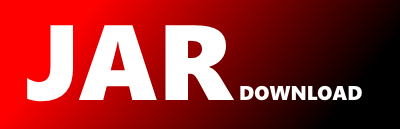
org.nerd4j.csv.field.CSVFieldProcessContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nerd4j-csv Show documentation
Show all versions of nerd4j-csv Show documentation
CSV manipulation library.
/*
* #%L
* Nerd4j CSV
* %%
* Copyright (C) 2013 Nerd4j
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nerd4j.csv.field;
import org.nerd4j.csv.CSVProcessContext;
import org.nerd4j.csv.CSVProcessError;
import org.nerd4j.csv.CSVProcessOperation;
/**
* Represents the execution context of the during
* the process of validating and converting fields.
*
*
* This context is used both during reading of a
* CSV source and during writing to a CSV target.
*
*
*
* This context keeps some information like:
*
* - The current row and column handled.
* - The original value of the field.
* - The value of the field after conversion.
*
*
*
* @author Nerd4j Team
*/
public final class CSVFieldProcessContext implements CSVProcessContext
{
/** Currently processed row index (0 based). */
private int rowIndex;
/** Currently processed column index (0 based). */
private int columnIndex;
/*
* Avoid to use generic types for value field
* because id makes the context uselessly
* complex to use.
*/
/** Value before processing. */
private Object originalValue;
/** Value after processing. */
private Object processedValue;
/** In case of failure contains the operator that has failed. */
private CSVProcessOperation failedOperation;
/** The CSV header containing the columns names. */
private String[] header;
/**
* Constructor with parameters.
*
* @param header the CSV header containing the columns names.
*/
public CSVFieldProcessContext( String[] header )
{
super();
this.header = header;
this.columnIndex = -1;
/* If the header has been read the row index starts at the second row. */
this.rowIndex = header == null ? -1 : 0;
this.originalValue = null;
this.processedValue = null;
this.failedOperation = null;
}
/* ******************* */
/* INTERFACE METHODS */
/* ******************* */
/**
* {@inheritDoc}
*/
@Override
public int getRowIndex()
{
return rowIndex;
}
/**
* {@inheritDoc}
*/
@Override
public int getColumnIndex()
{
return columnIndex;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isError()
{
return failedOperation != null;
}
/**
* {@inheritDoc}
*/
@Override
public CSVProcessError getError()
{
if( failedOperation != null )
return new CSVFieldProcessError( failedOperation, this );
else
return null;
}
/* ******************* */
/* GETTERS & SETTERS */
/* ******************* */
public Object getOriginalValue()
{
return originalValue;
}
public void setOriginalValue( final Object originalValue )
{
this.originalValue = originalValue;
}
public Object getProcessedValue()
{
return processedValue;
}
public void setProcessedValue( final Object processedValue )
{
this.processedValue = processedValue;
}
/* ***************** */
/* UTILITY METHODS */
/* ***************** */
/**
* Performs the steps needed to start a new row:
*
* - Increments the row count;
* - Clears the column count;
* - Clears previous values;
* - Clears previous errors:
*
*
*/
public void newRow()
{
++ this.rowIndex;
this.columnIndex = -1;
}
/**
* Performs the steps needed to process a new column:
*
* - Increments the column count;
* - Clears previous values;
* - Clears previous errors:
*
*
*/
public void newColumn()
{
++ this.columnIndex;
}
/**
* Returns the column name if exists otherwise
* returns the column index in string format.
*
* @return the column name.
*/
public String getColumnName()
{
String columnName = null;
if( header != null && columnIndex < header.length )
columnName = header[columnIndex];
return columnName != null ? columnName : String.valueOf( columnIndex );
}
/**
* Tells the context that the given operation
* has failed. This causes the {@link #isError()}
* method to return true
.
*
* @param failedOperation the operation that has failed.
*/
public void operationFailed( final CSVProcessOperation failedOperation )
{
this.failedOperation = failedOperation;
}
/**
* Clears the context errors.
*
*/
public void clear()
{
this.originalValue = null;
this.processedValue = null;
this.failedOperation = null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy