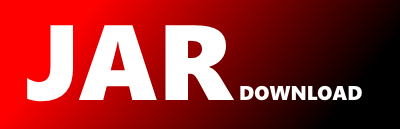
org.neo4j.kernel.impl.util.CappedOperation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neo4j-kernel Show documentation
Show all versions of neo4j-kernel Show documentation
Neo4j kernel is a lightweight, embedded Java database designed to
store data structured as graphs rather than tables. For more
information, see http://neo4j.org.
/*
* Copyright (c) 2002-2015 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.kernel.impl.util;
import java.util.concurrent.TimeUnit;
import org.neo4j.function.Predicate;
import org.neo4j.helpers.Clock;
public abstract class CappedOperation
{
public interface Switch extends Predicate
{
void reset();
}
private final Switch opener;
public CappedOperation( Switch... openers )
{
this.opener = firstOccurenceOf( openers );
}
public void event( T event )
{
if ( opener.test( event ) )
{
triggered( event );
}
}
protected abstract void triggered( T event );
@SuppressWarnings( "rawtypes" )
private static Switch firstOccurenceOf( final Switch... filters )
{
for ( Switch filter : filters )
{
filter.reset();
}
return new Switch()
{
@SuppressWarnings( "unchecked" )
@Override
public synchronized boolean test( T item )
{
boolean accepted = false;
// Pass it through all since they are probably stateful
for ( Switch filter : filters )
{
if ( filter.test( item ) )
{
accepted = true;
}
}
if ( accepted )
{
reset();
}
return accepted;
}
@SuppressWarnings( "unchecked" )
@Override
public synchronized void reset()
{
for ( Switch filter : filters )
{
filter.reset();
}
}
};
}
public static Switch time( final long time, TimeUnit unit )
{
return time( Clock.SYSTEM_CLOCK, time, unit );
}
public static Switch time( final Clock clock, long time, TimeUnit unit )
{
final long timeMillis = unit.toMillis( time );
return new Switch()
{
private long lastSeen = -1;
@Override
public boolean test( T item )
{
return clock.currentTimeMillis()-lastSeen >= timeMillis;
}
@Override
public void reset()
{
lastSeen = lastSeen == -1 ? 0 : clock.currentTimeMillis();
}
};
}
public static Switch count( final long maxCount )
{
return new Switch()
{
private long count;
@Override
public boolean test( T item )
{
return ++count >= maxCount;
}
@Override
public void reset()
{
count = 0;
}
};
}
public static Switch differentItems()
{
return new Switch()
{
private T lastSeenItem;
@Override
public boolean test( T item )
{
boolean accepted = lastSeenItem == null || !lastSeenItem.equals( item );
lastSeenItem = item;
return accepted;
}
@Override
public void reset()
{ // Don't reset
}
};
}
public static Switch differentItemClasses()
{
return new Switch()
{
private Class lastSeenItemClass;
@Override
public boolean test( T item )
{
boolean accepted = lastSeenItemClass == null || !lastSeenItemClass.equals( item.getClass() );
lastSeenItemClass = item.getClass();
return accepted;
}
@Override
public void reset()
{ // Don't reset
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy