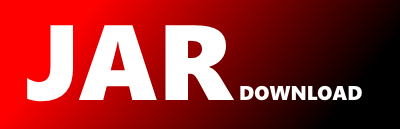
org.netbeans.modules.schema2beans.AttrProp Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.modules.schema2beans;
import java.util.*;
/**
* This class hold the information about a property attribute.
*
* This class is used by the TreeBuilder to build a representation of the
* attributes, by the BeanClass to convert these information into
* generated attribute code, and by the BeanProp class to hold at runtime
* the information about the attributes of a property.
*
*/
public class AttrProp implements BaseAttribute {
public static final int MASK_KIND = 0x00FF;
public static final int CDATA = 0x0001;
public static final int ENUM = 0x0002;
public static final int NMTOKEN = 0x0003;
public static final int ID = 0x0004;
public static final int IDREF = 0x0005;
public static final int IDREFS = 0x0006;
public static final int ENTITY = 0x0007;
public static final int ENTITIES = 0x0008;
public static final int NOTATION = 0x0009;
static final String[] kinds =
new String[] {"CDATA", "ENUM", "NMTOKEN", "ID", "IDREF", // NOI18N
"IDREFS", "ENTITY", "ENTITIES", "NOTATION"}; // NOI18N
static final int[] kindValues =
new int[] {CDATA, ENUM, NMTOKEN, ID, IDREF,
IDREFS, ENTITY, ENTITIES, NOTATION};
public static final int MASK_OPTION = 0x0F00;
public static final int REQUIRED = 0x0100;
public static final int IMPLIED = 0x0200;
public static final int FIXED = 0x0300;
public static final int TRANSIENT = 0x1000;
static final String[] options =
new String[] {"#REQUIRED", "#IMPLIED", "#FIXED"}; // NOI18N
static final int[] optionValues = new int[] {REQUIRED, IMPLIED, FIXED};
// Property this attribure belongs to
String propertyName;
// Name of the attribute
String name;
// Name of the attribute
String dtdName;
String namespace;
// Its type (CDATA, ID, ...)
int type;
// Proposed java class for it.
String javaType;
// Enum values if any (null if none)
ArrayList values;
// The default value of the attribute
String defaultValue;
//
// The attribute content is populated only calling addValue()
// assuming it is built from a left to right parsing. This state
// is used to know which value is being added.
//
private int state;
private int enumMode;
private List extraData;
//private GraphNode sourceGraphNode;
// The state values when the attribute is populated
private static final int NEED_NAME = 0;
private static final int NEED_TYPE = 1;
private static final int NEED_ENUM = 2;
private static final int NEED_OPTION = 3;
private static final int NEED_DEFVAL = 4;
private static final int NEED_VALUE = 5;
private static final int DONE = 6;
public AttrProp() {
this.values = null;
this.state = NEED_NAME;
this.type = 0;
this.enumMode = 0;
}
public AttrProp(String propName) {
this();
this.propertyName = propName;
}
public AttrProp(String propName, String dtdName, String name, int type,
String[] values, String defValue) {
this.dtdName = dtdName;
this.name = name;
this.propertyName = propName;
if (values != null && values.length > 0) {
this.values = new ArrayList();
for (int i=0; i 0)
return (String[])this.values.toArray(ret);
else
return ret;
}
public void setDefaultValue(String d) {
defaultValue = d;
}
public String getDefaultValue() {
return this.defaultValue;
}
public String getPropertyName() {
return this.propertyName;
}
public String getName() {
return this.name;
}
public void setName(String n) {
name = n;
}
/*
public void setSourceGraphNode(GraphNode node) {
sourceGraphNode = node;
}
public GraphNode getSourceGraphNode() {
return sourceGraphNode;
}
*/
public String getDtdName() {
return this.dtdName;
}
public String getNamespace() {
return this.namespace;
}
public String typeAsString() {
String str = "AttrProp." + // NOI18N
intToString(this.type & MASK_KIND, kinds, kindValues);
int opt = this.type & MASK_OPTION;
if (opt != 0) {
str += " | " + "AttrProp." + // NOI18N
intToString(opt, options, optionValues).substring(1);
}
return str;
}
String enumsToString() {
String[] e = this.getValues();
StringBuffer ret = new StringBuffer();
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy