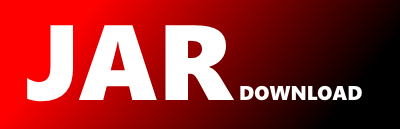
org.netbeans.modules.viewmodel.TreeModelHyperNode Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.netbeans.modules.viewmodel;
import java.awt.datatransfer.Transferable;
import java.lang.ref.WeakReference;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Set;
import java.util.concurrent.Executor;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.netbeans.spi.viewmodel.AsynchronousModelFilter;
import org.netbeans.spi.viewmodel.AsynchronousModelFilter.CALL;
import org.netbeans.spi.viewmodel.ColumnModel;
import org.netbeans.spi.viewmodel.Models;
import org.netbeans.spi.viewmodel.Models.CompoundModel;
import org.netbeans.spi.viewmodel.Models.TreeFeatures;
import org.netbeans.spi.viewmodel.TreeModelFilter;
import org.netbeans.spi.viewmodel.UnknownTypeException;
import org.openide.nodes.Children;
import org.openide.nodes.Node;
import org.openide.util.Exceptions;
import org.openide.util.datatransfer.PasteType;
/**
*
* @author Martin Entlicher
*/
public class TreeModelHyperNode extends TreeModelNode {
private HyperCompoundModel model;
public TreeModelHyperNode(
final HyperCompoundModel model,
final TreeModelRoot treeModelRoot,
final Object object
) {
super(
model.getMain(),
model.getColumns(),
null,
createChildren(model, treeModelRoot, object),
treeModelRoot,
object
);
this.model = model;
}
private static Children createChildren (
HyperCompoundModel model,
TreeModelRoot treeModelRoot,
Object object
) {
if (object == null) {
throw new NullPointerException ();
}
return new HyperModelChildren (model, treeModelRoot, object);
}
@Override
protected void refreshTheChildren(Set models, TreeModelChildren.RefreshingInfo refreshInfo) {
//System.err.println("HYPER node: refreshTheChildren("+model+", "+refreshInfo+")");
//Thread.dumpStack();
Children ch = getChildren();
if (ch instanceof TreeModelChildren) {
HyperModelChildren hch = (HyperModelChildren) ch;
//hch.cleanCachedChildren(model);
hch.refreshChildren(hch.new HyperRefreshingInfo(refreshInfo, models));
} else {
setChildren(new HyperModelChildren (this.model, treeModelRoot, object));
}
}
@Override
public PasteType getDropType(Transferable t, int action, int index) {
//System.err.println("\nTreeModelHyperNode.getDropType("+model+", \n"+action+", "+index+")");
if (index < 0) {
// Drop to an area outside models. Try to find some that accepts it:
PasteType p;
CompoundModel mm = model.getMain();
try {
p = mm.getDropType(object, t, action, index);
} catch (UnknownTypeException e) {
p = null;
}
if (p == null) {
for (CompoundModel m : model.getModels()) {
if (m != mm) {
try {
p = m.getDropType(object, t, action, index);
if (p != null) break;
} catch (UnknownTypeException ex) {
}
}
}
}
//System.err.println(" PasteType = "+p+"\n");
return p;
} else {
// Drop between nodes of some model.
PasteType p = null;
HyperModelChildren hch = (HyperModelChildren) getChildren();
int index1 = (index > 0) ? index - 1 : index; // node above
int[] modelIndexPtr = new int[] { -1 };
CompoundModel cm1 = hch.getRootModelByIndex(index1, modelIndexPtr);
if (cm1 != null) {
try {
if (index1 < index) modelIndexPtr[0]++;
//System.err.println("\nTreeModelHyperNode.getDropType("+cm1+", \n"+action+", "+modelIndexPtr[0]+")");
p = cm1.getDropType(object, t, action, modelIndexPtr[0]);
} catch (UnknownTypeException e) {
}
}
if (p == null && index1 < index) { // node below
CompoundModel cm2 = hch.getRootModelByIndex(index, modelIndexPtr);
if (cm2 != null && cm2 != cm1) {
try {
//System.err.println("\nTreeModelHyperNode.getDropType("+cm2+", \n"+action+", "+modelIndexPtr[0]+")");
p = cm2.getDropType(object, t, action, modelIndexPtr[0]);
} catch (UnknownTypeException e) {
}
}
}
//System.err.println(" PasteType = "+p+"\n");
return p;
}
}
private static final class HyperModelChildren extends TreeModelChildren {
private HyperCompoundModel model;
private final java.util.Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy