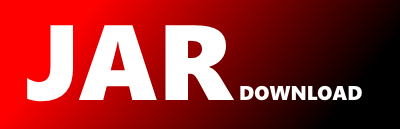
org.neo4j.helpers.Predicates Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neo4j-kernel Show documentation
Show all versions of neo4j-kernel Show documentation
Neo4j kernel is a lightweight, embedded Java database designed to
store data structured as graphs rather than tables. For more
information, see http://neo4j.org.
/*
* Copyright (c) 2002-2015 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.helpers;
import java.util.Arrays;
import java.util.Collection;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import org.neo4j.helpers.collection.Iterables;
/**
* Common predicates
*/
public class Predicates
{
public static Predicate TRUE()
{
return new Predicate()
{
@Override
public boolean accept( T instance )
{
return true;
}
};
}
public static Predicate not( final Predicate specification )
{
return new Predicate()
{
@Override
public boolean accept( T instance )
{
return !specification.accept( instance );
}
};
}
public static AndPredicate and( final Predicate... predicates )
{
return and( Arrays.asList( predicates ) );
}
public static AndPredicate and( final Iterable> predicates )
{
return new AndPredicate( predicates );
}
public static OrPredicate or( final Predicate... predicates )
{
return or( Arrays.asList( predicates ) );
}
public static OrPredicate or( final Iterable> predicates )
{
return new OrPredicate( predicates );
}
public static Predicate equalTo( final T allowed )
{
return new Predicate()
{
@Override
public boolean accept( T item )
{
return allowed == null ? item == null : allowed.equals( item );
}
};
}
public static Predicate in( final T... allowed )
{
return in( Arrays.asList( allowed ) );
}
public static Predicate in( final Iterable allowed )
{
return new Predicate()
{
@Override
public boolean accept( T item )
{
for ( T allow : allowed )
{
if ( allow.equals( item ) )
{
return true;
}
}
return false;
}
};
}
public static Predicate in( final Collection allowed )
{
return new Predicate()
{
@Override
public boolean accept( T item )
{
return allowed.contains( item );
}
};
}
@SuppressWarnings( "rawtypes" )
private static Predicate NOT_NULL = new Predicate()
{
@Override
public boolean accept( Object item )
{
return item != null;
}
};
@SuppressWarnings( "unchecked" )
public static Predicate notNull()
{
return NOT_NULL;
}
public static Predicate translate( final Function function,
final Predicate super TO> specification )
{
return new Predicate()
{
@Override
public boolean accept( FROM item )
{
return specification.accept( function.apply( item ) );
}
};
}
public static void await( Provider provider, Predicate predicate, long timeout, TimeUnit unit )
throws TimeoutException, InterruptedException
{
long sleep = Math.max( unit.toMillis( timeout ) / 100, 1 );
long deadline = System.currentTimeMillis() + unit.toMillis( timeout );
do
{
if ( predicate.accept( provider.instance() ) )
{
return;
}
Thread.sleep( sleep );
}
while ( System.currentTimeMillis() < deadline );
throw new TimeoutException( "Waited for " + timeout + " " + unit + ", but " + predicate + " was not accepted." );
}
public static class AndPredicate implements Predicate
{
private final Iterable> predicates;
private AndPredicate( Iterable> predicates )
{
this.predicates = predicates;
}
@Override
public boolean accept( T instance )
{
for ( Predicate specification : predicates )
{
if ( !specification.accept( instance ) )
{
return false;
}
}
return true;
}
public AndPredicate and( Predicate... predicates )
{
Iterable> iterable = Iterables.iterable( predicates );
Iterable> flatten = Iterables.flatten( this.predicates, iterable );
return Predicates.and( flatten );
}
public OrPredicate or( Predicate... predicates )
{
return Predicates.or( Iterables.prepend( this, Arrays.asList( predicates ) ) );
}
}
public static class OrPredicate implements Predicate
{
private final Iterable> predicates;
private OrPredicate( Iterable> predicates )
{
this.predicates = predicates;
}
@Override
public boolean accept( T instance )
{
for ( Predicate specification : predicates )
{
if ( specification.accept( instance ) )
{
return true;
}
}
return false;
}
public AndPredicate and( Predicate... predicates )
{
return Predicates.and( Iterables.prepend( this, Arrays.asList( predicates ) ) );
}
public OrPredicate or( Predicate... predicates )
{
Iterable> iterable = Iterables.iterable( predicates );
Iterable> flatten = Iterables.flatten( this.predicates, iterable );
return Predicates.or( flatten );
}
}
public static Predicate stringContains( final String string )
{
return new Predicate()
{
@Override
public boolean accept( String item )
{
return item.contains( string );
}
};
}
public static Predicate instanceOf( final Class clazz)
{
return new Predicate()
{
@Override
public boolean accept( T item )
{
return item != null && clazz.isInstance( item );
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy