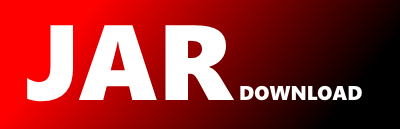
org.nerd4j.csv.writer.binding.ArrayToCSVBinderFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nerd4j-csv Show documentation
Show all versions of nerd4j-csv Show documentation
CSV manipulation library.
/*
* #%L
* Nerd4j CSV
* %%
* Copyright (C) 2013 Nerd4j
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nerd4j.csv.writer.binding;
import org.nerd4j.csv.exception.ModelToCSVBindingException;
import org.nerd4j.csv.field.CSVFieldMetadata;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Represents a Factory
able to build and configure
* {@link ModelToCSVBinder}s which reads data models and provides
* CSV record fields in the right order.
*
* @author Nerd4j Team
*/
public final class ArrayToCSVBinderFactory extends AbstractModelToCSVBinderFactory
© 2015 - 2025 Weber Informatics LLC | Privacy Policy