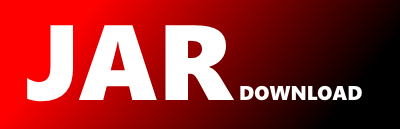
org.netbeans.modules.autoupdate.ui.UnitDetails Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.modules.autoupdate.ui;
import java.awt.Color;
import java.awt.Image;
import java.io.CharConversionException;
import java.net.URL;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.Action;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
import org.netbeans.api.autoupdate.UpdateElement;
import org.netbeans.api.autoupdate.UpdateUnit;
import org.openide.util.NbBundle;
import org.openide.util.RequestProcessor;
import org.openide.xml.XMLUtil;
/**
*
* @author Jiri Rechtacek
*/
public class UnitDetails extends DetailsPanel {
private static final Logger err = Logger.getLogger("org.netbeans.modules.autoupdate.ui.UnitDetails");
private RequestProcessor.Task unitDetailsTask = null;
static final RequestProcessor UNIT_DETAILS_PROCESSOR = new RequestProcessor("unit-details-processor", 1, true);
/** Creates a new instance of UnitDetails */
public UnitDetails() {
getAccessibleContext().setAccessibleName(org.openide.util.NbBundle.getMessage(UnitTable.class, "ACN_UnitDetails")); // NOI18N
}
public void setUnit(Unit u) {
setUnit(u, null);
}
public void setUnit(final Unit u, Action action) {
if (unitDetailsTask != null && !unitDetailsTask.isFinished()) {
unitDetailsTask.cancel();
}
if (u == null) {
getDetails().setText("" + getBundle("UnitDetails_Category_NoDescription") + ""); // NOI18N
setTitle(null);
} else {
try {
setTitle(XMLUtil.toElementContent(u.getDisplayName()));
} catch (CharConversionException e) {
err.log(Level.INFO, null, e);
return;
}
setActionListener(action);
setUnitText(u, getUnitText(u, false));
if (u instanceof Unit.Update) {
unitDetailsTask = UNIT_DETAILS_PROCESSOR.post(new Runnable() {
@Override
public void run() {
final StringBuilder text = getUnitText(u, true);
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
setUnitText(u, text);
}
});
}
});
}
}
}
private void buildUnitText(Unit u, StringBuilder text, boolean collectDependencies) {
if (u instanceof Unit.Available) {
Unit.Available u1 = (Unit.Available) u;
Image c = u1.getSourceIcon();
Object url = c.getProperty("url", null);
String categoryName = u1.getSourceDescription();
text.append("");
if (url instanceof URL) {
text.append(".append(url).append("\")
");
}
text.append(" ");
text.append(" ");
text.append("").append(categoryName).append(" ");
text.append("
");
}
if (Utilities.modulesOnly() || Utilities.showExtendedDescription()) {
text.append("").append(getBundle("UnitDetails_Plugin_CodeName")).append("").append(u.updateUnit.getCodeName()); // NOI18N
text.append("
");
}
String desc = null;
if (u instanceof Unit.Update) {
Unit.Update uu = ((Unit.Update) u);
text.append("").append(getBundle("UnitDetails_Plugin_InstalledVersion")).append("").append(uu.getInstalledVersion()).append("
"); // NOI18N
text.append("").append(getBundle("UnitDetails_Plugin_AvailableVersion")).append("").append(uu.getAvailableVersion()).append("
"); // NOI18N
desc = getDependencies(uu, collectDependencies);
} else {
text.append("").append(getBundle("UnitDetails_Plugin_Version")).append("").append(u.getDisplayVersion()).append("
"); // NOI18N
}
if (u.getAuthor() != null && u.getAuthor().length() > 0) {
text.append("").append(getBundle("UnitDetails_Plugin_Author")).append("").append(u.getAuthor()).append("
"); // NOI18N
}
if (u.getDisplayDate() != null && u.getDisplayDate().length() > 0) {
text.append("").append(getBundle("UnitDetails_Plugin_Date")).append("").append(u.getDisplayDate()).append("
"); // NOI18N
}
text.append("").append(getBundle("UnitDetails_Plugin_Source")).append("").append(u.getSource()).append("
"); // NOI18N
if (u.getHomepage() != null && u.getHomepage().length() > 0) {
text.append("").append(getBundle("UnitDetails_Plugin_Homepage")).append("").append(u.getHomepage()).append("
"); // NOI18N
}
if (u.getNotification() != null && u.getNotification().length() > 0) {
text.append("
").append(getBundle("UnitDetails_Plugin_Notification")).append("
"); // NOI18N
text.append(""); // NOI18N
text.append(u.getNotification());
text.append("
"); // NOI18N
}
if (u.getDescription() != null && u.getDescription().length() > 0) {
text.append("
").append(getBundle("UnitDetails_Plugin_Description")).append("
"); // NOI18N
String description = u.getDescription();
if(description.toLowerCase().startsWith("")) {
text.append(description.substring(6));
} else {
text.append(description);
}
}
if (desc != null && desc.length() > 0) {
text.append("
").append(getBundle("Unit_InternalUpdates_Title")).append("
"); // NOI18N
text.append(desc);
}
}
private void setUnitText(Unit u, StringBuilder text) {
getDetails().setText(text.toString());
setUnitHighlighing(u);
}
private StringBuilder getUnitText(Unit u, boolean collectDependencies) {
StringBuilder text = new StringBuilder();
for (int i = 0; ; i++) {
try {
buildUnitText(u, text, collectDependencies);
} catch (IllegalStateException ex) {
if (i > 100) {
throw ex;
}
Unit.log.log(Level.INFO, "Can't compute getUnitText for " + u, ex); // NOI18N
continue;
}
break;
}
return text;
}
private void setUnitHighlighing(Unit u) {
Color highlightColor = UIManager.getColor("nb.autoupdate.search.highlight");
highlightColor = highlightColor == null ? Color.YELLOW : highlightColor;
final ColorHighlighter highlighter = new ColorHighlighter(getDetails(), highlightColor);
int idx = highlighter.highlight(u.getFilter());
getDetails().setCaretPosition(idx > 0 ? idx : 0);
}
private String getDependencies(Unit.Update uu, boolean collectDependencies) {
if (!collectDependencies) {
return "" + getBundle("UnitDetails_Plugin_Collecting_Dependencies") + "
";
}
Unit u = uu;
if (u instanceof Unit.CompoundUpdate) {
Unit.CompoundUpdate cu = (Unit.CompoundUpdate) u;
StringBuilder desc = new StringBuilder();
for (UpdateUnit internalUnits : cu.getUpdateUnits()) {
appendInternalUpdates(desc, internalUnits.getAvailableUpdates().get(0));
}
return desc.toString();
} else {
return "";
}
}
private void appendInternalUpdates(StringBuilder desc, UpdateElement ue) {
desc.append(" ");
desc.append(ue.getDisplayName());
if (ue.getUpdateUnit().getInstalled() != null) {
desc.append(" [").append(ue.getUpdateUnit().getInstalled().getSpecificationVersion()).append("->");
} else {
desc.append(" ").append(getBundle("UnitDetails_New_Internal_Update_Mark")).append(" [");
}
desc.append(ue.getUpdateUnit().getAvailableUpdates().get(0).getSpecificationVersion());
desc.append("]
");
}
private static String getBundle(String key) {
return NbBundle.getMessage(UnitDetails.class, key);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy