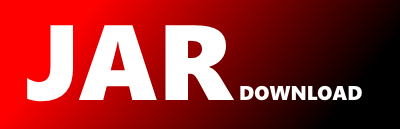
org.neo4j.cypher.internal.javacompat.ExecutionResult Maven / Gradle / Ivy
/*
* Copyright (c) 2002-2016 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.cypher.internal.javacompat;
import scala.collection.JavaConversions;
import java.io.PrintWriter;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.stream.Stream;
import org.neo4j.cypher.CypherException;
import org.neo4j.graphdb.ExecutionPlanDescription;
import org.neo4j.graphdb.Notification;
import org.neo4j.graphdb.QueryExecutionException;
import org.neo4j.graphdb.QueryExecutionType;
import org.neo4j.graphdb.QueryExecutionType.QueryType;
import org.neo4j.graphdb.ResourceIterable;
import org.neo4j.graphdb.ResourceIterator;
import org.neo4j.graphdb.Result;
import org.neo4j.kernel.impl.query.QueryExecutionKernelException;
import org.neo4j.kernel.impl.query.QuerySession;
/**
* Holds Cypher query result sets, in tabular form. Each row of the result is a map
* of column name to result object. Each column name correlates directly
* with the terms used in the "return" clause of the Cypher query.
* The result objects could be {@link org.neo4j.graphdb.Node Nodes},
* {@link org.neo4j.graphdb.Relationship Relationships} or java primitives.
*
*
* Either iterate directly over the ExecutionResult to retrieve each row of the result
* set, or use columnAs()
to access a single column with result objects
* cast to a type.
*/
public class ExecutionResult implements ResourceIterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy