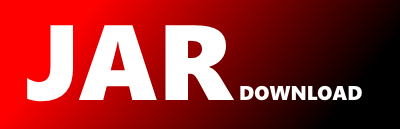
org.netbeans.modules.schema2beansdev.BeanClass Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.modules.schema2beansdev;
import java.util.*;
import java.io.*;
import org.netbeans.modules.schema2beans.*;
import org.netbeans.modules.schema2beansdev.metadd.*;
import org.netbeans.modules.schema2beansdev.gen.*;
//******************************************************************************
// BEGIN_NOI18N
//******************************************************************************
/**
* This class generates the bean classes code. There is one instance of
* this class per bean to generate.
*
* The BeanBuilder creates one instance of the BeanClass class for every
* bean class to generate, and for each of these instances,
* the BeanBuilder calls the addPropertyMethod() in order to populate the
* list of properties contained by the bean. Then it calls the process()
* method, which makes the class to generate its java output into
* the output stream specified in the process parameter.
*
* The BeanBuilder might give an optional MetaDD bean graph which contains
* extra information that didn't exist in the dtd. The content of this graph
* is used to generate extra information about the properties of the bean
* (for example the default values, well known values, wrapper class)
*/
public class BeanClass extends AbstractCodeGeneratorClass implements CodeGeneratorClass {
private boolean isVetoable;
private boolean genVetoListeners;
private boolean genGenericVetoListeners;
BeanClass(BeanBuilder.BeanElement be, GenBeans.Config config) {
init(be, config);
addExtraMethods();
}
void setBeanName(String n) {
this.className = n;
}
private void genVetoBegin() {
gencr("try"); begin();
}
private void genVetoEnd() {
end();
gencr("catch(BaseProperty.VetoException ve)");
begin();
gen("throw ve.getPropertyVetoException()"); eol();
end();
}
static final String VCL_FULL_CLASS_NAME = "java.beans.VetoableChangeListener";
static final String VCL = "VetoableChangeListener";
static final String PCL_FULL_CLASS_NAME = "java.beans.PropertyChangeListener";
static final String PCL = "PropertyChangeListener";
/**
* Generate the different parts of the class
*/
void genHeader(int out) throws IOException {
select(out);
gencr("/**");
gencr(" * This generated bean class " + this.className +
" matches the schema element '" + this.beanElement.node.getName()+"'.");
if (!beanElement.isRoot) {
jw.writecr(" * The root bean class is "+rootBeanElement.getClassType());
}
gencr(" *");
printComment(" * ");
if (config.isGenerateTimeStamp())
gencr(" * Generated on " + new Date());
if (this.beanElement.isRoot) {
gencr(" *");
gencr(" * This class matches the root element of the "+(config.getSchemaTypeNum() == GenBeans.Config.DTD ? "DTD" : "XML Schema")+",");
gencr(" * and is the root of the following bean graph:");
gencr(" *");
dumpBeanTree(jw, " *"+jw.getIndent(), jw.getIndent());
gencr(" *");
}
gencr(" * @"+Common.GENERATED_TAG);
gencr(" */"); cr();
}
void genPackage(int out) {
select(out);
if (this.packageName != null) {
gen(PACKAGE, this.packageName);
eol();
cr();
}
}
void genImports(int out) {
select(out);
gen(IMPORT, "org.w3c.dom.*"); eol();
gen(IMPORT, "org.netbeans.modules.schema2beans.*"); eol();
gen(IMPORT, "java.beans.*"); eol();
gen(IMPORT, "java.util.*"); eol();
//if (!this.config.isStandalone()) {
// gen(IMPORT, "org.openide.util.NbBundle"); eol();
//}
if (this.beanElement.isRoot) {
gen(IMPORT, "java.io.*"); eol();
}
String[] imps = null;
if (this.mdd != null) {
if (this.metaElement != null)
imps = this.metaElement.getImport();
if (imps == null || imps.length==0)
imps = this.mdd.getImport();
}
if (imps != null) {
for (int i=0; i 0)
gencr("if ((options & Common.USE_DEFAULT_VALUES) ",
"== Common.USE_DEFAULT_VALUES)");
if (values.length > 1)
begin();
for (int j=0; j 1)
end();
}
//
// Generate the well-known values
//
elts = 0;
values = null;
// Get the current property default values first
if (mp != null)
values = mp.getKnownValue();
if (values != null)
elts = values.length;
// If no default value, try to get the generic ones
if (elts == 0)
values = me.getKnownValue();
if (values != null) {
for (int j = 0; j < values.length; j++) {
jw.writeEol("addKnownValue("+constName+", ", JavaUtil.instanceFrom(JavaUtil.toObjectType(type), values[j]), ")");
}
}
}
}
boolean first = true;
for (int i = 0; i < size; i++) {
Property a = (Property)attrList.get(i);
boolean indexed = a.isIndexed();
String type = a.getType();
if (!indexed && a.getDefaultValue() != null && (a.elementInstance == Common.TYPE_1 || a.elementInstance == Common.TYPE_1_N)) {
if (!JavaUtil.checkValueToType(type, a.getDefaultValue())) {
config.messageOut.println(Common.getMessage("MSG_NotAGoodValue", a.getDefaultValue(), type));
}
if (first) {
first = false;
jw.beginIf("(options & Common.USE_DEFAULT_VALUES) == Common.USE_DEFAULT_VALUES");
}
jw.write(a.getWriteMethod(), "(");
jw.write(JavaUtil.instanceFrom(type, a.getDefaultValue()));
jw.writeEol(")");
}
}
if (!first)
jw.end();
}
void genDeclarations(int out) {
select(out);
cr();
gen("static Vector comparators = new Vector()"); eol();
if (this.metaElement != null) {
int size = this.metaElement.sizeComparatorClass();
if (size >0) {
gen(STATIC);
begin();
for (int i=0; i 0)");
begin();
gen("final int count = array.length - offset"); eol();
gen("out.writeUTF(new String(array, offset, count, SERIALIZATION_HELPER_CHARSET))"); eol();
end();
end();
gen("catch (Schema2BeansException ex)");
begin();
gen("throw new Schema2BeansRuntimeException(ex)"); eol();
end();
end();
comment("Special deserializer: read XML as deserialization");
gen(PRIVATE, VOID, "readObject(java.io.ObjectInputStream in) throws java.io.IOException, ClassNotFoundException");
begin();
gen("try");
begin();
gen("in.defaultReadObject()"); eol();
gen("init(comparators, runtimeVersion)"); eol();
comment("init(comparators, new GenBeans.Version(1, 0, 8))");
gen("final int numStrings = in.readInt()");eol();
gen("final int max_size = in.readInt()");eol();
if (config.isJava5()) {
gen("final StringBuilder sb = new StringBuilder(numStrings * max_size)"); eol();
} else {
gen("final StringBuffer sb = new StringBuffer(numStrings * max_size)"); eol();
}
gen("for (int i = 0; i < numStrings; i++)"); begin();
gen("sb.append(in.readUTF())"); eol();
end();
gen("ByteArrayInputStream bais = new ByteArrayInputStream(sb.toString().getBytes(SERIALIZATION_HELPER_CHARSET))"); eol();
gen("Document doc = GraphManager.createXmlDocument(bais, false)"); eol();
if (config.isSetDefaults()){
gen("initOptions(Common.USE_DEFAULT_VALUES)"); eol();
gen("initFromNode(doc, Common.USE_DEFAULT_VALUES)"); eol();
} else {
gen("initOptions(Common.NO_DEFAULT_VALUES)"); eol();
gen("initFromNode(doc, Common.NO_DEFAULT_VALUES)"); eol();
}
end();
gen("catch (Schema2BeansException e)");
begin();
gen("throw new RuntimeException(e)"); eol();
end();
end();
cr();
jw.beginMethod("_setSchemaLocation", "String location", null, "void", jw.PUBLIC);
jw.beginIf("beanProp().getAttrProp(\"xsi:schemaLocation\", true) == null");
jw.writeEol("createAttribute(\"xmlns:xsi\", \"xmlns:xsi\", AttrProp.CDATA | AttrProp.IMPLIED, null, \"http://www.w3.org/2001/XMLSchema-instance\")");
jw.writeEol("setAttributeValue(\"xmlns:xsi\", \"http://www.w3.org/2001/XMLSchema-instance\")");
jw.writeEol("createAttribute(\"xsi:schemaLocation\", \"xsi:schemaLocation\", AttrProp.CDATA | AttrProp.IMPLIED, null, location)");
jw.end();
jw.writeEol("setAttributeValue(\"xsi:schemaLocation\", location)");
jw.end();
jw.cr();
jw.beginMethod("_getSchemaLocation", "", null, "String", jw.PUBLIC);
jw.beginIf("beanProp().getAttrProp(\"xsi:schemaLocation\", true) == null");
jw.writeEol("createAttribute(\"xmlns:xsi\", \"xmlns:xsi\", AttrProp.CDATA | AttrProp.IMPLIED, null, \"http://www.w3.org/2001/XMLSchema-instance\")");
jw.writeEol("setAttributeValue(\"xmlns:xsi\", \"http://www.w3.org/2001/XMLSchema-instance\")");
jw.writeEol("createAttribute(\"xsi:schemaLocation\", \"xsi:schemaLocation\", AttrProp.CDATA | AttrProp.IMPLIED, null, null)");
jw.end();
jw.writeEol("return getAttributeValue(\"xsi:schemaLocation\")");
jw.end();
jw.cr();
}
select(TRAILER_SECTION);
comment("Dump the content of this bean returning it as a String");
gen(PUBLIC, VOID, "dump(StringBuffer str, String indent)");
begin();
gen("String s"); eol();
gen("Object o"); eol();
gen("org.netbeans.modules.schema2beans.BaseBean n"); eol();
select(CONSTRUCTOR_SECTION);
comment("Properties (see root bean comments for the bean graph)");
int propertyCount = 0;
for (int i = 0; i < size; i++) {
Property a = (Property) attrList.get(i);
if (!a.isAttribute()) {
++propertyCount;
}
}
jw.writeEol("initPropertyTables("+propertyCount, ")");
select(DECL_SECTION); cr();
//
// Assume that there is no property interested in veto events.
// We'll adjust later on in the above properties loop.
//
this.genVetoListeners = false;
// Find out if any element has a key
if (this.metaElement != null) {
MetaProperty[] mp = this.metaElement.getMetaProperty();
for (int i=0; i\\n\")"); eolNoI18N();
} else if (a.isBean) {
gen("n = (org.netbeans.modules.schema2beans.BaseBean) this.get", a.name, "(");
if (indexed) gen("i");
gencr(");");
gencr("if (n != null)");
gen("\tn.dump(str, indent + \"\\t\")"); eolNoI18N();
gencr("else");
gen("\tstr.append(indent+\"\\tnull\")"); eolNoI18N();
}
if (!a.isAttribute()) {
gen("this.dumpAttributes(", constName, ", ");
if (indexed)
gen("i");
else
gen("0");
gen(", str, indent)"); eol();
}
if (isScalar && config.isScalarException() && !Common.isBoolean(a.type)) {
end();
}
if (indexed) {
//gen("str.append(indent+\"\\t\")"); eol();
//gen("str.append(\"---\")"); eol();
end();
}
cr();
}
genAttributes(CONSTRUCTOR_SECTION, a.attributes, constName);
select(ACCESS_SECTION);
genDefaultsAccessable(a);
if (a.isBean) {
genNewMethod(a.getPropertyInterface(), a.getType());
}
}
// The root might have attributes
if (this.beanElement.isRoot)
genAttributes(CONSTRUCTOR_SECTION,
this.beanElement.node.getAttributes(), null);
select(DECL_SECTION); cr();
select(TRAILER_SECTION);
end(false); cr();
}
// Generate the createAttribute() code
void genAttributes(int out, AttrProp[] attributes, String name) {
select(out);
if (attributes == null)
return;
for (int j=0; j 0) {
gencr("new String[] {");
for (int k=0; k0) gencr(",");
gentab(5);
gen("\"", values[k], "\"");
}
cr(); gentab(4); gen("}, ");
}
else
gen("null, ");
String value = attr.getDefaultValue();
if (value != null)
gen("\"", value, "\")");
else
gen("null)");
eol();
}
void genBody(int out) throws IOException {
select(out);
//
// add/remove comparators
//
comment();
jw.beginMethod("addComparator", "org.netbeans.modules.schema2beans.BeanComparator c", null, "void", jw.PUBLIC | jw.STATIC);
gen("comparators.add(c)"); eol();
end(); cr();
comment();
jw.beginMethod("removeComparator", "org.netbeans.modules.schema2beans.BeanComparator c", null, "void", jw.PUBLIC | jw.STATIC);
gen("comparators.remove(c)"); eol();
end();
//
// Event registration methods
//
//
// the generation of addPropertyChange are in BaseBean.
//
if (this.genGenericVetoListeners) {
comment();
jw.beginMethod("add"+VCL, VCL_FULL_CLASS_NAME+" l", null, "void", jw.PUBLIC);
gengetprop();
gen("\tp.addVCListener(l)"); eol();
end(); cr();
comment();
jw.beginMethod("remove"+VCL, VCL_FULL_CLASS_NAME+" l", null, "void", jw.PUBLIC);
gengetprop();
gen("\tp.removeVCListener(l)"); eol();
end(); cr();
}
//
// Per property event registration method
//
//
// the generation of addPropertyChange are now in BaseBean.
//
if (this.genVetoListeners) {
comment();
jw.beginMethod("add"+VCL, "String n, "+VCL_FULL_CLASS_NAME+" l", null, "void", jw.PUBLIC);
gengetpropbyname();
gen("\tp.addVCListener(l)"); eol();
end(); cr();
comment();
jw.beginMethod("remove"+VCL, "String n, "+VCL_FULL_CLASS_NAME+" l", null, "void", jw.PUBLIC);
gengetpropbyname();
gen("\tp.removeVCListener(l)"); eol();
end(); cr();
}
if (this.beanElement.isRoot) {
// public void createBean(Node doc)
comment();
comment("This method returns the root of the bean graph");
comment("Each call creates a new bean graph from the specified DOM graph");
comment();
String thrownExceptions = null;
if (shouldThrowException()) {
thrownExceptions = "org.netbeans.modules.schema2beans.Schema2BeansException";
}
jw.beginMethod("createGraph", "org.w3c.dom.Node doc", thrownExceptions, className, jw.PUBLIC | jw.STATIC);
if (config.isSetDefaults())
gen("return new ", this.className, "(doc, Common.USE_DEFAULT_VALUES)");
else
gen("return new ", this.className, "(doc, Common.NO_DEFAULT_VALUES)");
eol();
end(); cr();
jw.beginMethod("createGraph", "java.io.File f",
(thrownExceptions == null) ? "java.io.IOException" :
thrownExceptions + ", " + "java.io.IOException",
className, jw.PUBLIC | jw.STATIC);
jw.writeEol("java.io.InputStream in = new java.io.FileInputStream(f)");
jw.beginTry();
gen("return createGraph(in, false)"); eol();
jw.endFinallyBegin();
jw.writeEol("in.close()");
jw.end();
jw.endMethod();
// public void createGraph(InputStream in)
jw.beginMethod("createGraph", "java.io.InputStream in", thrownExceptions, className, jw.PUBLIC | jw.STATIC);
gen("return createGraph(in, false)"); eol();
jw.endMethod();
// public void createGraph(InputStream in)
jw.beginMethod("createGraph", "java.io.InputStream in, boolean validate", thrownExceptions, className, jw.PUBLIC | jw.STATIC);
if (!shouldThrowException()) {
gen("try ");
begin();
}
gen("Document doc = GraphManager.createXmlDocument(in, validate)");
eol();
gen("return createGraph(doc)"); eol();
if (!shouldThrowException()) {
end();
gen("catch (Exception t) ");
begin();
if (this.config.isStandalone()) {
gencrNoI18N("throw new RuntimeException(\"DOM graph creation failed\", t);");
} else {
gencr("throw new RuntimeException(Common.getMessage(");
tabIn();
gencr("\"DOMGraphCreateFailed_msg\",");
tabIn();
gen("t))"); eol();
}
end();
}
end(); cr();
// public void createBean()
comment();
comment("This method returns the root for a new empty bean graph");
comment();
jw.beginMethod("createGraph", "", null, className, jw.PUBLIC | jw.STATIC);
if (shouldThrowException()) {
gen("try ");
begin();
}
gen("return new ", this.className, "()"); eol();
if (shouldThrowException()) {
end();
gen("catch (Schema2BeansException e) ");
begin();
gen("throw new RuntimeException(e)"); eol();
end();
}
end(); cr();
}
MetaElement me = getMetaElement(beanElement);
if (me != null && me.getUserCode() != null) {
String userCode = me.getUserCode();
cr(); gencr(userCode);
}
}
boolean shouldThrowException() {
return (config.isThrowErrors() || (mdd != null && mdd.isThrowExceptions()));
}
void genValidate() throws IOException {
select(BODY_SECTION);
jw.beginMethod("validate", "", "org.netbeans.modules.schema2beans.ValidateException", "void", jw.PUBLIC);
if (config.isGenerateValidate()) {
genValidateProperties();
}
end();
cr();
}
protected void genValidateFail(String detail, String name,
boolean quoteDetail,
ValidateException.FailureType ft,
JavaWriter out) throws IOException {
out.write("throw new org.netbeans.modules.schema2beans.ValidateException(");
if (quoteDetail)
out.write('"');
out.write(detail);
if (quoteDetail)
out.write('"');
out.write(", org.netbeans.modules.schema2beans.ValidateException.FailureType.",
ft.toString());
out.writeEolNoI18N(", \""+name+"\", this)");
}
void genTrailer(int out) {
select(out);
gen(PUBLIC, STRING, "dumpBeanNode()");
begin();
gen("StringBuffer str = new StringBuffer()"); eol();
gen("str.append(\"", this.className, "\\n\")"); eolNoI18N();
gen("this.dump(str, \"\\n \")"); eolNoI18N();
gen("return str.toString()"); eol();
end(false);
if (this.config.isDumpToString()) {
cr();
gencr(PUBLIC, STRING, "toString()");
begin();
gen("return this.dumpBeanNode()"); eol();
end();
}
}
/**
* Generate the java code in the out stream, using the optional
* metaDD bean graph.
*/
public void generate(OutputStream out, MetaDD mdd) throws IOException {
this.mdd = mdd;
this.metaElement = getMetaElement(beanElement);
if (this.metaElement != null && this.metaElement.isSkipGeneration()) {
config.messageOut.println("Skipping generation of class "
+ " (as specified in the mdd file)"); // NOI18N
return;
}
findAttributeOwners();
this.isVetoable = this.config.isVetoable();
// Find out if there is any veto specified in the mdd
if (this.mdd != null) {
//if (this.mdd.findPropertyValue("vetoable", Boolean.TRUE).length != 0) {
org.netbeans.modules.schema2beansdev.metadd.CommonBean[] beans = mdd.childBeans(true);
for (int beanPos = 0; beanPos < beans.length; ++beanPos) {
try {
if (((Boolean)beans[beanPos].fetchPropertyByName("vetoable")).booleanValue()) {
// Override information from the mdd file
this.isVetoable = this.mdd.isVetoable();
this.genGenericVetoListeners = true;
break;
}
} catch (IllegalArgumentException e) {
// This bean does not have that property
}
}
}
this.genHeader(HEADER_SECTION);
this.genPackage(HEADER_SECTION);
this.genImports(HEADER_SECTION);
select(HEADER_SECTION);
cr(); beginNoI18N(); cr();
this.genClassName(HEADER_SECTION);
this.genDeclarations(DECL_SECTION);
this.genConstructor(CONSTRUCTOR_SECTION);
this.genAccessors(ACCESS_SECTION);
this.genBody(BODY_SECTION);
this.genInitializer();
genValidate();
this.genTrailer(TRAILER_SECTION);
select(CONSTRUCTOR_SECTION);
gen("this.initialize(options)"); eol();
end(); cr();
select(INITIALIZE_SECTION);
cr(); end(); cr();
select(TRAILER_SECTION);
end(); cr();
endNoI18N(); cr();
try {
printSchema();
} catch (IOException ioe) {
// Do not generate the schema comment
}
printGenBuffers(out);
}
protected void addExtraMethods() {
// Most of these are taken from the public methods of BaseBean
jw.addToMethodStore("addComparator", "org.netbeans.modules.schema2beans.BeanComparator c", null, "void", jw.PUBLIC | jw.STATIC);
jw.addToMethodStore("removeComparator", "org.netbeans.modules.schema2beans.BeanComparator c", null, "void", jw.PUBLIC | jw.STATIC);
jw.addToMethodStore("getValue", "String name", null, "Object");
jw.addToMethodStore("getValue", "String name, int index", null, "Object");
jw.addToMethodStore("isNull", "String name", null, "boolean");
jw.addToMethodStore("isNull", "String name, int index", null, "boolean");
jw.addToMethodStore("getValues", "String name", null, "Object[]");
jw.addToMethodStore("setValue", "String name, Object value", null, "void");
jw.addToMethodStore("setValue", "String name, int index, Object value", null, "void");
jw.addToMethodStore("setValue", "String name, Object[] value", null, "void");
jw.addToMethodStore("addValue", "String name, Object value", null, "int");
jw.addToMethodStore("removeValue", "String name, Object value", null, "int");
jw.addToMethodStore("removeValue", "String name, int index", null, "void");
jw.addToMethodStore("indexOf", "String name, Object value", null, "int");
jw.addToMethodStore("size", "String name", null, "int");
jw.addToMethodStore("isChoiceProperty", "String name", null, "boolean");
jw.addToMethodStore("isChoiceProperty", "", null, "boolean");
jw.addToMethodStore("getAttributeValue", "String name", null, "String");
jw.addToMethodStore("getAttributeValue", "String propName, String name", null, "String");
jw.addToMethodStore("setAttributeValue", "String propName, int index, String name, String value", null, "void");
jw.addToMethodStore("getAttributeValue", "String propName, int index, String name", null, "String");
jw.addToMethodStore("getAttributeNames", "String propName", null, "String[]");
jw.addToMethodStore("getAttributeNames", "", null, "String[]");
jw.addToMethodStore("write", "java.io.OutputStream out", "java.io.IOException, org.netbeans.modules.schema2beans.Schema2BeansRuntimeException", "void");
jw.addToMethodStore("write", "java.io.OutputStream out, String encoding", "java.io.IOException, org.netbeans.modules.schema2beans.Schema2BeansException", "void");
jw.addToMethodStore("write", "java.io.Writer w", "java.io.IOException, org.netbeans.modules.schema2beans.Schema2BeansException", "void");
jw.addToMethodStore("write", "java.io.Writer w, String encoding", "java.io.IOException, org.netbeans.modules.schema2beans.Schema2BeansException", "void");
jw.addToMethodStore("writeNoReindent", "java.io.OutputStream out", "java.io.IOException, org.netbeans.modules.schema2beans.Schema2BeansException", "void");
jw.addToMethodStore("reindent", "", null, "void");
jw.addToMethodStore("clone", "", null, "Object");
jw.addToMethodStore("merge", "org.netbeans.modules.schema2beans.BaseBean bean, int mode", null, "void");
jw.addToMethodStore("merge", "org.netbeans.modules.schema2beans.BaseBean bean", null, "void");
jw.addToMethodStore("equals", "Object obj", null, "boolean");
jw.addToMethodStore("parent", "", null, "org.netbeans.modules.schema2beans.BaseBean");
jw.addToMethodStore("fullName", "", null, "String");
jw.addToMethodStore("isRoot", "", null, "boolean");
jw.addToMethodStore("name", "", null, "String");
jw.addToMethodStore("dtdName", "", null, "String");
jw.addToMethodStore("dump", "StringBuffer str, String indent", null, "void");
jw.addToMethodStore("createGraph", "Class clazz, java.io.InputStream in", "org.netbeans.modules.schema2beans.Schema2BeansException", "org.netbeans.modules.schema2beans.BaseBean", jw.PUBLIC | jw.STATIC);
jw.addToMethodStore("createGraph", "Class clazz, java.io.InputStream in, boolean validate", "org.netbeans.modules.schema2beans.Schema2BeansException", "org.netbeans.modules.schema2beans.BaseBean", jw.PUBLIC | jw.STATIC);
jw.addToMethodStore("createGraph", "Class clazz, java.io.InputStream in, boolean validate, org.xml.sax.EntityResolver er", "org.netbeans.modules.schema2beans.Schema2BeansException", "org.netbeans.modules.schema2beans.BaseBean", jw.PUBLIC | jw.STATIC);
jw.addToMethodStore("createGraph", "Class clazz, java.io.InputStream in, boolean validate, org.xml.sax.EntityResolver er, org.xml.sax.ErrorHandler eh", "org.netbeans.modules.schema2beans.Schema2BeansException", "org.netbeans.modules.schema2beans.BaseBean", jw.PUBLIC | jw.STATIC);
jw.addToMethodStore("addPropertyChangeListener", "java.beans.PropertyChangeListener l", null, "void");
jw.addToMethodStore("removePropertyChangeListener", "java.beans.PropertyChangeListener l", null, "void");
jw.addToMethodStore("addPropertyChangeListener", "String n, java.beans.PropertyChangeListener l", null, "void");
jw.addToMethodStore("removePropertyChangeListener", "String n, java.beans.PropertyChangeListener l", null, "void");
jw.addToMethodStore("comments", "", null, "org.w3c.dom.Comment[]");
jw.addToMethodStore("addComment", "String comment", null, "org.w3c.dom.Comment");
jw.addToMethodStore("removeComment", "org.w3c.dom.Comment comment", null, "void");
jw.addToMethodStore("childBeans", "boolean recursive", null, "org.netbeans.modules.schema2beans.BaseBean[]");
jw.addToMethodStore("childBeans", "boolean recursive, java.util.List beans", null, "void");
jw.addToMethodStore("setDefaultNamespace", "String namespace", null, "void");
jw.addToMethodStore("getDefaultNamespace", "", null, "String");
jw.addToMethodStore("toString", "", null, "String");
//jw.addToMethodStore("copyProperties", "org.netbeans.modules.schema2beans.BaseBean destBean, java.util.Map nameMapping", null, "void");
}
/*
protected Signatures getSystemSignatures() {
Signatures result = new Signatures();
Signature sig;
if (config.isGenerateValidate()) {
result.add(new Signature("void", "validate"));
}
sig = new Signature("void", "addComparator");
sig.addParameter("org.netbeans.modules.schema2beans.BeanComparator", "c");
sig.setStatic();
result.add(sig);
sig = new Signature("void", "removeComparator");
sig.addParameter("org.netbeans.modules.schema2beans.BeanComparator", "c");
sig.setStatic();
result.add(sig);
if (beanElement.isRoot) {
sig = new Signature(className, "createGraph");
sig.addParameter("org.w3c.dom.Node", "doc");
sig.setStatic();
result.add(sig);
sig = new Signature(className, "createGraph");
sig.addParameter("java.io.InputStream", "in");
sig.setStatic();
result.add(sig);
sig = new Signature(className, "createGraph");
sig.addParameter("java.io.InputStream", "in");
sig.addParameter("boolean", "validate");
sig.setStatic();
result.add(sig);
sig = new Signature(className, "createGraph");
sig.setStatic();
result.add(sig);
}
sig = new Signature("void", "write");
sig.addParameter("java.io.OutputStream", "out");
sig.addThrows("java.io.IOException");
sig.addThrows("org.netbeans.modules.schema2beans.Schema2BeansRuntimeException");
result.add(sig);
sig = new Signature("void", "write");
sig.addParameter("java.io.OutputStream", "out");
sig.addParameter("String", "encoding");
sig.addThrows("java.io.IOException");
sig.addThrows("org.netbeans.modules.schema2beans.Schema2BeansException");
result.add(sig);
sig = new Signature("void", "write");
sig.addParameter("java.io.Writer", "w");
sig.addThrows("java.io.IOException");
sig.addThrows("org.netbeans.modules.schema2beans.Schema2BeansException");
result.add(sig);
sig = new Signature("void", "write");
sig.addParameter("java.io.Writer", "w");
sig.addParameter("String", "encoding");
sig.addThrows("java.io.IOException");
sig.addThrows("org.netbeans.modules.schema2beans.Schema2BeansException");
result.add(sig);
sig = new Signature("void", "merge");
sig.addParameter("org.netbeans.modules.schema2beans.BaseBean", "bean");
result.add(sig);
sig = new Signature("boolean", "equals", OTHER);
sig.addParameter("Object", "obj");
result.add(sig);
result.add(new Signature("String", "toString"));
return result;
}
*/
public void setInvalidPropertyNames(Map invalidNames) {
invalidNames.put("Class", null);
invalidNames.put("Property", null);
invalidNames.put("AttributeNames", null);
}
protected String testIfPropertySet(Property prop) {
if (!prop.isAttribute() && prop.isScalar() &&
!Common.isBoolean(prop.type))
return "getValue("+prop.constName+") != null";
else
return super.testIfPropertySet(prop);
}
protected String testIfPropertyNotSet(Property prop) {
if (!prop.isAttribute() && prop.isScalar() &&
!Common.isBoolean(prop.type))
return "getValue("+prop.constName+") == null";
else
return super.testIfPropertyNotSet(prop);
}
}
//******************************************************************************
// END_NOI18N
// This class does not (and will not) cantain strings that need to be localized.
//******************************************************************************
© 2015 - 2025 Weber Informatics LLC | Privacy Policy