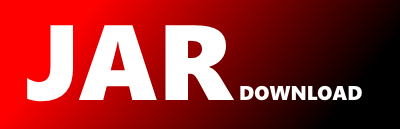
org.netbeans.api.autoupdate.InstallSupport Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.api.autoupdate;
import org.netbeans.api.autoupdate.OperationSupport.Restarter;
import org.netbeans.api.progress.ProgressHandle;
import org.netbeans.modules.autoupdate.services.InstallSupportImpl;
/**
* Performs all operations scheduled on instance of OperationContainer
.
* Instance of InstallSupport
can be obtained by calling {@link OperationContainer#getSupport}
*
*
* Typical scenario how to use:
*
* - Use instance of the
OperationContainer
created for chosen
* operation: {@link OperationContainer#createForInstall} or {@link OperationContainer#createForUninstall} and contained
* correct UpdateElement
s. See {@link OperationContainer}
* - Call the {@link #doDownload} for downloading install data.
* - Call the {@link #doValidate} for verify consistency of downloaded data.
* - Call the {@link #doInstall} for install contained
UpdateElement
.
* - If application restart is required for completing the Install/Update operation
* then call {@link #doRestart} or {@link #doRestartLater}.
*
*
* Code example:
*
* UpdateElement element = ...;
* OperationContainer<InstallSupport> container = createForInstall();
* ... add elements ...
* InstallSupport support = container.getSupport();
* Validator v = support.doDownload(null, false);
* Installer i = support.doValidate(v, null);
* Restarter r = support.doInstall(i, null);
* if (r != null) {
* support.doRestart(r, null);
* }
*
*
* @author Radek Matous, Jiri Rechtacek
*/
public final class InstallSupport {
InstallSupport () {
impl = new InstallSupportImpl (this);
}
/** Downloads all instances i.e. UpdateElement
s in corresponding OperationContainer
.
*
* @param progress ProgressHandle for notification progress in downloading, can be null
* @param isGlobal if true
then forces download instances into shared directories i.e. installation directory
* @return Validator
an instance of Validator which allows to verify downloaded instances in the next step
* @throws org.netbeans.api.autoupdate.OperationException
* @deprecated Use {@link #doDownload(ProgressHandle, Boolean, boolean)} instead.
*/
@Deprecated
public Validator doDownload(ProgressHandle progress/*or null*/, boolean isGlobal) throws OperationException {
if (impl.doDownload (progress, isGlobal ? Boolean.TRUE : null, false)) {
return new Validator ();
} else {
return null;
}
}
/** Downloads all instances i.e. UpdateElement
s in corresponding OperationContainer
.
*
* @param progress ProgressHandle for notification progress in downloading, can be null
* @param isGlobal if true
then forces download plugins into shared directories i.e. installation directory,
* if false
then download plugins into userdir
. If null
then download plugins in a default place.
* @param useUserdirAsFallback if true
then download plugins into userdir if no permission to write in shared directories
* @return Validator
an instance of Validator which allows to verify downloaded instances in the next step
* @throws org.netbeans.api.autoupdate.OperationException
* @since 1.33
*/
public Validator doDownload(ProgressHandle progress/*or null*/, Boolean isGlobal/*or null*/, boolean useUserdirAsFallback) throws OperationException {
if (impl.doDownload (progress, isGlobal, useUserdirAsFallback)) {
return new Validator ();
} else {
return null;
}
}
/** Validates all instances that have been downloaded in the previous step.
*
* @param validator an instance of Validator
that has been returned by {link @doDownload}. Mustn't be null.
* @param progress ProgressHandle for notification progress in validation, can be null
* @return Installer
an instance of Installer which allows to install all verified instances
* @throws org.netbeans.api.autoupdate.OperationException
* @see #doDownload
*/
public Installer doValidate(Validator validator, ProgressHandle progress/*or null*/) throws OperationException {
if (impl.doValidate (validator, progress)) {
return new Installer ();
} else {
return null;
}
}
/** Validates all instances that have been verified in the previous step.
*
* @param installer an instance of Installer
that has been returned by InstallSupport#doValidate. Mustn't be null.
* @param progress ProgressHandle for notification progress in installation, can be null
* @return Restarter
an instance of Restart if application restart is required for complete the install operation, or null
* @throws org.netbeans.api.autoupdate.OperationException
* @see #doValidate
*/
public Restarter doInstall(Installer installer ,ProgressHandle progress/*or null*/) throws OperationException {
Boolean restart = impl.doInstall (installer, progress, false);
if (restart == null /*was problem*/ || ! restart.booleanValue ()) {
return null;
} else {
return new OperationSupport.Restarter ();
}
}
/**
* Cancels changes done in previous calling methods.
* @throws org.netbeans.api.autoupdate.OperationException
* @see OperationException
*/
public void doCancel() throws OperationException {
// finds and deletes possible downloaded files
impl.doCancel ();
}
/**
* Completes the operation, applies all changes and ensures restart of the application immediately.
* If method {@link #doInstall} returns non null instance of Restarter
then
* this method must be called to apply all changes.
* @param restarter instance of Restarter
obtained from previous call {@link #doInstall}. Mustn't be null.
* @param progress instance of {@link ProgressHandle} or null
* @throws org.netbeans.api.autoupdate.OperationException
* @see OperationException
*/
public void doRestart(Restarter restarter,ProgressHandle progress/*or null*/) throws OperationException {
impl.doRestart (restarter, progress);
}
/**
* Finishes operation, all the changes will be completed after restart the application.
* If method {@link #doInstall} returns non null instance of Restarter
then
* this method must be called to apply all changes
* @param restarter instance of Restarter
obtained from previous call {@link #doInstall}.
* Mustn't be null.
*/
public void doRestartLater(Restarter restarter) {
impl.doRestartLater(restarter);
}
/** Returns java.security.cert.Certificate.toString() of given UpdateElement
.
*
* @param validator Installer
an instance of Installer has been returned by {link @doValidate}
* @param uElement UpdateElement
* @return content of UpdateElement's certificate
* @see #doValidate
*/
public String getCertificate(Installer validator, UpdateElement uElement) {
return impl.getCertificate (validator, uElement);
}
/** Returns if the UpdateElement
is trusted or not.
*
* @param validator Installer
an instance of Installer has been returned by {link @doValidate}
* @param uElement UpdateElement
* @return true for trusted UpdateElement
* @see #doValidate
* @see java.security.cert.Certificate
*/
public boolean isTrusted(Installer validator, UpdateElement uElement) {
return impl.isTrusted(validator, uElement);
}
/** Returns if the UpdateElement
is signed or not.
*
* @param validator Installer
an instance of Installer has been returned by {link @doValidate}
* @param uElement UpdateElement
* @return true for signed UpdateElement
* @see java.security.cert.Certificate
* @see #doValidate
*/
public boolean isSigned(Installer validator, UpdateElement uElement) {
return impl.isSignedVerified(validator, uElement) || impl.isSignedUnverified(validator, uElement);
}
/** Returns if the UpdateElement
is signed and verified or not.
*
* @param validator Installer
an instance of Installer has been returned by {link @doValidate}
* @param uElement UpdateElement
* @return true for signed and verified UpdateElement
* @see java.security.cert.Certificate
* @see #doValidate
* @since 1.50
*/
public boolean isSignedVerified(Installer validator, UpdateElement uElement) {
return impl.isSignedVerified(validator, uElement);
}
/** Returns if the UpdateElement
is signed but not verified or not.
*
* @param validator Installer
an instance of Installer has been returned by {link @doValidate}
* @param uElement UpdateElement
* @return true for signed but not verified UpdateElement
* @see java.security.cert.Certificate
* @see #doValidate
* @since 1.50
*/
public boolean isSignedUnverified(Installer validator, UpdateElement uElement) {
return impl.isSignedUnverified(validator, uElement);
}
/** Returns if the UpdateElement
is modified or not.
*
* @param validator Installer
an instance of Installer has been returned by {link @doValidate}
* @param uElement UpdateElement
* @return true for modified UpdateElement
* @see java.security.cert.Certificate
* @see #doValidate
* @since 1.50
*/
public boolean isContentModified(Installer validator, UpdateElement uElement) {
return impl.isContentModified(validator, uElement);
}
/** Returns the corresponing OperationContainer
.
*
* @return the OperationContainer
*/
public OperationContainer getContainer() {return container;}
/** A helper object returned by a {@link #doDownload} for invoke
* the method {@link #doValidate}
*
*/
public static final class Validator {private Validator() {}}
/** A helper object returned by a {@link #doValidate} for invoke
* the method {@link #doInstall}
*
*/
public static final class Installer {private Installer() {}}
//end of API - next just impl details
private OperationContainer container;
void setContainer(OperationContainer c) {container = c;}
InstallSupportImpl impl;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy