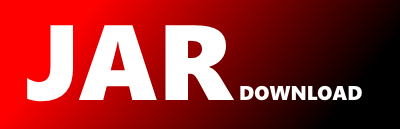
org.netbeans.api.autoupdate.OperationContainer Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.api.autoupdate;
import java.io.File;
import java.util.Collection;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.netbeans.modules.autoupdate.services.OperationContainerImpl;
import org.openide.modules.ModuleInfo;
/**
* An object that keeps requests for operations upon instances of UpdateEelement
* (like install, uninstall, update, enable, disable), provides checks whether
* chosen operation is allowed (e.g. already installed plugin cannot be scheduled for install again),
* provides information which additional plugins are
* required and so on.
*
* Typical scenario how to use:
*
* - use one of factory methods for creating instance of
OperationContainer
* for chosen operation: {@link #createForInstall}, {@link #createForUninstall},
* {@link #createForUpdate}, {@link #createForEnable},{@link #createForDisable}
* - add instances of
UpdateElement
(see {@link OperationContainer#add})
* - check if additional required instances of
UpdateElement
are needed
* ({@link OperationInfo#getRequiredElements}),
* if so then these required instances should be also added
* - next can be tested for broken dependencies ({@link OperationInfo#getBrokenDependencies})
* - call method {@link #getSupport} to get either {@link InstallSupport} or {@link OperationSupport}
* that can be used for performing operation
*
*
* Code example:
*
* UpdateElement element = ...;
* OperationContainer<OperationSupport> container = createForDirectInstall();
* OperationInfo<Support> info = container.add(element);
* Set<UpdateElement> required = info.getRequiredElements();
* container.add(required);
* OperationSupport support = container.getSupport();
* support.doOperation(null);
*
*
* @param the type of support for performing chosen operation like
* {@link OperationSupport} or {@link InstallSupport}
* @author Radek Matous, Jiri Rechtacek
*/
public final class OperationContainer {
/**
* The factory method to construct instance of OperationContainer
for install operation
* @return newly constructed instance of OperationContainer
for install operation
*/
public static OperationContainer createForInstall() {
OperationContainer retval =
new OperationContainer(OperationContainerImpl.createForInstall(), new InstallSupport());
retval.getSupportInner ().setContainer(retval);
return retval;
}
/**
* The factory method to construct instance of OperationContainer
for internal update operation
* @return newly constructed instance of OperationContainer
for internal update operation
* @since 1.11
*/
public static OperationContainer createForInternalUpdate() {
OperationContainer retval =
new OperationContainer(OperationContainerImpl.createForInternalUpdate(), new InstallSupport());
retval.getSupportInner ().setContainer(retval);
return retval;
}
/**
* The factory method to construct instance of OperationContainer
for install operation
* @return newly constructed instance of OperationContainer
for install operation
*/
public static OperationContainer createForDirectInstall() {
OperationContainer retval =
new OperationContainer (OperationContainerImpl.createForDirectInstall(), new OperationSupport());
retval.getSupportInner ().setContainer(retval);
return retval;
}
/**
* The factory method to construct instance of OperationContainer
for update operation
* @return newly constructed instance of OperationContainer
for update operation
*/
public static OperationContainer createForUpdate() {
OperationContainer retval =
new OperationContainer(OperationContainerImpl.createForUpdate(), new InstallSupport());
retval.getSupportInner ().setContainer(retval);
return retval;
}
/**
* The factory method to construct instance of OperationContainer
for update operation
* @return newly constructed instance of OperationContainer
for update operation
*/
public static OperationContainer createForDirectUpdate() {
OperationContainerImpl implContainerForDirectUpdate = OperationContainerImpl.createForDirectUpdate();
OperationContainer retval =
new OperationContainer(implContainerForDirectUpdate, new OperationSupport());
retval.getSupportInner ().setContainer(retval);
return retval;
}
/**
* The factory method to construct instance of OperationContainer
for uninstall operation
* @return newly constructed instance of OperationContainer
for uninstall operation
*/
public static OperationContainer createForUninstall() {
OperationContainer retval =
new OperationContainer(OperationContainerImpl.createForUninstall(), new OperationSupport());
retval.getSupportInner ().setContainer(retval);
return retval;
}
/**
* The factory method to construct instance of OperationContainer
for uninstall operation
* @return newly constructed instance of OperationContainer
for uninstall operation
*/
public static OperationContainer createForDirectUninstall() {
OperationContainer retval =
new OperationContainer(OperationContainerImpl.createForDirectUninstall(), new OperationSupport());
retval.getSupportInner ().setContainer(retval);
return retval;
}
/**
* The factory method to construct instance of OperationContainer
for enable operation
* @return newly constructed instance of OperationContainer
for enable operation
*/
public static OperationContainer createForEnable() {
OperationContainer retval =
new OperationContainer(OperationContainerImpl.createForEnable(), new OperationSupport());
retval.getSupportInner ().setContainer(retval);
return retval;
}
/**
* The factory method to construct instance of OperationContainer
for disable operation
* @return newly constructed instance of OperationContainer
for disable operation
*/
public static OperationContainer createForDisable() {
OperationContainer retval =
new OperationContainer(OperationContainerImpl.createForDisable(), new OperationSupport());
retval.getSupportInner ().setContainer(retval);
return retval;
}
/**
* The factory method to construct instance of OperationContainer
for disable operation
* @return newly constructed instance of OperationContainer
for disable operation
*/
public static OperationContainer createForDirectDisable() {
OperationContainer retval =
new OperationContainer(OperationContainerImpl.createForDirectDisable(), new OperationSupport());
retval.getSupportInner ().setContainer(retval);
return retval;
}
/**
* The factory method to construct instance of OperationContainer
for installation of custom component
* @return newly constructed instance of OperationContainer
for installation of custom component
*/
public static OperationContainer createForCustomInstallComponent () {
OperationContainer retval =
new OperationContainer (OperationContainerImpl.createForInstallNativeComponent (), new OperationSupport());
retval.getSupportInner ().setContainer (retval);
return retval;
}
/**
* The factory method to construct instance of OperationContainer
for uninstallation of custom component
* @return newly constructed instance of OperationContainer
for uninstallation of custom component
*/
public static OperationContainer createForCustomUninstallComponent () {
OperationContainer retval =
new OperationContainer (OperationContainerImpl.createForUninstallNativeComponent (), new OperationSupport());
retval.getSupportInner ().setContainer (retval);
return retval;
}
/**
* @return either {@link OperationSupport} or {@link InstallSupport} depending on type parameter of OperationContainer<Support>
or
* null
if the OperationContainer
is empty or contains any invalid elements
* @see #listAll
* @see #listInvalid
*
See the difference between {@link #createForInstall} and {@link #createForDirectInstall} for example
*/
public Support getSupport() {
if (upToDate != null && upToDate) {
return support;
} else {
if (listAll().size() > 0 && listInvalid().isEmpty()) {
upToDate = true;
return support;
} else {
return null;
}
}
}
Support getSupportInner () {
return support;
}
/**
* Check if updateElement
can be added ({@link #add})
* @param updateUnit
* @param updateElement to be inserted.
* @return true if chosen operation upon updateElement
is allowed
*/
public boolean canBeAdded(UpdateUnit updateUnit, UpdateElement updateElement) {
return impl.isValid(updateUnit, updateElement);
}
/**
* Adds all elems
* @param elems to be inserted.
*/
public void add(Collection elems) {
if (elems == null) throw new IllegalArgumentException("Cannot add null value.");
for (UpdateElement el : elems) {
add(el);
}
}
/**
* Adds all elems
* @param elems to be inserted.
*/
public void add(Map elems) {
if (elems == null) throw new IllegalArgumentException ("Cannot add null value.");
for (Map.Entry entry : elems.entrySet()) {
add(entry.getKey(), entry.getValue());
}
}
/**
* Adds updateElement
* @param updateUnit
* @param updateElement
* @return instance of {@link OperationInfo}<Support> or
* null
if the UpdateElement
is already present in the container
*/
public OperationInfo add(UpdateUnit updateUnit,UpdateElement updateElement) {
upToDate = false;
return impl.add (updateUnit, updateElement);
}
/**
* Adds updateElement
* @param updateElement
* @return instance of {@link OperationInfo}<Support> or
* null
if the UpdateElement
is already present in the container
*/
public OperationInfo add(UpdateElement updateElement) {
upToDate = false;
UpdateUnit updateUnit = updateElement.getUpdateUnit ();
return add (updateUnit, updateElement);
}
/**
* Removes all elems
* @param elems
*/
public void remove(Collection elems) {
if (elems == null) throw new IllegalArgumentException ("Cannot add null value.");
for (UpdateElement el : elems) {
remove (el);
}
}
/**
* Removes updateElement
* @param updateElement
* @return true if successfully added
*/
public boolean remove(UpdateElement updateElement) {
if (upToDate != null) {
upToDate = false;
}
return impl.remove(updateElement);
}
/**
* @param updateElement
* @return true if this instance of OperationContainer
* contains the specified updateElement
.
*/
public boolean contains(UpdateElement updateElement) {
return impl.contains(updateElement);
}
/**
* @return all instances of {@link OperationInfo}<Support> from this
* instance of OperationContainer
*/
public List> listAll() {
return impl.listAllWithPossibleEager ();
}
/**
* @return all invalid instances of {@link OperationInfo}<Support> from this
* instance of OperationContainer
*/
public List> listInvalid() {
return impl.listInvalid ();
}
/**
* Removes op
* @param op
*/
public void remove(OperationInfo op) {
if (upToDate != null) {
upToDate = false;
}
impl.remove (op);
}
/**
* Removes all content
*/
public void removeAll() {
if (upToDate != null) {
upToDate = false;
}
impl.removeAll ();
}
/** Specifies location of unpack200 executable. {@code unpack200} has been
* removed from JDK 14. As such it is not possible to unpack older NBM
* files without providing alternative JDK implementation of this file.
*
* @param executable path to the executable
* @since 1.65
*/
public final void setUnpack200(File executable) {
this.impl.setUnpack200(executable);
}
/**
* Provides additional information
* @param the type of support for performing chosen operation like
*/
public static final class OperationInfo {
OperationContainerImpl.OperationInfoImpl impl;
OperationInfo (OperationContainerImpl.OperationInfoImpl impl) {
this.impl = impl;
}
public UpdateElement getUpdateElement() {return impl.getUpdateElement();}
public UpdateUnit getUpdateUnit() {return impl.getUpdateUnit();}
/**
* @return all required elements. Each of them represented by instance of UpdateElement
*/
public Set getRequiredElements(){return new LinkedHashSet (impl.getRequiredElements());}
/**
* @return all broken dependencies. Each of them represented by the code name of the module
* @see ModuleInfo#getCodeNameBase()
*/
public Set getBrokenDependencies(){return impl.getBrokenDependencies();}
/**
* Reports parts missing from the installation. Will return codenames of required
* unknown modules (e.g. from catalogs not fetched yet). Note differences to {@link #getBrokenDependencies()},
* which report also broken requirements for packages or java version for specialized / optional modules.
* @return set of missing parts (modules). If nothing is missing, returns empty set.
* @since 1.57
*/
public Set getMissingParts() { return impl.getMissingParts(); }
@Override
public String toString () {
return "OperationInfo: " + impl.getUpdateElement ().toString (); // NOI18N
}
}
//end of API - next just impl details
/** Creates a new instance of OperationContainer */
private OperationContainer(OperationContainerImpl impl, Support t) {
this.impl = impl;
this.support = t;
}
final OperationContainerImpl impl;
private final Support support;
private Boolean upToDate = null;
@Override
public String toString() {
return super.toString() + "+" + impl;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy