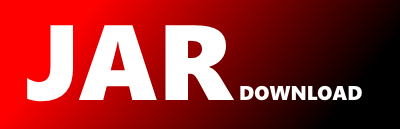
org.netbeans.api.languages.LibrarySupport Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.api.languages;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.xml.sax.Attributes;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
import org.xml.sax.XMLReader;
import org.xml.sax.helpers.DefaultHandler;
import org.openide.ErrorManager;
import org.openide.util.Lookup;
import org.openide.xml.XMLUtil;
/**
* Support for definition of libraries. Used for code completion.
*
* @author Jan Jancura
*/
public abstract class LibrarySupport {
/**
* Crates new Instance of LibrarySupport and reads library definition from give resource file.
*
* @param resourceName a name of resource file
*/
public static LibrarySupport create (String resourceName) {
return new LibraryImpl (resourceName);
}
/**
* Crates new Instance of LibrarySupport and reads library definition from give resource files.
*
* @param resourceNames names of resource files
*/
public static LibrarySupport create (List resourceNames) {
return new DelegatingLibrarySupport (resourceNames);
}
/**
* Returns list of items for given context (e.g. list of static methods
* for fiven class name).
*
* @param context
* @return list of items for given context
*/
public abstract List getItems (String context);
public abstract List getCompletionItems (String context);
/**
* Returns property for given item, context and property name.
*
* @param context a context
* @param item an item
* @param propertyName a name of property
*/
public abstract String getProperty (String context, String item, String propertyName);
private static class LibraryImpl extends LibrarySupport {
private String resourceName;
LibraryImpl (String resourceName) {
this.resourceName = resourceName;
}
private Map> keys = new HashMap> ();
public List getItems (String context) {
List k = keys.get (context);
if (k == null) {
Map>> m = getItems ().get (context);
if (m == null) return null;
k = new ArrayList (m.keySet ());
Collections.sort (k);
k = Collections.unmodifiableList (k);
keys.put (context, k);
}
return k;
}
public List getCompletionItems (String context) {
List result = new ArrayList ();
Map>> items = getItems ().get (context);
if (items == null) return result;
Iterator it = items.keySet ().iterator ();
while (it.hasNext ()) {
String name = it.next();
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy