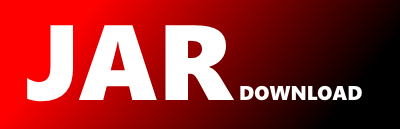
org.netbeans.jellytools.properties.Property Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.jellytools.properties;
import java.awt.Component;
import java.awt.event.ActionEvent;
import java.beans.PropertyEditor;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.concurrent.atomic.AtomicReference;
import javax.swing.Action;
import javax.swing.JComponent;
import javax.swing.JTable;
import javax.swing.table.TableCellRenderer;
import org.netbeans.jellytools.JellyVersion;
import org.netbeans.jemmy.JemmyException;
import org.netbeans.jemmy.QueueTool;
import org.netbeans.jemmy.Waitable;
import org.netbeans.jemmy.Waiter;
import org.netbeans.jemmy.operators.JTableOperator;
import org.openide.ErrorManager;
import org.openide.explorer.propertysheet.editors.EnhancedPropertyEditor;
import org.openide.nodes.Node;
/**
* Handles properties in IDE property sheets. Properties are grouped in
* property sheet. Their are identified by their display names. Once you
* have created a Property instance you can get value, set a new text value,
* set a new value by index of possible options or open custom editor.
*
* Usage:
*
PropertySheetOperator pso = new PropertySheetOperator("Properties of MyClass");
Property p = new Property(pso, "Name");
System.out.println("\nProperty name="+p.getName());
System.out.println("\nProperty value="+p.getValue());
p.setValue("ANewValue");
// set a new value by index where it is applicable
//p.setValue(2);
// open custom editor where it is applicable
//p.openEditor();
*
*
* @author [email protected]
* @see PropertySheetOperator
*/
public class Property {
/** Class name of string renderer. */
public static final String STRING_RENDERER = "org.openide.explorer.propertysheet.RendererFactory$StringRenderer"; // NOI18N
/** Class name of check box renderer. */
public static final String CHECKBOX_RENDERER = "org.openide.explorer.propertysheet.RendererFactory$CheckboxRenderer"; // NOI18N
/** Class name of combo box renderer. */
public static final String COMBOBOX_RENDERER = "org.openide.explorer.propertysheet.RendererFactory$ComboboxRenderer"; // NOI18N
/** Class name of radio button renderer. */
public static final String RADIOBUTTON_RENDERER = "org.openide.explorer.propertysheet.RendererFactory$RadioButtonRenderer"; // NOI18N
/** Class name of set renderer. */
public static final String SET_RENDERER = "org.openide.explorer.propertysheet.RendererFactory$SetRenderer"; // NOI18N
/** Instance of Node.Property. */
protected Node.Property property;
/** Property sheet where this property resides. */
protected PropertySheetOperator propertySheetOper;
static {
// Checks if you run on correct jemmy version. Writes message to jemmy log if not.
JellyVersion.checkJemmyVersion();
}
/** Waits for property with given name in specified property sheet.
* @param propertySheetOper PropertySheetOperator where to find property.
* @param name property display name
*/
public Property(PropertySheetOperator propertySheetOper, String name) {
this.propertySheetOper = propertySheetOper;
this.property = waitProperty(propertySheetOper, name);
}
/** Waits for index-th property in specified property sheet.
* @param propertySheetOper PropertySheetOperator where to find property.
* @param index index (row number) of property inside property sheet
* (starts at 0). If there categories shown in property sheet,
* rows occupied by their names must by added to index.
*/
public Property(PropertySheetOperator propertySheetOper, int index) {
this.propertySheetOper = propertySheetOper;
this.property = waitProperty(propertySheetOper, index);
}
/** Waits for property with given name in specified property sheet.
* @param propSheetOper PropertySheetOperator where to find property.
* @param name property display name
*/
private Node.Property waitProperty(final PropertySheetOperator propSheetOper, final String name) {
try {
Waiter waiter = new Waiter(new Waitable() {
public Object actionProduced(Object param) {
Node.Property property = null;
JTableOperator table = propSheetOper.tblSheet();
for(int row=0;row
* org.openide.explorer.propertysheet.RendererFactory$StringRenderer
* org.openide.explorer.propertysheet.RendererFactory$CheckboxRenderer
* org.openide.explorer.propertysheet.RendererFactory$ComboboxRenderer
* org.openide.explorer.propertysheet.RendererFactory$RadioButtonRenderer
* org.openide.explorer.propertysheet.RendererFactory$SetRenderer
*
* @see #STRING_RENDERER
* @see #CHECKBOX_RENDERER
* @see #COMBOBOX_RENDERER
* @see #RADIOBUTTON_RENDERER
* @see #SET_RENDERER
*/
public String getRendererName() {
return getRenderer().getClass().getName();
}
/** Returns component which represents renderer for this property. */
private Component getRenderer() {
final JTableOperator table = propertySheetOper.tblSheet();
int row = getRow();
// gets component used to render a value
TableCellRenderer renderer = table.getCellRenderer(row, 1);
Component comp = renderer.getTableCellRendererComponent(
(JTable)table.getSource(),
table.getValueAt(row, 1),
false,
false,
row,
1
);
// We need to find a real renderer because it can be embedded
// in ButtonPanel (supplies custom editor button "...")
// or IconPanel(supplies property marking).
try {
Class> clazz = Class.forName("org.openide.explorer.propertysheet.RendererPropertyDisplayer");
Method findInnermostRendererMethod = clazz.getDeclaredMethod("findInnermostRenderer", new Class[] {JComponent.class});
findInnermostRendererMethod.setAccessible(true);
comp = (Component)findInnermostRendererMethod.invoke(null, new Object[] {comp});
} catch (Exception e) {
throw new JemmyException("RendererPropertyDisplayer.findInnermostRenderer() by reflection failed.", e);
}
return comp;
}
/** Gets short description for this property. Short description is also
* used in tooltip.
* @return short description for this property.
*/
public String getShortDescription() {
return this.property.getShortDescription();
}
/*
* @return row number of property inside property sheet (starts at 0).
* If there are categories shown in property sheet, rows occupied by their
* names must by taken into account.
*/
public int getRow() {
JTableOperator table = this.propertySheetOper.tblSheet();
for(int row=0;row atomicReference = new AtomicReference();
new QueueTool().invokeSmoothly(new Runnable() {
@Override
public void run() {
try {
Class> clazz = Class.forName("org.openide.explorer.propertysheet.PropUtils");
Method getPropertyEditorMethod = clazz.getDeclaredMethod("getPropertyEditor", new Class[]{Node.Property.class});
getPropertyEditorMethod.setAccessible(true);
atomicReference.set((PropertyEditor) getPropertyEditorMethod.invoke(null, new Object[]{property}));
} catch (Exception e) {
throw new JemmyException("PropUtils.getPropertyEditor() by reflection failed.", e);
}
}
});
return atomicReference.get();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy