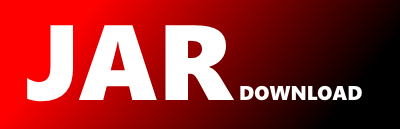
org.neo4j.index.impl.lucene.legacy.LuceneIndexImplementation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neo4j-lucene-index Show documentation
Show all versions of neo4j-lucene-index Show documentation
Integration layer between Neo4j and Lucene, providing one possible implementation of the Index API.
/*
* Copyright (c) 2002-2016 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.index.impl.lucene.legacy;
import java.io.File;
import java.io.IOException;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.function.Supplier;
import org.neo4j.graphdb.ResourceIterator;
import org.neo4j.graphdb.index.IndexManager;
import org.neo4j.helpers.collection.MapUtil;
import org.neo4j.io.fs.FileSystemAbstraction;
import org.neo4j.kernel.configuration.Config;
import org.neo4j.kernel.impl.api.TransactionApplier;
import org.neo4j.kernel.impl.index.IndexConfigStore;
import org.neo4j.kernel.lifecycle.LifecycleAdapter;
import org.neo4j.kernel.spi.legacyindex.IndexCommandFactory;
import org.neo4j.kernel.spi.legacyindex.IndexImplementation;
import org.neo4j.kernel.spi.legacyindex.LegacyIndexProviderTransaction;
public class LuceneIndexImplementation extends LifecycleAdapter implements IndexImplementation
{
static final String KEY_TYPE = "type";
static final String KEY_ANALYZER = "analyzer";
static final String KEY_TO_LOWER_CASE = "to_lower_case";
static final String KEY_SIMILARITY = "similarity";
public static final String SERVICE_NAME = "lucene";
public static final Map EXACT_CONFIG =
Collections.unmodifiableMap( MapUtil.stringMap(
IndexManager.PROVIDER, SERVICE_NAME, KEY_TYPE, "exact" ) );
public static final Map FULLTEXT_CONFIG =
Collections.unmodifiableMap( MapUtil.stringMap(
IndexManager.PROVIDER, SERVICE_NAME, KEY_TYPE, "fulltext",
KEY_TO_LOWER_CASE, "true" ) );
private LuceneDataSource dataSource;
private final File storeDir;
private final Config config;
private final Supplier indexStore;
private final FileSystemAbstraction fileSystemAbstraction;
public LuceneIndexImplementation( File storeDir, Config config, Supplier indexStore,
FileSystemAbstraction fileSystemAbstraction )
{
this.storeDir = storeDir;
this.config = config;
this.indexStore = indexStore;
this.fileSystemAbstraction = fileSystemAbstraction;
}
@Override
public void init() throws Throwable
{
this.dataSource = new LuceneDataSource( storeDir, config, indexStore.get(), fileSystemAbstraction );
this.dataSource.init();
}
@Override
public void start() throws Throwable
{
this.dataSource.start();
}
@Override
public void stop() throws Throwable
{
this.dataSource.stop();
}
@Override
public void shutdown() throws Throwable
{
this.dataSource.shutdown();
this.dataSource = null;
}
@Override
public File getIndexImplementationDirectory(File storeDir)
{
return LuceneDataSource.getLuceneIndexStoreDirectory(storeDir);
}
@Override
public LegacyIndexProviderTransaction newTransaction( IndexCommandFactory commandFactory )
{
return new LuceneLegacyIndexTransaction( dataSource, commandFactory );
}
@Override
public Map fillInDefaults( Map source )
{
Map result = source != null ?
new HashMap<>( source ) : new HashMap<>();
String analyzer = result.get( KEY_ANALYZER );
if ( analyzer == null )
{
// Type is only considered if "analyzer" isn't supplied
String type = result.get( KEY_TYPE );
if ( type == null )
{
type = "exact";
result.put( KEY_TYPE, type );
}
if ( type.equals( "fulltext" ) &&
!result.containsKey( LuceneIndexImplementation.KEY_TO_LOWER_CASE ) )
{
result.put( KEY_TO_LOWER_CASE, "true" );
}
}
// Try it on for size. Calling this will reveal configuration problems.
IndexType.getIndexType( result );
return result;
}
@Override
public boolean configMatches( Map storedConfig, Map config )
{
return match( storedConfig, config, KEY_TYPE, null ) &&
match( storedConfig, config, KEY_TO_LOWER_CASE, "true" ) &&
match( storedConfig, config, KEY_ANALYZER, null ) &&
match( storedConfig, config, KEY_SIMILARITY, null );
}
private boolean match( Map storedConfig, Map config,
String key, String defaultValue )
{
String value1 = storedConfig.get( key );
String value2 = config.get( key );
if ( value1 == null || value2 == null )
{
if ( value1 == value2 )
{
return true;
}
if ( defaultValue != null )
{
value1 = value1 != null ? value1 : defaultValue;
value2 = value2 != null ? value2 : defaultValue;
return value1.equals( value2 );
}
}
else
{
return value1.equals( value2 );
}
return false;
}
@Override
public TransactionApplier newApplier( boolean recovery )
{
return new LuceneCommandApplier( dataSource, recovery );
}
@Override
public ResourceIterator listStoreFiles() throws IOException
{
return dataSource.listStoreFiles();
}
@Override
public void force()
{
dataSource.force();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy