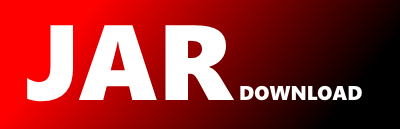
org.neo4j.kernel.impl.api.state.RelationshipState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neo4j-kernel Show documentation
Show all versions of neo4j-kernel Show documentation
Neo4j kernel is a lightweight, embedded Java database designed to
store data structured as graphs rather than tables. For more
information, see http://neo4j.org.
/*
* Copyright (c) 2002-2015 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.kernel.impl.api.state;
import java.util.Iterator;
import org.neo4j.cursor.Cursor;
import org.neo4j.function.Supplier;
import org.neo4j.helpers.collection.IteratorUtil;
import org.neo4j.kernel.api.EntityType;
import org.neo4j.kernel.api.cursor.PropertyItem;
import org.neo4j.kernel.api.properties.DefinedProperty;
import org.neo4j.kernel.impl.api.RelationshipVisitor;
import org.neo4j.kernel.impl.api.cursor.TxAllPropertyCursor;
import org.neo4j.kernel.impl.api.cursor.TxSinglePropertyCursor;
/**
* Represents the transactional changes to a relationship.
*
* @see PropertyContainerState
*/
public interface RelationshipState extends PropertyContainerState
{
long getId();
boolean accept( RelationshipVisitor visitor ) throws EX;
class Mutable extends PropertyContainerState.Mutable implements RelationshipState
{
private long startNode = -1;
private long endNode = -1;
private int type = -1;
private Mutable( long id )
{
super( id, EntityType.RELATIONSHIP );
}
public void setMetaData( long startNode, long endNode, int type )
{
this.startNode = startNode;
this.endNode = endNode;
this.type = type;
}
@Override
public boolean accept( RelationshipVisitor visitor ) throws EX
{
if ( type != -1 )
{
visitor.visit( getId(), type, startNode, endNode );
return true;
}
return false;
}
}
abstract class Defaults extends StateDefaults
{
@Override
Mutable createValue( Long id, TxState state )
{
return new Mutable( id );
}
@Override
RelationshipState defaultValue()
{
return DEFAULT;
}
private static final RelationshipState DEFAULT = new RelationshipState()
{
private UnsupportedOperationException notDefined( String field )
{
return new UnsupportedOperationException( field + " not defined" );
}
@Override
public long getId()
{
throw notDefined( "id" );
}
@Override
public boolean accept( RelationshipVisitor visitor ) throws EX
{
return false;
}
@Override
public Iterator addedProperties()
{
return IteratorUtil.emptyIterator();
}
@Override
public Iterator changedProperties()
{
return IteratorUtil.emptyIterator();
}
@Override
public Iterator removedProperties()
{
return IteratorUtil.emptyIterator();
}
@Override
public Iterator addedAndChangedProperties()
{
return IteratorUtil.emptyIterator();
}
@Override
public Iterator augmentProperties( Iterator iterator )
{
return iterator;
}
@Override
public Cursor augmentPropertyCursor( Supplier propertyCursor,
Cursor cursor )
{
return cursor;
}
@Override
public Cursor augmentSinglePropertyCursor( Supplier propertyCursor,
Cursor cursor,
int propertyKeyId )
{
return cursor;
}
@Override
public void accept( Visitor visitor )
{
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy