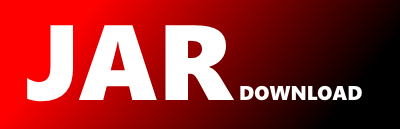
org.nerd4j.csv.writer.CSVWriterImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nerd4j-csv Show documentation
Show all versions of nerd4j-csv Show documentation
CSV manipulation library.
/*
* #%L
* Nerd4j CSV
* %%
* Copyright (C) 2013 Nerd4j
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nerd4j.csv.writer;
import java.io.IOException;
import org.nerd4j.csv.CSVProcessContext;
import org.nerd4j.csv.exception.CSVProcessException;
import org.nerd4j.csv.exception.ModelToCSVBindingException;
import org.nerd4j.csv.field.CSVField;
import org.nerd4j.csv.field.CSVFieldProcessContext;
import org.nerd4j.csv.formatter.CSVFormatter;
import org.nerd4j.csv.writer.binding.ModelToCSVBinder;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Reference implementation if the {@link CSVWriter} interface.
*
*
* Reads an object of type M that represents the
* data model and writes the corresponding CSV record
* to the given destination.
*
*
* @param type of the data model representing the CSV record.
*
* @author Nerd4J Team
*/
final class CSVWriterImpl implements CSVWriter
{
/** SLF4J Logging system. */
private static final Logger logger = LoggerFactory.getLogger( CSVWriterImpl.class );
/** Object able to format a source data into a CSV field. */
private final CSVFormatter formatter;
/** Object able to read the data model related to the CSV record. */
private final ModelToCSVBinder modelBinder;
/** Contains the fields used to manipulate data. */
private final CSVField
© 2015 - 2025 Weber Informatics LLC | Privacy Policy