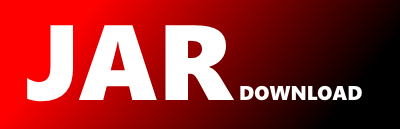
org.neo4j.jdbc.bolt.cache.BoltDriverCache Maven / Gradle / Ivy
Show all versions of neo4j-jdbc-bolt Show documentation
/*
* Copyright (c) 2016 LARUS Business Automation [http://www.larus-ba.it]
*
* This file is part of the "LARUS Integration Framework for Neo4j".
*
* The "LARUS Integration Framework for Neo4j" is licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Created on 02/10/18
*/
package org.neo4j.jdbc.bolt.cache;
import org.neo4j.driver.v1.AuthToken;
import org.neo4j.driver.v1.Config;
import org.neo4j.driver.v1.Driver;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Function;
/**
* A cache for the driver instances in order to reduce the number of them.
* In the JDBC specification the connection has the properties meanwhile in Neo4j they are on the driver.
*/
public class BoltDriverCache {
private Map cache;
private Function builder;
/**
* Setup the cache for the specific building function
* @param builder
*/
public BoltDriverCache(Function builder){
this.builder = builder;
this.cache = new ConcurrentHashMap<>();
}
/**
* Get driver from cache, building it if necessary
* @param routingUris
* @param config
* @param authToken
* @param info
* @return
* @throws URISyntaxException
*/
public Driver getDriver(List routingUris, Config config, AuthToken authToken, Properties info) throws URISyntaxException {
BoltDriverCacheKey key = new BoltDriverCacheKey(routingUris, config, authToken, info);
Driver driver = cache.get(key);
if(null == driver){
driver = new BoltDriverCached(builder.apply(key), this, key);
cache.put(key, driver);
}
return driver;
}
public Driver removeFromCache(BoltDriverCacheKey key){
return cache.remove(key);
}
/**
* The internal cache, for inspection only
* @return
*/
public Map getCache() {
return cache;
}
}