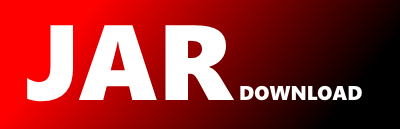
org.netbeans.modules.java.ui.FmtBraces Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.modules.java.ui;
import static org.netbeans.modules.java.ui.FmtOptions.*;
import static org.netbeans.modules.java.ui.CategorySupport.OPTION_ID;
import org.netbeans.modules.options.editor.spi.PreferencesCustomizer;
/**
*
* @author phrebejk
*/
public class FmtBraces extends javax.swing.JPanel {
/** Creates new form FmtAlignmentBraces */
public FmtBraces() {
initComponents();
moduleDeclCombo.putClientProperty(OPTION_ID, moduleDeclBracePlacement);
classDeclCombo.putClientProperty(OPTION_ID, classDeclBracePlacement);
methodDeclCombo.putClientProperty(OPTION_ID, methodDeclBracePlacement);
otherCombo.putClientProperty(OPTION_ID, otherBracePlacement);
specialElseIfCheckBox.putClientProperty(OPTION_ID, specialElseIf);
ifBracesCombo.putClientProperty(OPTION_ID, redundantIfBraces);
forBracesCombo.putClientProperty(OPTION_ID, redundantForBraces);
whileBracesCombo.putClientProperty(OPTION_ID, redundantWhileBraces);
doWhileBracesCombo.putClientProperty(OPTION_ID, redundantDoWhileBraces);
lambdaParensCheckBox.putClientProperty(OPTION_ID, parensAroundSingularLambdaParam);
}
public static PreferencesCustomizer.Factory getController() {
return new CategorySupport.Factory("braces", FmtBraces.class, //NOI18N
org.openide.util.NbBundle.getMessage(FmtBraces.class, "SAMPLE_Braces"), // NOI18N
new String[] { FmtOptions.blankLinesBeforeClass, "0" });
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
bracesPlacementLabel = new javax.swing.JLabel();
classDeclLabel = new javax.swing.JLabel();
classDeclCombo = new javax.swing.JComboBox();
methodDeclLabel = new javax.swing.JLabel();
methodDeclCombo = new javax.swing.JComboBox();
otherLabel = new javax.swing.JLabel();
otherCombo = new javax.swing.JComboBox();
specialElseIfCheckBox = new javax.swing.JCheckBox();
bracesGenerationLabel = new javax.swing.JLabel();
ifBracesLabel = new javax.swing.JLabel();
ifBracesCombo = new javax.swing.JComboBox();
forBracesLabel = new javax.swing.JLabel();
forBracesCombo = new javax.swing.JComboBox();
whileBracesLabel = new javax.swing.JLabel();
whileBracesCombo = new javax.swing.JComboBox();
doWhileBracesLabel = new javax.swing.JLabel();
doWhileBracesCombo = new javax.swing.JComboBox();
jSeparator1 = new javax.swing.JSeparator();
jSeparator2 = new javax.swing.JSeparator();
moduleDeclLabel = new javax.swing.JLabel();
moduleDeclCombo = new javax.swing.JComboBox<>();
jLabel1 = new javax.swing.JLabel();
jSeparator3 = new javax.swing.JSeparator();
lambdaParensCheckBox = new javax.swing.JCheckBox();
setName(org.openide.util.NbBundle.getMessage(FmtBraces.class, "LBL_Braces")); // NOI18N
setOpaque(false);
org.openide.awt.Mnemonics.setLocalizedText(bracesPlacementLabel, org.openide.util.NbBundle.getMessage(FmtBraces.class, "LBL_br_bracesPlacement")); // NOI18N
classDeclLabel.setLabelFor(classDeclCombo);
org.openide.awt.Mnemonics.setLocalizedText(classDeclLabel, org.openide.util.NbBundle.getMessage(FmtBraces.class, "LBL_bp_ClassDecl")); // NOI18N
classDeclCombo.setModel(new javax.swing.DefaultComboBoxModel(new String[] { "Item 1", "Item 2", "Item 3", "Item 4" }));
methodDeclLabel.setLabelFor(methodDeclCombo);
org.openide.awt.Mnemonics.setLocalizedText(methodDeclLabel, org.openide.util.NbBundle.getMessage(FmtBraces.class, "LBL_bp_MethodDecl")); // NOI18N
methodDeclCombo.setModel(new javax.swing.DefaultComboBoxModel(new String[] { "Item 1", "Item 2", "Item 3", "Item 4" }));
methodDeclCombo.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
methodDeclComboActionPerformed(evt);
}
});
otherLabel.setLabelFor(otherCombo);
org.openide.awt.Mnemonics.setLocalizedText(otherLabel, org.openide.util.NbBundle.getMessage(FmtBraces.class, "LBL_bp_Other")); // NOI18N
otherCombo.setModel(new javax.swing.DefaultComboBoxModel(new String[] { "Item 1", "Item 2", "Item 3", "Item 4" }));
org.openide.awt.Mnemonics.setLocalizedText(specialElseIfCheckBox, org.openide.util.NbBundle.getMessage(FmtBraces.class, "LBL_bp_SpecialElseIf")); // NOI18N
specialElseIfCheckBox.setBorder(javax.swing.BorderFactory.createEmptyBorder(0, 0, 0, 0));
specialElseIfCheckBox.setMargin(new java.awt.Insets(0, 0, 0, 0));
specialElseIfCheckBox.setOpaque(false);
org.openide.awt.Mnemonics.setLocalizedText(bracesGenerationLabel, org.openide.util.NbBundle.getMessage(FmtBraces.class, "LBL_br_bracesGeneration")); // NOI18N
ifBracesLabel.setLabelFor(ifBracesCombo);
org.openide.awt.Mnemonics.setLocalizedText(ifBracesLabel, org.openide.util.NbBundle.getMessage(FmtBraces.class, "LBL_bg_If")); // NOI18N
ifBracesCombo.setModel(new javax.swing.DefaultComboBoxModel(new String[] { "Item 1", "Item 2", "Item 3", "Item 4" }));
forBracesLabel.setLabelFor(forBracesCombo);
org.openide.awt.Mnemonics.setLocalizedText(forBracesLabel, org.openide.util.NbBundle.getMessage(FmtBraces.class, "LBL_bg_For")); // NOI18N
forBracesCombo.setModel(new javax.swing.DefaultComboBoxModel(new String[] { "Item 1", "Item 2", "Item 3", "Item 4" }));
whileBracesLabel.setLabelFor(whileBracesCombo);
org.openide.awt.Mnemonics.setLocalizedText(whileBracesLabel, org.openide.util.NbBundle.getMessage(FmtBraces.class, "LBL_bg_While")); // NOI18N
whileBracesCombo.setModel(new javax.swing.DefaultComboBoxModel(new String[] { "Item 1", "Item 2", "Item 3", "Item 4" }));
doWhileBracesLabel.setLabelFor(doWhileBracesCombo);
org.openide.awt.Mnemonics.setLocalizedText(doWhileBracesLabel, org.openide.util.NbBundle.getMessage(FmtBraces.class, "LBL_bg_DoWhile")); // NOI18N
doWhileBracesCombo.setModel(new javax.swing.DefaultComboBoxModel(new String[] { "Item 1", "Item 2", "Item 3", "Item 4" }));
moduleDeclLabel.setLabelFor(methodDeclCombo);
org.openide.awt.Mnemonics.setLocalizedText(moduleDeclLabel, org.openide.util.NbBundle.getMessage(FmtBraces.class, "LBL_bp_ModuleDecl")); // NOI18N
moduleDeclCombo.setModel(new javax.swing.DefaultComboBoxModel<>(new String[] { "Item 1", "Item 2", "Item 3", "Item 4" }));
org.openide.awt.Mnemonics.setLocalizedText(jLabel1, org.openide.util.NbBundle.getMessage(FmtBraces.class, "FmtBraces.jLabel1.text")); // NOI18N
org.openide.awt.Mnemonics.setLocalizedText(lambdaParensCheckBox, org.openide.util.NbBundle.getMessage(FmtBraces.class, "FmtBraces.lambdaParensCheckBox.text")); // NOI18N
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(bracesPlacementLabel)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jSeparator1))
.addGroup(layout.createSequentialGroup()
.addComponent(bracesGenerationLabel)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jSeparator2))
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel1)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jSeparator3))
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(doWhileBracesLabel)
.addComponent(otherLabel)
.addComponent(methodDeclLabel)
.addComponent(whileBracesLabel))
.addGap(12, 12, 12)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(otherCombo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(classDeclCombo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(ifBracesCombo, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(forBracesCombo, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(whileBracesCombo, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(moduleDeclCombo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addComponent(doWhileBracesCombo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(methodDeclCombo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addComponent(classDeclLabel)
.addComponent(specialElseIfCheckBox)
.addComponent(ifBracesLabel)
.addComponent(forBracesLabel)
.addComponent(moduleDeclLabel)
.addComponent(lambdaParensCheckBox))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
layout.linkSize(javax.swing.SwingConstants.HORIZONTAL, new java.awt.Component[] {classDeclCombo, doWhileBracesCombo, forBracesCombo, ifBracesCombo, methodDeclCombo, otherCombo, whileBracesCombo});
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(bracesPlacementLabel)
.addComponent(jSeparator1, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(moduleDeclLabel)
.addComponent(moduleDeclCombo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(classDeclLabel, javax.swing.GroupLayout.PREFERRED_SIZE, 18, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(classDeclCombo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(6, 6, 6)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(methodDeclLabel)
.addComponent(methodDeclCombo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(otherLabel)
.addComponent(otherCombo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(specialElseIfCheckBox)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(20, 20, 20)
.addComponent(bracesGenerationLabel))
.addGroup(layout.createSequentialGroup()
.addGap(28, 28, 28)
.addComponent(jSeparator2, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(ifBracesLabel)
.addComponent(ifBracesCombo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(forBracesLabel, javax.swing.GroupLayout.PREFERRED_SIZE, 16, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(forBracesCombo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(whileBracesLabel)
.addComponent(whileBracesCombo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(doWhileBracesLabel)
.addComponent(doWhileBracesCombo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jLabel1)
.addComponent(jSeparator3, javax.swing.GroupLayout.PREFERRED_SIZE, 8, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(lambdaParensCheckBox)
.addContainerGap(30, Short.MAX_VALUE))
);
}// //GEN-END:initComponents
private void methodDeclComboActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_methodDeclComboActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_methodDeclComboActionPerformed
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JLabel bracesGenerationLabel;
private javax.swing.JLabel bracesPlacementLabel;
private javax.swing.JComboBox classDeclCombo;
private javax.swing.JLabel classDeclLabel;
private javax.swing.JComboBox doWhileBracesCombo;
private javax.swing.JLabel doWhileBracesLabel;
private javax.swing.JComboBox forBracesCombo;
private javax.swing.JLabel forBracesLabel;
private javax.swing.JComboBox ifBracesCombo;
private javax.swing.JLabel ifBracesLabel;
private javax.swing.JLabel jLabel1;
private javax.swing.JSeparator jSeparator1;
private javax.swing.JSeparator jSeparator2;
private javax.swing.JSeparator jSeparator3;
private javax.swing.JCheckBox lambdaParensCheckBox;
private javax.swing.JComboBox methodDeclCombo;
private javax.swing.JLabel methodDeclLabel;
private javax.swing.JComboBox moduleDeclCombo;
private javax.swing.JLabel moduleDeclLabel;
private javax.swing.JComboBox otherCombo;
private javax.swing.JLabel otherLabel;
private javax.swing.JCheckBox specialElseIfCheckBox;
private javax.swing.JComboBox whileBracesCombo;
private javax.swing.JLabel whileBracesLabel;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy