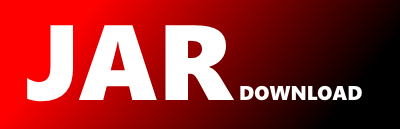
org.netbeans.modules.autoupdate.ui.Containers Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.modules.autoupdate.ui;
import java.io.File;
import java.lang.ref.Reference;
import java.lang.ref.WeakReference;
import org.netbeans.api.autoupdate.InstallSupport;
import org.netbeans.api.autoupdate.OperationContainer;
import org.netbeans.api.autoupdate.OperationSupport;
import org.openide.util.NbPreferences;
/**
*
* @author Radek Matous
*/
public class Containers {
private static Reference> INSTALL;
private static Reference> INTERNAL_UPDATE;
private static Reference> UPDATE;
private static Reference> INSTALL_FOR_NBMS;
private static Reference> UPDATE_FOR_NBMS;
private static Reference> UNINSTALL;
private static Reference> ENABLE;
private static Reference> DISABLE;
private static Reference> CUSTOM_INSTALL;
private static Reference> CUSTOM_UNINSTALL;
private Containers(){}
public static void initNotify() {
try {
forAvailableNbms().removeAll();
forUpdateNbms().removeAll();
forAvailable().removeAll();
forUninstall().removeAll();
forUpdate().removeAll();
forEnable().removeAll();
forDisable().removeAll();
forCustomInstall().removeAll();
forCustomUninstall().removeAll();
forInternalUpdate().removeAll();
} catch (NullPointerException npe) {
// doesn't matter, can ignore that
}
}
public static OperationContainer forAvailableNbms() {
synchronized(Containers.class) {
OperationContainer container = null;
if (INSTALL_FOR_NBMS != null) {
container = INSTALL_FOR_NBMS.get();
}
if (container==null) {
container = OperationContainer.createForInstall();
INSTALL_FOR_NBMS = new WeakReference>(container);
}
return useUnpack200(container);
}
}
public static OperationContainer forUpdateNbms() {
synchronized(Containers.class) {
OperationContainer container = null;
if (UPDATE_FOR_NBMS != null) {
container = UPDATE_FOR_NBMS.get();
}
if (container==null) {
container = OperationContainer.createForUpdate();
UPDATE_FOR_NBMS = new WeakReference>(container);
}
return useUnpack200(container);
}
}
public static OperationContainer forAvailable() {
synchronized(Containers.class) {
OperationContainer container = null;
if (INSTALL != null) {
container = INSTALL.get();
}
if (container == null) {
container = OperationContainer.createForInstall();
INSTALL = new WeakReference>(container);
}
return useUnpack200(container);
}
}
public static OperationContainer forUpdate() {
synchronized(Containers.class) {
OperationContainer container = null;
if (UPDATE != null) {
container = UPDATE.get();
}
if (container == null) {
container = OperationContainer.createForUpdate();
UPDATE = new WeakReference>(container);
}
return useUnpack200(container);
}
}
public static OperationContainer forUninstall() {
synchronized(Containers.class) {
OperationContainer container = null;
if (UNINSTALL != null) {
container = UNINSTALL.get();
}
if (container == null) {
container = OperationContainer.createForUninstall();
UNINSTALL = new WeakReference>(container);
}
return useUnpack200(container);
}
}
public static OperationContainer forEnable() {
synchronized(Containers.class) {
OperationContainer container = null;
if (ENABLE != null) {
container = ENABLE.get();
}
if(container == null) {
container = OperationContainer.createForEnable();
ENABLE = new WeakReference>(container);
}
return useUnpack200(container);
}
}
public static OperationContainer forDisable() {
synchronized(Containers.class) {
OperationContainer container = null;
if (DISABLE != null) {
container = DISABLE.get();
}
if(container == null) {
container = OperationContainer.createForDisable();
DISABLE = new WeakReference>(container);
}
return useUnpack200(container);
}
}
public static OperationContainer forCustomInstall () {
synchronized (Containers.class) {
OperationContainer container = null;
if (CUSTOM_INSTALL != null) {
container = CUSTOM_INSTALL.get ();
}
if(container == null) {
container = OperationContainer.createForCustomInstallComponent ();
CUSTOM_INSTALL = new WeakReference> (container);
}
return useUnpack200(container);
}
}
public static OperationContainer forCustomUninstall () {
synchronized (Containers.class) {
OperationContainer container = null;
if (CUSTOM_UNINSTALL != null) {
container = CUSTOM_UNINSTALL.get ();
}
if(container == null) {
container = OperationContainer.createForCustomUninstallComponent ();
CUSTOM_UNINSTALL = new WeakReference> (container);
}
return useUnpack200(container);
}
}
public static OperationContainer forInternalUpdate () {
synchronized (Containers.class) {
OperationContainer container = null;
if (INTERNAL_UPDATE != null) {
container = INTERNAL_UPDATE.get ();
}
if(container == null) {
container = OperationContainer.createForInternalUpdate();
INTERNAL_UPDATE = new WeakReference> (container);
}
return useUnpack200(container);
}
}
public static void defineUnpack200(File executable) {
NbPreferences.forModule(OperationContainer.class).put("unpack200", executable.getPath()); // NOI18N
INSTALL.clear();
INTERNAL_UPDATE.clear();
UPDATE.clear();
INSTALL_FOR_NBMS.clear();
UPDATE_FOR_NBMS.clear();
UNINSTALL.clear();
ENABLE.clear();
DISABLE.clear();
CUSTOM_INSTALL.clear();
CUSTOM_UNINSTALL.clear();
}
private static OperationContainer useUnpack200(OperationContainer container) {
String pack200 = NbPreferences.forModule(OperationContainer.class).get("unpack200", null); // NOI18N
if (pack200 != null) {
final File file = new File(pack200);
if (file.canExecute()) {
container.setUnpack200(file);
}
}
return container;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy