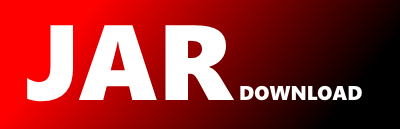
org.neo4j.ogm.drivers.bolt.response.BoltGraphRowModelAdapter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neo4j-ogm-bolt-driver Show documentation
Show all versions of neo4j-ogm-bolt-driver Show documentation
Neo4j-OGM transport that uses the official Java-Driver to connect to Neo4j via the Bolt-protocol.
/*
* Copyright (c) 2002-2016 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* This product is licensed to you under the Apache License, Version 2.0 (the "License").
* You may not use this product except in compliance with the License.
*
* This product may include a number of subcomponents with
* separate copyright notices and license terms. Your use of the source
* code for these subcomponents is subject to the terms and
* conditions of the subcomponent's license, as noted in the LICENSE file.
*/
package org.neo4j.ogm.drivers.bolt.response;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.neo4j.ogm.exception.ResultProcessingException;
import org.neo4j.ogm.model.GraphModel;
import org.neo4j.ogm.model.GraphRowModel;
import org.neo4j.ogm.response.model.DefaultGraphModel;
import org.neo4j.ogm.response.model.DefaultGraphRowModel;
import org.neo4j.ogm.response.model.DefaultRowModel;
import org.neo4j.ogm.result.adapter.AdapterUtils;
import org.neo4j.ogm.result.adapter.GraphRowModelAdapter;
/**
* @author Luanne Misquitta
*/
public class BoltGraphRowModelAdapter extends GraphRowModelAdapter {
private BoltGraphModelAdapter graphModelAdapter;
private List columns = new ArrayList<>();
public BoltGraphRowModelAdapter(BoltGraphModelAdapter graphModelAdapter) {
super(graphModelAdapter);
this.graphModelAdapter = graphModelAdapter;
}
@Override
public GraphRowModel adapt(Map data) {
if (columns == null) {
throw new ResultProcessingException("Column data cannot be null!");
}
Set nodeIdentities = new HashSet<>();
Set edgeIdentities = new HashSet<>();
GraphModel graphModel = new DefaultGraphModel();
List variables = new ArrayList<>();
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy