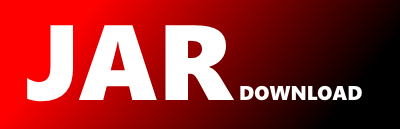
org.openide.WizardDescriptor Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.openide;
import java.awt.*;
import java.awt.datatransfer.Clipboard;
import java.awt.datatransfer.StringSelection;
import java.awt.event.*;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.io.IOException;
import java.net.URL;
import java.text.MessageFormat;
import java.util.*;
import java.util.List;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.accessibility.Accessible;
import javax.swing.*;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
import javax.swing.event.HyperlinkEvent;
import javax.swing.event.HyperlinkEvent.EventType;
import javax.swing.event.HyperlinkListener;
import javax.swing.text.html.HTMLEditorKit;
import javax.swing.text.html.StyleSheet;
import org.netbeans.api.progress.ProgressHandle;
import org.netbeans.api.progress.ProgressHandleFactory;
import org.openide.awt.HtmlBrowser;
import org.openide.awt.HtmlBrowser.URLDisplayer;
import org.openide.awt.Mnemonics;
import org.openide.util.*;
/**
* Implements a basic "wizard" GUI system.
* A list of wizard panels may be specified and these
* may be traversed at the proper times using the "Previous"
* and "Next" buttons (or "Finish" on the last one).
*
* Related Tutorial
*
*
*
* @see DialogDisplayer#createDialog
*/
public class WizardDescriptor extends DialogDescriptor {
/** "Next" button option.
* @see #setOptions */
public static final Object NEXT_OPTION = new String("NEXT_OPTION"); // NOI18N
/** "Finish" button option.
* @see #setOptions */
public static final Object FINISH_OPTION = OK_OPTION;
/** "Previous" button option.
* @see #setOptions */
public static final Object PREVIOUS_OPTION = new String("PREVIOUS_OPTION"); // NOI18N
private static final ActionListener CLOSE_PREVENTER = new ActionListener() {
@Override
public void actionPerformed(ActionEvent evt) {
}
@Override
public String toString() {
return "CLOSE_PREVENTER"; // NOI18N
}
};
/** Set to true
for enabling other properties. It is relevant only on
* initialization (client property in first panel). Recommended to be set to true
in most cases,
* then wizard can display wizard steps on the left side, create a subtitle on active panel,
* display of error messages and others. When false or not present in JComponent.getClientProperty(),
* then supplied panel is used directly without content, help or panel name auto layout.
*
* The value is taken from WizardDescriptor.getProperty()
or
* ((JComponent)Panel.getComponent()).getClientProperty()
in this order.
* Boolean
type property.
* @since 7.8
*/
public static final String PROP_AUTO_WIZARD_STYLE = "WizardPanel_autoWizardStyle"; // NOI18N
/** Set to true
for showing help pane (HTML browser) in the left pane. It is relevant only on
* initialization (client property in first panel). Help content will be taken from property PROP_HELP_URL
.
*
* The value is taken from WizardDescriptor.getProperty()
or
* ((JComponent)Panel.getComponent()).getClientProperty()
in this order.
* Boolean
type property.
* @since 7.8
*/
public static final String PROP_HELP_DISPLAYED = "WizardPanel_helpDisplayed"; // NOI18N
/** Set to true
for showing content pane (steps) in the left pane. It is relevant only on
* initialization (client property in first panel). Content will be constructed from property PROP_CONTENT_DATA
.
*
* The value is taken from WizardDescriptor.getProperty()
or
* ((JComponent)Panel.getComponent()).getClientProperty()
in this order.
* Boolean
type property.
* @since 7.8
*/
public static final String PROP_CONTENT_DISPLAYED = "WizardPanel_contentDisplayed"; // NOI18N
/** Set to true
for displaying numbers in the content. It is relevant only on
* initialization (client property in first panel).
*
* The value is taken from WizardDescriptor.getProperty()
or
* ((JComponent)Panel.getComponent()).getClientProperty()
in this order.
* Boolean
type property.
* @since 7.8
*/
public static final String PROP_CONTENT_NUMBERED = "WizardPanel_contentNumbered"; // NOI18N
/** Represents index of content item which will be highlighted.
*
* The value is taken from WizardDescriptor.getProperty()
or
* ((JComponent)Panel.getComponent()).getClientProperty()
in this order.
* Integer
type property.
* @since 7.8
*/
public static final String PROP_CONTENT_SELECTED_INDEX = "WizardPanel_contentSelectedIndex"; // NOI18N
/** Represents array of content items.
*
* The value is taken from WizardDescriptor.getProperty()
or
* ((JComponent)Panel.getComponent()).getClientProperty()
in this order.
* String[]
type property.
* @since 7.8
*/
public static final String PROP_CONTENT_DATA = "WizardPanel_contentData"; // NOI18N
/** Set background color of content pane.
*
* The value is taken from WizardDescriptor.getProperty()
or
* ((JComponent)Panel.getComponent()).getClientProperty()
in this order.
* Color
type property.
* @since 7.8
*/
public static final String PROP_CONTENT_BACK_COLOR = "WizardPanel_contentBackColor"; // NOI18N
/** Set foreground color of content pane.
*
* The value is taken from WizardDescriptor.getProperty()
or
* ((JComponent)Panel.getComponent()).getClientProperty()
in this order.
* Color
type property.
* @since 7.8
*/
public static final String PROP_CONTENT_FOREGROUND_COLOR = "WizardPanel_contentForegroundColor"; // NOI18N
/** Set the image which will be displayed in the left pane (behind the content).
*
* The value is taken from WizardDescriptor.getProperty()
or
* ((JComponent) Panel.getComponent()).getClientProperty()
in this order.
* java.awt.Image
type property.
* @since 7.8
*/
public static final String PROP_IMAGE = "WizardPanel_image"; // NOI18N
/** Set the side where the image should be drawn.
*
* The value is taken from WizardDescriptor.getProperty()
or
* ((JComponent) Panel.getComponent()).getClientProperty()
in this order.
* String
type property.
* @since 7.8
*/
public static final String PROP_IMAGE_ALIGNMENT = "WizardPanel_imageAlignment"; // NOI18N
/** Dimension of left pane, should be same as dimension of PROP_IMAGE
.
* It is relevant only on initialization (client property in first panel).
*
* The value is taken from WizardDescriptor.getProperty()
or
* ((JComponent) Panel.getComponent()).getClientProperty()
in this order.
* Dimension
type property.
* @since 7.8
*/
public static final String PROP_LEFT_DIMENSION = "WizardPanel_leftDimension"; // NOI18N
/** Represents URL of help displayed in left pane.
*
* The value is taken from WizardDescriptor.getProperty()
or
* ((JComponent) Panel.getComponent()).getClientProperty()
in this order.
* URL
type property.
* @since 7.8
*/
public static final String PROP_HELP_URL = "WizardPanel_helpURL"; // NOI18N
/** Error message that is displayed at the bottom of the wizard.
* Message informs user why the panel is invalid and possibly why the Next/Finish buttons were disabled.
* The property must be set to null value to clear the message.
*
* The value is taken from WizardDescriptor.getProperty()
.
* String
type property.
* @since 7.8
*/
public static final String PROP_ERROR_MESSAGE = "WizardPanel_errorMessage"; // NOI18N
/** Warning message that is displayed at the bottom of the wizard.
* Message informs user about possible non fatal problems with current enterd values in the wizard panel.
* Next/Finish buttons are usually enabled. The property must be set to null value to clear the message.
*
* The value is taken from WizardDescriptor.getProperty()
.
* String
type property.
* @since 7.8
*/
public static final String PROP_WARNING_MESSAGE = "WizardPanel_warningMessage"; // NOI18N
/** Informational message that is displayed at the bottom of the wizard.
* Message informs user usually about need to fill some field or similar requirements or other non fatal problems.
* Next/Finish button are usually enabled. The property must be set to null value to clear the message.
*
* The value is taken from WizardDescriptor.getProperty()
.
* String
type property.
* @since 7.8
*/
public static final String PROP_INFO_MESSAGE = "WizardPanel_infoMessage"; // NOI18N
private static Logger err = Logger.getLogger(WizardDescriptor.class.getName ());
/** real buttons to be placed instead of the options */
private final JButton nextButton = new JButton();
private final JButton finishButton = new JButton();
private final JButton cancelButton = new JButton();
private final JButton previousButton = new JButton();
private FinishAction finishOption;
private Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy