMITROWS:
case LOAD:
case LOOKUP:
case MANAGEMENT:
case MATCH:
case MERGE:
case NAME:
case NAMES:
case NEW:
case NODE:
case NODES:
case NONE:
case NOT:
case NOWAIT:
case NULL:
case OF:
case ON:
case ONLY:
case OPTIONAL:
case OPTIONS:
case OR:
case ORDER:
case OUTPUT:
case PASSWORD:
case PASSWORDS:
case PERIODIC:
case PLAINTEXT:
case POINT:
case POPULATED:
case PRIVILEGE:
case PRIVILEGES:
case PROCEDURE:
case PROCEDURES:
case PROPERTY:
case RANGE:
case READ:
case REDUCE:
case RENAME:
case REL:
case RELATIONSHIP:
case RELATIONSHIPS:
case REMOVE:
case REPLACE:
case REQUIRE:
case REQUIRED:
case RETURN:
case REVOKE:
case ROLE:
case ROLES:
case ROW:
case ROWS:
case SCAN:
case SEC:
case SECOND:
case SECONDS:
case SEEK:
case SET:
case SHORTEST_PATH:
case SHOW:
case SINGLE:
case SKIPROWS:
case START:
case STARTS:
case STATUS:
case STOP:
case SUSPENDED:
case TARGET:
case TERMINATE:
case TEXT:
case THEN:
case TO:
case TRANSACTION:
case TRANSACTIONS:
case TRAVERSE:
case TRUE:
case TYPE:
case TYPES:
case UNION:
case UNIQUE:
case UNWIND:
case USE:
case USER:
case USERS:
case USING:
case VERBOSE:
case WAIT:
case WHEN:
case WHERE:
case WITH:
case WRITE:
case XOR:
case YIELD:
case IDENTIFIER:{
userToken = SymbolicNameString();
user = userToken.image; currentUser = false;
break;
}
default:
jj_la1[198] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
break;
}
default:
jj_la1[199] = jj_gen;
;
}
break;
}
default:
jj_la1[200] = jj_gen;
;
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:
case YIELD:{
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case YIELD:{
yieldClause = YieldClause();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case RETURN:{
returnClause = ReturnClause();
break;
}
default:
jj_la1[201] = jj_gen;
;
}
break;
}
case WHERE:{
where = WhereClause();
break;
}
default:
jj_la1[202] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
break;
}
default:
jj_la1[203] = jj_gen;
;
}
if ( yieldClause != null )
{
clauses.add( astFactory.showFunctionClause( pos( start ), functionType, currentUser, user, where, true ) );
clauses.add( yieldClause );
if ( returnClause != null )
{
clauses.add( returnClause );
}
}
else
{
clauses.add( astFactory.showFunctionClause( pos( start ), functionType, currentUser, user, where, false ) );
}
{if ("" != null) return astFactory.newSingleQuery( pos( start ), clauses );}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT ShowTransactions(Token start, USE_CLAUSE useClause) throws ParseException {List clauses = new ArrayList<>();
if (useClause != null)
{
clauses.add( useClause );
}
List ids = new ArrayList<>();
PARAMETER param = null;
SimpleEither, PARAMETER> idEither = SimpleEither.left(ids);
WHERE where = null;
YIELD yieldClause = null;
RETURN_CLAUSE returnClause = null;
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case TRANSACTION:{
jj_consume_token(TRANSACTION);
break;
}
case TRANSACTIONS:{
jj_consume_token(TRANSACTIONS);
break;
}
default:
jj_la1[204] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case STRING_LITERAL1:
case STRING_LITERAL2:
case DOLLAR:
case WHERE:
case YIELD:{
if (jj_2_54(2147483647)) {
yieldClause = YieldClause();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case RETURN:{
returnClause = ReturnClause();
break;
}
default:
jj_la1[205] = jj_gen;
;
}
} else if (jj_2_55(2147483647)) {
where = WhereClause();
} else {
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case STRING_LITERAL1:
case STRING_LITERAL2:
case DOLLAR:{
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case STRING_LITERAL1:
case STRING_LITERAL2:{
ids = TransactionIdStringList();
idEither=SimpleEither.left(ids);
break;
}
case DOLLAR:{
param = Parameter(ParameterType.ANY);
idEither=SimpleEither.right(param);
break;
}
default:
jj_la1[206] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case WHERE:
case YIELD:{
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case YIELD:{
yieldClause = YieldClause();
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case RETURN:{
returnClause = ReturnClause();
break;
}
default:
jj_la1[207] = jj_gen;
;
}
break;
}
case WHERE:{
where = WhereClause();
break;
}
default:
jj_la1[208] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
break;
}
default:
jj_la1[209] = jj_gen;
;
}
break;
}
default:
jj_la1[210] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
break;
}
default:
jj_la1[211] = jj_gen;
;
}
if ( yieldClause != null )
{
clauses.add( astFactory.showTransactionsClause( pos( start ), idEither, where, true ) );
clauses.add( yieldClause );
if ( returnClause != null )
{
clauses.add( returnClause );
}
}
else
{
clauses.add( astFactory.showTransactionsClause( pos( start ), idEither, where, false ) );
}
{if ("" != null) return astFactory.newSingleQuery( pos( start ), clauses );}
throw new IllegalStateException ("Missing return statement in function");
}
final public STATEMENT TerminateTransactions(Token start, USE_CLAUSE useClause) throws ParseException {List clauses = new ArrayList<>();
if (useClause != null)
{
clauses.add( useClause );
}
List ids = new ArrayList<>();
PARAMETER param = null;
SimpleEither, PARAMETER> idEither = SimpleEither.left(ids);
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case TRANSACTION:{
jj_consume_token(TRANSACTION);
break;
}
case TRANSACTIONS:{
jj_consume_token(TRANSACTIONS);
break;
}
default:
jj_la1[212] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case STRING_LITERAL1:
case STRING_LITERAL2:
case DOLLAR:{
switch (jj_ntk == -1 ? jj_ntk_f() : jj_ntk) {
case STRING_LITERAL1:
case STRING_LITERAL2:{
ids = TransactionIdStringList();
idEither=SimpleEither.left(ids);
break;
}
case DOLLAR:{
param = Parameter(ParameterType.ANY);
idEither=SimpleEither.right(param);
break;
}
default:
jj_la1[213] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
break;
}
default:
jj_la1[214] = jj_gen;
;
}
clauses.add( astFactory.terminateTransactionsClause( pos( start ), idEither ) );
{if ("" != null) return astFactory.newSingleQuery( pos( start ), clauses );}
throw new IllegalStateException ("Missing return statement in function");
}
// Schema commands
// Constraint commands
final public
SCHEMA_COMMAND CreateConstraint(Token start, boolean replace) throws ParseException, Exception {String name = null;
boolean ifNotExists = false;
Token label;
VARIABLE variable = null;
List properties= new ArrayList<>();
SimpleEither
.
*/
public final boolean trace_enabled() {
return false;
}
/** Enable tracing. */
public final void enable_tracing() {}
/** Disable tracing. */
public final void disable_tracing() {}
private void jj_rescan_token() {
jj_rescan = true;
for (int i = 0; i < 73; i++) {
try {
JJCalls p = jj_2_rtns[i];
do {
if (p.gen > jj_gen) {
jj_la = p.arg;
jj_scanpos = p.first;
jj_lastpos = p.first;
switch (i) {
case 0: jj_3_1(); break;
case 1: jj_3_2(); break;
case 2: jj_3_3(); break;
case 3: jj_3_4(); break;
case 4: jj_3_5(); break;
case 5: jj_3_6(); break;
case 6: jj_3_7(); break;
case 7: jj_3_8(); break;
case 8: jj_3_9(); break;
case 9: jj_3_10(); break;
case 10: jj_3_11(); break;
case 11: jj_3_12(); break;
case 12: jj_3_13(); break;
case 13: jj_3_14(); break;
case 14: jj_3_15(); break;
case 15: jj_3_16(); break;
case 16: jj_3_17(); break;
case 17: jj_3_18(); break;
case 18: jj_3_19(); break;
case 19: jj_3_20(); break;
case 20: jj_3_21(); break;
case 21: jj_3_22(); break;
case 22: jj_3_23(); break;
case 23: jj_3_24(); break;
case 24: jj_3_25(); break;
case 25: jj_3_26(); break;
case 26: jj_3_27(); break;
case 27: jj_3_28(); break;
case 28: jj_3_29(); break;
case 29: jj_3_30(); break;
case 30: jj_3_31(); break;
case 31: jj_3_32(); break;
case 32: jj_3_33(); break;
case 33: jj_3_34(); break;
case 34: jj_3_35(); break;
case 35: jj_3_36(); break;
case 36: jj_3_37(); break;
case 37: jj_3_38(); break;
case 38: jj_3_39(); break;
case 39: jj_3_40(); break;
case 40: jj_3_41(); break;
case 41: jj_3_42(); break;
case 42: jj_3_43(); break;
case 43: jj_3_44(); break;
case 44: jj_3_45(); break;
case 45: jj_3_46(); break;
case 46: jj_3_47(); break;
case 47: jj_3_48(); break;
case 48: jj_3_49(); break;
case 49: jj_3_50(); break;
case 50: jj_3_51(); break;
case 51: jj_3_52(); break;
case 52: jj_3_53(); break;
case 53: jj_3_54(); break;
case 54: jj_3_55(); break;
case 55: jj_3_56(); break;
case 56: jj_3_57(); break;
case 57: jj_3_58(); break;
case 58: jj_3_59(); break;
case 59: jj_3_60(); break;
case 60: jj_3_61(); break;
case 61: jj_3_62(); break;
case 62: jj_3_63(); break;
case 63: jj_3_64(); break;
case 64: jj_3_65(); break;
case 65: jj_3_66(); break;
case 66: jj_3_67(); break;
case 67: jj_3_68(); break;
case 68: jj_3_69(); break;
case 69: jj_3_70(); break;
case 70: jj_3_71(); break;
case 71: jj_3_72(); break;
case 72: jj_3_73(); break;
}
}
p = p.next;
} while (p != null);
} catch(LookaheadSuccess ls) { /* ignore */ }
}
jj_rescan = false;
}
private void jj_save(int index, int xla) {
JJCalls p = jj_2_rtns[index];
while (p.gen > jj_gen) {
if (p.next == null) {
p.next = new JJCalls();
p = p.next;
break;
}
p = p.next;
}
p.gen = jj_gen + xla - jj_la;
p.first = token;
p.arg = xla;
}
static final class JJCalls {
int gen;
Token first;
int arg;
JJCalls next;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy