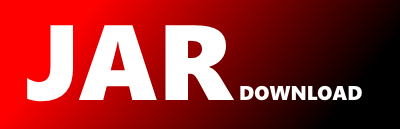
org.netbeans.modules.bugtracking.tasks.TaskNotificationPanel Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.modules.bugtracking.tasks;
import java.awt.GridBagConstraints;
import java.awt.Insets;
import java.awt.event.MouseEvent;
import java.util.ArrayList;
import java.util.List;
import javax.swing.Action;
import javax.swing.JLabel;
import org.netbeans.modules.bugtracking.IssueImpl;
import org.netbeans.modules.team.commons.treelist.LinkButton;
import org.openide.util.ImageUtilities;
import org.openide.util.NbBundle;
/**
*
* @author jpeska
*/
public class TaskNotificationPanel extends javax.swing.JPanel {
private final List tasks;
private final Action openAction;
private final int ROWS_COUNT = 4;
/**
* Creates new form TaskNotificationPanel
*/
public TaskNotificationPanel(List tasks, Action openAction) {
this.tasks = tasks;
this.openAction = openAction;
initComponents();
initTasks();
}
private void initTasks() {
for (int i = 0; i < ROWS_COUNT; i++) {
final IssueImpl task = getTask(i);
if (task == null) {
break;
}
JLabel lbl = new LinkLabel(DashboardUtils.getTaskDisplayName(task), ImageUtilities.image2Icon(task.getPriorityIcon())) {
@Override
public void mouseClicked(MouseEvent e) {
task.open();
}
};
GridBagConstraints gbc = new GridBagConstraints(0, i, 1, 1, 1.0, 0, GridBagConstraints.WEST, GridBagConstraints.NONE, new Insets(0, 5, 5, 0), 0, 0);
pnlTasks.add(lbl, gbc);
}
int overflow = tasks.size() - ROWS_COUNT;
if (overflow > 0) {
JLabel lblOthers = new JLabel(NbBundle.getMessage(TaskNotificationPanel.class, "LBL_Others", overflow));
GridBagConstraints gbc = new GridBagConstraints(0, ROWS_COUNT, 1, 1, 1.0, 0, GridBagConstraints.WEST, GridBagConstraints.NONE, new Insets(0, 5, 5, 0), 0, 0);
pnlTasks.add(lblOthers, gbc);
}
}
private IssueImpl getTask(int index) {
if (index < tasks.size()) {
return tasks.get(index);
}
return null;
}
/**
* This method is called from within the constructor to initialize the form. WARNING: Do NOT modify this code. The content of this method is always regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
pnlTasks = new javax.swing.JPanel();
jPanel1 = new javax.swing.JPanel();
btnAction = new LinkButton(NbBundle.getMessage(TaskNotificationPanel.class, "LBL_OpenAction"), openAction);
setOpaque(false);
setLayout(new java.awt.BorderLayout());
pnlTasks.setOpaque(false);
pnlTasks.setLayout(new java.awt.GridBagLayout());
add(pnlTasks, java.awt.BorderLayout.CENTER);
jPanel1.setOpaque(false);
javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1);
jPanel1.setLayout(jPanel1Layout);
jPanel1Layout.setHorizontalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(0, 0, 0)
.addComponent(btnAction))
);
jPanel1Layout.setVerticalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addContainerGap()
.addComponent(btnAction))
);
add(jPanel1, java.awt.BorderLayout.PAGE_END);
}// //GEN-END:initComponents
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton btnAction;
private javax.swing.JPanel jPanel1;
private javax.swing.JPanel pnlTasks;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy