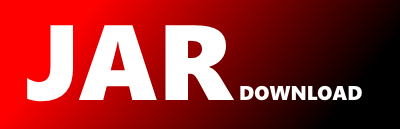
org.neo4j.visualization.SubgraphMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neo4j-graphviz Show documentation
Show all versions of neo4j-graphviz Show documentation
Utility component to generate Graphviz .dot notation from Neo4j graphs.
/*
* Copyright (c) 2002-2016 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.visualization;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import org.neo4j.graphdb.Node;
import org.neo4j.graphdb.Relationship;
import org.neo4j.walk.Visitor;
import org.neo4j.walk.Walker;
public interface SubgraphMapper
{
String getSubgraphFor( Node node );
public abstract class SubgraphMappingWalker extends Walker
{
private final SubgraphMapper mapper;
protected SubgraphMappingWalker( SubgraphMapper mapper )
{
this.mapper = mapper;
}
private Map> subgraphs;
private Collection generic;
private Collection relationships;
private synchronized void initialize()
{
if ( subgraphs != null ) return;
Map mappings = new HashMap();
subgraphs = new HashMap>();
generic = new ArrayList();
for ( Node node : nodes() )
{
if ( mappings.containsKey( node ) ) continue;
String subgraph = subgraphFor( node );
if ( !subgraphs.containsKey( subgraph ) && subgraph != null )
{
subgraphs.put( subgraph, new ArrayList() );
}
mappings.put( node, subgraph );
}
relationships = new ArrayList();
for ( Relationship rel : relationships() )
{
if ( mappings.containsKey( rel.getStartNode() )
&& mappings.containsKey( rel.getEndNode() ) )
{
relationships.add( rel );
}
}
for ( Map.Entry e : mappings.entrySet() )
{
( e.getValue() == null ? generic : subgraphs.get( e.getValue() ) ).add( e.getKey() );
}
}
private String subgraphFor( Node node )
{
return mapper == null ? null : mapper.getSubgraphFor( node );
}
@Override
public final R accept( Visitor visitor ) throws E
{
initialize();
for ( Map.Entry> subgraph : subgraphs.entrySet() )
{
Visitor subVisitor = visitor.visitSubgraph( subgraph.getKey() );
for ( Node node : subgraph.getValue() )
{
subVisitor.visitNode( node );
}
subVisitor.done();
}
for ( Node node : generic )
{
visitor.visitNode( node );
}
for ( Relationship relationship : relationships )
{
visitor.visitRelationship( relationship );
}
return visitor.done();
}
protected abstract Iterable nodes();
protected abstract Iterable relationships();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy