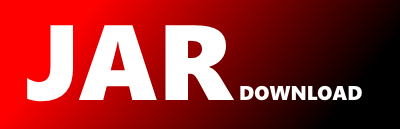
org.neo4j.cypher.export.SubGraphExporter Maven / Gradle / Ivy
/*
* Copyright (c) 2002-2016 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.cypher.export;
import java.io.PrintWriter;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Locale;
import org.neo4j.graphdb.Direction;
import org.neo4j.graphdb.Label;
import org.neo4j.graphdb.Node;
import org.neo4j.graphdb.PropertyContainer;
import org.neo4j.graphdb.Relationship;
import org.neo4j.graphdb.schema.ConstraintDefinition;
import org.neo4j.graphdb.schema.ConstraintType;
import org.neo4j.graphdb.schema.IndexDefinition;
import org.neo4j.helpers.collection.Iterables;
public class SubGraphExporter
{
private final SubGraph graph;
public SubGraphExporter( SubGraph graph )
{
this.graph = graph;
}
public void export( PrintWriter out )
{
export(out, null, null);
}
public void export( PrintWriter out, String begin, String commit )
{
output( out, begin );
appendIndexes( out );
appendConstraints( out );
output( out, commit, begin );
long nodes = appendNodes( out );
long relationships = appendRelationships( out );
if ( nodes + relationships > 0 )
{
out.println( ";" );
}
output( out, commit );
}
private void output( PrintWriter out, String ... commands )
{
for ( String command : commands )
{
if ( command == null ) continue;
out.println( command );
}
}
private Collection exportIndexes()
{
final List result = new ArrayList<>();
for ( IndexDefinition index : graph.getIndexes() )
{
if ( !index.isConstraintIndex() )
{
Iterator propertyKeys = index.getPropertyKeys().iterator();
if ( !propertyKeys.hasNext() )
{
throw new IllegalStateException( "Indexes should have at least one property key" );
}
String key = quote( propertyKeys.next() );
if ( propertyKeys.hasNext() )
{
throw new RuntimeException( "Exporting compound indexes is not implemented yet" );
}
String label = quote( index.getLabel().name() );
result.add( "create index on :" + label + "(" + key + ")" );
}
}
Collections.sort( result );
return result;
}
private Collection exportConstraints()
{
final List result = new ArrayList<>();
for ( ConstraintDefinition constraint : graph.getConstraints() )
{
if ( !constraint.isConstraintType( ConstraintType.UNIQUENESS ) )
{
throw new RuntimeException( "Exporting constraints other than uniqueness is not implemented yet" );
}
Iterator propertyKeys = constraint.getPropertyKeys().iterator();
if ( !propertyKeys.hasNext() )
{
throw new IllegalStateException( "Constraints should have at least one property key" );
}
String key = quote( propertyKeys.next() );
if ( propertyKeys.hasNext() )
{
throw new RuntimeException( "Exporting compound constraints is not implemented yet" );
}
String label = quote( constraint.getLabel().name() );
result.add( "create constraint on (n:" + label + ") assert n." + key + " is unique" );
}
Collections.sort( result );
return result;
}
private String quote( String id )
{
return "`" + id + "`";
}
private String labelString( Node node )
{
Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy