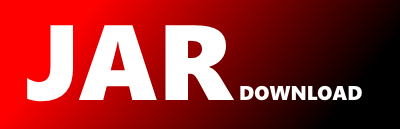
org.neo4j.kernel.AbstractAutoIndexerImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neo4j-kernel Show documentation
Show all versions of neo4j-kernel Show documentation
Neo4j kernel is a lightweight, embedded Java database designed to
store data structured as graphs rather than tables. For more
information, see http://neo4j.org.
/**
* Copyright (c) 2002-2013 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.kernel;
import java.util.Collections;
import java.util.HashSet;
import java.util.Set;
import java.util.StringTokenizer;
import org.neo4j.graphdb.GraphDatabaseService;
import org.neo4j.graphdb.PropertyContainer;
import org.neo4j.graphdb.index.AutoIndexer;
import org.neo4j.graphdb.index.Index;
import org.neo4j.graphdb.index.IndexHits;
import org.neo4j.graphdb.index.ReadableIndex;
import org.neo4j.kernel.lifecycle.Lifecycle;
/**
* Default implementation of the AutoIndexer, binding to the beforeCommit hook
* as a TransactionEventHandler
*
* @param The database primitive type auto indexed
*/
abstract class AbstractAutoIndexerImpl implements
PropertyTracker, AutoIndexer, Lifecycle
{
protected final Set propertyKeysToInclude = new HashSet();
private volatile boolean enabled;
public AbstractAutoIndexerImpl( )
{
}
public void propertyAdded( T primitive, String propertyName,
Object propertyValue )
{
if ( propertyKeysToInclude.contains( propertyName ) )
{
getIndexInternal().add( primitive, propertyName, propertyValue );
}
}
public void propertyChanged( T primitive, String propertyName,
Object oldValue, Object newValue )
{
if ( oldValue != null )
{
getIndexInternal().remove( primitive, propertyName, oldValue );
}
if ( propertyKeysToInclude.contains( propertyName ) )
{
getIndexInternal().add( primitive, propertyName, newValue );
}
}
public void propertyRemoved( T primitive, String propertyName,
Object propertyValue )
{
getIndexInternal().remove( primitive, propertyName );
}
@Override
public ReadableIndex getAutoIndex()
{
return new IndexWrapper( getIndexInternal() );
}
public void setEnabled( boolean enabled )
{
this.enabled = enabled;
}
@Override
public boolean isEnabled()
{
return enabled;
}
@Override
public void startAutoIndexingProperty( String propName )
{
propertyKeysToInclude.add(propName);
}
@Override
public void stopAutoIndexingProperty( String propName )
{
propertyKeysToInclude.remove( propName );
}
@Override
public Set getAutoIndexedProperties()
{
return Collections.unmodifiableSet( propertyKeysToInclude );
}
/**
* Returns the actual index used by the auto indexer. This is not supposed
* to
* leak unprotected to the outside world.
*
* @return The Index used by this AutoIndexer
*/
protected abstract Index getIndexInternal();
protected Set parseConfigList(String list)
{
if ( list == null )
{
return Collections.emptySet();
}
Set toReturn = new HashSet();
StringTokenizer tokenizer = new StringTokenizer(list, "," );
String currentToken;
while ( tokenizer.hasMoreTokens() )
{
currentToken = tokenizer.nextToken();
if ( ( currentToken = currentToken.trim() ).length() > 0 )
{
toReturn.add( currentToken );
}
}
return toReturn;
}
/**
* Simple implementation of the AutoIndex interface, as a wrapper around a
* normal Index that exposes the read-only operations.
*
* @param The type of database primitive this index holds
*/
private static class IndexWrapper implements
ReadableIndex
{
private final Index delegate;
IndexWrapper( Index delegate )
{
this.delegate = delegate;
}
@Override
public String getName()
{
return delegate.getName();
}
@Override
public Class getEntityType()
{
return delegate.getEntityType();
}
@Override
public IndexHits get( String key, Object value )
{
return delegate.get( key, value );
}
@Override
public IndexHits query( String key, Object queryOrQueryObject )
{
return delegate.query( key, queryOrQueryObject );
}
@Override
public IndexHits query( Object queryOrQueryObject )
{
return delegate.query( queryOrQueryObject );
}
@Override
public boolean isWriteable()
{
return false;
}
@Override
public GraphDatabaseService getGraphDatabase()
{
return delegate.getGraphDatabase();
}
}
/**
* A simple wrapper that makes a read only index into a read-write
* index with the unsupported operations throwing
* UnsupportedOperationException
* Useful primarily for returning the actually read-write but
* publicly read-only auto indexes from the get by name methods of the index
* manager.
*/
static class ReadOnlyIndexToIndexAdapter
implements Index
{
private final ReadableIndex delegate;
public ReadOnlyIndexToIndexAdapter( ReadableIndex delegate )
{
this.delegate = delegate;
}
@Override
public String getName()
{
return delegate.getName();
}
@Override
public Class getEntityType()
{
return delegate.getEntityType();
}
@Override
public IndexHits get( String key, Object value )
{
return delegate.get( key, value );
}
@Override
public IndexHits query( String key, Object queryOrQueryObject )
{
return delegate.query( key, queryOrQueryObject );
}
@Override
public IndexHits query( Object queryOrQueryObject )
{
return delegate.query( queryOrQueryObject );
}
private UnsupportedOperationException readOnlyIndex()
{
return new UnsupportedOperationException( "read only index" );
}
@Override
public void add( T entity, String key, Object value )
{
throw readOnlyIndex();
}
@Override
public T putIfAbsent( T entity, String key, Object value )
{
throw readOnlyIndex();
}
@Override
public void remove( T entity, String key, Object value )
{
throw readOnlyIndex();
}
@Override
public void remove( T entity, String key )
{
throw readOnlyIndex();
}
@Override
public void remove( T entity )
{
throw readOnlyIndex();
}
@Override
public void delete()
{
throw readOnlyIndex();
}
@Override
public boolean isWriteable()
{
return false;
}
@Override
public GraphDatabaseService getGraphDatabase()
{
return delegate.getGraphDatabase();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy