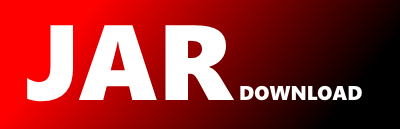
org.neo4j.ext.udc.impl.UdcKernelExtensionFactory Maven / Gradle / Ivy
/*
* Copyright (c) 2002-2016 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.ext.udc.impl;
import java.util.HashMap;
import java.util.Map;
import java.util.Timer;
import org.neo4j.ext.udc.UdcSettings;
import org.neo4j.helpers.Service;
import org.neo4j.helpers.collection.MapUtil;
import org.neo4j.kernel.configuration.Config;
import org.neo4j.kernel.extension.KernelExtensionFactory;
import org.neo4j.kernel.impl.core.StartupStatistics;
import org.neo4j.kernel.impl.spi.KernelContext;
import org.neo4j.kernel.impl.store.id.IdGeneratorFactory;
import org.neo4j.kernel.impl.transaction.state.DataSourceManager;
import org.neo4j.kernel.lifecycle.Lifecycle;
import org.neo4j.udc.UsageData;
/**
* Kernel extension for UDC, the Usage Data Collector. The UDC runs as a background
* daemon, waking up once a day to collect basic usage information about a long
* running graph database.
*
* The first update is delayed to avoid needless activity during integration
* testing and short-run applications. Subsequent updates are made at regular
* intervals. Both times are specified in milliseconds.
*/
@Service.Implementation(KernelExtensionFactory.class)
public class UdcKernelExtensionFactory extends KernelExtensionFactory
{
static final String KEY = "kernel udc";
public interface Dependencies
{
Config config();
DataSourceManager dataSourceManager();
UsageData usageData();
IdGeneratorFactory idGeneratorFactory();
StartupStatistics startupStats();
}
public UdcKernelExtensionFactory()
{
super( KEY );
}
@Override
public Class getSettingsClass()
{
return UdcSettings.class;
}
@Override
public Lifecycle newInstance( KernelContext kernelContext, UdcKernelExtensionFactory.Dependencies dependencies )
throws Throwable
{
return new UdcKernelExtension(
dependencies.config().with( loadUdcProperties() ),
dependencies.dataSourceManager(),
dependencies.idGeneratorFactory(),
dependencies.startupStats(),
dependencies.usageData(),
new Timer( "Neo4j UDC Timer", isAlwaysDaemon() ) );
}
private boolean isAlwaysDaemon()
{
return true;
}
private Map loadUdcProperties()
{
try
{
return MapUtil.load( getClass().getResourceAsStream( "/org/neo4j/ext/udc/udc.properties" ) );
}
catch ( Exception e )
{
return new HashMap<>();
}
}
}