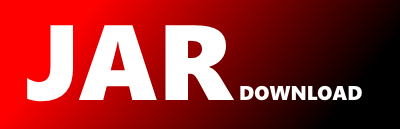
org.netbeans.modules.palette.Utils Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.modules.palette;
import java.awt.event.ActionListener;
import java.beans.BeanInfo;
import java.util.ResourceBundle;
import java.text.MessageFormat;
import java.awt.event.ActionEvent;
import java.awt.datatransfer.*;
import java.io.IOException;
import java.util.*;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.*;
import org.netbeans.spi.palette.PaletteController;
import org.netbeans.modules.palette.ui.PalettePanel;
import org.netbeans.spi.palette.PaletteActions;
import org.openide.*;
import org.openide.loaders.DataObject;
import org.openide.nodes.*;
import org.openide.filesystems.*;
import org.openide.util.*;
import org.openide.util.datatransfer.PasteType;
import org.openide.util.datatransfer.NewType;
import org.openide.util.datatransfer.ExClipboard;
import org.openide.windows.TopComponent;
/**
* Class providing various useful methods for palette classes.
*
* @author S Aubrecht
*/
public final class Utils {
private static final Logger ERR = Logger.getLogger( "org.netbeans.modules.palette" ); // NOI18N
private Utils() {
}
// -----------
public static ResourceBundle getBundle() {
return NbBundle.getBundle(Utils.class);
}
public static String getBundleString(String key) {
return getBundle().getString(key);
}
public static Action[] mergeActions( Action[] first, Action[] second ) {
if( null == first )
return second;
if( null == second )
return first;
Action[] res = new Action[first.length+second.length+1];
System.arraycopy( first, 0, res, 0, first.length );
res[first.length] = null;
System.arraycopy( second, 0, res, first.length+1, second.length );
return res;
}
public static boolean isReadonly( Node node ) {
return getBoolean(node, PaletteController.ATTR_IS_READONLY, !node.canDestroy());
}
public static boolean getBoolean( Node node, String attrName, boolean defaultValue ) {
Object val = node.getValue( attrName );
if( null == val ) {
DataObject dobj = (DataObject)node.getCookie( DataObject.class );
if( null != dobj ) {
val = dobj.getPrimaryFile().getAttribute( attrName );
}
}
if( null != val ) {
return Boolean.valueOf( val.toString() ).booleanValue();
} else {
return defaultValue;
}
}
public static HelpCtx getHelpCtx( Node node, HelpCtx defaultHelp ) {
HelpCtx retValue = defaultHelp;
if( null == retValue || HelpCtx.DEFAULT_HELP.equals( retValue ) ) {
Object val = node.getValue( PaletteController.ATTR_HELP_ID );
if( null == val ) {
DataObject dobj = (DataObject)node.getCookie( DataObject.class );
if( null != dobj ) {
val = dobj.getPrimaryFile().getAttribute( PaletteController.ATTR_HELP_ID );
}
}
if( null != val )
retValue = new HelpCtx( val.toString() );
}
return retValue;
}
public static void addCustomizationMenuItems( JPopupMenu popup, PaletteController controller, Settings settings ) {
popup.addSeparator();
popup.add( new ShowNamesAction( settings ) );
popup.add( new ChangeIconSizeAction( settings ) );
addResetMenuItem( popup, controller, settings );
popup.addSeparator();
popup.add( new ShowCustomizerAction( controller ) );
}
static void addResetMenuItem( JPopupMenu popup, final PaletteController controller, final Settings settings ) {
JMenuItem item = new JMenuItem( getBundleString( "CTL_ResetPalettePopup" ) );
item.addActionListener( new ActionListener() {
public void actionPerformed(ActionEvent e) {
resetPalette( controller, settings );
}
});
popup.add( item );
}
/**
* Find a Node representing the given category.
*
* @param root Palette's root node.
* @param categoryName Name of the category to search for.
* @return Category with the given name or null.
*/
public static Node findCategoryNode( Node root, String categoryName ) {
return root.getChildren().findChild( categoryName );
}
public static void resetPalette( final PaletteController controller, final Settings settings ) {
Node rootNode = (Node)controller.getRoot().lookup( Node.class );
if( null != rootNode ) {
PaletteActions customActions = rootNode.getLookup().lookup( PaletteActions.class );
Action resetAction = customActions.getResetAction();
if( null != resetAction ) {
settings.reset();
resetAction.actionPerformed( new ActionEvent( controller, 0, "reset" ) ); //NOI18N
controller.refresh();
} else {
resetPalette( rootNode, controller, settings );
}
}
}
public static void resetPalette( Node rootNode, PaletteController controller, Settings settings ) {
// first user confirmation...
NotifyDescriptor desc = new NotifyDescriptor.Confirmation(
getBundleString("MSG_ConfirmPaletteReset"), // NOI18N
getBundleString("CTL_ConfirmResetTitle"), // NOI18N
NotifyDescriptor.YES_NO_OPTION);
if( NotifyDescriptor.YES_OPTION.equals(
DialogDisplayer.getDefault().notify(desc)) ) {
settings.reset();
DataObject dob = (DataObject)rootNode.getLookup().lookup( DataObject.class );
if( null != dob ) {
FileObject primaryFile = dob.getPrimaryFile();
if( null != primaryFile && primaryFile.isFolder() ) {
try {
primaryFile.revert();
for( FileObject fo : primaryFile.getChildren() ) {
fo.setAttribute( "categoryName", null );
fo.setAttribute( "position", null );
}
} catch (IOException ex) {
ERR.log(Level.INFO, null, ex);
}
}
}
controller.refresh();
}
}
public static void setOpenedByUser( TopComponent tc, boolean userOpened ) {
tc.putClientProperty( "userOpened", Boolean.valueOf(userOpened) ); //NOI18N
}
public static boolean isOpenedByUser( TopComponent tc ) {
Object val = tc.getClientProperty( "userOpened" );
tc.putClientProperty("userOpened", null);
return val instanceof Boolean && ((Boolean) val).booleanValue();
}
/**
* An action to create a new palette category.
*/
public static class NewCategoryAction extends AbstractAction {
private Node paletteNode;
/**
* @param paletteRootNode Palette's root node.
*/
public NewCategoryAction( Node paletteRootNode ) {
putValue(Action.NAME, getBundleString("CTL_CreateCategory")); // NOI18N
this.paletteNode = paletteRootNode;
}
public void actionPerformed(ActionEvent event) {
NewType[] newTypes = paletteNode.getNewTypes();
try {
if( null != newTypes && newTypes.length > 0 ) {
newTypes[0].create();
}
} catch( IOException ioE ) {
ERR.log( Level.INFO, ioE.getLocalizedMessage(), ioE );
}
}
@Override
public boolean isEnabled() {
NewType[] newTypes = paletteNode.getNewTypes();
return null != newTypes && newTypes.length > 0;
}
}
/**
* An action to sort categories alphabetically.
*/
static class SortCategoriesAction extends AbstractAction {
private Node paletteNode;
public SortCategoriesAction( Node paletteNode ) {
putValue(Action.NAME, getBundleString("CTL_SortCategories")); // NOI18N
this.paletteNode = paletteNode;
}
public void actionPerformed(ActionEvent event) {
Index order = (Index)paletteNode.getCookie(Index.class);
if (order != null) {
final Node[] nodes = order.getNodes();
Arrays.sort( nodes, new Comparator() {
public int compare(Node n1, Node n2) {
return n1.getDisplayName().compareTo( n2.getDisplayName() );
}
} );
int[] perm = new int[nodes.length];
for( int i=0; i() {
public int compare(Node n1, Node n2) {
return n1.getDisplayName().compareTo( n2.getDisplayName() );
}
} );
int[] perm = new int[nodes.length];
for( int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy