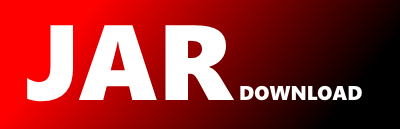
org.netbeans.api.xml.lexer.XMLTokenId Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.api.xml.lexer;
import java.util.Collection;
import java.util.EnumSet;
import java.util.HashMap;
import java.util.Map;
import org.netbeans.api.editor.mimelookup.MimeRegistration;
import org.netbeans.api.lexer.InputAttributes;
import org.netbeans.api.lexer.Language;
import org.netbeans.api.lexer.LanguagePath;
import org.netbeans.api.lexer.Token;
import org.netbeans.api.lexer.TokenId;
import org.netbeans.lib.xml.lexer.XMLLexer;
import org.netbeans.spi.lexer.LanguageEmbedding;
import org.netbeans.spi.lexer.LanguageHierarchy;
import org.netbeans.spi.lexer.Lexer;
import org.netbeans.spi.lexer.LexerRestartInfo;
/**
* Token ids of XML language
*/
public enum XMLTokenId implements TokenId {
/** Plain text */
TEXT("xml-text"),
/** Erroneous Text */
WS("xml-ws"),
/** Plain Text*/
ERROR("xml-error"),
/** XML Tag */
TAG("xml-tag"),
/** Argument of a tag */
ARGUMENT("xml-attribute"),
/** Operators - '=' between arg and value */
OPERATOR("xml-operator"),
/** Value - value of an argument */
VALUE("xml-value"),
/** Block comment */
BLOCK_COMMENT("xml-comment"),
/** SGML declaration in XML document - e.g. <!DOCTYPE> */
DECLARATION("xml-doctype"),
/** Character reference, e.g. < = < */
CHARACTER("xml-ref"),
/** End of line */
EOL("xml-EOL"),
/* PI start delimiter <?target content of pi ?> */
PI_START("xml-pi-start"),
/* PI target <?target content of pi ?> */
PI_TARGET("xml-pi-target"),
/* PI conetnt <?target content of pi ?> */
PI_CONTENT("xml-pi-content"),
/* PI end delimiter <?target ?> */
PI_END("xml-pi-end"),
/** Cdata section including its delimiters. */
CDATA_SECTION("xml-cdata-section");
private final String primaryCategory;
XMLTokenId() {
this(null);
}
XMLTokenId(String primaryCategory) {
this.primaryCategory = primaryCategory;
}
public String primaryCategory() {
return primaryCategory;
}
private static final Language language = new LanguageHierarchy() {
@Override
protected Collection createTokenIds() {
return EnumSet.allOf(XMLTokenId.class);
}
@Override
protected Map> createTokenCategories() {
Map> cats = new HashMap>();
// Incomplete literals
//cats.put("incomplete", EnumSet.of());
// Additional literals being a lexical error
//cats.put("error", EnumSet.of());
return cats;
}
@Override
public Lexer createLexer(LexerRestartInfo info) {
return new XMLLexer(info);
}
@Override
public LanguageEmbedding> embedding(
Token token, LanguagePath languagePath, InputAttributes inputAttributes) {
return null; // No embedding
}
@Override
public String mimeType() {
return "text/xml";
}
}.language();
@MimeRegistration(mimeType = "text/xml", service = Language.class)
public static Language language() {
return language;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy