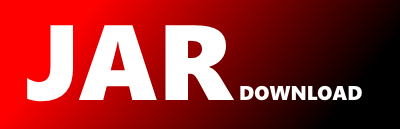
org.neo4j.helpers.collection.CollectionWrapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neo4j-kernel Show documentation
Show all versions of neo4j-kernel Show documentation
Neo4j kernel is a lightweight, embedded Java database designed to
store data structured as graphs rather than tables. For more
information, see http://neo4j.org.
/*
* Copyright (c) 2002-2015 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.helpers.collection;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
/**
* Wraps a {@link Collection}, making it look (and function) like a collection
* holding another type of items. The wrapper delegates to its underlying
* collection instead of keeping track of the items itself.
*
* @param the type of items
* @param the type of items of the underlying/wrapped collection
*/
public abstract class CollectionWrapper implements Collection
{
private Collection collection;
public CollectionWrapper( Collection underlyingCollection )
{
this.collection = underlyingCollection;
}
protected abstract U objectToUnderlyingObject( T object );
protected abstract T underlyingObjectToObject( U object );
public boolean add( T o )
{
return collection.add( objectToUnderlyingObject( o ) );
}
public void clear()
{
collection.clear();
}
public boolean contains( Object o )
{
return collection.contains( objectToUnderlyingObject( ( T ) o ) );
}
public boolean isEmpty()
{
return collection.isEmpty();
}
public Iterator iterator()
{
return new WrappingIterator( collection.iterator() );
}
public boolean remove( Object o )
{
return collection.remove( objectToUnderlyingObject( ( T ) o ) );
}
public int size()
{
return collection.size();
}
protected Collection convertCollection( Collection c )
{
Collection converted = new HashSet();
for ( Object item : c )
{
converted.add( objectToUnderlyingObject( ( T ) item ) );
}
return converted;
}
public boolean retainAll( Collection c )
{
return collection.retainAll( convertCollection( c ) );
}
public boolean addAll( Collection c )
{
return collection.addAll( convertCollection( c ) );
}
public boolean removeAll( Collection c )
{
return collection.removeAll( convertCollection( c ) );
}
public boolean containsAll( Collection c )
{
return collection.containsAll( convertCollection( c ) );
}
public Object[] toArray()
{
Object[] array = collection.toArray();
Object[] result = new Object[ array.length ];
for ( int i = 0; i < array.length; i++ )
{
result[ i ] = underlyingObjectToObject( ( U ) array[ i ] );
}
return result;
}
public R[] toArray( R[] a )
{
Object[] array = collection.toArray();
ArrayList result = new ArrayList();
for ( int i = 0; i < array.length; i++ )
{
result.add( ( R ) underlyingObjectToObject( ( U ) array[ i ] ) );
}
return result.toArray( a );
}
private class WrappingIterator extends IteratorWrapper
{
WrappingIterator( Iterator iterator )
{
super( iterator );
}
@Override
protected T underlyingObjectToObject( U object )
{
return CollectionWrapper.this.underlyingObjectToObject( object );
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy