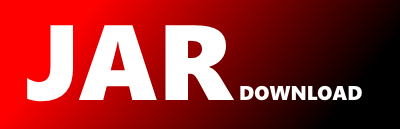
org.netbeans.modules.bugtracking.tasks.SchedulingPickerImpl Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.modules.bugtracking.tasks;
import java.awt.Point;
import java.awt.event.MouseEvent;
import java.text.DateFormat;
import java.text.ParseException;
import java.util.Calendar;
import java.util.Date;
import java.util.Locale;
import javax.swing.JComponent;
import javax.swing.JFormattedTextField;
import javax.swing.JMenuItem;
import javax.swing.JPopupMenu;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
import javax.swing.text.DateFormatter;
import javax.swing.text.DefaultFormatterFactory;
import org.netbeans.modules.bugtracking.spi.IssueScheduleInfo;
import org.openide.util.ChangeSupport;
import org.openide.util.NbBundle;
/**
* An implementation for {@link org.netbeans.modules.bugtracking.spi.SchedulingPicker }
* @author Ondrej Vrabec
*/
public class SchedulingPickerImpl extends javax.swing.JPanel {
private final ChangeSupport support;
private IssueScheduleInfo currentValue;
private static final DateFormat DATE_FORMAT = DateFormat.getDateInstance(DateFormat.MEDIUM);
private boolean setting;
/**
* Creates new form SchedulePickerImpl
*/
public SchedulingPickerImpl () {
support = new ChangeSupport(this);
initComponents();
}
public JComponent getComponent () {
return this;
}
public IssueScheduleInfo getScheduleDate () {
return currentValue;
}
public void setScheduleDate (IssueScheduleInfo info) {
currentValue = info;
setting = true;
scheduleTextComponent.setValue(info);
setting = false;
}
public void addChangeListener (ChangeListener listener) {
support.addChangeListener(listener);
}
public void removeChangeListener (ChangeListener listener) {
support.removeChangeListener(listener);
}
@Override
public void setEnabled (boolean enabled) {
super.setEnabled(enabled);
scheduleTextComponent.setEnabled(enabled);
popupButton.setEnabled(enabled);
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
scheduleTextComponent = new javax.swing.JFormattedTextField();
popupButton = new javax.swing.JButton();
scheduleTextComponent.setFormatterFactory(new DefaultFormatterFactory(new SchedulingDisplayFormatter(), null, new SchedulingEditFormatter(), null));
scheduleTextComponent.setMargin(new java.awt.Insets(2, 2, 2, 2));
scheduleTextComponent.addPropertyChangeListener(new java.beans.PropertyChangeListener() {
public void propertyChange(java.beans.PropertyChangeEvent evt) {
scheduleTextComponentPropertyChange(evt);
}
});
popupButton.setIcon(new javax.swing.ImageIcon(getClass().getResource("/org/netbeans/modules/bugtracking/tasks/resources/arrow-down.png"))); // NOI18N
popupButton.setMargin(new java.awt.Insets(2, 2, 2, 2));
popupButton.addMouseListener(new java.awt.event.MouseAdapter() {
public void mousePressed(java.awt.event.MouseEvent evt) {
popupButtonMousePressed(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(scheduleTextComponent, javax.swing.GroupLayout.PREFERRED_SIZE, 200, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(0, 0, 0)
.addComponent(popupButton)
.addGap(0, 0, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.CENTER)
.addComponent(scheduleTextComponent, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(popupButton, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGap(0, 0, 0))
);
}// //GEN-END:initComponents
private void popupButtonMousePressed(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_popupButtonMousePressed
if (evt.getButton() == MouseEvent.BUTTON1) {
showSchedulingPopup();
}
}//GEN-LAST:event_popupButtonMousePressed
private void scheduleTextComponentPropertyChange(java.beans.PropertyChangeEvent evt) {//GEN-FIRST:event_scheduleTextComponentPropertyChange
if ("value".equals(evt.getPropertyName())) { //NOI18N
if (evt.getOldValue() != null || evt.getNewValue() != null) {
updateCurrentValue((IssueScheduleInfo) scheduleTextComponent.getValue());
}
}
}//GEN-LAST:event_scheduleTextComponentPropertyChange
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton popupButton;
private javax.swing.JFormattedTextField scheduleTextComponent;
// End of variables declaration//GEN-END:variables
private void updateCurrentValue (IssueScheduleInfo info) {
if (!setting && (currentValue == null && info != null
|| currentValue != null && !currentValue.equals(info))) {
currentValue = info;
scheduleTextComponent.setValue(info);
support.fireChange();
}
}
private void showSchedulingPopup () {
final DashboardUtils.SchedulingMenu menu = new DashboardUtils.SchedulingMenu(currentValue);
menu.addChangeListener(new ChangeListener() {
@Override
public void stateChanged (ChangeEvent e) {
updateCurrentValue(menu.getScheduleInfo());
menu.removeChangeListener(this);
}
});
JPopupMenu popup = new JPopupMenu();
for (JMenuItem item : menu.getMenuItems()) {
if (item == null) {
popup.addSeparator();
} else {
popup.add(item);
}
}
Point p = scheduleTextComponent.getLocation();
popup.show(scheduleTextComponent.getParent(), p.x, p.y + scheduleTextComponent.getSize().height + 1);
}
@NbBundle.Messages({
"# {0} - date from", "# {1} - date until", "CTL_SpecificDates={0} - {1}"
})
private static String toDisplayable (IssueScheduleInfo info) {
Date date = info.getDate();
int interval = info.getInterval();
Calendar cal = Calendar.getInstance();
stripTime(cal);
Calendar scheduleDay = Calendar.getInstance();
scheduleDay.setTime(date);
stripTime(scheduleDay);
if (interval <= 1) {
String dayName = scheduleDay.getDisplayName(Calendar.DAY_OF_WEEK, Calendar.LONG, Locale.getDefault());
for (int i = 0; i < 7; ++i) {
if (cal.getTime().equals(scheduleDay.getTime())) {
if (i == 0) {
dayName = Bundle.CTL_Today(dayName);
}
return dayName;
}
cal.add(Calendar.DATE, 1);
}
return DATE_FORMAT.format(date);
} else {
scheduleDay.setTime(date);
stripTime(scheduleDay);
Calendar until = Calendar.getInstance();
until.setTime(date);
stripTime(until);
until.add(Calendar.DATE, interval);
if (isThisWeek(scheduleDay, until)) {
return Bundle.CTL_ThisWeek();
} else if (isNextWeek(scheduleDay, until)) {
return Bundle.CTL_NextWeek();
} else {
return Bundle.CTL_SpecificDates(DATE_FORMAT.format(scheduleDay.getTime()),
DATE_FORMAT.format(until.getTime()));
}
}
}
private static void stripTime (Calendar cal) {
cal.set(Calendar.HOUR_OF_DAY, 0);
cal.set(Calendar.MINUTE, 0);
cal.set(Calendar.SECOND, 0);
cal.set(Calendar.MILLISECOND, 0);
}
private static boolean isThisWeek (Calendar scheduleDay, Calendar until) {
Calendar weekStart = Calendar.getInstance();
stripTime(weekStart);
weekStart.set(Calendar.DAY_OF_WEEK, weekStart.getFirstDayOfWeek());
Calendar weekEnd = Calendar.getInstance();
weekEnd.setTime(weekStart.getTime());
weekEnd.add(Calendar.DATE, 7);
return scheduleDay.equals(weekStart) && until.equals(weekEnd);
}
private static boolean isNextWeek (Calendar scheduleDay, Calendar until) {
Calendar weekStart = Calendar.getInstance();
stripTime(weekStart);
weekStart.set(Calendar.DAY_OF_WEEK, weekStart.getFirstDayOfWeek());
weekStart.add(Calendar.DATE, 7);
Calendar weekEnd = Calendar.getInstance();
weekEnd.setTime(weekStart.getTime());
weekEnd.add(Calendar.DATE, 7);
return scheduleDay.equals(weekStart) && until.equals(weekEnd);
}
private static class SchedulingDisplayFormatter extends JFormattedTextField.AbstractFormatter {
@Override
public Object stringToValue (String text) throws ParseException {
return null;
}
@Override
public String valueToString (Object value) throws ParseException {
if (value instanceof IssueScheduleInfo) {
return toDisplayable((IssueScheduleInfo) value);
} else {
return Bundle.CTL_NotScheduled();
}
}
}
private static class SchedulingEditFormatter extends DateFormatter {
@Override
public Object stringToValue (String text) throws ParseException {
if (text == null || text.isEmpty()) {
return null;
} else {
Date date = (Date) super.stringToValue(text);
return new IssueScheduleInfo(date);
}
}
@Override
public String valueToString (Object value) throws ParseException {
if (value instanceof IssueScheduleInfo) {
return super.valueToString(((IssueScheduleInfo) value).getDate());
} else {
return null;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy